angular 6 unit testing Forkjoin service response
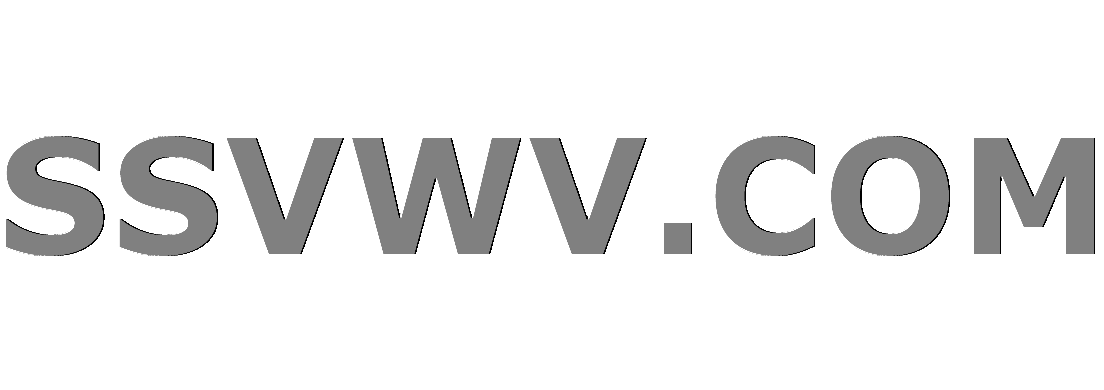
Multi tool use
i have a function in service in which i have 3 http get calls and i return the response with forkJoin making an array, how will i test this service funtcion in spec.ts ?
this is the function in my registration component which is calling the service,
this.register.registrationData()
.subscribe(data => {
this.types = data[0];
this.modules = data[1];
this.packages = data[2];
});
and this is my registration service function,
registrationData(): Observable<any> {
const companyTypes =this.http.get(ConstantService.apiRoutes.companyTypes);
const modules = this.http.get(ConstantService.apiRoutes.modules);
const packages = this.http.get(ConstantService.apiRoutes.packages);
return forkJoin([companyTypes, modules, packages]);
}
this is my spec file so far,
it('Should require registration data', () => {
loginRegService.registrationData().subscribe((data) => {
expect(data).toEqual(regData);
})
const reqCompany = httpTestingController.expectOne(`${environment.apiUrl}/anonymous/companyTypes/`);
const reqModule = httpTestingController.expectOne(`${environment.apiUrl}/anonymous/modules/`);
const reqPackage = httpTestingController.expectOne(`${environment.apiUrl}/anonymous/packages/`);
expect(reqCompany.request.method).toBe('GET');
expect(reqModule.request.method).toBe('GET');
expect(reqPackage.request.method).toBe('GET');
const e1 = forkJoin([reqCompany, reqModule, reqPackage]);
reqCompany.flush(companyType);
reqModule.flush(modules);
reqPackage.flush(packages);
});

add a comment |
i have a function in service in which i have 3 http get calls and i return the response with forkJoin making an array, how will i test this service funtcion in spec.ts ?
this is the function in my registration component which is calling the service,
this.register.registrationData()
.subscribe(data => {
this.types = data[0];
this.modules = data[1];
this.packages = data[2];
});
and this is my registration service function,
registrationData(): Observable<any> {
const companyTypes =this.http.get(ConstantService.apiRoutes.companyTypes);
const modules = this.http.get(ConstantService.apiRoutes.modules);
const packages = this.http.get(ConstantService.apiRoutes.packages);
return forkJoin([companyTypes, modules, packages]);
}
this is my spec file so far,
it('Should require registration data', () => {
loginRegService.registrationData().subscribe((data) => {
expect(data).toEqual(regData);
})
const reqCompany = httpTestingController.expectOne(`${environment.apiUrl}/anonymous/companyTypes/`);
const reqModule = httpTestingController.expectOne(`${environment.apiUrl}/anonymous/modules/`);
const reqPackage = httpTestingController.expectOne(`${environment.apiUrl}/anonymous/packages/`);
expect(reqCompany.request.method).toBe('GET');
expect(reqModule.request.method).toBe('GET');
expect(reqPackage.request.method).toBe('GET');
const e1 = forkJoin([reqCompany, reqModule, reqPackage]);
reqCompany.flush(companyType);
reqModule.flush(modules);
reqPackage.flush(packages);
});

expect(data.result[0]).toEqual(regData);, expect(data.result[1]).toEqual(regData);, expect(data.result[2]).toEqual(regData);
you tried?
– Just code
Jan 2 at 12:08
yes i did try this out and i changed my data accordingly to data and now its working fine ,let regData = [companyType, modules, packages];
– syed muttaqeen rizvi
Jan 3 at 9:04
add a comment |
i have a function in service in which i have 3 http get calls and i return the response with forkJoin making an array, how will i test this service funtcion in spec.ts ?
this is the function in my registration component which is calling the service,
this.register.registrationData()
.subscribe(data => {
this.types = data[0];
this.modules = data[1];
this.packages = data[2];
});
and this is my registration service function,
registrationData(): Observable<any> {
const companyTypes =this.http.get(ConstantService.apiRoutes.companyTypes);
const modules = this.http.get(ConstantService.apiRoutes.modules);
const packages = this.http.get(ConstantService.apiRoutes.packages);
return forkJoin([companyTypes, modules, packages]);
}
this is my spec file so far,
it('Should require registration data', () => {
loginRegService.registrationData().subscribe((data) => {
expect(data).toEqual(regData);
})
const reqCompany = httpTestingController.expectOne(`${environment.apiUrl}/anonymous/companyTypes/`);
const reqModule = httpTestingController.expectOne(`${environment.apiUrl}/anonymous/modules/`);
const reqPackage = httpTestingController.expectOne(`${environment.apiUrl}/anonymous/packages/`);
expect(reqCompany.request.method).toBe('GET');
expect(reqModule.request.method).toBe('GET');
expect(reqPackage.request.method).toBe('GET');
const e1 = forkJoin([reqCompany, reqModule, reqPackage]);
reqCompany.flush(companyType);
reqModule.flush(modules);
reqPackage.flush(packages);
});

i have a function in service in which i have 3 http get calls and i return the response with forkJoin making an array, how will i test this service funtcion in spec.ts ?
this is the function in my registration component which is calling the service,
this.register.registrationData()
.subscribe(data => {
this.types = data[0];
this.modules = data[1];
this.packages = data[2];
});
and this is my registration service function,
registrationData(): Observable<any> {
const companyTypes =this.http.get(ConstantService.apiRoutes.companyTypes);
const modules = this.http.get(ConstantService.apiRoutes.modules);
const packages = this.http.get(ConstantService.apiRoutes.packages);
return forkJoin([companyTypes, modules, packages]);
}
this is my spec file so far,
it('Should require registration data', () => {
loginRegService.registrationData().subscribe((data) => {
expect(data).toEqual(regData);
})
const reqCompany = httpTestingController.expectOne(`${environment.apiUrl}/anonymous/companyTypes/`);
const reqModule = httpTestingController.expectOne(`${environment.apiUrl}/anonymous/modules/`);
const reqPackage = httpTestingController.expectOne(`${environment.apiUrl}/anonymous/packages/`);
expect(reqCompany.request.method).toBe('GET');
expect(reqModule.request.method).toBe('GET');
expect(reqPackage.request.method).toBe('GET');
const e1 = forkJoin([reqCompany, reqModule, reqPackage]);
reqCompany.flush(companyType);
reqModule.flush(modules);
reqPackage.flush(packages);
});


asked Jan 2 at 11:19


syed muttaqeen rizvisyed muttaqeen rizvi
31
31
expect(data.result[0]).toEqual(regData);, expect(data.result[1]).toEqual(regData);, expect(data.result[2]).toEqual(regData);
you tried?
– Just code
Jan 2 at 12:08
yes i did try this out and i changed my data accordingly to data and now its working fine ,let regData = [companyType, modules, packages];
– syed muttaqeen rizvi
Jan 3 at 9:04
add a comment |
expect(data.result[0]).toEqual(regData);, expect(data.result[1]).toEqual(regData);, expect(data.result[2]).toEqual(regData);
you tried?
– Just code
Jan 2 at 12:08
yes i did try this out and i changed my data accordingly to data and now its working fine ,let regData = [companyType, modules, packages];
– syed muttaqeen rizvi
Jan 3 at 9:04
expect(data.result[0]).toEqual(regData);, expect(data.result[1]).toEqual(regData);, expect(data.result[2]).toEqual(regData);
you tried?– Just code
Jan 2 at 12:08
expect(data.result[0]).toEqual(regData);, expect(data.result[1]).toEqual(regData);, expect(data.result[2]).toEqual(regData);
you tried?– Just code
Jan 2 at 12:08
yes i did try this out and i changed my data accordingly to data and now its working fine ,
let regData = [companyType, modules, packages];
– syed muttaqeen rizvi
Jan 3 at 9:04
yes i did try this out and i changed my data accordingly to data and now its working fine ,
let regData = [companyType, modules, packages];
– syed muttaqeen rizvi
Jan 3 at 9:04
add a comment |
1 Answer
1
active
oldest
votes
In your approach to testing this, you are mocking up HTTP observables - which is a good way to test HTTP calls in Angular.
However if you are looking for a more generic way of testing rxjs, you can use their marbles library.
In fact I wrote an example using forkJoin which you can find here.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54005374%2fangular-6-unit-testing-forkjoin-service-response%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
In your approach to testing this, you are mocking up HTTP observables - which is a good way to test HTTP calls in Angular.
However if you are looking for a more generic way of testing rxjs, you can use their marbles library.
In fact I wrote an example using forkJoin which you can find here.
add a comment |
In your approach to testing this, you are mocking up HTTP observables - which is a good way to test HTTP calls in Angular.
However if you are looking for a more generic way of testing rxjs, you can use their marbles library.
In fact I wrote an example using forkJoin which you can find here.
add a comment |
In your approach to testing this, you are mocking up HTTP observables - which is a good way to test HTTP calls in Angular.
However if you are looking for a more generic way of testing rxjs, you can use their marbles library.
In fact I wrote an example using forkJoin which you can find here.
In your approach to testing this, you are mocking up HTTP observables - which is a good way to test HTTP calls in Angular.
However if you are looking for a more generic way of testing rxjs, you can use their marbles library.
In fact I wrote an example using forkJoin which you can find here.
answered Jan 2 at 12:16


hevans900hevans900
1877
1877
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54005374%2fangular-6-unit-testing-forkjoin-service-response%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
HgGQ,l4cQ,Y,9I,LRHIv
expect(data.result[0]).toEqual(regData);, expect(data.result[1]).toEqual(regData);, expect(data.result[2]).toEqual(regData);
you tried?– Just code
Jan 2 at 12:08
yes i did try this out and i changed my data accordingly to data and now its working fine ,
let regData = [companyType, modules, packages];
– syed muttaqeen rizvi
Jan 3 at 9:04