readQuery from cache results in Can't find field on object undefined
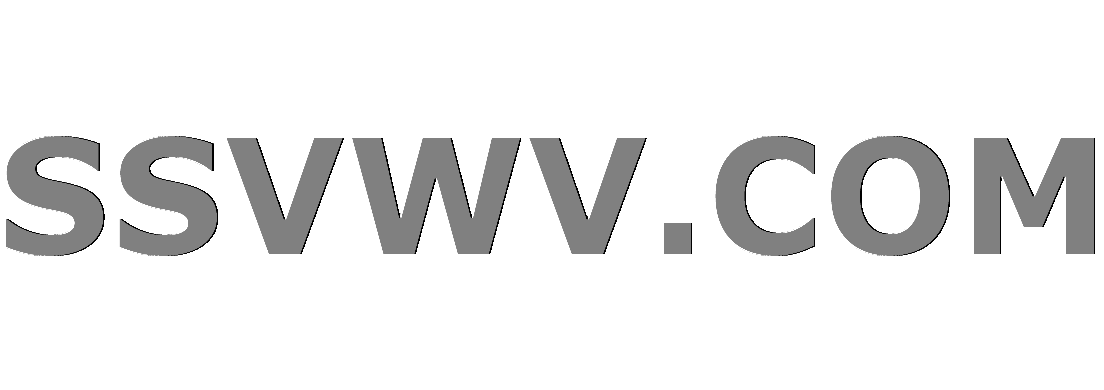
Multi tool use
I'm getting started with graphQL and apollo. I'm doing some simple queries and mutations fine but when I try to access data from apollo cache I keep getting "Can't find field me on object undefined" but when using apollo chrome plugin it returns data correct.
When logged into my app I run a simple query that fetches the user object from the server. It logs correct, I can see in chome dev apollo under queries and cache that my user data is there.
const ME_QUERY = gql`
query {
me {
_id
username
password
exchanges {
name
active
}
}
}
`;
<Query query={ME_QUERY}>
{({loading, error, data}) => {
if (error) {
return <Error error={error.message} />;
}
if (data) {
console.log(data, 'data');
}
return <div>asdf</div>
}}
</Query>
I have wrapped withApollo and I can log the client object fine in the component where I'm trying to query. I run the following code:
componentDidMount() {
console.log(this.props.client.readQuery, 'propps');
const user = this.props.client.readQuery({
query: gql`
{
me {
_id
username
password
exchanges {
name
active
}
}
}
`
});
console.log(user, 'user');
And that results in Uncaught Error: Can't find field me on object undefined Ive also tried with adding variables but it doesn't help.
Which object is undefined? Any idea why my query fails?
react-apollo apollo-client
add a comment |
I'm getting started with graphQL and apollo. I'm doing some simple queries and mutations fine but when I try to access data from apollo cache I keep getting "Can't find field me on object undefined" but when using apollo chrome plugin it returns data correct.
When logged into my app I run a simple query that fetches the user object from the server. It logs correct, I can see in chome dev apollo under queries and cache that my user data is there.
const ME_QUERY = gql`
query {
me {
_id
username
password
exchanges {
name
active
}
}
}
`;
<Query query={ME_QUERY}>
{({loading, error, data}) => {
if (error) {
return <Error error={error.message} />;
}
if (data) {
console.log(data, 'data');
}
return <div>asdf</div>
}}
</Query>
I have wrapped withApollo and I can log the client object fine in the component where I'm trying to query. I run the following code:
componentDidMount() {
console.log(this.props.client.readQuery, 'propps');
const user = this.props.client.readQuery({
query: gql`
{
me {
_id
username
password
exchanges {
name
active
}
}
}
`
});
console.log(user, 'user');
And that results in Uncaught Error: Can't find field me on object undefined Ive also tried with adding variables but it doesn't help.
Which object is undefined? Any idea why my query fails?
react-apollo apollo-client
Does adding wordquery
to the query in readQuery fix the problem?
– pyankoff
Jan 21 at 22:25
add a comment |
I'm getting started with graphQL and apollo. I'm doing some simple queries and mutations fine but when I try to access data from apollo cache I keep getting "Can't find field me on object undefined" but when using apollo chrome plugin it returns data correct.
When logged into my app I run a simple query that fetches the user object from the server. It logs correct, I can see in chome dev apollo under queries and cache that my user data is there.
const ME_QUERY = gql`
query {
me {
_id
username
password
exchanges {
name
active
}
}
}
`;
<Query query={ME_QUERY}>
{({loading, error, data}) => {
if (error) {
return <Error error={error.message} />;
}
if (data) {
console.log(data, 'data');
}
return <div>asdf</div>
}}
</Query>
I have wrapped withApollo and I can log the client object fine in the component where I'm trying to query. I run the following code:
componentDidMount() {
console.log(this.props.client.readQuery, 'propps');
const user = this.props.client.readQuery({
query: gql`
{
me {
_id
username
password
exchanges {
name
active
}
}
}
`
});
console.log(user, 'user');
And that results in Uncaught Error: Can't find field me on object undefined Ive also tried with adding variables but it doesn't help.
Which object is undefined? Any idea why my query fails?
react-apollo apollo-client
I'm getting started with graphQL and apollo. I'm doing some simple queries and mutations fine but when I try to access data from apollo cache I keep getting "Can't find field me on object undefined" but when using apollo chrome plugin it returns data correct.
When logged into my app I run a simple query that fetches the user object from the server. It logs correct, I can see in chome dev apollo under queries and cache that my user data is there.
const ME_QUERY = gql`
query {
me {
_id
username
password
exchanges {
name
active
}
}
}
`;
<Query query={ME_QUERY}>
{({loading, error, data}) => {
if (error) {
return <Error error={error.message} />;
}
if (data) {
console.log(data, 'data');
}
return <div>asdf</div>
}}
</Query>
I have wrapped withApollo and I can log the client object fine in the component where I'm trying to query. I run the following code:
componentDidMount() {
console.log(this.props.client.readQuery, 'propps');
const user = this.props.client.readQuery({
query: gql`
{
me {
_id
username
password
exchanges {
name
active
}
}
}
`
});
console.log(user, 'user');
And that results in Uncaught Error: Can't find field me on object undefined Ive also tried with adding variables but it doesn't help.
Which object is undefined? Any idea why my query fails?
react-apollo apollo-client
react-apollo apollo-client
asked Jan 1 at 12:04
PiranhaPiranha
63
63
Does adding wordquery
to the query in readQuery fix the problem?
– pyankoff
Jan 21 at 22:25
add a comment |
Does adding wordquery
to the query in readQuery fix the problem?
– pyankoff
Jan 21 at 22:25
Does adding word
query
to the query in readQuery fix the problem?– pyankoff
Jan 21 at 22:25
Does adding word
query
to the query in readQuery fix the problem?– pyankoff
Jan 21 at 22:25
add a comment |
1 Answer
1
active
oldest
votes
This is wrong
const ME_QUERY = gql`
query {
me {
_id
username
password
exchanges {
name
active
}
}
}
`;
Try this instead:
const ME_QUERY = gql`
{
me {
_id
username
password
exchanges {
name
active
}
}
}
`;
Which will lead you to this error Error: Can't find field me on object undefined
and that I can't help you with it unless you provide me with the schema for that specific query. But I guess it is _id
it should be id
instead or maybe a missing id
inside exchanges
too.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53995283%2freadquery-from-cache-results-in-cant-find-field-field-on-object-undefined%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This is wrong
const ME_QUERY = gql`
query {
me {
_id
username
password
exchanges {
name
active
}
}
}
`;
Try this instead:
const ME_QUERY = gql`
{
me {
_id
username
password
exchanges {
name
active
}
}
}
`;
Which will lead you to this error Error: Can't find field me on object undefined
and that I can't help you with it unless you provide me with the schema for that specific query. But I guess it is _id
it should be id
instead or maybe a missing id
inside exchanges
too.
add a comment |
This is wrong
const ME_QUERY = gql`
query {
me {
_id
username
password
exchanges {
name
active
}
}
}
`;
Try this instead:
const ME_QUERY = gql`
{
me {
_id
username
password
exchanges {
name
active
}
}
}
`;
Which will lead you to this error Error: Can't find field me on object undefined
and that I can't help you with it unless you provide me with the schema for that specific query. But I guess it is _id
it should be id
instead or maybe a missing id
inside exchanges
too.
add a comment |
This is wrong
const ME_QUERY = gql`
query {
me {
_id
username
password
exchanges {
name
active
}
}
}
`;
Try this instead:
const ME_QUERY = gql`
{
me {
_id
username
password
exchanges {
name
active
}
}
}
`;
Which will lead you to this error Error: Can't find field me on object undefined
and that I can't help you with it unless you provide me with the schema for that specific query. But I guess it is _id
it should be id
instead or maybe a missing id
inside exchanges
too.
This is wrong
const ME_QUERY = gql`
query {
me {
_id
username
password
exchanges {
name
active
}
}
}
`;
Try this instead:
const ME_QUERY = gql`
{
me {
_id
username
password
exchanges {
name
active
}
}
}
`;
Which will lead you to this error Error: Can't find field me on object undefined
and that I can't help you with it unless you provide me with the schema for that specific query. But I guess it is _id
it should be id
instead or maybe a missing id
inside exchanges
too.
answered Feb 15 at 22:15
Rachid RhafourRachid Rhafour
33418
33418
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53995283%2freadquery-from-cache-results-in-cant-find-field-field-on-object-undefined%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ky9hNgyZ
Does adding word
query
to the query in readQuery fix the problem?– pyankoff
Jan 21 at 22:25