Unable to reverse xticks inside matplotlib subplot
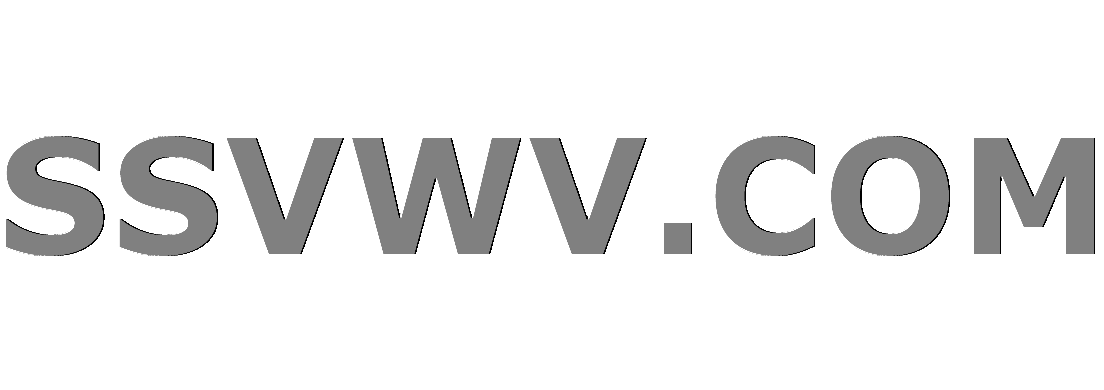
Multi tool use
I have designed a subplot using matplotlib
. I am trying to reverse the xticks
of the plot. Please see the sample code-
import numpy as np
import matplotlib.pyplot as plt
# generate the data
n = 6
y = np.random.randint(low=0, high=10, size=n)
x = np.arange(n)
# generate the ticks and reverse it
xticks = range(n)
xticks.reverse()
# plot the data
plt.figure()
ax = plt.subplot(111)
ax.bar(x, y)
print xticks # prints [5, 4, 3, 2, 1, 0]
ax.set_xticks(xticks)
plt.show()
Please see below the generated plot-
Please pay attention to the xticks
. Even though, ax.set_xticks(xticks)
is used but the xticks
haven't changed. Am I missing some function call to rerender the plot?
Below is the system information-
matplotlib.__version__
'2.1.1'
matplotlib.__version__numpy__
'1.7.1'
python --version
Python 2.7.15rc1
Please note that I just want to reverse the ticks and do not want to invert axis.
python matplotlib
add a comment |
I have designed a subplot using matplotlib
. I am trying to reverse the xticks
of the plot. Please see the sample code-
import numpy as np
import matplotlib.pyplot as plt
# generate the data
n = 6
y = np.random.randint(low=0, high=10, size=n)
x = np.arange(n)
# generate the ticks and reverse it
xticks = range(n)
xticks.reverse()
# plot the data
plt.figure()
ax = plt.subplot(111)
ax.bar(x, y)
print xticks # prints [5, 4, 3, 2, 1, 0]
ax.set_xticks(xticks)
plt.show()
Please see below the generated plot-
Please pay attention to the xticks
. Even though, ax.set_xticks(xticks)
is used but the xticks
haven't changed. Am I missing some function call to rerender the plot?
Below is the system information-
matplotlib.__version__
'2.1.1'
matplotlib.__version__numpy__
'1.7.1'
python --version
Python 2.7.15rc1
Please note that I just want to reverse the ticks and do not want to invert axis.
python matplotlib
add a comment |
I have designed a subplot using matplotlib
. I am trying to reverse the xticks
of the plot. Please see the sample code-
import numpy as np
import matplotlib.pyplot as plt
# generate the data
n = 6
y = np.random.randint(low=0, high=10, size=n)
x = np.arange(n)
# generate the ticks and reverse it
xticks = range(n)
xticks.reverse()
# plot the data
plt.figure()
ax = plt.subplot(111)
ax.bar(x, y)
print xticks # prints [5, 4, 3, 2, 1, 0]
ax.set_xticks(xticks)
plt.show()
Please see below the generated plot-
Please pay attention to the xticks
. Even though, ax.set_xticks(xticks)
is used but the xticks
haven't changed. Am I missing some function call to rerender the plot?
Below is the system information-
matplotlib.__version__
'2.1.1'
matplotlib.__version__numpy__
'1.7.1'
python --version
Python 2.7.15rc1
Please note that I just want to reverse the ticks and do not want to invert axis.
python matplotlib
I have designed a subplot using matplotlib
. I am trying to reverse the xticks
of the plot. Please see the sample code-
import numpy as np
import matplotlib.pyplot as plt
# generate the data
n = 6
y = np.random.randint(low=0, high=10, size=n)
x = np.arange(n)
# generate the ticks and reverse it
xticks = range(n)
xticks.reverse()
# plot the data
plt.figure()
ax = plt.subplot(111)
ax.bar(x, y)
print xticks # prints [5, 4, 3, 2, 1, 0]
ax.set_xticks(xticks)
plt.show()
Please see below the generated plot-
Please pay attention to the xticks
. Even though, ax.set_xticks(xticks)
is used but the xticks
haven't changed. Am I missing some function call to rerender the plot?
Below is the system information-
matplotlib.__version__
'2.1.1'
matplotlib.__version__numpy__
'1.7.1'
python --version
Python 2.7.15rc1
Please note that I just want to reverse the ticks and do not want to invert axis.
python matplotlib
python matplotlib
asked Jan 2 at 11:26
Ravi JoshiRavi Joshi
2,240124895
2,240124895
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
With ax.set_xticks
, you are currently specifying tick positions which is invariant to the order of the list. Either you pass [0, 1, 2, 3, 4, 5]
or you pass [5, 4, 3, 2, 1, 0]
. The difference will not be noticed in the ticks. What you instead want is to have reversed ticklabels for which you should do set_xticklabels(xticks[::-1])
. There are two ways to do it:
Way 1
Use plt.xticks
where the first argument specifies the location of the ticks and the second arguments specifies the respective ticklabels. Specifically, xticks
will provide the tick positions and xticks[::-1]
will label your plot with reversed ticklabels.
xticks = range(n)
# plot the data
plt.figure()
ax = plt.subplot(111)
ax.bar(x, y)
plt.xticks(xticks, xticks[::-1])
Way 2 using ax
where you need set_xticklabels
to get what you want
ax.set_xticks(xticks)
ax.set_xticklabels(xticks[::-1])
Thank you very much for the explanation! I realized my mistake. I liked the Way 2.
– Ravi Joshi
Jan 2 at 13:10
@RaviJoshi: You are welcome. Glad to help. The first approach is however direct
– Bazingaa
Jan 2 at 13:11
add a comment |
Use:
# generate the data
n = 6
y = np.random.randint(low=0, high=10, size=n)
x = np.arange(n)
# generate the ticks and reverse it
xticks = range(n)
# xticks.reverse()
# plot the data
plt.figure()
ax = plt.subplot(111)
ax.bar(x, y)
# print xticks # prints [5, 4, 3, 2, 1, 0]
ax.set_xticklabels(xticks[::-1]) # <- Changed
plt.show()
Thanks! It works, however, I couldn't understand the following: changedrange(n)
torange(n + 1)
– Ravi Joshi
Jan 2 at 13:05
n+1 is not needed here. range(6) generates 0, 1, 2, 3, 4, 5. Thats all what the OP needs.range(7)
will generate 0, 1, 2, 3, 4, 5, 6. Here6
is not needed
– Bazingaa
Jan 2 at 13:09
@Bazingaa well if you say so.
– meW
Jan 2 at 13:11
@meW: Not because I say so, but because there are only 6 bars. So you need indices starting from 0 up to 5 which are generated by range(6).
– Bazingaa
Jan 2 at 13:12
I agree. It's just that when I experimented, I didn't receive the last tick with n so used n+1. Either way I agree on range(6) too which makes sense
– meW
Jan 2 at 13:13
|
show 2 more comments
You can also reverse the order of the axis ax.set_xlim([5.5, -0.5])
import numpy as np
import matplotlib.pyplot as plt
n = 6
x = np.arange(n)
y = (x+1) **(1/2)
fig, axs = plt.subplots(1, 3, constrained_layout=True)
axs[0].bar(x, y)
axs[0].set_title('Original data')
axs[1].bar(x[::-1], y)
axs[1].set_xlim(5.5, -0.5)
axs[1].set_title('x index reversednand axis reversed')
axs[2].bar(x, y)
axs[2].set_xlim(5.5, -0.5)
axs[2].set_title('just axis reversed')
plt.show()
Not sure why this was voted down - its the proper way to reverse the order of the ticks, rather than changing all the labels.
– Jody Klymak
Jan 10 at 15:57
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54005499%2funable-to-reverse-xticks-inside-matplotlib-subplot%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
With ax.set_xticks
, you are currently specifying tick positions which is invariant to the order of the list. Either you pass [0, 1, 2, 3, 4, 5]
or you pass [5, 4, 3, 2, 1, 0]
. The difference will not be noticed in the ticks. What you instead want is to have reversed ticklabels for which you should do set_xticklabels(xticks[::-1])
. There are two ways to do it:
Way 1
Use plt.xticks
where the first argument specifies the location of the ticks and the second arguments specifies the respective ticklabels. Specifically, xticks
will provide the tick positions and xticks[::-1]
will label your plot with reversed ticklabels.
xticks = range(n)
# plot the data
plt.figure()
ax = plt.subplot(111)
ax.bar(x, y)
plt.xticks(xticks, xticks[::-1])
Way 2 using ax
where you need set_xticklabels
to get what you want
ax.set_xticks(xticks)
ax.set_xticklabels(xticks[::-1])
Thank you very much for the explanation! I realized my mistake. I liked the Way 2.
– Ravi Joshi
Jan 2 at 13:10
@RaviJoshi: You are welcome. Glad to help. The first approach is however direct
– Bazingaa
Jan 2 at 13:11
add a comment |
With ax.set_xticks
, you are currently specifying tick positions which is invariant to the order of the list. Either you pass [0, 1, 2, 3, 4, 5]
or you pass [5, 4, 3, 2, 1, 0]
. The difference will not be noticed in the ticks. What you instead want is to have reversed ticklabels for which you should do set_xticklabels(xticks[::-1])
. There are two ways to do it:
Way 1
Use plt.xticks
where the first argument specifies the location of the ticks and the second arguments specifies the respective ticklabels. Specifically, xticks
will provide the tick positions and xticks[::-1]
will label your plot with reversed ticklabels.
xticks = range(n)
# plot the data
plt.figure()
ax = plt.subplot(111)
ax.bar(x, y)
plt.xticks(xticks, xticks[::-1])
Way 2 using ax
where you need set_xticklabels
to get what you want
ax.set_xticks(xticks)
ax.set_xticklabels(xticks[::-1])
Thank you very much for the explanation! I realized my mistake. I liked the Way 2.
– Ravi Joshi
Jan 2 at 13:10
@RaviJoshi: You are welcome. Glad to help. The first approach is however direct
– Bazingaa
Jan 2 at 13:11
add a comment |
With ax.set_xticks
, you are currently specifying tick positions which is invariant to the order of the list. Either you pass [0, 1, 2, 3, 4, 5]
or you pass [5, 4, 3, 2, 1, 0]
. The difference will not be noticed in the ticks. What you instead want is to have reversed ticklabels for which you should do set_xticklabels(xticks[::-1])
. There are two ways to do it:
Way 1
Use plt.xticks
where the first argument specifies the location of the ticks and the second arguments specifies the respective ticklabels. Specifically, xticks
will provide the tick positions and xticks[::-1]
will label your plot with reversed ticklabels.
xticks = range(n)
# plot the data
plt.figure()
ax = plt.subplot(111)
ax.bar(x, y)
plt.xticks(xticks, xticks[::-1])
Way 2 using ax
where you need set_xticklabels
to get what you want
ax.set_xticks(xticks)
ax.set_xticklabels(xticks[::-1])
With ax.set_xticks
, you are currently specifying tick positions which is invariant to the order of the list. Either you pass [0, 1, 2, 3, 4, 5]
or you pass [5, 4, 3, 2, 1, 0]
. The difference will not be noticed in the ticks. What you instead want is to have reversed ticklabels for which you should do set_xticklabels(xticks[::-1])
. There are two ways to do it:
Way 1
Use plt.xticks
where the first argument specifies the location of the ticks and the second arguments specifies the respective ticklabels. Specifically, xticks
will provide the tick positions and xticks[::-1]
will label your plot with reversed ticklabels.
xticks = range(n)
# plot the data
plt.figure()
ax = plt.subplot(111)
ax.bar(x, y)
plt.xticks(xticks, xticks[::-1])
Way 2 using ax
where you need set_xticklabels
to get what you want
ax.set_xticks(xticks)
ax.set_xticklabels(xticks[::-1])
edited Jan 2 at 11:36
answered Jan 2 at 11:31


BazingaaBazingaa
16.2k21330
16.2k21330
Thank you very much for the explanation! I realized my mistake. I liked the Way 2.
– Ravi Joshi
Jan 2 at 13:10
@RaviJoshi: You are welcome. Glad to help. The first approach is however direct
– Bazingaa
Jan 2 at 13:11
add a comment |
Thank you very much for the explanation! I realized my mistake. I liked the Way 2.
– Ravi Joshi
Jan 2 at 13:10
@RaviJoshi: You are welcome. Glad to help. The first approach is however direct
– Bazingaa
Jan 2 at 13:11
Thank you very much for the explanation! I realized my mistake. I liked the Way 2.
– Ravi Joshi
Jan 2 at 13:10
Thank you very much for the explanation! I realized my mistake. I liked the Way 2.
– Ravi Joshi
Jan 2 at 13:10
@RaviJoshi: You are welcome. Glad to help. The first approach is however direct
– Bazingaa
Jan 2 at 13:11
@RaviJoshi: You are welcome. Glad to help. The first approach is however direct
– Bazingaa
Jan 2 at 13:11
add a comment |
Use:
# generate the data
n = 6
y = np.random.randint(low=0, high=10, size=n)
x = np.arange(n)
# generate the ticks and reverse it
xticks = range(n)
# xticks.reverse()
# plot the data
plt.figure()
ax = plt.subplot(111)
ax.bar(x, y)
# print xticks # prints [5, 4, 3, 2, 1, 0]
ax.set_xticklabels(xticks[::-1]) # <- Changed
plt.show()
Thanks! It works, however, I couldn't understand the following: changedrange(n)
torange(n + 1)
– Ravi Joshi
Jan 2 at 13:05
n+1 is not needed here. range(6) generates 0, 1, 2, 3, 4, 5. Thats all what the OP needs.range(7)
will generate 0, 1, 2, 3, 4, 5, 6. Here6
is not needed
– Bazingaa
Jan 2 at 13:09
@Bazingaa well if you say so.
– meW
Jan 2 at 13:11
@meW: Not because I say so, but because there are only 6 bars. So you need indices starting from 0 up to 5 which are generated by range(6).
– Bazingaa
Jan 2 at 13:12
I agree. It's just that when I experimented, I didn't receive the last tick with n so used n+1. Either way I agree on range(6) too which makes sense
– meW
Jan 2 at 13:13
|
show 2 more comments
Use:
# generate the data
n = 6
y = np.random.randint(low=0, high=10, size=n)
x = np.arange(n)
# generate the ticks and reverse it
xticks = range(n)
# xticks.reverse()
# plot the data
plt.figure()
ax = plt.subplot(111)
ax.bar(x, y)
# print xticks # prints [5, 4, 3, 2, 1, 0]
ax.set_xticklabels(xticks[::-1]) # <- Changed
plt.show()
Thanks! It works, however, I couldn't understand the following: changedrange(n)
torange(n + 1)
– Ravi Joshi
Jan 2 at 13:05
n+1 is not needed here. range(6) generates 0, 1, 2, 3, 4, 5. Thats all what the OP needs.range(7)
will generate 0, 1, 2, 3, 4, 5, 6. Here6
is not needed
– Bazingaa
Jan 2 at 13:09
@Bazingaa well if you say so.
– meW
Jan 2 at 13:11
@meW: Not because I say so, but because there are only 6 bars. So you need indices starting from 0 up to 5 which are generated by range(6).
– Bazingaa
Jan 2 at 13:12
I agree. It's just that when I experimented, I didn't receive the last tick with n so used n+1. Either way I agree on range(6) too which makes sense
– meW
Jan 2 at 13:13
|
show 2 more comments
Use:
# generate the data
n = 6
y = np.random.randint(low=0, high=10, size=n)
x = np.arange(n)
# generate the ticks and reverse it
xticks = range(n)
# xticks.reverse()
# plot the data
plt.figure()
ax = plt.subplot(111)
ax.bar(x, y)
# print xticks # prints [5, 4, 3, 2, 1, 0]
ax.set_xticklabels(xticks[::-1]) # <- Changed
plt.show()
Use:
# generate the data
n = 6
y = np.random.randint(low=0, high=10, size=n)
x = np.arange(n)
# generate the ticks and reverse it
xticks = range(n)
# xticks.reverse()
# plot the data
plt.figure()
ax = plt.subplot(111)
ax.bar(x, y)
# print xticks # prints [5, 4, 3, 2, 1, 0]
ax.set_xticklabels(xticks[::-1]) # <- Changed
plt.show()
edited Jan 2 at 13:15
answered Jan 2 at 11:30


meWmeW
2,798119
2,798119
Thanks! It works, however, I couldn't understand the following: changedrange(n)
torange(n + 1)
– Ravi Joshi
Jan 2 at 13:05
n+1 is not needed here. range(6) generates 0, 1, 2, 3, 4, 5. Thats all what the OP needs.range(7)
will generate 0, 1, 2, 3, 4, 5, 6. Here6
is not needed
– Bazingaa
Jan 2 at 13:09
@Bazingaa well if you say so.
– meW
Jan 2 at 13:11
@meW: Not because I say so, but because there are only 6 bars. So you need indices starting from 0 up to 5 which are generated by range(6).
– Bazingaa
Jan 2 at 13:12
I agree. It's just that when I experimented, I didn't receive the last tick with n so used n+1. Either way I agree on range(6) too which makes sense
– meW
Jan 2 at 13:13
|
show 2 more comments
Thanks! It works, however, I couldn't understand the following: changedrange(n)
torange(n + 1)
– Ravi Joshi
Jan 2 at 13:05
n+1 is not needed here. range(6) generates 0, 1, 2, 3, 4, 5. Thats all what the OP needs.range(7)
will generate 0, 1, 2, 3, 4, 5, 6. Here6
is not needed
– Bazingaa
Jan 2 at 13:09
@Bazingaa well if you say so.
– meW
Jan 2 at 13:11
@meW: Not because I say so, but because there are only 6 bars. So you need indices starting from 0 up to 5 which are generated by range(6).
– Bazingaa
Jan 2 at 13:12
I agree. It's just that when I experimented, I didn't receive the last tick with n so used n+1. Either way I agree on range(6) too which makes sense
– meW
Jan 2 at 13:13
Thanks! It works, however, I couldn't understand the following: changed
range(n)
to range(n + 1)
– Ravi Joshi
Jan 2 at 13:05
Thanks! It works, however, I couldn't understand the following: changed
range(n)
to range(n + 1)
– Ravi Joshi
Jan 2 at 13:05
n+1 is not needed here. range(6) generates 0, 1, 2, 3, 4, 5. Thats all what the OP needs.
range(7)
will generate 0, 1, 2, 3, 4, 5, 6. Here 6
is not needed– Bazingaa
Jan 2 at 13:09
n+1 is not needed here. range(6) generates 0, 1, 2, 3, 4, 5. Thats all what the OP needs.
range(7)
will generate 0, 1, 2, 3, 4, 5, 6. Here 6
is not needed– Bazingaa
Jan 2 at 13:09
@Bazingaa well if you say so.
– meW
Jan 2 at 13:11
@Bazingaa well if you say so.
– meW
Jan 2 at 13:11
@meW: Not because I say so, but because there are only 6 bars. So you need indices starting from 0 up to 5 which are generated by range(6).
– Bazingaa
Jan 2 at 13:12
@meW: Not because I say so, but because there are only 6 bars. So you need indices starting from 0 up to 5 which are generated by range(6).
– Bazingaa
Jan 2 at 13:12
I agree. It's just that when I experimented, I didn't receive the last tick with n so used n+1. Either way I agree on range(6) too which makes sense
– meW
Jan 2 at 13:13
I agree. It's just that when I experimented, I didn't receive the last tick with n so used n+1. Either way I agree on range(6) too which makes sense
– meW
Jan 2 at 13:13
|
show 2 more comments
You can also reverse the order of the axis ax.set_xlim([5.5, -0.5])
import numpy as np
import matplotlib.pyplot as plt
n = 6
x = np.arange(n)
y = (x+1) **(1/2)
fig, axs = plt.subplots(1, 3, constrained_layout=True)
axs[0].bar(x, y)
axs[0].set_title('Original data')
axs[1].bar(x[::-1], y)
axs[1].set_xlim(5.5, -0.5)
axs[1].set_title('x index reversednand axis reversed')
axs[2].bar(x, y)
axs[2].set_xlim(5.5, -0.5)
axs[2].set_title('just axis reversed')
plt.show()
Not sure why this was voted down - its the proper way to reverse the order of the ticks, rather than changing all the labels.
– Jody Klymak
Jan 10 at 15:57
add a comment |
You can also reverse the order of the axis ax.set_xlim([5.5, -0.5])
import numpy as np
import matplotlib.pyplot as plt
n = 6
x = np.arange(n)
y = (x+1) **(1/2)
fig, axs = plt.subplots(1, 3, constrained_layout=True)
axs[0].bar(x, y)
axs[0].set_title('Original data')
axs[1].bar(x[::-1], y)
axs[1].set_xlim(5.5, -0.5)
axs[1].set_title('x index reversednand axis reversed')
axs[2].bar(x, y)
axs[2].set_xlim(5.5, -0.5)
axs[2].set_title('just axis reversed')
plt.show()
Not sure why this was voted down - its the proper way to reverse the order of the ticks, rather than changing all the labels.
– Jody Klymak
Jan 10 at 15:57
add a comment |
You can also reverse the order of the axis ax.set_xlim([5.5, -0.5])
import numpy as np
import matplotlib.pyplot as plt
n = 6
x = np.arange(n)
y = (x+1) **(1/2)
fig, axs = plt.subplots(1, 3, constrained_layout=True)
axs[0].bar(x, y)
axs[0].set_title('Original data')
axs[1].bar(x[::-1], y)
axs[1].set_xlim(5.5, -0.5)
axs[1].set_title('x index reversednand axis reversed')
axs[2].bar(x, y)
axs[2].set_xlim(5.5, -0.5)
axs[2].set_title('just axis reversed')
plt.show()
You can also reverse the order of the axis ax.set_xlim([5.5, -0.5])
import numpy as np
import matplotlib.pyplot as plt
n = 6
x = np.arange(n)
y = (x+1) **(1/2)
fig, axs = plt.subplots(1, 3, constrained_layout=True)
axs[0].bar(x, y)
axs[0].set_title('Original data')
axs[1].bar(x[::-1], y)
axs[1].set_xlim(5.5, -0.5)
axs[1].set_title('x index reversednand axis reversed')
axs[2].bar(x, y)
axs[2].set_xlim(5.5, -0.5)
axs[2].set_title('just axis reversed')
plt.show()
edited Jan 10 at 17:51
answered Jan 10 at 6:29
Jody KlymakJody Klymak
1946
1946
Not sure why this was voted down - its the proper way to reverse the order of the ticks, rather than changing all the labels.
– Jody Klymak
Jan 10 at 15:57
add a comment |
Not sure why this was voted down - its the proper way to reverse the order of the ticks, rather than changing all the labels.
– Jody Klymak
Jan 10 at 15:57
Not sure why this was voted down - its the proper way to reverse the order of the ticks, rather than changing all the labels.
– Jody Klymak
Jan 10 at 15:57
Not sure why this was voted down - its the proper way to reverse the order of the ticks, rather than changing all the labels.
– Jody Klymak
Jan 10 at 15:57
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54005499%2funable-to-reverse-xticks-inside-matplotlib-subplot%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XAoGjAMvnJqzMZ,N4,eqrjRTjPjrrmII5J BxFcxacJo45rpKh1X 7pKkxdF 88GXm