How to fix the “Overlapping accesses to 'self', but modification requires exclusive access; consider...
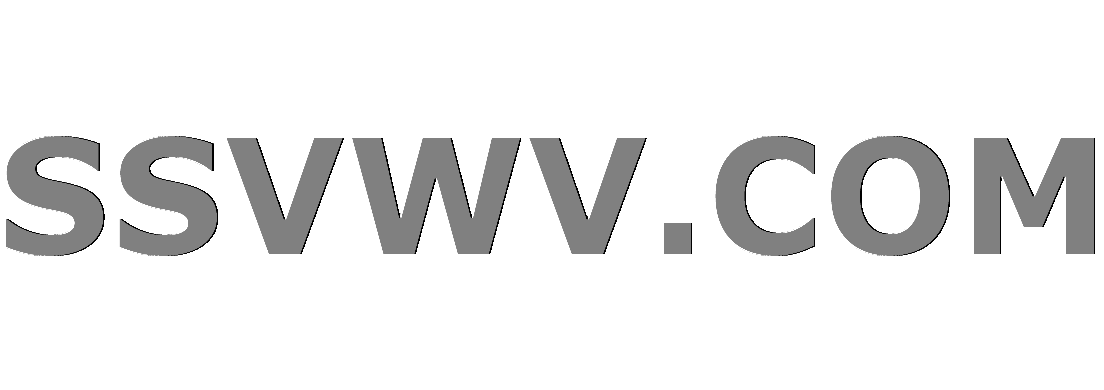
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I can't continue with my app and I can't test run it because something is wrong in my code that I don't know how to fix!
Here is the code:
import Foundation
extension Array {
mutating func shuffle() {
if count < 2 { return }
for i in 0..<(count - 1) {
let j = Int(arc4random_uniform(UInt32(count - i))) + i
customSwap(a: &self[i], b: &self[j])
}
}
}
func customSwap<T>(a:inout T, b:inout T) {
let temp = a
a = b
b = temp
}
ios swift xcode xcode10 swift4.2
add a comment |
I can't continue with my app and I can't test run it because something is wrong in my code that I don't know how to fix!
Here is the code:
import Foundation
extension Array {
mutating func shuffle() {
if count < 2 { return }
for i in 0..<(count - 1) {
let j = Int(arc4random_uniform(UInt32(count - i))) + i
customSwap(a: &self[i], b: &self[j])
}
}
}
func customSwap<T>(a:inout T, b:inout T) {
let temp = a
a = b
b = temp
}
ios swift xcode xcode10 swift4.2
1
Swift 4.2 has a built-in shuffle() method.
– Martin R
Jan 3 at 21:35
1
If you insist on writing your own shuffle, use the built-inswapAt
method:self.swapAt(i, j)
instead of callingcustomSwap
.
– vacawama
Jan 3 at 21:41
Thank a lot!! swapAt fixed the problem!! :)
– Love Örterström
Jan 3 at 21:58
add a comment |
I can't continue with my app and I can't test run it because something is wrong in my code that I don't know how to fix!
Here is the code:
import Foundation
extension Array {
mutating func shuffle() {
if count < 2 { return }
for i in 0..<(count - 1) {
let j = Int(arc4random_uniform(UInt32(count - i))) + i
customSwap(a: &self[i], b: &self[j])
}
}
}
func customSwap<T>(a:inout T, b:inout T) {
let temp = a
a = b
b = temp
}
ios swift xcode xcode10 swift4.2
I can't continue with my app and I can't test run it because something is wrong in my code that I don't know how to fix!
Here is the code:
import Foundation
extension Array {
mutating func shuffle() {
if count < 2 { return }
for i in 0..<(count - 1) {
let j = Int(arc4random_uniform(UInt32(count - i))) + i
customSwap(a: &self[i], b: &self[j])
}
}
}
func customSwap<T>(a:inout T, b:inout T) {
let temp = a
a = b
b = temp
}
ios swift xcode xcode10 swift4.2
ios swift xcode xcode10 swift4.2
asked Jan 3 at 21:32
Love ÖrterströmLove Örterström
62
62
1
Swift 4.2 has a built-in shuffle() method.
– Martin R
Jan 3 at 21:35
1
If you insist on writing your own shuffle, use the built-inswapAt
method:self.swapAt(i, j)
instead of callingcustomSwap
.
– vacawama
Jan 3 at 21:41
Thank a lot!! swapAt fixed the problem!! :)
– Love Örterström
Jan 3 at 21:58
add a comment |
1
Swift 4.2 has a built-in shuffle() method.
– Martin R
Jan 3 at 21:35
1
If you insist on writing your own shuffle, use the built-inswapAt
method:self.swapAt(i, j)
instead of callingcustomSwap
.
– vacawama
Jan 3 at 21:41
Thank a lot!! swapAt fixed the problem!! :)
– Love Örterström
Jan 3 at 21:58
1
1
Swift 4.2 has a built-in shuffle() method.
– Martin R
Jan 3 at 21:35
Swift 4.2 has a built-in shuffle() method.
– Martin R
Jan 3 at 21:35
1
1
If you insist on writing your own shuffle, use the built-in
swapAt
method: self.swapAt(i, j)
instead of calling customSwap
.– vacawama
Jan 3 at 21:41
If you insist on writing your own shuffle, use the built-in
swapAt
method: self.swapAt(i, j)
instead of calling customSwap
.– vacawama
Jan 3 at 21:41
Thank a lot!! swapAt fixed the problem!! :)
– Love Örterström
Jan 3 at 21:58
Thank a lot!! swapAt fixed the problem!! :)
– Love Örterström
Jan 3 at 21:58
add a comment |
1 Answer
1
active
oldest
votes
The problem is that an array is a value type, and when you modify one element you change the whole array. So your call to customSwap()
is passing in two references to the whole array which leads to the overlapping accesses to self error.
Instead, you could write customSwap()
to take one copy of the array and the indices you want to swap:
func customSwap<T>(_ array: inout [T], _ a: Int, _ b: Int) {
let temp = array[a]
array[a] = array[b]
array[b] = temp
}
and then call it like this:
customSwap(&self, i, j)
But you don't have to do that, because Array
has a built-in swapAt(_:_)
defined like this:
mutating func swapAt(_ i: Int, _ j: Int)
So you could replace your customSwap
call with:
self.swapAt(i, j)
But Array
has a built-in shuffle()
that you can just call instead of implementing it yourself.
1
Welcome to Stack Overflow. If this answered your question, please accept it by clicking on the gray checkmark to the left to turn it green.
– vacawama
Jan 4 at 1:31
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54030090%2fhow-to-fix-the-overlapping-accesses-to-self-but-modification-requires-exclus%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The problem is that an array is a value type, and when you modify one element you change the whole array. So your call to customSwap()
is passing in two references to the whole array which leads to the overlapping accesses to self error.
Instead, you could write customSwap()
to take one copy of the array and the indices you want to swap:
func customSwap<T>(_ array: inout [T], _ a: Int, _ b: Int) {
let temp = array[a]
array[a] = array[b]
array[b] = temp
}
and then call it like this:
customSwap(&self, i, j)
But you don't have to do that, because Array
has a built-in swapAt(_:_)
defined like this:
mutating func swapAt(_ i: Int, _ j: Int)
So you could replace your customSwap
call with:
self.swapAt(i, j)
But Array
has a built-in shuffle()
that you can just call instead of implementing it yourself.
1
Welcome to Stack Overflow. If this answered your question, please accept it by clicking on the gray checkmark to the left to turn it green.
– vacawama
Jan 4 at 1:31
add a comment |
The problem is that an array is a value type, and when you modify one element you change the whole array. So your call to customSwap()
is passing in two references to the whole array which leads to the overlapping accesses to self error.
Instead, you could write customSwap()
to take one copy of the array and the indices you want to swap:
func customSwap<T>(_ array: inout [T], _ a: Int, _ b: Int) {
let temp = array[a]
array[a] = array[b]
array[b] = temp
}
and then call it like this:
customSwap(&self, i, j)
But you don't have to do that, because Array
has a built-in swapAt(_:_)
defined like this:
mutating func swapAt(_ i: Int, _ j: Int)
So you could replace your customSwap
call with:
self.swapAt(i, j)
But Array
has a built-in shuffle()
that you can just call instead of implementing it yourself.
1
Welcome to Stack Overflow. If this answered your question, please accept it by clicking on the gray checkmark to the left to turn it green.
– vacawama
Jan 4 at 1:31
add a comment |
The problem is that an array is a value type, and when you modify one element you change the whole array. So your call to customSwap()
is passing in two references to the whole array which leads to the overlapping accesses to self error.
Instead, you could write customSwap()
to take one copy of the array and the indices you want to swap:
func customSwap<T>(_ array: inout [T], _ a: Int, _ b: Int) {
let temp = array[a]
array[a] = array[b]
array[b] = temp
}
and then call it like this:
customSwap(&self, i, j)
But you don't have to do that, because Array
has a built-in swapAt(_:_)
defined like this:
mutating func swapAt(_ i: Int, _ j: Int)
So you could replace your customSwap
call with:
self.swapAt(i, j)
But Array
has a built-in shuffle()
that you can just call instead of implementing it yourself.
The problem is that an array is a value type, and when you modify one element you change the whole array. So your call to customSwap()
is passing in two references to the whole array which leads to the overlapping accesses to self error.
Instead, you could write customSwap()
to take one copy of the array and the indices you want to swap:
func customSwap<T>(_ array: inout [T], _ a: Int, _ b: Int) {
let temp = array[a]
array[a] = array[b]
array[b] = temp
}
and then call it like this:
customSwap(&self, i, j)
But you don't have to do that, because Array
has a built-in swapAt(_:_)
defined like this:
mutating func swapAt(_ i: Int, _ j: Int)
So you could replace your customSwap
call with:
self.swapAt(i, j)
But Array
has a built-in shuffle()
that you can just call instead of implementing it yourself.
edited Jan 3 at 22:34
answered Jan 3 at 22:04


vacawamavacawama
99.7k15178204
99.7k15178204
1
Welcome to Stack Overflow. If this answered your question, please accept it by clicking on the gray checkmark to the left to turn it green.
– vacawama
Jan 4 at 1:31
add a comment |
1
Welcome to Stack Overflow. If this answered your question, please accept it by clicking on the gray checkmark to the left to turn it green.
– vacawama
Jan 4 at 1:31
1
1
Welcome to Stack Overflow. If this answered your question, please accept it by clicking on the gray checkmark to the left to turn it green.
– vacawama
Jan 4 at 1:31
Welcome to Stack Overflow. If this answered your question, please accept it by clicking on the gray checkmark to the left to turn it green.
– vacawama
Jan 4 at 1:31
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54030090%2fhow-to-fix-the-overlapping-accesses-to-self-but-modification-requires-exclus%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hziemyrZKHpsxNs qM,utm7,zD7
1
Swift 4.2 has a built-in shuffle() method.
– Martin R
Jan 3 at 21:35
1
If you insist on writing your own shuffle, use the built-in
swapAt
method:self.swapAt(i, j)
instead of callingcustomSwap
.– vacawama
Jan 3 at 21:41
Thank a lot!! swapAt fixed the problem!! :)
– Love Örterström
Jan 3 at 21:58