React Native error when passing size of ActivityIndicator as a prop
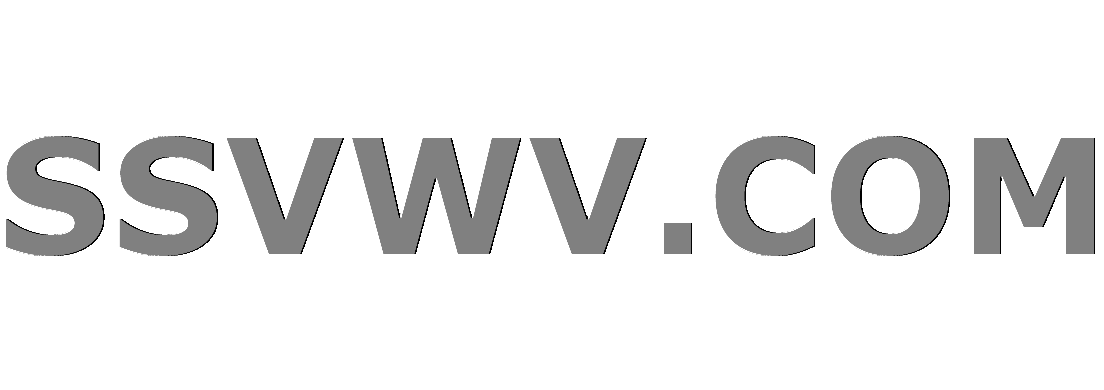
Multi tool use
I was creating a reusable spinner component for my project I had created 2 components: loginform and spinner, where i passed an enum as a prop from loginform to spinner when I reloaded the app it crashed on android but thankfully it displayed an error message on iOS saying "Error while updating property 'width' in shadow node of type: AndroidProgressBar".
I've tried removing the reference to the prop and instead specified the enum values i.e., 'small' or 'large' to the spinner component. It worked but that completely destroyed the whole point of creating a reusable component.
import React, { Component } from 'react';
import { View, Text } from 'react-native';
import firebase from 'firebase';
import { Button, Card, CardSection, Input, Spinner } from './common/';
class LoginForm extends Component {
state = { email: '', password: '', error: '', loading: false };
onButtonPress() {
const { email, password } = this.state;
this.setState({ error: '', loading: true });
firebase.auth().signInWithEmailAndPassword(email, password)
.catch(() => {
firebase.auth().createUserWithEmailAndPassword(email, password)
.catch(() => {
this.setState({ error: 'Authentication Failed!' });
});
});
}
renderButton() {
if (this.state.loading) {
return <Spinner size="small" />;
}
return <Button onPress={this.onButtonPress.bind(this)}>Log In</Button>;
}
render() {
return (
<View style={{ marginTop: 50 }}>
<Card>
<CardSection>
<Input
autoCorrect={false}
label='Email :'
placeholder='user@example.com'
value={this.state.email}
onChangeText={email => this.setState({ email })}
/>
</CardSection>
<CardSection>
<Input
secureTextEntry
placeholder='password'
label='Password :'
value={this.state.password}
onChangeText={password => this.setState({ password })}
/>
</CardSection>
<Text style={styles.errorTextStyle}>{this.state.error}</Text>
<CardSection style={{ flexDirection: 'column' }}>
{this.renderButton()}
</CardSection>
</Card>
</View>
);
}
}
const styles = {
errorTextStyle: {
fontSize: 20,
color: 'red',
alignSelf: 'center',
borderColor: 'white',
}
};
export default LoginForm;
The other component's code:
import React from 'react';
import { ActivityIndicator, View } from 'react-native';
// eslint-disable-next-line arrow-body-style
const Spinner = (size) => {
return (
<View style={styles.spinnerStyle}>
<ActivityIndicator size={size || 'large'} />
</View>
);
};
const styles = {
spinnerStyle: {
flex: 1,
justifyContent: 'center',
alignItems: 'center'
}
};
export { Spinner };
I ran the above code and on the expo app on android, it crashed and on ios it didn't crash but displayed the above mentioned error on the red screen.
javascript react-native
add a comment |
I was creating a reusable spinner component for my project I had created 2 components: loginform and spinner, where i passed an enum as a prop from loginform to spinner when I reloaded the app it crashed on android but thankfully it displayed an error message on iOS saying "Error while updating property 'width' in shadow node of type: AndroidProgressBar".
I've tried removing the reference to the prop and instead specified the enum values i.e., 'small' or 'large' to the spinner component. It worked but that completely destroyed the whole point of creating a reusable component.
import React, { Component } from 'react';
import { View, Text } from 'react-native';
import firebase from 'firebase';
import { Button, Card, CardSection, Input, Spinner } from './common/';
class LoginForm extends Component {
state = { email: '', password: '', error: '', loading: false };
onButtonPress() {
const { email, password } = this.state;
this.setState({ error: '', loading: true });
firebase.auth().signInWithEmailAndPassword(email, password)
.catch(() => {
firebase.auth().createUserWithEmailAndPassword(email, password)
.catch(() => {
this.setState({ error: 'Authentication Failed!' });
});
});
}
renderButton() {
if (this.state.loading) {
return <Spinner size="small" />;
}
return <Button onPress={this.onButtonPress.bind(this)}>Log In</Button>;
}
render() {
return (
<View style={{ marginTop: 50 }}>
<Card>
<CardSection>
<Input
autoCorrect={false}
label='Email :'
placeholder='user@example.com'
value={this.state.email}
onChangeText={email => this.setState({ email })}
/>
</CardSection>
<CardSection>
<Input
secureTextEntry
placeholder='password'
label='Password :'
value={this.state.password}
onChangeText={password => this.setState({ password })}
/>
</CardSection>
<Text style={styles.errorTextStyle}>{this.state.error}</Text>
<CardSection style={{ flexDirection: 'column' }}>
{this.renderButton()}
</CardSection>
</Card>
</View>
);
}
}
const styles = {
errorTextStyle: {
fontSize: 20,
color: 'red',
alignSelf: 'center',
borderColor: 'white',
}
};
export default LoginForm;
The other component's code:
import React from 'react';
import { ActivityIndicator, View } from 'react-native';
// eslint-disable-next-line arrow-body-style
const Spinner = (size) => {
return (
<View style={styles.spinnerStyle}>
<ActivityIndicator size={size || 'large'} />
</View>
);
};
const styles = {
spinnerStyle: {
flex: 1,
justifyContent: 'center',
alignItems: 'center'
}
};
export { Spinner };
I ran the above code and on the expo app on android, it crashed and on ios it didn't crash but displayed the above mentioned error on the red screen.
javascript react-native
add a comment |
I was creating a reusable spinner component for my project I had created 2 components: loginform and spinner, where i passed an enum as a prop from loginform to spinner when I reloaded the app it crashed on android but thankfully it displayed an error message on iOS saying "Error while updating property 'width' in shadow node of type: AndroidProgressBar".
I've tried removing the reference to the prop and instead specified the enum values i.e., 'small' or 'large' to the spinner component. It worked but that completely destroyed the whole point of creating a reusable component.
import React, { Component } from 'react';
import { View, Text } from 'react-native';
import firebase from 'firebase';
import { Button, Card, CardSection, Input, Spinner } from './common/';
class LoginForm extends Component {
state = { email: '', password: '', error: '', loading: false };
onButtonPress() {
const { email, password } = this.state;
this.setState({ error: '', loading: true });
firebase.auth().signInWithEmailAndPassword(email, password)
.catch(() => {
firebase.auth().createUserWithEmailAndPassword(email, password)
.catch(() => {
this.setState({ error: 'Authentication Failed!' });
});
});
}
renderButton() {
if (this.state.loading) {
return <Spinner size="small" />;
}
return <Button onPress={this.onButtonPress.bind(this)}>Log In</Button>;
}
render() {
return (
<View style={{ marginTop: 50 }}>
<Card>
<CardSection>
<Input
autoCorrect={false}
label='Email :'
placeholder='user@example.com'
value={this.state.email}
onChangeText={email => this.setState({ email })}
/>
</CardSection>
<CardSection>
<Input
secureTextEntry
placeholder='password'
label='Password :'
value={this.state.password}
onChangeText={password => this.setState({ password })}
/>
</CardSection>
<Text style={styles.errorTextStyle}>{this.state.error}</Text>
<CardSection style={{ flexDirection: 'column' }}>
{this.renderButton()}
</CardSection>
</Card>
</View>
);
}
}
const styles = {
errorTextStyle: {
fontSize: 20,
color: 'red',
alignSelf: 'center',
borderColor: 'white',
}
};
export default LoginForm;
The other component's code:
import React from 'react';
import { ActivityIndicator, View } from 'react-native';
// eslint-disable-next-line arrow-body-style
const Spinner = (size) => {
return (
<View style={styles.spinnerStyle}>
<ActivityIndicator size={size || 'large'} />
</View>
);
};
const styles = {
spinnerStyle: {
flex: 1,
justifyContent: 'center',
alignItems: 'center'
}
};
export { Spinner };
I ran the above code and on the expo app on android, it crashed and on ios it didn't crash but displayed the above mentioned error on the red screen.
javascript react-native
I was creating a reusable spinner component for my project I had created 2 components: loginform and spinner, where i passed an enum as a prop from loginform to spinner when I reloaded the app it crashed on android but thankfully it displayed an error message on iOS saying "Error while updating property 'width' in shadow node of type: AndroidProgressBar".
I've tried removing the reference to the prop and instead specified the enum values i.e., 'small' or 'large' to the spinner component. It worked but that completely destroyed the whole point of creating a reusable component.
import React, { Component } from 'react';
import { View, Text } from 'react-native';
import firebase from 'firebase';
import { Button, Card, CardSection, Input, Spinner } from './common/';
class LoginForm extends Component {
state = { email: '', password: '', error: '', loading: false };
onButtonPress() {
const { email, password } = this.state;
this.setState({ error: '', loading: true });
firebase.auth().signInWithEmailAndPassword(email, password)
.catch(() => {
firebase.auth().createUserWithEmailAndPassword(email, password)
.catch(() => {
this.setState({ error: 'Authentication Failed!' });
});
});
}
renderButton() {
if (this.state.loading) {
return <Spinner size="small" />;
}
return <Button onPress={this.onButtonPress.bind(this)}>Log In</Button>;
}
render() {
return (
<View style={{ marginTop: 50 }}>
<Card>
<CardSection>
<Input
autoCorrect={false}
label='Email :'
placeholder='user@example.com'
value={this.state.email}
onChangeText={email => this.setState({ email })}
/>
</CardSection>
<CardSection>
<Input
secureTextEntry
placeholder='password'
label='Password :'
value={this.state.password}
onChangeText={password => this.setState({ password })}
/>
</CardSection>
<Text style={styles.errorTextStyle}>{this.state.error}</Text>
<CardSection style={{ flexDirection: 'column' }}>
{this.renderButton()}
</CardSection>
</Card>
</View>
);
}
}
const styles = {
errorTextStyle: {
fontSize: 20,
color: 'red',
alignSelf: 'center',
borderColor: 'white',
}
};
export default LoginForm;
The other component's code:
import React from 'react';
import { ActivityIndicator, View } from 'react-native';
// eslint-disable-next-line arrow-body-style
const Spinner = (size) => {
return (
<View style={styles.spinnerStyle}>
<ActivityIndicator size={size || 'large'} />
</View>
);
};
const styles = {
spinnerStyle: {
flex: 1,
justifyContent: 'center',
alignItems: 'center'
}
};
export { Spinner };
I ran the above code and on the expo app on android, it crashed and on ios it didn't crash but displayed the above mentioned error on the red screen.
javascript react-native
javascript react-native
edited Dec 30 '18 at 20:14
Priyanshu Bharti
asked Dec 30 '18 at 20:02


Priyanshu BhartiPriyanshu Bharti
62
62
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Did you used "this.props.prop" to access the props, if so, an stateless component doesn't have the "this.props" property, instead you should pass the props like this
In your login screen use this normally
if (this.state.loading) {
return <Spinner prop="small" />;
}
In your component use this
const Spinner = (prop) => {
return (
<View style={styles.spinnerStyle}>
<ActivityIndicator size=prop />
</View>
);
};
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53981007%2freact-native-error-when-passing-size-of-activityindicator-as-a-prop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Did you used "this.props.prop" to access the props, if so, an stateless component doesn't have the "this.props" property, instead you should pass the props like this
In your login screen use this normally
if (this.state.loading) {
return <Spinner prop="small" />;
}
In your component use this
const Spinner = (prop) => {
return (
<View style={styles.spinnerStyle}>
<ActivityIndicator size=prop />
</View>
);
};
add a comment |
Did you used "this.props.prop" to access the props, if so, an stateless component doesn't have the "this.props" property, instead you should pass the props like this
In your login screen use this normally
if (this.state.loading) {
return <Spinner prop="small" />;
}
In your component use this
const Spinner = (prop) => {
return (
<View style={styles.spinnerStyle}>
<ActivityIndicator size=prop />
</View>
);
};
add a comment |
Did you used "this.props.prop" to access the props, if so, an stateless component doesn't have the "this.props" property, instead you should pass the props like this
In your login screen use this normally
if (this.state.loading) {
return <Spinner prop="small" />;
}
In your component use this
const Spinner = (prop) => {
return (
<View style={styles.spinnerStyle}>
<ActivityIndicator size=prop />
</View>
);
};
Did you used "this.props.prop" to access the props, if so, an stateless component doesn't have the "this.props" property, instead you should pass the props like this
In your login screen use this normally
if (this.state.loading) {
return <Spinner prop="small" />;
}
In your component use this
const Spinner = (prop) => {
return (
<View style={styles.spinnerStyle}>
<ActivityIndicator size=prop />
</View>
);
};
answered Dec 30 '18 at 20:18


ValdaXDValdaXD
4169
4169
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53981007%2freact-native-error-when-passing-size-of-activityindicator-as-a-prop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
K0bqR8fycAw