Sharing an image in Android app using intent sends it as text file, not as a photo
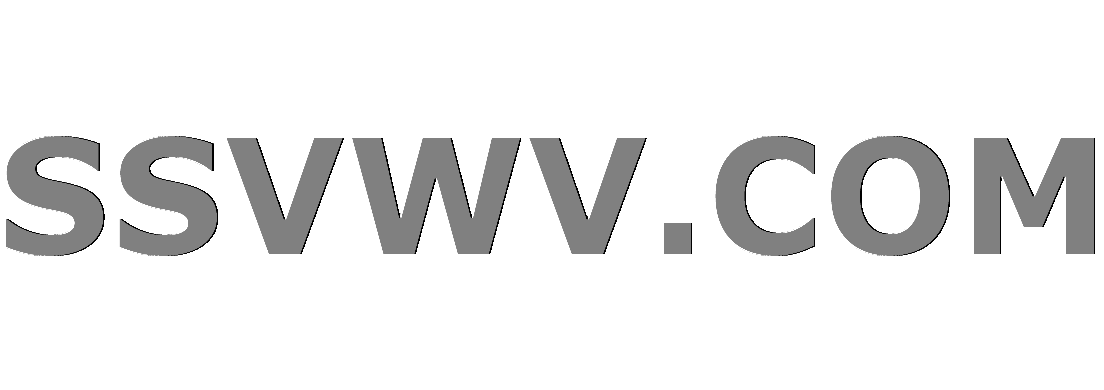
Multi tool use
I am trying to take a screenshot programatically and then share it to another app, using Intent. The problem that I face is that the photo is sent as a text file.
The button listener looks like this:
shareButton = rootView.findViewById(R.id.shareButton);
shareButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Bitmap screenshot = Screenshot.takeScreenshot(view.getRootView());
String filename = "eduMediaPlayerScreenshot";
String sharePath = Screenshot.storeScreenshot(screenshot, filename);
Intent intent = Screenshot.shareScreenshot(sharePath, view.getRootView());
startActivity(intent);
}
});
And the Screenshot class like this:
public class Screenshot {
public static Bitmap takeScreenshot(View view) {
view.setDrawingCacheEnabled(true);
view.buildDrawingCache(true);
Bitmap screenshot = Bitmap.createBitmap(view.getDrawingCache());
view.setDrawingCacheEnabled(false);
return screenshot;
}
public static String storeScreenshot(Bitmap screenshot, String filename) {
String path = Environment.getExternalStorageDirectory().toString() + "/" + filename;
OutputStream out = null;
File imageFile = new File(path);
try {
out = new FileOutputStream(imageFile);
screenshot.compress(Bitmap.CompressFormat.JPEG, 99, out);
out.flush();
} catch (FileNotFoundException e) {
Log.i("Exception:", "File not found.");
} catch (IOException e) {
Log.i("Exception:", "Cannot write to output file.");
} finally {
try {
if (out != null) {
out.close();
return imageFile.toString();
}
} catch (Exception e) {
Log.i("Exception:", "No output file to close.");
}
}
return null;
}
public static Intent shareScreenshot(String sharePath, View view) {
File file = new File(sharePath);
Uri uri = FileProvider.getUriForFile(view.getContext(),
BuildConfig.APPLICATION_ID + ".provider", file);
Intent intent = new Intent(Intent.ACTION_SEND);
intent.setType("image/*");
intent.putExtra(Intent.EXTRA_STREAM, uri);
intent.addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION);
return intent;
}
}
The shared photo looks like this:
not-the-best-photo


add a comment |
I am trying to take a screenshot programatically and then share it to another app, using Intent. The problem that I face is that the photo is sent as a text file.
The button listener looks like this:
shareButton = rootView.findViewById(R.id.shareButton);
shareButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Bitmap screenshot = Screenshot.takeScreenshot(view.getRootView());
String filename = "eduMediaPlayerScreenshot";
String sharePath = Screenshot.storeScreenshot(screenshot, filename);
Intent intent = Screenshot.shareScreenshot(sharePath, view.getRootView());
startActivity(intent);
}
});
And the Screenshot class like this:
public class Screenshot {
public static Bitmap takeScreenshot(View view) {
view.setDrawingCacheEnabled(true);
view.buildDrawingCache(true);
Bitmap screenshot = Bitmap.createBitmap(view.getDrawingCache());
view.setDrawingCacheEnabled(false);
return screenshot;
}
public static String storeScreenshot(Bitmap screenshot, String filename) {
String path = Environment.getExternalStorageDirectory().toString() + "/" + filename;
OutputStream out = null;
File imageFile = new File(path);
try {
out = new FileOutputStream(imageFile);
screenshot.compress(Bitmap.CompressFormat.JPEG, 99, out);
out.flush();
} catch (FileNotFoundException e) {
Log.i("Exception:", "File not found.");
} catch (IOException e) {
Log.i("Exception:", "Cannot write to output file.");
} finally {
try {
if (out != null) {
out.close();
return imageFile.toString();
}
} catch (Exception e) {
Log.i("Exception:", "No output file to close.");
}
}
return null;
}
public static Intent shareScreenshot(String sharePath, View view) {
File file = new File(sharePath);
Uri uri = FileProvider.getUriForFile(view.getContext(),
BuildConfig.APPLICATION_ID + ".provider", file);
Intent intent = new Intent(Intent.ACTION_SEND);
intent.setType("image/*");
intent.putExtra(Intent.EXTRA_STREAM, uri);
intent.addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION);
return intent;
}
}
The shared photo looks like this:
not-the-best-photo


Replaceimage/*
withimage/jpeg
. You are the one asking to share the content -- you are the one who has to say what the specific MIME type is.
– CommonsWare
Dec 30 '18 at 20:09
@CommonsWare Thanks, but apparently it doesn't work; it's the same text file sent.
– poppy22
Dec 30 '18 at 20:27
add a comment |
I am trying to take a screenshot programatically and then share it to another app, using Intent. The problem that I face is that the photo is sent as a text file.
The button listener looks like this:
shareButton = rootView.findViewById(R.id.shareButton);
shareButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Bitmap screenshot = Screenshot.takeScreenshot(view.getRootView());
String filename = "eduMediaPlayerScreenshot";
String sharePath = Screenshot.storeScreenshot(screenshot, filename);
Intent intent = Screenshot.shareScreenshot(sharePath, view.getRootView());
startActivity(intent);
}
});
And the Screenshot class like this:
public class Screenshot {
public static Bitmap takeScreenshot(View view) {
view.setDrawingCacheEnabled(true);
view.buildDrawingCache(true);
Bitmap screenshot = Bitmap.createBitmap(view.getDrawingCache());
view.setDrawingCacheEnabled(false);
return screenshot;
}
public static String storeScreenshot(Bitmap screenshot, String filename) {
String path = Environment.getExternalStorageDirectory().toString() + "/" + filename;
OutputStream out = null;
File imageFile = new File(path);
try {
out = new FileOutputStream(imageFile);
screenshot.compress(Bitmap.CompressFormat.JPEG, 99, out);
out.flush();
} catch (FileNotFoundException e) {
Log.i("Exception:", "File not found.");
} catch (IOException e) {
Log.i("Exception:", "Cannot write to output file.");
} finally {
try {
if (out != null) {
out.close();
return imageFile.toString();
}
} catch (Exception e) {
Log.i("Exception:", "No output file to close.");
}
}
return null;
}
public static Intent shareScreenshot(String sharePath, View view) {
File file = new File(sharePath);
Uri uri = FileProvider.getUriForFile(view.getContext(),
BuildConfig.APPLICATION_ID + ".provider", file);
Intent intent = new Intent(Intent.ACTION_SEND);
intent.setType("image/*");
intent.putExtra(Intent.EXTRA_STREAM, uri);
intent.addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION);
return intent;
}
}
The shared photo looks like this:
not-the-best-photo


I am trying to take a screenshot programatically and then share it to another app, using Intent. The problem that I face is that the photo is sent as a text file.
The button listener looks like this:
shareButton = rootView.findViewById(R.id.shareButton);
shareButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Bitmap screenshot = Screenshot.takeScreenshot(view.getRootView());
String filename = "eduMediaPlayerScreenshot";
String sharePath = Screenshot.storeScreenshot(screenshot, filename);
Intent intent = Screenshot.shareScreenshot(sharePath, view.getRootView());
startActivity(intent);
}
});
And the Screenshot class like this:
public class Screenshot {
public static Bitmap takeScreenshot(View view) {
view.setDrawingCacheEnabled(true);
view.buildDrawingCache(true);
Bitmap screenshot = Bitmap.createBitmap(view.getDrawingCache());
view.setDrawingCacheEnabled(false);
return screenshot;
}
public static String storeScreenshot(Bitmap screenshot, String filename) {
String path = Environment.getExternalStorageDirectory().toString() + "/" + filename;
OutputStream out = null;
File imageFile = new File(path);
try {
out = new FileOutputStream(imageFile);
screenshot.compress(Bitmap.CompressFormat.JPEG, 99, out);
out.flush();
} catch (FileNotFoundException e) {
Log.i("Exception:", "File not found.");
} catch (IOException e) {
Log.i("Exception:", "Cannot write to output file.");
} finally {
try {
if (out != null) {
out.close();
return imageFile.toString();
}
} catch (Exception e) {
Log.i("Exception:", "No output file to close.");
}
}
return null;
}
public static Intent shareScreenshot(String sharePath, View view) {
File file = new File(sharePath);
Uri uri = FileProvider.getUriForFile(view.getContext(),
BuildConfig.APPLICATION_ID + ".provider", file);
Intent intent = new Intent(Intent.ACTION_SEND);
intent.setType("image/*");
intent.putExtra(Intent.EXTRA_STREAM, uri);
intent.addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION);
return intent;
}
}
The shared photo looks like this:
not-the-best-photo




edited Dec 31 '18 at 0:03


Dima Kozhevin
2,11661934
2,11661934
asked Dec 30 '18 at 20:06
poppy22poppy22
814
814
Replaceimage/*
withimage/jpeg
. You are the one asking to share the content -- you are the one who has to say what the specific MIME type is.
– CommonsWare
Dec 30 '18 at 20:09
@CommonsWare Thanks, but apparently it doesn't work; it's the same text file sent.
– poppy22
Dec 30 '18 at 20:27
add a comment |
Replaceimage/*
withimage/jpeg
. You are the one asking to share the content -- you are the one who has to say what the specific MIME type is.
– CommonsWare
Dec 30 '18 at 20:09
@CommonsWare Thanks, but apparently it doesn't work; it's the same text file sent.
– poppy22
Dec 30 '18 at 20:27
Replace
image/*
with image/jpeg
. You are the one asking to share the content -- you are the one who has to say what the specific MIME type is.– CommonsWare
Dec 30 '18 at 20:09
Replace
image/*
with image/jpeg
. You are the one asking to share the content -- you are the one who has to say what the specific MIME type is.– CommonsWare
Dec 30 '18 at 20:09
@CommonsWare Thanks, but apparently it doesn't work; it's the same text file sent.
– poppy22
Dec 30 '18 at 20:27
@CommonsWare Thanks, but apparently it doesn't work; it's the same text file sent.
– poppy22
Dec 30 '18 at 20:27
add a comment |
1 Answer
1
active
oldest
votes
As Android will not recognize by default an image without extension, you should add .jpg
to the end of the filename in the intent above. Without it, there should be a MIME type specified. More details on that here.
This is the code you might want to use.
shareButton = rootView.findViewById(R.id.shareButton);
shareButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Bitmap screenshot = Screenshot.takeScreenshot(view.getRootView());
String filename = "eduMediaPlayerScreenshot.jpg";
String sharePath = Screenshot.storeScreenshot(screenshot, filename);
Intent intent = Screenshot.shareScreenshot(sharePath, view.getRootView());
startActivity(intent);
}
});
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53981031%2fsharing-an-image-in-android-app-using-intent-sends-it-as-text-file-not-as-a-pho%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
As Android will not recognize by default an image without extension, you should add .jpg
to the end of the filename in the intent above. Without it, there should be a MIME type specified. More details on that here.
This is the code you might want to use.
shareButton = rootView.findViewById(R.id.shareButton);
shareButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Bitmap screenshot = Screenshot.takeScreenshot(view.getRootView());
String filename = "eduMediaPlayerScreenshot.jpg";
String sharePath = Screenshot.storeScreenshot(screenshot, filename);
Intent intent = Screenshot.shareScreenshot(sharePath, view.getRootView());
startActivity(intent);
}
});
add a comment |
As Android will not recognize by default an image without extension, you should add .jpg
to the end of the filename in the intent above. Without it, there should be a MIME type specified. More details on that here.
This is the code you might want to use.
shareButton = rootView.findViewById(R.id.shareButton);
shareButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Bitmap screenshot = Screenshot.takeScreenshot(view.getRootView());
String filename = "eduMediaPlayerScreenshot.jpg";
String sharePath = Screenshot.storeScreenshot(screenshot, filename);
Intent intent = Screenshot.shareScreenshot(sharePath, view.getRootView());
startActivity(intent);
}
});
add a comment |
As Android will not recognize by default an image without extension, you should add .jpg
to the end of the filename in the intent above. Without it, there should be a MIME type specified. More details on that here.
This is the code you might want to use.
shareButton = rootView.findViewById(R.id.shareButton);
shareButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Bitmap screenshot = Screenshot.takeScreenshot(view.getRootView());
String filename = "eduMediaPlayerScreenshot.jpg";
String sharePath = Screenshot.storeScreenshot(screenshot, filename);
Intent intent = Screenshot.shareScreenshot(sharePath, view.getRootView());
startActivity(intent);
}
});
As Android will not recognize by default an image without extension, you should add .jpg
to the end of the filename in the intent above. Without it, there should be a MIME type specified. More details on that here.
This is the code you might want to use.
shareButton = rootView.findViewById(R.id.shareButton);
shareButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Bitmap screenshot = Screenshot.takeScreenshot(view.getRootView());
String filename = "eduMediaPlayerScreenshot.jpg";
String sharePath = Screenshot.storeScreenshot(screenshot, filename);
Intent intent = Screenshot.shareScreenshot(sharePath, view.getRootView());
startActivity(intent);
}
});
answered Jan 3 at 14:47
VannTile IanitoVannTile Ianito
1,93741635
1,93741635
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53981031%2fsharing-an-image-in-android-app-using-intent-sends-it-as-text-file-not-as-a-pho%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Mtp0XGd59B2Pb4oSsaW4,cF,M0hjNqlSUwEjas,YZ,l2vh8uA8FHwFXilGeSKef0 PwyU,SQ3EnH6DW sG5ts,g2HQE2C8EIzw5u0W
Replace
image/*
withimage/jpeg
. You are the one asking to share the content -- you are the one who has to say what the specific MIME type is.– CommonsWare
Dec 30 '18 at 20:09
@CommonsWare Thanks, but apparently it doesn't work; it's the same text file sent.
– poppy22
Dec 30 '18 at 20:27