How to assign roles to users using the website interface in ASP.NET Core 2.1
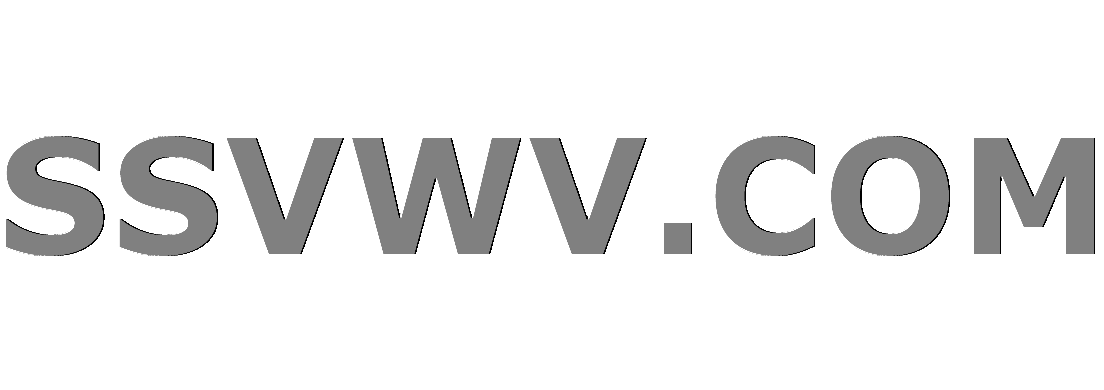
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I'm trying to work out how to assign roles to users in an admin interface of my web application using ASP.Net core 2.1.
I haven't come across an answer yet. My ID for application user is string-based not an integer.
Currently I am able to edit users, add new users, add new roles delete roles, edit role names, but am unable to assign roles to users.
Ideally what I am looking to do is have a view, that has two drop-down lists. One with all users in it and a list of the available roles that I can assign.
Does anyone have any tips on how to achieve this please?
Here's my current Roles controller. For context, I am using a repository pattern. And have implemented a few of the identity models, ApplicationUser, ApplicationUserRole and Application Role.
Roles controller.cs:
[Authorize(Roles = "Admin")]
public class RolesController : Controller
{
private readonly UserManager<ApplicationUser> _userManager;
private readonly RoleManager<ApplicationRole> _roleManager;
private IRepository _repo;
private readonly ApplicationDbContext _context;
public RolesController(UserManager<ApplicationUser> userManager,
RoleManager<ApplicationRole> roleManager, IRepository repo, ApplicationDbContext context)
{
_userManager = userManager;
_roleManager = roleManager;
_repo = repo;
_context = context;
}
public IActionResult Index()
{
List<RoleListViewModel> model = new List<RoleListViewModel>();
model = _roleManager.Roles.Select(r => new RoleListViewModel
{
RoleName = r.Name,
Description = r.Description,
Id = r.Id,
NumberOfUsers = r.UserRoles.Count
}).ToList();
return View(model);
}
[AutoValidateAntiforgeryToken]
public ActionResult Details(string id)
{
var role = _repo.GetRole((string)id);
if (role == null)
{
return RedirectToAction("Index");
}
return View(role);
}
[HttpGet]
public IActionResult Create()
{
return View();
}
[AutoValidateAntiforgeryToken]
[HttpPost]
public async Task<IActionResult> Create(RoleViewModel vm)
{
if (!ModelState.IsValid)
return View(vm);
{
var role = new ApplicationRole
{ Name = vm.Name };
var result = await _roleManager.CreateAsync(role);
if (result.Succeeded)
{
_repo.AddRole(role);
return RedirectToAction("Index");
}
else
foreach (var error in result.Errors)
{
ModelState.AddModelError("", error.Description);
}
return View(vm);
}
}
[HttpGet]
public ActionResult Delete(string Id)
{
var role = _context.Roles.Find(Id);
if (role == null)
{
return RedirectToAction("Index");
}
return View(role);
}
[ValidateAntiForgeryToken]
[HttpPost]
public async Task<ActionResult> Delete([Bind(include: "Id,Name")]ApplicationRole myRole)
{
ApplicationRole role = _context.Roles.Find(myRole.Id);
_context.Roles.Remove(role);
await _context.SaveChangesAsync();
return RedirectToAction("Index");
}
[HttpGet]
public IActionResult Edit(string Id)
{
var role = _repo.GetRole((string)Id);
if (role == null)
{
return RedirectToAction("Index");
}
return View(new RoleViewModel { Id = role.Id, Name = role.Name, Description = role.Description });
}
[HttpPost]
public async Task<IActionResult> Edit(RoleViewModel vm)
{
var role = await _roleManager.FindByIdAsync(vm.Id);
if (vm.Name != role.Name)
{
role.Name = vm.Name;
}
if(vm.Description != role.Description)
{
role.Description = vm.Description;
}
var result = _roleManager.UpdateAsync(role).Result;
if (result.Succeeded)
{
return RedirectToAction("Index", "Roles");
}
else return View(vm);
}
//[HttpGet]
//public async Task<IActionResult> AssignRole(string Id)
//{
// List<UserRolesViewModel> model = new List<UserRolesViewModel>();
// model = _userManager.Users.Select(r => new UserRolesViewModel
// {
// Email = u.Email,
// Description = r.Description,
// Id = r.Id,
// NumberOfUsers = r.UserRoles.Count
// }).ToList();
// return View(model);
//}`
ApplicationUser.cs:
public class ApplicationUser : IdentityUser
{
public string FirstName { get; internal set; }
public string LastName { get; internal set; }
public virtual ICollection<IdentityUserClaim<string>> Claims { get; set; }
public virtual ICollection<IdentityUserLogin<string>> Logins { get; set; }
public virtual ICollection<IdentityUserToken<string>> Tokens { get; set; }
public virtual IEnumerable<ApplicationRole> Roles { get; set; }
public ICollection<ApplicationUserRole> UserRoles { get; set; }
public ICollection<MainComment> MainComments { get; set; }
}
ApplicationUserRole.cs
public class ApplicationUserRole : IdentityUserRole<string>
{
public virtual ApplicationUser User { get; set; }
public virtual ApplicationRole Role { get; set; }
}
ApplicationRole.cs
public class ApplicationRole : IdentityRole
{
public ApplicationRole() : base() { }
public ApplicationRole(string name)
: base(name)
{ }
public virtual ICollection<ApplicationUserRole> UserRoles { get; set; }
public string Description { get; set; }
}
c# asp.net-core asp.net-core-identity
add a comment |
I'm trying to work out how to assign roles to users in an admin interface of my web application using ASP.Net core 2.1.
I haven't come across an answer yet. My ID for application user is string-based not an integer.
Currently I am able to edit users, add new users, add new roles delete roles, edit role names, but am unable to assign roles to users.
Ideally what I am looking to do is have a view, that has two drop-down lists. One with all users in it and a list of the available roles that I can assign.
Does anyone have any tips on how to achieve this please?
Here's my current Roles controller. For context, I am using a repository pattern. And have implemented a few of the identity models, ApplicationUser, ApplicationUserRole and Application Role.
Roles controller.cs:
[Authorize(Roles = "Admin")]
public class RolesController : Controller
{
private readonly UserManager<ApplicationUser> _userManager;
private readonly RoleManager<ApplicationRole> _roleManager;
private IRepository _repo;
private readonly ApplicationDbContext _context;
public RolesController(UserManager<ApplicationUser> userManager,
RoleManager<ApplicationRole> roleManager, IRepository repo, ApplicationDbContext context)
{
_userManager = userManager;
_roleManager = roleManager;
_repo = repo;
_context = context;
}
public IActionResult Index()
{
List<RoleListViewModel> model = new List<RoleListViewModel>();
model = _roleManager.Roles.Select(r => new RoleListViewModel
{
RoleName = r.Name,
Description = r.Description,
Id = r.Id,
NumberOfUsers = r.UserRoles.Count
}).ToList();
return View(model);
}
[AutoValidateAntiforgeryToken]
public ActionResult Details(string id)
{
var role = _repo.GetRole((string)id);
if (role == null)
{
return RedirectToAction("Index");
}
return View(role);
}
[HttpGet]
public IActionResult Create()
{
return View();
}
[AutoValidateAntiforgeryToken]
[HttpPost]
public async Task<IActionResult> Create(RoleViewModel vm)
{
if (!ModelState.IsValid)
return View(vm);
{
var role = new ApplicationRole
{ Name = vm.Name };
var result = await _roleManager.CreateAsync(role);
if (result.Succeeded)
{
_repo.AddRole(role);
return RedirectToAction("Index");
}
else
foreach (var error in result.Errors)
{
ModelState.AddModelError("", error.Description);
}
return View(vm);
}
}
[HttpGet]
public ActionResult Delete(string Id)
{
var role = _context.Roles.Find(Id);
if (role == null)
{
return RedirectToAction("Index");
}
return View(role);
}
[ValidateAntiForgeryToken]
[HttpPost]
public async Task<ActionResult> Delete([Bind(include: "Id,Name")]ApplicationRole myRole)
{
ApplicationRole role = _context.Roles.Find(myRole.Id);
_context.Roles.Remove(role);
await _context.SaveChangesAsync();
return RedirectToAction("Index");
}
[HttpGet]
public IActionResult Edit(string Id)
{
var role = _repo.GetRole((string)Id);
if (role == null)
{
return RedirectToAction("Index");
}
return View(new RoleViewModel { Id = role.Id, Name = role.Name, Description = role.Description });
}
[HttpPost]
public async Task<IActionResult> Edit(RoleViewModel vm)
{
var role = await _roleManager.FindByIdAsync(vm.Id);
if (vm.Name != role.Name)
{
role.Name = vm.Name;
}
if(vm.Description != role.Description)
{
role.Description = vm.Description;
}
var result = _roleManager.UpdateAsync(role).Result;
if (result.Succeeded)
{
return RedirectToAction("Index", "Roles");
}
else return View(vm);
}
//[HttpGet]
//public async Task<IActionResult> AssignRole(string Id)
//{
// List<UserRolesViewModel> model = new List<UserRolesViewModel>();
// model = _userManager.Users.Select(r => new UserRolesViewModel
// {
// Email = u.Email,
// Description = r.Description,
// Id = r.Id,
// NumberOfUsers = r.UserRoles.Count
// }).ToList();
// return View(model);
//}`
ApplicationUser.cs:
public class ApplicationUser : IdentityUser
{
public string FirstName { get; internal set; }
public string LastName { get; internal set; }
public virtual ICollection<IdentityUserClaim<string>> Claims { get; set; }
public virtual ICollection<IdentityUserLogin<string>> Logins { get; set; }
public virtual ICollection<IdentityUserToken<string>> Tokens { get; set; }
public virtual IEnumerable<ApplicationRole> Roles { get; set; }
public ICollection<ApplicationUserRole> UserRoles { get; set; }
public ICollection<MainComment> MainComments { get; set; }
}
ApplicationUserRole.cs
public class ApplicationUserRole : IdentityUserRole<string>
{
public virtual ApplicationUser User { get; set; }
public virtual ApplicationRole Role { get; set; }
}
ApplicationRole.cs
public class ApplicationRole : IdentityRole
{
public ApplicationRole() : base() { }
public ApplicationRole(string name)
: base(name)
{ }
public virtual ICollection<ApplicationUserRole> UserRoles { get; set; }
public string Description { get; set; }
}
c# asp.net-core asp.net-core-identity
Code is formatted by prefixing with 4 spaces. Looks like you tried to use a right angle bracket, if you want to clean that up.
– ToothlessRebel
Jan 3 at 22:49
Maybe some more information regarding your code and your code structure would be helpful for us. :)
– MF DOOM
Jan 4 at 0:24
add a comment |
I'm trying to work out how to assign roles to users in an admin interface of my web application using ASP.Net core 2.1.
I haven't come across an answer yet. My ID for application user is string-based not an integer.
Currently I am able to edit users, add new users, add new roles delete roles, edit role names, but am unable to assign roles to users.
Ideally what I am looking to do is have a view, that has two drop-down lists. One with all users in it and a list of the available roles that I can assign.
Does anyone have any tips on how to achieve this please?
Here's my current Roles controller. For context, I am using a repository pattern. And have implemented a few of the identity models, ApplicationUser, ApplicationUserRole and Application Role.
Roles controller.cs:
[Authorize(Roles = "Admin")]
public class RolesController : Controller
{
private readonly UserManager<ApplicationUser> _userManager;
private readonly RoleManager<ApplicationRole> _roleManager;
private IRepository _repo;
private readonly ApplicationDbContext _context;
public RolesController(UserManager<ApplicationUser> userManager,
RoleManager<ApplicationRole> roleManager, IRepository repo, ApplicationDbContext context)
{
_userManager = userManager;
_roleManager = roleManager;
_repo = repo;
_context = context;
}
public IActionResult Index()
{
List<RoleListViewModel> model = new List<RoleListViewModel>();
model = _roleManager.Roles.Select(r => new RoleListViewModel
{
RoleName = r.Name,
Description = r.Description,
Id = r.Id,
NumberOfUsers = r.UserRoles.Count
}).ToList();
return View(model);
}
[AutoValidateAntiforgeryToken]
public ActionResult Details(string id)
{
var role = _repo.GetRole((string)id);
if (role == null)
{
return RedirectToAction("Index");
}
return View(role);
}
[HttpGet]
public IActionResult Create()
{
return View();
}
[AutoValidateAntiforgeryToken]
[HttpPost]
public async Task<IActionResult> Create(RoleViewModel vm)
{
if (!ModelState.IsValid)
return View(vm);
{
var role = new ApplicationRole
{ Name = vm.Name };
var result = await _roleManager.CreateAsync(role);
if (result.Succeeded)
{
_repo.AddRole(role);
return RedirectToAction("Index");
}
else
foreach (var error in result.Errors)
{
ModelState.AddModelError("", error.Description);
}
return View(vm);
}
}
[HttpGet]
public ActionResult Delete(string Id)
{
var role = _context.Roles.Find(Id);
if (role == null)
{
return RedirectToAction("Index");
}
return View(role);
}
[ValidateAntiForgeryToken]
[HttpPost]
public async Task<ActionResult> Delete([Bind(include: "Id,Name")]ApplicationRole myRole)
{
ApplicationRole role = _context.Roles.Find(myRole.Id);
_context.Roles.Remove(role);
await _context.SaveChangesAsync();
return RedirectToAction("Index");
}
[HttpGet]
public IActionResult Edit(string Id)
{
var role = _repo.GetRole((string)Id);
if (role == null)
{
return RedirectToAction("Index");
}
return View(new RoleViewModel { Id = role.Id, Name = role.Name, Description = role.Description });
}
[HttpPost]
public async Task<IActionResult> Edit(RoleViewModel vm)
{
var role = await _roleManager.FindByIdAsync(vm.Id);
if (vm.Name != role.Name)
{
role.Name = vm.Name;
}
if(vm.Description != role.Description)
{
role.Description = vm.Description;
}
var result = _roleManager.UpdateAsync(role).Result;
if (result.Succeeded)
{
return RedirectToAction("Index", "Roles");
}
else return View(vm);
}
//[HttpGet]
//public async Task<IActionResult> AssignRole(string Id)
//{
// List<UserRolesViewModel> model = new List<UserRolesViewModel>();
// model = _userManager.Users.Select(r => new UserRolesViewModel
// {
// Email = u.Email,
// Description = r.Description,
// Id = r.Id,
// NumberOfUsers = r.UserRoles.Count
// }).ToList();
// return View(model);
//}`
ApplicationUser.cs:
public class ApplicationUser : IdentityUser
{
public string FirstName { get; internal set; }
public string LastName { get; internal set; }
public virtual ICollection<IdentityUserClaim<string>> Claims { get; set; }
public virtual ICollection<IdentityUserLogin<string>> Logins { get; set; }
public virtual ICollection<IdentityUserToken<string>> Tokens { get; set; }
public virtual IEnumerable<ApplicationRole> Roles { get; set; }
public ICollection<ApplicationUserRole> UserRoles { get; set; }
public ICollection<MainComment> MainComments { get; set; }
}
ApplicationUserRole.cs
public class ApplicationUserRole : IdentityUserRole<string>
{
public virtual ApplicationUser User { get; set; }
public virtual ApplicationRole Role { get; set; }
}
ApplicationRole.cs
public class ApplicationRole : IdentityRole
{
public ApplicationRole() : base() { }
public ApplicationRole(string name)
: base(name)
{ }
public virtual ICollection<ApplicationUserRole> UserRoles { get; set; }
public string Description { get; set; }
}
c# asp.net-core asp.net-core-identity
I'm trying to work out how to assign roles to users in an admin interface of my web application using ASP.Net core 2.1.
I haven't come across an answer yet. My ID for application user is string-based not an integer.
Currently I am able to edit users, add new users, add new roles delete roles, edit role names, but am unable to assign roles to users.
Ideally what I am looking to do is have a view, that has two drop-down lists. One with all users in it and a list of the available roles that I can assign.
Does anyone have any tips on how to achieve this please?
Here's my current Roles controller. For context, I am using a repository pattern. And have implemented a few of the identity models, ApplicationUser, ApplicationUserRole and Application Role.
Roles controller.cs:
[Authorize(Roles = "Admin")]
public class RolesController : Controller
{
private readonly UserManager<ApplicationUser> _userManager;
private readonly RoleManager<ApplicationRole> _roleManager;
private IRepository _repo;
private readonly ApplicationDbContext _context;
public RolesController(UserManager<ApplicationUser> userManager,
RoleManager<ApplicationRole> roleManager, IRepository repo, ApplicationDbContext context)
{
_userManager = userManager;
_roleManager = roleManager;
_repo = repo;
_context = context;
}
public IActionResult Index()
{
List<RoleListViewModel> model = new List<RoleListViewModel>();
model = _roleManager.Roles.Select(r => new RoleListViewModel
{
RoleName = r.Name,
Description = r.Description,
Id = r.Id,
NumberOfUsers = r.UserRoles.Count
}).ToList();
return View(model);
}
[AutoValidateAntiforgeryToken]
public ActionResult Details(string id)
{
var role = _repo.GetRole((string)id);
if (role == null)
{
return RedirectToAction("Index");
}
return View(role);
}
[HttpGet]
public IActionResult Create()
{
return View();
}
[AutoValidateAntiforgeryToken]
[HttpPost]
public async Task<IActionResult> Create(RoleViewModel vm)
{
if (!ModelState.IsValid)
return View(vm);
{
var role = new ApplicationRole
{ Name = vm.Name };
var result = await _roleManager.CreateAsync(role);
if (result.Succeeded)
{
_repo.AddRole(role);
return RedirectToAction("Index");
}
else
foreach (var error in result.Errors)
{
ModelState.AddModelError("", error.Description);
}
return View(vm);
}
}
[HttpGet]
public ActionResult Delete(string Id)
{
var role = _context.Roles.Find(Id);
if (role == null)
{
return RedirectToAction("Index");
}
return View(role);
}
[ValidateAntiForgeryToken]
[HttpPost]
public async Task<ActionResult> Delete([Bind(include: "Id,Name")]ApplicationRole myRole)
{
ApplicationRole role = _context.Roles.Find(myRole.Id);
_context.Roles.Remove(role);
await _context.SaveChangesAsync();
return RedirectToAction("Index");
}
[HttpGet]
public IActionResult Edit(string Id)
{
var role = _repo.GetRole((string)Id);
if (role == null)
{
return RedirectToAction("Index");
}
return View(new RoleViewModel { Id = role.Id, Name = role.Name, Description = role.Description });
}
[HttpPost]
public async Task<IActionResult> Edit(RoleViewModel vm)
{
var role = await _roleManager.FindByIdAsync(vm.Id);
if (vm.Name != role.Name)
{
role.Name = vm.Name;
}
if(vm.Description != role.Description)
{
role.Description = vm.Description;
}
var result = _roleManager.UpdateAsync(role).Result;
if (result.Succeeded)
{
return RedirectToAction("Index", "Roles");
}
else return View(vm);
}
//[HttpGet]
//public async Task<IActionResult> AssignRole(string Id)
//{
// List<UserRolesViewModel> model = new List<UserRolesViewModel>();
// model = _userManager.Users.Select(r => new UserRolesViewModel
// {
// Email = u.Email,
// Description = r.Description,
// Id = r.Id,
// NumberOfUsers = r.UserRoles.Count
// }).ToList();
// return View(model);
//}`
ApplicationUser.cs:
public class ApplicationUser : IdentityUser
{
public string FirstName { get; internal set; }
public string LastName { get; internal set; }
public virtual ICollection<IdentityUserClaim<string>> Claims { get; set; }
public virtual ICollection<IdentityUserLogin<string>> Logins { get; set; }
public virtual ICollection<IdentityUserToken<string>> Tokens { get; set; }
public virtual IEnumerable<ApplicationRole> Roles { get; set; }
public ICollection<ApplicationUserRole> UserRoles { get; set; }
public ICollection<MainComment> MainComments { get; set; }
}
ApplicationUserRole.cs
public class ApplicationUserRole : IdentityUserRole<string>
{
public virtual ApplicationUser User { get; set; }
public virtual ApplicationRole Role { get; set; }
}
ApplicationRole.cs
public class ApplicationRole : IdentityRole
{
public ApplicationRole() : base() { }
public ApplicationRole(string name)
: base(name)
{ }
public virtual ICollection<ApplicationUserRole> UserRoles { get; set; }
public string Description { get; set; }
}
c# asp.net-core asp.net-core-identity
c# asp.net-core asp.net-core-identity
edited Jan 4 at 8:18
Tasos K.
6,79553150
6,79553150
asked Jan 3 at 22:37


ZakaZaka
32
32
Code is formatted by prefixing with 4 spaces. Looks like you tried to use a right angle bracket, if you want to clean that up.
– ToothlessRebel
Jan 3 at 22:49
Maybe some more information regarding your code and your code structure would be helpful for us. :)
– MF DOOM
Jan 4 at 0:24
add a comment |
Code is formatted by prefixing with 4 spaces. Looks like you tried to use a right angle bracket, if you want to clean that up.
– ToothlessRebel
Jan 3 at 22:49
Maybe some more information regarding your code and your code structure would be helpful for us. :)
– MF DOOM
Jan 4 at 0:24
Code is formatted by prefixing with 4 spaces. Looks like you tried to use a right angle bracket, if you want to clean that up.
– ToothlessRebel
Jan 3 at 22:49
Code is formatted by prefixing with 4 spaces. Looks like you tried to use a right angle bracket, if you want to clean that up.
– ToothlessRebel
Jan 3 at 22:49
Maybe some more information regarding your code and your code structure would be helpful for us. :)
– MF DOOM
Jan 4 at 0:24
Maybe some more information regarding your code and your code structure would be helpful for us. :)
– MF DOOM
Jan 4 at 0:24
add a comment |
2 Answers
2
active
oldest
votes
If you want to assign a role to a user in MVC (tested in asp.net core 2.1), you can do the following. I have also created a user here, just to show the injection of the UserManager.
using Microsoft.AspNetCore.Identity;
using Microsoft.AspNetCore.Mvc;
using System.Threading.Tasks;
namespace MyApp.Controllers
{
public class RolesController : Controller
{
private readonly RoleManager<IdentityRole> _roleManager;
private readonly UserManager<IdentityUser> _userManager;
public RolesController(RoleManager<IdentityRole> roleManager, UserManager<IdentityUser> userManager)
{
_roleManager = roleManager;
_userManager = userManager;
}
[HttpPost]
public async Task<IActionResult> AssignRoleToUser(string _roleName, string _userName)
{
//Created a user
var user = new IdentityUser { UserName = _userName, Email = "xyz@somedomain.tld" };
var result = await _userManager.CreateAsync(user, "[SomePassword]");
if (result.Succeeded)
{
// assign an existing role to the newly created user
await _userManager.AddToRoleAsync(user, "Admin");
}
return View();
}
}
}
add a comment |
By no means this is the proper way of doing this or anything.
I made a role assigner not so long ago and this is what i came up with.
Also the "Admin" role cannot be assigned. It can be simply commented out/removed. and the class:
HomebreModel
only contains strings
The Assign view shows two dropdowns one for the user and another one for the role.
The Controller
[Authorize(AuthenticationSchemes = HomebrewModel.BothAuthSchemes, Roles = HomebrewModel.RoleAdmin)]
public class RoleController : Controller
{
private readonly RoleManager<IdentityRole> _roleManager;
private readonly UserManager<NutricionUser> _userManager;
public RoleController(RoleManager<IdentityRole> roleManager, UserManager<NutricionUser> userManager)
{
_roleManager = roleManager;
_userManager = userManager;
}
// GET: Role
public async Task<ActionResult> Index()
{
var adminRole = await _roleManager.FindByNameAsync(HomebrewModel.RoleAdmin);
var assignableRoles = _roleManager.Roles.ToList();
assignableRoles.RemoveAt(assignableRoles.IndexOf(adminRole));
return View(assignableRoles);
}
// GET: Role/Assign
public async Task<ActionResult> Assign()
{
var adminRole = await _roleManager.FindByNameAsync(HomebrewModel.RoleAdmin);
var assignableRoles = _roleManager.Roles.ToList();
assignableRoles.RemoveAt(assignableRoles.IndexOf(adminRole));
ViewData["Name"] = new SelectList(assignableRoles, "Name", "Name");
ViewData["UserName"] = new SelectList(_userManager.Users, "UserName", "UserName");
return View(new RoleModel());
}
// POST: Role/Assign
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<ActionResult> Assign(RoleModel roleModel)
{
if (ModelState.IsValid)
{
if(roleModel.Name == HomebrewModel.RoleAdmin)
{
ViewData["Message"] = "Invalid Request.";
return View("Info");
}
var user = await _userManager.FindByEmailAsync(roleModel.UserName);
if (user != null)
{
if (await _roleManager.RoleExistsAsync(roleModel.Name))
{
if(await _userManager.IsInRoleAsync(user, roleModel.Name))
{
ViewData["Message"] = $@"User {roleModel.UserName} already has the {roleModel.Name} role.";
return View("Info");
}
else
{
await _userManager.AddToRoleAsync(user, roleModel.Name);
ViewData["Message"] = $@"User {roleModel.UserName} was assigned the {roleModel.Name} role.";
return View("Info");
}
}
else
{
ViewData["Message"] = "Invalid Request.";
return View("Info");
}
}
else
{
ViewData["Message"] = "Invalid Request.";
return View("Info");
}
}
return View(roleModel);
}
}
And these are the views.
Index
@{
ViewData["Title"] = "Roles";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Roles</h2>
<p>
<a asp-action="Assign">Assign</a>
</p>
<table class="table">
<thead>
<tr>
<th>
Roles
</th>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<td>
@item.Name
</td>
</tr>
}
</tbody>
</table>
Assign
@model Models.RoleModel
@{
ViewData["Title"] = "Assign";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Assign</h2>
<hr />
<div class="row">
<div class="col-md-4">
<form asp-action="Assign">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="Name" class="control-label"></label>
<select asp-for="Name" asp-items="(SelectList)@ViewData["Name"]"></select>
</div>
<div class="form-group">
<label asp-for="UserName" class="control-label"></label>
<select asp-for="UserName" asp-items="(SelectList)@ViewData["UserName"]"></select>
</div>
<div class="form-group">
<input type="submit" value="Assign" class="btn btn-default" />
</div>
</form>
</div>
</div>
<div>
<a asp-action="Index">Back to list.</a>
</div>
And this is the RoleModel class
public class RoleModel
{
[Display(Name = "Name")]
public string Name { get; set; }
[Display(Name = "UserName")]
public string UserName { get; set; }
}
The Info view as well
@{
ViewData["Title"] = "Info";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Info</h2>
<h3>@ViewData["Message"]</h3>
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54030762%2fhow-to-assign-roles-to-users-using-the-website-interface-in-asp-net-core-2-1%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you want to assign a role to a user in MVC (tested in asp.net core 2.1), you can do the following. I have also created a user here, just to show the injection of the UserManager.
using Microsoft.AspNetCore.Identity;
using Microsoft.AspNetCore.Mvc;
using System.Threading.Tasks;
namespace MyApp.Controllers
{
public class RolesController : Controller
{
private readonly RoleManager<IdentityRole> _roleManager;
private readonly UserManager<IdentityUser> _userManager;
public RolesController(RoleManager<IdentityRole> roleManager, UserManager<IdentityUser> userManager)
{
_roleManager = roleManager;
_userManager = userManager;
}
[HttpPost]
public async Task<IActionResult> AssignRoleToUser(string _roleName, string _userName)
{
//Created a user
var user = new IdentityUser { UserName = _userName, Email = "xyz@somedomain.tld" };
var result = await _userManager.CreateAsync(user, "[SomePassword]");
if (result.Succeeded)
{
// assign an existing role to the newly created user
await _userManager.AddToRoleAsync(user, "Admin");
}
return View();
}
}
}
add a comment |
If you want to assign a role to a user in MVC (tested in asp.net core 2.1), you can do the following. I have also created a user here, just to show the injection of the UserManager.
using Microsoft.AspNetCore.Identity;
using Microsoft.AspNetCore.Mvc;
using System.Threading.Tasks;
namespace MyApp.Controllers
{
public class RolesController : Controller
{
private readonly RoleManager<IdentityRole> _roleManager;
private readonly UserManager<IdentityUser> _userManager;
public RolesController(RoleManager<IdentityRole> roleManager, UserManager<IdentityUser> userManager)
{
_roleManager = roleManager;
_userManager = userManager;
}
[HttpPost]
public async Task<IActionResult> AssignRoleToUser(string _roleName, string _userName)
{
//Created a user
var user = new IdentityUser { UserName = _userName, Email = "xyz@somedomain.tld" };
var result = await _userManager.CreateAsync(user, "[SomePassword]");
if (result.Succeeded)
{
// assign an existing role to the newly created user
await _userManager.AddToRoleAsync(user, "Admin");
}
return View();
}
}
}
add a comment |
If you want to assign a role to a user in MVC (tested in asp.net core 2.1), you can do the following. I have also created a user here, just to show the injection of the UserManager.
using Microsoft.AspNetCore.Identity;
using Microsoft.AspNetCore.Mvc;
using System.Threading.Tasks;
namespace MyApp.Controllers
{
public class RolesController : Controller
{
private readonly RoleManager<IdentityRole> _roleManager;
private readonly UserManager<IdentityUser> _userManager;
public RolesController(RoleManager<IdentityRole> roleManager, UserManager<IdentityUser> userManager)
{
_roleManager = roleManager;
_userManager = userManager;
}
[HttpPost]
public async Task<IActionResult> AssignRoleToUser(string _roleName, string _userName)
{
//Created a user
var user = new IdentityUser { UserName = _userName, Email = "xyz@somedomain.tld" };
var result = await _userManager.CreateAsync(user, "[SomePassword]");
if (result.Succeeded)
{
// assign an existing role to the newly created user
await _userManager.AddToRoleAsync(user, "Admin");
}
return View();
}
}
}
If you want to assign a role to a user in MVC (tested in asp.net core 2.1), you can do the following. I have also created a user here, just to show the injection of the UserManager.
using Microsoft.AspNetCore.Identity;
using Microsoft.AspNetCore.Mvc;
using System.Threading.Tasks;
namespace MyApp.Controllers
{
public class RolesController : Controller
{
private readonly RoleManager<IdentityRole> _roleManager;
private readonly UserManager<IdentityUser> _userManager;
public RolesController(RoleManager<IdentityRole> roleManager, UserManager<IdentityUser> userManager)
{
_roleManager = roleManager;
_userManager = userManager;
}
[HttpPost]
public async Task<IActionResult> AssignRoleToUser(string _roleName, string _userName)
{
//Created a user
var user = new IdentityUser { UserName = _userName, Email = "xyz@somedomain.tld" };
var result = await _userManager.CreateAsync(user, "[SomePassword]");
if (result.Succeeded)
{
// assign an existing role to the newly created user
await _userManager.AddToRoleAsync(user, "Admin");
}
return View();
}
}
}
answered Feb 14 at 7:47


netfednetfed
377513
377513
add a comment |
add a comment |
By no means this is the proper way of doing this or anything.
I made a role assigner not so long ago and this is what i came up with.
Also the "Admin" role cannot be assigned. It can be simply commented out/removed. and the class:
HomebreModel
only contains strings
The Assign view shows two dropdowns one for the user and another one for the role.
The Controller
[Authorize(AuthenticationSchemes = HomebrewModel.BothAuthSchemes, Roles = HomebrewModel.RoleAdmin)]
public class RoleController : Controller
{
private readonly RoleManager<IdentityRole> _roleManager;
private readonly UserManager<NutricionUser> _userManager;
public RoleController(RoleManager<IdentityRole> roleManager, UserManager<NutricionUser> userManager)
{
_roleManager = roleManager;
_userManager = userManager;
}
// GET: Role
public async Task<ActionResult> Index()
{
var adminRole = await _roleManager.FindByNameAsync(HomebrewModel.RoleAdmin);
var assignableRoles = _roleManager.Roles.ToList();
assignableRoles.RemoveAt(assignableRoles.IndexOf(adminRole));
return View(assignableRoles);
}
// GET: Role/Assign
public async Task<ActionResult> Assign()
{
var adminRole = await _roleManager.FindByNameAsync(HomebrewModel.RoleAdmin);
var assignableRoles = _roleManager.Roles.ToList();
assignableRoles.RemoveAt(assignableRoles.IndexOf(adminRole));
ViewData["Name"] = new SelectList(assignableRoles, "Name", "Name");
ViewData["UserName"] = new SelectList(_userManager.Users, "UserName", "UserName");
return View(new RoleModel());
}
// POST: Role/Assign
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<ActionResult> Assign(RoleModel roleModel)
{
if (ModelState.IsValid)
{
if(roleModel.Name == HomebrewModel.RoleAdmin)
{
ViewData["Message"] = "Invalid Request.";
return View("Info");
}
var user = await _userManager.FindByEmailAsync(roleModel.UserName);
if (user != null)
{
if (await _roleManager.RoleExistsAsync(roleModel.Name))
{
if(await _userManager.IsInRoleAsync(user, roleModel.Name))
{
ViewData["Message"] = $@"User {roleModel.UserName} already has the {roleModel.Name} role.";
return View("Info");
}
else
{
await _userManager.AddToRoleAsync(user, roleModel.Name);
ViewData["Message"] = $@"User {roleModel.UserName} was assigned the {roleModel.Name} role.";
return View("Info");
}
}
else
{
ViewData["Message"] = "Invalid Request.";
return View("Info");
}
}
else
{
ViewData["Message"] = "Invalid Request.";
return View("Info");
}
}
return View(roleModel);
}
}
And these are the views.
Index
@{
ViewData["Title"] = "Roles";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Roles</h2>
<p>
<a asp-action="Assign">Assign</a>
</p>
<table class="table">
<thead>
<tr>
<th>
Roles
</th>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<td>
@item.Name
</td>
</tr>
}
</tbody>
</table>
Assign
@model Models.RoleModel
@{
ViewData["Title"] = "Assign";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Assign</h2>
<hr />
<div class="row">
<div class="col-md-4">
<form asp-action="Assign">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="Name" class="control-label"></label>
<select asp-for="Name" asp-items="(SelectList)@ViewData["Name"]"></select>
</div>
<div class="form-group">
<label asp-for="UserName" class="control-label"></label>
<select asp-for="UserName" asp-items="(SelectList)@ViewData["UserName"]"></select>
</div>
<div class="form-group">
<input type="submit" value="Assign" class="btn btn-default" />
</div>
</form>
</div>
</div>
<div>
<a asp-action="Index">Back to list.</a>
</div>
And this is the RoleModel class
public class RoleModel
{
[Display(Name = "Name")]
public string Name { get; set; }
[Display(Name = "UserName")]
public string UserName { get; set; }
}
The Info view as well
@{
ViewData["Title"] = "Info";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Info</h2>
<h3>@ViewData["Message"]</h3>
add a comment |
By no means this is the proper way of doing this or anything.
I made a role assigner not so long ago and this is what i came up with.
Also the "Admin" role cannot be assigned. It can be simply commented out/removed. and the class:
HomebreModel
only contains strings
The Assign view shows two dropdowns one for the user and another one for the role.
The Controller
[Authorize(AuthenticationSchemes = HomebrewModel.BothAuthSchemes, Roles = HomebrewModel.RoleAdmin)]
public class RoleController : Controller
{
private readonly RoleManager<IdentityRole> _roleManager;
private readonly UserManager<NutricionUser> _userManager;
public RoleController(RoleManager<IdentityRole> roleManager, UserManager<NutricionUser> userManager)
{
_roleManager = roleManager;
_userManager = userManager;
}
// GET: Role
public async Task<ActionResult> Index()
{
var adminRole = await _roleManager.FindByNameAsync(HomebrewModel.RoleAdmin);
var assignableRoles = _roleManager.Roles.ToList();
assignableRoles.RemoveAt(assignableRoles.IndexOf(adminRole));
return View(assignableRoles);
}
// GET: Role/Assign
public async Task<ActionResult> Assign()
{
var adminRole = await _roleManager.FindByNameAsync(HomebrewModel.RoleAdmin);
var assignableRoles = _roleManager.Roles.ToList();
assignableRoles.RemoveAt(assignableRoles.IndexOf(adminRole));
ViewData["Name"] = new SelectList(assignableRoles, "Name", "Name");
ViewData["UserName"] = new SelectList(_userManager.Users, "UserName", "UserName");
return View(new RoleModel());
}
// POST: Role/Assign
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<ActionResult> Assign(RoleModel roleModel)
{
if (ModelState.IsValid)
{
if(roleModel.Name == HomebrewModel.RoleAdmin)
{
ViewData["Message"] = "Invalid Request.";
return View("Info");
}
var user = await _userManager.FindByEmailAsync(roleModel.UserName);
if (user != null)
{
if (await _roleManager.RoleExistsAsync(roleModel.Name))
{
if(await _userManager.IsInRoleAsync(user, roleModel.Name))
{
ViewData["Message"] = $@"User {roleModel.UserName} already has the {roleModel.Name} role.";
return View("Info");
}
else
{
await _userManager.AddToRoleAsync(user, roleModel.Name);
ViewData["Message"] = $@"User {roleModel.UserName} was assigned the {roleModel.Name} role.";
return View("Info");
}
}
else
{
ViewData["Message"] = "Invalid Request.";
return View("Info");
}
}
else
{
ViewData["Message"] = "Invalid Request.";
return View("Info");
}
}
return View(roleModel);
}
}
And these are the views.
Index
@{
ViewData["Title"] = "Roles";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Roles</h2>
<p>
<a asp-action="Assign">Assign</a>
</p>
<table class="table">
<thead>
<tr>
<th>
Roles
</th>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<td>
@item.Name
</td>
</tr>
}
</tbody>
</table>
Assign
@model Models.RoleModel
@{
ViewData["Title"] = "Assign";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Assign</h2>
<hr />
<div class="row">
<div class="col-md-4">
<form asp-action="Assign">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="Name" class="control-label"></label>
<select asp-for="Name" asp-items="(SelectList)@ViewData["Name"]"></select>
</div>
<div class="form-group">
<label asp-for="UserName" class="control-label"></label>
<select asp-for="UserName" asp-items="(SelectList)@ViewData["UserName"]"></select>
</div>
<div class="form-group">
<input type="submit" value="Assign" class="btn btn-default" />
</div>
</form>
</div>
</div>
<div>
<a asp-action="Index">Back to list.</a>
</div>
And this is the RoleModel class
public class RoleModel
{
[Display(Name = "Name")]
public string Name { get; set; }
[Display(Name = "UserName")]
public string UserName { get; set; }
}
The Info view as well
@{
ViewData["Title"] = "Info";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Info</h2>
<h3>@ViewData["Message"]</h3>
add a comment |
By no means this is the proper way of doing this or anything.
I made a role assigner not so long ago and this is what i came up with.
Also the "Admin" role cannot be assigned. It can be simply commented out/removed. and the class:
HomebreModel
only contains strings
The Assign view shows two dropdowns one for the user and another one for the role.
The Controller
[Authorize(AuthenticationSchemes = HomebrewModel.BothAuthSchemes, Roles = HomebrewModel.RoleAdmin)]
public class RoleController : Controller
{
private readonly RoleManager<IdentityRole> _roleManager;
private readonly UserManager<NutricionUser> _userManager;
public RoleController(RoleManager<IdentityRole> roleManager, UserManager<NutricionUser> userManager)
{
_roleManager = roleManager;
_userManager = userManager;
}
// GET: Role
public async Task<ActionResult> Index()
{
var adminRole = await _roleManager.FindByNameAsync(HomebrewModel.RoleAdmin);
var assignableRoles = _roleManager.Roles.ToList();
assignableRoles.RemoveAt(assignableRoles.IndexOf(adminRole));
return View(assignableRoles);
}
// GET: Role/Assign
public async Task<ActionResult> Assign()
{
var adminRole = await _roleManager.FindByNameAsync(HomebrewModel.RoleAdmin);
var assignableRoles = _roleManager.Roles.ToList();
assignableRoles.RemoveAt(assignableRoles.IndexOf(adminRole));
ViewData["Name"] = new SelectList(assignableRoles, "Name", "Name");
ViewData["UserName"] = new SelectList(_userManager.Users, "UserName", "UserName");
return View(new RoleModel());
}
// POST: Role/Assign
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<ActionResult> Assign(RoleModel roleModel)
{
if (ModelState.IsValid)
{
if(roleModel.Name == HomebrewModel.RoleAdmin)
{
ViewData["Message"] = "Invalid Request.";
return View("Info");
}
var user = await _userManager.FindByEmailAsync(roleModel.UserName);
if (user != null)
{
if (await _roleManager.RoleExistsAsync(roleModel.Name))
{
if(await _userManager.IsInRoleAsync(user, roleModel.Name))
{
ViewData["Message"] = $@"User {roleModel.UserName} already has the {roleModel.Name} role.";
return View("Info");
}
else
{
await _userManager.AddToRoleAsync(user, roleModel.Name);
ViewData["Message"] = $@"User {roleModel.UserName} was assigned the {roleModel.Name} role.";
return View("Info");
}
}
else
{
ViewData["Message"] = "Invalid Request.";
return View("Info");
}
}
else
{
ViewData["Message"] = "Invalid Request.";
return View("Info");
}
}
return View(roleModel);
}
}
And these are the views.
Index
@{
ViewData["Title"] = "Roles";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Roles</h2>
<p>
<a asp-action="Assign">Assign</a>
</p>
<table class="table">
<thead>
<tr>
<th>
Roles
</th>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<td>
@item.Name
</td>
</tr>
}
</tbody>
</table>
Assign
@model Models.RoleModel
@{
ViewData["Title"] = "Assign";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Assign</h2>
<hr />
<div class="row">
<div class="col-md-4">
<form asp-action="Assign">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="Name" class="control-label"></label>
<select asp-for="Name" asp-items="(SelectList)@ViewData["Name"]"></select>
</div>
<div class="form-group">
<label asp-for="UserName" class="control-label"></label>
<select asp-for="UserName" asp-items="(SelectList)@ViewData["UserName"]"></select>
</div>
<div class="form-group">
<input type="submit" value="Assign" class="btn btn-default" />
</div>
</form>
</div>
</div>
<div>
<a asp-action="Index">Back to list.</a>
</div>
And this is the RoleModel class
public class RoleModel
{
[Display(Name = "Name")]
public string Name { get; set; }
[Display(Name = "UserName")]
public string UserName { get; set; }
}
The Info view as well
@{
ViewData["Title"] = "Info";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Info</h2>
<h3>@ViewData["Message"]</h3>
By no means this is the proper way of doing this or anything.
I made a role assigner not so long ago and this is what i came up with.
Also the "Admin" role cannot be assigned. It can be simply commented out/removed. and the class:
HomebreModel
only contains strings
The Assign view shows two dropdowns one for the user and another one for the role.
The Controller
[Authorize(AuthenticationSchemes = HomebrewModel.BothAuthSchemes, Roles = HomebrewModel.RoleAdmin)]
public class RoleController : Controller
{
private readonly RoleManager<IdentityRole> _roleManager;
private readonly UserManager<NutricionUser> _userManager;
public RoleController(RoleManager<IdentityRole> roleManager, UserManager<NutricionUser> userManager)
{
_roleManager = roleManager;
_userManager = userManager;
}
// GET: Role
public async Task<ActionResult> Index()
{
var adminRole = await _roleManager.FindByNameAsync(HomebrewModel.RoleAdmin);
var assignableRoles = _roleManager.Roles.ToList();
assignableRoles.RemoveAt(assignableRoles.IndexOf(adminRole));
return View(assignableRoles);
}
// GET: Role/Assign
public async Task<ActionResult> Assign()
{
var adminRole = await _roleManager.FindByNameAsync(HomebrewModel.RoleAdmin);
var assignableRoles = _roleManager.Roles.ToList();
assignableRoles.RemoveAt(assignableRoles.IndexOf(adminRole));
ViewData["Name"] = new SelectList(assignableRoles, "Name", "Name");
ViewData["UserName"] = new SelectList(_userManager.Users, "UserName", "UserName");
return View(new RoleModel());
}
// POST: Role/Assign
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<ActionResult> Assign(RoleModel roleModel)
{
if (ModelState.IsValid)
{
if(roleModel.Name == HomebrewModel.RoleAdmin)
{
ViewData["Message"] = "Invalid Request.";
return View("Info");
}
var user = await _userManager.FindByEmailAsync(roleModel.UserName);
if (user != null)
{
if (await _roleManager.RoleExistsAsync(roleModel.Name))
{
if(await _userManager.IsInRoleAsync(user, roleModel.Name))
{
ViewData["Message"] = $@"User {roleModel.UserName} already has the {roleModel.Name} role.";
return View("Info");
}
else
{
await _userManager.AddToRoleAsync(user, roleModel.Name);
ViewData["Message"] = $@"User {roleModel.UserName} was assigned the {roleModel.Name} role.";
return View("Info");
}
}
else
{
ViewData["Message"] = "Invalid Request.";
return View("Info");
}
}
else
{
ViewData["Message"] = "Invalid Request.";
return View("Info");
}
}
return View(roleModel);
}
}
And these are the views.
Index
@{
ViewData["Title"] = "Roles";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Roles</h2>
<p>
<a asp-action="Assign">Assign</a>
</p>
<table class="table">
<thead>
<tr>
<th>
Roles
</th>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<td>
@item.Name
</td>
</tr>
}
</tbody>
</table>
Assign
@model Models.RoleModel
@{
ViewData["Title"] = "Assign";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Assign</h2>
<hr />
<div class="row">
<div class="col-md-4">
<form asp-action="Assign">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="Name" class="control-label"></label>
<select asp-for="Name" asp-items="(SelectList)@ViewData["Name"]"></select>
</div>
<div class="form-group">
<label asp-for="UserName" class="control-label"></label>
<select asp-for="UserName" asp-items="(SelectList)@ViewData["UserName"]"></select>
</div>
<div class="form-group">
<input type="submit" value="Assign" class="btn btn-default" />
</div>
</form>
</div>
</div>
<div>
<a asp-action="Index">Back to list.</a>
</div>
And this is the RoleModel class
public class RoleModel
{
[Display(Name = "Name")]
public string Name { get; set; }
[Display(Name = "UserName")]
public string UserName { get; set; }
}
The Info view as well
@{
ViewData["Title"] = "Info";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Info</h2>
<h3>@ViewData["Message"]</h3>
answered Jan 4 at 17:58
SwodniwSSwodniwS
5815
5815
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54030762%2fhow-to-assign-roles-to-users-using-the-website-interface-in-asp-net-core-2-1%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6eHlRz5,b2Dvqq53qEh,jq,E hQg9D5UGZkB H g8K3dv6lNr6UR8Sz3,zBkmx1M OTlcuo2T synOHTu4gtOIT203h2EL
Code is formatted by prefixing with 4 spaces. Looks like you tried to use a right angle bracket, if you want to clean that up.
– ToothlessRebel
Jan 3 at 22:49
Maybe some more information regarding your code and your code structure would be helpful for us. :)
– MF DOOM
Jan 4 at 0:24