React-Navigation: Cannot hide header with nested navigators
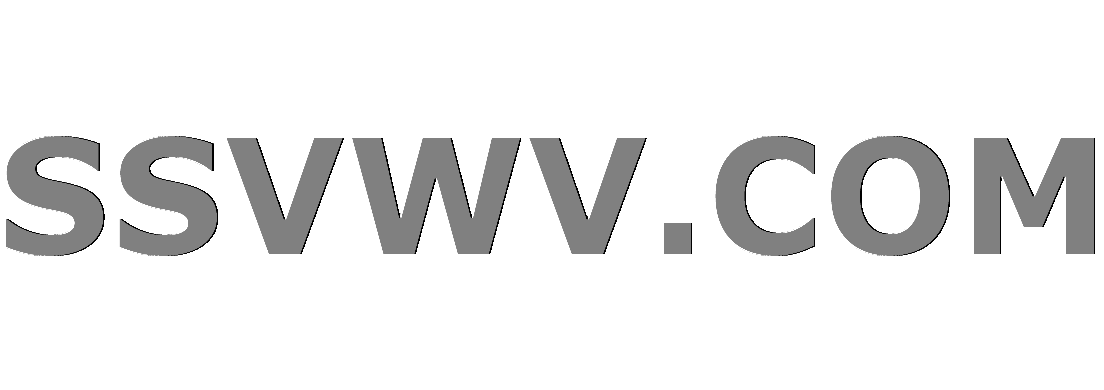
Multi tool use
I'm using the official react-navigation to handle my navigation. I have one main TabNavigator for the whole app with two tabs (called HitchhikingMapNavigator
and SettingsNavigator
below), and each tab has a nested StackNavigator:
const HitchhikingMapNavigator = StackNavigator({
hitchhikingMap: { screen: HitchhikingMapViewContainer },
spotDetails: { screen: SpotDetailsViewContainer }
}, {
navigationOptions: {
header: {
visible: false
}
}
});
const SettingsNavigator = StackNavigator({
// some other routes
});
export default AppNavigator = TabNavigator({
hitchhikingMap: { screen: HitchhikingMapNavigator },
settings: { screen: SettingsNavigator }
}, {
navigationOptions: {
header: {
visible: false,
},
},
});
As you can see, I put the headers' visilibility to false everywhere, even in my HitchhikingMapViewContainer
's view:
class HitchhikingMapView extends React.Component {
static navigationOptions = {
title: 'Map',
header: {
visible: false,
},
//...other options
}
And yet, the header bar is still visible:
If I don't nest the navigators (i.e. if I put this code, skipping the nested one):
export default AppNavigator = TabNavigator({
hitchhikingMap: { screen: HitchhikingMapViewContainer },
settings: { screen: SettingsNavigator }
});
then the header is correctly hidden.
So conclusion: I can't make a header not visible when I have two nested navigators. Any ideas?
javascript react-native react-navigation
add a comment |
I'm using the official react-navigation to handle my navigation. I have one main TabNavigator for the whole app with two tabs (called HitchhikingMapNavigator
and SettingsNavigator
below), and each tab has a nested StackNavigator:
const HitchhikingMapNavigator = StackNavigator({
hitchhikingMap: { screen: HitchhikingMapViewContainer },
spotDetails: { screen: SpotDetailsViewContainer }
}, {
navigationOptions: {
header: {
visible: false
}
}
});
const SettingsNavigator = StackNavigator({
// some other routes
});
export default AppNavigator = TabNavigator({
hitchhikingMap: { screen: HitchhikingMapNavigator },
settings: { screen: SettingsNavigator }
}, {
navigationOptions: {
header: {
visible: false,
},
},
});
As you can see, I put the headers' visilibility to false everywhere, even in my HitchhikingMapViewContainer
's view:
class HitchhikingMapView extends React.Component {
static navigationOptions = {
title: 'Map',
header: {
visible: false,
},
//...other options
}
And yet, the header bar is still visible:
If I don't nest the navigators (i.e. if I put this code, skipping the nested one):
export default AppNavigator = TabNavigator({
hitchhikingMap: { screen: HitchhikingMapViewContainer },
settings: { screen: SettingsNavigator }
});
then the header is correctly hidden.
So conclusion: I can't make a header not visible when I have two nested navigators. Any ideas?
javascript react-native react-navigation
add a comment |
I'm using the official react-navigation to handle my navigation. I have one main TabNavigator for the whole app with two tabs (called HitchhikingMapNavigator
and SettingsNavigator
below), and each tab has a nested StackNavigator:
const HitchhikingMapNavigator = StackNavigator({
hitchhikingMap: { screen: HitchhikingMapViewContainer },
spotDetails: { screen: SpotDetailsViewContainer }
}, {
navigationOptions: {
header: {
visible: false
}
}
});
const SettingsNavigator = StackNavigator({
// some other routes
});
export default AppNavigator = TabNavigator({
hitchhikingMap: { screen: HitchhikingMapNavigator },
settings: { screen: SettingsNavigator }
}, {
navigationOptions: {
header: {
visible: false,
},
},
});
As you can see, I put the headers' visilibility to false everywhere, even in my HitchhikingMapViewContainer
's view:
class HitchhikingMapView extends React.Component {
static navigationOptions = {
title: 'Map',
header: {
visible: false,
},
//...other options
}
And yet, the header bar is still visible:
If I don't nest the navigators (i.e. if I put this code, skipping the nested one):
export default AppNavigator = TabNavigator({
hitchhikingMap: { screen: HitchhikingMapViewContainer },
settings: { screen: SettingsNavigator }
});
then the header is correctly hidden.
So conclusion: I can't make a header not visible when I have two nested navigators. Any ideas?
javascript react-native react-navigation
I'm using the official react-navigation to handle my navigation. I have one main TabNavigator for the whole app with two tabs (called HitchhikingMapNavigator
and SettingsNavigator
below), and each tab has a nested StackNavigator:
const HitchhikingMapNavigator = StackNavigator({
hitchhikingMap: { screen: HitchhikingMapViewContainer },
spotDetails: { screen: SpotDetailsViewContainer }
}, {
navigationOptions: {
header: {
visible: false
}
}
});
const SettingsNavigator = StackNavigator({
// some other routes
});
export default AppNavigator = TabNavigator({
hitchhikingMap: { screen: HitchhikingMapNavigator },
settings: { screen: SettingsNavigator }
}, {
navigationOptions: {
header: {
visible: false,
},
},
});
As you can see, I put the headers' visilibility to false everywhere, even in my HitchhikingMapViewContainer
's view:
class HitchhikingMapView extends React.Component {
static navigationOptions = {
title: 'Map',
header: {
visible: false,
},
//...other options
}
And yet, the header bar is still visible:
If I don't nest the navigators (i.e. if I put this code, skipping the nested one):
export default AppNavigator = TabNavigator({
hitchhikingMap: { screen: HitchhikingMapViewContainer },
settings: { screen: SettingsNavigator }
});
then the header is correctly hidden.
So conclusion: I can't make a header not visible when I have two nested navigators. Any ideas?
javascript react-native react-navigation
javascript react-native react-navigation
edited Apr 4 '18 at 13:38
David Nathan
2,63132143
2,63132143
asked Feb 22 '17 at 17:01


amaurymartinyamaurymartiny
1,85811744
1,85811744
add a comment |
add a comment |
6 Answers
6
active
oldest
votes
For those who are still looking for the answer, I will post it here.
So two solutions:
1st solution: use headerMode: 'none'
in the StackNavigator. This will remove the header from ALL screens in the StackNavigator
2nd solution: use headerMode: 'screen'
in the StackNavigator and add header: { visible: false }
in the navigationOptions
of the screens where you want to hide the header.
More info can be found here: https://reactnavigation.org/docs/en/stack-navigator.html
5
As for React Navigation 1.0.0-beta.11 header : { visible : false} doesn't work. Use header : null instead.
– Andrei Beziazychnyi
May 26 '17 at 15:12
add a comment |
Starting from v1.0.0-beta.9
, use the following,
static navigationOptions = {
header: null
}
add a comment |
This worked for me:
headerMode: 'none'
1
doesn't work for me in"react-navigation": "^1.0.0-beta.7"
– Iliyass Hamza
Mar 15 '17 at 18:41
2
I have "react-navigation": "^1.0.0-beta.9" and the method -> static navigationOptions = { header: false } work for me.
– Daniel Arenas
May 9 '17 at 2:41
@DanielArenas You're my hero dude
– Guillaume Munsch
May 22 '17 at 15:51
add a comment |
This works for me to hide the navigation:
static navigationOptions = {
header: null
};
add a comment |
This Worked for me, i am working on android side in react native version 0.45
static navigationOptions = {
header: null
}
add a comment |
I have the same problem in "react-navigation": "^3.0.9" . What is the solution please ?
My code :
const MontanteStack = createStackNavigator(
{
Montante: {
screen: MontanteTab,
navigationOptions: ({navigation}) => ({
header: null,
}),
},
PronosticsDetails: {
screen: PronosticsDetailsScreen,
navigationOptions: ({navigation}) => ({
headerMode: 'screen',
headerTitle: 'Pronostics détails',
headerStyle: {
backgroundColor: '#000000',
textAlign: 'center',
},
headerTintColor: '#ffffff',
headerTitleStyle: {
color: '#c7943e',
textAlign: 'center',
alignSelf: 'center',
justifyContent: 'center',
flex: 1,
}
}),
},
},
{
initialRouteName: 'Montante',
}
);
export default createAppContainer(MontanteStack);
I would like hide the the topbar and the tabs because they are displayed above the title of PronosticsDetailsScreen
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f42397807%2freact-navigation-cannot-hide-header-with-nested-navigators%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
For those who are still looking for the answer, I will post it here.
So two solutions:
1st solution: use headerMode: 'none'
in the StackNavigator. This will remove the header from ALL screens in the StackNavigator
2nd solution: use headerMode: 'screen'
in the StackNavigator and add header: { visible: false }
in the navigationOptions
of the screens where you want to hide the header.
More info can be found here: https://reactnavigation.org/docs/en/stack-navigator.html
5
As for React Navigation 1.0.0-beta.11 header : { visible : false} doesn't work. Use header : null instead.
– Andrei Beziazychnyi
May 26 '17 at 15:12
add a comment |
For those who are still looking for the answer, I will post it here.
So two solutions:
1st solution: use headerMode: 'none'
in the StackNavigator. This will remove the header from ALL screens in the StackNavigator
2nd solution: use headerMode: 'screen'
in the StackNavigator and add header: { visible: false }
in the navigationOptions
of the screens where you want to hide the header.
More info can be found here: https://reactnavigation.org/docs/en/stack-navigator.html
5
As for React Navigation 1.0.0-beta.11 header : { visible : false} doesn't work. Use header : null instead.
– Andrei Beziazychnyi
May 26 '17 at 15:12
add a comment |
For those who are still looking for the answer, I will post it here.
So two solutions:
1st solution: use headerMode: 'none'
in the StackNavigator. This will remove the header from ALL screens in the StackNavigator
2nd solution: use headerMode: 'screen'
in the StackNavigator and add header: { visible: false }
in the navigationOptions
of the screens where you want to hide the header.
More info can be found here: https://reactnavigation.org/docs/en/stack-navigator.html
For those who are still looking for the answer, I will post it here.
So two solutions:
1st solution: use headerMode: 'none'
in the StackNavigator. This will remove the header from ALL screens in the StackNavigator
2nd solution: use headerMode: 'screen'
in the StackNavigator and add header: { visible: false }
in the navigationOptions
of the screens where you want to hide the header.
More info can be found here: https://reactnavigation.org/docs/en/stack-navigator.html
edited Sep 7 '18 at 15:01
Donut
76.4k14116135
76.4k14116135
answered Apr 5 '17 at 12:23


amaurymartinyamaurymartiny
1,85811744
1,85811744
5
As for React Navigation 1.0.0-beta.11 header : { visible : false} doesn't work. Use header : null instead.
– Andrei Beziazychnyi
May 26 '17 at 15:12
add a comment |
5
As for React Navigation 1.0.0-beta.11 header : { visible : false} doesn't work. Use header : null instead.
– Andrei Beziazychnyi
May 26 '17 at 15:12
5
5
As for React Navigation 1.0.0-beta.11 header : { visible : false} doesn't work. Use header : null instead.
– Andrei Beziazychnyi
May 26 '17 at 15:12
As for React Navigation 1.0.0-beta.11 header : { visible : false} doesn't work. Use header : null instead.
– Andrei Beziazychnyi
May 26 '17 at 15:12
add a comment |
Starting from v1.0.0-beta.9
, use the following,
static navigationOptions = {
header: null
}
add a comment |
Starting from v1.0.0-beta.9
, use the following,
static navigationOptions = {
header: null
}
add a comment |
Starting from v1.0.0-beta.9
, use the following,
static navigationOptions = {
header: null
}
Starting from v1.0.0-beta.9
, use the following,
static navigationOptions = {
header: null
}
edited Apr 4 '18 at 10:02
David Nathan
2,63132143
2,63132143
answered May 30 '17 at 2:50


h--nh--n
4,81542128
4,81542128
add a comment |
add a comment |
This worked for me:
headerMode: 'none'
1
doesn't work for me in"react-navigation": "^1.0.0-beta.7"
– Iliyass Hamza
Mar 15 '17 at 18:41
2
I have "react-navigation": "^1.0.0-beta.9" and the method -> static navigationOptions = { header: false } work for me.
– Daniel Arenas
May 9 '17 at 2:41
@DanielArenas You're my hero dude
– Guillaume Munsch
May 22 '17 at 15:51
add a comment |
This worked for me:
headerMode: 'none'
1
doesn't work for me in"react-navigation": "^1.0.0-beta.7"
– Iliyass Hamza
Mar 15 '17 at 18:41
2
I have "react-navigation": "^1.0.0-beta.9" and the method -> static navigationOptions = { header: false } work for me.
– Daniel Arenas
May 9 '17 at 2:41
@DanielArenas You're my hero dude
– Guillaume Munsch
May 22 '17 at 15:51
add a comment |
This worked for me:
headerMode: 'none'
This worked for me:
headerMode: 'none'
edited Apr 4 '18 at 9:10
David Nathan
2,63132143
2,63132143
answered Feb 24 '17 at 19:49
PeterPeter
292
292
1
doesn't work for me in"react-navigation": "^1.0.0-beta.7"
– Iliyass Hamza
Mar 15 '17 at 18:41
2
I have "react-navigation": "^1.0.0-beta.9" and the method -> static navigationOptions = { header: false } work for me.
– Daniel Arenas
May 9 '17 at 2:41
@DanielArenas You're my hero dude
– Guillaume Munsch
May 22 '17 at 15:51
add a comment |
1
doesn't work for me in"react-navigation": "^1.0.0-beta.7"
– Iliyass Hamza
Mar 15 '17 at 18:41
2
I have "react-navigation": "^1.0.0-beta.9" and the method -> static navigationOptions = { header: false } work for me.
– Daniel Arenas
May 9 '17 at 2:41
@DanielArenas You're my hero dude
– Guillaume Munsch
May 22 '17 at 15:51
1
1
doesn't work for me in
"react-navigation": "^1.0.0-beta.7"
– Iliyass Hamza
Mar 15 '17 at 18:41
doesn't work for me in
"react-navigation": "^1.0.0-beta.7"
– Iliyass Hamza
Mar 15 '17 at 18:41
2
2
I have "react-navigation": "^1.0.0-beta.9" and the method -> static navigationOptions = { header: false } work for me.
– Daniel Arenas
May 9 '17 at 2:41
I have "react-navigation": "^1.0.0-beta.9" and the method -> static navigationOptions = { header: false } work for me.
– Daniel Arenas
May 9 '17 at 2:41
@DanielArenas You're my hero dude
– Guillaume Munsch
May 22 '17 at 15:51
@DanielArenas You're my hero dude
– Guillaume Munsch
May 22 '17 at 15:51
add a comment |
This works for me to hide the navigation:
static navigationOptions = {
header: null
};
add a comment |
This works for me to hide the navigation:
static navigationOptions = {
header: null
};
add a comment |
This works for me to hide the navigation:
static navigationOptions = {
header: null
};
This works for me to hide the navigation:
static navigationOptions = {
header: null
};
edited Apr 4 '18 at 9:32
David Nathan
2,63132143
2,63132143
answered Aug 9 '17 at 8:03
ramkrishna samantaramkrishna samanta
1
1
add a comment |
add a comment |
This Worked for me, i am working on android side in react native version 0.45
static navigationOptions = {
header: null
}
add a comment |
This Worked for me, i am working on android side in react native version 0.45
static navigationOptions = {
header: null
}
add a comment |
This Worked for me, i am working on android side in react native version 0.45
static navigationOptions = {
header: null
}
This Worked for me, i am working on android side in react native version 0.45
static navigationOptions = {
header: null
}
edited Apr 4 '18 at 9:47
David Nathan
2,63132143
2,63132143
answered Jun 13 '17 at 20:53


Atif MukhtiarAtif Mukhtiar
1655
1655
add a comment |
add a comment |
I have the same problem in "react-navigation": "^3.0.9" . What is the solution please ?
My code :
const MontanteStack = createStackNavigator(
{
Montante: {
screen: MontanteTab,
navigationOptions: ({navigation}) => ({
header: null,
}),
},
PronosticsDetails: {
screen: PronosticsDetailsScreen,
navigationOptions: ({navigation}) => ({
headerMode: 'screen',
headerTitle: 'Pronostics détails',
headerStyle: {
backgroundColor: '#000000',
textAlign: 'center',
},
headerTintColor: '#ffffff',
headerTitleStyle: {
color: '#c7943e',
textAlign: 'center',
alignSelf: 'center',
justifyContent: 'center',
flex: 1,
}
}),
},
},
{
initialRouteName: 'Montante',
}
);
export default createAppContainer(MontanteStack);
I would like hide the the topbar and the tabs because they are displayed above the title of PronosticsDetailsScreen
add a comment |
I have the same problem in "react-navigation": "^3.0.9" . What is the solution please ?
My code :
const MontanteStack = createStackNavigator(
{
Montante: {
screen: MontanteTab,
navigationOptions: ({navigation}) => ({
header: null,
}),
},
PronosticsDetails: {
screen: PronosticsDetailsScreen,
navigationOptions: ({navigation}) => ({
headerMode: 'screen',
headerTitle: 'Pronostics détails',
headerStyle: {
backgroundColor: '#000000',
textAlign: 'center',
},
headerTintColor: '#ffffff',
headerTitleStyle: {
color: '#c7943e',
textAlign: 'center',
alignSelf: 'center',
justifyContent: 'center',
flex: 1,
}
}),
},
},
{
initialRouteName: 'Montante',
}
);
export default createAppContainer(MontanteStack);
I would like hide the the topbar and the tabs because they are displayed above the title of PronosticsDetailsScreen
add a comment |
I have the same problem in "react-navigation": "^3.0.9" . What is the solution please ?
My code :
const MontanteStack = createStackNavigator(
{
Montante: {
screen: MontanteTab,
navigationOptions: ({navigation}) => ({
header: null,
}),
},
PronosticsDetails: {
screen: PronosticsDetailsScreen,
navigationOptions: ({navigation}) => ({
headerMode: 'screen',
headerTitle: 'Pronostics détails',
headerStyle: {
backgroundColor: '#000000',
textAlign: 'center',
},
headerTintColor: '#ffffff',
headerTitleStyle: {
color: '#c7943e',
textAlign: 'center',
alignSelf: 'center',
justifyContent: 'center',
flex: 1,
}
}),
},
},
{
initialRouteName: 'Montante',
}
);
export default createAppContainer(MontanteStack);
I would like hide the the topbar and the tabs because they are displayed above the title of PronosticsDetailsScreen
I have the same problem in "react-navigation": "^3.0.9" . What is the solution please ?
My code :
const MontanteStack = createStackNavigator(
{
Montante: {
screen: MontanteTab,
navigationOptions: ({navigation}) => ({
header: null,
}),
},
PronosticsDetails: {
screen: PronosticsDetailsScreen,
navigationOptions: ({navigation}) => ({
headerMode: 'screen',
headerTitle: 'Pronostics détails',
headerStyle: {
backgroundColor: '#000000',
textAlign: 'center',
},
headerTintColor: '#ffffff',
headerTitleStyle: {
color: '#c7943e',
textAlign: 'center',
alignSelf: 'center',
justifyContent: 'center',
flex: 1,
}
}),
},
},
{
initialRouteName: 'Montante',
}
);
export default createAppContainer(MontanteStack);
I would like hide the the topbar and the tabs because they are displayed above the title of PronosticsDetailsScreen
answered Dec 30 '18 at 0:22
ElGecko_76ElGecko_76
1089
1089
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f42397807%2freact-navigation-cannot-hide-header-with-nested-navigators%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zzLE,MH9mNDucVrVrQDD mgCbnimWb7uWyFjD5ePpmVd