Cloud Functions - Function execution finished with status 'ok' before return
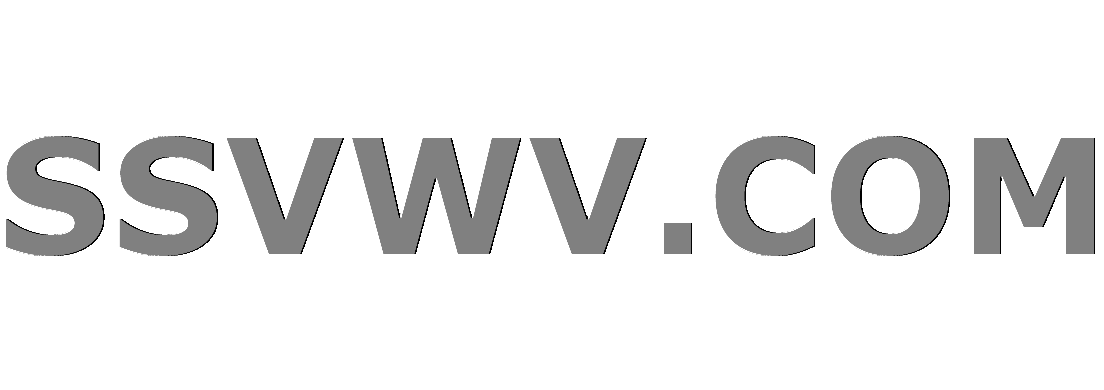
Multi tool use
I have a (GCP) Cloud Function that is meant to aggregate hourly data and write to Cloud Bigtable, however it seems to be returning with message: "Function execution took 100ms, finished with status: ok", before completing the full code, subsequent lines sometimes gets run, sometimes not. Would be great if anyone has any experience and can advise on this, thanks!
It works on my local machine when I'm running the script, only in Cloud Functions and I'm not sure what is triggering the termination of the code. I have tried adding in a try/catch block but it did not throw any errors either. Main parts of the code is reproduced below:
const Bigtable = require('@google-cloud/bigtable');
const bigtableOptions = { projectId: process.env.PROJECT_ID };
const bigtable = new Bigtable(bigtableOptions);
const cbt = bigtable.instance(process.env.BIGTABLE_INSTANCE);
const async = require("async");
const moment = require("moment");
require("moment-round");
const bigtableFetchRawDataForDmac = require("./fetchData").bigtableFetchRawDataForDmac;
exports.patchJob = (event, context) => {
const pubsubMsg = Buffer.from(event.data, 'base64').toString();
const jsonMsg = tryParseJSON(pubsubMsg); // msg in format { time: "2018-12-24T02:00:00.000Z", dmac: ["abc", "def", "ghi] }
if(!jsonMsg) return;
else {
if(!jsonMsg.time) {
console.log("Time not provided");
// res.status(400).json({ err: 'TimeNotProvided', msg: `Time parameter is not provided` });
return;
}
let date_range = {};
date_range.lower = moment(jsonMsg.time).toISOString();
date_range.upper = moment(jsonMsg.time).add(1,'hours').subtract(1,"milliseconds").toISOString();
let queryData = ;
let data = {};
for(let i=0; i<jsonMsg.dmac.length; i++){
data[jsonMsg.dmac[i]]=;
queryData.push(bigtableFetchRawDataForDmac(cbt, jsonMsg.dmac[i], date_range.lower, date_range.upper, data[jsonMsg.dmac[i]]));
}
async.parallel(queryData, function(err, result){
console.log("cookie trail...");
return;
}
}
}
For the bigtableFetchRawDataForDmac it is in a different folder:
function bigtableFetchRawDataForDmac(cbt, dmac, start, end, data) {
return async function(cb){
const table = cbt.table(process.env.BT_DATA_TABLE);
try { var bigtable = await fetchFromBigtable(table, process.env.BT_DATA_TABLE, dmac, start, end, data, ['push', 'mode', 'val']); }
catch (err) { console.log("bigtableFetchRawDataForDmac failed: ", err); cb(err); }
}
}
google-cloud-functions async.js
add a comment |
I have a (GCP) Cloud Function that is meant to aggregate hourly data and write to Cloud Bigtable, however it seems to be returning with message: "Function execution took 100ms, finished with status: ok", before completing the full code, subsequent lines sometimes gets run, sometimes not. Would be great if anyone has any experience and can advise on this, thanks!
It works on my local machine when I'm running the script, only in Cloud Functions and I'm not sure what is triggering the termination of the code. I have tried adding in a try/catch block but it did not throw any errors either. Main parts of the code is reproduced below:
const Bigtable = require('@google-cloud/bigtable');
const bigtableOptions = { projectId: process.env.PROJECT_ID };
const bigtable = new Bigtable(bigtableOptions);
const cbt = bigtable.instance(process.env.BIGTABLE_INSTANCE);
const async = require("async");
const moment = require("moment");
require("moment-round");
const bigtableFetchRawDataForDmac = require("./fetchData").bigtableFetchRawDataForDmac;
exports.patchJob = (event, context) => {
const pubsubMsg = Buffer.from(event.data, 'base64').toString();
const jsonMsg = tryParseJSON(pubsubMsg); // msg in format { time: "2018-12-24T02:00:00.000Z", dmac: ["abc", "def", "ghi] }
if(!jsonMsg) return;
else {
if(!jsonMsg.time) {
console.log("Time not provided");
// res.status(400).json({ err: 'TimeNotProvided', msg: `Time parameter is not provided` });
return;
}
let date_range = {};
date_range.lower = moment(jsonMsg.time).toISOString();
date_range.upper = moment(jsonMsg.time).add(1,'hours').subtract(1,"milliseconds").toISOString();
let queryData = ;
let data = {};
for(let i=0; i<jsonMsg.dmac.length; i++){
data[jsonMsg.dmac[i]]=;
queryData.push(bigtableFetchRawDataForDmac(cbt, jsonMsg.dmac[i], date_range.lower, date_range.upper, data[jsonMsg.dmac[i]]));
}
async.parallel(queryData, function(err, result){
console.log("cookie trail...");
return;
}
}
}
For the bigtableFetchRawDataForDmac it is in a different folder:
function bigtableFetchRawDataForDmac(cbt, dmac, start, end, data) {
return async function(cb){
const table = cbt.table(process.env.BT_DATA_TABLE);
try { var bigtable = await fetchFromBigtable(table, process.env.BT_DATA_TABLE, dmac, start, end, data, ['push', 'mode', 'val']); }
catch (err) { console.log("bigtableFetchRawDataForDmac failed: ", err); cb(err); }
}
}
google-cloud-functions async.js
add a comment |
I have a (GCP) Cloud Function that is meant to aggregate hourly data and write to Cloud Bigtable, however it seems to be returning with message: "Function execution took 100ms, finished with status: ok", before completing the full code, subsequent lines sometimes gets run, sometimes not. Would be great if anyone has any experience and can advise on this, thanks!
It works on my local machine when I'm running the script, only in Cloud Functions and I'm not sure what is triggering the termination of the code. I have tried adding in a try/catch block but it did not throw any errors either. Main parts of the code is reproduced below:
const Bigtable = require('@google-cloud/bigtable');
const bigtableOptions = { projectId: process.env.PROJECT_ID };
const bigtable = new Bigtable(bigtableOptions);
const cbt = bigtable.instance(process.env.BIGTABLE_INSTANCE);
const async = require("async");
const moment = require("moment");
require("moment-round");
const bigtableFetchRawDataForDmac = require("./fetchData").bigtableFetchRawDataForDmac;
exports.patchJob = (event, context) => {
const pubsubMsg = Buffer.from(event.data, 'base64').toString();
const jsonMsg = tryParseJSON(pubsubMsg); // msg in format { time: "2018-12-24T02:00:00.000Z", dmac: ["abc", "def", "ghi] }
if(!jsonMsg) return;
else {
if(!jsonMsg.time) {
console.log("Time not provided");
// res.status(400).json({ err: 'TimeNotProvided', msg: `Time parameter is not provided` });
return;
}
let date_range = {};
date_range.lower = moment(jsonMsg.time).toISOString();
date_range.upper = moment(jsonMsg.time).add(1,'hours').subtract(1,"milliseconds").toISOString();
let queryData = ;
let data = {};
for(let i=0; i<jsonMsg.dmac.length; i++){
data[jsonMsg.dmac[i]]=;
queryData.push(bigtableFetchRawDataForDmac(cbt, jsonMsg.dmac[i], date_range.lower, date_range.upper, data[jsonMsg.dmac[i]]));
}
async.parallel(queryData, function(err, result){
console.log("cookie trail...");
return;
}
}
}
For the bigtableFetchRawDataForDmac it is in a different folder:
function bigtableFetchRawDataForDmac(cbt, dmac, start, end, data) {
return async function(cb){
const table = cbt.table(process.env.BT_DATA_TABLE);
try { var bigtable = await fetchFromBigtable(table, process.env.BT_DATA_TABLE, dmac, start, end, data, ['push', 'mode', 'val']); }
catch (err) { console.log("bigtableFetchRawDataForDmac failed: ", err); cb(err); }
}
}
google-cloud-functions async.js
I have a (GCP) Cloud Function that is meant to aggregate hourly data and write to Cloud Bigtable, however it seems to be returning with message: "Function execution took 100ms, finished with status: ok", before completing the full code, subsequent lines sometimes gets run, sometimes not. Would be great if anyone has any experience and can advise on this, thanks!
It works on my local machine when I'm running the script, only in Cloud Functions and I'm not sure what is triggering the termination of the code. I have tried adding in a try/catch block but it did not throw any errors either. Main parts of the code is reproduced below:
const Bigtable = require('@google-cloud/bigtable');
const bigtableOptions = { projectId: process.env.PROJECT_ID };
const bigtable = new Bigtable(bigtableOptions);
const cbt = bigtable.instance(process.env.BIGTABLE_INSTANCE);
const async = require("async");
const moment = require("moment");
require("moment-round");
const bigtableFetchRawDataForDmac = require("./fetchData").bigtableFetchRawDataForDmac;
exports.patchJob = (event, context) => {
const pubsubMsg = Buffer.from(event.data, 'base64').toString();
const jsonMsg = tryParseJSON(pubsubMsg); // msg in format { time: "2018-12-24T02:00:00.000Z", dmac: ["abc", "def", "ghi] }
if(!jsonMsg) return;
else {
if(!jsonMsg.time) {
console.log("Time not provided");
// res.status(400).json({ err: 'TimeNotProvided', msg: `Time parameter is not provided` });
return;
}
let date_range = {};
date_range.lower = moment(jsonMsg.time).toISOString();
date_range.upper = moment(jsonMsg.time).add(1,'hours').subtract(1,"milliseconds").toISOString();
let queryData = ;
let data = {};
for(let i=0; i<jsonMsg.dmac.length; i++){
data[jsonMsg.dmac[i]]=;
queryData.push(bigtableFetchRawDataForDmac(cbt, jsonMsg.dmac[i], date_range.lower, date_range.upper, data[jsonMsg.dmac[i]]));
}
async.parallel(queryData, function(err, result){
console.log("cookie trail...");
return;
}
}
}
For the bigtableFetchRawDataForDmac it is in a different folder:
function bigtableFetchRawDataForDmac(cbt, dmac, start, end, data) {
return async function(cb){
const table = cbt.table(process.env.BT_DATA_TABLE);
try { var bigtable = await fetchFromBigtable(table, process.env.BT_DATA_TABLE, dmac, start, end, data, ['push', 'mode', 'val']); }
catch (err) { console.log("bigtableFetchRawDataForDmac failed: ", err); cb(err); }
}
}
google-cloud-functions async.js
google-cloud-functions async.js
edited Jan 2 at 5:07
jlyh
asked Jan 2 at 4:48
jlyhjlyh
135113
135113
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
A pubsub Cloud Function receives an event
and callback
parameter. You're supposed to call the callback method to terminate the function when all the work is complete, as is the case for all types of background Cloud Functions.
You've called the callback context
. And you're not using it to terminate the function at all. You may alternatively return a promise that resolves when all the work is complete, but you're not doing that either.
You're going to have to figure out a way to properly terminate your function only after all the async work is complete, or it won't work the way you expect.
Hi Doug, thanks for your comments. Sorry I forgot to show the return; from my code, edited above. The issue is more of early termination, not non-termination though. Will look into the control flow again, but just a question, I'm using the async library. Would that somehow interfere with the way Cloud Function runs? i.e. Would it somehow assume the callbacks enclosed with the callback functions to be run in async.parallel are triggers for early termination? I ran this as a normal nodeJS script and the control flow works fine though ;(
– jlyh
Jan 2 at 5:15
Everything I said still mostly applies. The return from your callback passed to parallel isn't doing what you expect. Your overall function is still not either calling the callback function provided by Cloud Functions (again, you called it "context"), nor is it returning a promise. I think you'll be better off ditching the async library and just work with the promises directly using Promise.all() to generate a new promise that resolves when everything else resolves.
– Doug Stevenson
Jan 2 at 5:24
That ("context") was what was provided as default template in gcp console. Anyway, I tested the below and it worked, so its likely not an issue with async library. Thanks for your advice though, will look through the control flow in more detail again. var async = require("async"); exports.ps = (event, context) => { async.parallel({ one: function(callback) { setTimeout(function() { callback(null, 1);}, 200); }, two: function(callback) { setTimeout(function() { callback(null, 2);}, 100); } }, function(err, ret) { // results: {one: 1, two: 2} console.log(ret); }); };
– jlyh
Jan 2 at 5:42
What you got in the console for callback doesn't at all match the documentation (or reality). I'd consider it a bug in the console. If you're using setTimeout in a function, it's almost certainly not the right thing to do. Just delaying the callback is fragile, and I'd expect that to fail intermittently. If you terminate the function before all the work is fully complete, Cloud Functions will shut down that work forcibly.
– Doug Stevenson
Jan 2 at 5:54
Doug, setTimeout above was just a watered down piece of code used to check if async library works in Cloud Functions. You're right about the bug, I was trying to call callback() just now but it didn't work because the parameters are supposed to be (data, context, callback), instead of (data, context) as provided in the default template - cloud.google.com/functions/docs/writing/…. Now everything works as intended with the 3 parameters provided.
– jlyh
Jan 2 at 6:57
add a comment |
In Nodejs 8 (Beta) runtime, 3 parameters should be provided (data, context, callback) instead of 2 as provided in the default template in the inline editor of Cloud Functions console. (Documentation reference:
https://cloud.google.com/functions/docs/writing/background#functions_background_parameters-node8).
Code should be something like:
exports.patchJob = (event, context, callback) => {
doSomething();
callback(); // To terminate Cloud Functions
}
Thanks @Doug for the hint!
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54001332%2fcloud-functions-function-execution-finished-with-status-ok-before-return%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
A pubsub Cloud Function receives an event
and callback
parameter. You're supposed to call the callback method to terminate the function when all the work is complete, as is the case for all types of background Cloud Functions.
You've called the callback context
. And you're not using it to terminate the function at all. You may alternatively return a promise that resolves when all the work is complete, but you're not doing that either.
You're going to have to figure out a way to properly terminate your function only after all the async work is complete, or it won't work the way you expect.
Hi Doug, thanks for your comments. Sorry I forgot to show the return; from my code, edited above. The issue is more of early termination, not non-termination though. Will look into the control flow again, but just a question, I'm using the async library. Would that somehow interfere with the way Cloud Function runs? i.e. Would it somehow assume the callbacks enclosed with the callback functions to be run in async.parallel are triggers for early termination? I ran this as a normal nodeJS script and the control flow works fine though ;(
– jlyh
Jan 2 at 5:15
Everything I said still mostly applies. The return from your callback passed to parallel isn't doing what you expect. Your overall function is still not either calling the callback function provided by Cloud Functions (again, you called it "context"), nor is it returning a promise. I think you'll be better off ditching the async library and just work with the promises directly using Promise.all() to generate a new promise that resolves when everything else resolves.
– Doug Stevenson
Jan 2 at 5:24
That ("context") was what was provided as default template in gcp console. Anyway, I tested the below and it worked, so its likely not an issue with async library. Thanks for your advice though, will look through the control flow in more detail again. var async = require("async"); exports.ps = (event, context) => { async.parallel({ one: function(callback) { setTimeout(function() { callback(null, 1);}, 200); }, two: function(callback) { setTimeout(function() { callback(null, 2);}, 100); } }, function(err, ret) { // results: {one: 1, two: 2} console.log(ret); }); };
– jlyh
Jan 2 at 5:42
What you got in the console for callback doesn't at all match the documentation (or reality). I'd consider it a bug in the console. If you're using setTimeout in a function, it's almost certainly not the right thing to do. Just delaying the callback is fragile, and I'd expect that to fail intermittently. If you terminate the function before all the work is fully complete, Cloud Functions will shut down that work forcibly.
– Doug Stevenson
Jan 2 at 5:54
Doug, setTimeout above was just a watered down piece of code used to check if async library works in Cloud Functions. You're right about the bug, I was trying to call callback() just now but it didn't work because the parameters are supposed to be (data, context, callback), instead of (data, context) as provided in the default template - cloud.google.com/functions/docs/writing/…. Now everything works as intended with the 3 parameters provided.
– jlyh
Jan 2 at 6:57
add a comment |
A pubsub Cloud Function receives an event
and callback
parameter. You're supposed to call the callback method to terminate the function when all the work is complete, as is the case for all types of background Cloud Functions.
You've called the callback context
. And you're not using it to terminate the function at all. You may alternatively return a promise that resolves when all the work is complete, but you're not doing that either.
You're going to have to figure out a way to properly terminate your function only after all the async work is complete, or it won't work the way you expect.
Hi Doug, thanks for your comments. Sorry I forgot to show the return; from my code, edited above. The issue is more of early termination, not non-termination though. Will look into the control flow again, but just a question, I'm using the async library. Would that somehow interfere with the way Cloud Function runs? i.e. Would it somehow assume the callbacks enclosed with the callback functions to be run in async.parallel are triggers for early termination? I ran this as a normal nodeJS script and the control flow works fine though ;(
– jlyh
Jan 2 at 5:15
Everything I said still mostly applies. The return from your callback passed to parallel isn't doing what you expect. Your overall function is still not either calling the callback function provided by Cloud Functions (again, you called it "context"), nor is it returning a promise. I think you'll be better off ditching the async library and just work with the promises directly using Promise.all() to generate a new promise that resolves when everything else resolves.
– Doug Stevenson
Jan 2 at 5:24
That ("context") was what was provided as default template in gcp console. Anyway, I tested the below and it worked, so its likely not an issue with async library. Thanks for your advice though, will look through the control flow in more detail again. var async = require("async"); exports.ps = (event, context) => { async.parallel({ one: function(callback) { setTimeout(function() { callback(null, 1);}, 200); }, two: function(callback) { setTimeout(function() { callback(null, 2);}, 100); } }, function(err, ret) { // results: {one: 1, two: 2} console.log(ret); }); };
– jlyh
Jan 2 at 5:42
What you got in the console for callback doesn't at all match the documentation (or reality). I'd consider it a bug in the console. If you're using setTimeout in a function, it's almost certainly not the right thing to do. Just delaying the callback is fragile, and I'd expect that to fail intermittently. If you terminate the function before all the work is fully complete, Cloud Functions will shut down that work forcibly.
– Doug Stevenson
Jan 2 at 5:54
Doug, setTimeout above was just a watered down piece of code used to check if async library works in Cloud Functions. You're right about the bug, I was trying to call callback() just now but it didn't work because the parameters are supposed to be (data, context, callback), instead of (data, context) as provided in the default template - cloud.google.com/functions/docs/writing/…. Now everything works as intended with the 3 parameters provided.
– jlyh
Jan 2 at 6:57
add a comment |
A pubsub Cloud Function receives an event
and callback
parameter. You're supposed to call the callback method to terminate the function when all the work is complete, as is the case for all types of background Cloud Functions.
You've called the callback context
. And you're not using it to terminate the function at all. You may alternatively return a promise that resolves when all the work is complete, but you're not doing that either.
You're going to have to figure out a way to properly terminate your function only after all the async work is complete, or it won't work the way you expect.
A pubsub Cloud Function receives an event
and callback
parameter. You're supposed to call the callback method to terminate the function when all the work is complete, as is the case for all types of background Cloud Functions.
You've called the callback context
. And you're not using it to terminate the function at all. You may alternatively return a promise that resolves when all the work is complete, but you're not doing that either.
You're going to have to figure out a way to properly terminate your function only after all the async work is complete, or it won't work the way you expect.
answered Jan 2 at 5:01


Doug StevensonDoug Stevenson
79.2k994112
79.2k994112
Hi Doug, thanks for your comments. Sorry I forgot to show the return; from my code, edited above. The issue is more of early termination, not non-termination though. Will look into the control flow again, but just a question, I'm using the async library. Would that somehow interfere with the way Cloud Function runs? i.e. Would it somehow assume the callbacks enclosed with the callback functions to be run in async.parallel are triggers for early termination? I ran this as a normal nodeJS script and the control flow works fine though ;(
– jlyh
Jan 2 at 5:15
Everything I said still mostly applies. The return from your callback passed to parallel isn't doing what you expect. Your overall function is still not either calling the callback function provided by Cloud Functions (again, you called it "context"), nor is it returning a promise. I think you'll be better off ditching the async library and just work with the promises directly using Promise.all() to generate a new promise that resolves when everything else resolves.
– Doug Stevenson
Jan 2 at 5:24
That ("context") was what was provided as default template in gcp console. Anyway, I tested the below and it worked, so its likely not an issue with async library. Thanks for your advice though, will look through the control flow in more detail again. var async = require("async"); exports.ps = (event, context) => { async.parallel({ one: function(callback) { setTimeout(function() { callback(null, 1);}, 200); }, two: function(callback) { setTimeout(function() { callback(null, 2);}, 100); } }, function(err, ret) { // results: {one: 1, two: 2} console.log(ret); }); };
– jlyh
Jan 2 at 5:42
What you got in the console for callback doesn't at all match the documentation (or reality). I'd consider it a bug in the console. If you're using setTimeout in a function, it's almost certainly not the right thing to do. Just delaying the callback is fragile, and I'd expect that to fail intermittently. If you terminate the function before all the work is fully complete, Cloud Functions will shut down that work forcibly.
– Doug Stevenson
Jan 2 at 5:54
Doug, setTimeout above was just a watered down piece of code used to check if async library works in Cloud Functions. You're right about the bug, I was trying to call callback() just now but it didn't work because the parameters are supposed to be (data, context, callback), instead of (data, context) as provided in the default template - cloud.google.com/functions/docs/writing/…. Now everything works as intended with the 3 parameters provided.
– jlyh
Jan 2 at 6:57
add a comment |
Hi Doug, thanks for your comments. Sorry I forgot to show the return; from my code, edited above. The issue is more of early termination, not non-termination though. Will look into the control flow again, but just a question, I'm using the async library. Would that somehow interfere with the way Cloud Function runs? i.e. Would it somehow assume the callbacks enclosed with the callback functions to be run in async.parallel are triggers for early termination? I ran this as a normal nodeJS script and the control flow works fine though ;(
– jlyh
Jan 2 at 5:15
Everything I said still mostly applies. The return from your callback passed to parallel isn't doing what you expect. Your overall function is still not either calling the callback function provided by Cloud Functions (again, you called it "context"), nor is it returning a promise. I think you'll be better off ditching the async library and just work with the promises directly using Promise.all() to generate a new promise that resolves when everything else resolves.
– Doug Stevenson
Jan 2 at 5:24
That ("context") was what was provided as default template in gcp console. Anyway, I tested the below and it worked, so its likely not an issue with async library. Thanks for your advice though, will look through the control flow in more detail again. var async = require("async"); exports.ps = (event, context) => { async.parallel({ one: function(callback) { setTimeout(function() { callback(null, 1);}, 200); }, two: function(callback) { setTimeout(function() { callback(null, 2);}, 100); } }, function(err, ret) { // results: {one: 1, two: 2} console.log(ret); }); };
– jlyh
Jan 2 at 5:42
What you got in the console for callback doesn't at all match the documentation (or reality). I'd consider it a bug in the console. If you're using setTimeout in a function, it's almost certainly not the right thing to do. Just delaying the callback is fragile, and I'd expect that to fail intermittently. If you terminate the function before all the work is fully complete, Cloud Functions will shut down that work forcibly.
– Doug Stevenson
Jan 2 at 5:54
Doug, setTimeout above was just a watered down piece of code used to check if async library works in Cloud Functions. You're right about the bug, I was trying to call callback() just now but it didn't work because the parameters are supposed to be (data, context, callback), instead of (data, context) as provided in the default template - cloud.google.com/functions/docs/writing/…. Now everything works as intended with the 3 parameters provided.
– jlyh
Jan 2 at 6:57
Hi Doug, thanks for your comments. Sorry I forgot to show the return; from my code, edited above. The issue is more of early termination, not non-termination though. Will look into the control flow again, but just a question, I'm using the async library. Would that somehow interfere with the way Cloud Function runs? i.e. Would it somehow assume the callbacks enclosed with the callback functions to be run in async.parallel are triggers for early termination? I ran this as a normal nodeJS script and the control flow works fine though ;(
– jlyh
Jan 2 at 5:15
Hi Doug, thanks for your comments. Sorry I forgot to show the return; from my code, edited above. The issue is more of early termination, not non-termination though. Will look into the control flow again, but just a question, I'm using the async library. Would that somehow interfere with the way Cloud Function runs? i.e. Would it somehow assume the callbacks enclosed with the callback functions to be run in async.parallel are triggers for early termination? I ran this as a normal nodeJS script and the control flow works fine though ;(
– jlyh
Jan 2 at 5:15
Everything I said still mostly applies. The return from your callback passed to parallel isn't doing what you expect. Your overall function is still not either calling the callback function provided by Cloud Functions (again, you called it "context"), nor is it returning a promise. I think you'll be better off ditching the async library and just work with the promises directly using Promise.all() to generate a new promise that resolves when everything else resolves.
– Doug Stevenson
Jan 2 at 5:24
Everything I said still mostly applies. The return from your callback passed to parallel isn't doing what you expect. Your overall function is still not either calling the callback function provided by Cloud Functions (again, you called it "context"), nor is it returning a promise. I think you'll be better off ditching the async library and just work with the promises directly using Promise.all() to generate a new promise that resolves when everything else resolves.
– Doug Stevenson
Jan 2 at 5:24
That ("context") was what was provided as default template in gcp console. Anyway, I tested the below and it worked, so its likely not an issue with async library. Thanks for your advice though, will look through the control flow in more detail again. var async = require("async"); exports.ps = (event, context) => { async.parallel({ one: function(callback) { setTimeout(function() { callback(null, 1);}, 200); }, two: function(callback) { setTimeout(function() { callback(null, 2);}, 100); } }, function(err, ret) { // results: {one: 1, two: 2} console.log(ret); }); };
– jlyh
Jan 2 at 5:42
That ("context") was what was provided as default template in gcp console. Anyway, I tested the below and it worked, so its likely not an issue with async library. Thanks for your advice though, will look through the control flow in more detail again. var async = require("async"); exports.ps = (event, context) => { async.parallel({ one: function(callback) { setTimeout(function() { callback(null, 1);}, 200); }, two: function(callback) { setTimeout(function() { callback(null, 2);}, 100); } }, function(err, ret) { // results: {one: 1, two: 2} console.log(ret); }); };
– jlyh
Jan 2 at 5:42
What you got in the console for callback doesn't at all match the documentation (or reality). I'd consider it a bug in the console. If you're using setTimeout in a function, it's almost certainly not the right thing to do. Just delaying the callback is fragile, and I'd expect that to fail intermittently. If you terminate the function before all the work is fully complete, Cloud Functions will shut down that work forcibly.
– Doug Stevenson
Jan 2 at 5:54
What you got in the console for callback doesn't at all match the documentation (or reality). I'd consider it a bug in the console. If you're using setTimeout in a function, it's almost certainly not the right thing to do. Just delaying the callback is fragile, and I'd expect that to fail intermittently. If you terminate the function before all the work is fully complete, Cloud Functions will shut down that work forcibly.
– Doug Stevenson
Jan 2 at 5:54
Doug, setTimeout above was just a watered down piece of code used to check if async library works in Cloud Functions. You're right about the bug, I was trying to call callback() just now but it didn't work because the parameters are supposed to be (data, context, callback), instead of (data, context) as provided in the default template - cloud.google.com/functions/docs/writing/…. Now everything works as intended with the 3 parameters provided.
– jlyh
Jan 2 at 6:57
Doug, setTimeout above was just a watered down piece of code used to check if async library works in Cloud Functions. You're right about the bug, I was trying to call callback() just now but it didn't work because the parameters are supposed to be (data, context, callback), instead of (data, context) as provided in the default template - cloud.google.com/functions/docs/writing/…. Now everything works as intended with the 3 parameters provided.
– jlyh
Jan 2 at 6:57
add a comment |
In Nodejs 8 (Beta) runtime, 3 parameters should be provided (data, context, callback) instead of 2 as provided in the default template in the inline editor of Cloud Functions console. (Documentation reference:
https://cloud.google.com/functions/docs/writing/background#functions_background_parameters-node8).
Code should be something like:
exports.patchJob = (event, context, callback) => {
doSomething();
callback(); // To terminate Cloud Functions
}
Thanks @Doug for the hint!
add a comment |
In Nodejs 8 (Beta) runtime, 3 parameters should be provided (data, context, callback) instead of 2 as provided in the default template in the inline editor of Cloud Functions console. (Documentation reference:
https://cloud.google.com/functions/docs/writing/background#functions_background_parameters-node8).
Code should be something like:
exports.patchJob = (event, context, callback) => {
doSomething();
callback(); // To terminate Cloud Functions
}
Thanks @Doug for the hint!
add a comment |
In Nodejs 8 (Beta) runtime, 3 parameters should be provided (data, context, callback) instead of 2 as provided in the default template in the inline editor of Cloud Functions console. (Documentation reference:
https://cloud.google.com/functions/docs/writing/background#functions_background_parameters-node8).
Code should be something like:
exports.patchJob = (event, context, callback) => {
doSomething();
callback(); // To terminate Cloud Functions
}
Thanks @Doug for the hint!
In Nodejs 8 (Beta) runtime, 3 parameters should be provided (data, context, callback) instead of 2 as provided in the default template in the inline editor of Cloud Functions console. (Documentation reference:
https://cloud.google.com/functions/docs/writing/background#functions_background_parameters-node8).
Code should be something like:
exports.patchJob = (event, context, callback) => {
doSomething();
callback(); // To terminate Cloud Functions
}
Thanks @Doug for the hint!
answered Jan 2 at 7:06
jlyhjlyh
135113
135113
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54001332%2fcloud-functions-function-execution-finished-with-status-ok-before-return%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vGv,MKXG,azix33nPYo8D8K7,Gr