Unity IoC database transaction, not fetching updated data, using ContainerControlledLifetimeManager
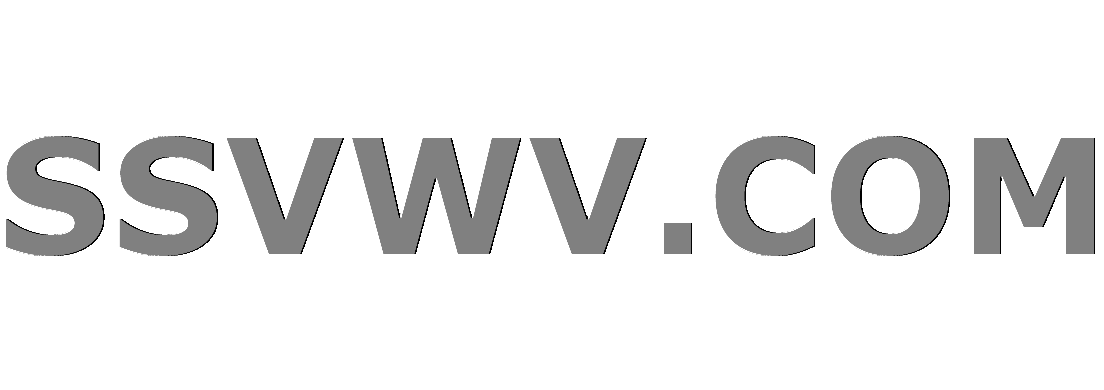
Multi tool use
I am using Unity IoC in C#, and DbContext with ContainerControlledLifetimeManager for application context with three layer architecture using Entity Framework. I have to ask either this pattern is better in a Windows Forms application or is there any better suggestion. I also face a problem in getting latest data when data is updated in database and after refreshing window form it loads old data.
As I am also using transactions using Entity Framework and therefore I can not use TransientLifetimeManager for DbContext class. I need same context in different DA classes to perform multiple operations in a single transaction. Is there a better approach to tackle this kind of situation?
Here is the project link for demonstration:
Sample Project
Generic Repository
public interface IRepository<ModelClass, EntityClass>
where ModelClass : class
where EntityClass : class
{
void Add(ModelClass entity);
void Delete(ModelClass entity);
void Update(ModelClass entity,int id);
bool Save();
}
Generic Repository implementation
public class GenericRepository<ModelClass, EntityClass> : IRepository<ModelClass, EntityClass>
where ModelClass : class
where EntityClass : class
{
private ApplicationContext context = null;
private DbSet<EntityClass> Entity = null;
public GenericRepository(ApplicationContext context)
{
this.context = context;
Entity = context.Set<EntityClass>();
}
public void Add(ModelClass model)
{
EntityClass enityClass = AutoMapper.Mapper.Map<ModelClass, EntityClass>(model);
this.Entity.Add(enityClass);
}
public void Delete(ModelClass model)
{
EntityClass enityClass = AutoMapper.Mapper.Map<ModelClass, EntityClass>(model);
this.Entity.Remove(enityClass);
context.SaveChanges();
}
public void Update(ModelClass model, int id)
{
EntityClass dbEntity = Entity.Find(id);
EntityClass entityClass = AutoMapper.Mapper.Map<ModelClass, EntityClass>(model);
context.Entry(dbEntity).CurrentValues.SetValues(entityClass);
context.Entry(dbEntity).State = EntityState.Modified;
}
public bool Save()
{
bool status = false;
try
{
int result = context.SaveChanges();
if (result > 0)
{
return status = true;
}
else
return status;
}
catch (DbEntityValidationException dbEx)
{
throw;
}
return status;
}
Student data access interface
public interface IStudent : IRepository<Student, StudentEntity>
{
List<Student> GetStudentById(int studentId);
}
// Implementation of student access interfaces
public class StudentAccess : GenericRepository<Student, StudentEntity>, IStudent
{
public StudentAccess(ApplicationContext context)
: base(context)
{
this.context = context;
}
Public void Add(student std)
{
base.Add(std);
base.save();
}
Public void Update(student std,int studentid)
{
base.update(std,studentId);
base.save();
}
public List<student> GetStudentById()
{
var data=context.student.ToList();
return data;
}
}
Student service layer
public class StudentService
{
ApplicationContext Context;
IStudent student;
public StudentService(ApplicationContext Context, IStudent student)
{
this.Context = Context;
this.student = student;
}
Public void SaveStudent(student std)
{
this.student.Add(std);
}
Public void UpdateStudent(student std,int studentId)
{
this.student.Update(std,studentId);
}
}
Implementation of unity container
public class DependencyOfDependency : UnityContainerExtension
{
protected override void Initialize()
{
// Register Data access layer
Container.RegisterType<DbContext, LogisticsERP.DA.Entities.ApplicationContext>(new ContainerControlledLifetimeManager());
Container.RegisterType< IStudent,StudentAccess>();
//Register Service Layer Classes
Container.RegisterType<StudentService>();
}
}
sql c#-4.0 dependency-injection entity-framework-6 unity-container
add a comment |
I am using Unity IoC in C#, and DbContext with ContainerControlledLifetimeManager for application context with three layer architecture using Entity Framework. I have to ask either this pattern is better in a Windows Forms application or is there any better suggestion. I also face a problem in getting latest data when data is updated in database and after refreshing window form it loads old data.
As I am also using transactions using Entity Framework and therefore I can not use TransientLifetimeManager for DbContext class. I need same context in different DA classes to perform multiple operations in a single transaction. Is there a better approach to tackle this kind of situation?
Here is the project link for demonstration:
Sample Project
Generic Repository
public interface IRepository<ModelClass, EntityClass>
where ModelClass : class
where EntityClass : class
{
void Add(ModelClass entity);
void Delete(ModelClass entity);
void Update(ModelClass entity,int id);
bool Save();
}
Generic Repository implementation
public class GenericRepository<ModelClass, EntityClass> : IRepository<ModelClass, EntityClass>
where ModelClass : class
where EntityClass : class
{
private ApplicationContext context = null;
private DbSet<EntityClass> Entity = null;
public GenericRepository(ApplicationContext context)
{
this.context = context;
Entity = context.Set<EntityClass>();
}
public void Add(ModelClass model)
{
EntityClass enityClass = AutoMapper.Mapper.Map<ModelClass, EntityClass>(model);
this.Entity.Add(enityClass);
}
public void Delete(ModelClass model)
{
EntityClass enityClass = AutoMapper.Mapper.Map<ModelClass, EntityClass>(model);
this.Entity.Remove(enityClass);
context.SaveChanges();
}
public void Update(ModelClass model, int id)
{
EntityClass dbEntity = Entity.Find(id);
EntityClass entityClass = AutoMapper.Mapper.Map<ModelClass, EntityClass>(model);
context.Entry(dbEntity).CurrentValues.SetValues(entityClass);
context.Entry(dbEntity).State = EntityState.Modified;
}
public bool Save()
{
bool status = false;
try
{
int result = context.SaveChanges();
if (result > 0)
{
return status = true;
}
else
return status;
}
catch (DbEntityValidationException dbEx)
{
throw;
}
return status;
}
Student data access interface
public interface IStudent : IRepository<Student, StudentEntity>
{
List<Student> GetStudentById(int studentId);
}
// Implementation of student access interfaces
public class StudentAccess : GenericRepository<Student, StudentEntity>, IStudent
{
public StudentAccess(ApplicationContext context)
: base(context)
{
this.context = context;
}
Public void Add(student std)
{
base.Add(std);
base.save();
}
Public void Update(student std,int studentid)
{
base.update(std,studentId);
base.save();
}
public List<student> GetStudentById()
{
var data=context.student.ToList();
return data;
}
}
Student service layer
public class StudentService
{
ApplicationContext Context;
IStudent student;
public StudentService(ApplicationContext Context, IStudent student)
{
this.Context = Context;
this.student = student;
}
Public void SaveStudent(student std)
{
this.student.Add(std);
}
Public void UpdateStudent(student std,int studentId)
{
this.student.Update(std,studentId);
}
}
Implementation of unity container
public class DependencyOfDependency : UnityContainerExtension
{
protected override void Initialize()
{
// Register Data access layer
Container.RegisterType<DbContext, LogisticsERP.DA.Entities.ApplicationContext>(new ContainerControlledLifetimeManager());
Container.RegisterType< IStudent,StudentAccess>();
//Register Service Layer Classes
Container.RegisterType<StudentService>();
}
}
sql c#-4.0 dependency-injection entity-framework-6 unity-container
add a comment |
I am using Unity IoC in C#, and DbContext with ContainerControlledLifetimeManager for application context with three layer architecture using Entity Framework. I have to ask either this pattern is better in a Windows Forms application or is there any better suggestion. I also face a problem in getting latest data when data is updated in database and after refreshing window form it loads old data.
As I am also using transactions using Entity Framework and therefore I can not use TransientLifetimeManager for DbContext class. I need same context in different DA classes to perform multiple operations in a single transaction. Is there a better approach to tackle this kind of situation?
Here is the project link for demonstration:
Sample Project
Generic Repository
public interface IRepository<ModelClass, EntityClass>
where ModelClass : class
where EntityClass : class
{
void Add(ModelClass entity);
void Delete(ModelClass entity);
void Update(ModelClass entity,int id);
bool Save();
}
Generic Repository implementation
public class GenericRepository<ModelClass, EntityClass> : IRepository<ModelClass, EntityClass>
where ModelClass : class
where EntityClass : class
{
private ApplicationContext context = null;
private DbSet<EntityClass> Entity = null;
public GenericRepository(ApplicationContext context)
{
this.context = context;
Entity = context.Set<EntityClass>();
}
public void Add(ModelClass model)
{
EntityClass enityClass = AutoMapper.Mapper.Map<ModelClass, EntityClass>(model);
this.Entity.Add(enityClass);
}
public void Delete(ModelClass model)
{
EntityClass enityClass = AutoMapper.Mapper.Map<ModelClass, EntityClass>(model);
this.Entity.Remove(enityClass);
context.SaveChanges();
}
public void Update(ModelClass model, int id)
{
EntityClass dbEntity = Entity.Find(id);
EntityClass entityClass = AutoMapper.Mapper.Map<ModelClass, EntityClass>(model);
context.Entry(dbEntity).CurrentValues.SetValues(entityClass);
context.Entry(dbEntity).State = EntityState.Modified;
}
public bool Save()
{
bool status = false;
try
{
int result = context.SaveChanges();
if (result > 0)
{
return status = true;
}
else
return status;
}
catch (DbEntityValidationException dbEx)
{
throw;
}
return status;
}
Student data access interface
public interface IStudent : IRepository<Student, StudentEntity>
{
List<Student> GetStudentById(int studentId);
}
// Implementation of student access interfaces
public class StudentAccess : GenericRepository<Student, StudentEntity>, IStudent
{
public StudentAccess(ApplicationContext context)
: base(context)
{
this.context = context;
}
Public void Add(student std)
{
base.Add(std);
base.save();
}
Public void Update(student std,int studentid)
{
base.update(std,studentId);
base.save();
}
public List<student> GetStudentById()
{
var data=context.student.ToList();
return data;
}
}
Student service layer
public class StudentService
{
ApplicationContext Context;
IStudent student;
public StudentService(ApplicationContext Context, IStudent student)
{
this.Context = Context;
this.student = student;
}
Public void SaveStudent(student std)
{
this.student.Add(std);
}
Public void UpdateStudent(student std,int studentId)
{
this.student.Update(std,studentId);
}
}
Implementation of unity container
public class DependencyOfDependency : UnityContainerExtension
{
protected override void Initialize()
{
// Register Data access layer
Container.RegisterType<DbContext, LogisticsERP.DA.Entities.ApplicationContext>(new ContainerControlledLifetimeManager());
Container.RegisterType< IStudent,StudentAccess>();
//Register Service Layer Classes
Container.RegisterType<StudentService>();
}
}
sql c#-4.0 dependency-injection entity-framework-6 unity-container
I am using Unity IoC in C#, and DbContext with ContainerControlledLifetimeManager for application context with three layer architecture using Entity Framework. I have to ask either this pattern is better in a Windows Forms application or is there any better suggestion. I also face a problem in getting latest data when data is updated in database and after refreshing window form it loads old data.
As I am also using transactions using Entity Framework and therefore I can not use TransientLifetimeManager for DbContext class. I need same context in different DA classes to perform multiple operations in a single transaction. Is there a better approach to tackle this kind of situation?
Here is the project link for demonstration:
Sample Project
Generic Repository
public interface IRepository<ModelClass, EntityClass>
where ModelClass : class
where EntityClass : class
{
void Add(ModelClass entity);
void Delete(ModelClass entity);
void Update(ModelClass entity,int id);
bool Save();
}
Generic Repository implementation
public class GenericRepository<ModelClass, EntityClass> : IRepository<ModelClass, EntityClass>
where ModelClass : class
where EntityClass : class
{
private ApplicationContext context = null;
private DbSet<EntityClass> Entity = null;
public GenericRepository(ApplicationContext context)
{
this.context = context;
Entity = context.Set<EntityClass>();
}
public void Add(ModelClass model)
{
EntityClass enityClass = AutoMapper.Mapper.Map<ModelClass, EntityClass>(model);
this.Entity.Add(enityClass);
}
public void Delete(ModelClass model)
{
EntityClass enityClass = AutoMapper.Mapper.Map<ModelClass, EntityClass>(model);
this.Entity.Remove(enityClass);
context.SaveChanges();
}
public void Update(ModelClass model, int id)
{
EntityClass dbEntity = Entity.Find(id);
EntityClass entityClass = AutoMapper.Mapper.Map<ModelClass, EntityClass>(model);
context.Entry(dbEntity).CurrentValues.SetValues(entityClass);
context.Entry(dbEntity).State = EntityState.Modified;
}
public bool Save()
{
bool status = false;
try
{
int result = context.SaveChanges();
if (result > 0)
{
return status = true;
}
else
return status;
}
catch (DbEntityValidationException dbEx)
{
throw;
}
return status;
}
Student data access interface
public interface IStudent : IRepository<Student, StudentEntity>
{
List<Student> GetStudentById(int studentId);
}
// Implementation of student access interfaces
public class StudentAccess : GenericRepository<Student, StudentEntity>, IStudent
{
public StudentAccess(ApplicationContext context)
: base(context)
{
this.context = context;
}
Public void Add(student std)
{
base.Add(std);
base.save();
}
Public void Update(student std,int studentid)
{
base.update(std,studentId);
base.save();
}
public List<student> GetStudentById()
{
var data=context.student.ToList();
return data;
}
}
Student service layer
public class StudentService
{
ApplicationContext Context;
IStudent student;
public StudentService(ApplicationContext Context, IStudent student)
{
this.Context = Context;
this.student = student;
}
Public void SaveStudent(student std)
{
this.student.Add(std);
}
Public void UpdateStudent(student std,int studentId)
{
this.student.Update(std,studentId);
}
}
Implementation of unity container
public class DependencyOfDependency : UnityContainerExtension
{
protected override void Initialize()
{
// Register Data access layer
Container.RegisterType<DbContext, LogisticsERP.DA.Entities.ApplicationContext>(new ContainerControlledLifetimeManager());
Container.RegisterType< IStudent,StudentAccess>();
//Register Service Layer Classes
Container.RegisterType<StudentService>();
}
}
sql c#-4.0 dependency-injection entity-framework-6 unity-container
sql c#-4.0 dependency-injection entity-framework-6 unity-container
edited Jan 3 at 7:02


Peter Mortensen
13.8k1986113
13.8k1986113
asked Jan 2 at 15:51


amir javaidamir javaid
11
11
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54009293%2funity-ioc-database-transaction-not-fetching-updated-data-using-containercontro%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54009293%2funity-ioc-database-transaction-not-fetching-updated-data-using-containercontro%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ngR2RyK,GJYUv4X383g63JneF0rA,7 Q4HAXv7iW KrAw,RiK,3LCo,9py3,kF50on94