How to create “RoleManager” in ASP.Net Core?
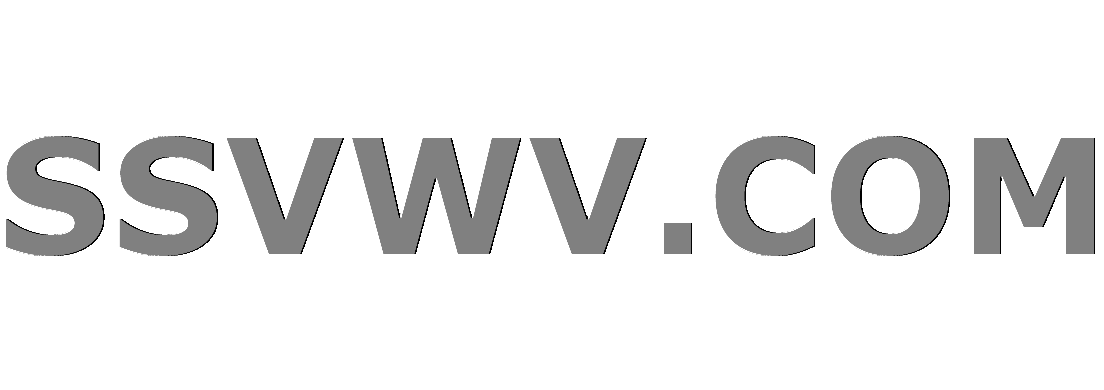
Multi tool use
I am unable to use RoleManager in my application. I am trying to seed an Admin user in to a .NET Core web application, and I want this user to have a role called "canEdit". What I've got currently produces this error:
System.InvalidOperationException: 'No service for type 'Microsoft.AspNetCore.Identity.RoleManager`1[Microsoft.AspNetCore.Identity.IdentityRole]' has been registered.'
I have tried various ways of doing this. I have tried using Service Provider to do it, dependency injection, etc. but they all give the same error.
ServiceProvider in the Configure method of Startup.cs:
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseCookiePolicy();
app.UseAuthentication();
var scopeFactory = app.ApplicationServices.GetRequiredService<IServiceScopeFactory>();
var scope = scopeFactory.CreateScope();
var roleManager = scope.ServiceProvider.GetRequiredService<RoleManager<IdentityRole>>();
DbInitializer dbi = new DbInitializer(roleManager);
dbi.Initialize(context, userManager);
DBInitializer Class:
Constructor:
private readonly RoleManager<IdentityRole> rm;
public DbInitializer(RoleManager<IdentityRole> rm)
{
this.rm = rm;
}
CreateAdmin method:
private async Task CreateAdmin(UserManager<ApplicationUser> userManager, ApplicationDbContext context)
{
IdentityResult ir;
ir = await rm.CreateAsync(new IdentityRole("canEdit"));
IdentityRole canEdit = new IdentityRole("canEdit");
ApplicationUser admin = new ApplicationUser
{
UserName = "Member1@email.com"
};
if (context.Users.Where(u => u.UserName == admin.UserName).Count() == 0)
{
userManager.CreateAsync(admin, "Password123!").Wait();
userManager.AddToRoleAsync(admin, "canEdit").Wait();
}
}
CreateUsers method:
private void CreateUsers(UserManager<ApplicationUser> userManager, ApplicationDbContext context)
{
CreateAdmin(userManager, context).Wait();
ApplicationUser customer1 = new ApplicationUser
{
UserName = "Customer1@email.com"
};
if (context.Users.Where(u => u.UserName == customer1.UserName).Count() > 0)
{
userManager.CreateAsync(customer1, "Password123!").Wait();
}
This repeats for customer2, 3, 4, 5. I can optimise this by removing the need to create an object which I may not need (in the case where the email exists), but I was trying to knock up this method and the CreateAdmin method quickly, then optimise later. Unfortunately I then ran in to an error which I have been unable to fix.
The end goal is for the DbInitializer class to seed 5 regular users, and 1 admin user with extra permissions/claims.
c# asp.net-mvc
add a comment |
I am unable to use RoleManager in my application. I am trying to seed an Admin user in to a .NET Core web application, and I want this user to have a role called "canEdit". What I've got currently produces this error:
System.InvalidOperationException: 'No service for type 'Microsoft.AspNetCore.Identity.RoleManager`1[Microsoft.AspNetCore.Identity.IdentityRole]' has been registered.'
I have tried various ways of doing this. I have tried using Service Provider to do it, dependency injection, etc. but they all give the same error.
ServiceProvider in the Configure method of Startup.cs:
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseCookiePolicy();
app.UseAuthentication();
var scopeFactory = app.ApplicationServices.GetRequiredService<IServiceScopeFactory>();
var scope = scopeFactory.CreateScope();
var roleManager = scope.ServiceProvider.GetRequiredService<RoleManager<IdentityRole>>();
DbInitializer dbi = new DbInitializer(roleManager);
dbi.Initialize(context, userManager);
DBInitializer Class:
Constructor:
private readonly RoleManager<IdentityRole> rm;
public DbInitializer(RoleManager<IdentityRole> rm)
{
this.rm = rm;
}
CreateAdmin method:
private async Task CreateAdmin(UserManager<ApplicationUser> userManager, ApplicationDbContext context)
{
IdentityResult ir;
ir = await rm.CreateAsync(new IdentityRole("canEdit"));
IdentityRole canEdit = new IdentityRole("canEdit");
ApplicationUser admin = new ApplicationUser
{
UserName = "Member1@email.com"
};
if (context.Users.Where(u => u.UserName == admin.UserName).Count() == 0)
{
userManager.CreateAsync(admin, "Password123!").Wait();
userManager.AddToRoleAsync(admin, "canEdit").Wait();
}
}
CreateUsers method:
private void CreateUsers(UserManager<ApplicationUser> userManager, ApplicationDbContext context)
{
CreateAdmin(userManager, context).Wait();
ApplicationUser customer1 = new ApplicationUser
{
UserName = "Customer1@email.com"
};
if (context.Users.Where(u => u.UserName == customer1.UserName).Count() > 0)
{
userManager.CreateAsync(customer1, "Password123!").Wait();
}
This repeats for customer2, 3, 4, 5. I can optimise this by removing the need to create an object which I may not need (in the case where the email exists), but I was trying to knock up this method and the CreateAdmin method quickly, then optimise later. Unfortunately I then ran in to an error which I have been unable to fix.
The end goal is for the DbInitializer class to seed 5 regular users, and 1 admin user with extra permissions/claims.
c# asp.net-mvc
add a comment |
I am unable to use RoleManager in my application. I am trying to seed an Admin user in to a .NET Core web application, and I want this user to have a role called "canEdit". What I've got currently produces this error:
System.InvalidOperationException: 'No service for type 'Microsoft.AspNetCore.Identity.RoleManager`1[Microsoft.AspNetCore.Identity.IdentityRole]' has been registered.'
I have tried various ways of doing this. I have tried using Service Provider to do it, dependency injection, etc. but they all give the same error.
ServiceProvider in the Configure method of Startup.cs:
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseCookiePolicy();
app.UseAuthentication();
var scopeFactory = app.ApplicationServices.GetRequiredService<IServiceScopeFactory>();
var scope = scopeFactory.CreateScope();
var roleManager = scope.ServiceProvider.GetRequiredService<RoleManager<IdentityRole>>();
DbInitializer dbi = new DbInitializer(roleManager);
dbi.Initialize(context, userManager);
DBInitializer Class:
Constructor:
private readonly RoleManager<IdentityRole> rm;
public DbInitializer(RoleManager<IdentityRole> rm)
{
this.rm = rm;
}
CreateAdmin method:
private async Task CreateAdmin(UserManager<ApplicationUser> userManager, ApplicationDbContext context)
{
IdentityResult ir;
ir = await rm.CreateAsync(new IdentityRole("canEdit"));
IdentityRole canEdit = new IdentityRole("canEdit");
ApplicationUser admin = new ApplicationUser
{
UserName = "Member1@email.com"
};
if (context.Users.Where(u => u.UserName == admin.UserName).Count() == 0)
{
userManager.CreateAsync(admin, "Password123!").Wait();
userManager.AddToRoleAsync(admin, "canEdit").Wait();
}
}
CreateUsers method:
private void CreateUsers(UserManager<ApplicationUser> userManager, ApplicationDbContext context)
{
CreateAdmin(userManager, context).Wait();
ApplicationUser customer1 = new ApplicationUser
{
UserName = "Customer1@email.com"
};
if (context.Users.Where(u => u.UserName == customer1.UserName).Count() > 0)
{
userManager.CreateAsync(customer1, "Password123!").Wait();
}
This repeats for customer2, 3, 4, 5. I can optimise this by removing the need to create an object which I may not need (in the case where the email exists), but I was trying to knock up this method and the CreateAdmin method quickly, then optimise later. Unfortunately I then ran in to an error which I have been unable to fix.
The end goal is for the DbInitializer class to seed 5 regular users, and 1 admin user with extra permissions/claims.
c# asp.net-mvc
I am unable to use RoleManager in my application. I am trying to seed an Admin user in to a .NET Core web application, and I want this user to have a role called "canEdit". What I've got currently produces this error:
System.InvalidOperationException: 'No service for type 'Microsoft.AspNetCore.Identity.RoleManager`1[Microsoft.AspNetCore.Identity.IdentityRole]' has been registered.'
I have tried various ways of doing this. I have tried using Service Provider to do it, dependency injection, etc. but they all give the same error.
ServiceProvider in the Configure method of Startup.cs:
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseCookiePolicy();
app.UseAuthentication();
var scopeFactory = app.ApplicationServices.GetRequiredService<IServiceScopeFactory>();
var scope = scopeFactory.CreateScope();
var roleManager = scope.ServiceProvider.GetRequiredService<RoleManager<IdentityRole>>();
DbInitializer dbi = new DbInitializer(roleManager);
dbi.Initialize(context, userManager);
DBInitializer Class:
Constructor:
private readonly RoleManager<IdentityRole> rm;
public DbInitializer(RoleManager<IdentityRole> rm)
{
this.rm = rm;
}
CreateAdmin method:
private async Task CreateAdmin(UserManager<ApplicationUser> userManager, ApplicationDbContext context)
{
IdentityResult ir;
ir = await rm.CreateAsync(new IdentityRole("canEdit"));
IdentityRole canEdit = new IdentityRole("canEdit");
ApplicationUser admin = new ApplicationUser
{
UserName = "Member1@email.com"
};
if (context.Users.Where(u => u.UserName == admin.UserName).Count() == 0)
{
userManager.CreateAsync(admin, "Password123!").Wait();
userManager.AddToRoleAsync(admin, "canEdit").Wait();
}
}
CreateUsers method:
private void CreateUsers(UserManager<ApplicationUser> userManager, ApplicationDbContext context)
{
CreateAdmin(userManager, context).Wait();
ApplicationUser customer1 = new ApplicationUser
{
UserName = "Customer1@email.com"
};
if (context.Users.Where(u => u.UserName == customer1.UserName).Count() > 0)
{
userManager.CreateAsync(customer1, "Password123!").Wait();
}
This repeats for customer2, 3, 4, 5. I can optimise this by removing the need to create an object which I may not need (in the case where the email exists), but I was trying to knock up this method and the CreateAdmin method quickly, then optimise later. Unfortunately I then ran in to an error which I have been unable to fix.
The end goal is for the DbInitializer class to seed 5 regular users, and 1 admin user with extra permissions/claims.
c# asp.net-mvc
c# asp.net-mvc
asked Dec 30 '18 at 20:32
JedJed
54
54
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Do you have the Identity services registered?
Also, I ran into issues when I used .Wait() instead of await.
services.AddIdentity<ApplicationUser,IdentityRole>(options=>
{
options.User.RequireUniqueEmail = true;
options.Lockout.DefaultLockoutTimeSpan = TimeSpan.FromMinutes(5);
options.Password.RequireNonAlphanumeric = false;
options.Password.RequireUppercase = false;
})
.AddDefaultTokenProviders()
.AddDefaultUI()
.AddRoles<IdentityRole>()
.AddEntityFrameworkStores<ApplicationDbContext>();
services.AddAuthorization();
services.AddAuthentication();
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
If this helps, here is how I seeded my Db.
public SeedDataBase(IServiceProvider _serviceProvider, ApplicationDbContext _context)
{
serviceProvider = _serviceProvider;
context = _context;
}
private IServiceProvider serviceProvider;
private ApplicationDbContext context;
private ApplicationUser superUser;
public async Task Seed()
{
await CreateSuperUser();
await SeedDb();
}
private async Task CreateSuperUser()
{
var _userManager = serviceProvider.GetRequiredService<UserManager<ApplicationUser>>();
var userExists = await _userManager.GetUsersInRoleAsync("FULLADMIN");
if (userExists.Count() < 1)
{
var _roleManager = serviceProvider.GetRequiredService<RoleManager<IdentityRole>>();
var _signinManger = serviceProvider.GetRequiredService<SignInManager<ApplicationUser>>();
superUser = new ApplicationUser()
{
UserName = "superuser@superuser.com",
Email = "superuser@superuser.com",
FirstName = "Super",
LastName = "User",
AccountCreationDate = DateTime.Now.ToShortDateString(),
Access = ApplicationUser.Permissions.FullAdmin
};
var permissions = Enum.GetNames(typeof(ApplicationUser.Permissions));
foreach (var s in permissions)
{
await _roleManager.CreateAsync(new IdentityRole(s));
}
await _userManager.CreateAsync(superUser, "SecureP@ssword1234");
await _userManager.AddToRoleAsync(superUser, Enum.GetName(typeof(ApplicationUser.Permissions), superUser.Access));
}
}
In the ConfigureServices method, I have: services.AddDefaultIdentity<ApplicationUser>() .AddEntityFrameworkStores<ApplicationDbContext>(); I will try editing it to see if that works.
– Jed
Dec 30 '18 at 21:07
Sure thing, I updated to show how I seeded my db. Not the prettiest code (its old), but it may help.
– Rye
Dec 30 '18 at 21:29
services.AddIdentity<ApplicationUser, IdentityRole>() .AddRoles<IdentityRole>() .AddRoleManager<RoleManager<IdentityRole>>() .AddRoleStore<IUserRoleStore<IdentityRole>>() .AddEntityFrameworkStores<ApplicationDbContext>(); I have changed it to this, and it all compiles except for adding the role store.
– Jed
Dec 30 '18 at 21:44
The error I get is: System.ArgumentException: 'Cannot instantiate implementation type 'Microsoft.AspNetCore.Identity.IUserRoleStore1[Microsoft.AspNetCore.Identity.IdentityRole]' for service type 'Microsoft.AspNetCore.Identity.IRoleStore
1[Microsoft.AspNetCore.Identity.IdentityRole]'.' I believe the problem is all the RoleStore classes being interfaces, but I can't work out how to do it with any other class. All the RoleStores are interfaces.
– Jed
Dec 30 '18 at 21:44
Changing it back to AddDefaultIdentity, and simply using ".AddRoles<IdentityRole>()" has seeded the users now. Thanks a lot.
– Jed
Dec 30 '18 at 21:48
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53981203%2fhow-to-create-rolemanageridentityrole-in-asp-net-core%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Do you have the Identity services registered?
Also, I ran into issues when I used .Wait() instead of await.
services.AddIdentity<ApplicationUser,IdentityRole>(options=>
{
options.User.RequireUniqueEmail = true;
options.Lockout.DefaultLockoutTimeSpan = TimeSpan.FromMinutes(5);
options.Password.RequireNonAlphanumeric = false;
options.Password.RequireUppercase = false;
})
.AddDefaultTokenProviders()
.AddDefaultUI()
.AddRoles<IdentityRole>()
.AddEntityFrameworkStores<ApplicationDbContext>();
services.AddAuthorization();
services.AddAuthentication();
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
If this helps, here is how I seeded my Db.
public SeedDataBase(IServiceProvider _serviceProvider, ApplicationDbContext _context)
{
serviceProvider = _serviceProvider;
context = _context;
}
private IServiceProvider serviceProvider;
private ApplicationDbContext context;
private ApplicationUser superUser;
public async Task Seed()
{
await CreateSuperUser();
await SeedDb();
}
private async Task CreateSuperUser()
{
var _userManager = serviceProvider.GetRequiredService<UserManager<ApplicationUser>>();
var userExists = await _userManager.GetUsersInRoleAsync("FULLADMIN");
if (userExists.Count() < 1)
{
var _roleManager = serviceProvider.GetRequiredService<RoleManager<IdentityRole>>();
var _signinManger = serviceProvider.GetRequiredService<SignInManager<ApplicationUser>>();
superUser = new ApplicationUser()
{
UserName = "superuser@superuser.com",
Email = "superuser@superuser.com",
FirstName = "Super",
LastName = "User",
AccountCreationDate = DateTime.Now.ToShortDateString(),
Access = ApplicationUser.Permissions.FullAdmin
};
var permissions = Enum.GetNames(typeof(ApplicationUser.Permissions));
foreach (var s in permissions)
{
await _roleManager.CreateAsync(new IdentityRole(s));
}
await _userManager.CreateAsync(superUser, "SecureP@ssword1234");
await _userManager.AddToRoleAsync(superUser, Enum.GetName(typeof(ApplicationUser.Permissions), superUser.Access));
}
}
In the ConfigureServices method, I have: services.AddDefaultIdentity<ApplicationUser>() .AddEntityFrameworkStores<ApplicationDbContext>(); I will try editing it to see if that works.
– Jed
Dec 30 '18 at 21:07
Sure thing, I updated to show how I seeded my db. Not the prettiest code (its old), but it may help.
– Rye
Dec 30 '18 at 21:29
services.AddIdentity<ApplicationUser, IdentityRole>() .AddRoles<IdentityRole>() .AddRoleManager<RoleManager<IdentityRole>>() .AddRoleStore<IUserRoleStore<IdentityRole>>() .AddEntityFrameworkStores<ApplicationDbContext>(); I have changed it to this, and it all compiles except for adding the role store.
– Jed
Dec 30 '18 at 21:44
The error I get is: System.ArgumentException: 'Cannot instantiate implementation type 'Microsoft.AspNetCore.Identity.IUserRoleStore1[Microsoft.AspNetCore.Identity.IdentityRole]' for service type 'Microsoft.AspNetCore.Identity.IRoleStore
1[Microsoft.AspNetCore.Identity.IdentityRole]'.' I believe the problem is all the RoleStore classes being interfaces, but I can't work out how to do it with any other class. All the RoleStores are interfaces.
– Jed
Dec 30 '18 at 21:44
Changing it back to AddDefaultIdentity, and simply using ".AddRoles<IdentityRole>()" has seeded the users now. Thanks a lot.
– Jed
Dec 30 '18 at 21:48
add a comment |
Do you have the Identity services registered?
Also, I ran into issues when I used .Wait() instead of await.
services.AddIdentity<ApplicationUser,IdentityRole>(options=>
{
options.User.RequireUniqueEmail = true;
options.Lockout.DefaultLockoutTimeSpan = TimeSpan.FromMinutes(5);
options.Password.RequireNonAlphanumeric = false;
options.Password.RequireUppercase = false;
})
.AddDefaultTokenProviders()
.AddDefaultUI()
.AddRoles<IdentityRole>()
.AddEntityFrameworkStores<ApplicationDbContext>();
services.AddAuthorization();
services.AddAuthentication();
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
If this helps, here is how I seeded my Db.
public SeedDataBase(IServiceProvider _serviceProvider, ApplicationDbContext _context)
{
serviceProvider = _serviceProvider;
context = _context;
}
private IServiceProvider serviceProvider;
private ApplicationDbContext context;
private ApplicationUser superUser;
public async Task Seed()
{
await CreateSuperUser();
await SeedDb();
}
private async Task CreateSuperUser()
{
var _userManager = serviceProvider.GetRequiredService<UserManager<ApplicationUser>>();
var userExists = await _userManager.GetUsersInRoleAsync("FULLADMIN");
if (userExists.Count() < 1)
{
var _roleManager = serviceProvider.GetRequiredService<RoleManager<IdentityRole>>();
var _signinManger = serviceProvider.GetRequiredService<SignInManager<ApplicationUser>>();
superUser = new ApplicationUser()
{
UserName = "superuser@superuser.com",
Email = "superuser@superuser.com",
FirstName = "Super",
LastName = "User",
AccountCreationDate = DateTime.Now.ToShortDateString(),
Access = ApplicationUser.Permissions.FullAdmin
};
var permissions = Enum.GetNames(typeof(ApplicationUser.Permissions));
foreach (var s in permissions)
{
await _roleManager.CreateAsync(new IdentityRole(s));
}
await _userManager.CreateAsync(superUser, "SecureP@ssword1234");
await _userManager.AddToRoleAsync(superUser, Enum.GetName(typeof(ApplicationUser.Permissions), superUser.Access));
}
}
In the ConfigureServices method, I have: services.AddDefaultIdentity<ApplicationUser>() .AddEntityFrameworkStores<ApplicationDbContext>(); I will try editing it to see if that works.
– Jed
Dec 30 '18 at 21:07
Sure thing, I updated to show how I seeded my db. Not the prettiest code (its old), but it may help.
– Rye
Dec 30 '18 at 21:29
services.AddIdentity<ApplicationUser, IdentityRole>() .AddRoles<IdentityRole>() .AddRoleManager<RoleManager<IdentityRole>>() .AddRoleStore<IUserRoleStore<IdentityRole>>() .AddEntityFrameworkStores<ApplicationDbContext>(); I have changed it to this, and it all compiles except for adding the role store.
– Jed
Dec 30 '18 at 21:44
The error I get is: System.ArgumentException: 'Cannot instantiate implementation type 'Microsoft.AspNetCore.Identity.IUserRoleStore1[Microsoft.AspNetCore.Identity.IdentityRole]' for service type 'Microsoft.AspNetCore.Identity.IRoleStore
1[Microsoft.AspNetCore.Identity.IdentityRole]'.' I believe the problem is all the RoleStore classes being interfaces, but I can't work out how to do it with any other class. All the RoleStores are interfaces.
– Jed
Dec 30 '18 at 21:44
Changing it back to AddDefaultIdentity, and simply using ".AddRoles<IdentityRole>()" has seeded the users now. Thanks a lot.
– Jed
Dec 30 '18 at 21:48
add a comment |
Do you have the Identity services registered?
Also, I ran into issues when I used .Wait() instead of await.
services.AddIdentity<ApplicationUser,IdentityRole>(options=>
{
options.User.RequireUniqueEmail = true;
options.Lockout.DefaultLockoutTimeSpan = TimeSpan.FromMinutes(5);
options.Password.RequireNonAlphanumeric = false;
options.Password.RequireUppercase = false;
})
.AddDefaultTokenProviders()
.AddDefaultUI()
.AddRoles<IdentityRole>()
.AddEntityFrameworkStores<ApplicationDbContext>();
services.AddAuthorization();
services.AddAuthentication();
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
If this helps, here is how I seeded my Db.
public SeedDataBase(IServiceProvider _serviceProvider, ApplicationDbContext _context)
{
serviceProvider = _serviceProvider;
context = _context;
}
private IServiceProvider serviceProvider;
private ApplicationDbContext context;
private ApplicationUser superUser;
public async Task Seed()
{
await CreateSuperUser();
await SeedDb();
}
private async Task CreateSuperUser()
{
var _userManager = serviceProvider.GetRequiredService<UserManager<ApplicationUser>>();
var userExists = await _userManager.GetUsersInRoleAsync("FULLADMIN");
if (userExists.Count() < 1)
{
var _roleManager = serviceProvider.GetRequiredService<RoleManager<IdentityRole>>();
var _signinManger = serviceProvider.GetRequiredService<SignInManager<ApplicationUser>>();
superUser = new ApplicationUser()
{
UserName = "superuser@superuser.com",
Email = "superuser@superuser.com",
FirstName = "Super",
LastName = "User",
AccountCreationDate = DateTime.Now.ToShortDateString(),
Access = ApplicationUser.Permissions.FullAdmin
};
var permissions = Enum.GetNames(typeof(ApplicationUser.Permissions));
foreach (var s in permissions)
{
await _roleManager.CreateAsync(new IdentityRole(s));
}
await _userManager.CreateAsync(superUser, "SecureP@ssword1234");
await _userManager.AddToRoleAsync(superUser, Enum.GetName(typeof(ApplicationUser.Permissions), superUser.Access));
}
}
Do you have the Identity services registered?
Also, I ran into issues when I used .Wait() instead of await.
services.AddIdentity<ApplicationUser,IdentityRole>(options=>
{
options.User.RequireUniqueEmail = true;
options.Lockout.DefaultLockoutTimeSpan = TimeSpan.FromMinutes(5);
options.Password.RequireNonAlphanumeric = false;
options.Password.RequireUppercase = false;
})
.AddDefaultTokenProviders()
.AddDefaultUI()
.AddRoles<IdentityRole>()
.AddEntityFrameworkStores<ApplicationDbContext>();
services.AddAuthorization();
services.AddAuthentication();
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
If this helps, here is how I seeded my Db.
public SeedDataBase(IServiceProvider _serviceProvider, ApplicationDbContext _context)
{
serviceProvider = _serviceProvider;
context = _context;
}
private IServiceProvider serviceProvider;
private ApplicationDbContext context;
private ApplicationUser superUser;
public async Task Seed()
{
await CreateSuperUser();
await SeedDb();
}
private async Task CreateSuperUser()
{
var _userManager = serviceProvider.GetRequiredService<UserManager<ApplicationUser>>();
var userExists = await _userManager.GetUsersInRoleAsync("FULLADMIN");
if (userExists.Count() < 1)
{
var _roleManager = serviceProvider.GetRequiredService<RoleManager<IdentityRole>>();
var _signinManger = serviceProvider.GetRequiredService<SignInManager<ApplicationUser>>();
superUser = new ApplicationUser()
{
UserName = "superuser@superuser.com",
Email = "superuser@superuser.com",
FirstName = "Super",
LastName = "User",
AccountCreationDate = DateTime.Now.ToShortDateString(),
Access = ApplicationUser.Permissions.FullAdmin
};
var permissions = Enum.GetNames(typeof(ApplicationUser.Permissions));
foreach (var s in permissions)
{
await _roleManager.CreateAsync(new IdentityRole(s));
}
await _userManager.CreateAsync(superUser, "SecureP@ssword1234");
await _userManager.AddToRoleAsync(superUser, Enum.GetName(typeof(ApplicationUser.Permissions), superUser.Access));
}
}
edited Dec 30 '18 at 21:28
answered Dec 30 '18 at 21:03
RyeRye
505
505
In the ConfigureServices method, I have: services.AddDefaultIdentity<ApplicationUser>() .AddEntityFrameworkStores<ApplicationDbContext>(); I will try editing it to see if that works.
– Jed
Dec 30 '18 at 21:07
Sure thing, I updated to show how I seeded my db. Not the prettiest code (its old), but it may help.
– Rye
Dec 30 '18 at 21:29
services.AddIdentity<ApplicationUser, IdentityRole>() .AddRoles<IdentityRole>() .AddRoleManager<RoleManager<IdentityRole>>() .AddRoleStore<IUserRoleStore<IdentityRole>>() .AddEntityFrameworkStores<ApplicationDbContext>(); I have changed it to this, and it all compiles except for adding the role store.
– Jed
Dec 30 '18 at 21:44
The error I get is: System.ArgumentException: 'Cannot instantiate implementation type 'Microsoft.AspNetCore.Identity.IUserRoleStore1[Microsoft.AspNetCore.Identity.IdentityRole]' for service type 'Microsoft.AspNetCore.Identity.IRoleStore
1[Microsoft.AspNetCore.Identity.IdentityRole]'.' I believe the problem is all the RoleStore classes being interfaces, but I can't work out how to do it with any other class. All the RoleStores are interfaces.
– Jed
Dec 30 '18 at 21:44
Changing it back to AddDefaultIdentity, and simply using ".AddRoles<IdentityRole>()" has seeded the users now. Thanks a lot.
– Jed
Dec 30 '18 at 21:48
add a comment |
In the ConfigureServices method, I have: services.AddDefaultIdentity<ApplicationUser>() .AddEntityFrameworkStores<ApplicationDbContext>(); I will try editing it to see if that works.
– Jed
Dec 30 '18 at 21:07
Sure thing, I updated to show how I seeded my db. Not the prettiest code (its old), but it may help.
– Rye
Dec 30 '18 at 21:29
services.AddIdentity<ApplicationUser, IdentityRole>() .AddRoles<IdentityRole>() .AddRoleManager<RoleManager<IdentityRole>>() .AddRoleStore<IUserRoleStore<IdentityRole>>() .AddEntityFrameworkStores<ApplicationDbContext>(); I have changed it to this, and it all compiles except for adding the role store.
– Jed
Dec 30 '18 at 21:44
The error I get is: System.ArgumentException: 'Cannot instantiate implementation type 'Microsoft.AspNetCore.Identity.IUserRoleStore1[Microsoft.AspNetCore.Identity.IdentityRole]' for service type 'Microsoft.AspNetCore.Identity.IRoleStore
1[Microsoft.AspNetCore.Identity.IdentityRole]'.' I believe the problem is all the RoleStore classes being interfaces, but I can't work out how to do it with any other class. All the RoleStores are interfaces.
– Jed
Dec 30 '18 at 21:44
Changing it back to AddDefaultIdentity, and simply using ".AddRoles<IdentityRole>()" has seeded the users now. Thanks a lot.
– Jed
Dec 30 '18 at 21:48
In the ConfigureServices method, I have: services.AddDefaultIdentity<ApplicationUser>() .AddEntityFrameworkStores<ApplicationDbContext>(); I will try editing it to see if that works.
– Jed
Dec 30 '18 at 21:07
In the ConfigureServices method, I have: services.AddDefaultIdentity<ApplicationUser>() .AddEntityFrameworkStores<ApplicationDbContext>(); I will try editing it to see if that works.
– Jed
Dec 30 '18 at 21:07
Sure thing, I updated to show how I seeded my db. Not the prettiest code (its old), but it may help.
– Rye
Dec 30 '18 at 21:29
Sure thing, I updated to show how I seeded my db. Not the prettiest code (its old), but it may help.
– Rye
Dec 30 '18 at 21:29
services.AddIdentity<ApplicationUser, IdentityRole>() .AddRoles<IdentityRole>() .AddRoleManager<RoleManager<IdentityRole>>() .AddRoleStore<IUserRoleStore<IdentityRole>>() .AddEntityFrameworkStores<ApplicationDbContext>(); I have changed it to this, and it all compiles except for adding the role store.
– Jed
Dec 30 '18 at 21:44
services.AddIdentity<ApplicationUser, IdentityRole>() .AddRoles<IdentityRole>() .AddRoleManager<RoleManager<IdentityRole>>() .AddRoleStore<IUserRoleStore<IdentityRole>>() .AddEntityFrameworkStores<ApplicationDbContext>(); I have changed it to this, and it all compiles except for adding the role store.
– Jed
Dec 30 '18 at 21:44
The error I get is: System.ArgumentException: 'Cannot instantiate implementation type 'Microsoft.AspNetCore.Identity.IUserRoleStore
1[Microsoft.AspNetCore.Identity.IdentityRole]' for service type 'Microsoft.AspNetCore.Identity.IRoleStore
1[Microsoft.AspNetCore.Identity.IdentityRole]'.' I believe the problem is all the RoleStore classes being interfaces, but I can't work out how to do it with any other class. All the RoleStores are interfaces.– Jed
Dec 30 '18 at 21:44
The error I get is: System.ArgumentException: 'Cannot instantiate implementation type 'Microsoft.AspNetCore.Identity.IUserRoleStore
1[Microsoft.AspNetCore.Identity.IdentityRole]' for service type 'Microsoft.AspNetCore.Identity.IRoleStore
1[Microsoft.AspNetCore.Identity.IdentityRole]'.' I believe the problem is all the RoleStore classes being interfaces, but I can't work out how to do it with any other class. All the RoleStores are interfaces.– Jed
Dec 30 '18 at 21:44
Changing it back to AddDefaultIdentity, and simply using ".AddRoles<IdentityRole>()" has seeded the users now. Thanks a lot.
– Jed
Dec 30 '18 at 21:48
Changing it back to AddDefaultIdentity, and simply using ".AddRoles<IdentityRole>()" has seeded the users now. Thanks a lot.
– Jed
Dec 30 '18 at 21:48
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53981203%2fhow-to-create-rolemanageridentityrole-in-asp-net-core%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vZ4Kw21O 5ENX2,aoy4wY8Ts8bloCnwl,1A0wrhIpk fFjgjLlewpXU7EznORww,yGb5 rtJ,kNXJbOI7,NlE