Enable GLSL 3.3 on Mac
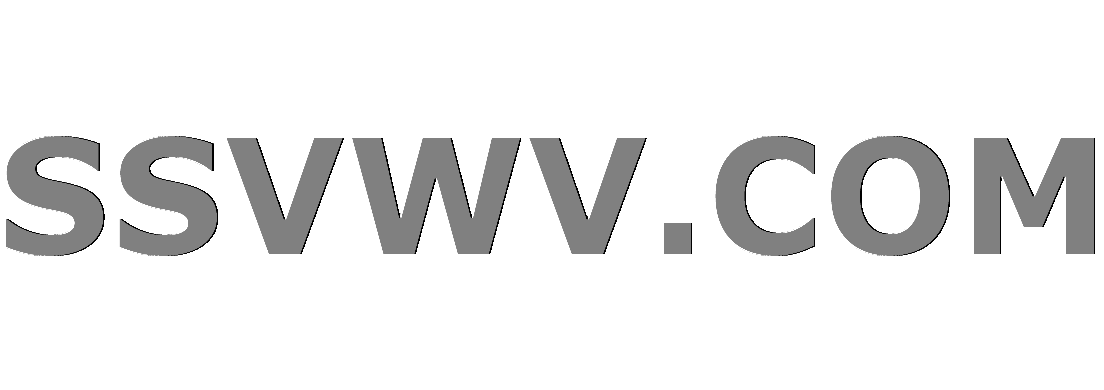
Multi tool use
I tried to use GLSL 3.3 but the system indicates the supported version is 1.2.
I tried to use SDL_GL_SetAttribute
to set major and minor version but glGetString(GL_SHADING_LANGUAGE_VERSION)
was still 1.2.
I'm not sure what's wrong with my code. Any help would be appreciated!
#include <iostream>
#include <GL/glew.h>
#include <GLFW/glfw3.h>
#include <SDL2/SDL.h>
unsigned int CompileShader(unsigned int type, const std::string& source)
{
unsigned int id = glCreateShader(type);
const char* src = source.c_str();
glShaderSource(id, 1, &src, nullptr);
glCompileShader(id);
int result;
glGetShaderiv(id, GL_COMPILE_STATUS, &result);
if (result != GL_TRUE)
{
int length;
glGetShaderiv(id, GL_INFO_LOG_LENGTH, &length);
char* message = (char*)alloca(length * sizeof(char));
glGetShaderInfoLog(id, length, &length, message);
std::cout << "Fail to compile " << (type == GL_VERTEX_SHADER ? "vertex" : "fragment") <<
" shader!" << std::endl;
printf("Supported GLSL version is %s.n", (char *)glGetString(GL_SHADING_LANGUAGE_VERSION));
std::cout << message << std::endl;
return 0;
}
return id;
}
static unsigned int CreateShader(const std::string& vertexShader, const std::string& fragmentShader)
{...}
int main(void)
{
SDL_Init(SDL_INIT_EVERYTHING);
SDL_GL_SetAttribute(SDL_GL_CONTEXT_MAJOR_VERSION, 3);
SDL_GL_SetAttribute(SDL_GL_CONTEXT_MINOR_VERSION, 1);
SDL_GL_SetAttribute(SDL_GL_CONTEXT_PROFILE_MASK, SDL_GL_CONTEXT_PROFILE_CORE);
// init window, context
// balabala
std::string vertexShader =
"#version 330 coren"
"n"
"layout (location = 0) in vec4 position;n"
"n"
"void main()n"
"{n"
" gl_position = position;n"
"}n";
std::string fragmentShader =
"#version 330 coren"
"n"
"layout (location = 0) out vec4 color;n"
"n"
"void main()n"
"{n"
" color = vec4(1.0,0.0,0.0,1.0);n"
"}n";
unsigned int shader = CreateShader(vertexShader, fragmentShader);
glUseProgram(shader);
// Loop until the user closes the window
// balabala
SDL_Quit();
return 0;
}
And below is the outputted error message:
@"Fail to compile vertex shader!rn"
@"Supported GLSL version is 1.20.rn"
@"ERROR: 0:1: '' : version '330' is not supportedrn"
@"ERROR: 0:1: '' : syntax error: #versionrn"
@"ERROR: 0:3: 'layout' : syntax error: syntax errorrn"
@"rn"
@"Fail to compile fragment shader!rn"
@"Supported GLSL version is 1.20.rn"
@"ERROR: 0:1: '' : version '330' is not supportedrn"
@"ERROR: 0:1: '' : syntax error: #versionrn"
@"ERROR: 0:3: 'layout' : syntax error: syntax errorrn"
@"rn"
Thanks!
c++ opengl glfw glew
add a comment |
I tried to use GLSL 3.3 but the system indicates the supported version is 1.2.
I tried to use SDL_GL_SetAttribute
to set major and minor version but glGetString(GL_SHADING_LANGUAGE_VERSION)
was still 1.2.
I'm not sure what's wrong with my code. Any help would be appreciated!
#include <iostream>
#include <GL/glew.h>
#include <GLFW/glfw3.h>
#include <SDL2/SDL.h>
unsigned int CompileShader(unsigned int type, const std::string& source)
{
unsigned int id = glCreateShader(type);
const char* src = source.c_str();
glShaderSource(id, 1, &src, nullptr);
glCompileShader(id);
int result;
glGetShaderiv(id, GL_COMPILE_STATUS, &result);
if (result != GL_TRUE)
{
int length;
glGetShaderiv(id, GL_INFO_LOG_LENGTH, &length);
char* message = (char*)alloca(length * sizeof(char));
glGetShaderInfoLog(id, length, &length, message);
std::cout << "Fail to compile " << (type == GL_VERTEX_SHADER ? "vertex" : "fragment") <<
" shader!" << std::endl;
printf("Supported GLSL version is %s.n", (char *)glGetString(GL_SHADING_LANGUAGE_VERSION));
std::cout << message << std::endl;
return 0;
}
return id;
}
static unsigned int CreateShader(const std::string& vertexShader, const std::string& fragmentShader)
{...}
int main(void)
{
SDL_Init(SDL_INIT_EVERYTHING);
SDL_GL_SetAttribute(SDL_GL_CONTEXT_MAJOR_VERSION, 3);
SDL_GL_SetAttribute(SDL_GL_CONTEXT_MINOR_VERSION, 1);
SDL_GL_SetAttribute(SDL_GL_CONTEXT_PROFILE_MASK, SDL_GL_CONTEXT_PROFILE_CORE);
// init window, context
// balabala
std::string vertexShader =
"#version 330 coren"
"n"
"layout (location = 0) in vec4 position;n"
"n"
"void main()n"
"{n"
" gl_position = position;n"
"}n";
std::string fragmentShader =
"#version 330 coren"
"n"
"layout (location = 0) out vec4 color;n"
"n"
"void main()n"
"{n"
" color = vec4(1.0,0.0,0.0,1.0);n"
"}n";
unsigned int shader = CreateShader(vertexShader, fragmentShader);
glUseProgram(shader);
// Loop until the user closes the window
// balabala
SDL_Quit();
return 0;
}
And below is the outputted error message:
@"Fail to compile vertex shader!rn"
@"Supported GLSL version is 1.20.rn"
@"ERROR: 0:1: '' : version '330' is not supportedrn"
@"ERROR: 0:1: '' : syntax error: #versionrn"
@"ERROR: 0:3: 'layout' : syntax error: syntax errorrn"
@"rn"
@"Fail to compile fragment shader!rn"
@"Supported GLSL version is 1.20.rn"
@"ERROR: 0:1: '' : version '330' is not supportedrn"
@"ERROR: 0:1: '' : syntax error: #versionrn"
@"ERROR: 0:3: 'layout' : syntax error: syntax errorrn"
@"rn"
Thanks!
c++ opengl glfw glew
I can’t speak for SDL, but with OpenGL you have to create a dummy context to get the OpenGL version so that you can create an appropriately versioned context. Usually you set attributes before initializing a context with a library. You’d have to look at the documentation, but you may have to move theSDL_GL_SetAttribute
calls to above theSDL_Init
call.
– van dench
Jan 3 at 2:33
4
There is no 3.1 core profile. Profiles were introduced in 3.2. When you want to use#version 330 core
, then you'll have to set minor and major version to 3 anyway for a 3.3 core profile.
– BDL
Jan 3 at 9:04
add a comment |
I tried to use GLSL 3.3 but the system indicates the supported version is 1.2.
I tried to use SDL_GL_SetAttribute
to set major and minor version but glGetString(GL_SHADING_LANGUAGE_VERSION)
was still 1.2.
I'm not sure what's wrong with my code. Any help would be appreciated!
#include <iostream>
#include <GL/glew.h>
#include <GLFW/glfw3.h>
#include <SDL2/SDL.h>
unsigned int CompileShader(unsigned int type, const std::string& source)
{
unsigned int id = glCreateShader(type);
const char* src = source.c_str();
glShaderSource(id, 1, &src, nullptr);
glCompileShader(id);
int result;
glGetShaderiv(id, GL_COMPILE_STATUS, &result);
if (result != GL_TRUE)
{
int length;
glGetShaderiv(id, GL_INFO_LOG_LENGTH, &length);
char* message = (char*)alloca(length * sizeof(char));
glGetShaderInfoLog(id, length, &length, message);
std::cout << "Fail to compile " << (type == GL_VERTEX_SHADER ? "vertex" : "fragment") <<
" shader!" << std::endl;
printf("Supported GLSL version is %s.n", (char *)glGetString(GL_SHADING_LANGUAGE_VERSION));
std::cout << message << std::endl;
return 0;
}
return id;
}
static unsigned int CreateShader(const std::string& vertexShader, const std::string& fragmentShader)
{...}
int main(void)
{
SDL_Init(SDL_INIT_EVERYTHING);
SDL_GL_SetAttribute(SDL_GL_CONTEXT_MAJOR_VERSION, 3);
SDL_GL_SetAttribute(SDL_GL_CONTEXT_MINOR_VERSION, 1);
SDL_GL_SetAttribute(SDL_GL_CONTEXT_PROFILE_MASK, SDL_GL_CONTEXT_PROFILE_CORE);
// init window, context
// balabala
std::string vertexShader =
"#version 330 coren"
"n"
"layout (location = 0) in vec4 position;n"
"n"
"void main()n"
"{n"
" gl_position = position;n"
"}n";
std::string fragmentShader =
"#version 330 coren"
"n"
"layout (location = 0) out vec4 color;n"
"n"
"void main()n"
"{n"
" color = vec4(1.0,0.0,0.0,1.0);n"
"}n";
unsigned int shader = CreateShader(vertexShader, fragmentShader);
glUseProgram(shader);
// Loop until the user closes the window
// balabala
SDL_Quit();
return 0;
}
And below is the outputted error message:
@"Fail to compile vertex shader!rn"
@"Supported GLSL version is 1.20.rn"
@"ERROR: 0:1: '' : version '330' is not supportedrn"
@"ERROR: 0:1: '' : syntax error: #versionrn"
@"ERROR: 0:3: 'layout' : syntax error: syntax errorrn"
@"rn"
@"Fail to compile fragment shader!rn"
@"Supported GLSL version is 1.20.rn"
@"ERROR: 0:1: '' : version '330' is not supportedrn"
@"ERROR: 0:1: '' : syntax error: #versionrn"
@"ERROR: 0:3: 'layout' : syntax error: syntax errorrn"
@"rn"
Thanks!
c++ opengl glfw glew
I tried to use GLSL 3.3 but the system indicates the supported version is 1.2.
I tried to use SDL_GL_SetAttribute
to set major and minor version but glGetString(GL_SHADING_LANGUAGE_VERSION)
was still 1.2.
I'm not sure what's wrong with my code. Any help would be appreciated!
#include <iostream>
#include <GL/glew.h>
#include <GLFW/glfw3.h>
#include <SDL2/SDL.h>
unsigned int CompileShader(unsigned int type, const std::string& source)
{
unsigned int id = glCreateShader(type);
const char* src = source.c_str();
glShaderSource(id, 1, &src, nullptr);
glCompileShader(id);
int result;
glGetShaderiv(id, GL_COMPILE_STATUS, &result);
if (result != GL_TRUE)
{
int length;
glGetShaderiv(id, GL_INFO_LOG_LENGTH, &length);
char* message = (char*)alloca(length * sizeof(char));
glGetShaderInfoLog(id, length, &length, message);
std::cout << "Fail to compile " << (type == GL_VERTEX_SHADER ? "vertex" : "fragment") <<
" shader!" << std::endl;
printf("Supported GLSL version is %s.n", (char *)glGetString(GL_SHADING_LANGUAGE_VERSION));
std::cout << message << std::endl;
return 0;
}
return id;
}
static unsigned int CreateShader(const std::string& vertexShader, const std::string& fragmentShader)
{...}
int main(void)
{
SDL_Init(SDL_INIT_EVERYTHING);
SDL_GL_SetAttribute(SDL_GL_CONTEXT_MAJOR_VERSION, 3);
SDL_GL_SetAttribute(SDL_GL_CONTEXT_MINOR_VERSION, 1);
SDL_GL_SetAttribute(SDL_GL_CONTEXT_PROFILE_MASK, SDL_GL_CONTEXT_PROFILE_CORE);
// init window, context
// balabala
std::string vertexShader =
"#version 330 coren"
"n"
"layout (location = 0) in vec4 position;n"
"n"
"void main()n"
"{n"
" gl_position = position;n"
"}n";
std::string fragmentShader =
"#version 330 coren"
"n"
"layout (location = 0) out vec4 color;n"
"n"
"void main()n"
"{n"
" color = vec4(1.0,0.0,0.0,1.0);n"
"}n";
unsigned int shader = CreateShader(vertexShader, fragmentShader);
glUseProgram(shader);
// Loop until the user closes the window
// balabala
SDL_Quit();
return 0;
}
And below is the outputted error message:
@"Fail to compile vertex shader!rn"
@"Supported GLSL version is 1.20.rn"
@"ERROR: 0:1: '' : version '330' is not supportedrn"
@"ERROR: 0:1: '' : syntax error: #versionrn"
@"ERROR: 0:3: 'layout' : syntax error: syntax errorrn"
@"rn"
@"Fail to compile fragment shader!rn"
@"Supported GLSL version is 1.20.rn"
@"ERROR: 0:1: '' : version '330' is not supportedrn"
@"ERROR: 0:1: '' : syntax error: #versionrn"
@"ERROR: 0:3: 'layout' : syntax error: syntax errorrn"
@"rn"
Thanks!
c++ opengl glfw glew
c++ opengl glfw glew
asked Jan 3 at 2:10
LHY_iS_LearningLHY_iS_Learning
43
43
I can’t speak for SDL, but with OpenGL you have to create a dummy context to get the OpenGL version so that you can create an appropriately versioned context. Usually you set attributes before initializing a context with a library. You’d have to look at the documentation, but you may have to move theSDL_GL_SetAttribute
calls to above theSDL_Init
call.
– van dench
Jan 3 at 2:33
4
There is no 3.1 core profile. Profiles were introduced in 3.2. When you want to use#version 330 core
, then you'll have to set minor and major version to 3 anyway for a 3.3 core profile.
– BDL
Jan 3 at 9:04
add a comment |
I can’t speak for SDL, but with OpenGL you have to create a dummy context to get the OpenGL version so that you can create an appropriately versioned context. Usually you set attributes before initializing a context with a library. You’d have to look at the documentation, but you may have to move theSDL_GL_SetAttribute
calls to above theSDL_Init
call.
– van dench
Jan 3 at 2:33
4
There is no 3.1 core profile. Profiles were introduced in 3.2. When you want to use#version 330 core
, then you'll have to set minor and major version to 3 anyway for a 3.3 core profile.
– BDL
Jan 3 at 9:04
I can’t speak for SDL, but with OpenGL you have to create a dummy context to get the OpenGL version so that you can create an appropriately versioned context. Usually you set attributes before initializing a context with a library. You’d have to look at the documentation, but you may have to move the
SDL_GL_SetAttribute
calls to above the SDL_Init
call.– van dench
Jan 3 at 2:33
I can’t speak for SDL, but with OpenGL you have to create a dummy context to get the OpenGL version so that you can create an appropriately versioned context. Usually you set attributes before initializing a context with a library. You’d have to look at the documentation, but you may have to move the
SDL_GL_SetAttribute
calls to above the SDL_Init
call.– van dench
Jan 3 at 2:33
4
4
There is no 3.1 core profile. Profiles were introduced in 3.2. When you want to use
#version 330 core
, then you'll have to set minor and major version to 3 anyway for a 3.3 core profile.– BDL
Jan 3 at 9:04
There is no 3.1 core profile. Profiles were introduced in 3.2. When you want to use
#version 330 core
, then you'll have to set minor and major version to 3 anyway for a 3.3 core profile.– BDL
Jan 3 at 9:04
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54015492%2fenable-glsl-3-3-on-mac%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54015492%2fenable-glsl-3-3-on-mac%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JBy,6uTZT5ImK8g3Io czL0VGiZebCAw56OXR 8
I can’t speak for SDL, but with OpenGL you have to create a dummy context to get the OpenGL version so that you can create an appropriately versioned context. Usually you set attributes before initializing a context with a library. You’d have to look at the documentation, but you may have to move the
SDL_GL_SetAttribute
calls to above theSDL_Init
call.– van dench
Jan 3 at 2:33
4
There is no 3.1 core profile. Profiles were introduced in 3.2. When you want to use
#version 330 core
, then you'll have to set minor and major version to 3 anyway for a 3.3 core profile.– BDL
Jan 3 at 9:04