Why is my call to “owner” in a “has_many” relationship not working?
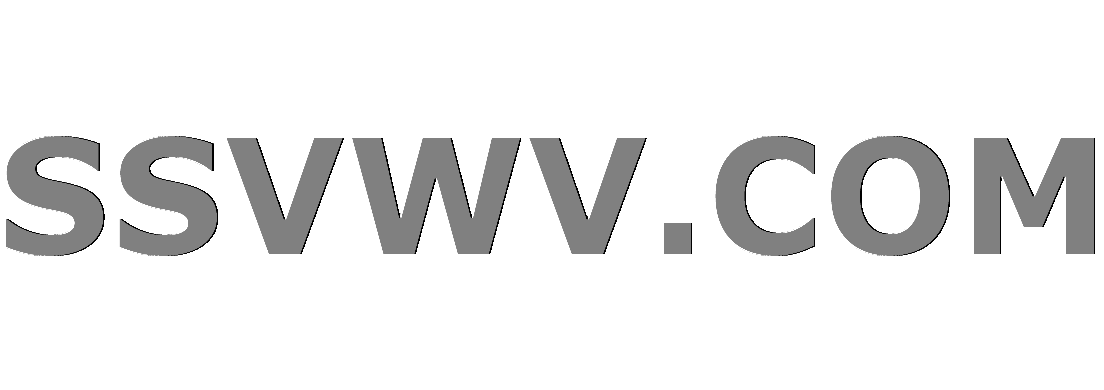
Multi tool use
I'm using Rails 5.0.7.1, and I see in the docs that my CollectionProxy instance should have access to an "@owner" instance variable:
Association proxies in Active Record are middlemen between the object
that holds the association, known as the @owner, and the actual
associated object, known as the @target. The kind of association any
proxy is about is available in @reflection. That's an instance of the
class ActiveRecord::Reflection::AssociationReflection.
the association proxy in blog.posts has the object in blog as @owner,
the collection of its posts as @target, and the @reflection object
represents a :has_many macro.
This class delegates unknown methods to @target via method_missing.
In my Rails app, I've got the following (rather unrealistic) test code:
class Post < ApplicationRecord
has_many :comments do
def number_five
if owner.is_a? Post
Comment.where(id: 5, post_id: self.id)
end
end
end
end
class Comment < ApplicationRecord
belongs_to :post
end
When I call Post.last.commments.number_five
, I get the following error:
NameError (undefined local variable or method `owner' for #
<Comment::ActiveRecord_Associations_CollectionProxy:0x00007fcbb9106120>)
When I add byebug
to the line in between def number_five
and owner.is_a? Post
, and I check the value of self
, I see it's ActiveRecord::Associations::CollectionProxy
, so I think I'm calling owner
in a scope where it should be defined.
I've tried Post.last.comments.instance_variables
, and I don't see :@owner
, only the following:
[:@association, :@klass, :@table, :@values, :@offsets,
:@loaded, :@predicate_builder, :@scope]
I've also tried the following:
comments = Post.last.comments
def comments.get_owner
self.owner
end
This returns the same NameError
as above.
For what it's worth, when I run Post.last.comments.class
, I see it's Comment::ActiveRecord_Associations_CollectionProxy
.
Given how the docs read, I'd expect to be able to call either Post.last.comments.owner
or @owner
from within Post.last.comments
(both of which I've tried), and have it return the value of Post.last
. Is my expectation incorrect, or is my code wrong, or is it something else entirely?
ruby-on-rails activerecord rails-activerecord
add a comment |
I'm using Rails 5.0.7.1, and I see in the docs that my CollectionProxy instance should have access to an "@owner" instance variable:
Association proxies in Active Record are middlemen between the object
that holds the association, known as the @owner, and the actual
associated object, known as the @target. The kind of association any
proxy is about is available in @reflection. That's an instance of the
class ActiveRecord::Reflection::AssociationReflection.
the association proxy in blog.posts has the object in blog as @owner,
the collection of its posts as @target, and the @reflection object
represents a :has_many macro.
This class delegates unknown methods to @target via method_missing.
In my Rails app, I've got the following (rather unrealistic) test code:
class Post < ApplicationRecord
has_many :comments do
def number_five
if owner.is_a? Post
Comment.where(id: 5, post_id: self.id)
end
end
end
end
class Comment < ApplicationRecord
belongs_to :post
end
When I call Post.last.commments.number_five
, I get the following error:
NameError (undefined local variable or method `owner' for #
<Comment::ActiveRecord_Associations_CollectionProxy:0x00007fcbb9106120>)
When I add byebug
to the line in between def number_five
and owner.is_a? Post
, and I check the value of self
, I see it's ActiveRecord::Associations::CollectionProxy
, so I think I'm calling owner
in a scope where it should be defined.
I've tried Post.last.comments.instance_variables
, and I don't see :@owner
, only the following:
[:@association, :@klass, :@table, :@values, :@offsets,
:@loaded, :@predicate_builder, :@scope]
I've also tried the following:
comments = Post.last.comments
def comments.get_owner
self.owner
end
This returns the same NameError
as above.
For what it's worth, when I run Post.last.comments.class
, I see it's Comment::ActiveRecord_Associations_CollectionProxy
.
Given how the docs read, I'd expect to be able to call either Post.last.comments.owner
or @owner
from within Post.last.comments
(both of which I've tried), and have it return the value of Post.last
. Is my expectation incorrect, or is my code wrong, or is it something else entirely?
ruby-on-rails activerecord rails-activerecord
add a comment |
I'm using Rails 5.0.7.1, and I see in the docs that my CollectionProxy instance should have access to an "@owner" instance variable:
Association proxies in Active Record are middlemen between the object
that holds the association, known as the @owner, and the actual
associated object, known as the @target. The kind of association any
proxy is about is available in @reflection. That's an instance of the
class ActiveRecord::Reflection::AssociationReflection.
the association proxy in blog.posts has the object in blog as @owner,
the collection of its posts as @target, and the @reflection object
represents a :has_many macro.
This class delegates unknown methods to @target via method_missing.
In my Rails app, I've got the following (rather unrealistic) test code:
class Post < ApplicationRecord
has_many :comments do
def number_five
if owner.is_a? Post
Comment.where(id: 5, post_id: self.id)
end
end
end
end
class Comment < ApplicationRecord
belongs_to :post
end
When I call Post.last.commments.number_five
, I get the following error:
NameError (undefined local variable or method `owner' for #
<Comment::ActiveRecord_Associations_CollectionProxy:0x00007fcbb9106120>)
When I add byebug
to the line in between def number_five
and owner.is_a? Post
, and I check the value of self
, I see it's ActiveRecord::Associations::CollectionProxy
, so I think I'm calling owner
in a scope where it should be defined.
I've tried Post.last.comments.instance_variables
, and I don't see :@owner
, only the following:
[:@association, :@klass, :@table, :@values, :@offsets,
:@loaded, :@predicate_builder, :@scope]
I've also tried the following:
comments = Post.last.comments
def comments.get_owner
self.owner
end
This returns the same NameError
as above.
For what it's worth, when I run Post.last.comments.class
, I see it's Comment::ActiveRecord_Associations_CollectionProxy
.
Given how the docs read, I'd expect to be able to call either Post.last.comments.owner
or @owner
from within Post.last.comments
(both of which I've tried), and have it return the value of Post.last
. Is my expectation incorrect, or is my code wrong, or is it something else entirely?
ruby-on-rails activerecord rails-activerecord
I'm using Rails 5.0.7.1, and I see in the docs that my CollectionProxy instance should have access to an "@owner" instance variable:
Association proxies in Active Record are middlemen between the object
that holds the association, known as the @owner, and the actual
associated object, known as the @target. The kind of association any
proxy is about is available in @reflection. That's an instance of the
class ActiveRecord::Reflection::AssociationReflection.
the association proxy in blog.posts has the object in blog as @owner,
the collection of its posts as @target, and the @reflection object
represents a :has_many macro.
This class delegates unknown methods to @target via method_missing.
In my Rails app, I've got the following (rather unrealistic) test code:
class Post < ApplicationRecord
has_many :comments do
def number_five
if owner.is_a? Post
Comment.where(id: 5, post_id: self.id)
end
end
end
end
class Comment < ApplicationRecord
belongs_to :post
end
When I call Post.last.commments.number_five
, I get the following error:
NameError (undefined local variable or method `owner' for #
<Comment::ActiveRecord_Associations_CollectionProxy:0x00007fcbb9106120>)
When I add byebug
to the line in between def number_five
and owner.is_a? Post
, and I check the value of self
, I see it's ActiveRecord::Associations::CollectionProxy
, so I think I'm calling owner
in a scope where it should be defined.
I've tried Post.last.comments.instance_variables
, and I don't see :@owner
, only the following:
[:@association, :@klass, :@table, :@values, :@offsets,
:@loaded, :@predicate_builder, :@scope]
I've also tried the following:
comments = Post.last.comments
def comments.get_owner
self.owner
end
This returns the same NameError
as above.
For what it's worth, when I run Post.last.comments.class
, I see it's Comment::ActiveRecord_Associations_CollectionProxy
.
Given how the docs read, I'd expect to be able to call either Post.last.comments.owner
or @owner
from within Post.last.comments
(both of which I've tried), and have it return the value of Post.last
. Is my expectation incorrect, or is my code wrong, or is it something else entirely?
ruby-on-rails activerecord rails-activerecord
ruby-on-rails activerecord rails-activerecord
edited Dec 30 '18 at 4:02
Richie Thomas
asked Dec 30 '18 at 0:53


Richie ThomasRichie Thomas
1,22422037
1,22422037
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
The documentation is a little confusing. I remember having to spend a few hours guessing, reading the Rails source, and experimenting to figure this out the first time I need to get outside the association from an extension method.
owner
is what you're after but that's a method on the association and you get the association via proxy_association
(which is just an accessor method for @association
):
has_many :comments do
def number_five
if proxy_association.owner.is_a? Post
#...
end
end
end
I'm not sure if this is the "right" or "official" way to do this but this is what I've been doing since Rails 4.
1
Confirmed the above code works. If there's a more canonical, official way to do this then I'm all ears, otherwise I'm accepting this as an answer. In the meantime, I may attempt a PR on the Rails docs specifying thatowner
is an attr on@association
, not onCollectionProxy
. Then the core team could either confirm this is the "best" way or suggest a better way.
– Richie Thomas
Dec 30 '18 at 18:57
1
I'd be interested in the result of such a PR.
– mu is too short
Dec 30 '18 at 20:32
Issue report here- github.com/rails/rails/issues/34829. Any feedback on the wording or clarity of the issue would be appreciated. Will follow up with a PR if the core team agrees with the change.
– Richie Thomas
Dec 31 '18 at 0:05
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53974500%2fwhy-is-my-call-to-owner-in-a-has-many-relationship-not-working%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The documentation is a little confusing. I remember having to spend a few hours guessing, reading the Rails source, and experimenting to figure this out the first time I need to get outside the association from an extension method.
owner
is what you're after but that's a method on the association and you get the association via proxy_association
(which is just an accessor method for @association
):
has_many :comments do
def number_five
if proxy_association.owner.is_a? Post
#...
end
end
end
I'm not sure if this is the "right" or "official" way to do this but this is what I've been doing since Rails 4.
1
Confirmed the above code works. If there's a more canonical, official way to do this then I'm all ears, otherwise I'm accepting this as an answer. In the meantime, I may attempt a PR on the Rails docs specifying thatowner
is an attr on@association
, not onCollectionProxy
. Then the core team could either confirm this is the "best" way or suggest a better way.
– Richie Thomas
Dec 30 '18 at 18:57
1
I'd be interested in the result of such a PR.
– mu is too short
Dec 30 '18 at 20:32
Issue report here- github.com/rails/rails/issues/34829. Any feedback on the wording or clarity of the issue would be appreciated. Will follow up with a PR if the core team agrees with the change.
– Richie Thomas
Dec 31 '18 at 0:05
add a comment |
The documentation is a little confusing. I remember having to spend a few hours guessing, reading the Rails source, and experimenting to figure this out the first time I need to get outside the association from an extension method.
owner
is what you're after but that's a method on the association and you get the association via proxy_association
(which is just an accessor method for @association
):
has_many :comments do
def number_five
if proxy_association.owner.is_a? Post
#...
end
end
end
I'm not sure if this is the "right" or "official" way to do this but this is what I've been doing since Rails 4.
1
Confirmed the above code works. If there's a more canonical, official way to do this then I'm all ears, otherwise I'm accepting this as an answer. In the meantime, I may attempt a PR on the Rails docs specifying thatowner
is an attr on@association
, not onCollectionProxy
. Then the core team could either confirm this is the "best" way or suggest a better way.
– Richie Thomas
Dec 30 '18 at 18:57
1
I'd be interested in the result of such a PR.
– mu is too short
Dec 30 '18 at 20:32
Issue report here- github.com/rails/rails/issues/34829. Any feedback on the wording or clarity of the issue would be appreciated. Will follow up with a PR if the core team agrees with the change.
– Richie Thomas
Dec 31 '18 at 0:05
add a comment |
The documentation is a little confusing. I remember having to spend a few hours guessing, reading the Rails source, and experimenting to figure this out the first time I need to get outside the association from an extension method.
owner
is what you're after but that's a method on the association and you get the association via proxy_association
(which is just an accessor method for @association
):
has_many :comments do
def number_five
if proxy_association.owner.is_a? Post
#...
end
end
end
I'm not sure if this is the "right" or "official" way to do this but this is what I've been doing since Rails 4.
The documentation is a little confusing. I remember having to spend a few hours guessing, reading the Rails source, and experimenting to figure this out the first time I need to get outside the association from an extension method.
owner
is what you're after but that's a method on the association and you get the association via proxy_association
(which is just an accessor method for @association
):
has_many :comments do
def number_five
if proxy_association.owner.is_a? Post
#...
end
end
end
I'm not sure if this is the "right" or "official" way to do this but this is what I've been doing since Rails 4.
answered Dec 30 '18 at 2:28


mu is too shortmu is too short
350k58688667
350k58688667
1
Confirmed the above code works. If there's a more canonical, official way to do this then I'm all ears, otherwise I'm accepting this as an answer. In the meantime, I may attempt a PR on the Rails docs specifying thatowner
is an attr on@association
, not onCollectionProxy
. Then the core team could either confirm this is the "best" way or suggest a better way.
– Richie Thomas
Dec 30 '18 at 18:57
1
I'd be interested in the result of such a PR.
– mu is too short
Dec 30 '18 at 20:32
Issue report here- github.com/rails/rails/issues/34829. Any feedback on the wording or clarity of the issue would be appreciated. Will follow up with a PR if the core team agrees with the change.
– Richie Thomas
Dec 31 '18 at 0:05
add a comment |
1
Confirmed the above code works. If there's a more canonical, official way to do this then I'm all ears, otherwise I'm accepting this as an answer. In the meantime, I may attempt a PR on the Rails docs specifying thatowner
is an attr on@association
, not onCollectionProxy
. Then the core team could either confirm this is the "best" way or suggest a better way.
– Richie Thomas
Dec 30 '18 at 18:57
1
I'd be interested in the result of such a PR.
– mu is too short
Dec 30 '18 at 20:32
Issue report here- github.com/rails/rails/issues/34829. Any feedback on the wording or clarity of the issue would be appreciated. Will follow up with a PR if the core team agrees with the change.
– Richie Thomas
Dec 31 '18 at 0:05
1
1
Confirmed the above code works. If there's a more canonical, official way to do this then I'm all ears, otherwise I'm accepting this as an answer. In the meantime, I may attempt a PR on the Rails docs specifying that
owner
is an attr on @association
, not on CollectionProxy
. Then the core team could either confirm this is the "best" way or suggest a better way.– Richie Thomas
Dec 30 '18 at 18:57
Confirmed the above code works. If there's a more canonical, official way to do this then I'm all ears, otherwise I'm accepting this as an answer. In the meantime, I may attempt a PR on the Rails docs specifying that
owner
is an attr on @association
, not on CollectionProxy
. Then the core team could either confirm this is the "best" way or suggest a better way.– Richie Thomas
Dec 30 '18 at 18:57
1
1
I'd be interested in the result of such a PR.
– mu is too short
Dec 30 '18 at 20:32
I'd be interested in the result of such a PR.
– mu is too short
Dec 30 '18 at 20:32
Issue report here- github.com/rails/rails/issues/34829. Any feedback on the wording or clarity of the issue would be appreciated. Will follow up with a PR if the core team agrees with the change.
– Richie Thomas
Dec 31 '18 at 0:05
Issue report here- github.com/rails/rails/issues/34829. Any feedback on the wording or clarity of the issue would be appreciated. Will follow up with a PR if the core team agrees with the change.
– Richie Thomas
Dec 31 '18 at 0:05
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53974500%2fwhy-is-my-call-to-owner-in-a-has-many-relationship-not-working%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
X Slgg7vBg97Qb,Dkjexuj,0 f4sxQ9J6XcOpRXpfTIQ