Is there a way to display live calculation with an information taken from mysql database?
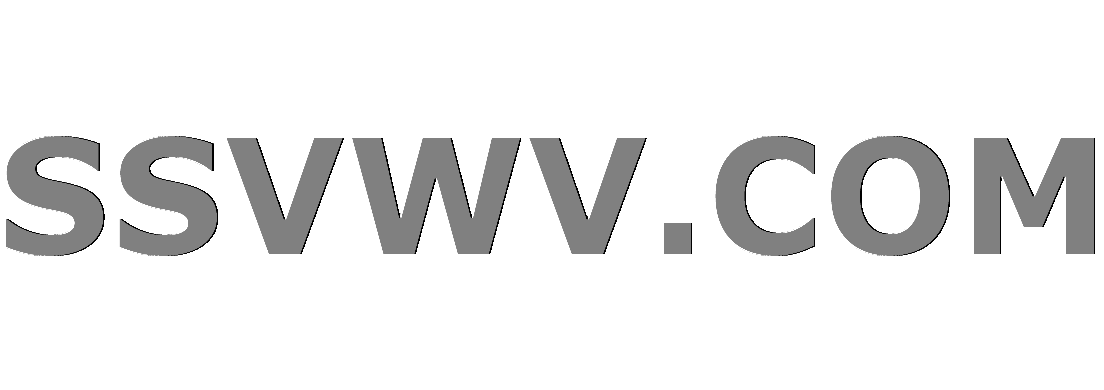
Multi tool use
I am developing a web application, and I need to display a live calculation as soon as the fields are filled with the information.
What I mean by a live calculation is something as live as a ng-model in a ng-app. The problem goes like this:
The user chooses a product from a dropdown list, which list's choices are coming from a mysql database and the price of the chosen product should be the first multiplyer, while the second multiplyer is the next input field where the customer should enter how many pieces they want.
I tried to take the price from the table where product is selected but I believe that php needs a submission in order to process the data and actually see which product has been selected.
So is there any way to display a live calculation and inform the customer how much their order will cost before they submit the form?
Thank you!
Update:
I came few steps further along the way but i got stuck again... so now i do retrieve the value of the chosen product but i fail to do the calculation because javascript says that the retrieved value is not a number (NaN) as soon as i try to do the operation.
This is the code that shows the value of the chosen product
<form action="#" method="POST">
<input type="text" name="clientname" class="fields" placeholder="Enter your name " required/>
<input type="text" name="contactinfo" class="fields" placeholder="Phone or Email" required/>
<input type="text" name="address" class="fields" placeholder="Street Address" required/>
<select class='dropdown' name='ordertype' id='ordertype' onchange='productPrice()' required>
<option value="" disabled selected>Select Product</option>
<?php
$query = "SELECT * FROM products";
$query_run = mysqli_query($mysqli, $query);
while($row = $query_run->fetch_assoc()){
echo"<option value=".$row['price'].">".$row['name']."</option>";
}
?>
</select>
<input ng-model="price" type="number" name="amount" class="fields" placeholder="How many pieces?" required/>
<button name="placeorder" class="regbutton">Place Order</button>
</form>
<script type="text/javascript">
function productPrice(){
var option = document.getElementById("ordertype").selectedIndex;
var value = document.getElementById("ordertype").options;
var result = (value[option].value);
document.getElementById("result").innerHTML = result + "$";
}
document.write('<p id="result"></p>' );
</script>
but the moment i try to do the calculation and multiply the given output * {{price}} or even when i try to multiply it * 2 it says NaN on page load (it has nothing to do with the dollar sign).
So do you know why is this happening and how can i fix it?
Thanks again!
javascript php mysql
add a comment |
I am developing a web application, and I need to display a live calculation as soon as the fields are filled with the information.
What I mean by a live calculation is something as live as a ng-model in a ng-app. The problem goes like this:
The user chooses a product from a dropdown list, which list's choices are coming from a mysql database and the price of the chosen product should be the first multiplyer, while the second multiplyer is the next input field where the customer should enter how many pieces they want.
I tried to take the price from the table where product is selected but I believe that php needs a submission in order to process the data and actually see which product has been selected.
So is there any way to display a live calculation and inform the customer how much their order will cost before they submit the form?
Thank you!
Update:
I came few steps further along the way but i got stuck again... so now i do retrieve the value of the chosen product but i fail to do the calculation because javascript says that the retrieved value is not a number (NaN) as soon as i try to do the operation.
This is the code that shows the value of the chosen product
<form action="#" method="POST">
<input type="text" name="clientname" class="fields" placeholder="Enter your name " required/>
<input type="text" name="contactinfo" class="fields" placeholder="Phone or Email" required/>
<input type="text" name="address" class="fields" placeholder="Street Address" required/>
<select class='dropdown' name='ordertype' id='ordertype' onchange='productPrice()' required>
<option value="" disabled selected>Select Product</option>
<?php
$query = "SELECT * FROM products";
$query_run = mysqli_query($mysqli, $query);
while($row = $query_run->fetch_assoc()){
echo"<option value=".$row['price'].">".$row['name']."</option>";
}
?>
</select>
<input ng-model="price" type="number" name="amount" class="fields" placeholder="How many pieces?" required/>
<button name="placeorder" class="regbutton">Place Order</button>
</form>
<script type="text/javascript">
function productPrice(){
var option = document.getElementById("ordertype").selectedIndex;
var value = document.getElementById("ordertype").options;
var result = (value[option].value);
document.getElementById("result").innerHTML = result + "$";
}
document.write('<p id="result"></p>' );
</script>
but the moment i try to do the calculation and multiply the given output * {{price}} or even when i try to multiply it * 2 it says NaN on page load (it has nothing to do with the dollar sign).
So do you know why is this happening and how can i fix it?
Thanks again!
javascript php mysql
You need to look on Ajax
– executable
Jan 2 at 11:48
You can use AJAX to run an asynchronous process, for example, you can get the price of the product and then multiply it with the quantity.
– Marcos Riveros
Jan 2 at 11:48
try to look eventsource
– Kamran Sohail
Jan 2 at 11:52
add a comment |
I am developing a web application, and I need to display a live calculation as soon as the fields are filled with the information.
What I mean by a live calculation is something as live as a ng-model in a ng-app. The problem goes like this:
The user chooses a product from a dropdown list, which list's choices are coming from a mysql database and the price of the chosen product should be the first multiplyer, while the second multiplyer is the next input field where the customer should enter how many pieces they want.
I tried to take the price from the table where product is selected but I believe that php needs a submission in order to process the data and actually see which product has been selected.
So is there any way to display a live calculation and inform the customer how much their order will cost before they submit the form?
Thank you!
Update:
I came few steps further along the way but i got stuck again... so now i do retrieve the value of the chosen product but i fail to do the calculation because javascript says that the retrieved value is not a number (NaN) as soon as i try to do the operation.
This is the code that shows the value of the chosen product
<form action="#" method="POST">
<input type="text" name="clientname" class="fields" placeholder="Enter your name " required/>
<input type="text" name="contactinfo" class="fields" placeholder="Phone or Email" required/>
<input type="text" name="address" class="fields" placeholder="Street Address" required/>
<select class='dropdown' name='ordertype' id='ordertype' onchange='productPrice()' required>
<option value="" disabled selected>Select Product</option>
<?php
$query = "SELECT * FROM products";
$query_run = mysqli_query($mysqli, $query);
while($row = $query_run->fetch_assoc()){
echo"<option value=".$row['price'].">".$row['name']."</option>";
}
?>
</select>
<input ng-model="price" type="number" name="amount" class="fields" placeholder="How many pieces?" required/>
<button name="placeorder" class="regbutton">Place Order</button>
</form>
<script type="text/javascript">
function productPrice(){
var option = document.getElementById("ordertype").selectedIndex;
var value = document.getElementById("ordertype").options;
var result = (value[option].value);
document.getElementById("result").innerHTML = result + "$";
}
document.write('<p id="result"></p>' );
</script>
but the moment i try to do the calculation and multiply the given output * {{price}} or even when i try to multiply it * 2 it says NaN on page load (it has nothing to do with the dollar sign).
So do you know why is this happening and how can i fix it?
Thanks again!
javascript php mysql
I am developing a web application, and I need to display a live calculation as soon as the fields are filled with the information.
What I mean by a live calculation is something as live as a ng-model in a ng-app. The problem goes like this:
The user chooses a product from a dropdown list, which list's choices are coming from a mysql database and the price of the chosen product should be the first multiplyer, while the second multiplyer is the next input field where the customer should enter how many pieces they want.
I tried to take the price from the table where product is selected but I believe that php needs a submission in order to process the data and actually see which product has been selected.
So is there any way to display a live calculation and inform the customer how much their order will cost before they submit the form?
Thank you!
Update:
I came few steps further along the way but i got stuck again... so now i do retrieve the value of the chosen product but i fail to do the calculation because javascript says that the retrieved value is not a number (NaN) as soon as i try to do the operation.
This is the code that shows the value of the chosen product
<form action="#" method="POST">
<input type="text" name="clientname" class="fields" placeholder="Enter your name " required/>
<input type="text" name="contactinfo" class="fields" placeholder="Phone or Email" required/>
<input type="text" name="address" class="fields" placeholder="Street Address" required/>
<select class='dropdown' name='ordertype' id='ordertype' onchange='productPrice()' required>
<option value="" disabled selected>Select Product</option>
<?php
$query = "SELECT * FROM products";
$query_run = mysqli_query($mysqli, $query);
while($row = $query_run->fetch_assoc()){
echo"<option value=".$row['price'].">".$row['name']."</option>";
}
?>
</select>
<input ng-model="price" type="number" name="amount" class="fields" placeholder="How many pieces?" required/>
<button name="placeorder" class="regbutton">Place Order</button>
</form>
<script type="text/javascript">
function productPrice(){
var option = document.getElementById("ordertype").selectedIndex;
var value = document.getElementById("ordertype").options;
var result = (value[option].value);
document.getElementById("result").innerHTML = result + "$";
}
document.write('<p id="result"></p>' );
</script>
but the moment i try to do the calculation and multiply the given output * {{price}} or even when i try to multiply it * 2 it says NaN on page load (it has nothing to do with the dollar sign).
So do you know why is this happening and how can i fix it?
Thanks again!
javascript php mysql
javascript php mysql
edited Jan 3 at 9:59
asked Jan 2 at 11:32
user8007596
You need to look on Ajax
– executable
Jan 2 at 11:48
You can use AJAX to run an asynchronous process, for example, you can get the price of the product and then multiply it with the quantity.
– Marcos Riveros
Jan 2 at 11:48
try to look eventsource
– Kamran Sohail
Jan 2 at 11:52
add a comment |
You need to look on Ajax
– executable
Jan 2 at 11:48
You can use AJAX to run an asynchronous process, for example, you can get the price of the product and then multiply it with the quantity.
– Marcos Riveros
Jan 2 at 11:48
try to look eventsource
– Kamran Sohail
Jan 2 at 11:52
You need to look on Ajax
– executable
Jan 2 at 11:48
You need to look on Ajax
– executable
Jan 2 at 11:48
You can use AJAX to run an asynchronous process, for example, you can get the price of the product and then multiply it with the quantity.
– Marcos Riveros
Jan 2 at 11:48
You can use AJAX to run an asynchronous process, for example, you can get the price of the product and then multiply it with the quantity.
– Marcos Riveros
Jan 2 at 11:48
try to look eventsource
– Kamran Sohail
Jan 2 at 11:52
try to look eventsource
– Kamran Sohail
Jan 2 at 11:52
add a comment |
3 Answers
3
active
oldest
votes
Your PHP code isn't going to know when the data changes, so even though you could use comet or websockets to get data immediately from the PHP code to the browser, you still need to poll the database for changes. It's simpler to just trigger the polling on a schedule from the browser using Ajax.
add a comment |
This will give you idea,
var source = new EventSource("demo_sse.php");
source.onmessage = function(event) {
document.getElementById("result").innerHTML += event.data + "<br>";
};
<div id="result"></div>
demo_sse.php
<?php
header('Content-Type: text/event-stream');
header('Cache-Control: no-cache');
$time = date('r');
echo "data: The server time is: {$time}nn";
flush();
?>
add a comment |
use jQuery ajax call.
go step by step.
1st get the qty of any product or any thing that the have changed.
on ajax call i guess you are creating a cart. let every thing same just add product or any thing to the cart of your. and get the hole data.
append the recent value.
in this way the amount you have stored in php that you can have at your client side using ajax call
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54005578%2fis-there-a-way-to-display-live-calculation-with-an-information-taken-from-mysql%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your PHP code isn't going to know when the data changes, so even though you could use comet or websockets to get data immediately from the PHP code to the browser, you still need to poll the database for changes. It's simpler to just trigger the polling on a schedule from the browser using Ajax.
add a comment |
Your PHP code isn't going to know when the data changes, so even though you could use comet or websockets to get data immediately from the PHP code to the browser, you still need to poll the database for changes. It's simpler to just trigger the polling on a schedule from the browser using Ajax.
add a comment |
Your PHP code isn't going to know when the data changes, so even though you could use comet or websockets to get data immediately from the PHP code to the browser, you still need to poll the database for changes. It's simpler to just trigger the polling on a schedule from the browser using Ajax.
Your PHP code isn't going to know when the data changes, so even though you could use comet or websockets to get data immediately from the PHP code to the browser, you still need to poll the database for changes. It's simpler to just trigger the polling on a schedule from the browser using Ajax.
answered Jan 2 at 11:49
symcbeansymcbean
41.3k54076
41.3k54076
add a comment |
add a comment |
This will give you idea,
var source = new EventSource("demo_sse.php");
source.onmessage = function(event) {
document.getElementById("result").innerHTML += event.data + "<br>";
};
<div id="result"></div>
demo_sse.php
<?php
header('Content-Type: text/event-stream');
header('Cache-Control: no-cache');
$time = date('r');
echo "data: The server time is: {$time}nn";
flush();
?>
add a comment |
This will give you idea,
var source = new EventSource("demo_sse.php");
source.onmessage = function(event) {
document.getElementById("result").innerHTML += event.data + "<br>";
};
<div id="result"></div>
demo_sse.php
<?php
header('Content-Type: text/event-stream');
header('Cache-Control: no-cache');
$time = date('r');
echo "data: The server time is: {$time}nn";
flush();
?>
add a comment |
This will give you idea,
var source = new EventSource("demo_sse.php");
source.onmessage = function(event) {
document.getElementById("result").innerHTML += event.data + "<br>";
};
<div id="result"></div>
demo_sse.php
<?php
header('Content-Type: text/event-stream');
header('Cache-Control: no-cache');
$time = date('r');
echo "data: The server time is: {$time}nn";
flush();
?>
This will give you idea,
var source = new EventSource("demo_sse.php");
source.onmessage = function(event) {
document.getElementById("result").innerHTML += event.data + "<br>";
};
<div id="result"></div>
demo_sse.php
<?php
header('Content-Type: text/event-stream');
header('Cache-Control: no-cache');
$time = date('r');
echo "data: The server time is: {$time}nn";
flush();
?>
answered Jan 2 at 11:57
Kamran SohailKamran Sohail
35528
35528
add a comment |
add a comment |
use jQuery ajax call.
go step by step.
1st get the qty of any product or any thing that the have changed.
on ajax call i guess you are creating a cart. let every thing same just add product or any thing to the cart of your. and get the hole data.
append the recent value.
in this way the amount you have stored in php that you can have at your client side using ajax call
add a comment |
use jQuery ajax call.
go step by step.
1st get the qty of any product or any thing that the have changed.
on ajax call i guess you are creating a cart. let every thing same just add product or any thing to the cart of your. and get the hole data.
append the recent value.
in this way the amount you have stored in php that you can have at your client side using ajax call
add a comment |
use jQuery ajax call.
go step by step.
1st get the qty of any product or any thing that the have changed.
on ajax call i guess you are creating a cart. let every thing same just add product or any thing to the cart of your. and get the hole data.
append the recent value.
in this way the amount you have stored in php that you can have at your client side using ajax call
use jQuery ajax call.
go step by step.
1st get the qty of any product or any thing that the have changed.
on ajax call i guess you are creating a cart. let every thing same just add product or any thing to the cart of your. and get the hole data.
append the recent value.
in this way the amount you have stored in php that you can have at your client side using ajax call
answered Jan 3 at 11:13
Masih AnsariMasih Ansari
3288
3288
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54005578%2fis-there-a-way-to-display-live-calculation-with-an-information-taken-from-mysql%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DwniP4ec49T,xCf jMQ0PDpRcDRG9KsYu,6VvbX koHs hmuLHmKqjJn9DAiVNNsWyLWEeay W5DcylNesp2P4
You need to look on Ajax
– executable
Jan 2 at 11:48
You can use AJAX to run an asynchronous process, for example, you can get the price of the product and then multiply it with the quantity.
– Marcos Riveros
Jan 2 at 11:48
try to look eventsource
– Kamran Sohail
Jan 2 at 11:52