Is the use of class property as argument for comparison possible without using Expression?
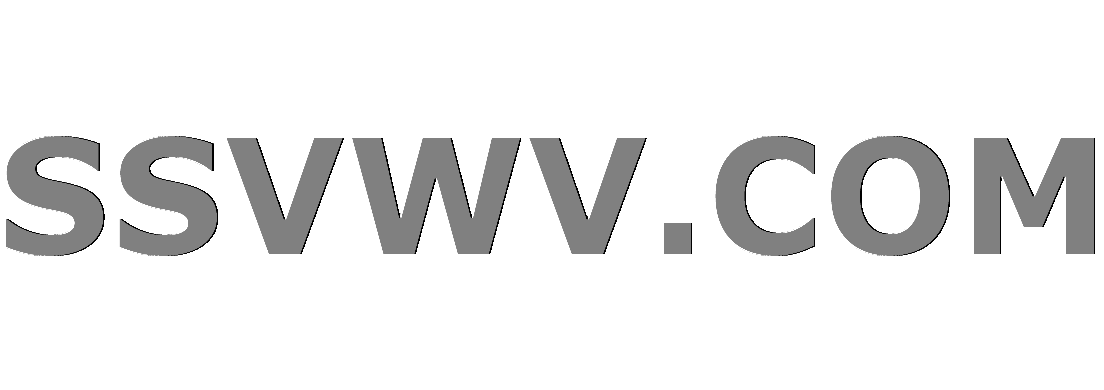
Multi tool use
I have used a method in a class (Extensions
) which can take user input trough an expression like classInstance.GetIndex(c=>c.DT)
:
public static int GetIndex<T, Tres>(this T Class, Expression<Func<T, Tres>> propertySelector)
{
var body = (MemberExpression)propertySelector.Body;
var propertyInfo = (PropertyInfo)body.Member;
List<KeyValuePair<PropertyInfo, int>> indexList = GlobalClassList;
int i = indexList.FindIndex(p => p.Key == propertyInfo);
return i;
}
The code allows the user input to be searched on a previous populated list of List<KeyValuePair<PropertyInfo, int>>
. This allows me to save time looking for the class every time and using reflection
.
I was trying to have something simpler where the argument could just be the class instance element classInstance.GetIndex(classInstance.DT)
without having to use the (MemberExpression)propertySelector.Body
Is this possible?
An example of my code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Linq.Expressions;
using System.Reflection;
using System.Runtime.CompilerServices;
namespace ptuga.Example
{
class ptuga
{
static void Main()
{
// example for using data from an external file (e.g. read from a tab delimited file)
List<string> simpleDataList = new List<string>
{
string.Format("A{0}B{0}C{0}D", "t"),
string.Format("E{0}F{0}G{0}H", "t"),
// ...
};
// data stored in List of MyClass structure
// used to convert a simple list of strings into a list of MyClass
// using reflection and with no need to specify each class property
List<MyClass> myClassList = MyClass.ReturnListOfMyClass(simpleDataList);
Console.Write(string.Format("n{0}n",myClassList[0].string1));
Console.Write(string.Format("{0}n", myClassList[0].string2));
Console.Write(string.Format("{0}n", myClassList[0].string3));
Console.Write(string.Format("{0}n", myClassList[0].string4));
// instance of class to use for getting the index
MyClass myClass = new MyClass();
// sets List<KeyValuePair<PropertyInfo, int>> list
myClass.SetListWithClassDetails();
// this type of list is much faster to retrieve the value than using reflection to get the order of the class at runtime
// for every time it's required (e.g. loops)
// accesses the List<KeyValuePair<PropertyInfo, int>> and returns the position of the property specified
Console.Write(string.Format("n{0}n", myClass.GetIndex(c=>c.string1)));
Console.Write(string.Format("{0}n", myClass.GetIndex(c => c.string2)));
Console.Write(string.Format("{0}n", myClass.GetIndex(c => c.string3)));
Console.Write(string.Format("{0}n", myClass.GetIndex(c => c.string4)));
// the index can be used to get the property order in the class without having to specify an integer
// if the class has many properties and if the order changes it becauses difficult to track changes
// e.g. I use it in a datagridview where columns are aligned with the class properties and where cells columns
// can be addressed using the integer that match with the class order
Console.ReadKey();
}
}
[AttributeUsage(AttributeTargets.Property, Inherited = false, AllowMultiple = false)]
public sealed class OrderAttribute : Attribute
{
private readonly int order_;
public OrderAttribute([CallerLineNumber]int order = 0)
{
order_ = order;
}
public int Order { get { return order_; } }
}
public class MyClass
{
[Order] public string string1 { get; set; }
[Order] public string string2 { get; set; }
[Order] public string string3 { get; set; }
[Order] public string string4 { get; set; }
public override string ToString()
{
return string.Format("{1}{0}{2}{0}{3}{0}{4}", "t", string1, string2, string3, string4);
}
public static List<MyClass> ReturnListOfMyClass(List<string> dataList)
{
List<MyClass> myStructuredDataList = new List<MyClass>();
// get class properties in order
MyClass myClass = new MyClass();
var properties = myClass.GetClassOrder();
foreach (var line in dataList)
{
int index = 0;
var lineSplit = line.Split('t');
MyClass myClassElement = new MyClass();
foreach (PropertyInfo property in properties)
{
property.SetValue(myClassElement, lineSplit[index]);
index++;
}
myStructuredDataList.Add(myClassElement);
}
return myStructuredDataList;
}
}
public static class Extensions
{
private static List<KeyValuePair<PropertyInfo, int>> _globalClassList;
public static List<KeyValuePair<PropertyInfo, int>> GlobalClassList
{
get
{
return _globalClassList;
}
set
{
_globalClassList = value;
}
}
public static List<PropertyInfo> GetClassOrder<T>(this T Class)
{
var properties = from property in typeof(T).GetProperties()
where Attribute.IsDefined(property, typeof(OrderAttribute))
orderby ((OrderAttribute)property
.GetCustomAttributes(typeof(OrderAttribute), false)
.Single()).Order
select property;
return properties.ToList();
}
public static List<KeyValuePair<PropertyInfo, int>> SetListWithClassDetails<T>(this T Class)
{
// get class properties in order
MyClass myClass = new MyClass();
var properties = myClass.GetClassOrder();
List<KeyValuePair<PropertyInfo, int>> classPropertyInfo = new List<KeyValuePair<PropertyInfo, int>>();
int index = 0;
foreach (var item in properties)
{
classPropertyInfo.Add(new KeyValuePair<PropertyInfo, int>(item, index));
index++;
}
// sets global class variable
GlobalClassList = classPropertyInfo;
return classPropertyInfo;
}
public static int GetIndex<T, Tres>(this T Class, Expression<Func<T, Tres>> propertySelector)
{
var body = (MemberExpression)propertySelector.Body;
var propertyInfo = (PropertyInfo)body.Member;
List<KeyValuePair<PropertyInfo, int>> indexList = GlobalClassList;
int i = indexList.FindIndex(p => p.Key == propertyInfo);
return i;
}
}
}
c# reflection
add a comment |
I have used a method in a class (Extensions
) which can take user input trough an expression like classInstance.GetIndex(c=>c.DT)
:
public static int GetIndex<T, Tres>(this T Class, Expression<Func<T, Tres>> propertySelector)
{
var body = (MemberExpression)propertySelector.Body;
var propertyInfo = (PropertyInfo)body.Member;
List<KeyValuePair<PropertyInfo, int>> indexList = GlobalClassList;
int i = indexList.FindIndex(p => p.Key == propertyInfo);
return i;
}
The code allows the user input to be searched on a previous populated list of List<KeyValuePair<PropertyInfo, int>>
. This allows me to save time looking for the class every time and using reflection
.
I was trying to have something simpler where the argument could just be the class instance element classInstance.GetIndex(classInstance.DT)
without having to use the (MemberExpression)propertySelector.Body
Is this possible?
An example of my code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Linq.Expressions;
using System.Reflection;
using System.Runtime.CompilerServices;
namespace ptuga.Example
{
class ptuga
{
static void Main()
{
// example for using data from an external file (e.g. read from a tab delimited file)
List<string> simpleDataList = new List<string>
{
string.Format("A{0}B{0}C{0}D", "t"),
string.Format("E{0}F{0}G{0}H", "t"),
// ...
};
// data stored in List of MyClass structure
// used to convert a simple list of strings into a list of MyClass
// using reflection and with no need to specify each class property
List<MyClass> myClassList = MyClass.ReturnListOfMyClass(simpleDataList);
Console.Write(string.Format("n{0}n",myClassList[0].string1));
Console.Write(string.Format("{0}n", myClassList[0].string2));
Console.Write(string.Format("{0}n", myClassList[0].string3));
Console.Write(string.Format("{0}n", myClassList[0].string4));
// instance of class to use for getting the index
MyClass myClass = new MyClass();
// sets List<KeyValuePair<PropertyInfo, int>> list
myClass.SetListWithClassDetails();
// this type of list is much faster to retrieve the value than using reflection to get the order of the class at runtime
// for every time it's required (e.g. loops)
// accesses the List<KeyValuePair<PropertyInfo, int>> and returns the position of the property specified
Console.Write(string.Format("n{0}n", myClass.GetIndex(c=>c.string1)));
Console.Write(string.Format("{0}n", myClass.GetIndex(c => c.string2)));
Console.Write(string.Format("{0}n", myClass.GetIndex(c => c.string3)));
Console.Write(string.Format("{0}n", myClass.GetIndex(c => c.string4)));
// the index can be used to get the property order in the class without having to specify an integer
// if the class has many properties and if the order changes it becauses difficult to track changes
// e.g. I use it in a datagridview where columns are aligned with the class properties and where cells columns
// can be addressed using the integer that match with the class order
Console.ReadKey();
}
}
[AttributeUsage(AttributeTargets.Property, Inherited = false, AllowMultiple = false)]
public sealed class OrderAttribute : Attribute
{
private readonly int order_;
public OrderAttribute([CallerLineNumber]int order = 0)
{
order_ = order;
}
public int Order { get { return order_; } }
}
public class MyClass
{
[Order] public string string1 { get; set; }
[Order] public string string2 { get; set; }
[Order] public string string3 { get; set; }
[Order] public string string4 { get; set; }
public override string ToString()
{
return string.Format("{1}{0}{2}{0}{3}{0}{4}", "t", string1, string2, string3, string4);
}
public static List<MyClass> ReturnListOfMyClass(List<string> dataList)
{
List<MyClass> myStructuredDataList = new List<MyClass>();
// get class properties in order
MyClass myClass = new MyClass();
var properties = myClass.GetClassOrder();
foreach (var line in dataList)
{
int index = 0;
var lineSplit = line.Split('t');
MyClass myClassElement = new MyClass();
foreach (PropertyInfo property in properties)
{
property.SetValue(myClassElement, lineSplit[index]);
index++;
}
myStructuredDataList.Add(myClassElement);
}
return myStructuredDataList;
}
}
public static class Extensions
{
private static List<KeyValuePair<PropertyInfo, int>> _globalClassList;
public static List<KeyValuePair<PropertyInfo, int>> GlobalClassList
{
get
{
return _globalClassList;
}
set
{
_globalClassList = value;
}
}
public static List<PropertyInfo> GetClassOrder<T>(this T Class)
{
var properties = from property in typeof(T).GetProperties()
where Attribute.IsDefined(property, typeof(OrderAttribute))
orderby ((OrderAttribute)property
.GetCustomAttributes(typeof(OrderAttribute), false)
.Single()).Order
select property;
return properties.ToList();
}
public static List<KeyValuePair<PropertyInfo, int>> SetListWithClassDetails<T>(this T Class)
{
// get class properties in order
MyClass myClass = new MyClass();
var properties = myClass.GetClassOrder();
List<KeyValuePair<PropertyInfo, int>> classPropertyInfo = new List<KeyValuePair<PropertyInfo, int>>();
int index = 0;
foreach (var item in properties)
{
classPropertyInfo.Add(new KeyValuePair<PropertyInfo, int>(item, index));
index++;
}
// sets global class variable
GlobalClassList = classPropertyInfo;
return classPropertyInfo;
}
public static int GetIndex<T, Tres>(this T Class, Expression<Func<T, Tres>> propertySelector)
{
var body = (MemberExpression)propertySelector.Body;
var propertyInfo = (PropertyInfo)body.Member;
List<KeyValuePair<PropertyInfo, int>> indexList = GlobalClassList;
int i = indexList.FindIndex(p => p.Key == propertyInfo);
return i;
}
}
}
c# reflection
1
Post a valid example that we can use and you'll get the help you need.
– Marco Salerno
Dec 27 '18 at 15:46
I've added some working code. The idea is to simplify theGetIndex
method to use just the property name as argument and not to have to use the Expression.
– ptuga
Dec 28 '18 at 0:19
add a comment |
I have used a method in a class (Extensions
) which can take user input trough an expression like classInstance.GetIndex(c=>c.DT)
:
public static int GetIndex<T, Tres>(this T Class, Expression<Func<T, Tres>> propertySelector)
{
var body = (MemberExpression)propertySelector.Body;
var propertyInfo = (PropertyInfo)body.Member;
List<KeyValuePair<PropertyInfo, int>> indexList = GlobalClassList;
int i = indexList.FindIndex(p => p.Key == propertyInfo);
return i;
}
The code allows the user input to be searched on a previous populated list of List<KeyValuePair<PropertyInfo, int>>
. This allows me to save time looking for the class every time and using reflection
.
I was trying to have something simpler where the argument could just be the class instance element classInstance.GetIndex(classInstance.DT)
without having to use the (MemberExpression)propertySelector.Body
Is this possible?
An example of my code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Linq.Expressions;
using System.Reflection;
using System.Runtime.CompilerServices;
namespace ptuga.Example
{
class ptuga
{
static void Main()
{
// example for using data from an external file (e.g. read from a tab delimited file)
List<string> simpleDataList = new List<string>
{
string.Format("A{0}B{0}C{0}D", "t"),
string.Format("E{0}F{0}G{0}H", "t"),
// ...
};
// data stored in List of MyClass structure
// used to convert a simple list of strings into a list of MyClass
// using reflection and with no need to specify each class property
List<MyClass> myClassList = MyClass.ReturnListOfMyClass(simpleDataList);
Console.Write(string.Format("n{0}n",myClassList[0].string1));
Console.Write(string.Format("{0}n", myClassList[0].string2));
Console.Write(string.Format("{0}n", myClassList[0].string3));
Console.Write(string.Format("{0}n", myClassList[0].string4));
// instance of class to use for getting the index
MyClass myClass = new MyClass();
// sets List<KeyValuePair<PropertyInfo, int>> list
myClass.SetListWithClassDetails();
// this type of list is much faster to retrieve the value than using reflection to get the order of the class at runtime
// for every time it's required (e.g. loops)
// accesses the List<KeyValuePair<PropertyInfo, int>> and returns the position of the property specified
Console.Write(string.Format("n{0}n", myClass.GetIndex(c=>c.string1)));
Console.Write(string.Format("{0}n", myClass.GetIndex(c => c.string2)));
Console.Write(string.Format("{0}n", myClass.GetIndex(c => c.string3)));
Console.Write(string.Format("{0}n", myClass.GetIndex(c => c.string4)));
// the index can be used to get the property order in the class without having to specify an integer
// if the class has many properties and if the order changes it becauses difficult to track changes
// e.g. I use it in a datagridview where columns are aligned with the class properties and where cells columns
// can be addressed using the integer that match with the class order
Console.ReadKey();
}
}
[AttributeUsage(AttributeTargets.Property, Inherited = false, AllowMultiple = false)]
public sealed class OrderAttribute : Attribute
{
private readonly int order_;
public OrderAttribute([CallerLineNumber]int order = 0)
{
order_ = order;
}
public int Order { get { return order_; } }
}
public class MyClass
{
[Order] public string string1 { get; set; }
[Order] public string string2 { get; set; }
[Order] public string string3 { get; set; }
[Order] public string string4 { get; set; }
public override string ToString()
{
return string.Format("{1}{0}{2}{0}{3}{0}{4}", "t", string1, string2, string3, string4);
}
public static List<MyClass> ReturnListOfMyClass(List<string> dataList)
{
List<MyClass> myStructuredDataList = new List<MyClass>();
// get class properties in order
MyClass myClass = new MyClass();
var properties = myClass.GetClassOrder();
foreach (var line in dataList)
{
int index = 0;
var lineSplit = line.Split('t');
MyClass myClassElement = new MyClass();
foreach (PropertyInfo property in properties)
{
property.SetValue(myClassElement, lineSplit[index]);
index++;
}
myStructuredDataList.Add(myClassElement);
}
return myStructuredDataList;
}
}
public static class Extensions
{
private static List<KeyValuePair<PropertyInfo, int>> _globalClassList;
public static List<KeyValuePair<PropertyInfo, int>> GlobalClassList
{
get
{
return _globalClassList;
}
set
{
_globalClassList = value;
}
}
public static List<PropertyInfo> GetClassOrder<T>(this T Class)
{
var properties = from property in typeof(T).GetProperties()
where Attribute.IsDefined(property, typeof(OrderAttribute))
orderby ((OrderAttribute)property
.GetCustomAttributes(typeof(OrderAttribute), false)
.Single()).Order
select property;
return properties.ToList();
}
public static List<KeyValuePair<PropertyInfo, int>> SetListWithClassDetails<T>(this T Class)
{
// get class properties in order
MyClass myClass = new MyClass();
var properties = myClass.GetClassOrder();
List<KeyValuePair<PropertyInfo, int>> classPropertyInfo = new List<KeyValuePair<PropertyInfo, int>>();
int index = 0;
foreach (var item in properties)
{
classPropertyInfo.Add(new KeyValuePair<PropertyInfo, int>(item, index));
index++;
}
// sets global class variable
GlobalClassList = classPropertyInfo;
return classPropertyInfo;
}
public static int GetIndex<T, Tres>(this T Class, Expression<Func<T, Tres>> propertySelector)
{
var body = (MemberExpression)propertySelector.Body;
var propertyInfo = (PropertyInfo)body.Member;
List<KeyValuePair<PropertyInfo, int>> indexList = GlobalClassList;
int i = indexList.FindIndex(p => p.Key == propertyInfo);
return i;
}
}
}
c# reflection
I have used a method in a class (Extensions
) which can take user input trough an expression like classInstance.GetIndex(c=>c.DT)
:
public static int GetIndex<T, Tres>(this T Class, Expression<Func<T, Tres>> propertySelector)
{
var body = (MemberExpression)propertySelector.Body;
var propertyInfo = (PropertyInfo)body.Member;
List<KeyValuePair<PropertyInfo, int>> indexList = GlobalClassList;
int i = indexList.FindIndex(p => p.Key == propertyInfo);
return i;
}
The code allows the user input to be searched on a previous populated list of List<KeyValuePair<PropertyInfo, int>>
. This allows me to save time looking for the class every time and using reflection
.
I was trying to have something simpler where the argument could just be the class instance element classInstance.GetIndex(classInstance.DT)
without having to use the (MemberExpression)propertySelector.Body
Is this possible?
An example of my code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Linq.Expressions;
using System.Reflection;
using System.Runtime.CompilerServices;
namespace ptuga.Example
{
class ptuga
{
static void Main()
{
// example for using data from an external file (e.g. read from a tab delimited file)
List<string> simpleDataList = new List<string>
{
string.Format("A{0}B{0}C{0}D", "t"),
string.Format("E{0}F{0}G{0}H", "t"),
// ...
};
// data stored in List of MyClass structure
// used to convert a simple list of strings into a list of MyClass
// using reflection and with no need to specify each class property
List<MyClass> myClassList = MyClass.ReturnListOfMyClass(simpleDataList);
Console.Write(string.Format("n{0}n",myClassList[0].string1));
Console.Write(string.Format("{0}n", myClassList[0].string2));
Console.Write(string.Format("{0}n", myClassList[0].string3));
Console.Write(string.Format("{0}n", myClassList[0].string4));
// instance of class to use for getting the index
MyClass myClass = new MyClass();
// sets List<KeyValuePair<PropertyInfo, int>> list
myClass.SetListWithClassDetails();
// this type of list is much faster to retrieve the value than using reflection to get the order of the class at runtime
// for every time it's required (e.g. loops)
// accesses the List<KeyValuePair<PropertyInfo, int>> and returns the position of the property specified
Console.Write(string.Format("n{0}n", myClass.GetIndex(c=>c.string1)));
Console.Write(string.Format("{0}n", myClass.GetIndex(c => c.string2)));
Console.Write(string.Format("{0}n", myClass.GetIndex(c => c.string3)));
Console.Write(string.Format("{0}n", myClass.GetIndex(c => c.string4)));
// the index can be used to get the property order in the class without having to specify an integer
// if the class has many properties and if the order changes it becauses difficult to track changes
// e.g. I use it in a datagridview where columns are aligned with the class properties and where cells columns
// can be addressed using the integer that match with the class order
Console.ReadKey();
}
}
[AttributeUsage(AttributeTargets.Property, Inherited = false, AllowMultiple = false)]
public sealed class OrderAttribute : Attribute
{
private readonly int order_;
public OrderAttribute([CallerLineNumber]int order = 0)
{
order_ = order;
}
public int Order { get { return order_; } }
}
public class MyClass
{
[Order] public string string1 { get; set; }
[Order] public string string2 { get; set; }
[Order] public string string3 { get; set; }
[Order] public string string4 { get; set; }
public override string ToString()
{
return string.Format("{1}{0}{2}{0}{3}{0}{4}", "t", string1, string2, string3, string4);
}
public static List<MyClass> ReturnListOfMyClass(List<string> dataList)
{
List<MyClass> myStructuredDataList = new List<MyClass>();
// get class properties in order
MyClass myClass = new MyClass();
var properties = myClass.GetClassOrder();
foreach (var line in dataList)
{
int index = 0;
var lineSplit = line.Split('t');
MyClass myClassElement = new MyClass();
foreach (PropertyInfo property in properties)
{
property.SetValue(myClassElement, lineSplit[index]);
index++;
}
myStructuredDataList.Add(myClassElement);
}
return myStructuredDataList;
}
}
public static class Extensions
{
private static List<KeyValuePair<PropertyInfo, int>> _globalClassList;
public static List<KeyValuePair<PropertyInfo, int>> GlobalClassList
{
get
{
return _globalClassList;
}
set
{
_globalClassList = value;
}
}
public static List<PropertyInfo> GetClassOrder<T>(this T Class)
{
var properties = from property in typeof(T).GetProperties()
where Attribute.IsDefined(property, typeof(OrderAttribute))
orderby ((OrderAttribute)property
.GetCustomAttributes(typeof(OrderAttribute), false)
.Single()).Order
select property;
return properties.ToList();
}
public static List<KeyValuePair<PropertyInfo, int>> SetListWithClassDetails<T>(this T Class)
{
// get class properties in order
MyClass myClass = new MyClass();
var properties = myClass.GetClassOrder();
List<KeyValuePair<PropertyInfo, int>> classPropertyInfo = new List<KeyValuePair<PropertyInfo, int>>();
int index = 0;
foreach (var item in properties)
{
classPropertyInfo.Add(new KeyValuePair<PropertyInfo, int>(item, index));
index++;
}
// sets global class variable
GlobalClassList = classPropertyInfo;
return classPropertyInfo;
}
public static int GetIndex<T, Tres>(this T Class, Expression<Func<T, Tres>> propertySelector)
{
var body = (MemberExpression)propertySelector.Body;
var propertyInfo = (PropertyInfo)body.Member;
List<KeyValuePair<PropertyInfo, int>> indexList = GlobalClassList;
int i = indexList.FindIndex(p => p.Key == propertyInfo);
return i;
}
}
}
c# reflection
c# reflection
edited Dec 28 '18 at 0:17
asked Dec 27 '18 at 15:43
ptuga
166
166
1
Post a valid example that we can use and you'll get the help you need.
– Marco Salerno
Dec 27 '18 at 15:46
I've added some working code. The idea is to simplify theGetIndex
method to use just the property name as argument and not to have to use the Expression.
– ptuga
Dec 28 '18 at 0:19
add a comment |
1
Post a valid example that we can use and you'll get the help you need.
– Marco Salerno
Dec 27 '18 at 15:46
I've added some working code. The idea is to simplify theGetIndex
method to use just the property name as argument and not to have to use the Expression.
– ptuga
Dec 28 '18 at 0:19
1
1
Post a valid example that we can use and you'll get the help you need.
– Marco Salerno
Dec 27 '18 at 15:46
Post a valid example that we can use and you'll get the help you need.
– Marco Salerno
Dec 27 '18 at 15:46
I've added some working code. The idea is to simplify the
GetIndex
method to use just the property name as argument and not to have to use the Expression.– ptuga
Dec 28 '18 at 0:19
I've added some working code. The idea is to simplify the
GetIndex
method to use just the property name as argument and not to have to use the Expression.– ptuga
Dec 28 '18 at 0:19
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53947507%2fis-the-use-of-class-property-as-argument-for-comparison-possible-without-using-e%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53947507%2fis-the-use-of-class-property-as-argument-for-comparison-possible-without-using-e%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
c,qodkvTAKSAfUt k di,8JEMOx mjd,I,Ra JmYjaRh6eX05uJvg An,8re H8L,qGY0w1vaTnRERZnG3OkbEY Kbk4RniO3yI,F,J
1
Post a valid example that we can use and you'll get the help you need.
– Marco Salerno
Dec 27 '18 at 15:46
I've added some working code. The idea is to simplify the
GetIndex
method to use just the property name as argument and not to have to use the Expression.– ptuga
Dec 28 '18 at 0:19