Laravel many to many with input pivot table
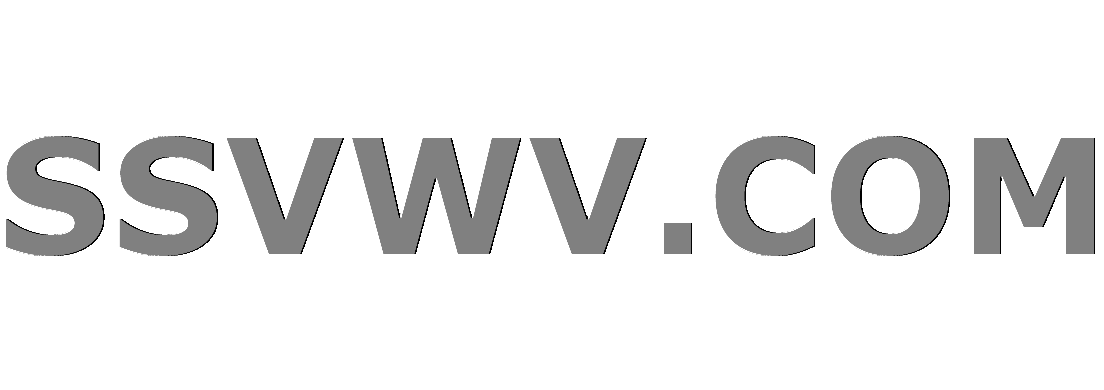
Multi tool use
This is a system for borrowing laboratory equipment, where users are required to fill in their personal data first before being able to borrow laboratory equipment. There is a tool lending form consisting of, name and address that will be stored in the "loan_equipment" table, selecting a list of tools taken from the "lab_equipment" table, and the data will be stored in the "loan_lists" table with the many to many method.
I have 3 tables
loan_equipment table (id, name, address),
lab_equipment table (id, name),
loan_lists table (loan_equipment_id, lab_equipment_id, amount)
This is the LoanEquipment.php model:
public function labEquipment()
{
return $this->belongsToMany('AppLabEquipment', 'loan_lists', 'loan_equipment_id',
'lab_equipment_id')->withTimestamps();
}
This is the LabEquipment.php model:
public function loanEquipment()
{
return $this->hasMany('AppLoanEquipment');
}
This is the LoanEquipmentController.php controller:
public function store(Request $request)
{
$this->validate($request, [
'name' => 'required|max:128',
'address' => 'required',
'labEquipment' => 'required'
]);
$input = LoanEquipment::create($request->all());
$input->labEquipment()->attach($request->input('labEquipment'));
Session::flash("flash_notification", [
"level" => "success",
"message" => "Success $input->name."
]);
return redirect()->route('loan.index');
}
This is a view or form to fill in the loan list tool form.blade.php:
Input Name:
<div class="form-group {{ $errors->has('name') ? 'has-error' : '' }}">
{!! Form::label('name', 'Nama', ['class' => 'col-md-2 control-label']) !!}
<div class="col-md-6">
{!! Form::text('name', null, ['class' => 'form-control']) !!}
<strong>{!! $errors->first('name', '<p class="help-block">:message</p>') !!}</strong>
</div>
Input Address:
<div class="form-group {{ $errors->has('address') ? 'has-error' : '' }}">
{!! Form::label('address', 'Address', ['class' => 'col-md-2 control-label']) !!}
<div class="col-md-6">
{!! Form::text('address', null, ['class' => 'form-control']) !!}
<strong>{!! $errors->first('address', '<p class="help-block">:message</p>') !!}</strong>
</div>
Selected and Input labEquipment and amount
<div class="form-group {{ $errors->has('labEquipment') ? 'has-error' : '' }}">
{!! Form::label('labEquipment', 'Daftar Alat Laboratorium', ['class' => 'col-md-2 control-label']) !!}
<div class="col-md-6">
@if(count($listsLabEquipment) > 0)
@foreach($listsLabEquipment as $key => $value)
<div class="checkbox">
<label for="">{!! Form::checkbox('labEquipment', $key, null) !!}
{{ $value }}
</label>
</div>
{!! Form::text('amount', null, ['class' => 'form-control']) !!}
@endforeach
@endif
<strong>{!! $errors->first('labEquipment', '<p class="help-block">:message</p>') !!}</strong>
</div>
How can I add or enter data on the number of borrowed tools through the tool lending form? And stored in the "loan_lists" table with the name "amount". How should the code I make in the controller, model, and view?
Thank you for your help
php laravel laravel-5
add a comment |
This is a system for borrowing laboratory equipment, where users are required to fill in their personal data first before being able to borrow laboratory equipment. There is a tool lending form consisting of, name and address that will be stored in the "loan_equipment" table, selecting a list of tools taken from the "lab_equipment" table, and the data will be stored in the "loan_lists" table with the many to many method.
I have 3 tables
loan_equipment table (id, name, address),
lab_equipment table (id, name),
loan_lists table (loan_equipment_id, lab_equipment_id, amount)
This is the LoanEquipment.php model:
public function labEquipment()
{
return $this->belongsToMany('AppLabEquipment', 'loan_lists', 'loan_equipment_id',
'lab_equipment_id')->withTimestamps();
}
This is the LabEquipment.php model:
public function loanEquipment()
{
return $this->hasMany('AppLoanEquipment');
}
This is the LoanEquipmentController.php controller:
public function store(Request $request)
{
$this->validate($request, [
'name' => 'required|max:128',
'address' => 'required',
'labEquipment' => 'required'
]);
$input = LoanEquipment::create($request->all());
$input->labEquipment()->attach($request->input('labEquipment'));
Session::flash("flash_notification", [
"level" => "success",
"message" => "Success $input->name."
]);
return redirect()->route('loan.index');
}
This is a view or form to fill in the loan list tool form.blade.php:
Input Name:
<div class="form-group {{ $errors->has('name') ? 'has-error' : '' }}">
{!! Form::label('name', 'Nama', ['class' => 'col-md-2 control-label']) !!}
<div class="col-md-6">
{!! Form::text('name', null, ['class' => 'form-control']) !!}
<strong>{!! $errors->first('name', '<p class="help-block">:message</p>') !!}</strong>
</div>
Input Address:
<div class="form-group {{ $errors->has('address') ? 'has-error' : '' }}">
{!! Form::label('address', 'Address', ['class' => 'col-md-2 control-label']) !!}
<div class="col-md-6">
{!! Form::text('address', null, ['class' => 'form-control']) !!}
<strong>{!! $errors->first('address', '<p class="help-block">:message</p>') !!}</strong>
</div>
Selected and Input labEquipment and amount
<div class="form-group {{ $errors->has('labEquipment') ? 'has-error' : '' }}">
{!! Form::label('labEquipment', 'Daftar Alat Laboratorium', ['class' => 'col-md-2 control-label']) !!}
<div class="col-md-6">
@if(count($listsLabEquipment) > 0)
@foreach($listsLabEquipment as $key => $value)
<div class="checkbox">
<label for="">{!! Form::checkbox('labEquipment', $key, null) !!}
{{ $value }}
</label>
</div>
{!! Form::text('amount', null, ['class' => 'form-control']) !!}
@endforeach
@endif
<strong>{!! $errors->first('labEquipment', '<p class="help-block">:message</p>') !!}</strong>
</div>
How can I add or enter data on the number of borrowed tools through the tool lending form? And stored in the "loan_lists" table with the name "amount". How should the code I make in the controller, model, and view?
Thank you for your help
php laravel laravel-5
add a comment |
This is a system for borrowing laboratory equipment, where users are required to fill in their personal data first before being able to borrow laboratory equipment. There is a tool lending form consisting of, name and address that will be stored in the "loan_equipment" table, selecting a list of tools taken from the "lab_equipment" table, and the data will be stored in the "loan_lists" table with the many to many method.
I have 3 tables
loan_equipment table (id, name, address),
lab_equipment table (id, name),
loan_lists table (loan_equipment_id, lab_equipment_id, amount)
This is the LoanEquipment.php model:
public function labEquipment()
{
return $this->belongsToMany('AppLabEquipment', 'loan_lists', 'loan_equipment_id',
'lab_equipment_id')->withTimestamps();
}
This is the LabEquipment.php model:
public function loanEquipment()
{
return $this->hasMany('AppLoanEquipment');
}
This is the LoanEquipmentController.php controller:
public function store(Request $request)
{
$this->validate($request, [
'name' => 'required|max:128',
'address' => 'required',
'labEquipment' => 'required'
]);
$input = LoanEquipment::create($request->all());
$input->labEquipment()->attach($request->input('labEquipment'));
Session::flash("flash_notification", [
"level" => "success",
"message" => "Success $input->name."
]);
return redirect()->route('loan.index');
}
This is a view or form to fill in the loan list tool form.blade.php:
Input Name:
<div class="form-group {{ $errors->has('name') ? 'has-error' : '' }}">
{!! Form::label('name', 'Nama', ['class' => 'col-md-2 control-label']) !!}
<div class="col-md-6">
{!! Form::text('name', null, ['class' => 'form-control']) !!}
<strong>{!! $errors->first('name', '<p class="help-block">:message</p>') !!}</strong>
</div>
Input Address:
<div class="form-group {{ $errors->has('address') ? 'has-error' : '' }}">
{!! Form::label('address', 'Address', ['class' => 'col-md-2 control-label']) !!}
<div class="col-md-6">
{!! Form::text('address', null, ['class' => 'form-control']) !!}
<strong>{!! $errors->first('address', '<p class="help-block">:message</p>') !!}</strong>
</div>
Selected and Input labEquipment and amount
<div class="form-group {{ $errors->has('labEquipment') ? 'has-error' : '' }}">
{!! Form::label('labEquipment', 'Daftar Alat Laboratorium', ['class' => 'col-md-2 control-label']) !!}
<div class="col-md-6">
@if(count($listsLabEquipment) > 0)
@foreach($listsLabEquipment as $key => $value)
<div class="checkbox">
<label for="">{!! Form::checkbox('labEquipment', $key, null) !!}
{{ $value }}
</label>
</div>
{!! Form::text('amount', null, ['class' => 'form-control']) !!}
@endforeach
@endif
<strong>{!! $errors->first('labEquipment', '<p class="help-block">:message</p>') !!}</strong>
</div>
How can I add or enter data on the number of borrowed tools through the tool lending form? And stored in the "loan_lists" table with the name "amount". How should the code I make in the controller, model, and view?
Thank you for your help
php laravel laravel-5
This is a system for borrowing laboratory equipment, where users are required to fill in their personal data first before being able to borrow laboratory equipment. There is a tool lending form consisting of, name and address that will be stored in the "loan_equipment" table, selecting a list of tools taken from the "lab_equipment" table, and the data will be stored in the "loan_lists" table with the many to many method.
I have 3 tables
loan_equipment table (id, name, address),
lab_equipment table (id, name),
loan_lists table (loan_equipment_id, lab_equipment_id, amount)
This is the LoanEquipment.php model:
public function labEquipment()
{
return $this->belongsToMany('AppLabEquipment', 'loan_lists', 'loan_equipment_id',
'lab_equipment_id')->withTimestamps();
}
This is the LabEquipment.php model:
public function loanEquipment()
{
return $this->hasMany('AppLoanEquipment');
}
This is the LoanEquipmentController.php controller:
public function store(Request $request)
{
$this->validate($request, [
'name' => 'required|max:128',
'address' => 'required',
'labEquipment' => 'required'
]);
$input = LoanEquipment::create($request->all());
$input->labEquipment()->attach($request->input('labEquipment'));
Session::flash("flash_notification", [
"level" => "success",
"message" => "Success $input->name."
]);
return redirect()->route('loan.index');
}
This is a view or form to fill in the loan list tool form.blade.php:
Input Name:
<div class="form-group {{ $errors->has('name') ? 'has-error' : '' }}">
{!! Form::label('name', 'Nama', ['class' => 'col-md-2 control-label']) !!}
<div class="col-md-6">
{!! Form::text('name', null, ['class' => 'form-control']) !!}
<strong>{!! $errors->first('name', '<p class="help-block">:message</p>') !!}</strong>
</div>
Input Address:
<div class="form-group {{ $errors->has('address') ? 'has-error' : '' }}">
{!! Form::label('address', 'Address', ['class' => 'col-md-2 control-label']) !!}
<div class="col-md-6">
{!! Form::text('address', null, ['class' => 'form-control']) !!}
<strong>{!! $errors->first('address', '<p class="help-block">:message</p>') !!}</strong>
</div>
Selected and Input labEquipment and amount
<div class="form-group {{ $errors->has('labEquipment') ? 'has-error' : '' }}">
{!! Form::label('labEquipment', 'Daftar Alat Laboratorium', ['class' => 'col-md-2 control-label']) !!}
<div class="col-md-6">
@if(count($listsLabEquipment) > 0)
@foreach($listsLabEquipment as $key => $value)
<div class="checkbox">
<label for="">{!! Form::checkbox('labEquipment', $key, null) !!}
{{ $value }}
</label>
</div>
{!! Form::text('amount', null, ['class' => 'form-control']) !!}
@endforeach
@endif
<strong>{!! $errors->first('labEquipment', '<p class="help-block">:message</p>') !!}</strong>
</div>
How can I add or enter data on the number of borrowed tools through the tool lending form? And stored in the "loan_lists" table with the name "amount". How should the code I make in the controller, model, and view?
Thank you for your help
php laravel laravel-5
php laravel laravel-5
asked Jan 2 at 10:10
Agus SuparmanAgus Suparman
315
315
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
If i understand correctly you need a many to many relatioship between lab_equipment and loan_equipment. To define a many to many relationship you need to add in both models a belongs to many relationship. As it refers to laravel docs. https://laravel.com/docs/5.7/eloquent-relationships#many-to-many . If you use a custom convention in column names and table names you have to pass them as arguments in relationship declaration as you have already done in your first relationship declaration. To access the pivot table you do something like this
public function labEquipment()
{
return $this->belongsToMany('AppLabEquipment', 'loan_lists', 'loan_equipment_id',
'lab_equipment_id')->withTimestamps()->withPivot('column1', 'column2');
}
what about the form in viewform.blade.php
SelectedlabEquipment
and inputamount
?
– Agus Suparman
Jan 2 at 11:29
By using this $loanEquipment->labEquipment you get all the related data. Then you can check if the specific equipement exists in these data. You can do this with many ways. Please read the laravel docs on how to define a relatioship and how to retrieve it. laravel.com/docs/5.7/eloquent-relationships#many-to-many . Also note that laravel collective forms as i see supports until version 5.4 and i am not a fan of this packages anyway. Last but not least consider using eager loading to avoid n + 1 problems.
– Vaggelis Ksps
Jan 2 at 13:15
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54004440%2flaravel-many-to-many-with-input-pivot-table%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If i understand correctly you need a many to many relatioship between lab_equipment and loan_equipment. To define a many to many relationship you need to add in both models a belongs to many relationship. As it refers to laravel docs. https://laravel.com/docs/5.7/eloquent-relationships#many-to-many . If you use a custom convention in column names and table names you have to pass them as arguments in relationship declaration as you have already done in your first relationship declaration. To access the pivot table you do something like this
public function labEquipment()
{
return $this->belongsToMany('AppLabEquipment', 'loan_lists', 'loan_equipment_id',
'lab_equipment_id')->withTimestamps()->withPivot('column1', 'column2');
}
what about the form in viewform.blade.php
SelectedlabEquipment
and inputamount
?
– Agus Suparman
Jan 2 at 11:29
By using this $loanEquipment->labEquipment you get all the related data. Then you can check if the specific equipement exists in these data. You can do this with many ways. Please read the laravel docs on how to define a relatioship and how to retrieve it. laravel.com/docs/5.7/eloquent-relationships#many-to-many . Also note that laravel collective forms as i see supports until version 5.4 and i am not a fan of this packages anyway. Last but not least consider using eager loading to avoid n + 1 problems.
– Vaggelis Ksps
Jan 2 at 13:15
add a comment |
If i understand correctly you need a many to many relatioship between lab_equipment and loan_equipment. To define a many to many relationship you need to add in both models a belongs to many relationship. As it refers to laravel docs. https://laravel.com/docs/5.7/eloquent-relationships#many-to-many . If you use a custom convention in column names and table names you have to pass them as arguments in relationship declaration as you have already done in your first relationship declaration. To access the pivot table you do something like this
public function labEquipment()
{
return $this->belongsToMany('AppLabEquipment', 'loan_lists', 'loan_equipment_id',
'lab_equipment_id')->withTimestamps()->withPivot('column1', 'column2');
}
what about the form in viewform.blade.php
SelectedlabEquipment
and inputamount
?
– Agus Suparman
Jan 2 at 11:29
By using this $loanEquipment->labEquipment you get all the related data. Then you can check if the specific equipement exists in these data. You can do this with many ways. Please read the laravel docs on how to define a relatioship and how to retrieve it. laravel.com/docs/5.7/eloquent-relationships#many-to-many . Also note that laravel collective forms as i see supports until version 5.4 and i am not a fan of this packages anyway. Last but not least consider using eager loading to avoid n + 1 problems.
– Vaggelis Ksps
Jan 2 at 13:15
add a comment |
If i understand correctly you need a many to many relatioship between lab_equipment and loan_equipment. To define a many to many relationship you need to add in both models a belongs to many relationship. As it refers to laravel docs. https://laravel.com/docs/5.7/eloquent-relationships#many-to-many . If you use a custom convention in column names and table names you have to pass them as arguments in relationship declaration as you have already done in your first relationship declaration. To access the pivot table you do something like this
public function labEquipment()
{
return $this->belongsToMany('AppLabEquipment', 'loan_lists', 'loan_equipment_id',
'lab_equipment_id')->withTimestamps()->withPivot('column1', 'column2');
}
If i understand correctly you need a many to many relatioship between lab_equipment and loan_equipment. To define a many to many relationship you need to add in both models a belongs to many relationship. As it refers to laravel docs. https://laravel.com/docs/5.7/eloquent-relationships#many-to-many . If you use a custom convention in column names and table names you have to pass them as arguments in relationship declaration as you have already done in your first relationship declaration. To access the pivot table you do something like this
public function labEquipment()
{
return $this->belongsToMany('AppLabEquipment', 'loan_lists', 'loan_equipment_id',
'lab_equipment_id')->withTimestamps()->withPivot('column1', 'column2');
}
answered Jan 2 at 10:29
Vaggelis KspsVaggelis Ksps
19019
19019
what about the form in viewform.blade.php
SelectedlabEquipment
and inputamount
?
– Agus Suparman
Jan 2 at 11:29
By using this $loanEquipment->labEquipment you get all the related data. Then you can check if the specific equipement exists in these data. You can do this with many ways. Please read the laravel docs on how to define a relatioship and how to retrieve it. laravel.com/docs/5.7/eloquent-relationships#many-to-many . Also note that laravel collective forms as i see supports until version 5.4 and i am not a fan of this packages anyway. Last but not least consider using eager loading to avoid n + 1 problems.
– Vaggelis Ksps
Jan 2 at 13:15
add a comment |
what about the form in viewform.blade.php
SelectedlabEquipment
and inputamount
?
– Agus Suparman
Jan 2 at 11:29
By using this $loanEquipment->labEquipment you get all the related data. Then you can check if the specific equipement exists in these data. You can do this with many ways. Please read the laravel docs on how to define a relatioship and how to retrieve it. laravel.com/docs/5.7/eloquent-relationships#many-to-many . Also note that laravel collective forms as i see supports until version 5.4 and i am not a fan of this packages anyway. Last but not least consider using eager loading to avoid n + 1 problems.
– Vaggelis Ksps
Jan 2 at 13:15
what about the form in view
form.blade.php
Selected labEquipment
and input amount
?– Agus Suparman
Jan 2 at 11:29
what about the form in view
form.blade.php
Selected labEquipment
and input amount
?– Agus Suparman
Jan 2 at 11:29
By using this $loanEquipment->labEquipment you get all the related data. Then you can check if the specific equipement exists in these data. You can do this with many ways. Please read the laravel docs on how to define a relatioship and how to retrieve it. laravel.com/docs/5.7/eloquent-relationships#many-to-many . Also note that laravel collective forms as i see supports until version 5.4 and i am not a fan of this packages anyway. Last but not least consider using eager loading to avoid n + 1 problems.
– Vaggelis Ksps
Jan 2 at 13:15
By using this $loanEquipment->labEquipment you get all the related data. Then you can check if the specific equipement exists in these data. You can do this with many ways. Please read the laravel docs on how to define a relatioship and how to retrieve it. laravel.com/docs/5.7/eloquent-relationships#many-to-many . Also note that laravel collective forms as i see supports until version 5.4 and i am not a fan of this packages anyway. Last but not least consider using eager loading to avoid n + 1 problems.
– Vaggelis Ksps
Jan 2 at 13:15
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54004440%2flaravel-many-to-many-with-input-pivot-table%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Y8V8tEm32sVzz,ZY8cu6 S,W3qAN,9St4Gs0a