Calculating superellipse shape in dart
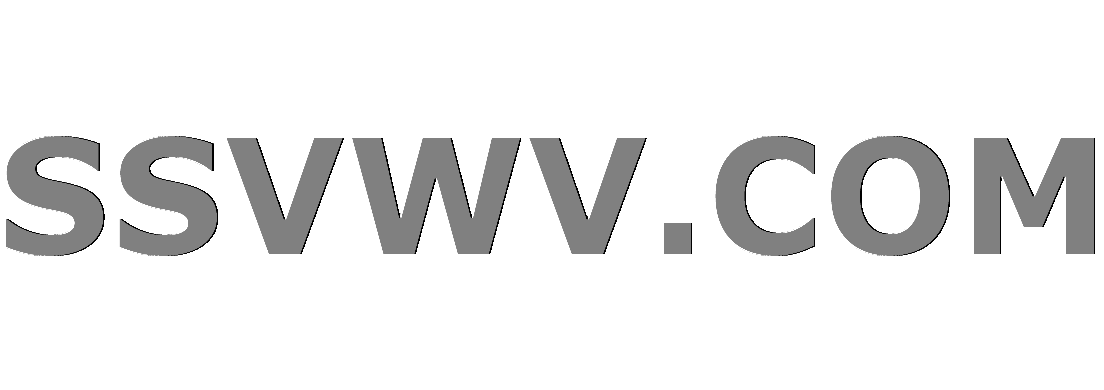
Multi tool use
I want to create a superellipse shape in Flutter for a widget.
I found an article about creating superellipses written in python and java, but i can't quite get the code to work.
Link to article
class SuperEllipse extends ShapeBorder {
final BorderSide side;
final double n;
SuperEllipse({
@required this.n,
this.side = BorderSide.none,
}) : assert(side != null);
@override
EdgeInsetsGeometry get dimensions => EdgeInsets.all(side.width);
@override
ShapeBorder scale(double t) {
return SuperEllipse(
side: side.scale(t),
n: n,
);
}
@override
Path getInnerPath(Rect rect, {TextDirection textDirection}) {
return _superEllipsePath(rect, n);
}
@override
Path getOuterPath(Rect rect, {TextDirection textDirection}) {
return _superEllipsePath(rect, n);
}
static Path _superEllipsePath(Rect rect, double n) {
final int a = 200;
List<double> points = [a + 1.0];
Path path = new Path();
path.moveTo(a.toDouble(), 0);
// Calculate first quadrant.
for (int x = a; x >= 0; x--) {
points[x] = pow(pow(a, n) - pow(x, n), 1 / n);
path.lineTo(x.toDouble(), -points[x]);
}
// Mirror to other quadrants.
for (int x = 0; x <= a; x++) {
path.lineTo(x.toDouble(), points[x]);
}
for (int x = a; x >= 0; x--) {
path.lineTo(-x.toDouble(), points[x]);
}
for (int x = 0; x <= a; x++) {
path.lineTo(-x.toDouble(), -points[x]);
}
return path;
}
@override
void paint(Canvas canvas, Rect rect, {TextDirection textDirection}) {
Path path = getOuterPath(rect.deflate(side.width / 2.0), textDirection: textDirection);
canvas.drawPath(path, side.toPaint());
}
}
I want to return the correct shape, but instead I get an Exception: Invalid value: Only valid value is 0: 200.
For some reason the variable a
isn't allowed to be 200? I don't know why, and changing it to 0 doesn't produce any errors, but then there is no shape either.
Does anyone know if there is a better way of doing this?
math dart

|
show 18 more comments
I want to create a superellipse shape in Flutter for a widget.
I found an article about creating superellipses written in python and java, but i can't quite get the code to work.
Link to article
class SuperEllipse extends ShapeBorder {
final BorderSide side;
final double n;
SuperEllipse({
@required this.n,
this.side = BorderSide.none,
}) : assert(side != null);
@override
EdgeInsetsGeometry get dimensions => EdgeInsets.all(side.width);
@override
ShapeBorder scale(double t) {
return SuperEllipse(
side: side.scale(t),
n: n,
);
}
@override
Path getInnerPath(Rect rect, {TextDirection textDirection}) {
return _superEllipsePath(rect, n);
}
@override
Path getOuterPath(Rect rect, {TextDirection textDirection}) {
return _superEllipsePath(rect, n);
}
static Path _superEllipsePath(Rect rect, double n) {
final int a = 200;
List<double> points = [a + 1.0];
Path path = new Path();
path.moveTo(a.toDouble(), 0);
// Calculate first quadrant.
for (int x = a; x >= 0; x--) {
points[x] = pow(pow(a, n) - pow(x, n), 1 / n);
path.lineTo(x.toDouble(), -points[x]);
}
// Mirror to other quadrants.
for (int x = 0; x <= a; x++) {
path.lineTo(x.toDouble(), points[x]);
}
for (int x = a; x >= 0; x--) {
path.lineTo(-x.toDouble(), points[x]);
}
for (int x = 0; x <= a; x++) {
path.lineTo(-x.toDouble(), -points[x]);
}
return path;
}
@override
void paint(Canvas canvas, Rect rect, {TextDirection textDirection}) {
Path path = getOuterPath(rect.deflate(side.width / 2.0), textDirection: textDirection);
canvas.drawPath(path, side.toPaint());
}
}
I want to return the correct shape, but instead I get an Exception: Invalid value: Only valid value is 0: 200.
For some reason the variable a
isn't allowed to be 200? I don't know why, and changing it to 0 doesn't produce any errors, but then there is no shape either.
Does anyone know if there is a better way of doing this?
math dart

see figma.com/blog/desperately-seeking-squircles
– pskink
Jan 2 at 12:00
I'm not sure how that article is helpful... I need help with my code, I understand what a superellipse and a squircle is.
– Sander Dalby Larsen
Jan 2 at 12:08
i though you really wanted the case where n >= 4
– pskink
Jan 2 at 12:17
That's not the problem, The problem is that I'm getting an Exception when I use the Shape
– Sander Dalby Larsen
Jan 2 at 12:19
ok so what is you exact stacktrace (3-4 top frames)?
– pskink
Jan 2 at 12:24
|
show 18 more comments
I want to create a superellipse shape in Flutter for a widget.
I found an article about creating superellipses written in python and java, but i can't quite get the code to work.
Link to article
class SuperEllipse extends ShapeBorder {
final BorderSide side;
final double n;
SuperEllipse({
@required this.n,
this.side = BorderSide.none,
}) : assert(side != null);
@override
EdgeInsetsGeometry get dimensions => EdgeInsets.all(side.width);
@override
ShapeBorder scale(double t) {
return SuperEllipse(
side: side.scale(t),
n: n,
);
}
@override
Path getInnerPath(Rect rect, {TextDirection textDirection}) {
return _superEllipsePath(rect, n);
}
@override
Path getOuterPath(Rect rect, {TextDirection textDirection}) {
return _superEllipsePath(rect, n);
}
static Path _superEllipsePath(Rect rect, double n) {
final int a = 200;
List<double> points = [a + 1.0];
Path path = new Path();
path.moveTo(a.toDouble(), 0);
// Calculate first quadrant.
for (int x = a; x >= 0; x--) {
points[x] = pow(pow(a, n) - pow(x, n), 1 / n);
path.lineTo(x.toDouble(), -points[x]);
}
// Mirror to other quadrants.
for (int x = 0; x <= a; x++) {
path.lineTo(x.toDouble(), points[x]);
}
for (int x = a; x >= 0; x--) {
path.lineTo(-x.toDouble(), points[x]);
}
for (int x = 0; x <= a; x++) {
path.lineTo(-x.toDouble(), -points[x]);
}
return path;
}
@override
void paint(Canvas canvas, Rect rect, {TextDirection textDirection}) {
Path path = getOuterPath(rect.deflate(side.width / 2.0), textDirection: textDirection);
canvas.drawPath(path, side.toPaint());
}
}
I want to return the correct shape, but instead I get an Exception: Invalid value: Only valid value is 0: 200.
For some reason the variable a
isn't allowed to be 200? I don't know why, and changing it to 0 doesn't produce any errors, but then there is no shape either.
Does anyone know if there is a better way of doing this?
math dart

I want to create a superellipse shape in Flutter for a widget.
I found an article about creating superellipses written in python and java, but i can't quite get the code to work.
Link to article
class SuperEllipse extends ShapeBorder {
final BorderSide side;
final double n;
SuperEllipse({
@required this.n,
this.side = BorderSide.none,
}) : assert(side != null);
@override
EdgeInsetsGeometry get dimensions => EdgeInsets.all(side.width);
@override
ShapeBorder scale(double t) {
return SuperEllipse(
side: side.scale(t),
n: n,
);
}
@override
Path getInnerPath(Rect rect, {TextDirection textDirection}) {
return _superEllipsePath(rect, n);
}
@override
Path getOuterPath(Rect rect, {TextDirection textDirection}) {
return _superEllipsePath(rect, n);
}
static Path _superEllipsePath(Rect rect, double n) {
final int a = 200;
List<double> points = [a + 1.0];
Path path = new Path();
path.moveTo(a.toDouble(), 0);
// Calculate first quadrant.
for (int x = a; x >= 0; x--) {
points[x] = pow(pow(a, n) - pow(x, n), 1 / n);
path.lineTo(x.toDouble(), -points[x]);
}
// Mirror to other quadrants.
for (int x = 0; x <= a; x++) {
path.lineTo(x.toDouble(), points[x]);
}
for (int x = a; x >= 0; x--) {
path.lineTo(-x.toDouble(), points[x]);
}
for (int x = 0; x <= a; x++) {
path.lineTo(-x.toDouble(), -points[x]);
}
return path;
}
@override
void paint(Canvas canvas, Rect rect, {TextDirection textDirection}) {
Path path = getOuterPath(rect.deflate(side.width / 2.0), textDirection: textDirection);
canvas.drawPath(path, side.toPaint());
}
}
I want to return the correct shape, but instead I get an Exception: Invalid value: Only valid value is 0: 200.
For some reason the variable a
isn't allowed to be 200? I don't know why, and changing it to 0 doesn't produce any errors, but then there is no shape either.
Does anyone know if there is a better way of doing this?
math dart

math dart

asked Jan 2 at 11:32


Sander Dalby LarsenSander Dalby Larsen
13
13
see figma.com/blog/desperately-seeking-squircles
– pskink
Jan 2 at 12:00
I'm not sure how that article is helpful... I need help with my code, I understand what a superellipse and a squircle is.
– Sander Dalby Larsen
Jan 2 at 12:08
i though you really wanted the case where n >= 4
– pskink
Jan 2 at 12:17
That's not the problem, The problem is that I'm getting an Exception when I use the Shape
– Sander Dalby Larsen
Jan 2 at 12:19
ok so what is you exact stacktrace (3-4 top frames)?
– pskink
Jan 2 at 12:24
|
show 18 more comments
see figma.com/blog/desperately-seeking-squircles
– pskink
Jan 2 at 12:00
I'm not sure how that article is helpful... I need help with my code, I understand what a superellipse and a squircle is.
– Sander Dalby Larsen
Jan 2 at 12:08
i though you really wanted the case where n >= 4
– pskink
Jan 2 at 12:17
That's not the problem, The problem is that I'm getting an Exception when I use the Shape
– Sander Dalby Larsen
Jan 2 at 12:19
ok so what is you exact stacktrace (3-4 top frames)?
– pskink
Jan 2 at 12:24
see figma.com/blog/desperately-seeking-squircles
– pskink
Jan 2 at 12:00
see figma.com/blog/desperately-seeking-squircles
– pskink
Jan 2 at 12:00
I'm not sure how that article is helpful... I need help with my code, I understand what a superellipse and a squircle is.
– Sander Dalby Larsen
Jan 2 at 12:08
I'm not sure how that article is helpful... I need help with my code, I understand what a superellipse and a squircle is.
– Sander Dalby Larsen
Jan 2 at 12:08
i though you really wanted the case where n >= 4
– pskink
Jan 2 at 12:17
i though you really wanted the case where n >= 4
– pskink
Jan 2 at 12:17
That's not the problem, The problem is that I'm getting an Exception when I use the Shape
– Sander Dalby Larsen
Jan 2 at 12:19
That's not the problem, The problem is that I'm getting an Exception when I use the Shape
– Sander Dalby Larsen
Jan 2 at 12:19
ok so what is you exact stacktrace (3-4 top frames)?
– pskink
Jan 2 at 12:24
ok so what is you exact stacktrace (3-4 top frames)?
– pskink
Jan 2 at 12:24
|
show 18 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54005570%2fcalculating-superellipse-shape-in-dart%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54005570%2fcalculating-superellipse-shape-in-dart%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
w,J5ZeUyD 4e
see figma.com/blog/desperately-seeking-squircles
– pskink
Jan 2 at 12:00
I'm not sure how that article is helpful... I need help with my code, I understand what a superellipse and a squircle is.
– Sander Dalby Larsen
Jan 2 at 12:08
i though you really wanted the case where n >= 4
– pskink
Jan 2 at 12:17
That's not the problem, The problem is that I'm getting an Exception when I use the Shape
– Sander Dalby Larsen
Jan 2 at 12:19
ok so what is you exact stacktrace (3-4 top frames)?
– pskink
Jan 2 at 12:24