Why isn't my code reading the correct integers from a file?
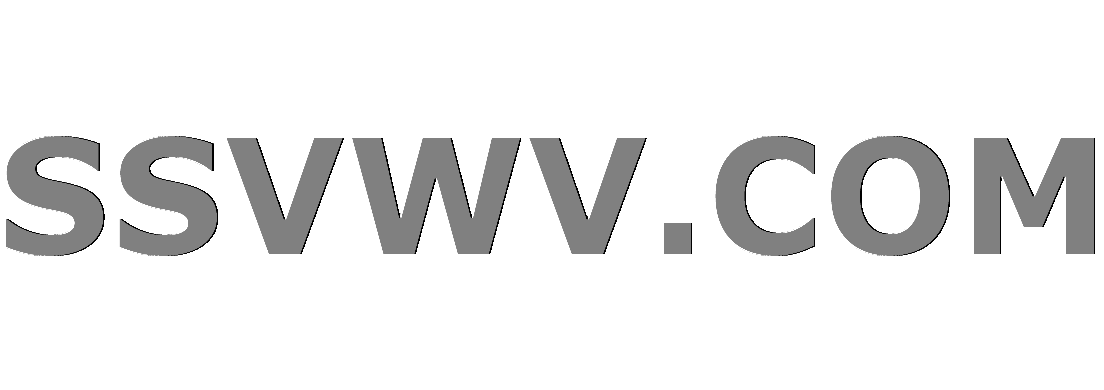
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
So after playing a little bit with Craig Estey's answer, I managed to get the following piece of code:
#include <string.h>
#include <stdio.h>
#include <stdlib.h>
#include <errno.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
int main(int argc,char **argv){
char *filename = argv[1];
if(filename == NULL) {
printf("Please specify a filename.n");
exit(1);
}
FILE *fi = fopen(filename,"r");
printf("Finding size...n");
int size = 0;
while(fscanf(fi,"%*d") != -1) size++;
rewind(fi);
int numbers[size];
while(fscanf(fi,"%d",&numbers[size]) != 1);
fclose(fi);
printf("Size of array: %dnn", size);
int idx=0,idx2=1,idx3=1,idx4=1;
printf("Elements: n");
while (idx < size){
printf("%dn",numbers[idx]);
idx++;
}
int maximum = numbers[0];
while (idx2 < size){
if (numbers[idx2] > maximum){
maximum = numbers[idx2];
}
idx2++;
}
printf("nMax: %dn",maximum);
int minimum = numbers[0];
while (idx3 < size){
if (numbers[idx3] < minimum){
minimum = numbers[idx3];
}
idx3++;
}
printf("Min: %dn",minimum);
int sum = numbers[0];
while (idx4 < size){
sum = sum + numbers[idx4];
idx4++;
}
printf("Total: %dn",sum);
}
Problem is, now it executes without halting, but still doesn't provide the right answer. My 'abcd.txt' file contains the following numbers:
5564
4324
863
98743
However, my result after executing reader.c is:
./reader abcd.txt
Finding size...
Size of array: 4
Elements:
0
0
1384783917
32713
Max: 1384783917
Min: 0
Total: 1384816630
Why is that, now? I cannot find why is it different than in the answer below. If I execute the exact code in the answer, it does return the right answer. Thanks in advance.
c
|
show 11 more comments
So after playing a little bit with Craig Estey's answer, I managed to get the following piece of code:
#include <string.h>
#include <stdio.h>
#include <stdlib.h>
#include <errno.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
int main(int argc,char **argv){
char *filename = argv[1];
if(filename == NULL) {
printf("Please specify a filename.n");
exit(1);
}
FILE *fi = fopen(filename,"r");
printf("Finding size...n");
int size = 0;
while(fscanf(fi,"%*d") != -1) size++;
rewind(fi);
int numbers[size];
while(fscanf(fi,"%d",&numbers[size]) != 1);
fclose(fi);
printf("Size of array: %dnn", size);
int idx=0,idx2=1,idx3=1,idx4=1;
printf("Elements: n");
while (idx < size){
printf("%dn",numbers[idx]);
idx++;
}
int maximum = numbers[0];
while (idx2 < size){
if (numbers[idx2] > maximum){
maximum = numbers[idx2];
}
idx2++;
}
printf("nMax: %dn",maximum);
int minimum = numbers[0];
while (idx3 < size){
if (numbers[idx3] < minimum){
minimum = numbers[idx3];
}
idx3++;
}
printf("Min: %dn",minimum);
int sum = numbers[0];
while (idx4 < size){
sum = sum + numbers[idx4];
idx4++;
}
printf("Total: %dn",sum);
}
Problem is, now it executes without halting, but still doesn't provide the right answer. My 'abcd.txt' file contains the following numbers:
5564
4324
863
98743
However, my result after executing reader.c is:
./reader abcd.txt
Finding size...
Size of array: 4
Elements:
0
0
1384783917
32713
Max: 1384783917
Min: 0
Total: 1384816630
Why is that, now? I cannot find why is it different than in the answer below. If I execute the exact code in the answer, it does return the right answer. Thanks in advance.
c
6
void main (char *filename)
isn’t a correct signature formain
– you should have learnedint main(int argc, char* argv)
. Might also want to read How to Ask
– Ry-♦
Jan 3 at 22:45
1
Ry- found [at least] your initial problem. Definemain
asint main(int argc,char **argv)
and then dofopen(argv[1],"r")
. As you've definedmain
, yourfilename
pointer will be given [silently] a value of 0x02 [or some other worse/garbage value] which causes a segfault due to an invalid pointer dereference.
– Craig Estey
Jan 3 at 22:51
1
You should edit your question and post your sample input file in a separate code block. This allows us to download, compile, link, and run your program with enough input to reproduce your problem [beyond the fix for the segfault]. Note that you should do:char *filename = argv[1];
to handle all places wherefilename
is used. Note that this is a quick fix because it doesn't handle the case where the program argument is omitted (e.g.argc
is 1 andargv[1] == NULL
)
– Craig Estey
Jan 3 at 22:54
5
Turn on compiler warnings and use a debugger.
– Broman
Jan 3 at 23:02
1
numbers[idx] = (int *) p_data;
perhaps you meantnumbers[idx] = *(int *) p_data;
? The compiler will not compile the original statement.
– Paul Rooney
Jan 3 at 23:09
|
show 11 more comments
So after playing a little bit with Craig Estey's answer, I managed to get the following piece of code:
#include <string.h>
#include <stdio.h>
#include <stdlib.h>
#include <errno.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
int main(int argc,char **argv){
char *filename = argv[1];
if(filename == NULL) {
printf("Please specify a filename.n");
exit(1);
}
FILE *fi = fopen(filename,"r");
printf("Finding size...n");
int size = 0;
while(fscanf(fi,"%*d") != -1) size++;
rewind(fi);
int numbers[size];
while(fscanf(fi,"%d",&numbers[size]) != 1);
fclose(fi);
printf("Size of array: %dnn", size);
int idx=0,idx2=1,idx3=1,idx4=1;
printf("Elements: n");
while (idx < size){
printf("%dn",numbers[idx]);
idx++;
}
int maximum = numbers[0];
while (idx2 < size){
if (numbers[idx2] > maximum){
maximum = numbers[idx2];
}
idx2++;
}
printf("nMax: %dn",maximum);
int minimum = numbers[0];
while (idx3 < size){
if (numbers[idx3] < minimum){
minimum = numbers[idx3];
}
idx3++;
}
printf("Min: %dn",minimum);
int sum = numbers[0];
while (idx4 < size){
sum = sum + numbers[idx4];
idx4++;
}
printf("Total: %dn",sum);
}
Problem is, now it executes without halting, but still doesn't provide the right answer. My 'abcd.txt' file contains the following numbers:
5564
4324
863
98743
However, my result after executing reader.c is:
./reader abcd.txt
Finding size...
Size of array: 4
Elements:
0
0
1384783917
32713
Max: 1384783917
Min: 0
Total: 1384816630
Why is that, now? I cannot find why is it different than in the answer below. If I execute the exact code in the answer, it does return the right answer. Thanks in advance.
c
So after playing a little bit with Craig Estey's answer, I managed to get the following piece of code:
#include <string.h>
#include <stdio.h>
#include <stdlib.h>
#include <errno.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
int main(int argc,char **argv){
char *filename = argv[1];
if(filename == NULL) {
printf("Please specify a filename.n");
exit(1);
}
FILE *fi = fopen(filename,"r");
printf("Finding size...n");
int size = 0;
while(fscanf(fi,"%*d") != -1) size++;
rewind(fi);
int numbers[size];
while(fscanf(fi,"%d",&numbers[size]) != 1);
fclose(fi);
printf("Size of array: %dnn", size);
int idx=0,idx2=1,idx3=1,idx4=1;
printf("Elements: n");
while (idx < size){
printf("%dn",numbers[idx]);
idx++;
}
int maximum = numbers[0];
while (idx2 < size){
if (numbers[idx2] > maximum){
maximum = numbers[idx2];
}
idx2++;
}
printf("nMax: %dn",maximum);
int minimum = numbers[0];
while (idx3 < size){
if (numbers[idx3] < minimum){
minimum = numbers[idx3];
}
idx3++;
}
printf("Min: %dn",minimum);
int sum = numbers[0];
while (idx4 < size){
sum = sum + numbers[idx4];
idx4++;
}
printf("Total: %dn",sum);
}
Problem is, now it executes without halting, but still doesn't provide the right answer. My 'abcd.txt' file contains the following numbers:
5564
4324
863
98743
However, my result after executing reader.c is:
./reader abcd.txt
Finding size...
Size of array: 4
Elements:
0
0
1384783917
32713
Max: 1384783917
Min: 0
Total: 1384816630
Why is that, now? I cannot find why is it different than in the answer below. If I execute the exact code in the answer, it does return the right answer. Thanks in advance.
c
c
edited Jan 6 at 13:02
Beroun
asked Jan 3 at 22:41
BerounBeroun
83
83
6
void main (char *filename)
isn’t a correct signature formain
– you should have learnedint main(int argc, char* argv)
. Might also want to read How to Ask
– Ry-♦
Jan 3 at 22:45
1
Ry- found [at least] your initial problem. Definemain
asint main(int argc,char **argv)
and then dofopen(argv[1],"r")
. As you've definedmain
, yourfilename
pointer will be given [silently] a value of 0x02 [or some other worse/garbage value] which causes a segfault due to an invalid pointer dereference.
– Craig Estey
Jan 3 at 22:51
1
You should edit your question and post your sample input file in a separate code block. This allows us to download, compile, link, and run your program with enough input to reproduce your problem [beyond the fix for the segfault]. Note that you should do:char *filename = argv[1];
to handle all places wherefilename
is used. Note that this is a quick fix because it doesn't handle the case where the program argument is omitted (e.g.argc
is 1 andargv[1] == NULL
)
– Craig Estey
Jan 3 at 22:54
5
Turn on compiler warnings and use a debugger.
– Broman
Jan 3 at 23:02
1
numbers[idx] = (int *) p_data;
perhaps you meantnumbers[idx] = *(int *) p_data;
? The compiler will not compile the original statement.
– Paul Rooney
Jan 3 at 23:09
|
show 11 more comments
6
void main (char *filename)
isn’t a correct signature formain
– you should have learnedint main(int argc, char* argv)
. Might also want to read How to Ask
– Ry-♦
Jan 3 at 22:45
1
Ry- found [at least] your initial problem. Definemain
asint main(int argc,char **argv)
and then dofopen(argv[1],"r")
. As you've definedmain
, yourfilename
pointer will be given [silently] a value of 0x02 [or some other worse/garbage value] which causes a segfault due to an invalid pointer dereference.
– Craig Estey
Jan 3 at 22:51
1
You should edit your question and post your sample input file in a separate code block. This allows us to download, compile, link, and run your program with enough input to reproduce your problem [beyond the fix for the segfault]. Note that you should do:char *filename = argv[1];
to handle all places wherefilename
is used. Note that this is a quick fix because it doesn't handle the case where the program argument is omitted (e.g.argc
is 1 andargv[1] == NULL
)
– Craig Estey
Jan 3 at 22:54
5
Turn on compiler warnings and use a debugger.
– Broman
Jan 3 at 23:02
1
numbers[idx] = (int *) p_data;
perhaps you meantnumbers[idx] = *(int *) p_data;
? The compiler will not compile the original statement.
– Paul Rooney
Jan 3 at 23:09
6
6
void main (char *filename)
isn’t a correct signature for main
– you should have learned int main(int argc, char* argv)
. Might also want to read How to Ask– Ry-♦
Jan 3 at 22:45
void main (char *filename)
isn’t a correct signature for main
– you should have learned int main(int argc, char* argv)
. Might also want to read How to Ask– Ry-♦
Jan 3 at 22:45
1
1
Ry- found [at least] your initial problem. Define
main
as int main(int argc,char **argv)
and then do fopen(argv[1],"r")
. As you've defined main
, your filename
pointer will be given [silently] a value of 0x02 [or some other worse/garbage value] which causes a segfault due to an invalid pointer dereference.– Craig Estey
Jan 3 at 22:51
Ry- found [at least] your initial problem. Define
main
as int main(int argc,char **argv)
and then do fopen(argv[1],"r")
. As you've defined main
, your filename
pointer will be given [silently] a value of 0x02 [or some other worse/garbage value] which causes a segfault due to an invalid pointer dereference.– Craig Estey
Jan 3 at 22:51
1
1
You should edit your question and post your sample input file in a separate code block. This allows us to download, compile, link, and run your program with enough input to reproduce your problem [beyond the fix for the segfault]. Note that you should do:
char *filename = argv[1];
to handle all places where filename
is used. Note that this is a quick fix because it doesn't handle the case where the program argument is omitted (e.g. argc
is 1 and argv[1] == NULL
)– Craig Estey
Jan 3 at 22:54
You should edit your question and post your sample input file in a separate code block. This allows us to download, compile, link, and run your program with enough input to reproduce your problem [beyond the fix for the segfault]. Note that you should do:
char *filename = argv[1];
to handle all places where filename
is used. Note that this is a quick fix because it doesn't handle the case where the program argument is omitted (e.g. argc
is 1 and argv[1] == NULL
)– Craig Estey
Jan 3 at 22:54
5
5
Turn on compiler warnings and use a debugger.
– Broman
Jan 3 at 23:02
Turn on compiler warnings and use a debugger.
– Broman
Jan 3 at 23:02
1
1
numbers[idx] = (int *) p_data;
perhaps you meant numbers[idx] = *(int *) p_data;
? The compiler will not compile the original statement.– Paul Rooney
Jan 3 at 23:09
numbers[idx] = (int *) p_data;
perhaps you meant numbers[idx] = *(int *) p_data;
? The compiler will not compile the original statement.– Paul Rooney
Jan 3 at 23:09
|
show 11 more comments
1 Answer
1
active
oldest
votes
This is prefaced by my top comments.
You can't do: printf(numbers[idx2]);
(i.e. the first argument has to be a format string). So, do: printf("%dn",numbers[idx2]);
Doing sizeof(numbers) / sizeof(int)
does not give an accurate count of the number actually filled in. It gives the maximum which will have garbage values at the end.
Because, there were so many errors, I couldn't list them all. I had to completely refactor your code. Just compare it in detail to yours to see the difference. You'll learn much more and more quickly than trying to patch what you already have.
#include <errno.h>
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <unistd.h>
long int
findSize(char *filename)
{
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
printf("findSize: unable to open file '%s' -- %sn",
filename,strerror(errno));
exit(1);
}
fseek(fp, 0L, SEEK_END);
long int res = ftell(fp);
fclose(fp);
return res;
}
int
main(int argc, char **argv)
{
char *filename = argv[1];
if (filename == NULL) {
printf("please specify a filenamen");
exit(1);
}
printf("findSizen");
int numbers[findSize(filename)];
printf("scanfn");
FILE *fi = fopen(filename,"r");
int size = 0;
while (1) {
if (fscanf(fi,"%d",&numbers[size]) != 1)
break;
printf("scanf: %dn",numbers[size]);
++size;
}
fclose(fi);
printf("size: %dn",size);
for (int idx2 = 0; idx2 < size; ++idx2)
printf("%dn",numbers[idx2]);
int maximum = numbers[0];
for (int idx3 = 1; idx3 < size; ++idx3) {
if (numbers[idx3] > maximum)
maximum = numbers[idx3];
}
printf("Max: %dn", maximum);
int minimum = numbers[0];
for (int idx4 = 1; idx4 < size; ++idx4) {
if (numbers[idx4] < minimum)
minimum = numbers[idx4];
}
printf("Min: %dn", minimum);
int sum = 0;
for (int idx5 = 0; idx5 < size; ++idx5)
sum = sum + numbers[idx5];
printf("Total: %dn", sum);
return 0;
}
UPDATE:
First of all, thanks a lot for your work. Looking at your code (I cannot call it anymore my code as it's completely different)
Yes, it was intended as a guide for you to write/rewrite your own code, which you've done.
I see that the findSize function is mostly irrelevant.
It is technically correct, but would allocate [much] more space than was needed.
I tried to put another fscanf instead of calling a findSize function, but I don't know how this function works exactly.
Good for you in figuring out this alternate sizing algorithm.
Let me see if I get it: If there is a third argument, it stores each read element into the third argument (which should be a variable). But if there is no third element, and you put an * in the second argument, it doesn't store the read elements anywhere. Am I right?
Believe it or not, I don't use fscanf
too much as it can be slow, so I'm not familiar with some of the more esoteric options. I've tested your new sizing loop and it appears to work, so I think your analysis may be correct.
Also, we could simply introduce an extra scalar int
variable and do:
int size = 0;
while (1) {
int val;
if (fscanf(fi,"%d",&val) != 1)
break;
++size;
}
However, you're actual second fscanf
loop that is used to read in the numbers will not work:
while (fscanf(fi, "%d", &numbers[size]) != 1);
This puts all numbers in the same index, namely size
, which is one beyond the end of the numbers
array, so this is undefined behavior.
Also, the loop condition is reversed from what it should be, so only one number would be filled in.
To fix this, we need an additional index variable and need to change the sense of the while
loop:
int idx0 = 0;
while (fscanf(fi, "%d", &numbers[idx0]) == 1)
++idx0;
After this change, the rest of your updated code [min/max/sum] works fine.
Some notes on style:
As you noticed, my original posted code was a bit different (e.g. replacing while
loops with for
loops).
But, one thing I didn't do was reuse the index variable. That is, there isn't a need to have separate ones for each loop. They could all be changed to a single idx
variable.
Using different symbols isn't wrong, and can add to clarity. But, idx
, idx1
, idx2
aren't as descriptive as they could be.
If you'd like to keep separate variables, I'd suggest more descriptive names such as: inpidx
, minidx
, maxidx
, and sumidx
Why the DV? The guidelines for DV on SO are an answer that is egregiously wrong.
– Craig Estey
Jan 4 at 0:17
First of all, thanks a lot for your work. Looking at your code (I cannot call it anymore my code as it's completely different) I see that the findSize function is mostly irrelevant. I tried to put another fscanf instead of calling a findSize function, but I don't know how this function works exactly. Let me see if I get it: If there is a third argument, it stores each read element into the third argument (which should be a variable). But if there is no third element, and you put an * in the second argument, it doesn't store the read elements anywhere. Am I right? (continuation below)
– Beroun
Jan 5 at 17:27
Thanks! These 2 errors (reversing the loop condition and forgetting to reset size before the loop and increase inside the loop) were quite embarassing. Now it works perfectly. The while are there because for some reason, if I use 'for', the console spits a warning saying 'You need to compile in C99 mode'. I obviously can add -std=c99 to the end, but I preferred to just use whiles instead (I don't like warnings). Thanks again!
– Beroun
Jan 5 at 22:01
@CraigEstey it’s probably a punishment down vote. Someone’s way of saying don’t answer out of scope questions. Personally if people want to put in the effort to answers these question, more power to them.
– Paul Rooney
Jan 7 at 22:05
@PaulRooney Thanks. The DV came early (before the update section). I suspect it was punishment--from the "don't answer homework questions" camp [which I'm not a member of]. IMO, when a question is so far away, merely "hints" aren't going to help an OP much. Normally, I try to remain [somewhat] faithful to an OP's code, give an explanation of errors, annotate the code with the bugs [and fixes] and sometimes present a second cleaned up version. A number of OPs like this approach. And, here, OP was able to take my version as a guide to fixing his own code [just what I had hoped for].
– Craig Estey
Jan 7 at 22:32
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54030811%2fwhy-isnt-my-code-reading-the-correct-integers-from-a-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This is prefaced by my top comments.
You can't do: printf(numbers[idx2]);
(i.e. the first argument has to be a format string). So, do: printf("%dn",numbers[idx2]);
Doing sizeof(numbers) / sizeof(int)
does not give an accurate count of the number actually filled in. It gives the maximum which will have garbage values at the end.
Because, there were so many errors, I couldn't list them all. I had to completely refactor your code. Just compare it in detail to yours to see the difference. You'll learn much more and more quickly than trying to patch what you already have.
#include <errno.h>
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <unistd.h>
long int
findSize(char *filename)
{
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
printf("findSize: unable to open file '%s' -- %sn",
filename,strerror(errno));
exit(1);
}
fseek(fp, 0L, SEEK_END);
long int res = ftell(fp);
fclose(fp);
return res;
}
int
main(int argc, char **argv)
{
char *filename = argv[1];
if (filename == NULL) {
printf("please specify a filenamen");
exit(1);
}
printf("findSizen");
int numbers[findSize(filename)];
printf("scanfn");
FILE *fi = fopen(filename,"r");
int size = 0;
while (1) {
if (fscanf(fi,"%d",&numbers[size]) != 1)
break;
printf("scanf: %dn",numbers[size]);
++size;
}
fclose(fi);
printf("size: %dn",size);
for (int idx2 = 0; idx2 < size; ++idx2)
printf("%dn",numbers[idx2]);
int maximum = numbers[0];
for (int idx3 = 1; idx3 < size; ++idx3) {
if (numbers[idx3] > maximum)
maximum = numbers[idx3];
}
printf("Max: %dn", maximum);
int minimum = numbers[0];
for (int idx4 = 1; idx4 < size; ++idx4) {
if (numbers[idx4] < minimum)
minimum = numbers[idx4];
}
printf("Min: %dn", minimum);
int sum = 0;
for (int idx5 = 0; idx5 < size; ++idx5)
sum = sum + numbers[idx5];
printf("Total: %dn", sum);
return 0;
}
UPDATE:
First of all, thanks a lot for your work. Looking at your code (I cannot call it anymore my code as it's completely different)
Yes, it was intended as a guide for you to write/rewrite your own code, which you've done.
I see that the findSize function is mostly irrelevant.
It is technically correct, but would allocate [much] more space than was needed.
I tried to put another fscanf instead of calling a findSize function, but I don't know how this function works exactly.
Good for you in figuring out this alternate sizing algorithm.
Let me see if I get it: If there is a third argument, it stores each read element into the third argument (which should be a variable). But if there is no third element, and you put an * in the second argument, it doesn't store the read elements anywhere. Am I right?
Believe it or not, I don't use fscanf
too much as it can be slow, so I'm not familiar with some of the more esoteric options. I've tested your new sizing loop and it appears to work, so I think your analysis may be correct.
Also, we could simply introduce an extra scalar int
variable and do:
int size = 0;
while (1) {
int val;
if (fscanf(fi,"%d",&val) != 1)
break;
++size;
}
However, you're actual second fscanf
loop that is used to read in the numbers will not work:
while (fscanf(fi, "%d", &numbers[size]) != 1);
This puts all numbers in the same index, namely size
, which is one beyond the end of the numbers
array, so this is undefined behavior.
Also, the loop condition is reversed from what it should be, so only one number would be filled in.
To fix this, we need an additional index variable and need to change the sense of the while
loop:
int idx0 = 0;
while (fscanf(fi, "%d", &numbers[idx0]) == 1)
++idx0;
After this change, the rest of your updated code [min/max/sum] works fine.
Some notes on style:
As you noticed, my original posted code was a bit different (e.g. replacing while
loops with for
loops).
But, one thing I didn't do was reuse the index variable. That is, there isn't a need to have separate ones for each loop. They could all be changed to a single idx
variable.
Using different symbols isn't wrong, and can add to clarity. But, idx
, idx1
, idx2
aren't as descriptive as they could be.
If you'd like to keep separate variables, I'd suggest more descriptive names such as: inpidx
, minidx
, maxidx
, and sumidx
Why the DV? The guidelines for DV on SO are an answer that is egregiously wrong.
– Craig Estey
Jan 4 at 0:17
First of all, thanks a lot for your work. Looking at your code (I cannot call it anymore my code as it's completely different) I see that the findSize function is mostly irrelevant. I tried to put another fscanf instead of calling a findSize function, but I don't know how this function works exactly. Let me see if I get it: If there is a third argument, it stores each read element into the third argument (which should be a variable). But if there is no third element, and you put an * in the second argument, it doesn't store the read elements anywhere. Am I right? (continuation below)
– Beroun
Jan 5 at 17:27
Thanks! These 2 errors (reversing the loop condition and forgetting to reset size before the loop and increase inside the loop) were quite embarassing. Now it works perfectly. The while are there because for some reason, if I use 'for', the console spits a warning saying 'You need to compile in C99 mode'. I obviously can add -std=c99 to the end, but I preferred to just use whiles instead (I don't like warnings). Thanks again!
– Beroun
Jan 5 at 22:01
@CraigEstey it’s probably a punishment down vote. Someone’s way of saying don’t answer out of scope questions. Personally if people want to put in the effort to answers these question, more power to them.
– Paul Rooney
Jan 7 at 22:05
@PaulRooney Thanks. The DV came early (before the update section). I suspect it was punishment--from the "don't answer homework questions" camp [which I'm not a member of]. IMO, when a question is so far away, merely "hints" aren't going to help an OP much. Normally, I try to remain [somewhat] faithful to an OP's code, give an explanation of errors, annotate the code with the bugs [and fixes] and sometimes present a second cleaned up version. A number of OPs like this approach. And, here, OP was able to take my version as a guide to fixing his own code [just what I had hoped for].
– Craig Estey
Jan 7 at 22:32
add a comment |
This is prefaced by my top comments.
You can't do: printf(numbers[idx2]);
(i.e. the first argument has to be a format string). So, do: printf("%dn",numbers[idx2]);
Doing sizeof(numbers) / sizeof(int)
does not give an accurate count of the number actually filled in. It gives the maximum which will have garbage values at the end.
Because, there were so many errors, I couldn't list them all. I had to completely refactor your code. Just compare it in detail to yours to see the difference. You'll learn much more and more quickly than trying to patch what you already have.
#include <errno.h>
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <unistd.h>
long int
findSize(char *filename)
{
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
printf("findSize: unable to open file '%s' -- %sn",
filename,strerror(errno));
exit(1);
}
fseek(fp, 0L, SEEK_END);
long int res = ftell(fp);
fclose(fp);
return res;
}
int
main(int argc, char **argv)
{
char *filename = argv[1];
if (filename == NULL) {
printf("please specify a filenamen");
exit(1);
}
printf("findSizen");
int numbers[findSize(filename)];
printf("scanfn");
FILE *fi = fopen(filename,"r");
int size = 0;
while (1) {
if (fscanf(fi,"%d",&numbers[size]) != 1)
break;
printf("scanf: %dn",numbers[size]);
++size;
}
fclose(fi);
printf("size: %dn",size);
for (int idx2 = 0; idx2 < size; ++idx2)
printf("%dn",numbers[idx2]);
int maximum = numbers[0];
for (int idx3 = 1; idx3 < size; ++idx3) {
if (numbers[idx3] > maximum)
maximum = numbers[idx3];
}
printf("Max: %dn", maximum);
int minimum = numbers[0];
for (int idx4 = 1; idx4 < size; ++idx4) {
if (numbers[idx4] < minimum)
minimum = numbers[idx4];
}
printf("Min: %dn", minimum);
int sum = 0;
for (int idx5 = 0; idx5 < size; ++idx5)
sum = sum + numbers[idx5];
printf("Total: %dn", sum);
return 0;
}
UPDATE:
First of all, thanks a lot for your work. Looking at your code (I cannot call it anymore my code as it's completely different)
Yes, it was intended as a guide for you to write/rewrite your own code, which you've done.
I see that the findSize function is mostly irrelevant.
It is technically correct, but would allocate [much] more space than was needed.
I tried to put another fscanf instead of calling a findSize function, but I don't know how this function works exactly.
Good for you in figuring out this alternate sizing algorithm.
Let me see if I get it: If there is a third argument, it stores each read element into the third argument (which should be a variable). But if there is no third element, and you put an * in the second argument, it doesn't store the read elements anywhere. Am I right?
Believe it or not, I don't use fscanf
too much as it can be slow, so I'm not familiar with some of the more esoteric options. I've tested your new sizing loop and it appears to work, so I think your analysis may be correct.
Also, we could simply introduce an extra scalar int
variable and do:
int size = 0;
while (1) {
int val;
if (fscanf(fi,"%d",&val) != 1)
break;
++size;
}
However, you're actual second fscanf
loop that is used to read in the numbers will not work:
while (fscanf(fi, "%d", &numbers[size]) != 1);
This puts all numbers in the same index, namely size
, which is one beyond the end of the numbers
array, so this is undefined behavior.
Also, the loop condition is reversed from what it should be, so only one number would be filled in.
To fix this, we need an additional index variable and need to change the sense of the while
loop:
int idx0 = 0;
while (fscanf(fi, "%d", &numbers[idx0]) == 1)
++idx0;
After this change, the rest of your updated code [min/max/sum] works fine.
Some notes on style:
As you noticed, my original posted code was a bit different (e.g. replacing while
loops with for
loops).
But, one thing I didn't do was reuse the index variable. That is, there isn't a need to have separate ones for each loop. They could all be changed to a single idx
variable.
Using different symbols isn't wrong, and can add to clarity. But, idx
, idx1
, idx2
aren't as descriptive as they could be.
If you'd like to keep separate variables, I'd suggest more descriptive names such as: inpidx
, minidx
, maxidx
, and sumidx
Why the DV? The guidelines for DV on SO are an answer that is egregiously wrong.
– Craig Estey
Jan 4 at 0:17
First of all, thanks a lot for your work. Looking at your code (I cannot call it anymore my code as it's completely different) I see that the findSize function is mostly irrelevant. I tried to put another fscanf instead of calling a findSize function, but I don't know how this function works exactly. Let me see if I get it: If there is a third argument, it stores each read element into the third argument (which should be a variable). But if there is no third element, and you put an * in the second argument, it doesn't store the read elements anywhere. Am I right? (continuation below)
– Beroun
Jan 5 at 17:27
Thanks! These 2 errors (reversing the loop condition and forgetting to reset size before the loop and increase inside the loop) were quite embarassing. Now it works perfectly. The while are there because for some reason, if I use 'for', the console spits a warning saying 'You need to compile in C99 mode'. I obviously can add -std=c99 to the end, but I preferred to just use whiles instead (I don't like warnings). Thanks again!
– Beroun
Jan 5 at 22:01
@CraigEstey it’s probably a punishment down vote. Someone’s way of saying don’t answer out of scope questions. Personally if people want to put in the effort to answers these question, more power to them.
– Paul Rooney
Jan 7 at 22:05
@PaulRooney Thanks. The DV came early (before the update section). I suspect it was punishment--from the "don't answer homework questions" camp [which I'm not a member of]. IMO, when a question is so far away, merely "hints" aren't going to help an OP much. Normally, I try to remain [somewhat] faithful to an OP's code, give an explanation of errors, annotate the code with the bugs [and fixes] and sometimes present a second cleaned up version. A number of OPs like this approach. And, here, OP was able to take my version as a guide to fixing his own code [just what I had hoped for].
– Craig Estey
Jan 7 at 22:32
add a comment |
This is prefaced by my top comments.
You can't do: printf(numbers[idx2]);
(i.e. the first argument has to be a format string). So, do: printf("%dn",numbers[idx2]);
Doing sizeof(numbers) / sizeof(int)
does not give an accurate count of the number actually filled in. It gives the maximum which will have garbage values at the end.
Because, there were so many errors, I couldn't list them all. I had to completely refactor your code. Just compare it in detail to yours to see the difference. You'll learn much more and more quickly than trying to patch what you already have.
#include <errno.h>
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <unistd.h>
long int
findSize(char *filename)
{
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
printf("findSize: unable to open file '%s' -- %sn",
filename,strerror(errno));
exit(1);
}
fseek(fp, 0L, SEEK_END);
long int res = ftell(fp);
fclose(fp);
return res;
}
int
main(int argc, char **argv)
{
char *filename = argv[1];
if (filename == NULL) {
printf("please specify a filenamen");
exit(1);
}
printf("findSizen");
int numbers[findSize(filename)];
printf("scanfn");
FILE *fi = fopen(filename,"r");
int size = 0;
while (1) {
if (fscanf(fi,"%d",&numbers[size]) != 1)
break;
printf("scanf: %dn",numbers[size]);
++size;
}
fclose(fi);
printf("size: %dn",size);
for (int idx2 = 0; idx2 < size; ++idx2)
printf("%dn",numbers[idx2]);
int maximum = numbers[0];
for (int idx3 = 1; idx3 < size; ++idx3) {
if (numbers[idx3] > maximum)
maximum = numbers[idx3];
}
printf("Max: %dn", maximum);
int minimum = numbers[0];
for (int idx4 = 1; idx4 < size; ++idx4) {
if (numbers[idx4] < minimum)
minimum = numbers[idx4];
}
printf("Min: %dn", minimum);
int sum = 0;
for (int idx5 = 0; idx5 < size; ++idx5)
sum = sum + numbers[idx5];
printf("Total: %dn", sum);
return 0;
}
UPDATE:
First of all, thanks a lot for your work. Looking at your code (I cannot call it anymore my code as it's completely different)
Yes, it was intended as a guide for you to write/rewrite your own code, which you've done.
I see that the findSize function is mostly irrelevant.
It is technically correct, but would allocate [much] more space than was needed.
I tried to put another fscanf instead of calling a findSize function, but I don't know how this function works exactly.
Good for you in figuring out this alternate sizing algorithm.
Let me see if I get it: If there is a third argument, it stores each read element into the third argument (which should be a variable). But if there is no third element, and you put an * in the second argument, it doesn't store the read elements anywhere. Am I right?
Believe it or not, I don't use fscanf
too much as it can be slow, so I'm not familiar with some of the more esoteric options. I've tested your new sizing loop and it appears to work, so I think your analysis may be correct.
Also, we could simply introduce an extra scalar int
variable and do:
int size = 0;
while (1) {
int val;
if (fscanf(fi,"%d",&val) != 1)
break;
++size;
}
However, you're actual second fscanf
loop that is used to read in the numbers will not work:
while (fscanf(fi, "%d", &numbers[size]) != 1);
This puts all numbers in the same index, namely size
, which is one beyond the end of the numbers
array, so this is undefined behavior.
Also, the loop condition is reversed from what it should be, so only one number would be filled in.
To fix this, we need an additional index variable and need to change the sense of the while
loop:
int idx0 = 0;
while (fscanf(fi, "%d", &numbers[idx0]) == 1)
++idx0;
After this change, the rest of your updated code [min/max/sum] works fine.
Some notes on style:
As you noticed, my original posted code was a bit different (e.g. replacing while
loops with for
loops).
But, one thing I didn't do was reuse the index variable. That is, there isn't a need to have separate ones for each loop. They could all be changed to a single idx
variable.
Using different symbols isn't wrong, and can add to clarity. But, idx
, idx1
, idx2
aren't as descriptive as they could be.
If you'd like to keep separate variables, I'd suggest more descriptive names such as: inpidx
, minidx
, maxidx
, and sumidx
This is prefaced by my top comments.
You can't do: printf(numbers[idx2]);
(i.e. the first argument has to be a format string). So, do: printf("%dn",numbers[idx2]);
Doing sizeof(numbers) / sizeof(int)
does not give an accurate count of the number actually filled in. It gives the maximum which will have garbage values at the end.
Because, there were so many errors, I couldn't list them all. I had to completely refactor your code. Just compare it in detail to yours to see the difference. You'll learn much more and more quickly than trying to patch what you already have.
#include <errno.h>
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <unistd.h>
long int
findSize(char *filename)
{
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
printf("findSize: unable to open file '%s' -- %sn",
filename,strerror(errno));
exit(1);
}
fseek(fp, 0L, SEEK_END);
long int res = ftell(fp);
fclose(fp);
return res;
}
int
main(int argc, char **argv)
{
char *filename = argv[1];
if (filename == NULL) {
printf("please specify a filenamen");
exit(1);
}
printf("findSizen");
int numbers[findSize(filename)];
printf("scanfn");
FILE *fi = fopen(filename,"r");
int size = 0;
while (1) {
if (fscanf(fi,"%d",&numbers[size]) != 1)
break;
printf("scanf: %dn",numbers[size]);
++size;
}
fclose(fi);
printf("size: %dn",size);
for (int idx2 = 0; idx2 < size; ++idx2)
printf("%dn",numbers[idx2]);
int maximum = numbers[0];
for (int idx3 = 1; idx3 < size; ++idx3) {
if (numbers[idx3] > maximum)
maximum = numbers[idx3];
}
printf("Max: %dn", maximum);
int minimum = numbers[0];
for (int idx4 = 1; idx4 < size; ++idx4) {
if (numbers[idx4] < minimum)
minimum = numbers[idx4];
}
printf("Min: %dn", minimum);
int sum = 0;
for (int idx5 = 0; idx5 < size; ++idx5)
sum = sum + numbers[idx5];
printf("Total: %dn", sum);
return 0;
}
UPDATE:
First of all, thanks a lot for your work. Looking at your code (I cannot call it anymore my code as it's completely different)
Yes, it was intended as a guide for you to write/rewrite your own code, which you've done.
I see that the findSize function is mostly irrelevant.
It is technically correct, but would allocate [much] more space than was needed.
I tried to put another fscanf instead of calling a findSize function, but I don't know how this function works exactly.
Good for you in figuring out this alternate sizing algorithm.
Let me see if I get it: If there is a third argument, it stores each read element into the third argument (which should be a variable). But if there is no third element, and you put an * in the second argument, it doesn't store the read elements anywhere. Am I right?
Believe it or not, I don't use fscanf
too much as it can be slow, so I'm not familiar with some of the more esoteric options. I've tested your new sizing loop and it appears to work, so I think your analysis may be correct.
Also, we could simply introduce an extra scalar int
variable and do:
int size = 0;
while (1) {
int val;
if (fscanf(fi,"%d",&val) != 1)
break;
++size;
}
However, you're actual second fscanf
loop that is used to read in the numbers will not work:
while (fscanf(fi, "%d", &numbers[size]) != 1);
This puts all numbers in the same index, namely size
, which is one beyond the end of the numbers
array, so this is undefined behavior.
Also, the loop condition is reversed from what it should be, so only one number would be filled in.
To fix this, we need an additional index variable and need to change the sense of the while
loop:
int idx0 = 0;
while (fscanf(fi, "%d", &numbers[idx0]) == 1)
++idx0;
After this change, the rest of your updated code [min/max/sum] works fine.
Some notes on style:
As you noticed, my original posted code was a bit different (e.g. replacing while
loops with for
loops).
But, one thing I didn't do was reuse the index variable. That is, there isn't a need to have separate ones for each loop. They could all be changed to a single idx
variable.
Using different symbols isn't wrong, and can add to clarity. But, idx
, idx1
, idx2
aren't as descriptive as they could be.
If you'd like to keep separate variables, I'd suggest more descriptive names such as: inpidx
, minidx
, maxidx
, and sumidx
edited Jan 5 at 20:17
answered Jan 3 at 23:57
Craig EsteyCraig Estey
15.3k21131
15.3k21131
Why the DV? The guidelines for DV on SO are an answer that is egregiously wrong.
– Craig Estey
Jan 4 at 0:17
First of all, thanks a lot for your work. Looking at your code (I cannot call it anymore my code as it's completely different) I see that the findSize function is mostly irrelevant. I tried to put another fscanf instead of calling a findSize function, but I don't know how this function works exactly. Let me see if I get it: If there is a third argument, it stores each read element into the third argument (which should be a variable). But if there is no third element, and you put an * in the second argument, it doesn't store the read elements anywhere. Am I right? (continuation below)
– Beroun
Jan 5 at 17:27
Thanks! These 2 errors (reversing the loop condition and forgetting to reset size before the loop and increase inside the loop) were quite embarassing. Now it works perfectly. The while are there because for some reason, if I use 'for', the console spits a warning saying 'You need to compile in C99 mode'. I obviously can add -std=c99 to the end, but I preferred to just use whiles instead (I don't like warnings). Thanks again!
– Beroun
Jan 5 at 22:01
@CraigEstey it’s probably a punishment down vote. Someone’s way of saying don’t answer out of scope questions. Personally if people want to put in the effort to answers these question, more power to them.
– Paul Rooney
Jan 7 at 22:05
@PaulRooney Thanks. The DV came early (before the update section). I suspect it was punishment--from the "don't answer homework questions" camp [which I'm not a member of]. IMO, when a question is so far away, merely "hints" aren't going to help an OP much. Normally, I try to remain [somewhat] faithful to an OP's code, give an explanation of errors, annotate the code with the bugs [and fixes] and sometimes present a second cleaned up version. A number of OPs like this approach. And, here, OP was able to take my version as a guide to fixing his own code [just what I had hoped for].
– Craig Estey
Jan 7 at 22:32
add a comment |
Why the DV? The guidelines for DV on SO are an answer that is egregiously wrong.
– Craig Estey
Jan 4 at 0:17
First of all, thanks a lot for your work. Looking at your code (I cannot call it anymore my code as it's completely different) I see that the findSize function is mostly irrelevant. I tried to put another fscanf instead of calling a findSize function, but I don't know how this function works exactly. Let me see if I get it: If there is a third argument, it stores each read element into the third argument (which should be a variable). But if there is no third element, and you put an * in the second argument, it doesn't store the read elements anywhere. Am I right? (continuation below)
– Beroun
Jan 5 at 17:27
Thanks! These 2 errors (reversing the loop condition and forgetting to reset size before the loop and increase inside the loop) were quite embarassing. Now it works perfectly. The while are there because for some reason, if I use 'for', the console spits a warning saying 'You need to compile in C99 mode'. I obviously can add -std=c99 to the end, but I preferred to just use whiles instead (I don't like warnings). Thanks again!
– Beroun
Jan 5 at 22:01
@CraigEstey it’s probably a punishment down vote. Someone’s way of saying don’t answer out of scope questions. Personally if people want to put in the effort to answers these question, more power to them.
– Paul Rooney
Jan 7 at 22:05
@PaulRooney Thanks. The DV came early (before the update section). I suspect it was punishment--from the "don't answer homework questions" camp [which I'm not a member of]. IMO, when a question is so far away, merely "hints" aren't going to help an OP much. Normally, I try to remain [somewhat] faithful to an OP's code, give an explanation of errors, annotate the code with the bugs [and fixes] and sometimes present a second cleaned up version. A number of OPs like this approach. And, here, OP was able to take my version as a guide to fixing his own code [just what I had hoped for].
– Craig Estey
Jan 7 at 22:32
Why the DV? The guidelines for DV on SO are an answer that is egregiously wrong.
– Craig Estey
Jan 4 at 0:17
Why the DV? The guidelines for DV on SO are an answer that is egregiously wrong.
– Craig Estey
Jan 4 at 0:17
First of all, thanks a lot for your work. Looking at your code (I cannot call it anymore my code as it's completely different) I see that the findSize function is mostly irrelevant. I tried to put another fscanf instead of calling a findSize function, but I don't know how this function works exactly. Let me see if I get it: If there is a third argument, it stores each read element into the third argument (which should be a variable). But if there is no third element, and you put an * in the second argument, it doesn't store the read elements anywhere. Am I right? (continuation below)
– Beroun
Jan 5 at 17:27
First of all, thanks a lot for your work. Looking at your code (I cannot call it anymore my code as it's completely different) I see that the findSize function is mostly irrelevant. I tried to put another fscanf instead of calling a findSize function, but I don't know how this function works exactly. Let me see if I get it: If there is a third argument, it stores each read element into the third argument (which should be a variable). But if there is no third element, and you put an * in the second argument, it doesn't store the read elements anywhere. Am I right? (continuation below)
– Beroun
Jan 5 at 17:27
Thanks! These 2 errors (reversing the loop condition and forgetting to reset size before the loop and increase inside the loop) were quite embarassing. Now it works perfectly. The while are there because for some reason, if I use 'for', the console spits a warning saying 'You need to compile in C99 mode'. I obviously can add -std=c99 to the end, but I preferred to just use whiles instead (I don't like warnings). Thanks again!
– Beroun
Jan 5 at 22:01
Thanks! These 2 errors (reversing the loop condition and forgetting to reset size before the loop and increase inside the loop) were quite embarassing. Now it works perfectly. The while are there because for some reason, if I use 'for', the console spits a warning saying 'You need to compile in C99 mode'. I obviously can add -std=c99 to the end, but I preferred to just use whiles instead (I don't like warnings). Thanks again!
– Beroun
Jan 5 at 22:01
@CraigEstey it’s probably a punishment down vote. Someone’s way of saying don’t answer out of scope questions. Personally if people want to put in the effort to answers these question, more power to them.
– Paul Rooney
Jan 7 at 22:05
@CraigEstey it’s probably a punishment down vote. Someone’s way of saying don’t answer out of scope questions. Personally if people want to put in the effort to answers these question, more power to them.
– Paul Rooney
Jan 7 at 22:05
@PaulRooney Thanks. The DV came early (before the update section). I suspect it was punishment--from the "don't answer homework questions" camp [which I'm not a member of]. IMO, when a question is so far away, merely "hints" aren't going to help an OP much. Normally, I try to remain [somewhat] faithful to an OP's code, give an explanation of errors, annotate the code with the bugs [and fixes] and sometimes present a second cleaned up version. A number of OPs like this approach. And, here, OP was able to take my version as a guide to fixing his own code [just what I had hoped for].
– Craig Estey
Jan 7 at 22:32
@PaulRooney Thanks. The DV came early (before the update section). I suspect it was punishment--from the "don't answer homework questions" camp [which I'm not a member of]. IMO, when a question is so far away, merely "hints" aren't going to help an OP much. Normally, I try to remain [somewhat] faithful to an OP's code, give an explanation of errors, annotate the code with the bugs [and fixes] and sometimes present a second cleaned up version. A number of OPs like this approach. And, here, OP was able to take my version as a guide to fixing his own code [just what I had hoped for].
– Craig Estey
Jan 7 at 22:32
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54030811%2fwhy-isnt-my-code-reading-the-correct-integers-from-a-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
q8,Nn DlzVqvS8T9
6
void main (char *filename)
isn’t a correct signature formain
– you should have learnedint main(int argc, char* argv)
. Might also want to read How to Ask– Ry-♦
Jan 3 at 22:45
1
Ry- found [at least] your initial problem. Define
main
asint main(int argc,char **argv)
and then dofopen(argv[1],"r")
. As you've definedmain
, yourfilename
pointer will be given [silently] a value of 0x02 [or some other worse/garbage value] which causes a segfault due to an invalid pointer dereference.– Craig Estey
Jan 3 at 22:51
1
You should edit your question and post your sample input file in a separate code block. This allows us to download, compile, link, and run your program with enough input to reproduce your problem [beyond the fix for the segfault]. Note that you should do:
char *filename = argv[1];
to handle all places wherefilename
is used. Note that this is a quick fix because it doesn't handle the case where the program argument is omitted (e.g.argc
is 1 andargv[1] == NULL
)– Craig Estey
Jan 3 at 22:54
5
Turn on compiler warnings and use a debugger.
– Broman
Jan 3 at 23:02
1
numbers[idx] = (int *) p_data;
perhaps you meantnumbers[idx] = *(int *) p_data;
? The compiler will not compile the original statement.– Paul Rooney
Jan 3 at 23:09