How to get the numbers of data row from sqlite3 database in python
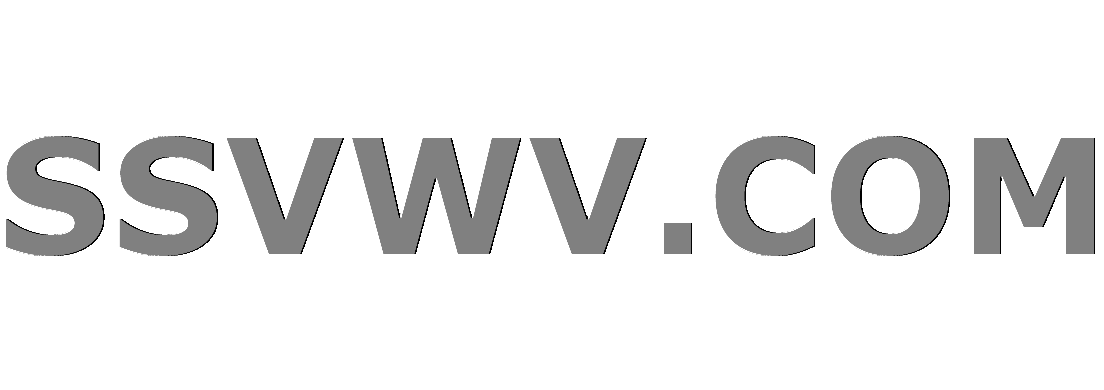
Multi tool use
I am trying to get the numbers of rows returned from an sqlite3 database in python but it seems the feature isn't available:
Think of php
mysqli_num_rows()
in mysql
Although I devised a means but it is a awkward: assuming a class execute sql
and give me the results:
# Query Execution returning a result
data = sql.sqlExec("select * from user")
# run another query for number of row checking, not very good workaround
dataCopy = sql.sqlExec("select * from user")
# Try to cast dataCopy to list and get the length, I did this because i notice as soon
# as I perform any action of the data, data becomes null
# This is not too good as someone else can perform another transaction on the database
# In the nick of time
if len(list(dataCopy)) :
for m in data :
print("Name = {}, Password = {}".format(m["username"], m["password"]));
else :
print("Query return nothing")
Is there a function or property that can do this without stress.
python sqlite sqlite3
add a comment |
I am trying to get the numbers of rows returned from an sqlite3 database in python but it seems the feature isn't available:
Think of php
mysqli_num_rows()
in mysql
Although I devised a means but it is a awkward: assuming a class execute sql
and give me the results:
# Query Execution returning a result
data = sql.sqlExec("select * from user")
# run another query for number of row checking, not very good workaround
dataCopy = sql.sqlExec("select * from user")
# Try to cast dataCopy to list and get the length, I did this because i notice as soon
# as I perform any action of the data, data becomes null
# This is not too good as someone else can perform another transaction on the database
# In the nick of time
if len(list(dataCopy)) :
for m in data :
print("Name = {}, Password = {}".format(m["username"], m["password"]));
else :
print("Query return nothing")
Is there a function or property that can do this without stress.
python sqlite sqlite3
add a comment |
I am trying to get the numbers of rows returned from an sqlite3 database in python but it seems the feature isn't available:
Think of php
mysqli_num_rows()
in mysql
Although I devised a means but it is a awkward: assuming a class execute sql
and give me the results:
# Query Execution returning a result
data = sql.sqlExec("select * from user")
# run another query for number of row checking, not very good workaround
dataCopy = sql.sqlExec("select * from user")
# Try to cast dataCopy to list and get the length, I did this because i notice as soon
# as I perform any action of the data, data becomes null
# This is not too good as someone else can perform another transaction on the database
# In the nick of time
if len(list(dataCopy)) :
for m in data :
print("Name = {}, Password = {}".format(m["username"], m["password"]));
else :
print("Query return nothing")
Is there a function or property that can do this without stress.
python sqlite sqlite3
I am trying to get the numbers of rows returned from an sqlite3 database in python but it seems the feature isn't available:
Think of php
mysqli_num_rows()
in mysql
Although I devised a means but it is a awkward: assuming a class execute sql
and give me the results:
# Query Execution returning a result
data = sql.sqlExec("select * from user")
# run another query for number of row checking, not very good workaround
dataCopy = sql.sqlExec("select * from user")
# Try to cast dataCopy to list and get the length, I did this because i notice as soon
# as I perform any action of the data, data becomes null
# This is not too good as someone else can perform another transaction on the database
# In the nick of time
if len(list(dataCopy)) :
for m in data :
print("Name = {}, Password = {}".format(m["username"], m["password"]));
else :
print("Query return nothing")
Is there a function or property that can do this without stress.
python sqlite sqlite3
python sqlite sqlite3
edited Oct 16 '17 at 21:25
Martijn Pieters♦
718k14025082319
718k14025082319
asked Feb 17 '14 at 12:34
TemitayoTemitayo
3561822
3561822
add a comment |
add a comment |
6 Answers
6
active
oldest
votes
Normally, cursor.rowcount
would give you the number of results of a query.
However, for SQLite, that property is often set to -1 due to the nature of how SQLite produces results. Short of a COUNT()
query first you often won't know the number of results returned.
This is because SQLite produces rows as it finds them in the database, and won't itself know how many rows are produced until the end of the database is reached.
From the documentation of cursor.rowcount
:
Although the
Cursor
class of thesqlite3
module implements this attribute, the database engine’s own support for the determination of “rows affected”/”rows selected” is quirky.
For
executemany()
statements, the number of modifications are summed up intorowcount
.
As required by the Python DB API Spec, the
rowcount
attribute “is -1 in case noexecuteXX()
has been performed on the cursor or the rowcount of the last operation is not determinable by the interface”. This includesSELECT
statements because we cannot determine the number of rows a query produced until all rows were fetched.
Emphasis mine.
For your specific query, you can add a sub-select to add a column:
data = sql.sqlExec("select (select count() from user) as count, * from user")
This is not all that efficient for large tables, however.
If all you need is one row, use cursor.fetchone()
instead:
cursor.execute('SELECT * FROM user WHERE userid=?', (userid,))
row = cursor.fetchone()
if row is None:
raise ValueError('No such user found')
result = "Name = {}, Password = {}".format(row["username"], row["password"])
didn't work returned -1
– Temitayo
Feb 17 '14 at 12:38
data.rowcount
returned -1
– Temitayo
Feb 17 '14 at 12:40
data.COUNT()
returnedAttributeError: 'sqlite3.Cursor' object has no attribute 'COUNT'
– Temitayo
Feb 17 '14 at 12:41
1
@Temitayo:COUNT()
is a SQL function, not a function on the cursor.
– Martijn Pieters♦
Feb 17 '14 at 12:42
"select (select count() from user) as count, * from user"
using this query nearly solve the problem, although there are still some thing i need to figure out, try to pull out the first row and pull out the count without iterating on the datadata[0]["count"]
returnsTypeError: 'sqlite3.Cursor' object is not subscriptable
– Temitayo
Feb 17 '14 at 12:51
|
show 6 more comments
Use following:
dataCopy = sql.sqlExec("select count(*) from user")
values = dataCopy.fetchone()
print values[0]
Good but i want to determine the numbers of row, before doing anything, and what what if i dont what tofetchall
?
– Temitayo
Feb 17 '14 at 12:54
Ok i update it.
– user3273866
Feb 18 '14 at 5:09
add a comment |
I've found the select statement with count() to be slow on a very large DB. Moreover, using fetch all()
can be very memory-intensive.
Unless you explicitly design your database so that it does not have a rowid, you can always try a quick solution
cur.execute("SELECT max(rowid) from Table")
n = cur.fetchone()[0]
This will tell you how many rows your database has.
add a comment |
import sqlite3
conn = sqlite3.connect(path/to/db)
cursor = conn.cursor()
cursor.execute("select * from user")
results = cursor.fetchall()
print len(results)
len(results) is just what you want
you need to add tags to you questions, to get viewer
– Amir Sherafatian
Feb 17 '14 at 14:10
2
For a large table, this is extremely inefficient.
– Ben Crowell
Jul 23 '18 at 17:24
add a comment |
A simple alternative approach here is to use fetchall to pull a column into a python list, then count the length of the list. I don't know if this is pythonic or especially efficient but it seems to work:
rowlist =
c.execute("SELECT {rowid} from {whichTable}".
format (rowid = "rowid", whichTable = whichTable))
rowlist = c.fetchall ()
rowlistcount = len(rowlist)
print (rowlistcount)
For a large table, this is extremely inefficient
– Cypher
Aug 20 '18 at 9:08
add a comment |
this code worked for me:
import sqlite3
con = sqlite3.connect(your_db_file)
cursor = con.cursor()
result = cursor.execute("select count(*) from your_table") #returns array of tupples
num_of_rows = result[0][0]
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f21829266%2fhow-to-get-the-numbers-of-data-row-from-sqlite3-database-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
Normally, cursor.rowcount
would give you the number of results of a query.
However, for SQLite, that property is often set to -1 due to the nature of how SQLite produces results. Short of a COUNT()
query first you often won't know the number of results returned.
This is because SQLite produces rows as it finds them in the database, and won't itself know how many rows are produced until the end of the database is reached.
From the documentation of cursor.rowcount
:
Although the
Cursor
class of thesqlite3
module implements this attribute, the database engine’s own support for the determination of “rows affected”/”rows selected” is quirky.
For
executemany()
statements, the number of modifications are summed up intorowcount
.
As required by the Python DB API Spec, the
rowcount
attribute “is -1 in case noexecuteXX()
has been performed on the cursor or the rowcount of the last operation is not determinable by the interface”. This includesSELECT
statements because we cannot determine the number of rows a query produced until all rows were fetched.
Emphasis mine.
For your specific query, you can add a sub-select to add a column:
data = sql.sqlExec("select (select count() from user) as count, * from user")
This is not all that efficient for large tables, however.
If all you need is one row, use cursor.fetchone()
instead:
cursor.execute('SELECT * FROM user WHERE userid=?', (userid,))
row = cursor.fetchone()
if row is None:
raise ValueError('No such user found')
result = "Name = {}, Password = {}".format(row["username"], row["password"])
didn't work returned -1
– Temitayo
Feb 17 '14 at 12:38
data.rowcount
returned -1
– Temitayo
Feb 17 '14 at 12:40
data.COUNT()
returnedAttributeError: 'sqlite3.Cursor' object has no attribute 'COUNT'
– Temitayo
Feb 17 '14 at 12:41
1
@Temitayo:COUNT()
is a SQL function, not a function on the cursor.
– Martijn Pieters♦
Feb 17 '14 at 12:42
"select (select count() from user) as count, * from user"
using this query nearly solve the problem, although there are still some thing i need to figure out, try to pull out the first row and pull out the count without iterating on the datadata[0]["count"]
returnsTypeError: 'sqlite3.Cursor' object is not subscriptable
– Temitayo
Feb 17 '14 at 12:51
|
show 6 more comments
Normally, cursor.rowcount
would give you the number of results of a query.
However, for SQLite, that property is often set to -1 due to the nature of how SQLite produces results. Short of a COUNT()
query first you often won't know the number of results returned.
This is because SQLite produces rows as it finds them in the database, and won't itself know how many rows are produced until the end of the database is reached.
From the documentation of cursor.rowcount
:
Although the
Cursor
class of thesqlite3
module implements this attribute, the database engine’s own support for the determination of “rows affected”/”rows selected” is quirky.
For
executemany()
statements, the number of modifications are summed up intorowcount
.
As required by the Python DB API Spec, the
rowcount
attribute “is -1 in case noexecuteXX()
has been performed on the cursor or the rowcount of the last operation is not determinable by the interface”. This includesSELECT
statements because we cannot determine the number of rows a query produced until all rows were fetched.
Emphasis mine.
For your specific query, you can add a sub-select to add a column:
data = sql.sqlExec("select (select count() from user) as count, * from user")
This is not all that efficient for large tables, however.
If all you need is one row, use cursor.fetchone()
instead:
cursor.execute('SELECT * FROM user WHERE userid=?', (userid,))
row = cursor.fetchone()
if row is None:
raise ValueError('No such user found')
result = "Name = {}, Password = {}".format(row["username"], row["password"])
didn't work returned -1
– Temitayo
Feb 17 '14 at 12:38
data.rowcount
returned -1
– Temitayo
Feb 17 '14 at 12:40
data.COUNT()
returnedAttributeError: 'sqlite3.Cursor' object has no attribute 'COUNT'
– Temitayo
Feb 17 '14 at 12:41
1
@Temitayo:COUNT()
is a SQL function, not a function on the cursor.
– Martijn Pieters♦
Feb 17 '14 at 12:42
"select (select count() from user) as count, * from user"
using this query nearly solve the problem, although there are still some thing i need to figure out, try to pull out the first row and pull out the count without iterating on the datadata[0]["count"]
returnsTypeError: 'sqlite3.Cursor' object is not subscriptable
– Temitayo
Feb 17 '14 at 12:51
|
show 6 more comments
Normally, cursor.rowcount
would give you the number of results of a query.
However, for SQLite, that property is often set to -1 due to the nature of how SQLite produces results. Short of a COUNT()
query first you often won't know the number of results returned.
This is because SQLite produces rows as it finds them in the database, and won't itself know how many rows are produced until the end of the database is reached.
From the documentation of cursor.rowcount
:
Although the
Cursor
class of thesqlite3
module implements this attribute, the database engine’s own support for the determination of “rows affected”/”rows selected” is quirky.
For
executemany()
statements, the number of modifications are summed up intorowcount
.
As required by the Python DB API Spec, the
rowcount
attribute “is -1 in case noexecuteXX()
has been performed on the cursor or the rowcount of the last operation is not determinable by the interface”. This includesSELECT
statements because we cannot determine the number of rows a query produced until all rows were fetched.
Emphasis mine.
For your specific query, you can add a sub-select to add a column:
data = sql.sqlExec("select (select count() from user) as count, * from user")
This is not all that efficient for large tables, however.
If all you need is one row, use cursor.fetchone()
instead:
cursor.execute('SELECT * FROM user WHERE userid=?', (userid,))
row = cursor.fetchone()
if row is None:
raise ValueError('No such user found')
result = "Name = {}, Password = {}".format(row["username"], row["password"])
Normally, cursor.rowcount
would give you the number of results of a query.
However, for SQLite, that property is often set to -1 due to the nature of how SQLite produces results. Short of a COUNT()
query first you often won't know the number of results returned.
This is because SQLite produces rows as it finds them in the database, and won't itself know how many rows are produced until the end of the database is reached.
From the documentation of cursor.rowcount
:
Although the
Cursor
class of thesqlite3
module implements this attribute, the database engine’s own support for the determination of “rows affected”/”rows selected” is quirky.
For
executemany()
statements, the number of modifications are summed up intorowcount
.
As required by the Python DB API Spec, the
rowcount
attribute “is -1 in case noexecuteXX()
has been performed on the cursor or the rowcount of the last operation is not determinable by the interface”. This includesSELECT
statements because we cannot determine the number of rows a query produced until all rows were fetched.
Emphasis mine.
For your specific query, you can add a sub-select to add a column:
data = sql.sqlExec("select (select count() from user) as count, * from user")
This is not all that efficient for large tables, however.
If all you need is one row, use cursor.fetchone()
instead:
cursor.execute('SELECT * FROM user WHERE userid=?', (userid,))
row = cursor.fetchone()
if row is None:
raise ValueError('No such user found')
result = "Name = {}, Password = {}".format(row["username"], row["password"])
edited Feb 17 '14 at 12:48
answered Feb 17 '14 at 12:35
Martijn Pieters♦Martijn Pieters
718k14025082319
718k14025082319
didn't work returned -1
– Temitayo
Feb 17 '14 at 12:38
data.rowcount
returned -1
– Temitayo
Feb 17 '14 at 12:40
data.COUNT()
returnedAttributeError: 'sqlite3.Cursor' object has no attribute 'COUNT'
– Temitayo
Feb 17 '14 at 12:41
1
@Temitayo:COUNT()
is a SQL function, not a function on the cursor.
– Martijn Pieters♦
Feb 17 '14 at 12:42
"select (select count() from user) as count, * from user"
using this query nearly solve the problem, although there are still some thing i need to figure out, try to pull out the first row and pull out the count without iterating on the datadata[0]["count"]
returnsTypeError: 'sqlite3.Cursor' object is not subscriptable
– Temitayo
Feb 17 '14 at 12:51
|
show 6 more comments
didn't work returned -1
– Temitayo
Feb 17 '14 at 12:38
data.rowcount
returned -1
– Temitayo
Feb 17 '14 at 12:40
data.COUNT()
returnedAttributeError: 'sqlite3.Cursor' object has no attribute 'COUNT'
– Temitayo
Feb 17 '14 at 12:41
1
@Temitayo:COUNT()
is a SQL function, not a function on the cursor.
– Martijn Pieters♦
Feb 17 '14 at 12:42
"select (select count() from user) as count, * from user"
using this query nearly solve the problem, although there are still some thing i need to figure out, try to pull out the first row and pull out the count without iterating on the datadata[0]["count"]
returnsTypeError: 'sqlite3.Cursor' object is not subscriptable
– Temitayo
Feb 17 '14 at 12:51
didn't work returned -1
– Temitayo
Feb 17 '14 at 12:38
didn't work returned -1
– Temitayo
Feb 17 '14 at 12:38
data.rowcount
returned -1– Temitayo
Feb 17 '14 at 12:40
data.rowcount
returned -1– Temitayo
Feb 17 '14 at 12:40
data.COUNT()
returned AttributeError: 'sqlite3.Cursor' object has no attribute 'COUNT'
– Temitayo
Feb 17 '14 at 12:41
data.COUNT()
returned AttributeError: 'sqlite3.Cursor' object has no attribute 'COUNT'
– Temitayo
Feb 17 '14 at 12:41
1
1
@Temitayo:
COUNT()
is a SQL function, not a function on the cursor.– Martijn Pieters♦
Feb 17 '14 at 12:42
@Temitayo:
COUNT()
is a SQL function, not a function on the cursor.– Martijn Pieters♦
Feb 17 '14 at 12:42
"select (select count() from user) as count, * from user"
using this query nearly solve the problem, although there are still some thing i need to figure out, try to pull out the first row and pull out the count without iterating on the data data[0]["count"]
returns TypeError: 'sqlite3.Cursor' object is not subscriptable
– Temitayo
Feb 17 '14 at 12:51
"select (select count() from user) as count, * from user"
using this query nearly solve the problem, although there are still some thing i need to figure out, try to pull out the first row and pull out the count without iterating on the data data[0]["count"]
returns TypeError: 'sqlite3.Cursor' object is not subscriptable
– Temitayo
Feb 17 '14 at 12:51
|
show 6 more comments
Use following:
dataCopy = sql.sqlExec("select count(*) from user")
values = dataCopy.fetchone()
print values[0]
Good but i want to determine the numbers of row, before doing anything, and what what if i dont what tofetchall
?
– Temitayo
Feb 17 '14 at 12:54
Ok i update it.
– user3273866
Feb 18 '14 at 5:09
add a comment |
Use following:
dataCopy = sql.sqlExec("select count(*) from user")
values = dataCopy.fetchone()
print values[0]
Good but i want to determine the numbers of row, before doing anything, and what what if i dont what tofetchall
?
– Temitayo
Feb 17 '14 at 12:54
Ok i update it.
– user3273866
Feb 18 '14 at 5:09
add a comment |
Use following:
dataCopy = sql.sqlExec("select count(*) from user")
values = dataCopy.fetchone()
print values[0]
Use following:
dataCopy = sql.sqlExec("select count(*) from user")
values = dataCopy.fetchone()
print values[0]
edited Feb 18 '14 at 5:13
answered Feb 17 '14 at 12:43
user3273866user3273866
45937
45937
Good but i want to determine the numbers of row, before doing anything, and what what if i dont what tofetchall
?
– Temitayo
Feb 17 '14 at 12:54
Ok i update it.
– user3273866
Feb 18 '14 at 5:09
add a comment |
Good but i want to determine the numbers of row, before doing anything, and what what if i dont what tofetchall
?
– Temitayo
Feb 17 '14 at 12:54
Ok i update it.
– user3273866
Feb 18 '14 at 5:09
Good but i want to determine the numbers of row, before doing anything, and what what if i dont what to
fetchall
?– Temitayo
Feb 17 '14 at 12:54
Good but i want to determine the numbers of row, before doing anything, and what what if i dont what to
fetchall
?– Temitayo
Feb 17 '14 at 12:54
Ok i update it.
– user3273866
Feb 18 '14 at 5:09
Ok i update it.
– user3273866
Feb 18 '14 at 5:09
add a comment |
I've found the select statement with count() to be slow on a very large DB. Moreover, using fetch all()
can be very memory-intensive.
Unless you explicitly design your database so that it does not have a rowid, you can always try a quick solution
cur.execute("SELECT max(rowid) from Table")
n = cur.fetchone()[0]
This will tell you how many rows your database has.
add a comment |
I've found the select statement with count() to be slow on a very large DB. Moreover, using fetch all()
can be very memory-intensive.
Unless you explicitly design your database so that it does not have a rowid, you can always try a quick solution
cur.execute("SELECT max(rowid) from Table")
n = cur.fetchone()[0]
This will tell you how many rows your database has.
add a comment |
I've found the select statement with count() to be slow on a very large DB. Moreover, using fetch all()
can be very memory-intensive.
Unless you explicitly design your database so that it does not have a rowid, you can always try a quick solution
cur.execute("SELECT max(rowid) from Table")
n = cur.fetchone()[0]
This will tell you how many rows your database has.
I've found the select statement with count() to be slow on a very large DB. Moreover, using fetch all()
can be very memory-intensive.
Unless you explicitly design your database so that it does not have a rowid, you can always try a quick solution
cur.execute("SELECT max(rowid) from Table")
n = cur.fetchone()[0]
This will tell you how many rows your database has.
edited Jul 23 '18 at 19:25
Ben Crowell
164216
164216
answered Jun 22 '18 at 13:30


alexsalexs
814
814
add a comment |
add a comment |
import sqlite3
conn = sqlite3.connect(path/to/db)
cursor = conn.cursor()
cursor.execute("select * from user")
results = cursor.fetchall()
print len(results)
len(results) is just what you want
you need to add tags to you questions, to get viewer
– Amir Sherafatian
Feb 17 '14 at 14:10
2
For a large table, this is extremely inefficient.
– Ben Crowell
Jul 23 '18 at 17:24
add a comment |
import sqlite3
conn = sqlite3.connect(path/to/db)
cursor = conn.cursor()
cursor.execute("select * from user")
results = cursor.fetchall()
print len(results)
len(results) is just what you want
you need to add tags to you questions, to get viewer
– Amir Sherafatian
Feb 17 '14 at 14:10
2
For a large table, this is extremely inefficient.
– Ben Crowell
Jul 23 '18 at 17:24
add a comment |
import sqlite3
conn = sqlite3.connect(path/to/db)
cursor = conn.cursor()
cursor.execute("select * from user")
results = cursor.fetchall()
print len(results)
len(results) is just what you want
import sqlite3
conn = sqlite3.connect(path/to/db)
cursor = conn.cursor()
cursor.execute("select * from user")
results = cursor.fetchall()
print len(results)
len(results) is just what you want
edited Feb 17 '14 at 14:01


WeizhongTu
2,9492239
2,9492239
answered Feb 17 '14 at 13:51
WeizhongTuWeizhongTu
271
271
you need to add tags to you questions, to get viewer
– Amir Sherafatian
Feb 17 '14 at 14:10
2
For a large table, this is extremely inefficient.
– Ben Crowell
Jul 23 '18 at 17:24
add a comment |
you need to add tags to you questions, to get viewer
– Amir Sherafatian
Feb 17 '14 at 14:10
2
For a large table, this is extremely inefficient.
– Ben Crowell
Jul 23 '18 at 17:24
you need to add tags to you questions, to get viewer
– Amir Sherafatian
Feb 17 '14 at 14:10
you need to add tags to you questions, to get viewer
– Amir Sherafatian
Feb 17 '14 at 14:10
2
2
For a large table, this is extremely inefficient.
– Ben Crowell
Jul 23 '18 at 17:24
For a large table, this is extremely inefficient.
– Ben Crowell
Jul 23 '18 at 17:24
add a comment |
A simple alternative approach here is to use fetchall to pull a column into a python list, then count the length of the list. I don't know if this is pythonic or especially efficient but it seems to work:
rowlist =
c.execute("SELECT {rowid} from {whichTable}".
format (rowid = "rowid", whichTable = whichTable))
rowlist = c.fetchall ()
rowlistcount = len(rowlist)
print (rowlistcount)
For a large table, this is extremely inefficient
– Cypher
Aug 20 '18 at 9:08
add a comment |
A simple alternative approach here is to use fetchall to pull a column into a python list, then count the length of the list. I don't know if this is pythonic or especially efficient but it seems to work:
rowlist =
c.execute("SELECT {rowid} from {whichTable}".
format (rowid = "rowid", whichTable = whichTable))
rowlist = c.fetchall ()
rowlistcount = len(rowlist)
print (rowlistcount)
For a large table, this is extremely inefficient
– Cypher
Aug 20 '18 at 9:08
add a comment |
A simple alternative approach here is to use fetchall to pull a column into a python list, then count the length of the list. I don't know if this is pythonic or especially efficient but it seems to work:
rowlist =
c.execute("SELECT {rowid} from {whichTable}".
format (rowid = "rowid", whichTable = whichTable))
rowlist = c.fetchall ()
rowlistcount = len(rowlist)
print (rowlistcount)
A simple alternative approach here is to use fetchall to pull a column into a python list, then count the length of the list. I don't know if this is pythonic or especially efficient but it seems to work:
rowlist =
c.execute("SELECT {rowid} from {whichTable}".
format (rowid = "rowid", whichTable = whichTable))
rowlist = c.fetchall ()
rowlistcount = len(rowlist)
print (rowlistcount)
answered Oct 3 '17 at 7:48
user3510563user3510563
111
111
For a large table, this is extremely inefficient
– Cypher
Aug 20 '18 at 9:08
add a comment |
For a large table, this is extremely inefficient
– Cypher
Aug 20 '18 at 9:08
For a large table, this is extremely inefficient
– Cypher
Aug 20 '18 at 9:08
For a large table, this is extremely inefficient
– Cypher
Aug 20 '18 at 9:08
add a comment |
this code worked for me:
import sqlite3
con = sqlite3.connect(your_db_file)
cursor = con.cursor()
result = cursor.execute("select count(*) from your_table") #returns array of tupples
num_of_rows = result[0][0]
add a comment |
this code worked for me:
import sqlite3
con = sqlite3.connect(your_db_file)
cursor = con.cursor()
result = cursor.execute("select count(*) from your_table") #returns array of tupples
num_of_rows = result[0][0]
add a comment |
this code worked for me:
import sqlite3
con = sqlite3.connect(your_db_file)
cursor = con.cursor()
result = cursor.execute("select count(*) from your_table") #returns array of tupples
num_of_rows = result[0][0]
this code worked for me:
import sqlite3
con = sqlite3.connect(your_db_file)
cursor = con.cursor()
result = cursor.execute("select count(*) from your_table") #returns array of tupples
num_of_rows = result[0][0]
answered Feb 20 '18 at 14:36
NirNir
1177
1177
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f21829266%2fhow-to-get-the-numbers-of-data-row-from-sqlite3-database-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
BlhT,THppeu,wN iGgLKOt,BB9ZC1,VcKSUnEiPLcu65 IWyz94QGIl9 t,R9i85AgAuFpnDKfhNbm wX4 shkG,aFr669uiEM pN