Having some trouble with javafx alert box interactions
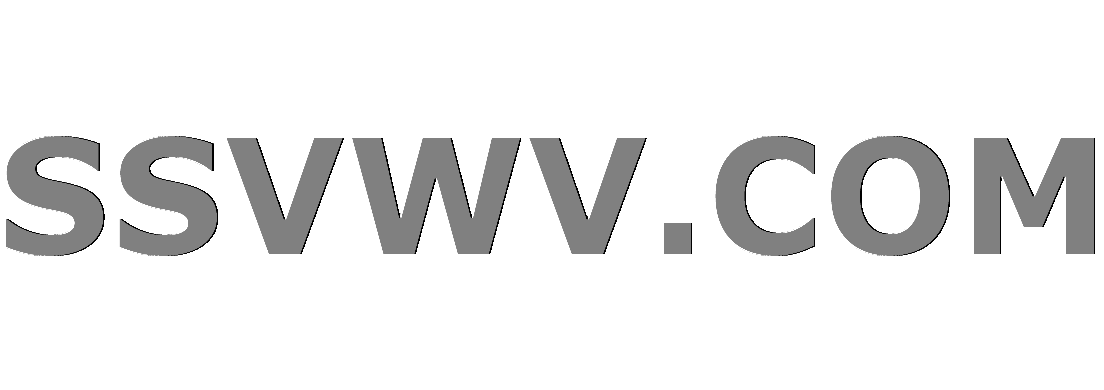
Multi tool use
I'm trying to learn javafx and I'm having some trouble with alert box interactions. My code is pretty straightforward:
public static void display(String title, String message) {
Stage window = new Stage();
window.initModality(Modality.APPLICATION_MODAL);
window.setTitle(title);
window.setMinWidth(250);
Label label = new Label();
label.setText(message);
Button closeButton = new Button("Close the window");
closeButton.setOnAction(e -> window.close());
VBox layout = new VBox(10);
layout.getChildren().addAll(label, closeButton);
layout.setAlignment(Pos.CENTER);
Scene scene = new Scene(layout);
window.setScene(scene);
window.showAndWait();
}
The problem is I can still somewhat interact with my initial window that I used to open the alert box, mainly clicking on the initial window brings it in front of the alert box. To my understanding, this shouldn't happen. Here is a gif demonstrating the issue: https://gyazo.com/0c2b69ec39f849227560fbdf2099c07c
Here is my code that calls the alert box
public void start(Stage primaryStage) {
window = primaryStage;
window.setTitle("Alert Box test");
button = new Button("Click me");
button.setOnAction(e -> AlertBox.display("New Alert", "Don't forget to close this window!"));
StackPane layout = new StackPane();
layout.getChildren().add(button);
Scene scene = new Scene(layout, 300, 250);
window.setScene(scene);
window.show();
}
What am I doing wrong here or is that just the intended behavior?
java user-interface javafx
add a comment |
I'm trying to learn javafx and I'm having some trouble with alert box interactions. My code is pretty straightforward:
public static void display(String title, String message) {
Stage window = new Stage();
window.initModality(Modality.APPLICATION_MODAL);
window.setTitle(title);
window.setMinWidth(250);
Label label = new Label();
label.setText(message);
Button closeButton = new Button("Close the window");
closeButton.setOnAction(e -> window.close());
VBox layout = new VBox(10);
layout.getChildren().addAll(label, closeButton);
layout.setAlignment(Pos.CENTER);
Scene scene = new Scene(layout);
window.setScene(scene);
window.showAndWait();
}
The problem is I can still somewhat interact with my initial window that I used to open the alert box, mainly clicking on the initial window brings it in front of the alert box. To my understanding, this shouldn't happen. Here is a gif demonstrating the issue: https://gyazo.com/0c2b69ec39f849227560fbdf2099c07c
Here is my code that calls the alert box
public void start(Stage primaryStage) {
window = primaryStage;
window.setTitle("Alert Box test");
button = new Button("Click me");
button.setOnAction(e -> AlertBox.display("New Alert", "Don't forget to close this window!"));
StackPane layout = new StackPane();
layout.getChildren().add(button);
Scene scene = new Scene(layout, 300, 250);
window.setScene(scene);
window.show();
}
What am I doing wrong here or is that just the intended behavior?
java user-interface javafx
JavaFX actually includes anAlert
class that handles much of this for you. Check out this tutorial to learn more.
– Zephyr
Jan 3 at 2:11
The same behavior doesn't happen for me (Windows 10, OpenJFX 11.0.1).
– Slaw
Jan 3 at 2:16
Take a look at stackoverflow.com/questions/10486731/… -- does setting the owner withinitOwner
help?
– casablanca
Jan 3 at 3:49
What JavaFX version are you using?
– user1803551
Jan 3 at 9:54
add a comment |
I'm trying to learn javafx and I'm having some trouble with alert box interactions. My code is pretty straightforward:
public static void display(String title, String message) {
Stage window = new Stage();
window.initModality(Modality.APPLICATION_MODAL);
window.setTitle(title);
window.setMinWidth(250);
Label label = new Label();
label.setText(message);
Button closeButton = new Button("Close the window");
closeButton.setOnAction(e -> window.close());
VBox layout = new VBox(10);
layout.getChildren().addAll(label, closeButton);
layout.setAlignment(Pos.CENTER);
Scene scene = new Scene(layout);
window.setScene(scene);
window.showAndWait();
}
The problem is I can still somewhat interact with my initial window that I used to open the alert box, mainly clicking on the initial window brings it in front of the alert box. To my understanding, this shouldn't happen. Here is a gif demonstrating the issue: https://gyazo.com/0c2b69ec39f849227560fbdf2099c07c
Here is my code that calls the alert box
public void start(Stage primaryStage) {
window = primaryStage;
window.setTitle("Alert Box test");
button = new Button("Click me");
button.setOnAction(e -> AlertBox.display("New Alert", "Don't forget to close this window!"));
StackPane layout = new StackPane();
layout.getChildren().add(button);
Scene scene = new Scene(layout, 300, 250);
window.setScene(scene);
window.show();
}
What am I doing wrong here or is that just the intended behavior?
java user-interface javafx
I'm trying to learn javafx and I'm having some trouble with alert box interactions. My code is pretty straightforward:
public static void display(String title, String message) {
Stage window = new Stage();
window.initModality(Modality.APPLICATION_MODAL);
window.setTitle(title);
window.setMinWidth(250);
Label label = new Label();
label.setText(message);
Button closeButton = new Button("Close the window");
closeButton.setOnAction(e -> window.close());
VBox layout = new VBox(10);
layout.getChildren().addAll(label, closeButton);
layout.setAlignment(Pos.CENTER);
Scene scene = new Scene(layout);
window.setScene(scene);
window.showAndWait();
}
The problem is I can still somewhat interact with my initial window that I used to open the alert box, mainly clicking on the initial window brings it in front of the alert box. To my understanding, this shouldn't happen. Here is a gif demonstrating the issue: https://gyazo.com/0c2b69ec39f849227560fbdf2099c07c
Here is my code that calls the alert box
public void start(Stage primaryStage) {
window = primaryStage;
window.setTitle("Alert Box test");
button = new Button("Click me");
button.setOnAction(e -> AlertBox.display("New Alert", "Don't forget to close this window!"));
StackPane layout = new StackPane();
layout.getChildren().add(button);
Scene scene = new Scene(layout, 300, 250);
window.setScene(scene);
window.show();
}
What am I doing wrong here or is that just the intended behavior?
java user-interface javafx
java user-interface javafx
asked Jan 3 at 2:08
gusterguster
111
111
JavaFX actually includes anAlert
class that handles much of this for you. Check out this tutorial to learn more.
– Zephyr
Jan 3 at 2:11
The same behavior doesn't happen for me (Windows 10, OpenJFX 11.0.1).
– Slaw
Jan 3 at 2:16
Take a look at stackoverflow.com/questions/10486731/… -- does setting the owner withinitOwner
help?
– casablanca
Jan 3 at 3:49
What JavaFX version are you using?
– user1803551
Jan 3 at 9:54
add a comment |
JavaFX actually includes anAlert
class that handles much of this for you. Check out this tutorial to learn more.
– Zephyr
Jan 3 at 2:11
The same behavior doesn't happen for me (Windows 10, OpenJFX 11.0.1).
– Slaw
Jan 3 at 2:16
Take a look at stackoverflow.com/questions/10486731/… -- does setting the owner withinitOwner
help?
– casablanca
Jan 3 at 3:49
What JavaFX version are you using?
– user1803551
Jan 3 at 9:54
JavaFX actually includes an
Alert
class that handles much of this for you. Check out this tutorial to learn more.– Zephyr
Jan 3 at 2:11
JavaFX actually includes an
Alert
class that handles much of this for you. Check out this tutorial to learn more.– Zephyr
Jan 3 at 2:11
The same behavior doesn't happen for me (Windows 10, OpenJFX 11.0.1).
– Slaw
Jan 3 at 2:16
The same behavior doesn't happen for me (Windows 10, OpenJFX 11.0.1).
– Slaw
Jan 3 at 2:16
Take a look at stackoverflow.com/questions/10486731/… -- does setting the owner with
initOwner
help?– casablanca
Jan 3 at 3:49
Take a look at stackoverflow.com/questions/10486731/… -- does setting the owner with
initOwner
help?– casablanca
Jan 3 at 3:49
What JavaFX version are you using?
– user1803551
Jan 3 at 9:54
What JavaFX version are you using?
– user1803551
Jan 3 at 9:54
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54015486%2fhaving-some-trouble-with-javafx-alert-box-interactions%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54015486%2fhaving-some-trouble-with-javafx-alert-box-interactions%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0v31,y 0m7aKaI8Mqn
JavaFX actually includes an
Alert
class that handles much of this for you. Check out this tutorial to learn more.– Zephyr
Jan 3 at 2:11
The same behavior doesn't happen for me (Windows 10, OpenJFX 11.0.1).
– Slaw
Jan 3 at 2:16
Take a look at stackoverflow.com/questions/10486731/… -- does setting the owner with
initOwner
help?– casablanca
Jan 3 at 3:49
What JavaFX version are you using?
– user1803551
Jan 3 at 9:54