Camera “Bitmap imageBitmap = (Bitmap) extras.get(”data“);” gives Nullpointer error
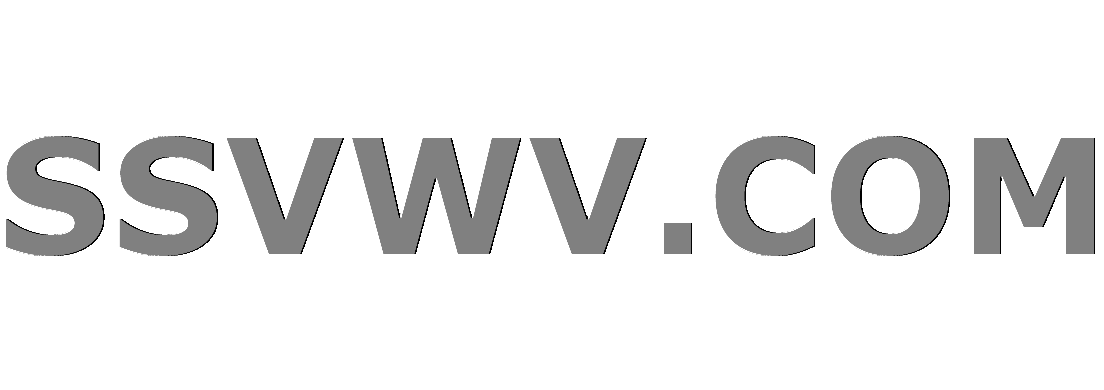
Multi tool use
I am following the Android developer tutorials on camera here: https://developer.android.com/training/camera/photobasics#java
I however get an error in the method
onActivityResult:,java.lang.NullPointerException: Attempt to invoke
virtual method 'java.lang.Object
android.os.Bundle.get(java.lang.String)' on a null object reference
This line is giving the error:
Bitmap imageBitmap = (Bitmap) extras.get("data");
Somehow when I comment out this line then the app works:
takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
My entire code is located here:
https://github.com/europa9/EanScannerForAndroid
MainActivity.java
package one.askit.eanscanner;
import android.Manifest;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.graphics.Bitmap;
import android.icu.text.SimpleDateFormat;
import android.net.Uri;
import android.os.Build;
import android.os.Bundle;
import android.os.Environment;
import android.provider.MediaStore;
import android.support.v4.content.FileProvider;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
import android.widget.FrameLayout;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import java.io.File;
import java.io.IOException;
import java.util.Date;
public class MainActivity extends AppCompatActivity {
static final int REQUEST_IMAGE_CAPTURE = 1;
static final int REQUEST_TAKE_PHOTO = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission
checkPermissionExternalRead();
checkPermissionExternalWrite();
checkPermissionCamera();
// Listeners
listeners();
FrameLayout frameLayoutCameraPreview = findViewById(R.id.frameLayoutCameraPreview);
frameLayoutCameraPreview.setVisibility(View.GONE);
}
/*- Check permission Read ------------------------------------------------------------------- */
// Pops up message to user for reading
private void checkPermissionExternalRead(){
int MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE = 1;
if (checkSelfPermission(android.Manifest.permission.READ_EXTERNAL_STORAGE)
!= PackageManager.PERMISSION_GRANTED) {
// Should we show an explanation?
if (shouldShowRequestPermissionRationale(
android.Manifest.permission.READ_EXTERNAL_STORAGE)) {
// Explain to the user why we need to read the contacts
}
requestPermissions(new String{android.Manifest.permission.READ_EXTERNAL_STORAGE},
MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE);
// MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE is an
// app-defined int constant that should be quite unique
return;
}
} // checkPermissionRead
/*- Check permission Write ------------------------------------------------------------------ */
// Pops up message to user for writing
private void checkPermissionExternalWrite(){
int MY_PERMISSIONS_REQUEST_WRITE_EXTERNAL_STORAGE = 1;
if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE)
!= PackageManager.PERMISSION_GRANTED) {
// Should we show an explanation?
if (shouldShowRequestPermissionRationale(
android.Manifest.permission.WRITE_EXTERNAL_STORAGE)) {
// Explain to the user why we need to read the contacts
}
requestPermissions(new String{android.Manifest.permission.WRITE_EXTERNAL_STORAGE},
MY_PERMISSIONS_REQUEST_WRITE_EXTERNAL_STORAGE);
// MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE is an
// app-defined int constant that should be quite unique
return;
}
} // checkPermissionWrite
/*- Check permission Camera ----------------------------------------------------------------- */
public void checkPermissionCamera(){
int MY_PERMISSIONS_REQUEST_CAMERA = 1;
if (checkSelfPermission(Manifest.permission.CAMERA)
!= PackageManager.PERMISSION_GRANTED) {
// Should we show an explanation?
if (shouldShowRequestPermissionRationale(
Manifest.permission.CAMERA)) {
// Explain to the user why we need to read the contacts
}
requestPermissions(new String{android.Manifest.permission.CAMERA},
MY_PERMISSIONS_REQUEST_CAMERA);
// MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE is an
// app-defined int constant that should be quite unique
return;
}
} // checkPermissionInternalRead
public void listeners(){
Button buttonScan = findViewById(R.id.buttonScan);
buttonScan.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
buttonScanClicked();
}
});
}
public void buttonScanClicked(){
// Scan text
TextView TextViewScan = findViewById(R.id.TextViewScan);
TextViewScan.setText("Now scanning");
// Take picture
dispatchTakePictureIntent();
}
private void dispatchTakePictureIntent() {
Intent picIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE).addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION | Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
String file_path = Environment.getExternalStorageDirectory().toString() +
"/" + this.getResources().getString(R.string.app_name);
File dir = new File(file_path);
if (!dir.exists())
dir.mkdirs();
// IMAGE_PATH = new File(dir, mContext.getResources().getString(R.string.app_name) + AppConstants.USER_ID + System.currentTimeMillis() + ".png");
File IMAGE_PATH = new File(dir, this.getResources().getString(R.string.app_name) + System.currentTimeMillis() + ".png");
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, FileProvider.getUriForFile(this, this.getPackageName() + ".fileprovider", IMAGE_PATH));
}
else {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, Uri.fromFile(IMAGE_PATH));
}
startActivityForResult(picIntent, REQUEST_TAKE_PHOTO);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == REQUEST_IMAGE_CAPTURE && resultCode == RESULT_OK) {
Bundle extras = data.getExtras();
if (extras != null) {
//Do your logic
Bitmap imageBitmap = (Bitmap) extras.get("data");
ImageView imageViewScanPreview = findViewById(R.id.imageViewScanPreview);
imageViewScanPreview.setImageBitmap(imageBitmap);
} else {
//Do something else
Toast.makeText(this, "Its null!", Toast.LENGTH_LONG).show();
}
}
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<FrameLayout
android:id="@+id/frameLayoutCameraPreview"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1" />
<ImageView
android:id="@+id/imageViewScanPreview"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:srcCompat="?attr/colorAccent" />
<TextView
android:id="@+id/TextViewScan"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Doint nothing"></TextView>
<Button
android:id="@+id/buttonScan"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="Scan" />
</LinearLayout>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="one.askit.eanscanner">
<uses-feature android:name="android.hardware.camera" android:required="true" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<provider
android:name="android.support.v4.content.FileProvider"
android:authorities="${applicationId}.fileprovider"
android:exported="false"
android:grantUriPermissions="true">
<meta-data
android:name="android.support.FILE_PROVIDER_PATHS"
android:resource="@xml/file_paths" />
</provider>
</application>
</manifest>
file_paths.xml
<?xml version="1.0" encoding="utf-8"?>
<paths xmlns:android="http://schemas.android.com/apk/res/android">
<external-path name="images" path="."/>
<external-path name="external_files" path="."/>
</paths>
java

add a comment |
I am following the Android developer tutorials on camera here: https://developer.android.com/training/camera/photobasics#java
I however get an error in the method
onActivityResult:,java.lang.NullPointerException: Attempt to invoke
virtual method 'java.lang.Object
android.os.Bundle.get(java.lang.String)' on a null object reference
This line is giving the error:
Bitmap imageBitmap = (Bitmap) extras.get("data");
Somehow when I comment out this line then the app works:
takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
My entire code is located here:
https://github.com/europa9/EanScannerForAndroid
MainActivity.java
package one.askit.eanscanner;
import android.Manifest;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.graphics.Bitmap;
import android.icu.text.SimpleDateFormat;
import android.net.Uri;
import android.os.Build;
import android.os.Bundle;
import android.os.Environment;
import android.provider.MediaStore;
import android.support.v4.content.FileProvider;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
import android.widget.FrameLayout;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import java.io.File;
import java.io.IOException;
import java.util.Date;
public class MainActivity extends AppCompatActivity {
static final int REQUEST_IMAGE_CAPTURE = 1;
static final int REQUEST_TAKE_PHOTO = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission
checkPermissionExternalRead();
checkPermissionExternalWrite();
checkPermissionCamera();
// Listeners
listeners();
FrameLayout frameLayoutCameraPreview = findViewById(R.id.frameLayoutCameraPreview);
frameLayoutCameraPreview.setVisibility(View.GONE);
}
/*- Check permission Read ------------------------------------------------------------------- */
// Pops up message to user for reading
private void checkPermissionExternalRead(){
int MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE = 1;
if (checkSelfPermission(android.Manifest.permission.READ_EXTERNAL_STORAGE)
!= PackageManager.PERMISSION_GRANTED) {
// Should we show an explanation?
if (shouldShowRequestPermissionRationale(
android.Manifest.permission.READ_EXTERNAL_STORAGE)) {
// Explain to the user why we need to read the contacts
}
requestPermissions(new String{android.Manifest.permission.READ_EXTERNAL_STORAGE},
MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE);
// MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE is an
// app-defined int constant that should be quite unique
return;
}
} // checkPermissionRead
/*- Check permission Write ------------------------------------------------------------------ */
// Pops up message to user for writing
private void checkPermissionExternalWrite(){
int MY_PERMISSIONS_REQUEST_WRITE_EXTERNAL_STORAGE = 1;
if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE)
!= PackageManager.PERMISSION_GRANTED) {
// Should we show an explanation?
if (shouldShowRequestPermissionRationale(
android.Manifest.permission.WRITE_EXTERNAL_STORAGE)) {
// Explain to the user why we need to read the contacts
}
requestPermissions(new String{android.Manifest.permission.WRITE_EXTERNAL_STORAGE},
MY_PERMISSIONS_REQUEST_WRITE_EXTERNAL_STORAGE);
// MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE is an
// app-defined int constant that should be quite unique
return;
}
} // checkPermissionWrite
/*- Check permission Camera ----------------------------------------------------------------- */
public void checkPermissionCamera(){
int MY_PERMISSIONS_REQUEST_CAMERA = 1;
if (checkSelfPermission(Manifest.permission.CAMERA)
!= PackageManager.PERMISSION_GRANTED) {
// Should we show an explanation?
if (shouldShowRequestPermissionRationale(
Manifest.permission.CAMERA)) {
// Explain to the user why we need to read the contacts
}
requestPermissions(new String{android.Manifest.permission.CAMERA},
MY_PERMISSIONS_REQUEST_CAMERA);
// MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE is an
// app-defined int constant that should be quite unique
return;
}
} // checkPermissionInternalRead
public void listeners(){
Button buttonScan = findViewById(R.id.buttonScan);
buttonScan.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
buttonScanClicked();
}
});
}
public void buttonScanClicked(){
// Scan text
TextView TextViewScan = findViewById(R.id.TextViewScan);
TextViewScan.setText("Now scanning");
// Take picture
dispatchTakePictureIntent();
}
private void dispatchTakePictureIntent() {
Intent picIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE).addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION | Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
String file_path = Environment.getExternalStorageDirectory().toString() +
"/" + this.getResources().getString(R.string.app_name);
File dir = new File(file_path);
if (!dir.exists())
dir.mkdirs();
// IMAGE_PATH = new File(dir, mContext.getResources().getString(R.string.app_name) + AppConstants.USER_ID + System.currentTimeMillis() + ".png");
File IMAGE_PATH = new File(dir, this.getResources().getString(R.string.app_name) + System.currentTimeMillis() + ".png");
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, FileProvider.getUriForFile(this, this.getPackageName() + ".fileprovider", IMAGE_PATH));
}
else {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, Uri.fromFile(IMAGE_PATH));
}
startActivityForResult(picIntent, REQUEST_TAKE_PHOTO);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == REQUEST_IMAGE_CAPTURE && resultCode == RESULT_OK) {
Bundle extras = data.getExtras();
if (extras != null) {
//Do your logic
Bitmap imageBitmap = (Bitmap) extras.get("data");
ImageView imageViewScanPreview = findViewById(R.id.imageViewScanPreview);
imageViewScanPreview.setImageBitmap(imageBitmap);
} else {
//Do something else
Toast.makeText(this, "Its null!", Toast.LENGTH_LONG).show();
}
}
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<FrameLayout
android:id="@+id/frameLayoutCameraPreview"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1" />
<ImageView
android:id="@+id/imageViewScanPreview"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:srcCompat="?attr/colorAccent" />
<TextView
android:id="@+id/TextViewScan"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Doint nothing"></TextView>
<Button
android:id="@+id/buttonScan"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="Scan" />
</LinearLayout>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="one.askit.eanscanner">
<uses-feature android:name="android.hardware.camera" android:required="true" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<provider
android:name="android.support.v4.content.FileProvider"
android:authorities="${applicationId}.fileprovider"
android:exported="false"
android:grantUriPermissions="true">
<meta-data
android:name="android.support.FILE_PROVIDER_PATHS"
android:resource="@xml/file_paths" />
</provider>
</application>
</manifest>
file_paths.xml
<?xml version="1.0" encoding="utf-8"?>
<paths xmlns:android="http://schemas.android.com/apk/res/android">
<external-path name="images" path="."/>
<external-path name="external_files" path="."/>
</paths>
java

as you are using apps private folder to store your images you need to use<files-path>
instead of<external-path>
– Karan Mer
Jan 2 at 11:53
Duplicate of What is a NullPointerException, and how do I fix it?
– Fantômas
Jan 2 at 13:43
add a comment |
I am following the Android developer tutorials on camera here: https://developer.android.com/training/camera/photobasics#java
I however get an error in the method
onActivityResult:,java.lang.NullPointerException: Attempt to invoke
virtual method 'java.lang.Object
android.os.Bundle.get(java.lang.String)' on a null object reference
This line is giving the error:
Bitmap imageBitmap = (Bitmap) extras.get("data");
Somehow when I comment out this line then the app works:
takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
My entire code is located here:
https://github.com/europa9/EanScannerForAndroid
MainActivity.java
package one.askit.eanscanner;
import android.Manifest;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.graphics.Bitmap;
import android.icu.text.SimpleDateFormat;
import android.net.Uri;
import android.os.Build;
import android.os.Bundle;
import android.os.Environment;
import android.provider.MediaStore;
import android.support.v4.content.FileProvider;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
import android.widget.FrameLayout;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import java.io.File;
import java.io.IOException;
import java.util.Date;
public class MainActivity extends AppCompatActivity {
static final int REQUEST_IMAGE_CAPTURE = 1;
static final int REQUEST_TAKE_PHOTO = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission
checkPermissionExternalRead();
checkPermissionExternalWrite();
checkPermissionCamera();
// Listeners
listeners();
FrameLayout frameLayoutCameraPreview = findViewById(R.id.frameLayoutCameraPreview);
frameLayoutCameraPreview.setVisibility(View.GONE);
}
/*- Check permission Read ------------------------------------------------------------------- */
// Pops up message to user for reading
private void checkPermissionExternalRead(){
int MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE = 1;
if (checkSelfPermission(android.Manifest.permission.READ_EXTERNAL_STORAGE)
!= PackageManager.PERMISSION_GRANTED) {
// Should we show an explanation?
if (shouldShowRequestPermissionRationale(
android.Manifest.permission.READ_EXTERNAL_STORAGE)) {
// Explain to the user why we need to read the contacts
}
requestPermissions(new String{android.Manifest.permission.READ_EXTERNAL_STORAGE},
MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE);
// MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE is an
// app-defined int constant that should be quite unique
return;
}
} // checkPermissionRead
/*- Check permission Write ------------------------------------------------------------------ */
// Pops up message to user for writing
private void checkPermissionExternalWrite(){
int MY_PERMISSIONS_REQUEST_WRITE_EXTERNAL_STORAGE = 1;
if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE)
!= PackageManager.PERMISSION_GRANTED) {
// Should we show an explanation?
if (shouldShowRequestPermissionRationale(
android.Manifest.permission.WRITE_EXTERNAL_STORAGE)) {
// Explain to the user why we need to read the contacts
}
requestPermissions(new String{android.Manifest.permission.WRITE_EXTERNAL_STORAGE},
MY_PERMISSIONS_REQUEST_WRITE_EXTERNAL_STORAGE);
// MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE is an
// app-defined int constant that should be quite unique
return;
}
} // checkPermissionWrite
/*- Check permission Camera ----------------------------------------------------------------- */
public void checkPermissionCamera(){
int MY_PERMISSIONS_REQUEST_CAMERA = 1;
if (checkSelfPermission(Manifest.permission.CAMERA)
!= PackageManager.PERMISSION_GRANTED) {
// Should we show an explanation?
if (shouldShowRequestPermissionRationale(
Manifest.permission.CAMERA)) {
// Explain to the user why we need to read the contacts
}
requestPermissions(new String{android.Manifest.permission.CAMERA},
MY_PERMISSIONS_REQUEST_CAMERA);
// MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE is an
// app-defined int constant that should be quite unique
return;
}
} // checkPermissionInternalRead
public void listeners(){
Button buttonScan = findViewById(R.id.buttonScan);
buttonScan.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
buttonScanClicked();
}
});
}
public void buttonScanClicked(){
// Scan text
TextView TextViewScan = findViewById(R.id.TextViewScan);
TextViewScan.setText("Now scanning");
// Take picture
dispatchTakePictureIntent();
}
private void dispatchTakePictureIntent() {
Intent picIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE).addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION | Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
String file_path = Environment.getExternalStorageDirectory().toString() +
"/" + this.getResources().getString(R.string.app_name);
File dir = new File(file_path);
if (!dir.exists())
dir.mkdirs();
// IMAGE_PATH = new File(dir, mContext.getResources().getString(R.string.app_name) + AppConstants.USER_ID + System.currentTimeMillis() + ".png");
File IMAGE_PATH = new File(dir, this.getResources().getString(R.string.app_name) + System.currentTimeMillis() + ".png");
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, FileProvider.getUriForFile(this, this.getPackageName() + ".fileprovider", IMAGE_PATH));
}
else {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, Uri.fromFile(IMAGE_PATH));
}
startActivityForResult(picIntent, REQUEST_TAKE_PHOTO);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == REQUEST_IMAGE_CAPTURE && resultCode == RESULT_OK) {
Bundle extras = data.getExtras();
if (extras != null) {
//Do your logic
Bitmap imageBitmap = (Bitmap) extras.get("data");
ImageView imageViewScanPreview = findViewById(R.id.imageViewScanPreview);
imageViewScanPreview.setImageBitmap(imageBitmap);
} else {
//Do something else
Toast.makeText(this, "Its null!", Toast.LENGTH_LONG).show();
}
}
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<FrameLayout
android:id="@+id/frameLayoutCameraPreview"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1" />
<ImageView
android:id="@+id/imageViewScanPreview"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:srcCompat="?attr/colorAccent" />
<TextView
android:id="@+id/TextViewScan"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Doint nothing"></TextView>
<Button
android:id="@+id/buttonScan"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="Scan" />
</LinearLayout>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="one.askit.eanscanner">
<uses-feature android:name="android.hardware.camera" android:required="true" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<provider
android:name="android.support.v4.content.FileProvider"
android:authorities="${applicationId}.fileprovider"
android:exported="false"
android:grantUriPermissions="true">
<meta-data
android:name="android.support.FILE_PROVIDER_PATHS"
android:resource="@xml/file_paths" />
</provider>
</application>
</manifest>
file_paths.xml
<?xml version="1.0" encoding="utf-8"?>
<paths xmlns:android="http://schemas.android.com/apk/res/android">
<external-path name="images" path="."/>
<external-path name="external_files" path="."/>
</paths>
java

I am following the Android developer tutorials on camera here: https://developer.android.com/training/camera/photobasics#java
I however get an error in the method
onActivityResult:,java.lang.NullPointerException: Attempt to invoke
virtual method 'java.lang.Object
android.os.Bundle.get(java.lang.String)' on a null object reference
This line is giving the error:
Bitmap imageBitmap = (Bitmap) extras.get("data");
Somehow when I comment out this line then the app works:
takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
My entire code is located here:
https://github.com/europa9/EanScannerForAndroid
MainActivity.java
package one.askit.eanscanner;
import android.Manifest;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.graphics.Bitmap;
import android.icu.text.SimpleDateFormat;
import android.net.Uri;
import android.os.Build;
import android.os.Bundle;
import android.os.Environment;
import android.provider.MediaStore;
import android.support.v4.content.FileProvider;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
import android.widget.FrameLayout;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import java.io.File;
import java.io.IOException;
import java.util.Date;
public class MainActivity extends AppCompatActivity {
static final int REQUEST_IMAGE_CAPTURE = 1;
static final int REQUEST_TAKE_PHOTO = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission
checkPermissionExternalRead();
checkPermissionExternalWrite();
checkPermissionCamera();
// Listeners
listeners();
FrameLayout frameLayoutCameraPreview = findViewById(R.id.frameLayoutCameraPreview);
frameLayoutCameraPreview.setVisibility(View.GONE);
}
/*- Check permission Read ------------------------------------------------------------------- */
// Pops up message to user for reading
private void checkPermissionExternalRead(){
int MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE = 1;
if (checkSelfPermission(android.Manifest.permission.READ_EXTERNAL_STORAGE)
!= PackageManager.PERMISSION_GRANTED) {
// Should we show an explanation?
if (shouldShowRequestPermissionRationale(
android.Manifest.permission.READ_EXTERNAL_STORAGE)) {
// Explain to the user why we need to read the contacts
}
requestPermissions(new String{android.Manifest.permission.READ_EXTERNAL_STORAGE},
MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE);
// MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE is an
// app-defined int constant that should be quite unique
return;
}
} // checkPermissionRead
/*- Check permission Write ------------------------------------------------------------------ */
// Pops up message to user for writing
private void checkPermissionExternalWrite(){
int MY_PERMISSIONS_REQUEST_WRITE_EXTERNAL_STORAGE = 1;
if (checkSelfPermission(Manifest.permission.WRITE_EXTERNAL_STORAGE)
!= PackageManager.PERMISSION_GRANTED) {
// Should we show an explanation?
if (shouldShowRequestPermissionRationale(
android.Manifest.permission.WRITE_EXTERNAL_STORAGE)) {
// Explain to the user why we need to read the contacts
}
requestPermissions(new String{android.Manifest.permission.WRITE_EXTERNAL_STORAGE},
MY_PERMISSIONS_REQUEST_WRITE_EXTERNAL_STORAGE);
// MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE is an
// app-defined int constant that should be quite unique
return;
}
} // checkPermissionWrite
/*- Check permission Camera ----------------------------------------------------------------- */
public void checkPermissionCamera(){
int MY_PERMISSIONS_REQUEST_CAMERA = 1;
if (checkSelfPermission(Manifest.permission.CAMERA)
!= PackageManager.PERMISSION_GRANTED) {
// Should we show an explanation?
if (shouldShowRequestPermissionRationale(
Manifest.permission.CAMERA)) {
// Explain to the user why we need to read the contacts
}
requestPermissions(new String{android.Manifest.permission.CAMERA},
MY_PERMISSIONS_REQUEST_CAMERA);
// MY_PERMISSIONS_REQUEST_READ_EXTERNAL_STORAGE is an
// app-defined int constant that should be quite unique
return;
}
} // checkPermissionInternalRead
public void listeners(){
Button buttonScan = findViewById(R.id.buttonScan);
buttonScan.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
buttonScanClicked();
}
});
}
public void buttonScanClicked(){
// Scan text
TextView TextViewScan = findViewById(R.id.TextViewScan);
TextViewScan.setText("Now scanning");
// Take picture
dispatchTakePictureIntent();
}
private void dispatchTakePictureIntent() {
Intent picIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE).addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION | Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
String file_path = Environment.getExternalStorageDirectory().toString() +
"/" + this.getResources().getString(R.string.app_name);
File dir = new File(file_path);
if (!dir.exists())
dir.mkdirs();
// IMAGE_PATH = new File(dir, mContext.getResources().getString(R.string.app_name) + AppConstants.USER_ID + System.currentTimeMillis() + ".png");
File IMAGE_PATH = new File(dir, this.getResources().getString(R.string.app_name) + System.currentTimeMillis() + ".png");
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, FileProvider.getUriForFile(this, this.getPackageName() + ".fileprovider", IMAGE_PATH));
}
else {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, Uri.fromFile(IMAGE_PATH));
}
startActivityForResult(picIntent, REQUEST_TAKE_PHOTO);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == REQUEST_IMAGE_CAPTURE && resultCode == RESULT_OK) {
Bundle extras = data.getExtras();
if (extras != null) {
//Do your logic
Bitmap imageBitmap = (Bitmap) extras.get("data");
ImageView imageViewScanPreview = findViewById(R.id.imageViewScanPreview);
imageViewScanPreview.setImageBitmap(imageBitmap);
} else {
//Do something else
Toast.makeText(this, "Its null!", Toast.LENGTH_LONG).show();
}
}
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<FrameLayout
android:id="@+id/frameLayoutCameraPreview"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1" />
<ImageView
android:id="@+id/imageViewScanPreview"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:srcCompat="?attr/colorAccent" />
<TextView
android:id="@+id/TextViewScan"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Doint nothing"></TextView>
<Button
android:id="@+id/buttonScan"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="Scan" />
</LinearLayout>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="one.askit.eanscanner">
<uses-feature android:name="android.hardware.camera" android:required="true" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<provider
android:name="android.support.v4.content.FileProvider"
android:authorities="${applicationId}.fileprovider"
android:exported="false"
android:grantUriPermissions="true">
<meta-data
android:name="android.support.FILE_PROVIDER_PATHS"
android:resource="@xml/file_paths" />
</provider>
</application>
</manifest>
file_paths.xml
<?xml version="1.0" encoding="utf-8"?>
<paths xmlns:android="http://schemas.android.com/apk/res/android">
<external-path name="images" path="."/>
<external-path name="external_files" path="."/>
</paths>
java

java

edited Jan 2 at 13:41


Fantômas
32.8k156390
32.8k156390
asked Jan 2 at 11:32
EuropaEuropa
768
768
as you are using apps private folder to store your images you need to use<files-path>
instead of<external-path>
– Karan Mer
Jan 2 at 11:53
Duplicate of What is a NullPointerException, and how do I fix it?
– Fantômas
Jan 2 at 13:43
add a comment |
as you are using apps private folder to store your images you need to use<files-path>
instead of<external-path>
– Karan Mer
Jan 2 at 11:53
Duplicate of What is a NullPointerException, and how do I fix it?
– Fantômas
Jan 2 at 13:43
as you are using apps private folder to store your images you need to use
<files-path>
instead of <external-path>
– Karan Mer
Jan 2 at 11:53
as you are using apps private folder to store your images you need to use
<files-path>
instead of <external-path>
– Karan Mer
Jan 2 at 11:53
Duplicate of What is a NullPointerException, and how do I fix it?
– Fantômas
Jan 2 at 13:43
Duplicate of What is a NullPointerException, and how do I fix it?
– Fantômas
Jan 2 at 13:43
add a comment |
2 Answers
2
active
oldest
votes
Change your file provider
like below
<provider
android:name="android.support.v4.content.FileProvider"
android:authorities="${applicationId}.fileprovider"
android:exported="false"
android:grantUriPermissions="true">
<meta-data
android:name="android.support.FILE_PROVIDER_PATHS"
android:resource="@xml/file_paths" />
</provider>
And your file_path.xml be like below
<?xml version="1.0" encoding="utf-8"?>
<paths xmlns:android="http://schemas.android.com/apk/res/android">
<external-path name="images" path="."/>
<external-path name="external_files" path="."/>
</paths>
For image capture use this Intent
Intent picIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE).addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION | Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
String file_path = Environment.getExternalStorageDirectory().toString() +
"/" + mContext.getResources().getString(R.string.app_name);
File dir = new File(file_path);
if (!dir.exists())
dir.mkdirs();
// IMAGE_PATH = new File(dir, mContext.getResources().getString(R.string.app_name) + AppConstants.USER_ID + System.currentTimeMillis() + ".png");
IMAGE_PATH = new File(dir, mContext.getResources().getString(R.string.app_name) + System.currentTimeMillis() + ".png");
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, FileProvider.getUriForFile(mContext, mContext.getPackageName()+".fileprovider", IMAGE_PATH));
}
else {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, Uri.fromFile(IMAGE_PATH));
}
((Activity) mContext).startActivityForResult(picIntent, CAMERA_REQUEST);
And inside the onActivityREsult
, you need to change the code as follow
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
int cropperType = 1;
if (requestCode == REQUEST_IMAGE_CAPTURE) {
switch (resultCode) {
case Activity.RESULT_OK:
String imagePAth= Uri.fromFile(IMAGE_PATH);
GlideApp.with(this).load(imagePAth).diskCacheStrategy(DiskCacheStrategy.ALL).skipMemoryCache(false).
placeholder(R.drawable.default_picture).error(R.drawable.default_picture).dontAnimate().into(YOUR_IMAGEVIEW);
break;
case Activity.RESULT_CANCELED:
break;
}
}
}
And if you want the Bitmap
from the onActivityResult
than you need to refer this link Click here
"Bundle extras = data.getExtras();" is still null after taking a picture.
– Europa
Jan 2 at 11:40
Have u change the provider ,as i have suggested
– Ravindra Kushwaha
Jan 2 at 11:40
Yes. I have updated them. When I comment out this line then the app works: "takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);"
– Europa
Jan 2 at 11:42
1
Wait i am updating naswer
– Ravindra Kushwaha
Jan 2 at 11:42
1
Happy to help you dost @Europa ..Keep happy coding
– Ravindra Kushwaha
Jan 2 at 13:06
|
show 11 more comments
There are different ways to receive a bitmap from the camera.
You are trying to get a Bitmap from the extras that is not there. Since you are casting the null to Bitmap the error happens at the moment of accessing the data and not the moment you try to work with the bitmap.
If your are supplying a output URI via takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
most Android phones will store the photo at this URI and you have to access the bitmap through the file that was stored there. If you remove the URI the photo is supplied in the extra in the Intent, that is why the code works if you remove that line.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54005582%2fcamera-bitmap-imagebitmap-bitmap-extras-getdata-gives-nullpointer-err%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Change your file provider
like below
<provider
android:name="android.support.v4.content.FileProvider"
android:authorities="${applicationId}.fileprovider"
android:exported="false"
android:grantUriPermissions="true">
<meta-data
android:name="android.support.FILE_PROVIDER_PATHS"
android:resource="@xml/file_paths" />
</provider>
And your file_path.xml be like below
<?xml version="1.0" encoding="utf-8"?>
<paths xmlns:android="http://schemas.android.com/apk/res/android">
<external-path name="images" path="."/>
<external-path name="external_files" path="."/>
</paths>
For image capture use this Intent
Intent picIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE).addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION | Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
String file_path = Environment.getExternalStorageDirectory().toString() +
"/" + mContext.getResources().getString(R.string.app_name);
File dir = new File(file_path);
if (!dir.exists())
dir.mkdirs();
// IMAGE_PATH = new File(dir, mContext.getResources().getString(R.string.app_name) + AppConstants.USER_ID + System.currentTimeMillis() + ".png");
IMAGE_PATH = new File(dir, mContext.getResources().getString(R.string.app_name) + System.currentTimeMillis() + ".png");
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, FileProvider.getUriForFile(mContext, mContext.getPackageName()+".fileprovider", IMAGE_PATH));
}
else {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, Uri.fromFile(IMAGE_PATH));
}
((Activity) mContext).startActivityForResult(picIntent, CAMERA_REQUEST);
And inside the onActivityREsult
, you need to change the code as follow
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
int cropperType = 1;
if (requestCode == REQUEST_IMAGE_CAPTURE) {
switch (resultCode) {
case Activity.RESULT_OK:
String imagePAth= Uri.fromFile(IMAGE_PATH);
GlideApp.with(this).load(imagePAth).diskCacheStrategy(DiskCacheStrategy.ALL).skipMemoryCache(false).
placeholder(R.drawable.default_picture).error(R.drawable.default_picture).dontAnimate().into(YOUR_IMAGEVIEW);
break;
case Activity.RESULT_CANCELED:
break;
}
}
}
And if you want the Bitmap
from the onActivityResult
than you need to refer this link Click here
"Bundle extras = data.getExtras();" is still null after taking a picture.
– Europa
Jan 2 at 11:40
Have u change the provider ,as i have suggested
– Ravindra Kushwaha
Jan 2 at 11:40
Yes. I have updated them. When I comment out this line then the app works: "takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);"
– Europa
Jan 2 at 11:42
1
Wait i am updating naswer
– Ravindra Kushwaha
Jan 2 at 11:42
1
Happy to help you dost @Europa ..Keep happy coding
– Ravindra Kushwaha
Jan 2 at 13:06
|
show 11 more comments
Change your file provider
like below
<provider
android:name="android.support.v4.content.FileProvider"
android:authorities="${applicationId}.fileprovider"
android:exported="false"
android:grantUriPermissions="true">
<meta-data
android:name="android.support.FILE_PROVIDER_PATHS"
android:resource="@xml/file_paths" />
</provider>
And your file_path.xml be like below
<?xml version="1.0" encoding="utf-8"?>
<paths xmlns:android="http://schemas.android.com/apk/res/android">
<external-path name="images" path="."/>
<external-path name="external_files" path="."/>
</paths>
For image capture use this Intent
Intent picIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE).addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION | Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
String file_path = Environment.getExternalStorageDirectory().toString() +
"/" + mContext.getResources().getString(R.string.app_name);
File dir = new File(file_path);
if (!dir.exists())
dir.mkdirs();
// IMAGE_PATH = new File(dir, mContext.getResources().getString(R.string.app_name) + AppConstants.USER_ID + System.currentTimeMillis() + ".png");
IMAGE_PATH = new File(dir, mContext.getResources().getString(R.string.app_name) + System.currentTimeMillis() + ".png");
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, FileProvider.getUriForFile(mContext, mContext.getPackageName()+".fileprovider", IMAGE_PATH));
}
else {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, Uri.fromFile(IMAGE_PATH));
}
((Activity) mContext).startActivityForResult(picIntent, CAMERA_REQUEST);
And inside the onActivityREsult
, you need to change the code as follow
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
int cropperType = 1;
if (requestCode == REQUEST_IMAGE_CAPTURE) {
switch (resultCode) {
case Activity.RESULT_OK:
String imagePAth= Uri.fromFile(IMAGE_PATH);
GlideApp.with(this).load(imagePAth).diskCacheStrategy(DiskCacheStrategy.ALL).skipMemoryCache(false).
placeholder(R.drawable.default_picture).error(R.drawable.default_picture).dontAnimate().into(YOUR_IMAGEVIEW);
break;
case Activity.RESULT_CANCELED:
break;
}
}
}
And if you want the Bitmap
from the onActivityResult
than you need to refer this link Click here
"Bundle extras = data.getExtras();" is still null after taking a picture.
– Europa
Jan 2 at 11:40
Have u change the provider ,as i have suggested
– Ravindra Kushwaha
Jan 2 at 11:40
Yes. I have updated them. When I comment out this line then the app works: "takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);"
– Europa
Jan 2 at 11:42
1
Wait i am updating naswer
– Ravindra Kushwaha
Jan 2 at 11:42
1
Happy to help you dost @Europa ..Keep happy coding
– Ravindra Kushwaha
Jan 2 at 13:06
|
show 11 more comments
Change your file provider
like below
<provider
android:name="android.support.v4.content.FileProvider"
android:authorities="${applicationId}.fileprovider"
android:exported="false"
android:grantUriPermissions="true">
<meta-data
android:name="android.support.FILE_PROVIDER_PATHS"
android:resource="@xml/file_paths" />
</provider>
And your file_path.xml be like below
<?xml version="1.0" encoding="utf-8"?>
<paths xmlns:android="http://schemas.android.com/apk/res/android">
<external-path name="images" path="."/>
<external-path name="external_files" path="."/>
</paths>
For image capture use this Intent
Intent picIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE).addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION | Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
String file_path = Environment.getExternalStorageDirectory().toString() +
"/" + mContext.getResources().getString(R.string.app_name);
File dir = new File(file_path);
if (!dir.exists())
dir.mkdirs();
// IMAGE_PATH = new File(dir, mContext.getResources().getString(R.string.app_name) + AppConstants.USER_ID + System.currentTimeMillis() + ".png");
IMAGE_PATH = new File(dir, mContext.getResources().getString(R.string.app_name) + System.currentTimeMillis() + ".png");
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, FileProvider.getUriForFile(mContext, mContext.getPackageName()+".fileprovider", IMAGE_PATH));
}
else {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, Uri.fromFile(IMAGE_PATH));
}
((Activity) mContext).startActivityForResult(picIntent, CAMERA_REQUEST);
And inside the onActivityREsult
, you need to change the code as follow
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
int cropperType = 1;
if (requestCode == REQUEST_IMAGE_CAPTURE) {
switch (resultCode) {
case Activity.RESULT_OK:
String imagePAth= Uri.fromFile(IMAGE_PATH);
GlideApp.with(this).load(imagePAth).diskCacheStrategy(DiskCacheStrategy.ALL).skipMemoryCache(false).
placeholder(R.drawable.default_picture).error(R.drawable.default_picture).dontAnimate().into(YOUR_IMAGEVIEW);
break;
case Activity.RESULT_CANCELED:
break;
}
}
}
And if you want the Bitmap
from the onActivityResult
than you need to refer this link Click here
Change your file provider
like below
<provider
android:name="android.support.v4.content.FileProvider"
android:authorities="${applicationId}.fileprovider"
android:exported="false"
android:grantUriPermissions="true">
<meta-data
android:name="android.support.FILE_PROVIDER_PATHS"
android:resource="@xml/file_paths" />
</provider>
And your file_path.xml be like below
<?xml version="1.0" encoding="utf-8"?>
<paths xmlns:android="http://schemas.android.com/apk/res/android">
<external-path name="images" path="."/>
<external-path name="external_files" path="."/>
</paths>
For image capture use this Intent
Intent picIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE).addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION | Intent.FLAG_GRANT_WRITE_URI_PERMISSION);
String file_path = Environment.getExternalStorageDirectory().toString() +
"/" + mContext.getResources().getString(R.string.app_name);
File dir = new File(file_path);
if (!dir.exists())
dir.mkdirs();
// IMAGE_PATH = new File(dir, mContext.getResources().getString(R.string.app_name) + AppConstants.USER_ID + System.currentTimeMillis() + ".png");
IMAGE_PATH = new File(dir, mContext.getResources().getString(R.string.app_name) + System.currentTimeMillis() + ".png");
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, FileProvider.getUriForFile(mContext, mContext.getPackageName()+".fileprovider", IMAGE_PATH));
}
else {
picIntent.putExtra(MediaStore.EXTRA_OUTPUT, Uri.fromFile(IMAGE_PATH));
}
((Activity) mContext).startActivityForResult(picIntent, CAMERA_REQUEST);
And inside the onActivityREsult
, you need to change the code as follow
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
int cropperType = 1;
if (requestCode == REQUEST_IMAGE_CAPTURE) {
switch (resultCode) {
case Activity.RESULT_OK:
String imagePAth= Uri.fromFile(IMAGE_PATH);
GlideApp.with(this).load(imagePAth).diskCacheStrategy(DiskCacheStrategy.ALL).skipMemoryCache(false).
placeholder(R.drawable.default_picture).error(R.drawable.default_picture).dontAnimate().into(YOUR_IMAGEVIEW);
break;
case Activity.RESULT_CANCELED:
break;
}
}
}
And if you want the Bitmap
from the onActivityResult
than you need to refer this link Click here
edited Jan 2 at 12:35
answered Jan 2 at 11:38


Ravindra KushwahaRavindra Kushwaha
3,56652562
3,56652562
"Bundle extras = data.getExtras();" is still null after taking a picture.
– Europa
Jan 2 at 11:40
Have u change the provider ,as i have suggested
– Ravindra Kushwaha
Jan 2 at 11:40
Yes. I have updated them. When I comment out this line then the app works: "takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);"
– Europa
Jan 2 at 11:42
1
Wait i am updating naswer
– Ravindra Kushwaha
Jan 2 at 11:42
1
Happy to help you dost @Europa ..Keep happy coding
– Ravindra Kushwaha
Jan 2 at 13:06
|
show 11 more comments
"Bundle extras = data.getExtras();" is still null after taking a picture.
– Europa
Jan 2 at 11:40
Have u change the provider ,as i have suggested
– Ravindra Kushwaha
Jan 2 at 11:40
Yes. I have updated them. When I comment out this line then the app works: "takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);"
– Europa
Jan 2 at 11:42
1
Wait i am updating naswer
– Ravindra Kushwaha
Jan 2 at 11:42
1
Happy to help you dost @Europa ..Keep happy coding
– Ravindra Kushwaha
Jan 2 at 13:06
"Bundle extras = data.getExtras();" is still null after taking a picture.
– Europa
Jan 2 at 11:40
"Bundle extras = data.getExtras();" is still null after taking a picture.
– Europa
Jan 2 at 11:40
Have u change the provider ,as i have suggested
– Ravindra Kushwaha
Jan 2 at 11:40
Have u change the provider ,as i have suggested
– Ravindra Kushwaha
Jan 2 at 11:40
Yes. I have updated them. When I comment out this line then the app works: "takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);"
– Europa
Jan 2 at 11:42
Yes. I have updated them. When I comment out this line then the app works: "takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);"
– Europa
Jan 2 at 11:42
1
1
Wait i am updating naswer
– Ravindra Kushwaha
Jan 2 at 11:42
Wait i am updating naswer
– Ravindra Kushwaha
Jan 2 at 11:42
1
1
Happy to help you dost @Europa ..Keep happy coding
– Ravindra Kushwaha
Jan 2 at 13:06
Happy to help you dost @Europa ..Keep happy coding
– Ravindra Kushwaha
Jan 2 at 13:06
|
show 11 more comments
There are different ways to receive a bitmap from the camera.
You are trying to get a Bitmap from the extras that is not there. Since you are casting the null to Bitmap the error happens at the moment of accessing the data and not the moment you try to work with the bitmap.
If your are supplying a output URI via takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
most Android phones will store the photo at this URI and you have to access the bitmap through the file that was stored there. If you remove the URI the photo is supplied in the extra in the Intent, that is why the code works if you remove that line.
add a comment |
There are different ways to receive a bitmap from the camera.
You are trying to get a Bitmap from the extras that is not there. Since you are casting the null to Bitmap the error happens at the moment of accessing the data and not the moment you try to work with the bitmap.
If your are supplying a output URI via takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
most Android phones will store the photo at this URI and you have to access the bitmap through the file that was stored there. If you remove the URI the photo is supplied in the extra in the Intent, that is why the code works if you remove that line.
add a comment |
There are different ways to receive a bitmap from the camera.
You are trying to get a Bitmap from the extras that is not there. Since you are casting the null to Bitmap the error happens at the moment of accessing the data and not the moment you try to work with the bitmap.
If your are supplying a output URI via takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
most Android phones will store the photo at this URI and you have to access the bitmap through the file that was stored there. If you remove the URI the photo is supplied in the extra in the Intent, that is why the code works if you remove that line.
There are different ways to receive a bitmap from the camera.
You are trying to get a Bitmap from the extras that is not there. Since you are casting the null to Bitmap the error happens at the moment of accessing the data and not the moment you try to work with the bitmap.
If your are supplying a output URI via takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
most Android phones will store the photo at this URI and you have to access the bitmap through the file that was stored there. If you remove the URI the photo is supplied in the extra in the Intent, that is why the code works if you remove that line.
answered Jan 2 at 12:33


JanuszJanusz
107k103279355
107k103279355
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54005582%2fcamera-bitmap-imagebitmap-bitmap-extras-getdata-gives-nullpointer-err%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Fi G4Wi,1QBhEhq5e2FkbfUplj8T2fueMXG7bQ8TYqtB Qn
as you are using apps private folder to store your images you need to use
<files-path>
instead of<external-path>
– Karan Mer
Jan 2 at 11:53
Duplicate of What is a NullPointerException, and how do I fix it?
– Fantômas
Jan 2 at 13:43