Changing the src of an image with javascript
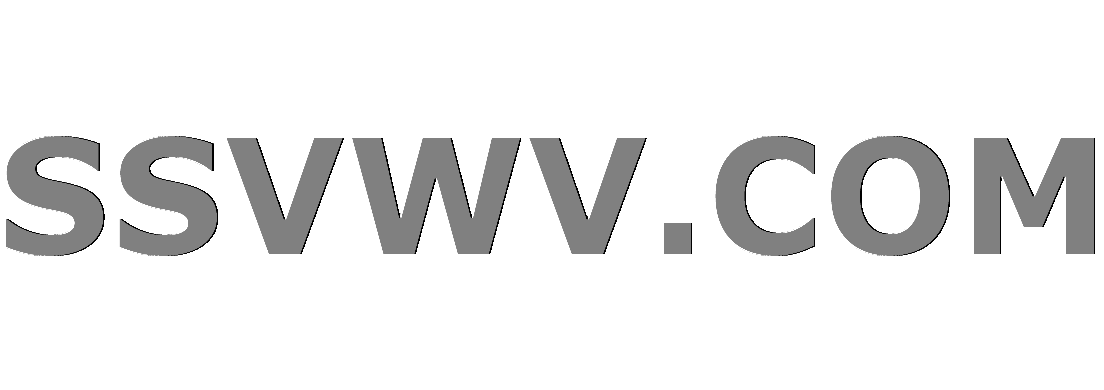
Multi tool use
I have been trying to use the following piece of code to change the src of an image
document.getElementById('test').src='images/test2.png';
My code looks like this. There's some CSS and other things in there but the important parts are the div and the img, and the function.
<div id='splashScreen' class='splash'>
<button class='bigButton' onclick='introSplash()'>Hi</button>
<img class='splashImages' src='images/splashScreen1.png'>
</div>
this is my image with a page-sized transparent button over the top of it
<script>
function introSplash() {
document.getElementById('splashImages').src='images/splashScreen2.png';
}
</script>
this function should be replacing the image under the button.
Pressing the button with this code returns the following error message:
Uncaught TypeError: Cannot set property 'src' of null
at introSplash
all of the images are locally saved and exist with those names
I'm not sure why this is occurring, and would appreciate any help.
javascript html css
add a comment |
I have been trying to use the following piece of code to change the src of an image
document.getElementById('test').src='images/test2.png';
My code looks like this. There's some CSS and other things in there but the important parts are the div and the img, and the function.
<div id='splashScreen' class='splash'>
<button class='bigButton' onclick='introSplash()'>Hi</button>
<img class='splashImages' src='images/splashScreen1.png'>
</div>
this is my image with a page-sized transparent button over the top of it
<script>
function introSplash() {
document.getElementById('splashImages').src='images/splashScreen2.png';
}
</script>
this function should be replacing the image under the button.
Pressing the button with this code returns the following error message:
Uncaught TypeError: Cannot set property 'src' of null
at introSplash
all of the images are locally saved and exist with those names
I'm not sure why this is occurring, and would appreciate any help.
javascript html css
I see you are new... I would strongly recommend you to use JQuery to do this kind of things... bye!
– Ari Waisberg
Dec 30 '18 at 1:03
add a comment |
I have been trying to use the following piece of code to change the src of an image
document.getElementById('test').src='images/test2.png';
My code looks like this. There's some CSS and other things in there but the important parts are the div and the img, and the function.
<div id='splashScreen' class='splash'>
<button class='bigButton' onclick='introSplash()'>Hi</button>
<img class='splashImages' src='images/splashScreen1.png'>
</div>
this is my image with a page-sized transparent button over the top of it
<script>
function introSplash() {
document.getElementById('splashImages').src='images/splashScreen2.png';
}
</script>
this function should be replacing the image under the button.
Pressing the button with this code returns the following error message:
Uncaught TypeError: Cannot set property 'src' of null
at introSplash
all of the images are locally saved and exist with those names
I'm not sure why this is occurring, and would appreciate any help.
javascript html css
I have been trying to use the following piece of code to change the src of an image
document.getElementById('test').src='images/test2.png';
My code looks like this. There's some CSS and other things in there but the important parts are the div and the img, and the function.
<div id='splashScreen' class='splash'>
<button class='bigButton' onclick='introSplash()'>Hi</button>
<img class='splashImages' src='images/splashScreen1.png'>
</div>
this is my image with a page-sized transparent button over the top of it
<script>
function introSplash() {
document.getElementById('splashImages').src='images/splashScreen2.png';
}
</script>
this function should be replacing the image under the button.
Pressing the button with this code returns the following error message:
Uncaught TypeError: Cannot set property 'src' of null
at introSplash
all of the images are locally saved and exist with those names
I'm not sure why this is occurring, and would appreciate any help.
javascript html css
javascript html css
edited Dec 30 '18 at 3:18
Jack Bashford
7,04531337
7,04531337
asked Dec 30 '18 at 0:52


FascismSaladFascismSalad
84
84
I see you are new... I would strongly recommend you to use JQuery to do this kind of things... bye!
– Ari Waisberg
Dec 30 '18 at 1:03
add a comment |
I see you are new... I would strongly recommend you to use JQuery to do this kind of things... bye!
– Ari Waisberg
Dec 30 '18 at 1:03
I see you are new... I would strongly recommend you to use JQuery to do this kind of things... bye!
– Ari Waisberg
Dec 30 '18 at 1:03
I see you are new... I would strongly recommend you to use JQuery to do this kind of things... bye!
– Ari Waisberg
Dec 30 '18 at 1:03
add a comment |
4 Answers
4
active
oldest
votes
You need to add the ID test
to the <img>
element:
<img id='test' class='splashImages' src='images/splashScreen1.png' />
Now your code will work.
The alternative, without adding any IDs, would be to use document.getElementsByClassName
like so:
document.getElementsByClassName('splashImages')[0].src='images/test2.png';
add a comment |
Please verify you are using document.getElementById('splashImages')
but the html element has not ID. Add the id attribute
<img id="splashImages" class='splashImages' src='images/splashScreen1.png'>
Please try this and let me know how it works :)
add a comment |
You try to get the element by id, but only a class="splashImages"
is given.
add a comment |
document.getElementById('splashImages')
returns null
because the element you want does not have the correct id. Use getElementsByClassName
instead, or give it the correct id.
So you should implement one of following changes:
<script>
function introSplash() {
document.getElementsByClassName('splashImages').src='images/splashScreen2.png';
}
</script>
or
<div id='splashScreen' class='splash'>
<button class='bigButton' onclick='introSplash()'>Hi</button>
<img id='splashImages' class='splashImages' src='images/splashScreen1.png'>
</div>
Oh my gosh I feel silly now. Of course it wouldn't work it was a class not an ID. So sorry for wasting all of your time lol.
– FascismSalad
Dec 30 '18 at 0:59
We've all been there :)
– Laurens Deprost
Dec 30 '18 at 1:01
Yes... Everyone ve all been there expending time un a simple comma. Semicolon or id. :)
– Manuel Eduardo Romero
Dec 30 '18 at 3:08
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53974498%2fchanging-the-src-of-an-image-with-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
You need to add the ID test
to the <img>
element:
<img id='test' class='splashImages' src='images/splashScreen1.png' />
Now your code will work.
The alternative, without adding any IDs, would be to use document.getElementsByClassName
like so:
document.getElementsByClassName('splashImages')[0].src='images/test2.png';
add a comment |
You need to add the ID test
to the <img>
element:
<img id='test' class='splashImages' src='images/splashScreen1.png' />
Now your code will work.
The alternative, without adding any IDs, would be to use document.getElementsByClassName
like so:
document.getElementsByClassName('splashImages')[0].src='images/test2.png';
add a comment |
You need to add the ID test
to the <img>
element:
<img id='test' class='splashImages' src='images/splashScreen1.png' />
Now your code will work.
The alternative, without adding any IDs, would be to use document.getElementsByClassName
like so:
document.getElementsByClassName('splashImages')[0].src='images/test2.png';
You need to add the ID test
to the <img>
element:
<img id='test' class='splashImages' src='images/splashScreen1.png' />
Now your code will work.
The alternative, without adding any IDs, would be to use document.getElementsByClassName
like so:
document.getElementsByClassName('splashImages')[0].src='images/test2.png';
answered Dec 30 '18 at 0:55
Jack BashfordJack Bashford
7,04531337
7,04531337
add a comment |
add a comment |
Please verify you are using document.getElementById('splashImages')
but the html element has not ID. Add the id attribute
<img id="splashImages" class='splashImages' src='images/splashScreen1.png'>
Please try this and let me know how it works :)
add a comment |
Please verify you are using document.getElementById('splashImages')
but the html element has not ID. Add the id attribute
<img id="splashImages" class='splashImages' src='images/splashScreen1.png'>
Please try this and let me know how it works :)
add a comment |
Please verify you are using document.getElementById('splashImages')
but the html element has not ID. Add the id attribute
<img id="splashImages" class='splashImages' src='images/splashScreen1.png'>
Please try this and let me know how it works :)
Please verify you are using document.getElementById('splashImages')
but the html element has not ID. Add the id attribute
<img id="splashImages" class='splashImages' src='images/splashScreen1.png'>
Please try this and let me know how it works :)
answered Dec 30 '18 at 0:57


Manuel Eduardo RomeroManuel Eduardo Romero
20016
20016
add a comment |
add a comment |
You try to get the element by id, but only a class="splashImages"
is given.
add a comment |
You try to get the element by id, but only a class="splashImages"
is given.
add a comment |
You try to get the element by id, but only a class="splashImages"
is given.
You try to get the element by id, but only a class="splashImages"
is given.
answered Dec 30 '18 at 0:54


TyrTyr
2,4811718
2,4811718
add a comment |
add a comment |
document.getElementById('splashImages')
returns null
because the element you want does not have the correct id. Use getElementsByClassName
instead, or give it the correct id.
So you should implement one of following changes:
<script>
function introSplash() {
document.getElementsByClassName('splashImages').src='images/splashScreen2.png';
}
</script>
or
<div id='splashScreen' class='splash'>
<button class='bigButton' onclick='introSplash()'>Hi</button>
<img id='splashImages' class='splashImages' src='images/splashScreen1.png'>
</div>
Oh my gosh I feel silly now. Of course it wouldn't work it was a class not an ID. So sorry for wasting all of your time lol.
– FascismSalad
Dec 30 '18 at 0:59
We've all been there :)
– Laurens Deprost
Dec 30 '18 at 1:01
Yes... Everyone ve all been there expending time un a simple comma. Semicolon or id. :)
– Manuel Eduardo Romero
Dec 30 '18 at 3:08
add a comment |
document.getElementById('splashImages')
returns null
because the element you want does not have the correct id. Use getElementsByClassName
instead, or give it the correct id.
So you should implement one of following changes:
<script>
function introSplash() {
document.getElementsByClassName('splashImages').src='images/splashScreen2.png';
}
</script>
or
<div id='splashScreen' class='splash'>
<button class='bigButton' onclick='introSplash()'>Hi</button>
<img id='splashImages' class='splashImages' src='images/splashScreen1.png'>
</div>
Oh my gosh I feel silly now. Of course it wouldn't work it was a class not an ID. So sorry for wasting all of your time lol.
– FascismSalad
Dec 30 '18 at 0:59
We've all been there :)
– Laurens Deprost
Dec 30 '18 at 1:01
Yes... Everyone ve all been there expending time un a simple comma. Semicolon or id. :)
– Manuel Eduardo Romero
Dec 30 '18 at 3:08
add a comment |
document.getElementById('splashImages')
returns null
because the element you want does not have the correct id. Use getElementsByClassName
instead, or give it the correct id.
So you should implement one of following changes:
<script>
function introSplash() {
document.getElementsByClassName('splashImages').src='images/splashScreen2.png';
}
</script>
or
<div id='splashScreen' class='splash'>
<button class='bigButton' onclick='introSplash()'>Hi</button>
<img id='splashImages' class='splashImages' src='images/splashScreen1.png'>
</div>
document.getElementById('splashImages')
returns null
because the element you want does not have the correct id. Use getElementsByClassName
instead, or give it the correct id.
So you should implement one of following changes:
<script>
function introSplash() {
document.getElementsByClassName('splashImages').src='images/splashScreen2.png';
}
</script>
or
<div id='splashScreen' class='splash'>
<button class='bigButton' onclick='introSplash()'>Hi</button>
<img id='splashImages' class='splashImages' src='images/splashScreen1.png'>
</div>
answered Dec 30 '18 at 0:55


Laurens DeprostLaurens Deprost
978114
978114
Oh my gosh I feel silly now. Of course it wouldn't work it was a class not an ID. So sorry for wasting all of your time lol.
– FascismSalad
Dec 30 '18 at 0:59
We've all been there :)
– Laurens Deprost
Dec 30 '18 at 1:01
Yes... Everyone ve all been there expending time un a simple comma. Semicolon or id. :)
– Manuel Eduardo Romero
Dec 30 '18 at 3:08
add a comment |
Oh my gosh I feel silly now. Of course it wouldn't work it was a class not an ID. So sorry for wasting all of your time lol.
– FascismSalad
Dec 30 '18 at 0:59
We've all been there :)
– Laurens Deprost
Dec 30 '18 at 1:01
Yes... Everyone ve all been there expending time un a simple comma. Semicolon or id. :)
– Manuel Eduardo Romero
Dec 30 '18 at 3:08
Oh my gosh I feel silly now. Of course it wouldn't work it was a class not an ID. So sorry for wasting all of your time lol.
– FascismSalad
Dec 30 '18 at 0:59
Oh my gosh I feel silly now. Of course it wouldn't work it was a class not an ID. So sorry for wasting all of your time lol.
– FascismSalad
Dec 30 '18 at 0:59
We've all been there :)
– Laurens Deprost
Dec 30 '18 at 1:01
We've all been there :)
– Laurens Deprost
Dec 30 '18 at 1:01
Yes... Everyone ve all been there expending time un a simple comma. Semicolon or id. :)
– Manuel Eduardo Romero
Dec 30 '18 at 3:08
Yes... Everyone ve all been there expending time un a simple comma. Semicolon or id. :)
– Manuel Eduardo Romero
Dec 30 '18 at 3:08
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53974498%2fchanging-the-src-of-an-image-with-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Mo,5bMD,AMET,f2OTV cTsaKI8SGX,J HQP 7,XsJiOICnRau mxvh3qS7H3Kq m,MY,H RoOaOxd2M OM8wS2SetQ0a2ZEBOfQ8S,CwxroNX37B
I see you are new... I would strongly recommend you to use JQuery to do this kind of things... bye!
– Ari Waisberg
Dec 30 '18 at 1:03