Python - Where is the space at the end of the string coming from?
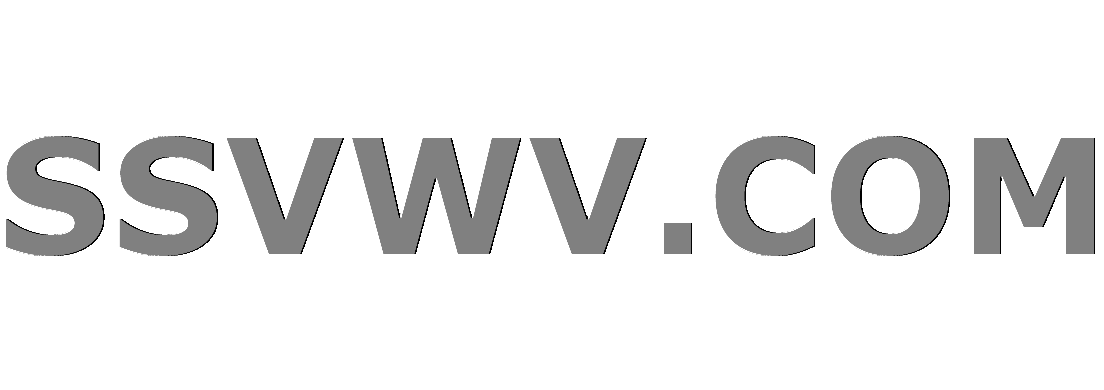
Multi tool use
TL;DR - what generates a white space at the end of a string stored in a set?
Problem description:
Imagine writing a script to crawl trough a directory and act on specific changes to the directory mentioned in a filter file.
Due to some architectural constraints, you choose to have the script map the dir structure and store it in a text file line by line. Example:
./Test
./Test/foo
./Test/foo/bar
Then, when you run the script again, it will compare the new text file generated on the nth run to the old text file from the n-1 run. If there were any changes, it will act on them by comparing the changes to the filter text file which holds directories of interest.
When the script is run, however, it fails. Upon debugging, it turns out that a white space was added just before the end of the strings stored in the "changes" set as follows:
changed_path = {str}'.\Test\foo\bar n' # Detected Path change
watched_path = {str}'.\Test\foo\barn' # Path as detailed in filter file
Any idea what's causing this?
The issue seems to be in the "checker" function
Full Code:
# Script
################
# Relevant Imports
###############
import os
################
# Parameter definitions:
###############
PATH = "." # default is the root folder holding the script
FILTERS = "./Filters/" # Default is a folder named "Filters" in root folder
SUFFIX = ".txt"
DIFF =
################
# Function definitions
###############
def return_as_list(filter_file):
"""Creates a list of strings out of a text file, line by line"""
with open(FILTERS+filter_file, 'r') as filter:
results = filter.readlines()
return results
def checker(filter_as_list, changes_as_set):
"""Compares the filter list to the changes set, and returns
the difference if there is any"""
#changes_set = set(filter_as_list).intersection(changes_as_set)
changes_list =
for watched_path in filter_as_list:
for changed_path in changes_as_set:
if watched_path == changed_path:
changes_list.append(watched_path)
if not changes_list: # If the list is empty
print('changes_list is empty!')
pass # Skips loop since there is no match between filter and Changelog
else:
return (changes_list)
###############
# Generate log of changes made to the directory structure:
###############
# Check if log exists, if so designate it as old for comparison later
if os.path.isfile('log_current.txt'):
if not os.path.isfile('log_old.txt'):
os.rename('log_current.txt', 'log_old.txt')
# Fill log_current with current directory structure
for root, dirs, files in os.walk(PATH, topdown=True):
for name in dirs:
os.system('echo ' + os.path.join(root, name) + ' >> log_current.txt')
# If two logs exist, create a log of all differences between the two
if (os.path.isfile('log_current.txt') and os.path.isfile('log_old.txt')):
with open('log_current.txt', 'r') as current:
with open('log_old.txt', 'r') as old:
DIFF = set(current).symmetric_difference(old)
# Create a text file containing all changes
with open('log_changes.txt', 'w+') as changes:
for line in DIFF:
changes.write(line)
###############
# Script start
###############
# Check if the changes set is empty
if not DIFF:
print('Set is empty, no changes')
if os.path.isfile('log_old.txt'):
os.remove('log_old.txt')
if os.path.isfile('log_changes.txt'):
os.remove('log_changes.txt')
else:
# Cycle through the filters and compare them to the changes log
for filename in os.listdir(FILTERS):
if filename.endswith(SUFFIX):
# Retrieve email address and send file to be processed
filter_file_as_list = return_as_list(filename)
email_address = filter_file_as_list.pop(0)
# Get a list of changes that are mentioned in the filter file
list_of_changed_paths = checker(filter_file_as_list, DIFF)
# If there was no match
if not list_of_changed_paths:
pass
else:
paths_for_email = ("n".join(list_of_changed_paths))
# Create text file for Email script
with open('message.txt', 'w+') as msg:
msg.write("The following paths have changed:n " + paths_for_email)
print(paths_for_email)
#os.system("./outlook-sendmail.exe " + email_address + ' "Changes" ' + ' "message.txt"')
# Clean up
if os.path.isfile('message.txt'):
os.remove("message.txt")
if os.path.isfile('log_old.txt'):
os.remove('log_old.txt')
if os.path.isfile('log_changes.txt'):
os.remove('log_changes.txt')
python string list set
add a comment |
TL;DR - what generates a white space at the end of a string stored in a set?
Problem description:
Imagine writing a script to crawl trough a directory and act on specific changes to the directory mentioned in a filter file.
Due to some architectural constraints, you choose to have the script map the dir structure and store it in a text file line by line. Example:
./Test
./Test/foo
./Test/foo/bar
Then, when you run the script again, it will compare the new text file generated on the nth run to the old text file from the n-1 run. If there were any changes, it will act on them by comparing the changes to the filter text file which holds directories of interest.
When the script is run, however, it fails. Upon debugging, it turns out that a white space was added just before the end of the strings stored in the "changes" set as follows:
changed_path = {str}'.\Test\foo\bar n' # Detected Path change
watched_path = {str}'.\Test\foo\barn' # Path as detailed in filter file
Any idea what's causing this?
The issue seems to be in the "checker" function
Full Code:
# Script
################
# Relevant Imports
###############
import os
################
# Parameter definitions:
###############
PATH = "." # default is the root folder holding the script
FILTERS = "./Filters/" # Default is a folder named "Filters" in root folder
SUFFIX = ".txt"
DIFF =
################
# Function definitions
###############
def return_as_list(filter_file):
"""Creates a list of strings out of a text file, line by line"""
with open(FILTERS+filter_file, 'r') as filter:
results = filter.readlines()
return results
def checker(filter_as_list, changes_as_set):
"""Compares the filter list to the changes set, and returns
the difference if there is any"""
#changes_set = set(filter_as_list).intersection(changes_as_set)
changes_list =
for watched_path in filter_as_list:
for changed_path in changes_as_set:
if watched_path == changed_path:
changes_list.append(watched_path)
if not changes_list: # If the list is empty
print('changes_list is empty!')
pass # Skips loop since there is no match between filter and Changelog
else:
return (changes_list)
###############
# Generate log of changes made to the directory structure:
###############
# Check if log exists, if so designate it as old for comparison later
if os.path.isfile('log_current.txt'):
if not os.path.isfile('log_old.txt'):
os.rename('log_current.txt', 'log_old.txt')
# Fill log_current with current directory structure
for root, dirs, files in os.walk(PATH, topdown=True):
for name in dirs:
os.system('echo ' + os.path.join(root, name) + ' >> log_current.txt')
# If two logs exist, create a log of all differences between the two
if (os.path.isfile('log_current.txt') and os.path.isfile('log_old.txt')):
with open('log_current.txt', 'r') as current:
with open('log_old.txt', 'r') as old:
DIFF = set(current).symmetric_difference(old)
# Create a text file containing all changes
with open('log_changes.txt', 'w+') as changes:
for line in DIFF:
changes.write(line)
###############
# Script start
###############
# Check if the changes set is empty
if not DIFF:
print('Set is empty, no changes')
if os.path.isfile('log_old.txt'):
os.remove('log_old.txt')
if os.path.isfile('log_changes.txt'):
os.remove('log_changes.txt')
else:
# Cycle through the filters and compare them to the changes log
for filename in os.listdir(FILTERS):
if filename.endswith(SUFFIX):
# Retrieve email address and send file to be processed
filter_file_as_list = return_as_list(filename)
email_address = filter_file_as_list.pop(0)
# Get a list of changes that are mentioned in the filter file
list_of_changed_paths = checker(filter_file_as_list, DIFF)
# If there was no match
if not list_of_changed_paths:
pass
else:
paths_for_email = ("n".join(list_of_changed_paths))
# Create text file for Email script
with open('message.txt', 'w+') as msg:
msg.write("The following paths have changed:n " + paths_for_email)
print(paths_for_email)
#os.system("./outlook-sendmail.exe " + email_address + ' "Changes" ' + ' "message.txt"')
# Clean up
if os.path.isfile('message.txt'):
os.remove("message.txt")
if os.path.isfile('log_old.txt'):
os.remove('log_old.txt')
if os.path.isfile('log_changes.txt'):
os.remove('log_changes.txt')
python string list set
I would be quite suspicious of thatecho
; does it make any difference if you dowith open("log_current.txt", "a") as f; f.write(os.path.join(root, name))
?
– snakecharmerb
Jan 3 at 10:47
add a comment |
TL;DR - what generates a white space at the end of a string stored in a set?
Problem description:
Imagine writing a script to crawl trough a directory and act on specific changes to the directory mentioned in a filter file.
Due to some architectural constraints, you choose to have the script map the dir structure and store it in a text file line by line. Example:
./Test
./Test/foo
./Test/foo/bar
Then, when you run the script again, it will compare the new text file generated on the nth run to the old text file from the n-1 run. If there were any changes, it will act on them by comparing the changes to the filter text file which holds directories of interest.
When the script is run, however, it fails. Upon debugging, it turns out that a white space was added just before the end of the strings stored in the "changes" set as follows:
changed_path = {str}'.\Test\foo\bar n' # Detected Path change
watched_path = {str}'.\Test\foo\barn' # Path as detailed in filter file
Any idea what's causing this?
The issue seems to be in the "checker" function
Full Code:
# Script
################
# Relevant Imports
###############
import os
################
# Parameter definitions:
###############
PATH = "." # default is the root folder holding the script
FILTERS = "./Filters/" # Default is a folder named "Filters" in root folder
SUFFIX = ".txt"
DIFF =
################
# Function definitions
###############
def return_as_list(filter_file):
"""Creates a list of strings out of a text file, line by line"""
with open(FILTERS+filter_file, 'r') as filter:
results = filter.readlines()
return results
def checker(filter_as_list, changes_as_set):
"""Compares the filter list to the changes set, and returns
the difference if there is any"""
#changes_set = set(filter_as_list).intersection(changes_as_set)
changes_list =
for watched_path in filter_as_list:
for changed_path in changes_as_set:
if watched_path == changed_path:
changes_list.append(watched_path)
if not changes_list: # If the list is empty
print('changes_list is empty!')
pass # Skips loop since there is no match between filter and Changelog
else:
return (changes_list)
###############
# Generate log of changes made to the directory structure:
###############
# Check if log exists, if so designate it as old for comparison later
if os.path.isfile('log_current.txt'):
if not os.path.isfile('log_old.txt'):
os.rename('log_current.txt', 'log_old.txt')
# Fill log_current with current directory structure
for root, dirs, files in os.walk(PATH, topdown=True):
for name in dirs:
os.system('echo ' + os.path.join(root, name) + ' >> log_current.txt')
# If two logs exist, create a log of all differences between the two
if (os.path.isfile('log_current.txt') and os.path.isfile('log_old.txt')):
with open('log_current.txt', 'r') as current:
with open('log_old.txt', 'r') as old:
DIFF = set(current).symmetric_difference(old)
# Create a text file containing all changes
with open('log_changes.txt', 'w+') as changes:
for line in DIFF:
changes.write(line)
###############
# Script start
###############
# Check if the changes set is empty
if not DIFF:
print('Set is empty, no changes')
if os.path.isfile('log_old.txt'):
os.remove('log_old.txt')
if os.path.isfile('log_changes.txt'):
os.remove('log_changes.txt')
else:
# Cycle through the filters and compare them to the changes log
for filename in os.listdir(FILTERS):
if filename.endswith(SUFFIX):
# Retrieve email address and send file to be processed
filter_file_as_list = return_as_list(filename)
email_address = filter_file_as_list.pop(0)
# Get a list of changes that are mentioned in the filter file
list_of_changed_paths = checker(filter_file_as_list, DIFF)
# If there was no match
if not list_of_changed_paths:
pass
else:
paths_for_email = ("n".join(list_of_changed_paths))
# Create text file for Email script
with open('message.txt', 'w+') as msg:
msg.write("The following paths have changed:n " + paths_for_email)
print(paths_for_email)
#os.system("./outlook-sendmail.exe " + email_address + ' "Changes" ' + ' "message.txt"')
# Clean up
if os.path.isfile('message.txt'):
os.remove("message.txt")
if os.path.isfile('log_old.txt'):
os.remove('log_old.txt')
if os.path.isfile('log_changes.txt'):
os.remove('log_changes.txt')
python string list set
TL;DR - what generates a white space at the end of a string stored in a set?
Problem description:
Imagine writing a script to crawl trough a directory and act on specific changes to the directory mentioned in a filter file.
Due to some architectural constraints, you choose to have the script map the dir structure and store it in a text file line by line. Example:
./Test
./Test/foo
./Test/foo/bar
Then, when you run the script again, it will compare the new text file generated on the nth run to the old text file from the n-1 run. If there were any changes, it will act on them by comparing the changes to the filter text file which holds directories of interest.
When the script is run, however, it fails. Upon debugging, it turns out that a white space was added just before the end of the strings stored in the "changes" set as follows:
changed_path = {str}'.\Test\foo\bar n' # Detected Path change
watched_path = {str}'.\Test\foo\barn' # Path as detailed in filter file
Any idea what's causing this?
The issue seems to be in the "checker" function
Full Code:
# Script
################
# Relevant Imports
###############
import os
################
# Parameter definitions:
###############
PATH = "." # default is the root folder holding the script
FILTERS = "./Filters/" # Default is a folder named "Filters" in root folder
SUFFIX = ".txt"
DIFF =
################
# Function definitions
###############
def return_as_list(filter_file):
"""Creates a list of strings out of a text file, line by line"""
with open(FILTERS+filter_file, 'r') as filter:
results = filter.readlines()
return results
def checker(filter_as_list, changes_as_set):
"""Compares the filter list to the changes set, and returns
the difference if there is any"""
#changes_set = set(filter_as_list).intersection(changes_as_set)
changes_list =
for watched_path in filter_as_list:
for changed_path in changes_as_set:
if watched_path == changed_path:
changes_list.append(watched_path)
if not changes_list: # If the list is empty
print('changes_list is empty!')
pass # Skips loop since there is no match between filter and Changelog
else:
return (changes_list)
###############
# Generate log of changes made to the directory structure:
###############
# Check if log exists, if so designate it as old for comparison later
if os.path.isfile('log_current.txt'):
if not os.path.isfile('log_old.txt'):
os.rename('log_current.txt', 'log_old.txt')
# Fill log_current with current directory structure
for root, dirs, files in os.walk(PATH, topdown=True):
for name in dirs:
os.system('echo ' + os.path.join(root, name) + ' >> log_current.txt')
# If two logs exist, create a log of all differences between the two
if (os.path.isfile('log_current.txt') and os.path.isfile('log_old.txt')):
with open('log_current.txt', 'r') as current:
with open('log_old.txt', 'r') as old:
DIFF = set(current).symmetric_difference(old)
# Create a text file containing all changes
with open('log_changes.txt', 'w+') as changes:
for line in DIFF:
changes.write(line)
###############
# Script start
###############
# Check if the changes set is empty
if not DIFF:
print('Set is empty, no changes')
if os.path.isfile('log_old.txt'):
os.remove('log_old.txt')
if os.path.isfile('log_changes.txt'):
os.remove('log_changes.txt')
else:
# Cycle through the filters and compare them to the changes log
for filename in os.listdir(FILTERS):
if filename.endswith(SUFFIX):
# Retrieve email address and send file to be processed
filter_file_as_list = return_as_list(filename)
email_address = filter_file_as_list.pop(0)
# Get a list of changes that are mentioned in the filter file
list_of_changed_paths = checker(filter_file_as_list, DIFF)
# If there was no match
if not list_of_changed_paths:
pass
else:
paths_for_email = ("n".join(list_of_changed_paths))
# Create text file for Email script
with open('message.txt', 'w+') as msg:
msg.write("The following paths have changed:n " + paths_for_email)
print(paths_for_email)
#os.system("./outlook-sendmail.exe " + email_address + ' "Changes" ' + ' "message.txt"')
# Clean up
if os.path.isfile('message.txt'):
os.remove("message.txt")
if os.path.isfile('log_old.txt'):
os.remove('log_old.txt')
if os.path.isfile('log_changes.txt'):
os.remove('log_changes.txt')
python string list set
python string list set
asked Jan 2 at 11:36
user4285059
I would be quite suspicious of thatecho
; does it make any difference if you dowith open("log_current.txt", "a") as f; f.write(os.path.join(root, name))
?
– snakecharmerb
Jan 3 at 10:47
add a comment |
I would be quite suspicious of thatecho
; does it make any difference if you dowith open("log_current.txt", "a") as f; f.write(os.path.join(root, name))
?
– snakecharmerb
Jan 3 at 10:47
I would be quite suspicious of that
echo
; does it make any difference if you do with open("log_current.txt", "a") as f; f.write(os.path.join(root, name))
?– snakecharmerb
Jan 3 at 10:47
I would be quite suspicious of that
echo
; does it make any difference if you do with open("log_current.txt", "a") as f; f.write(os.path.join(root, name))
?– snakecharmerb
Jan 3 at 10:47
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54005637%2fpython-where-is-the-space-at-the-end-of-the-string-coming-from%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54005637%2fpython-where-is-the-space-at-the-end-of-the-string-coming-from%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
E7ZKZWKK20ZnyYX9LagBNXAa0O2,LrPvkE1qhuWFg0vxXstij fCQvPPZdTAqDgQun8i8cz7lxe,GH OPknQ7 qgPXJ6XtHe 840
I would be quite suspicious of that
echo
; does it make any difference if you dowith open("log_current.txt", "a") as f; f.write(os.path.join(root, name))
?– snakecharmerb
Jan 3 at 10:47