Java Fx Splash screen with spring boot
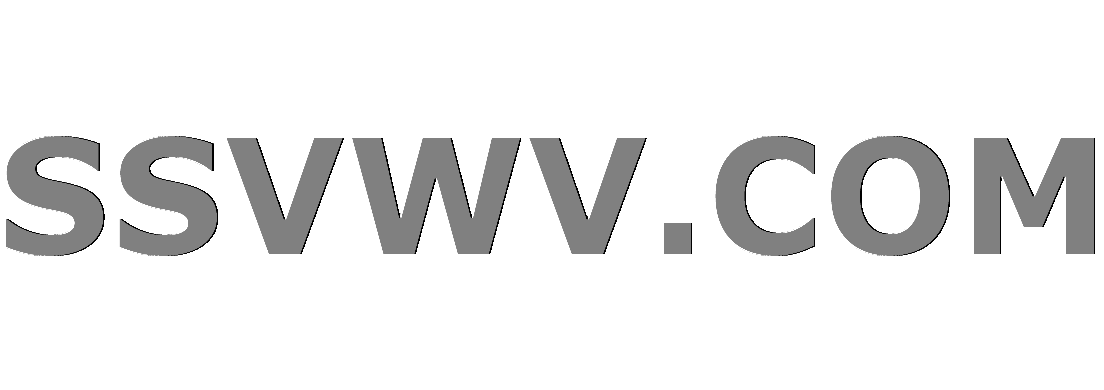
Multi tool use
JavaFX 11 and Spring Boot 2.0.
I want to display a splash screen until Spring inits all of its necessary beans, and in the spring.run() I want to close the splash stage(or at least after x amount of seconds). Such that connecting to the DB creating POJOs etc. But when I try to show my splash screen before FX thread kicks in so it throws:
Caused by: java.lang.IllegalStateException: This operation is permitted on the event thread only; currentThread = main()
I even tried in the Platform.runLater()
but still did not work out. Is there any work around for this problem? Thanks.
public class StartUp extends Application{
public static void main(String args) {
loadSplashScreen();
appContext = SpringApplication.run(StartUp.class);
launch(args);
}
@Override
public void start(Stage stage) {
Parent root = FXMLLoader.load(getClass().getResource("sample.fxml"));
stage.setScene(new Scene(root, 300, 275));
stage.show();
}
static void loadSplashScreen() {
Stage splashStage = new Stage();
try {
BorderPane splashPane = FXMLLoader.load(getClass().getResource("splash.fxml"));
Scene splashScene = new Scene(splashPane);
splashStage.setScene(splashScene);
splashStage.show();
setFadeInOut(splashPane, splashStage);
} catch (IOException e) {
e.printStackTrace();
}
}
static void setFadeInOut(Parent splashScene, Stage splashStage) {
FadeTransition fadeIn = new FadeTransition(Duration.seconds(3), splashScene);
fadeIn.setFromValue(0);
fadeIn.setToValue(1);
fadeIn.setCycleCount(1);
FadeTransition fadeOut = new FadeTransition(Duration.seconds(3), splashScene);
fadeOut.setFromValue(1);
fadeOut.setToValue(0);
fadeOut.setCycleCount(1);
fadeIn.play();
fadeIn.setOnFinished((e) -> fadeOut.play());
fadeOut.setOnFinished((e) -> splashStage.close());
}
}
java spring-boot javafx javafx-11
add a comment |
JavaFX 11 and Spring Boot 2.0.
I want to display a splash screen until Spring inits all of its necessary beans, and in the spring.run() I want to close the splash stage(or at least after x amount of seconds). Such that connecting to the DB creating POJOs etc. But when I try to show my splash screen before FX thread kicks in so it throws:
Caused by: java.lang.IllegalStateException: This operation is permitted on the event thread only; currentThread = main()
I even tried in the Platform.runLater()
but still did not work out. Is there any work around for this problem? Thanks.
public class StartUp extends Application{
public static void main(String args) {
loadSplashScreen();
appContext = SpringApplication.run(StartUp.class);
launch(args);
}
@Override
public void start(Stage stage) {
Parent root = FXMLLoader.load(getClass().getResource("sample.fxml"));
stage.setScene(new Scene(root, 300, 275));
stage.show();
}
static void loadSplashScreen() {
Stage splashStage = new Stage();
try {
BorderPane splashPane = FXMLLoader.load(getClass().getResource("splash.fxml"));
Scene splashScene = new Scene(splashPane);
splashStage.setScene(splashScene);
splashStage.show();
setFadeInOut(splashPane, splashStage);
} catch (IOException e) {
e.printStackTrace();
}
}
static void setFadeInOut(Parent splashScene, Stage splashStage) {
FadeTransition fadeIn = new FadeTransition(Duration.seconds(3), splashScene);
fadeIn.setFromValue(0);
fadeIn.setToValue(1);
fadeIn.setCycleCount(1);
FadeTransition fadeOut = new FadeTransition(Duration.seconds(3), splashScene);
fadeOut.setFromValue(1);
fadeOut.setToValue(0);
fadeOut.setCycleCount(1);
fadeIn.play();
fadeIn.setOnFinished((e) -> fadeOut.play());
fadeOut.setOnFinished((e) -> splashStage.close());
}
}
java spring-boot javafx javafx-11
Have you considered using aPreloader
? See this answer. APreloader
is part of the JavaFX life-cycle so you won't be able to show it "before FX thread kicks in". However, I'm not familiar with Spring Boot but if you can bootstrap it from theApplication.init
method it may fit nicely withPreloader
.
– Slaw
Jan 3 at 4:51
you already have the solution?
– EsopMx
Feb 20 at 6:25
add a comment |
JavaFX 11 and Spring Boot 2.0.
I want to display a splash screen until Spring inits all of its necessary beans, and in the spring.run() I want to close the splash stage(or at least after x amount of seconds). Such that connecting to the DB creating POJOs etc. But when I try to show my splash screen before FX thread kicks in so it throws:
Caused by: java.lang.IllegalStateException: This operation is permitted on the event thread only; currentThread = main()
I even tried in the Platform.runLater()
but still did not work out. Is there any work around for this problem? Thanks.
public class StartUp extends Application{
public static void main(String args) {
loadSplashScreen();
appContext = SpringApplication.run(StartUp.class);
launch(args);
}
@Override
public void start(Stage stage) {
Parent root = FXMLLoader.load(getClass().getResource("sample.fxml"));
stage.setScene(new Scene(root, 300, 275));
stage.show();
}
static void loadSplashScreen() {
Stage splashStage = new Stage();
try {
BorderPane splashPane = FXMLLoader.load(getClass().getResource("splash.fxml"));
Scene splashScene = new Scene(splashPane);
splashStage.setScene(splashScene);
splashStage.show();
setFadeInOut(splashPane, splashStage);
} catch (IOException e) {
e.printStackTrace();
}
}
static void setFadeInOut(Parent splashScene, Stage splashStage) {
FadeTransition fadeIn = new FadeTransition(Duration.seconds(3), splashScene);
fadeIn.setFromValue(0);
fadeIn.setToValue(1);
fadeIn.setCycleCount(1);
FadeTransition fadeOut = new FadeTransition(Duration.seconds(3), splashScene);
fadeOut.setFromValue(1);
fadeOut.setToValue(0);
fadeOut.setCycleCount(1);
fadeIn.play();
fadeIn.setOnFinished((e) -> fadeOut.play());
fadeOut.setOnFinished((e) -> splashStage.close());
}
}
java spring-boot javafx javafx-11
JavaFX 11 and Spring Boot 2.0.
I want to display a splash screen until Spring inits all of its necessary beans, and in the spring.run() I want to close the splash stage(or at least after x amount of seconds). Such that connecting to the DB creating POJOs etc. But when I try to show my splash screen before FX thread kicks in so it throws:
Caused by: java.lang.IllegalStateException: This operation is permitted on the event thread only; currentThread = main()
I even tried in the Platform.runLater()
but still did not work out. Is there any work around for this problem? Thanks.
public class StartUp extends Application{
public static void main(String args) {
loadSplashScreen();
appContext = SpringApplication.run(StartUp.class);
launch(args);
}
@Override
public void start(Stage stage) {
Parent root = FXMLLoader.load(getClass().getResource("sample.fxml"));
stage.setScene(new Scene(root, 300, 275));
stage.show();
}
static void loadSplashScreen() {
Stage splashStage = new Stage();
try {
BorderPane splashPane = FXMLLoader.load(getClass().getResource("splash.fxml"));
Scene splashScene = new Scene(splashPane);
splashStage.setScene(splashScene);
splashStage.show();
setFadeInOut(splashPane, splashStage);
} catch (IOException e) {
e.printStackTrace();
}
}
static void setFadeInOut(Parent splashScene, Stage splashStage) {
FadeTransition fadeIn = new FadeTransition(Duration.seconds(3), splashScene);
fadeIn.setFromValue(0);
fadeIn.setToValue(1);
fadeIn.setCycleCount(1);
FadeTransition fadeOut = new FadeTransition(Duration.seconds(3), splashScene);
fadeOut.setFromValue(1);
fadeOut.setToValue(0);
fadeOut.setCycleCount(1);
fadeIn.play();
fadeIn.setOnFinished((e) -> fadeOut.play());
fadeOut.setOnFinished((e) -> splashStage.close());
}
}
java spring-boot javafx javafx-11
java spring-boot javafx javafx-11
edited Jan 3 at 4:48
Slaw
9,28131235
9,28131235
asked Jan 2 at 15:58
AkanshaAkansha
104
104
Have you considered using aPreloader
? See this answer. APreloader
is part of the JavaFX life-cycle so you won't be able to show it "before FX thread kicks in". However, I'm not familiar with Spring Boot but if you can bootstrap it from theApplication.init
method it may fit nicely withPreloader
.
– Slaw
Jan 3 at 4:51
you already have the solution?
– EsopMx
Feb 20 at 6:25
add a comment |
Have you considered using aPreloader
? See this answer. APreloader
is part of the JavaFX life-cycle so you won't be able to show it "before FX thread kicks in". However, I'm not familiar with Spring Boot but if you can bootstrap it from theApplication.init
method it may fit nicely withPreloader
.
– Slaw
Jan 3 at 4:51
you already have the solution?
– EsopMx
Feb 20 at 6:25
Have you considered using a
Preloader
? See this answer. A Preloader
is part of the JavaFX life-cycle so you won't be able to show it "before FX thread kicks in". However, I'm not familiar with Spring Boot but if you can bootstrap it from the Application.init
method it may fit nicely with Preloader
.– Slaw
Jan 3 at 4:51
Have you considered using a
Preloader
? See this answer. A Preloader
is part of the JavaFX life-cycle so you won't be able to show it "before FX thread kicks in". However, I'm not familiar with Spring Boot but if you can bootstrap it from the Application.init
method it may fit nicely with Preloader
.– Slaw
Jan 3 at 4:51
you already have the solution?
– EsopMx
Feb 20 at 6:25
you already have the solution?
– EsopMx
Feb 20 at 6:25
add a comment |
1 Answer
1
active
oldest
votes
In your code you have a method called loadSplashScreen()
which you call before the Application.launch()
. It will be the call to the launch
method that starts the JavaFX thread which is why your loadSplashScreen()
method fails i.e. the JavaFX thread hasn't even started when this method is called.
You might want to take a look here at this Oracle tutorial on PreLoaders to understand how to understand a basic example before you try work with starting JavaFX with Spring Boot.
Although I haven't booted JavaFX from Spring Boot, I have done similar in an OSGi bundle and you might like to take a look at my FlexFx GitHub repo here which might give you a few pointers on how to use a pre-loader with Spring Boot but note I currently do not have the ability to display a splash screen in my project.
Finally, your issue would happen on JavaFX-8, 9 or 10. It's not specific to JavaFX-11.
Thanks for the suggestion. But PreLoaders does not work in this matter. If I find out a solution I will post here.
– Akansha
Jan 4 at 19:52
1
@Akansha, what did you try with the Preloader? It should work fine. Instead of initializing Spring Boot in the main() method, you'd do it in the Application init() method. You can also look at this GitHub repo that shows Spring Boot + Preloader: github.com/thomasdarimont/spring-labs/tree/master/…
– Ted M. Young
Jan 4 at 20:17
@Akansha If you don't want to use a preloader then the only other way is to do make a splash screen yourself as if it were a regular scene object and handle the creation and destruction of it yourself but you still must do it on the JavaFX thread i.e. all of the above still applies.
– Kerry
Jan 5 at 9:07
@ted-m-young, that suggestion worked. Created a preloader and placed spring run into init(). Thanks
– Akansha
Jan 12 at 3:04
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54009386%2fjava-fx-splash-screen-with-spring-boot%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
In your code you have a method called loadSplashScreen()
which you call before the Application.launch()
. It will be the call to the launch
method that starts the JavaFX thread which is why your loadSplashScreen()
method fails i.e. the JavaFX thread hasn't even started when this method is called.
You might want to take a look here at this Oracle tutorial on PreLoaders to understand how to understand a basic example before you try work with starting JavaFX with Spring Boot.
Although I haven't booted JavaFX from Spring Boot, I have done similar in an OSGi bundle and you might like to take a look at my FlexFx GitHub repo here which might give you a few pointers on how to use a pre-loader with Spring Boot but note I currently do not have the ability to display a splash screen in my project.
Finally, your issue would happen on JavaFX-8, 9 or 10. It's not specific to JavaFX-11.
Thanks for the suggestion. But PreLoaders does not work in this matter. If I find out a solution I will post here.
– Akansha
Jan 4 at 19:52
1
@Akansha, what did you try with the Preloader? It should work fine. Instead of initializing Spring Boot in the main() method, you'd do it in the Application init() method. You can also look at this GitHub repo that shows Spring Boot + Preloader: github.com/thomasdarimont/spring-labs/tree/master/…
– Ted M. Young
Jan 4 at 20:17
@Akansha If you don't want to use a preloader then the only other way is to do make a splash screen yourself as if it were a regular scene object and handle the creation and destruction of it yourself but you still must do it on the JavaFX thread i.e. all of the above still applies.
– Kerry
Jan 5 at 9:07
@ted-m-young, that suggestion worked. Created a preloader and placed spring run into init(). Thanks
– Akansha
Jan 12 at 3:04
add a comment |
In your code you have a method called loadSplashScreen()
which you call before the Application.launch()
. It will be the call to the launch
method that starts the JavaFX thread which is why your loadSplashScreen()
method fails i.e. the JavaFX thread hasn't even started when this method is called.
You might want to take a look here at this Oracle tutorial on PreLoaders to understand how to understand a basic example before you try work with starting JavaFX with Spring Boot.
Although I haven't booted JavaFX from Spring Boot, I have done similar in an OSGi bundle and you might like to take a look at my FlexFx GitHub repo here which might give you a few pointers on how to use a pre-loader with Spring Boot but note I currently do not have the ability to display a splash screen in my project.
Finally, your issue would happen on JavaFX-8, 9 or 10. It's not specific to JavaFX-11.
Thanks for the suggestion. But PreLoaders does not work in this matter. If I find out a solution I will post here.
– Akansha
Jan 4 at 19:52
1
@Akansha, what did you try with the Preloader? It should work fine. Instead of initializing Spring Boot in the main() method, you'd do it in the Application init() method. You can also look at this GitHub repo that shows Spring Boot + Preloader: github.com/thomasdarimont/spring-labs/tree/master/…
– Ted M. Young
Jan 4 at 20:17
@Akansha If you don't want to use a preloader then the only other way is to do make a splash screen yourself as if it were a regular scene object and handle the creation and destruction of it yourself but you still must do it on the JavaFX thread i.e. all of the above still applies.
– Kerry
Jan 5 at 9:07
@ted-m-young, that suggestion worked. Created a preloader and placed spring run into init(). Thanks
– Akansha
Jan 12 at 3:04
add a comment |
In your code you have a method called loadSplashScreen()
which you call before the Application.launch()
. It will be the call to the launch
method that starts the JavaFX thread which is why your loadSplashScreen()
method fails i.e. the JavaFX thread hasn't even started when this method is called.
You might want to take a look here at this Oracle tutorial on PreLoaders to understand how to understand a basic example before you try work with starting JavaFX with Spring Boot.
Although I haven't booted JavaFX from Spring Boot, I have done similar in an OSGi bundle and you might like to take a look at my FlexFx GitHub repo here which might give you a few pointers on how to use a pre-loader with Spring Boot but note I currently do not have the ability to display a splash screen in my project.
Finally, your issue would happen on JavaFX-8, 9 or 10. It's not specific to JavaFX-11.
In your code you have a method called loadSplashScreen()
which you call before the Application.launch()
. It will be the call to the launch
method that starts the JavaFX thread which is why your loadSplashScreen()
method fails i.e. the JavaFX thread hasn't even started when this method is called.
You might want to take a look here at this Oracle tutorial on PreLoaders to understand how to understand a basic example before you try work with starting JavaFX with Spring Boot.
Although I haven't booted JavaFX from Spring Boot, I have done similar in an OSGi bundle and you might like to take a look at my FlexFx GitHub repo here which might give you a few pointers on how to use a pre-loader with Spring Boot but note I currently do not have the ability to display a splash screen in my project.
Finally, your issue would happen on JavaFX-8, 9 or 10. It's not specific to JavaFX-11.
answered Jan 3 at 8:13


KerryKerry
4,14464179
4,14464179
Thanks for the suggestion. But PreLoaders does not work in this matter. If I find out a solution I will post here.
– Akansha
Jan 4 at 19:52
1
@Akansha, what did you try with the Preloader? It should work fine. Instead of initializing Spring Boot in the main() method, you'd do it in the Application init() method. You can also look at this GitHub repo that shows Spring Boot + Preloader: github.com/thomasdarimont/spring-labs/tree/master/…
– Ted M. Young
Jan 4 at 20:17
@Akansha If you don't want to use a preloader then the only other way is to do make a splash screen yourself as if it were a regular scene object and handle the creation and destruction of it yourself but you still must do it on the JavaFX thread i.e. all of the above still applies.
– Kerry
Jan 5 at 9:07
@ted-m-young, that suggestion worked. Created a preloader and placed spring run into init(). Thanks
– Akansha
Jan 12 at 3:04
add a comment |
Thanks for the suggestion. But PreLoaders does not work in this matter. If I find out a solution I will post here.
– Akansha
Jan 4 at 19:52
1
@Akansha, what did you try with the Preloader? It should work fine. Instead of initializing Spring Boot in the main() method, you'd do it in the Application init() method. You can also look at this GitHub repo that shows Spring Boot + Preloader: github.com/thomasdarimont/spring-labs/tree/master/…
– Ted M. Young
Jan 4 at 20:17
@Akansha If you don't want to use a preloader then the only other way is to do make a splash screen yourself as if it were a regular scene object and handle the creation and destruction of it yourself but you still must do it on the JavaFX thread i.e. all of the above still applies.
– Kerry
Jan 5 at 9:07
@ted-m-young, that suggestion worked. Created a preloader and placed spring run into init(). Thanks
– Akansha
Jan 12 at 3:04
Thanks for the suggestion. But PreLoaders does not work in this matter. If I find out a solution I will post here.
– Akansha
Jan 4 at 19:52
Thanks for the suggestion. But PreLoaders does not work in this matter. If I find out a solution I will post here.
– Akansha
Jan 4 at 19:52
1
1
@Akansha, what did you try with the Preloader? It should work fine. Instead of initializing Spring Boot in the main() method, you'd do it in the Application init() method. You can also look at this GitHub repo that shows Spring Boot + Preloader: github.com/thomasdarimont/spring-labs/tree/master/…
– Ted M. Young
Jan 4 at 20:17
@Akansha, what did you try with the Preloader? It should work fine. Instead of initializing Spring Boot in the main() method, you'd do it in the Application init() method. You can also look at this GitHub repo that shows Spring Boot + Preloader: github.com/thomasdarimont/spring-labs/tree/master/…
– Ted M. Young
Jan 4 at 20:17
@Akansha If you don't want to use a preloader then the only other way is to do make a splash screen yourself as if it were a regular scene object and handle the creation and destruction of it yourself but you still must do it on the JavaFX thread i.e. all of the above still applies.
– Kerry
Jan 5 at 9:07
@Akansha If you don't want to use a preloader then the only other way is to do make a splash screen yourself as if it were a regular scene object and handle the creation and destruction of it yourself but you still must do it on the JavaFX thread i.e. all of the above still applies.
– Kerry
Jan 5 at 9:07
@ted-m-young, that suggestion worked. Created a preloader and placed spring run into init(). Thanks
– Akansha
Jan 12 at 3:04
@ted-m-young, that suggestion worked. Created a preloader and placed spring run into init(). Thanks
– Akansha
Jan 12 at 3:04
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54009386%2fjava-fx-splash-screen-with-spring-boot%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
2 ZEoRfewOlEP9L kpf0IoijB,HkS4RJbL6b,0l,Ia1 4LABhk3OSY,u
Have you considered using a
Preloader
? See this answer. APreloader
is part of the JavaFX life-cycle so you won't be able to show it "before FX thread kicks in". However, I'm not familiar with Spring Boot but if you can bootstrap it from theApplication.init
method it may fit nicely withPreloader
.– Slaw
Jan 3 at 4:51
you already have the solution?
– EsopMx
Feb 20 at 6:25