Spring Boot 2 OAuth2 Resource Server Does not hit authorization server for access token validation
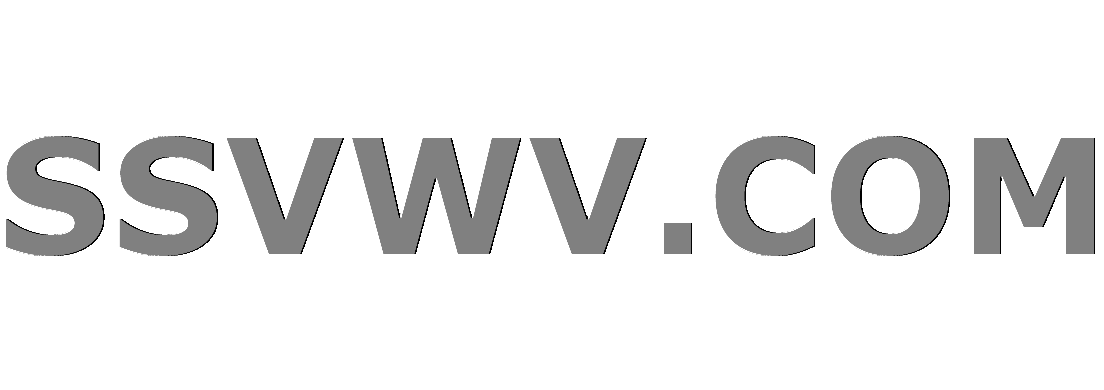
Multi tool use
I have implemented Spring boot 2 + OAuth2 Oauthorization server.
I only want to use Client_credential to secure resource Server
I am able to get access token from Auth server, but when I pass this to access rest api, resource server does not validate it from authorization server and gives invalid access token error, I am using postman to get access token and to quest resource server.
Authorization Server
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class AuthorizationApplication {
public static void main(String args) {
SpringApplication.run(AuthorizationApplication.class, args);
}
}
Authorization server config
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.oauth2.config.annotation.configurers.ClientDetailsServiceConfigurer;
import org.springframework.security.oauth2.config.annotation.web.configuration.AuthorizationServerConfigurerAdapter;
import org.springframework.security.oauth2.config.annotation.web.configuration.EnableAuthorizationServer;
import org.springframework.security.oauth2.config.annotation.web.configurers.AuthorizationServerEndpointsConfigurer;
import org.springframework.security.oauth2.config.annotation.web.configurers.AuthorizationServerSecurityConfigurer;
import org.springframework.security.oauth2.provider.token.store.InMemoryTokenStore;
@Configuration
@EnableAuthorizationServer
public class AuthorizationServerConfig extends AuthorizationServerConfigurerAdapter {
private static final String SERVER_RESOURCE_ID = "oauth2-server";
private static InMemoryTokenStore tokenStore = new InMemoryTokenStore();
@Autowired
@Qualifier("authenticationManagerBean")
private AuthenticationManager authenticationManager;
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints.authenticationManager(authenticationManager).tokenStore(tokenStore).approvalStoreDisabled();
}
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.inMemory().withClient("clientABC").secret("{noop}secretXYZ")
.authorizedGrantTypes("client_credentials")
.scopes("resource-server-read", "resource-server-write")
.resourceIds(SERVER_RESOURCE_ID);
}
@Override
public void configure(AuthorizationServerSecurityConfigurer security) throws Exception {
security.tokenKeyAccess("permitAll()")
.checkTokenAccess("isAuthenticated()");
}
}
Authorization server web config
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
public class AuthorizationServerWebConfig extends WebSecurityConfigurerAdapter {
@Bean
@Override
public AuthenticationManager authenticationManagerBean() throws Exception {
return super.authenticationManagerBean();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests().anyRequest().permitAll();
}
}
Controller
import java.security.Principal;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class AuthorizationServerController {
@RequestMapping("/user")
public Principal user(Principal user) {
return user;
}
}
Application.yml
server:
port: 8090
logging:
file: /logs/AuthourizationServer.log
level:
org.springframework: DEBUG
pattern:
file: "%clr(%d{yyyy-MM-dd HH:mm:ss.SSS}){faint} %clr(%5p) %clr(${PID}){magenta} %clr(---){faint} %clr([%15.15t]){faint} %clr(%-40.40logger{39}){cyan} %clr(:){faint} %m%n%wEx"
security:
basic:
enabled: false
Resource Server
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class ResourceApplication {
public static void main(String args) {
SpringApplication.run(ResourceApplication.class, args);
}
}
Web security config
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.actuate.autoconfigure.endpoint.web.WebEndpointProperties;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.oauth2.config.annotation.web.configuration.EnableResourceServer;
import org.springframework.security.oauth2.config.annotation.web.configuration.ResourceServerConfigurerAdapter;
@EnableResourceServer
@Configuration
public class ResourceApplicationWebSecurityConfig extends ResourceServerConfigurerAdapter {
private static final String AUTH_WHITELIST = {
// -- swagger ui
"/v2/api-docs",
"/swagger-resources",
"/swagger-resources/**",
"/configuration/ui",
"/configuration/security",
"/swagger-ui.html",
"/webjars/**"
// other public endpoints of your API may be appended to this array
};
@Autowired
private WebEndpointProperties webEndpointProperties;
@Override
public void configure(HttpSecurity http) throws Exception{
http.authorizeRequests().antMatchers(webEndpointProperties.getBasePath()+"/health").permitAll().and()
.authorizeRequests().antMatchers("/swagger-ui.html").permitAll().and()
.authorizeRequests().antMatchers(AUTH_WHITELIST).permitAll().and()
.authorizeRequests().antMatchers("/nonsecured").permitAll()
.anyRequest().authenticated();
}
}
Rest Controller
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ResourceServerController {
@RequestMapping(value = "/secured", method = RequestMethod.GET)
public String securedResource() {
return "This is Secured Resource";
}
@RequestMapping(value = "/nonsecured", method = RequestMethod.GET)
public String nonSecuredResource() {
return "This is Non Secured Resource";
}
}
Application.yml
server:
port: 8092
security:
oauth2:
resource:
user-info-uri: http://localhost:8090/user
basic:
enabled: false
logging:
file: /logs/ResourceServer.log
level:
org.springframework: DEBUG
pattern:
file: "%clr(%d{yyyy-MM-dd HH:mm:ss.SSS}){faint} %clr(%5p) %clr(${PID}){magenta} %clr(---){faint} %clr([%15.15t]){faint} %clr(%-40.40logger{39}){cyan} %clr(:){faint} %m%n%wEx"
Postman : getting token from Authorization server
Postman : getting token from Authorization server
Querying secured service and getting erro
AuthorizationServer logs
2018-12-30 21:04:40.599 INFO 5144 --- [nio-8090-exec-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring DispatcherServlet 'dispatcherServlet'
2018-12-30 21:04:40.599 INFO 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : Initializing Servlet 'dispatcherServlet'
2018-12-30 21:04:40.599 DEBUG 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : Detected StandardServletMultipartResolver
2018-12-30 21:04:40.599 DEBUG 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : enableLoggingRequestDetails='false': request parameters and headers will be masked to prevent unsafe logging of potentially sensitive data
2018-12-30 21:04:40.599 INFO 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : Completed initialization in 0 ms
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/oauth/token']
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Checking match of request : '/oauth/token'; against '/oauth/token'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : matched
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 1 of 11 in additional filter chain; firing Filter: 'WebAsyncManagerIntegrationFilter'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 2 of 11 in additional filter chain; firing Filter: 'SecurityContextPersistenceFilter'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 3 of 11 in additional filter chain; firing Filter: 'HeaderWriterFilter'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 4 of 11 in additional filter chain; firing Filter: 'LogoutFilter'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', GET]
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'POST /oauth/token' doesn't match 'GET /logout'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', POST]
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Checking match of request : '/oauth/token'; against '/logout'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', PUT]
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'POST /oauth/token' doesn't match 'PUT /logout'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', DELETE]
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'POST /oauth/token' doesn't match 'DELETE /logout'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : No matches found
2018-12-30 21:04:40.615 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 5 of 11 in additional filter chain; firing Filter: 'BasicAuthenticationFilter'
2018-12-30 21:04:40.615 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.www.BasicAuthenticationFilter : Basic Authentication Authorization header found for user 'clientABC'
2018-12-30 21:04:40.631 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.authentication.ProviderManager : Authentication attempt using org.springframework.security.authentication.dao.DaoAuthenticationProvider
2018-12-30 21:04:40.742 DEBUG 5144 --- [nio-8090-exec-1] o.s.b.f.s.DefaultListableBeanFactory : Creating shared instance of singleton bean 'scopedTarget.clientDetailsService'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.b.a.audit.listener.AuditListener : AuditEvent [timestamp=2018-12-30T20:04:40.758Z, principal=clientABC, type=AUTHENTICATION_SUCCESS, data={details=org.springframework.security.web.authentication.WebAuthenticationDetails@b364: RemoteIpAddress: 0:0:0:0:0:0:0:1; SessionId: null}]
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.www.BasicAuthenticationFilter : Authentication success: org.springframework.security.authentication.UsernamePasswordAuthenticationToken@717e3c37: Principal: org.springframework.security.core.userdetails.User@8e817097: Username: clientABC; Password: [PROTECTED]; Enabled: true; AccountNonExpired: true; credentialsNonExpired: true; AccountNonLocked: true; Not granted any authorities; Credentials: [PROTECTED]; Authenticated: true; Details: org.springframework.security.web.authentication.WebAuthenticationDetails@b364: RemoteIpAddress: 0:0:0:0:0:0:0:1; SessionId: null; Not granted any authorities
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 6 of 11 in additional filter chain; firing Filter: 'RequestCacheAwareFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.s.HttpSessionRequestCache : saved request doesn't match
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 7 of 11 in additional filter chain; firing Filter: 'SecurityContextHolderAwareRequestFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 8 of 11 in additional filter chain; firing Filter: 'AnonymousAuthenticationFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.AnonymousAuthenticationFilter : SecurityContextHolder not populated with anonymous token, as it already contained: 'org.springframework.security.authentication.UsernamePasswordAuthenticationToken@717e3c37: Principal: org.springframework.security.core.userdetails.User@8e817097: Username: clientABC; Password: [PROTECTED]; Enabled: true; AccountNonExpired: true; credentialsNonExpired: true; AccountNonLocked: true; Not granted any authorities; Credentials: [PROTECTED]; Authenticated: true; Details: org.springframework.security.web.authentication.WebAuthenticationDetails@b364: RemoteIpAddress: 0:0:0:0:0:0:0:1; SessionId: null; Not granted any authorities'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 9 of 11 in additional filter chain; firing Filter: 'SessionManagementFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] s.CompositeSessionAuthenticationStrategy : Delegating to org.springframework.security.web.authentication.session.ChangeSessionIdAuthenticationStrategy@5a1c3cb4
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 10 of 11 in additional filter chain; firing Filter: 'ExceptionTranslationFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 11 of 11 in additional filter chain; firing Filter: 'FilterSecurityInterceptor'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Checking match of request : '/oauth/token'; against '/oauth/token'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.i.FilterSecurityInterceptor : Secure object: FilterInvocation: URL: /oauth/token; Attributes: [fullyAuthenticated]
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.i.FilterSecurityInterceptor : Previously Authenticated: org.springframework.security.authentication.UsernamePasswordAuthenticationToken@717e3c37: Principal: org.springframework.security.core.userdetails.User@8e817097: Username: clientABC; Password: [PROTECTED]; Enabled: true; AccountNonExpired: true; credentialsNonExpired: true; AccountNonLocked: true; Not granted any authorities; Credentials: [PROTECTED]; Authenticated: true; Details: org.springframework.security.web.authentication.WebAuthenticationDetails@b364: RemoteIpAddress: 0:0:0:0:0:0:0:1; SessionId: null; Not granted any authorities
2018-12-30 21:04:40.773 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.access.vote.AffirmativeBased : Voter: org.springframework.security.web.access.expression.WebExpressionVoter@76d8c502, returned: 1
2018-12-30 21:04:40.773 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.i.FilterSecurityInterceptor : Authorization successful
2018-12-30 21:04:40.773 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.i.FilterSecurityInterceptor : RunAsManager did not change Authentication object
2018-12-30 21:04:40.773 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token reached end of additional filter chain; proceeding with original chain
2018-12-30 21:04:40.789 DEBUG 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : POST "/oauth/token", parameters={masked}
2018-12-30 21:04:40.790 DEBUG 5144 --- [nio-8090-exec-1] .s.o.p.e.FrameworkEndpointHandlerMapping : Mapped to public org.springframework.http.ResponseEntity<org.springframework.security.oauth2.common.OAuth2AccessToken> org.springframework.security.oauth2.provider.endpoint.TokenEndpoint.postAccessToken(java.security.Principal,java.util.Map<java.lang.String, java.lang.String>) throws org.springframework.web.HttpRequestMethodNotSupportedException
2018-12-30 21:04:40.842 DEBUG 5144 --- [nio-8090-exec-1] .s.s.o.p.c.ClientCredentialsTokenGranter : Getting access token for: clientABC
2018-12-30 21:04:40.858 DEBUG 5144 --- [nio-8090-exec-1] o.s.w.s.m.m.a.HttpEntityMethodProcessor : Found 'Content-Type:application/json;charset=UTF-8' in response
2018-12-30 21:04:40.905 DEBUG 5144 --- [nio-8090-exec-1] o.s.w.s.m.m.a.HttpEntityMethodProcessor : Writing [087b1986-b76e-4ff5-8e84-63ecd62e9583]
2018-12-30 21:04:40.920 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.header.writers.HstsHeaderWriter : Not injecting HSTS header since it did not match the requestMatcher org.springframework.security.web.header.writers.HstsHeaderWriter$SecureRequestMatcher@38dba2a
2018-12-30 21:04:40.921 DEBUG 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : Completed 200 OK
2018-12-30 21:04:40.921 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.ExceptionTranslationFilter : Chain processed normally
2018-12-30 21:04:40.921 DEBUG 5144 --- [nio-8090-exec-1] s.s.w.c.SecurityContextPersistenceFilter : SecurityContextHolder now cleared, as request processing completed
Resource Server logs
2018-12-30 21:04:54.158 INFO 8512 --- [nio-8092-exec-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring DispatcherServlet 'dispatcherServlet'
2018-12-30 21:04:54.158 INFO 8512 --- [nio-8092-exec-1] o.s.web.servlet.DispatcherServlet : Initializing Servlet 'dispatcherServlet'
2018-12-30 21:04:54.158 DEBUG 8512 --- [nio-8092-exec-1] o.s.web.servlet.DispatcherServlet : Detected StandardServletMultipartResolver
2018-12-30 21:04:54.159 DEBUG 8512 --- [nio-8092-exec-1] o.s.web.servlet.DispatcherServlet : enableLoggingRequestDetails='false': request parameters and headers will be masked to prevent unsafe logging of potentially sensitive data
2018-12-30 21:04:54.159 INFO 8512 --- [nio-8092-exec-1] o.s.web.servlet.DispatcherServlet : Completed initialization in 1 ms
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 1 of 11 in additional filter chain; firing Filter: 'WebAsyncManagerIntegrationFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 2 of 11 in additional filter chain; firing Filter: 'SecurityContextPersistenceFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 3 of 11 in additional filter chain; firing Filter: 'HeaderWriterFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 4 of 11 in additional filter chain; firing Filter: 'LogoutFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', GET]
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Checking match of request : '/secured'; against '/logout'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', POST]
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'GET /secured' doesn't match 'POST /logout'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', PUT]
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'GET /secured' doesn't match 'PUT /logout'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', DELETE]
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'GET /secured' doesn't match 'DELETE /logout'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : No matches found
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 5 of 11 in additional filter chain; firing Filter: 'OAuth2AuthenticationProcessingFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.o.p.a.BearerTokenExtractor : Token not found in headers. Trying request parameters.
2018-12-30 21:04:54.206 DEBUG 8512 --- [nio-8092-exec-1] p.a.OAuth2AuthenticationProcessingFilter : Authentication request failed: error="invalid_token", error_description="Invalid access token: 087b1986-b76e-4ff5-8e84-63ecd62e9583"
2018-12-30 21:04:54.206 DEBUG 8512 --- [nio-8092-exec-1] o.s.b.a.audit.listener.AuditListener : AuditEvent [timestamp=2018-12-30T20:04:54.206Z, principal=access-token, type=AUTHENTICATION_FAILURE, data={type=org.springframework.security.authentication.BadCredentialsException, message=Invalid access token: 087b1986-b76e-4ff5-8e84-63ecd62e9583}]
2018-12-30 21:04:54.300 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.header.writers.HstsHeaderWriter : Not injecting HSTS header since it did not match the requestMatcher org.springframework.security.web.header.writers.HstsHeaderWriter$SecureRequestMatcher@582fa201
2018-12-30 21:04:54.300 DEBUG 8512 --- [nio-8092-exec-1] s.s.o.p.e.DefaultOAuth2ExceptionRenderer : Written [error="invalid_token", error_description="Invalid access token: 087b1986-b76e-4ff5-8e84-63ecd62e9583"] as "application/json;charset=UTF-8" using [org.springframework.http.converter.json.MappingJackson2HttpMessageConverter@29184e62]
2018-12-30 21:04:54.300 DEBUG 8512 --- [nio-8092-exec-1] s.s.w.c.SecurityContextPersistenceFilter : SecurityContextHolder now cleared, as request processing completed
complete code is available in gihub
https://github.com/harsh-hardaha/springboot2Oauth2
Please ignore Admin server and swagger configuration code in github
spring spring-boot spring-security oauth-2.0 spring-security-oauth2
add a comment |
I have implemented Spring boot 2 + OAuth2 Oauthorization server.
I only want to use Client_credential to secure resource Server
I am able to get access token from Auth server, but when I pass this to access rest api, resource server does not validate it from authorization server and gives invalid access token error, I am using postman to get access token and to quest resource server.
Authorization Server
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class AuthorizationApplication {
public static void main(String args) {
SpringApplication.run(AuthorizationApplication.class, args);
}
}
Authorization server config
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.oauth2.config.annotation.configurers.ClientDetailsServiceConfigurer;
import org.springframework.security.oauth2.config.annotation.web.configuration.AuthorizationServerConfigurerAdapter;
import org.springframework.security.oauth2.config.annotation.web.configuration.EnableAuthorizationServer;
import org.springframework.security.oauth2.config.annotation.web.configurers.AuthorizationServerEndpointsConfigurer;
import org.springframework.security.oauth2.config.annotation.web.configurers.AuthorizationServerSecurityConfigurer;
import org.springframework.security.oauth2.provider.token.store.InMemoryTokenStore;
@Configuration
@EnableAuthorizationServer
public class AuthorizationServerConfig extends AuthorizationServerConfigurerAdapter {
private static final String SERVER_RESOURCE_ID = "oauth2-server";
private static InMemoryTokenStore tokenStore = new InMemoryTokenStore();
@Autowired
@Qualifier("authenticationManagerBean")
private AuthenticationManager authenticationManager;
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints.authenticationManager(authenticationManager).tokenStore(tokenStore).approvalStoreDisabled();
}
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.inMemory().withClient("clientABC").secret("{noop}secretXYZ")
.authorizedGrantTypes("client_credentials")
.scopes("resource-server-read", "resource-server-write")
.resourceIds(SERVER_RESOURCE_ID);
}
@Override
public void configure(AuthorizationServerSecurityConfigurer security) throws Exception {
security.tokenKeyAccess("permitAll()")
.checkTokenAccess("isAuthenticated()");
}
}
Authorization server web config
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
public class AuthorizationServerWebConfig extends WebSecurityConfigurerAdapter {
@Bean
@Override
public AuthenticationManager authenticationManagerBean() throws Exception {
return super.authenticationManagerBean();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests().anyRequest().permitAll();
}
}
Controller
import java.security.Principal;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class AuthorizationServerController {
@RequestMapping("/user")
public Principal user(Principal user) {
return user;
}
}
Application.yml
server:
port: 8090
logging:
file: /logs/AuthourizationServer.log
level:
org.springframework: DEBUG
pattern:
file: "%clr(%d{yyyy-MM-dd HH:mm:ss.SSS}){faint} %clr(%5p) %clr(${PID}){magenta} %clr(---){faint} %clr([%15.15t]){faint} %clr(%-40.40logger{39}){cyan} %clr(:){faint} %m%n%wEx"
security:
basic:
enabled: false
Resource Server
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class ResourceApplication {
public static void main(String args) {
SpringApplication.run(ResourceApplication.class, args);
}
}
Web security config
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.actuate.autoconfigure.endpoint.web.WebEndpointProperties;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.oauth2.config.annotation.web.configuration.EnableResourceServer;
import org.springframework.security.oauth2.config.annotation.web.configuration.ResourceServerConfigurerAdapter;
@EnableResourceServer
@Configuration
public class ResourceApplicationWebSecurityConfig extends ResourceServerConfigurerAdapter {
private static final String AUTH_WHITELIST = {
// -- swagger ui
"/v2/api-docs",
"/swagger-resources",
"/swagger-resources/**",
"/configuration/ui",
"/configuration/security",
"/swagger-ui.html",
"/webjars/**"
// other public endpoints of your API may be appended to this array
};
@Autowired
private WebEndpointProperties webEndpointProperties;
@Override
public void configure(HttpSecurity http) throws Exception{
http.authorizeRequests().antMatchers(webEndpointProperties.getBasePath()+"/health").permitAll().and()
.authorizeRequests().antMatchers("/swagger-ui.html").permitAll().and()
.authorizeRequests().antMatchers(AUTH_WHITELIST).permitAll().and()
.authorizeRequests().antMatchers("/nonsecured").permitAll()
.anyRequest().authenticated();
}
}
Rest Controller
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ResourceServerController {
@RequestMapping(value = "/secured", method = RequestMethod.GET)
public String securedResource() {
return "This is Secured Resource";
}
@RequestMapping(value = "/nonsecured", method = RequestMethod.GET)
public String nonSecuredResource() {
return "This is Non Secured Resource";
}
}
Application.yml
server:
port: 8092
security:
oauth2:
resource:
user-info-uri: http://localhost:8090/user
basic:
enabled: false
logging:
file: /logs/ResourceServer.log
level:
org.springframework: DEBUG
pattern:
file: "%clr(%d{yyyy-MM-dd HH:mm:ss.SSS}){faint} %clr(%5p) %clr(${PID}){magenta} %clr(---){faint} %clr([%15.15t]){faint} %clr(%-40.40logger{39}){cyan} %clr(:){faint} %m%n%wEx"
Postman : getting token from Authorization server
Postman : getting token from Authorization server
Querying secured service and getting erro
AuthorizationServer logs
2018-12-30 21:04:40.599 INFO 5144 --- [nio-8090-exec-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring DispatcherServlet 'dispatcherServlet'
2018-12-30 21:04:40.599 INFO 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : Initializing Servlet 'dispatcherServlet'
2018-12-30 21:04:40.599 DEBUG 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : Detected StandardServletMultipartResolver
2018-12-30 21:04:40.599 DEBUG 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : enableLoggingRequestDetails='false': request parameters and headers will be masked to prevent unsafe logging of potentially sensitive data
2018-12-30 21:04:40.599 INFO 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : Completed initialization in 0 ms
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/oauth/token']
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Checking match of request : '/oauth/token'; against '/oauth/token'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : matched
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 1 of 11 in additional filter chain; firing Filter: 'WebAsyncManagerIntegrationFilter'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 2 of 11 in additional filter chain; firing Filter: 'SecurityContextPersistenceFilter'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 3 of 11 in additional filter chain; firing Filter: 'HeaderWriterFilter'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 4 of 11 in additional filter chain; firing Filter: 'LogoutFilter'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', GET]
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'POST /oauth/token' doesn't match 'GET /logout'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', POST]
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Checking match of request : '/oauth/token'; against '/logout'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', PUT]
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'POST /oauth/token' doesn't match 'PUT /logout'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', DELETE]
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'POST /oauth/token' doesn't match 'DELETE /logout'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : No matches found
2018-12-30 21:04:40.615 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 5 of 11 in additional filter chain; firing Filter: 'BasicAuthenticationFilter'
2018-12-30 21:04:40.615 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.www.BasicAuthenticationFilter : Basic Authentication Authorization header found for user 'clientABC'
2018-12-30 21:04:40.631 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.authentication.ProviderManager : Authentication attempt using org.springframework.security.authentication.dao.DaoAuthenticationProvider
2018-12-30 21:04:40.742 DEBUG 5144 --- [nio-8090-exec-1] o.s.b.f.s.DefaultListableBeanFactory : Creating shared instance of singleton bean 'scopedTarget.clientDetailsService'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.b.a.audit.listener.AuditListener : AuditEvent [timestamp=2018-12-30T20:04:40.758Z, principal=clientABC, type=AUTHENTICATION_SUCCESS, data={details=org.springframework.security.web.authentication.WebAuthenticationDetails@b364: RemoteIpAddress: 0:0:0:0:0:0:0:1; SessionId: null}]
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.www.BasicAuthenticationFilter : Authentication success: org.springframework.security.authentication.UsernamePasswordAuthenticationToken@717e3c37: Principal: org.springframework.security.core.userdetails.User@8e817097: Username: clientABC; Password: [PROTECTED]; Enabled: true; AccountNonExpired: true; credentialsNonExpired: true; AccountNonLocked: true; Not granted any authorities; Credentials: [PROTECTED]; Authenticated: true; Details: org.springframework.security.web.authentication.WebAuthenticationDetails@b364: RemoteIpAddress: 0:0:0:0:0:0:0:1; SessionId: null; Not granted any authorities
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 6 of 11 in additional filter chain; firing Filter: 'RequestCacheAwareFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.s.HttpSessionRequestCache : saved request doesn't match
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 7 of 11 in additional filter chain; firing Filter: 'SecurityContextHolderAwareRequestFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 8 of 11 in additional filter chain; firing Filter: 'AnonymousAuthenticationFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.AnonymousAuthenticationFilter : SecurityContextHolder not populated with anonymous token, as it already contained: 'org.springframework.security.authentication.UsernamePasswordAuthenticationToken@717e3c37: Principal: org.springframework.security.core.userdetails.User@8e817097: Username: clientABC; Password: [PROTECTED]; Enabled: true; AccountNonExpired: true; credentialsNonExpired: true; AccountNonLocked: true; Not granted any authorities; Credentials: [PROTECTED]; Authenticated: true; Details: org.springframework.security.web.authentication.WebAuthenticationDetails@b364: RemoteIpAddress: 0:0:0:0:0:0:0:1; SessionId: null; Not granted any authorities'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 9 of 11 in additional filter chain; firing Filter: 'SessionManagementFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] s.CompositeSessionAuthenticationStrategy : Delegating to org.springframework.security.web.authentication.session.ChangeSessionIdAuthenticationStrategy@5a1c3cb4
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 10 of 11 in additional filter chain; firing Filter: 'ExceptionTranslationFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 11 of 11 in additional filter chain; firing Filter: 'FilterSecurityInterceptor'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Checking match of request : '/oauth/token'; against '/oauth/token'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.i.FilterSecurityInterceptor : Secure object: FilterInvocation: URL: /oauth/token; Attributes: [fullyAuthenticated]
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.i.FilterSecurityInterceptor : Previously Authenticated: org.springframework.security.authentication.UsernamePasswordAuthenticationToken@717e3c37: Principal: org.springframework.security.core.userdetails.User@8e817097: Username: clientABC; Password: [PROTECTED]; Enabled: true; AccountNonExpired: true; credentialsNonExpired: true; AccountNonLocked: true; Not granted any authorities; Credentials: [PROTECTED]; Authenticated: true; Details: org.springframework.security.web.authentication.WebAuthenticationDetails@b364: RemoteIpAddress: 0:0:0:0:0:0:0:1; SessionId: null; Not granted any authorities
2018-12-30 21:04:40.773 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.access.vote.AffirmativeBased : Voter: org.springframework.security.web.access.expression.WebExpressionVoter@76d8c502, returned: 1
2018-12-30 21:04:40.773 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.i.FilterSecurityInterceptor : Authorization successful
2018-12-30 21:04:40.773 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.i.FilterSecurityInterceptor : RunAsManager did not change Authentication object
2018-12-30 21:04:40.773 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token reached end of additional filter chain; proceeding with original chain
2018-12-30 21:04:40.789 DEBUG 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : POST "/oauth/token", parameters={masked}
2018-12-30 21:04:40.790 DEBUG 5144 --- [nio-8090-exec-1] .s.o.p.e.FrameworkEndpointHandlerMapping : Mapped to public org.springframework.http.ResponseEntity<org.springframework.security.oauth2.common.OAuth2AccessToken> org.springframework.security.oauth2.provider.endpoint.TokenEndpoint.postAccessToken(java.security.Principal,java.util.Map<java.lang.String, java.lang.String>) throws org.springframework.web.HttpRequestMethodNotSupportedException
2018-12-30 21:04:40.842 DEBUG 5144 --- [nio-8090-exec-1] .s.s.o.p.c.ClientCredentialsTokenGranter : Getting access token for: clientABC
2018-12-30 21:04:40.858 DEBUG 5144 --- [nio-8090-exec-1] o.s.w.s.m.m.a.HttpEntityMethodProcessor : Found 'Content-Type:application/json;charset=UTF-8' in response
2018-12-30 21:04:40.905 DEBUG 5144 --- [nio-8090-exec-1] o.s.w.s.m.m.a.HttpEntityMethodProcessor : Writing [087b1986-b76e-4ff5-8e84-63ecd62e9583]
2018-12-30 21:04:40.920 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.header.writers.HstsHeaderWriter : Not injecting HSTS header since it did not match the requestMatcher org.springframework.security.web.header.writers.HstsHeaderWriter$SecureRequestMatcher@38dba2a
2018-12-30 21:04:40.921 DEBUG 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : Completed 200 OK
2018-12-30 21:04:40.921 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.ExceptionTranslationFilter : Chain processed normally
2018-12-30 21:04:40.921 DEBUG 5144 --- [nio-8090-exec-1] s.s.w.c.SecurityContextPersistenceFilter : SecurityContextHolder now cleared, as request processing completed
Resource Server logs
2018-12-30 21:04:54.158 INFO 8512 --- [nio-8092-exec-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring DispatcherServlet 'dispatcherServlet'
2018-12-30 21:04:54.158 INFO 8512 --- [nio-8092-exec-1] o.s.web.servlet.DispatcherServlet : Initializing Servlet 'dispatcherServlet'
2018-12-30 21:04:54.158 DEBUG 8512 --- [nio-8092-exec-1] o.s.web.servlet.DispatcherServlet : Detected StandardServletMultipartResolver
2018-12-30 21:04:54.159 DEBUG 8512 --- [nio-8092-exec-1] o.s.web.servlet.DispatcherServlet : enableLoggingRequestDetails='false': request parameters and headers will be masked to prevent unsafe logging of potentially sensitive data
2018-12-30 21:04:54.159 INFO 8512 --- [nio-8092-exec-1] o.s.web.servlet.DispatcherServlet : Completed initialization in 1 ms
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 1 of 11 in additional filter chain; firing Filter: 'WebAsyncManagerIntegrationFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 2 of 11 in additional filter chain; firing Filter: 'SecurityContextPersistenceFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 3 of 11 in additional filter chain; firing Filter: 'HeaderWriterFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 4 of 11 in additional filter chain; firing Filter: 'LogoutFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', GET]
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Checking match of request : '/secured'; against '/logout'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', POST]
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'GET /secured' doesn't match 'POST /logout'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', PUT]
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'GET /secured' doesn't match 'PUT /logout'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', DELETE]
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'GET /secured' doesn't match 'DELETE /logout'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : No matches found
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 5 of 11 in additional filter chain; firing Filter: 'OAuth2AuthenticationProcessingFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.o.p.a.BearerTokenExtractor : Token not found in headers. Trying request parameters.
2018-12-30 21:04:54.206 DEBUG 8512 --- [nio-8092-exec-1] p.a.OAuth2AuthenticationProcessingFilter : Authentication request failed: error="invalid_token", error_description="Invalid access token: 087b1986-b76e-4ff5-8e84-63ecd62e9583"
2018-12-30 21:04:54.206 DEBUG 8512 --- [nio-8092-exec-1] o.s.b.a.audit.listener.AuditListener : AuditEvent [timestamp=2018-12-30T20:04:54.206Z, principal=access-token, type=AUTHENTICATION_FAILURE, data={type=org.springframework.security.authentication.BadCredentialsException, message=Invalid access token: 087b1986-b76e-4ff5-8e84-63ecd62e9583}]
2018-12-30 21:04:54.300 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.header.writers.HstsHeaderWriter : Not injecting HSTS header since it did not match the requestMatcher org.springframework.security.web.header.writers.HstsHeaderWriter$SecureRequestMatcher@582fa201
2018-12-30 21:04:54.300 DEBUG 8512 --- [nio-8092-exec-1] s.s.o.p.e.DefaultOAuth2ExceptionRenderer : Written [error="invalid_token", error_description="Invalid access token: 087b1986-b76e-4ff5-8e84-63ecd62e9583"] as "application/json;charset=UTF-8" using [org.springframework.http.converter.json.MappingJackson2HttpMessageConverter@29184e62]
2018-12-30 21:04:54.300 DEBUG 8512 --- [nio-8092-exec-1] s.s.w.c.SecurityContextPersistenceFilter : SecurityContextHolder now cleared, as request processing completed
complete code is available in gihub
https://github.com/harsh-hardaha/springboot2Oauth2
Please ignore Admin server and swagger configuration code in github
spring spring-boot spring-security oauth-2.0 spring-security-oauth2
Do you use the same token repository for authorization server and resource server? You didn't configure atoken-info-uri
.
– dur
Dec 30 '18 at 20:25
1
I have debugged code on Resource server side, and found that method public Authentication authenticate(Authentication authentication) in org.springframework.security.oauth2.provider.authentication.OAuth2AuthenticationManager uses Defaulttokenservice which uses inmemorytokenstore in resource server side which is empty.
– Harsh Hardaha
Dec 30 '18 at 20:36
@dur No I do not use same token repository, both server are independent. can you please let me know what will be implementation of token-info-uri endpoint in authorization server side?
– Harsh Hardaha
Dec 30 '18 at 20:38
add a comment |
I have implemented Spring boot 2 + OAuth2 Oauthorization server.
I only want to use Client_credential to secure resource Server
I am able to get access token from Auth server, but when I pass this to access rest api, resource server does not validate it from authorization server and gives invalid access token error, I am using postman to get access token and to quest resource server.
Authorization Server
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class AuthorizationApplication {
public static void main(String args) {
SpringApplication.run(AuthorizationApplication.class, args);
}
}
Authorization server config
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.oauth2.config.annotation.configurers.ClientDetailsServiceConfigurer;
import org.springframework.security.oauth2.config.annotation.web.configuration.AuthorizationServerConfigurerAdapter;
import org.springframework.security.oauth2.config.annotation.web.configuration.EnableAuthorizationServer;
import org.springframework.security.oauth2.config.annotation.web.configurers.AuthorizationServerEndpointsConfigurer;
import org.springframework.security.oauth2.config.annotation.web.configurers.AuthorizationServerSecurityConfigurer;
import org.springframework.security.oauth2.provider.token.store.InMemoryTokenStore;
@Configuration
@EnableAuthorizationServer
public class AuthorizationServerConfig extends AuthorizationServerConfigurerAdapter {
private static final String SERVER_RESOURCE_ID = "oauth2-server";
private static InMemoryTokenStore tokenStore = new InMemoryTokenStore();
@Autowired
@Qualifier("authenticationManagerBean")
private AuthenticationManager authenticationManager;
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints.authenticationManager(authenticationManager).tokenStore(tokenStore).approvalStoreDisabled();
}
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.inMemory().withClient("clientABC").secret("{noop}secretXYZ")
.authorizedGrantTypes("client_credentials")
.scopes("resource-server-read", "resource-server-write")
.resourceIds(SERVER_RESOURCE_ID);
}
@Override
public void configure(AuthorizationServerSecurityConfigurer security) throws Exception {
security.tokenKeyAccess("permitAll()")
.checkTokenAccess("isAuthenticated()");
}
}
Authorization server web config
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
public class AuthorizationServerWebConfig extends WebSecurityConfigurerAdapter {
@Bean
@Override
public AuthenticationManager authenticationManagerBean() throws Exception {
return super.authenticationManagerBean();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests().anyRequest().permitAll();
}
}
Controller
import java.security.Principal;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class AuthorizationServerController {
@RequestMapping("/user")
public Principal user(Principal user) {
return user;
}
}
Application.yml
server:
port: 8090
logging:
file: /logs/AuthourizationServer.log
level:
org.springframework: DEBUG
pattern:
file: "%clr(%d{yyyy-MM-dd HH:mm:ss.SSS}){faint} %clr(%5p) %clr(${PID}){magenta} %clr(---){faint} %clr([%15.15t]){faint} %clr(%-40.40logger{39}){cyan} %clr(:){faint} %m%n%wEx"
security:
basic:
enabled: false
Resource Server
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class ResourceApplication {
public static void main(String args) {
SpringApplication.run(ResourceApplication.class, args);
}
}
Web security config
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.actuate.autoconfigure.endpoint.web.WebEndpointProperties;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.oauth2.config.annotation.web.configuration.EnableResourceServer;
import org.springframework.security.oauth2.config.annotation.web.configuration.ResourceServerConfigurerAdapter;
@EnableResourceServer
@Configuration
public class ResourceApplicationWebSecurityConfig extends ResourceServerConfigurerAdapter {
private static final String AUTH_WHITELIST = {
// -- swagger ui
"/v2/api-docs",
"/swagger-resources",
"/swagger-resources/**",
"/configuration/ui",
"/configuration/security",
"/swagger-ui.html",
"/webjars/**"
// other public endpoints of your API may be appended to this array
};
@Autowired
private WebEndpointProperties webEndpointProperties;
@Override
public void configure(HttpSecurity http) throws Exception{
http.authorizeRequests().antMatchers(webEndpointProperties.getBasePath()+"/health").permitAll().and()
.authorizeRequests().antMatchers("/swagger-ui.html").permitAll().and()
.authorizeRequests().antMatchers(AUTH_WHITELIST).permitAll().and()
.authorizeRequests().antMatchers("/nonsecured").permitAll()
.anyRequest().authenticated();
}
}
Rest Controller
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ResourceServerController {
@RequestMapping(value = "/secured", method = RequestMethod.GET)
public String securedResource() {
return "This is Secured Resource";
}
@RequestMapping(value = "/nonsecured", method = RequestMethod.GET)
public String nonSecuredResource() {
return "This is Non Secured Resource";
}
}
Application.yml
server:
port: 8092
security:
oauth2:
resource:
user-info-uri: http://localhost:8090/user
basic:
enabled: false
logging:
file: /logs/ResourceServer.log
level:
org.springframework: DEBUG
pattern:
file: "%clr(%d{yyyy-MM-dd HH:mm:ss.SSS}){faint} %clr(%5p) %clr(${PID}){magenta} %clr(---){faint} %clr([%15.15t]){faint} %clr(%-40.40logger{39}){cyan} %clr(:){faint} %m%n%wEx"
Postman : getting token from Authorization server
Postman : getting token from Authorization server
Querying secured service and getting erro
AuthorizationServer logs
2018-12-30 21:04:40.599 INFO 5144 --- [nio-8090-exec-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring DispatcherServlet 'dispatcherServlet'
2018-12-30 21:04:40.599 INFO 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : Initializing Servlet 'dispatcherServlet'
2018-12-30 21:04:40.599 DEBUG 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : Detected StandardServletMultipartResolver
2018-12-30 21:04:40.599 DEBUG 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : enableLoggingRequestDetails='false': request parameters and headers will be masked to prevent unsafe logging of potentially sensitive data
2018-12-30 21:04:40.599 INFO 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : Completed initialization in 0 ms
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/oauth/token']
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Checking match of request : '/oauth/token'; against '/oauth/token'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : matched
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 1 of 11 in additional filter chain; firing Filter: 'WebAsyncManagerIntegrationFilter'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 2 of 11 in additional filter chain; firing Filter: 'SecurityContextPersistenceFilter'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 3 of 11 in additional filter chain; firing Filter: 'HeaderWriterFilter'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 4 of 11 in additional filter chain; firing Filter: 'LogoutFilter'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', GET]
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'POST /oauth/token' doesn't match 'GET /logout'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', POST]
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Checking match of request : '/oauth/token'; against '/logout'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', PUT]
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'POST /oauth/token' doesn't match 'PUT /logout'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', DELETE]
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'POST /oauth/token' doesn't match 'DELETE /logout'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : No matches found
2018-12-30 21:04:40.615 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 5 of 11 in additional filter chain; firing Filter: 'BasicAuthenticationFilter'
2018-12-30 21:04:40.615 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.www.BasicAuthenticationFilter : Basic Authentication Authorization header found for user 'clientABC'
2018-12-30 21:04:40.631 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.authentication.ProviderManager : Authentication attempt using org.springframework.security.authentication.dao.DaoAuthenticationProvider
2018-12-30 21:04:40.742 DEBUG 5144 --- [nio-8090-exec-1] o.s.b.f.s.DefaultListableBeanFactory : Creating shared instance of singleton bean 'scopedTarget.clientDetailsService'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.b.a.audit.listener.AuditListener : AuditEvent [timestamp=2018-12-30T20:04:40.758Z, principal=clientABC, type=AUTHENTICATION_SUCCESS, data={details=org.springframework.security.web.authentication.WebAuthenticationDetails@b364: RemoteIpAddress: 0:0:0:0:0:0:0:1; SessionId: null}]
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.www.BasicAuthenticationFilter : Authentication success: org.springframework.security.authentication.UsernamePasswordAuthenticationToken@717e3c37: Principal: org.springframework.security.core.userdetails.User@8e817097: Username: clientABC; Password: [PROTECTED]; Enabled: true; AccountNonExpired: true; credentialsNonExpired: true; AccountNonLocked: true; Not granted any authorities; Credentials: [PROTECTED]; Authenticated: true; Details: org.springframework.security.web.authentication.WebAuthenticationDetails@b364: RemoteIpAddress: 0:0:0:0:0:0:0:1; SessionId: null; Not granted any authorities
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 6 of 11 in additional filter chain; firing Filter: 'RequestCacheAwareFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.s.HttpSessionRequestCache : saved request doesn't match
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 7 of 11 in additional filter chain; firing Filter: 'SecurityContextHolderAwareRequestFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 8 of 11 in additional filter chain; firing Filter: 'AnonymousAuthenticationFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.AnonymousAuthenticationFilter : SecurityContextHolder not populated with anonymous token, as it already contained: 'org.springframework.security.authentication.UsernamePasswordAuthenticationToken@717e3c37: Principal: org.springframework.security.core.userdetails.User@8e817097: Username: clientABC; Password: [PROTECTED]; Enabled: true; AccountNonExpired: true; credentialsNonExpired: true; AccountNonLocked: true; Not granted any authorities; Credentials: [PROTECTED]; Authenticated: true; Details: org.springframework.security.web.authentication.WebAuthenticationDetails@b364: RemoteIpAddress: 0:0:0:0:0:0:0:1; SessionId: null; Not granted any authorities'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 9 of 11 in additional filter chain; firing Filter: 'SessionManagementFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] s.CompositeSessionAuthenticationStrategy : Delegating to org.springframework.security.web.authentication.session.ChangeSessionIdAuthenticationStrategy@5a1c3cb4
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 10 of 11 in additional filter chain; firing Filter: 'ExceptionTranslationFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 11 of 11 in additional filter chain; firing Filter: 'FilterSecurityInterceptor'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Checking match of request : '/oauth/token'; against '/oauth/token'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.i.FilterSecurityInterceptor : Secure object: FilterInvocation: URL: /oauth/token; Attributes: [fullyAuthenticated]
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.i.FilterSecurityInterceptor : Previously Authenticated: org.springframework.security.authentication.UsernamePasswordAuthenticationToken@717e3c37: Principal: org.springframework.security.core.userdetails.User@8e817097: Username: clientABC; Password: [PROTECTED]; Enabled: true; AccountNonExpired: true; credentialsNonExpired: true; AccountNonLocked: true; Not granted any authorities; Credentials: [PROTECTED]; Authenticated: true; Details: org.springframework.security.web.authentication.WebAuthenticationDetails@b364: RemoteIpAddress: 0:0:0:0:0:0:0:1; SessionId: null; Not granted any authorities
2018-12-30 21:04:40.773 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.access.vote.AffirmativeBased : Voter: org.springframework.security.web.access.expression.WebExpressionVoter@76d8c502, returned: 1
2018-12-30 21:04:40.773 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.i.FilterSecurityInterceptor : Authorization successful
2018-12-30 21:04:40.773 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.i.FilterSecurityInterceptor : RunAsManager did not change Authentication object
2018-12-30 21:04:40.773 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token reached end of additional filter chain; proceeding with original chain
2018-12-30 21:04:40.789 DEBUG 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : POST "/oauth/token", parameters={masked}
2018-12-30 21:04:40.790 DEBUG 5144 --- [nio-8090-exec-1] .s.o.p.e.FrameworkEndpointHandlerMapping : Mapped to public org.springframework.http.ResponseEntity<org.springframework.security.oauth2.common.OAuth2AccessToken> org.springframework.security.oauth2.provider.endpoint.TokenEndpoint.postAccessToken(java.security.Principal,java.util.Map<java.lang.String, java.lang.String>) throws org.springframework.web.HttpRequestMethodNotSupportedException
2018-12-30 21:04:40.842 DEBUG 5144 --- [nio-8090-exec-1] .s.s.o.p.c.ClientCredentialsTokenGranter : Getting access token for: clientABC
2018-12-30 21:04:40.858 DEBUG 5144 --- [nio-8090-exec-1] o.s.w.s.m.m.a.HttpEntityMethodProcessor : Found 'Content-Type:application/json;charset=UTF-8' in response
2018-12-30 21:04:40.905 DEBUG 5144 --- [nio-8090-exec-1] o.s.w.s.m.m.a.HttpEntityMethodProcessor : Writing [087b1986-b76e-4ff5-8e84-63ecd62e9583]
2018-12-30 21:04:40.920 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.header.writers.HstsHeaderWriter : Not injecting HSTS header since it did not match the requestMatcher org.springframework.security.web.header.writers.HstsHeaderWriter$SecureRequestMatcher@38dba2a
2018-12-30 21:04:40.921 DEBUG 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : Completed 200 OK
2018-12-30 21:04:40.921 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.ExceptionTranslationFilter : Chain processed normally
2018-12-30 21:04:40.921 DEBUG 5144 --- [nio-8090-exec-1] s.s.w.c.SecurityContextPersistenceFilter : SecurityContextHolder now cleared, as request processing completed
Resource Server logs
2018-12-30 21:04:54.158 INFO 8512 --- [nio-8092-exec-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring DispatcherServlet 'dispatcherServlet'
2018-12-30 21:04:54.158 INFO 8512 --- [nio-8092-exec-1] o.s.web.servlet.DispatcherServlet : Initializing Servlet 'dispatcherServlet'
2018-12-30 21:04:54.158 DEBUG 8512 --- [nio-8092-exec-1] o.s.web.servlet.DispatcherServlet : Detected StandardServletMultipartResolver
2018-12-30 21:04:54.159 DEBUG 8512 --- [nio-8092-exec-1] o.s.web.servlet.DispatcherServlet : enableLoggingRequestDetails='false': request parameters and headers will be masked to prevent unsafe logging of potentially sensitive data
2018-12-30 21:04:54.159 INFO 8512 --- [nio-8092-exec-1] o.s.web.servlet.DispatcherServlet : Completed initialization in 1 ms
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 1 of 11 in additional filter chain; firing Filter: 'WebAsyncManagerIntegrationFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 2 of 11 in additional filter chain; firing Filter: 'SecurityContextPersistenceFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 3 of 11 in additional filter chain; firing Filter: 'HeaderWriterFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 4 of 11 in additional filter chain; firing Filter: 'LogoutFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', GET]
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Checking match of request : '/secured'; against '/logout'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', POST]
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'GET /secured' doesn't match 'POST /logout'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', PUT]
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'GET /secured' doesn't match 'PUT /logout'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', DELETE]
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'GET /secured' doesn't match 'DELETE /logout'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : No matches found
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 5 of 11 in additional filter chain; firing Filter: 'OAuth2AuthenticationProcessingFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.o.p.a.BearerTokenExtractor : Token not found in headers. Trying request parameters.
2018-12-30 21:04:54.206 DEBUG 8512 --- [nio-8092-exec-1] p.a.OAuth2AuthenticationProcessingFilter : Authentication request failed: error="invalid_token", error_description="Invalid access token: 087b1986-b76e-4ff5-8e84-63ecd62e9583"
2018-12-30 21:04:54.206 DEBUG 8512 --- [nio-8092-exec-1] o.s.b.a.audit.listener.AuditListener : AuditEvent [timestamp=2018-12-30T20:04:54.206Z, principal=access-token, type=AUTHENTICATION_FAILURE, data={type=org.springframework.security.authentication.BadCredentialsException, message=Invalid access token: 087b1986-b76e-4ff5-8e84-63ecd62e9583}]
2018-12-30 21:04:54.300 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.header.writers.HstsHeaderWriter : Not injecting HSTS header since it did not match the requestMatcher org.springframework.security.web.header.writers.HstsHeaderWriter$SecureRequestMatcher@582fa201
2018-12-30 21:04:54.300 DEBUG 8512 --- [nio-8092-exec-1] s.s.o.p.e.DefaultOAuth2ExceptionRenderer : Written [error="invalid_token", error_description="Invalid access token: 087b1986-b76e-4ff5-8e84-63ecd62e9583"] as "application/json;charset=UTF-8" using [org.springframework.http.converter.json.MappingJackson2HttpMessageConverter@29184e62]
2018-12-30 21:04:54.300 DEBUG 8512 --- [nio-8092-exec-1] s.s.w.c.SecurityContextPersistenceFilter : SecurityContextHolder now cleared, as request processing completed
complete code is available in gihub
https://github.com/harsh-hardaha/springboot2Oauth2
Please ignore Admin server and swagger configuration code in github
spring spring-boot spring-security oauth-2.0 spring-security-oauth2
I have implemented Spring boot 2 + OAuth2 Oauthorization server.
I only want to use Client_credential to secure resource Server
I am able to get access token from Auth server, but when I pass this to access rest api, resource server does not validate it from authorization server and gives invalid access token error, I am using postman to get access token and to quest resource server.
Authorization Server
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class AuthorizationApplication {
public static void main(String args) {
SpringApplication.run(AuthorizationApplication.class, args);
}
}
Authorization server config
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.oauth2.config.annotation.configurers.ClientDetailsServiceConfigurer;
import org.springframework.security.oauth2.config.annotation.web.configuration.AuthorizationServerConfigurerAdapter;
import org.springframework.security.oauth2.config.annotation.web.configuration.EnableAuthorizationServer;
import org.springframework.security.oauth2.config.annotation.web.configurers.AuthorizationServerEndpointsConfigurer;
import org.springframework.security.oauth2.config.annotation.web.configurers.AuthorizationServerSecurityConfigurer;
import org.springframework.security.oauth2.provider.token.store.InMemoryTokenStore;
@Configuration
@EnableAuthorizationServer
public class AuthorizationServerConfig extends AuthorizationServerConfigurerAdapter {
private static final String SERVER_RESOURCE_ID = "oauth2-server";
private static InMemoryTokenStore tokenStore = new InMemoryTokenStore();
@Autowired
@Qualifier("authenticationManagerBean")
private AuthenticationManager authenticationManager;
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints.authenticationManager(authenticationManager).tokenStore(tokenStore).approvalStoreDisabled();
}
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.inMemory().withClient("clientABC").secret("{noop}secretXYZ")
.authorizedGrantTypes("client_credentials")
.scopes("resource-server-read", "resource-server-write")
.resourceIds(SERVER_RESOURCE_ID);
}
@Override
public void configure(AuthorizationServerSecurityConfigurer security) throws Exception {
security.tokenKeyAccess("permitAll()")
.checkTokenAccess("isAuthenticated()");
}
}
Authorization server web config
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
public class AuthorizationServerWebConfig extends WebSecurityConfigurerAdapter {
@Bean
@Override
public AuthenticationManager authenticationManagerBean() throws Exception {
return super.authenticationManagerBean();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests().anyRequest().permitAll();
}
}
Controller
import java.security.Principal;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class AuthorizationServerController {
@RequestMapping("/user")
public Principal user(Principal user) {
return user;
}
}
Application.yml
server:
port: 8090
logging:
file: /logs/AuthourizationServer.log
level:
org.springframework: DEBUG
pattern:
file: "%clr(%d{yyyy-MM-dd HH:mm:ss.SSS}){faint} %clr(%5p) %clr(${PID}){magenta} %clr(---){faint} %clr([%15.15t]){faint} %clr(%-40.40logger{39}){cyan} %clr(:){faint} %m%n%wEx"
security:
basic:
enabled: false
Resource Server
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class ResourceApplication {
public static void main(String args) {
SpringApplication.run(ResourceApplication.class, args);
}
}
Web security config
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.actuate.autoconfigure.endpoint.web.WebEndpointProperties;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.oauth2.config.annotation.web.configuration.EnableResourceServer;
import org.springframework.security.oauth2.config.annotation.web.configuration.ResourceServerConfigurerAdapter;
@EnableResourceServer
@Configuration
public class ResourceApplicationWebSecurityConfig extends ResourceServerConfigurerAdapter {
private static final String AUTH_WHITELIST = {
// -- swagger ui
"/v2/api-docs",
"/swagger-resources",
"/swagger-resources/**",
"/configuration/ui",
"/configuration/security",
"/swagger-ui.html",
"/webjars/**"
// other public endpoints of your API may be appended to this array
};
@Autowired
private WebEndpointProperties webEndpointProperties;
@Override
public void configure(HttpSecurity http) throws Exception{
http.authorizeRequests().antMatchers(webEndpointProperties.getBasePath()+"/health").permitAll().and()
.authorizeRequests().antMatchers("/swagger-ui.html").permitAll().and()
.authorizeRequests().antMatchers(AUTH_WHITELIST).permitAll().and()
.authorizeRequests().antMatchers("/nonsecured").permitAll()
.anyRequest().authenticated();
}
}
Rest Controller
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ResourceServerController {
@RequestMapping(value = "/secured", method = RequestMethod.GET)
public String securedResource() {
return "This is Secured Resource";
}
@RequestMapping(value = "/nonsecured", method = RequestMethod.GET)
public String nonSecuredResource() {
return "This is Non Secured Resource";
}
}
Application.yml
server:
port: 8092
security:
oauth2:
resource:
user-info-uri: http://localhost:8090/user
basic:
enabled: false
logging:
file: /logs/ResourceServer.log
level:
org.springframework: DEBUG
pattern:
file: "%clr(%d{yyyy-MM-dd HH:mm:ss.SSS}){faint} %clr(%5p) %clr(${PID}){magenta} %clr(---){faint} %clr([%15.15t]){faint} %clr(%-40.40logger{39}){cyan} %clr(:){faint} %m%n%wEx"
Postman : getting token from Authorization server
Postman : getting token from Authorization server
Querying secured service and getting erro
AuthorizationServer logs
2018-12-30 21:04:40.599 INFO 5144 --- [nio-8090-exec-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring DispatcherServlet 'dispatcherServlet'
2018-12-30 21:04:40.599 INFO 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : Initializing Servlet 'dispatcherServlet'
2018-12-30 21:04:40.599 DEBUG 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : Detected StandardServletMultipartResolver
2018-12-30 21:04:40.599 DEBUG 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : enableLoggingRequestDetails='false': request parameters and headers will be masked to prevent unsafe logging of potentially sensitive data
2018-12-30 21:04:40.599 INFO 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : Completed initialization in 0 ms
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/oauth/token']
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Checking match of request : '/oauth/token'; against '/oauth/token'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : matched
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 1 of 11 in additional filter chain; firing Filter: 'WebAsyncManagerIntegrationFilter'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 2 of 11 in additional filter chain; firing Filter: 'SecurityContextPersistenceFilter'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 3 of 11 in additional filter chain; firing Filter: 'HeaderWriterFilter'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 4 of 11 in additional filter chain; firing Filter: 'LogoutFilter'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', GET]
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'POST /oauth/token' doesn't match 'GET /logout'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', POST]
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Checking match of request : '/oauth/token'; against '/logout'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', PUT]
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'POST /oauth/token' doesn't match 'PUT /logout'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', DELETE]
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'POST /oauth/token' doesn't match 'DELETE /logout'
2018-12-30 21:04:40.600 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : No matches found
2018-12-30 21:04:40.615 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 5 of 11 in additional filter chain; firing Filter: 'BasicAuthenticationFilter'
2018-12-30 21:04:40.615 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.www.BasicAuthenticationFilter : Basic Authentication Authorization header found for user 'clientABC'
2018-12-30 21:04:40.631 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.authentication.ProviderManager : Authentication attempt using org.springframework.security.authentication.dao.DaoAuthenticationProvider
2018-12-30 21:04:40.742 DEBUG 5144 --- [nio-8090-exec-1] o.s.b.f.s.DefaultListableBeanFactory : Creating shared instance of singleton bean 'scopedTarget.clientDetailsService'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.b.a.audit.listener.AuditListener : AuditEvent [timestamp=2018-12-30T20:04:40.758Z, principal=clientABC, type=AUTHENTICATION_SUCCESS, data={details=org.springframework.security.web.authentication.WebAuthenticationDetails@b364: RemoteIpAddress: 0:0:0:0:0:0:0:1; SessionId: null}]
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.www.BasicAuthenticationFilter : Authentication success: org.springframework.security.authentication.UsernamePasswordAuthenticationToken@717e3c37: Principal: org.springframework.security.core.userdetails.User@8e817097: Username: clientABC; Password: [PROTECTED]; Enabled: true; AccountNonExpired: true; credentialsNonExpired: true; AccountNonLocked: true; Not granted any authorities; Credentials: [PROTECTED]; Authenticated: true; Details: org.springframework.security.web.authentication.WebAuthenticationDetails@b364: RemoteIpAddress: 0:0:0:0:0:0:0:1; SessionId: null; Not granted any authorities
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 6 of 11 in additional filter chain; firing Filter: 'RequestCacheAwareFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.s.HttpSessionRequestCache : saved request doesn't match
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 7 of 11 in additional filter chain; firing Filter: 'SecurityContextHolderAwareRequestFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 8 of 11 in additional filter chain; firing Filter: 'AnonymousAuthenticationFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.AnonymousAuthenticationFilter : SecurityContextHolder not populated with anonymous token, as it already contained: 'org.springframework.security.authentication.UsernamePasswordAuthenticationToken@717e3c37: Principal: org.springframework.security.core.userdetails.User@8e817097: Username: clientABC; Password: [PROTECTED]; Enabled: true; AccountNonExpired: true; credentialsNonExpired: true; AccountNonLocked: true; Not granted any authorities; Credentials: [PROTECTED]; Authenticated: true; Details: org.springframework.security.web.authentication.WebAuthenticationDetails@b364: RemoteIpAddress: 0:0:0:0:0:0:0:1; SessionId: null; Not granted any authorities'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 9 of 11 in additional filter chain; firing Filter: 'SessionManagementFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] s.CompositeSessionAuthenticationStrategy : Delegating to org.springframework.security.web.authentication.session.ChangeSessionIdAuthenticationStrategy@5a1c3cb4
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 10 of 11 in additional filter chain; firing Filter: 'ExceptionTranslationFilter'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token at position 11 of 11 in additional filter chain; firing Filter: 'FilterSecurityInterceptor'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Checking match of request : '/oauth/token'; against '/oauth/token'
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.i.FilterSecurityInterceptor : Secure object: FilterInvocation: URL: /oauth/token; Attributes: [fullyAuthenticated]
2018-12-30 21:04:40.758 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.i.FilterSecurityInterceptor : Previously Authenticated: org.springframework.security.authentication.UsernamePasswordAuthenticationToken@717e3c37: Principal: org.springframework.security.core.userdetails.User@8e817097: Username: clientABC; Password: [PROTECTED]; Enabled: true; AccountNonExpired: true; credentialsNonExpired: true; AccountNonLocked: true; Not granted any authorities; Credentials: [PROTECTED]; Authenticated: true; Details: org.springframework.security.web.authentication.WebAuthenticationDetails@b364: RemoteIpAddress: 0:0:0:0:0:0:0:1; SessionId: null; Not granted any authorities
2018-12-30 21:04:40.773 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.access.vote.AffirmativeBased : Voter: org.springframework.security.web.access.expression.WebExpressionVoter@76d8c502, returned: 1
2018-12-30 21:04:40.773 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.i.FilterSecurityInterceptor : Authorization successful
2018-12-30 21:04:40.773 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.i.FilterSecurityInterceptor : RunAsManager did not change Authentication object
2018-12-30 21:04:40.773 DEBUG 5144 --- [nio-8090-exec-1] o.s.security.web.FilterChainProxy : /oauth/token reached end of additional filter chain; proceeding with original chain
2018-12-30 21:04:40.789 DEBUG 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : POST "/oauth/token", parameters={masked}
2018-12-30 21:04:40.790 DEBUG 5144 --- [nio-8090-exec-1] .s.o.p.e.FrameworkEndpointHandlerMapping : Mapped to public org.springframework.http.ResponseEntity<org.springframework.security.oauth2.common.OAuth2AccessToken> org.springframework.security.oauth2.provider.endpoint.TokenEndpoint.postAccessToken(java.security.Principal,java.util.Map<java.lang.String, java.lang.String>) throws org.springframework.web.HttpRequestMethodNotSupportedException
2018-12-30 21:04:40.842 DEBUG 5144 --- [nio-8090-exec-1] .s.s.o.p.c.ClientCredentialsTokenGranter : Getting access token for: clientABC
2018-12-30 21:04:40.858 DEBUG 5144 --- [nio-8090-exec-1] o.s.w.s.m.m.a.HttpEntityMethodProcessor : Found 'Content-Type:application/json;charset=UTF-8' in response
2018-12-30 21:04:40.905 DEBUG 5144 --- [nio-8090-exec-1] o.s.w.s.m.m.a.HttpEntityMethodProcessor : Writing [087b1986-b76e-4ff5-8e84-63ecd62e9583]
2018-12-30 21:04:40.920 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.header.writers.HstsHeaderWriter : Not injecting HSTS header since it did not match the requestMatcher org.springframework.security.web.header.writers.HstsHeaderWriter$SecureRequestMatcher@38dba2a
2018-12-30 21:04:40.921 DEBUG 5144 --- [nio-8090-exec-1] o.s.web.servlet.DispatcherServlet : Completed 200 OK
2018-12-30 21:04:40.921 DEBUG 5144 --- [nio-8090-exec-1] o.s.s.w.a.ExceptionTranslationFilter : Chain processed normally
2018-12-30 21:04:40.921 DEBUG 5144 --- [nio-8090-exec-1] s.s.w.c.SecurityContextPersistenceFilter : SecurityContextHolder now cleared, as request processing completed
Resource Server logs
2018-12-30 21:04:54.158 INFO 8512 --- [nio-8092-exec-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring DispatcherServlet 'dispatcherServlet'
2018-12-30 21:04:54.158 INFO 8512 --- [nio-8092-exec-1] o.s.web.servlet.DispatcherServlet : Initializing Servlet 'dispatcherServlet'
2018-12-30 21:04:54.158 DEBUG 8512 --- [nio-8092-exec-1] o.s.web.servlet.DispatcherServlet : Detected StandardServletMultipartResolver
2018-12-30 21:04:54.159 DEBUG 8512 --- [nio-8092-exec-1] o.s.web.servlet.DispatcherServlet : enableLoggingRequestDetails='false': request parameters and headers will be masked to prevent unsafe logging of potentially sensitive data
2018-12-30 21:04:54.159 INFO 8512 --- [nio-8092-exec-1] o.s.web.servlet.DispatcherServlet : Completed initialization in 1 ms
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 1 of 11 in additional filter chain; firing Filter: 'WebAsyncManagerIntegrationFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 2 of 11 in additional filter chain; firing Filter: 'SecurityContextPersistenceFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 3 of 11 in additional filter chain; firing Filter: 'HeaderWriterFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 4 of 11 in additional filter chain; firing Filter: 'LogoutFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', GET]
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Checking match of request : '/secured'; against '/logout'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', POST]
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'GET /secured' doesn't match 'POST /logout'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', PUT]
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'GET /secured' doesn't match 'PUT /logout'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : Trying to match using Ant [pattern='/logout', DELETE]
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.u.matcher.AntPathRequestMatcher : Request 'GET /secured' doesn't match 'DELETE /logout'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.web.util.matcher.OrRequestMatcher : No matches found
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.security.web.FilterChainProxy : /secured?access_token=087b1986-b76e-4ff5-8e84-63ecd62e9583 at position 5 of 11 in additional filter chain; firing Filter: 'OAuth2AuthenticationProcessingFilter'
2018-12-30 21:04:54.190 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.o.p.a.BearerTokenExtractor : Token not found in headers. Trying request parameters.
2018-12-30 21:04:54.206 DEBUG 8512 --- [nio-8092-exec-1] p.a.OAuth2AuthenticationProcessingFilter : Authentication request failed: error="invalid_token", error_description="Invalid access token: 087b1986-b76e-4ff5-8e84-63ecd62e9583"
2018-12-30 21:04:54.206 DEBUG 8512 --- [nio-8092-exec-1] o.s.b.a.audit.listener.AuditListener : AuditEvent [timestamp=2018-12-30T20:04:54.206Z, principal=access-token, type=AUTHENTICATION_FAILURE, data={type=org.springframework.security.authentication.BadCredentialsException, message=Invalid access token: 087b1986-b76e-4ff5-8e84-63ecd62e9583}]
2018-12-30 21:04:54.300 DEBUG 8512 --- [nio-8092-exec-1] o.s.s.w.header.writers.HstsHeaderWriter : Not injecting HSTS header since it did not match the requestMatcher org.springframework.security.web.header.writers.HstsHeaderWriter$SecureRequestMatcher@582fa201
2018-12-30 21:04:54.300 DEBUG 8512 --- [nio-8092-exec-1] s.s.o.p.e.DefaultOAuth2ExceptionRenderer : Written [error="invalid_token", error_description="Invalid access token: 087b1986-b76e-4ff5-8e84-63ecd62e9583"] as "application/json;charset=UTF-8" using [org.springframework.http.converter.json.MappingJackson2HttpMessageConverter@29184e62]
2018-12-30 21:04:54.300 DEBUG 8512 --- [nio-8092-exec-1] s.s.w.c.SecurityContextPersistenceFilter : SecurityContextHolder now cleared, as request processing completed
complete code is available in gihub
https://github.com/harsh-hardaha/springboot2Oauth2
Please ignore Admin server and swagger configuration code in github
spring spring-boot spring-security oauth-2.0 spring-security-oauth2
spring spring-boot spring-security oauth-2.0 spring-security-oauth2
edited Dec 30 '18 at 20:21
Harsh Hardaha
asked Dec 30 '18 at 20:13


Harsh HardahaHarsh Hardaha
63
63
Do you use the same token repository for authorization server and resource server? You didn't configure atoken-info-uri
.
– dur
Dec 30 '18 at 20:25
1
I have debugged code on Resource server side, and found that method public Authentication authenticate(Authentication authentication) in org.springframework.security.oauth2.provider.authentication.OAuth2AuthenticationManager uses Defaulttokenservice which uses inmemorytokenstore in resource server side which is empty.
– Harsh Hardaha
Dec 30 '18 at 20:36
@dur No I do not use same token repository, both server are independent. can you please let me know what will be implementation of token-info-uri endpoint in authorization server side?
– Harsh Hardaha
Dec 30 '18 at 20:38
add a comment |
Do you use the same token repository for authorization server and resource server? You didn't configure atoken-info-uri
.
– dur
Dec 30 '18 at 20:25
1
I have debugged code on Resource server side, and found that method public Authentication authenticate(Authentication authentication) in org.springframework.security.oauth2.provider.authentication.OAuth2AuthenticationManager uses Defaulttokenservice which uses inmemorytokenstore in resource server side which is empty.
– Harsh Hardaha
Dec 30 '18 at 20:36
@dur No I do not use same token repository, both server are independent. can you please let me know what will be implementation of token-info-uri endpoint in authorization server side?
– Harsh Hardaha
Dec 30 '18 at 20:38
Do you use the same token repository for authorization server and resource server? You didn't configure a
token-info-uri
.– dur
Dec 30 '18 at 20:25
Do you use the same token repository for authorization server and resource server? You didn't configure a
token-info-uri
.– dur
Dec 30 '18 at 20:25
1
1
I have debugged code on Resource server side, and found that method public Authentication authenticate(Authentication authentication) in org.springframework.security.oauth2.provider.authentication.OAuth2AuthenticationManager uses Defaulttokenservice which uses inmemorytokenstore in resource server side which is empty.
– Harsh Hardaha
Dec 30 '18 at 20:36
I have debugged code on Resource server side, and found that method public Authentication authenticate(Authentication authentication) in org.springframework.security.oauth2.provider.authentication.OAuth2AuthenticationManager uses Defaulttokenservice which uses inmemorytokenstore in resource server side which is empty.
– Harsh Hardaha
Dec 30 '18 at 20:36
@dur No I do not use same token repository, both server are independent. can you please let me know what will be implementation of token-info-uri endpoint in authorization server side?
– Harsh Hardaha
Dec 30 '18 at 20:38
@dur No I do not use same token repository, both server are independent. can you please let me know what will be implementation of token-info-uri endpoint in authorization server side?
– Harsh Hardaha
Dec 30 '18 at 20:38
add a comment |
1 Answer
1
active
oldest
votes
After Some more inspection and debug I found Solution, I have to make below changes in resource server configuration
Application.yml
security:
oauth2:
client:
clientId: clientABC
clientSecret: secretXYZ
resource:
user-info-uri: http://localhost:8090/user
token-info-uri: http://localhost:8090/oauth/check_token
preferTokenInfo: true
filter-order: 3
basic:
enabled: false
included dependency in resource server
<dependency>
<groupId>org.springframework.security.oauth.boot</groupId>
<artifactId>spring-security-oauth2-autoconfigure</artifactId>
<version>2.1.1.RELEASE</version>
</dependency>
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53981076%2fspring-boot-2-oauth2-resource-server-does-not-hit-authorization-server-for-acces%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
After Some more inspection and debug I found Solution, I have to make below changes in resource server configuration
Application.yml
security:
oauth2:
client:
clientId: clientABC
clientSecret: secretXYZ
resource:
user-info-uri: http://localhost:8090/user
token-info-uri: http://localhost:8090/oauth/check_token
preferTokenInfo: true
filter-order: 3
basic:
enabled: false
included dependency in resource server
<dependency>
<groupId>org.springframework.security.oauth.boot</groupId>
<artifactId>spring-security-oauth2-autoconfigure</artifactId>
<version>2.1.1.RELEASE</version>
</dependency>
add a comment |
After Some more inspection and debug I found Solution, I have to make below changes in resource server configuration
Application.yml
security:
oauth2:
client:
clientId: clientABC
clientSecret: secretXYZ
resource:
user-info-uri: http://localhost:8090/user
token-info-uri: http://localhost:8090/oauth/check_token
preferTokenInfo: true
filter-order: 3
basic:
enabled: false
included dependency in resource server
<dependency>
<groupId>org.springframework.security.oauth.boot</groupId>
<artifactId>spring-security-oauth2-autoconfigure</artifactId>
<version>2.1.1.RELEASE</version>
</dependency>
add a comment |
After Some more inspection and debug I found Solution, I have to make below changes in resource server configuration
Application.yml
security:
oauth2:
client:
clientId: clientABC
clientSecret: secretXYZ
resource:
user-info-uri: http://localhost:8090/user
token-info-uri: http://localhost:8090/oauth/check_token
preferTokenInfo: true
filter-order: 3
basic:
enabled: false
included dependency in resource server
<dependency>
<groupId>org.springframework.security.oauth.boot</groupId>
<artifactId>spring-security-oauth2-autoconfigure</artifactId>
<version>2.1.1.RELEASE</version>
</dependency>
After Some more inspection and debug I found Solution, I have to make below changes in resource server configuration
Application.yml
security:
oauth2:
client:
clientId: clientABC
clientSecret: secretXYZ
resource:
user-info-uri: http://localhost:8090/user
token-info-uri: http://localhost:8090/oauth/check_token
preferTokenInfo: true
filter-order: 3
basic:
enabled: false
included dependency in resource server
<dependency>
<groupId>org.springframework.security.oauth.boot</groupId>
<artifactId>spring-security-oauth2-autoconfigure</artifactId>
<version>2.1.1.RELEASE</version>
</dependency>
answered Dec 30 '18 at 23:10


Harsh HardahaHarsh Hardaha
63
63
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53981076%2fspring-boot-2-oauth2-resource-server-does-not-hit-authorization-server-for-acces%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7ECEcVGk SfyPayw
Do you use the same token repository for authorization server and resource server? You didn't configure a
token-info-uri
.– dur
Dec 30 '18 at 20:25
1
I have debugged code on Resource server side, and found that method public Authentication authenticate(Authentication authentication) in org.springframework.security.oauth2.provider.authentication.OAuth2AuthenticationManager uses Defaulttokenservice which uses inmemorytokenstore in resource server side which is empty.
– Harsh Hardaha
Dec 30 '18 at 20:36
@dur No I do not use same token repository, both server are independent. can you please let me know what will be implementation of token-info-uri endpoint in authorization server side?
– Harsh Hardaha
Dec 30 '18 at 20:38