How To Handle conditional Routing or Component Navigation Without React Router
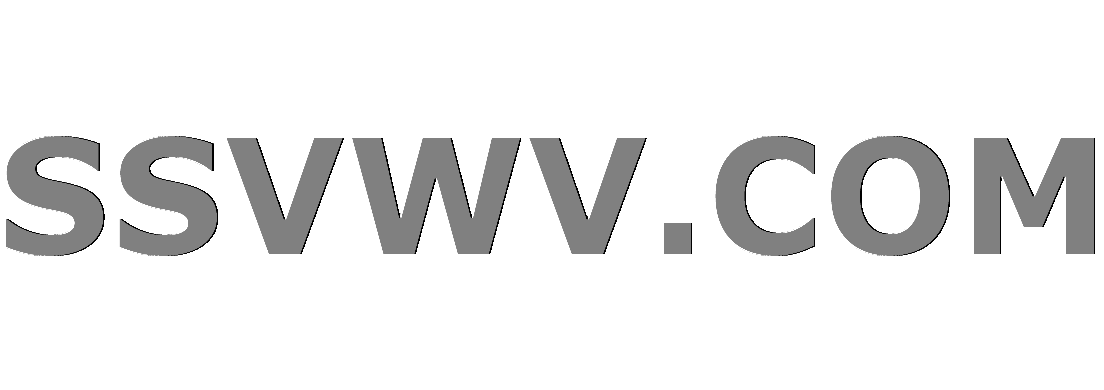
Multi tool use
I need to navigate between components based on several conditions, and I do not want routes to be displayed in the browser, say localhost:3000/step1 or localhost:3000/step2. The whole application is guided so that a user have to answer all the steps to reach the final result.
ATM I have a main container which handles the component rendering based on the Redux store value.
import React, { Component } from "react";
class Home extends Component {
renderComponent = screen => {
switch (screen) {
case 'SCREEN_A':
return <ScreenA />;
case 'SCREEN_B':
return <ScreenB />;
case 'SCREEN_C':
return <ScreenC />;
case 'SCREEN_D':
return <ScreenD />;
default:
return <ScreenA />;
}
};
render() {
return <div>{this.renderComponent(this.props.currentScreen)}</div>;
}
}
function mapStateToProps(storeData) {
return {
store: storeData,
currentScreen: storeData.appState.currentScreen,
userData: storeData.userData
};
}
export default connect(mapStateToProps)(Home);
The problem is I have to use dispatch to trigger navigation
navigateTo(screens[destination])
navigateBackward(currentScreen)
navigateForward(currentScreen)
in almost all components. I do have a predefined JSON for each component which contains the destination for each screen.
screens : {
SCREEN_A:{
id: 1,
name: 'SCREEN_A',
next: 'SCREEN_B',
back: 'WELCOME_SCREEN',
activeLoader: true,
},
SCREEN_B:{
id: 2,
name: 'SCREEN_B',
next: 'SCREEN_C',
back: 'WELCOME_SCREEN',
activeLoader: true,
},
SCREEN_C:{
id: 3,
name: 'SCREEN_C',
next: 'SCREEN_D',
back: 'SCREEN_A',
activeLoader: true,
},
SCREEN_D:{
id: 4,
name: 'SCREEN_D',
next: 'SCREEN_E',
back: 'SCREEN_D',
activeLoader: true,
},
}
And there are protected screens which makes things way more complicated. Is there a better way of doing this with redux? or should I create a middleware and intercept each state change and calculate the next screen.
reactjs react-redux
add a comment |
I need to navigate between components based on several conditions, and I do not want routes to be displayed in the browser, say localhost:3000/step1 or localhost:3000/step2. The whole application is guided so that a user have to answer all the steps to reach the final result.
ATM I have a main container which handles the component rendering based on the Redux store value.
import React, { Component } from "react";
class Home extends Component {
renderComponent = screen => {
switch (screen) {
case 'SCREEN_A':
return <ScreenA />;
case 'SCREEN_B':
return <ScreenB />;
case 'SCREEN_C':
return <ScreenC />;
case 'SCREEN_D':
return <ScreenD />;
default:
return <ScreenA />;
}
};
render() {
return <div>{this.renderComponent(this.props.currentScreen)}</div>;
}
}
function mapStateToProps(storeData) {
return {
store: storeData,
currentScreen: storeData.appState.currentScreen,
userData: storeData.userData
};
}
export default connect(mapStateToProps)(Home);
The problem is I have to use dispatch to trigger navigation
navigateTo(screens[destination])
navigateBackward(currentScreen)
navigateForward(currentScreen)
in almost all components. I do have a predefined JSON for each component which contains the destination for each screen.
screens : {
SCREEN_A:{
id: 1,
name: 'SCREEN_A',
next: 'SCREEN_B',
back: 'WELCOME_SCREEN',
activeLoader: true,
},
SCREEN_B:{
id: 2,
name: 'SCREEN_B',
next: 'SCREEN_C',
back: 'WELCOME_SCREEN',
activeLoader: true,
},
SCREEN_C:{
id: 3,
name: 'SCREEN_C',
next: 'SCREEN_D',
back: 'SCREEN_A',
activeLoader: true,
},
SCREEN_D:{
id: 4,
name: 'SCREEN_D',
next: 'SCREEN_E',
back: 'SCREEN_D',
activeLoader: true,
},
}
And there are protected screens which makes things way more complicated. Is there a better way of doing this with redux? or should I create a middleware and intercept each state change and calculate the next screen.
reactjs react-redux
add a comment |
I need to navigate between components based on several conditions, and I do not want routes to be displayed in the browser, say localhost:3000/step1 or localhost:3000/step2. The whole application is guided so that a user have to answer all the steps to reach the final result.
ATM I have a main container which handles the component rendering based on the Redux store value.
import React, { Component } from "react";
class Home extends Component {
renderComponent = screen => {
switch (screen) {
case 'SCREEN_A':
return <ScreenA />;
case 'SCREEN_B':
return <ScreenB />;
case 'SCREEN_C':
return <ScreenC />;
case 'SCREEN_D':
return <ScreenD />;
default:
return <ScreenA />;
}
};
render() {
return <div>{this.renderComponent(this.props.currentScreen)}</div>;
}
}
function mapStateToProps(storeData) {
return {
store: storeData,
currentScreen: storeData.appState.currentScreen,
userData: storeData.userData
};
}
export default connect(mapStateToProps)(Home);
The problem is I have to use dispatch to trigger navigation
navigateTo(screens[destination])
navigateBackward(currentScreen)
navigateForward(currentScreen)
in almost all components. I do have a predefined JSON for each component which contains the destination for each screen.
screens : {
SCREEN_A:{
id: 1,
name: 'SCREEN_A',
next: 'SCREEN_B',
back: 'WELCOME_SCREEN',
activeLoader: true,
},
SCREEN_B:{
id: 2,
name: 'SCREEN_B',
next: 'SCREEN_C',
back: 'WELCOME_SCREEN',
activeLoader: true,
},
SCREEN_C:{
id: 3,
name: 'SCREEN_C',
next: 'SCREEN_D',
back: 'SCREEN_A',
activeLoader: true,
},
SCREEN_D:{
id: 4,
name: 'SCREEN_D',
next: 'SCREEN_E',
back: 'SCREEN_D',
activeLoader: true,
},
}
And there are protected screens which makes things way more complicated. Is there a better way of doing this with redux? or should I create a middleware and intercept each state change and calculate the next screen.
reactjs react-redux
I need to navigate between components based on several conditions, and I do not want routes to be displayed in the browser, say localhost:3000/step1 or localhost:3000/step2. The whole application is guided so that a user have to answer all the steps to reach the final result.
ATM I have a main container which handles the component rendering based on the Redux store value.
import React, { Component } from "react";
class Home extends Component {
renderComponent = screen => {
switch (screen) {
case 'SCREEN_A':
return <ScreenA />;
case 'SCREEN_B':
return <ScreenB />;
case 'SCREEN_C':
return <ScreenC />;
case 'SCREEN_D':
return <ScreenD />;
default:
return <ScreenA />;
}
};
render() {
return <div>{this.renderComponent(this.props.currentScreen)}</div>;
}
}
function mapStateToProps(storeData) {
return {
store: storeData,
currentScreen: storeData.appState.currentScreen,
userData: storeData.userData
};
}
export default connect(mapStateToProps)(Home);
The problem is I have to use dispatch to trigger navigation
navigateTo(screens[destination])
navigateBackward(currentScreen)
navigateForward(currentScreen)
in almost all components. I do have a predefined JSON for each component which contains the destination for each screen.
screens : {
SCREEN_A:{
id: 1,
name: 'SCREEN_A',
next: 'SCREEN_B',
back: 'WELCOME_SCREEN',
activeLoader: true,
},
SCREEN_B:{
id: 2,
name: 'SCREEN_B',
next: 'SCREEN_C',
back: 'WELCOME_SCREEN',
activeLoader: true,
},
SCREEN_C:{
id: 3,
name: 'SCREEN_C',
next: 'SCREEN_D',
back: 'SCREEN_A',
activeLoader: true,
},
SCREEN_D:{
id: 4,
name: 'SCREEN_D',
next: 'SCREEN_E',
back: 'SCREEN_D',
activeLoader: true,
},
}
And there are protected screens which makes things way more complicated. Is there a better way of doing this with redux? or should I create a middleware and intercept each state change and calculate the next screen.
reactjs react-redux
reactjs react-redux
asked Jan 2 at 15:54


shankara narayananshankara narayanan
566
566
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I would change a few things:
Make your steps/screens dynamic. By putting them into an Array and using the index to determine the current step it removes a lot of code and will make it easier to add/move steps.
Store the steps/screens config in the redux store.
Optionally, you can pass the nextStep and previousStep to the StepComponent. e.g.
<StepComponent nextStep={nextStep} previousStep={previousStep} />
.
In your last step, you probably want to call a different action instead of nextStep.
Here's what my solution would look like:
// Home.jsx
import React, { Component } from 'react';
import * as types from '../../redux/Actor/Actor.types';
class Home extends Component {
stepComponents = [
ScreenA,
ScreenB,
ScreenC,
ScreenD,
];
render() {
const { step, steps } = this.props;
const StepComponent = this.stepComponents[step];
return (
<div>
<StepComponent {...steps[step]} />
</div>
);
}
}
// store.jsx
export default {
step : 0,
steps: [
{
id : 1,
name : 'SCREEN_A',
activeLoader: true,
},
....
],
};
// actions.jsx
export const nextStep = () => ({ type: 'NEXT_STEP' });
export const previousStep = () => ({ type: 'PREVIOUS_STEP' });
// reducers.jsx
export const nextStep = state => ({ ...state, step: state.step + 1 });
export const previousStep = state => ({ ...state, step: state.step - 1 });
thanks for the answer, Sorry took some time to actually try this solution. It works especially the dynamic component rendering part. Is it possible to build a navigation system that watches the store and decides what should be the next step. example: I am doing an Api call and setting getData.isFetching = true, then getData.status = 200, then oh you got some data from getData so navigate to screenD. is this possible with reselect or any other approach ?
– shankara narayanan
Jan 7 at 19:33
Sure, you could use an extra library like redux-sagas/redux-thunk or you could make the call in the component and handle the response from there. You would need to add a new action likeGOTO_STEP
with anindex
parameter and call this action after the response.
– Christiaan
Jan 9 at 9:21
Great thanks, I am using thunks for async but I have to manually mention which screen it should go next. Let’s say the index should be passed after each api call and based on the output I have to navigate to index {n}. Where n should be calculated based on the current state
– shankara narayanan
Jan 9 at 9:35
Yes, that's also possible. In this case, that logic should live in a reducer. If you dispatch aRESPONSE_SUCCESS
action and pass the response, you should be able to calculate the next step using the current state and response data.
– Christiaan
Jan 9 at 9:46
Oh, I was doing the same in another actionCreator. So I did if(response.message) dispatch(navigateTo(currentId)), so I use current ID to compute the next destination and dispatch another action
– shankara narayanan
Jan 9 at 9:49
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54009323%2fhow-to-handle-conditional-routing-or-component-navigation-without-react-router%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I would change a few things:
Make your steps/screens dynamic. By putting them into an Array and using the index to determine the current step it removes a lot of code and will make it easier to add/move steps.
Store the steps/screens config in the redux store.
Optionally, you can pass the nextStep and previousStep to the StepComponent. e.g.
<StepComponent nextStep={nextStep} previousStep={previousStep} />
.
In your last step, you probably want to call a different action instead of nextStep.
Here's what my solution would look like:
// Home.jsx
import React, { Component } from 'react';
import * as types from '../../redux/Actor/Actor.types';
class Home extends Component {
stepComponents = [
ScreenA,
ScreenB,
ScreenC,
ScreenD,
];
render() {
const { step, steps } = this.props;
const StepComponent = this.stepComponents[step];
return (
<div>
<StepComponent {...steps[step]} />
</div>
);
}
}
// store.jsx
export default {
step : 0,
steps: [
{
id : 1,
name : 'SCREEN_A',
activeLoader: true,
},
....
],
};
// actions.jsx
export const nextStep = () => ({ type: 'NEXT_STEP' });
export const previousStep = () => ({ type: 'PREVIOUS_STEP' });
// reducers.jsx
export const nextStep = state => ({ ...state, step: state.step + 1 });
export const previousStep = state => ({ ...state, step: state.step - 1 });
thanks for the answer, Sorry took some time to actually try this solution. It works especially the dynamic component rendering part. Is it possible to build a navigation system that watches the store and decides what should be the next step. example: I am doing an Api call and setting getData.isFetching = true, then getData.status = 200, then oh you got some data from getData so navigate to screenD. is this possible with reselect or any other approach ?
– shankara narayanan
Jan 7 at 19:33
Sure, you could use an extra library like redux-sagas/redux-thunk or you could make the call in the component and handle the response from there. You would need to add a new action likeGOTO_STEP
with anindex
parameter and call this action after the response.
– Christiaan
Jan 9 at 9:21
Great thanks, I am using thunks for async but I have to manually mention which screen it should go next. Let’s say the index should be passed after each api call and based on the output I have to navigate to index {n}. Where n should be calculated based on the current state
– shankara narayanan
Jan 9 at 9:35
Yes, that's also possible. In this case, that logic should live in a reducer. If you dispatch aRESPONSE_SUCCESS
action and pass the response, you should be able to calculate the next step using the current state and response data.
– Christiaan
Jan 9 at 9:46
Oh, I was doing the same in another actionCreator. So I did if(response.message) dispatch(navigateTo(currentId)), so I use current ID to compute the next destination and dispatch another action
– shankara narayanan
Jan 9 at 9:49
|
show 1 more comment
I would change a few things:
Make your steps/screens dynamic. By putting them into an Array and using the index to determine the current step it removes a lot of code and will make it easier to add/move steps.
Store the steps/screens config in the redux store.
Optionally, you can pass the nextStep and previousStep to the StepComponent. e.g.
<StepComponent nextStep={nextStep} previousStep={previousStep} />
.
In your last step, you probably want to call a different action instead of nextStep.
Here's what my solution would look like:
// Home.jsx
import React, { Component } from 'react';
import * as types from '../../redux/Actor/Actor.types';
class Home extends Component {
stepComponents = [
ScreenA,
ScreenB,
ScreenC,
ScreenD,
];
render() {
const { step, steps } = this.props;
const StepComponent = this.stepComponents[step];
return (
<div>
<StepComponent {...steps[step]} />
</div>
);
}
}
// store.jsx
export default {
step : 0,
steps: [
{
id : 1,
name : 'SCREEN_A',
activeLoader: true,
},
....
],
};
// actions.jsx
export const nextStep = () => ({ type: 'NEXT_STEP' });
export const previousStep = () => ({ type: 'PREVIOUS_STEP' });
// reducers.jsx
export const nextStep = state => ({ ...state, step: state.step + 1 });
export const previousStep = state => ({ ...state, step: state.step - 1 });
thanks for the answer, Sorry took some time to actually try this solution. It works especially the dynamic component rendering part. Is it possible to build a navigation system that watches the store and decides what should be the next step. example: I am doing an Api call and setting getData.isFetching = true, then getData.status = 200, then oh you got some data from getData so navigate to screenD. is this possible with reselect or any other approach ?
– shankara narayanan
Jan 7 at 19:33
Sure, you could use an extra library like redux-sagas/redux-thunk or you could make the call in the component and handle the response from there. You would need to add a new action likeGOTO_STEP
with anindex
parameter and call this action after the response.
– Christiaan
Jan 9 at 9:21
Great thanks, I am using thunks for async but I have to manually mention which screen it should go next. Let’s say the index should be passed after each api call and based on the output I have to navigate to index {n}. Where n should be calculated based on the current state
– shankara narayanan
Jan 9 at 9:35
Yes, that's also possible. In this case, that logic should live in a reducer. If you dispatch aRESPONSE_SUCCESS
action and pass the response, you should be able to calculate the next step using the current state and response data.
– Christiaan
Jan 9 at 9:46
Oh, I was doing the same in another actionCreator. So I did if(response.message) dispatch(navigateTo(currentId)), so I use current ID to compute the next destination and dispatch another action
– shankara narayanan
Jan 9 at 9:49
|
show 1 more comment
I would change a few things:
Make your steps/screens dynamic. By putting them into an Array and using the index to determine the current step it removes a lot of code and will make it easier to add/move steps.
Store the steps/screens config in the redux store.
Optionally, you can pass the nextStep and previousStep to the StepComponent. e.g.
<StepComponent nextStep={nextStep} previousStep={previousStep} />
.
In your last step, you probably want to call a different action instead of nextStep.
Here's what my solution would look like:
// Home.jsx
import React, { Component } from 'react';
import * as types from '../../redux/Actor/Actor.types';
class Home extends Component {
stepComponents = [
ScreenA,
ScreenB,
ScreenC,
ScreenD,
];
render() {
const { step, steps } = this.props;
const StepComponent = this.stepComponents[step];
return (
<div>
<StepComponent {...steps[step]} />
</div>
);
}
}
// store.jsx
export default {
step : 0,
steps: [
{
id : 1,
name : 'SCREEN_A',
activeLoader: true,
},
....
],
};
// actions.jsx
export const nextStep = () => ({ type: 'NEXT_STEP' });
export const previousStep = () => ({ type: 'PREVIOUS_STEP' });
// reducers.jsx
export const nextStep = state => ({ ...state, step: state.step + 1 });
export const previousStep = state => ({ ...state, step: state.step - 1 });
I would change a few things:
Make your steps/screens dynamic. By putting them into an Array and using the index to determine the current step it removes a lot of code and will make it easier to add/move steps.
Store the steps/screens config in the redux store.
Optionally, you can pass the nextStep and previousStep to the StepComponent. e.g.
<StepComponent nextStep={nextStep} previousStep={previousStep} />
.
In your last step, you probably want to call a different action instead of nextStep.
Here's what my solution would look like:
// Home.jsx
import React, { Component } from 'react';
import * as types from '../../redux/Actor/Actor.types';
class Home extends Component {
stepComponents = [
ScreenA,
ScreenB,
ScreenC,
ScreenD,
];
render() {
const { step, steps } = this.props;
const StepComponent = this.stepComponents[step];
return (
<div>
<StepComponent {...steps[step]} />
</div>
);
}
}
// store.jsx
export default {
step : 0,
steps: [
{
id : 1,
name : 'SCREEN_A',
activeLoader: true,
},
....
],
};
// actions.jsx
export const nextStep = () => ({ type: 'NEXT_STEP' });
export const previousStep = () => ({ type: 'PREVIOUS_STEP' });
// reducers.jsx
export const nextStep = state => ({ ...state, step: state.step + 1 });
export const previousStep = state => ({ ...state, step: state.step - 1 });
answered Jan 2 at 16:43
ChristiaanChristiaan
76136
76136
thanks for the answer, Sorry took some time to actually try this solution. It works especially the dynamic component rendering part. Is it possible to build a navigation system that watches the store and decides what should be the next step. example: I am doing an Api call and setting getData.isFetching = true, then getData.status = 200, then oh you got some data from getData so navigate to screenD. is this possible with reselect or any other approach ?
– shankara narayanan
Jan 7 at 19:33
Sure, you could use an extra library like redux-sagas/redux-thunk or you could make the call in the component and handle the response from there. You would need to add a new action likeGOTO_STEP
with anindex
parameter and call this action after the response.
– Christiaan
Jan 9 at 9:21
Great thanks, I am using thunks for async but I have to manually mention which screen it should go next. Let’s say the index should be passed after each api call and based on the output I have to navigate to index {n}. Where n should be calculated based on the current state
– shankara narayanan
Jan 9 at 9:35
Yes, that's also possible. In this case, that logic should live in a reducer. If you dispatch aRESPONSE_SUCCESS
action and pass the response, you should be able to calculate the next step using the current state and response data.
– Christiaan
Jan 9 at 9:46
Oh, I was doing the same in another actionCreator. So I did if(response.message) dispatch(navigateTo(currentId)), so I use current ID to compute the next destination and dispatch another action
– shankara narayanan
Jan 9 at 9:49
|
show 1 more comment
thanks for the answer, Sorry took some time to actually try this solution. It works especially the dynamic component rendering part. Is it possible to build a navigation system that watches the store and decides what should be the next step. example: I am doing an Api call and setting getData.isFetching = true, then getData.status = 200, then oh you got some data from getData so navigate to screenD. is this possible with reselect or any other approach ?
– shankara narayanan
Jan 7 at 19:33
Sure, you could use an extra library like redux-sagas/redux-thunk or you could make the call in the component and handle the response from there. You would need to add a new action likeGOTO_STEP
with anindex
parameter and call this action after the response.
– Christiaan
Jan 9 at 9:21
Great thanks, I am using thunks for async but I have to manually mention which screen it should go next. Let’s say the index should be passed after each api call and based on the output I have to navigate to index {n}. Where n should be calculated based on the current state
– shankara narayanan
Jan 9 at 9:35
Yes, that's also possible. In this case, that logic should live in a reducer. If you dispatch aRESPONSE_SUCCESS
action and pass the response, you should be able to calculate the next step using the current state and response data.
– Christiaan
Jan 9 at 9:46
Oh, I was doing the same in another actionCreator. So I did if(response.message) dispatch(navigateTo(currentId)), so I use current ID to compute the next destination and dispatch another action
– shankara narayanan
Jan 9 at 9:49
thanks for the answer, Sorry took some time to actually try this solution. It works especially the dynamic component rendering part. Is it possible to build a navigation system that watches the store and decides what should be the next step. example: I am doing an Api call and setting getData.isFetching = true, then getData.status = 200, then oh you got some data from getData so navigate to screenD. is this possible with reselect or any other approach ?
– shankara narayanan
Jan 7 at 19:33
thanks for the answer, Sorry took some time to actually try this solution. It works especially the dynamic component rendering part. Is it possible to build a navigation system that watches the store and decides what should be the next step. example: I am doing an Api call and setting getData.isFetching = true, then getData.status = 200, then oh you got some data from getData so navigate to screenD. is this possible with reselect or any other approach ?
– shankara narayanan
Jan 7 at 19:33
Sure, you could use an extra library like redux-sagas/redux-thunk or you could make the call in the component and handle the response from there. You would need to add a new action like
GOTO_STEP
with an index
parameter and call this action after the response.– Christiaan
Jan 9 at 9:21
Sure, you could use an extra library like redux-sagas/redux-thunk or you could make the call in the component and handle the response from there. You would need to add a new action like
GOTO_STEP
with an index
parameter and call this action after the response.– Christiaan
Jan 9 at 9:21
Great thanks, I am using thunks for async but I have to manually mention which screen it should go next. Let’s say the index should be passed after each api call and based on the output I have to navigate to index {n}. Where n should be calculated based on the current state
– shankara narayanan
Jan 9 at 9:35
Great thanks, I am using thunks for async but I have to manually mention which screen it should go next. Let’s say the index should be passed after each api call and based on the output I have to navigate to index {n}. Where n should be calculated based on the current state
– shankara narayanan
Jan 9 at 9:35
Yes, that's also possible. In this case, that logic should live in a reducer. If you dispatch a
RESPONSE_SUCCESS
action and pass the response, you should be able to calculate the next step using the current state and response data.– Christiaan
Jan 9 at 9:46
Yes, that's also possible. In this case, that logic should live in a reducer. If you dispatch a
RESPONSE_SUCCESS
action and pass the response, you should be able to calculate the next step using the current state and response data.– Christiaan
Jan 9 at 9:46
Oh, I was doing the same in another actionCreator. So I did if(response.message) dispatch(navigateTo(currentId)), so I use current ID to compute the next destination and dispatch another action
– shankara narayanan
Jan 9 at 9:49
Oh, I was doing the same in another actionCreator. So I did if(response.message) dispatch(navigateTo(currentId)), so I use current ID to compute the next destination and dispatch another action
– shankara narayanan
Jan 9 at 9:49
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54009323%2fhow-to-handle-conditional-routing-or-component-navigation-without-react-router%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
HqjWx2fa sI8BB