Printing copies of document from form textbox
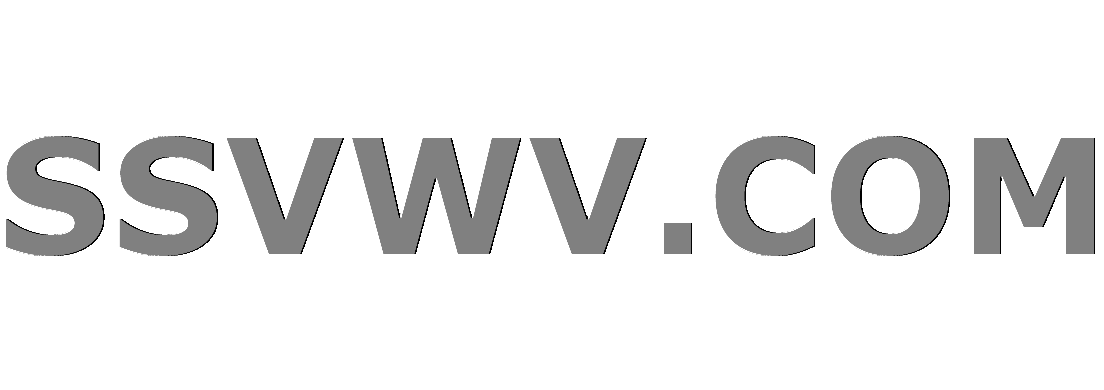
Multi tool use
Is there a way to print the number of copies given in a textbox in a C# windows form application?
With my current code the document gets printed the required number of prints, but after the first print there is a dialog that says that the document already is opened and I have to open a copy. When I open this copy, the document prints again, but only if I accept to open the copy. Is there a way to print the document multiple times without getting the dialog of the copy of the document every time?
When the form is filled in correct, I want it to be stored on my hard drive and want it to print the number given in a textbox on a previous form. The value from this textbox is stored in the variable intAantalPoorten.
Thanks a lot!
Regards Bert
CreateWordDocument(@"N:De wienesProductieformulierenSjablonenPoortblad.docx", @"N:De wienesProductieformulierenProducties poortbladenPoortblad " + txtKlantnaam.Text + "-" + txtReferentie.Text + ".docx");
ProcessStartInfo info = new ProcessStartInfo(@"N:De wienesProductieformulierenProducties poortbladenPoortblad " + txtKlantnaam.Text + "-" + txtReferentie.Text + ".docx");
for (intAAntalPrints = 0; intAAntalPrints <= intAantalPoorten; intAAntalPrints++)
{
info.Verb = "Print";
info.CreateNoWindow = true;
info.WindowStyle = ProcessWindowStyle.Hidden;
Process.Start(info);
}
c# winforms
add a comment |
Is there a way to print the number of copies given in a textbox in a C# windows form application?
With my current code the document gets printed the required number of prints, but after the first print there is a dialog that says that the document already is opened and I have to open a copy. When I open this copy, the document prints again, but only if I accept to open the copy. Is there a way to print the document multiple times without getting the dialog of the copy of the document every time?
When the form is filled in correct, I want it to be stored on my hard drive and want it to print the number given in a textbox on a previous form. The value from this textbox is stored in the variable intAantalPoorten.
Thanks a lot!
Regards Bert
CreateWordDocument(@"N:De wienesProductieformulierenSjablonenPoortblad.docx", @"N:De wienesProductieformulierenProducties poortbladenPoortblad " + txtKlantnaam.Text + "-" + txtReferentie.Text + ".docx");
ProcessStartInfo info = new ProcessStartInfo(@"N:De wienesProductieformulierenProducties poortbladenPoortblad " + txtKlantnaam.Text + "-" + txtReferentie.Text + ".docx");
for (intAAntalPrints = 0; intAAntalPrints <= intAantalPoorten; intAAntalPrints++)
{
info.Verb = "Print";
info.CreateNoWindow = true;
info.WindowStyle = ProcessWindowStyle.Hidden;
Process.Start(info);
}
c# winforms
add a comment |
Is there a way to print the number of copies given in a textbox in a C# windows form application?
With my current code the document gets printed the required number of prints, but after the first print there is a dialog that says that the document already is opened and I have to open a copy. When I open this copy, the document prints again, but only if I accept to open the copy. Is there a way to print the document multiple times without getting the dialog of the copy of the document every time?
When the form is filled in correct, I want it to be stored on my hard drive and want it to print the number given in a textbox on a previous form. The value from this textbox is stored in the variable intAantalPoorten.
Thanks a lot!
Regards Bert
CreateWordDocument(@"N:De wienesProductieformulierenSjablonenPoortblad.docx", @"N:De wienesProductieformulierenProducties poortbladenPoortblad " + txtKlantnaam.Text + "-" + txtReferentie.Text + ".docx");
ProcessStartInfo info = new ProcessStartInfo(@"N:De wienesProductieformulierenProducties poortbladenPoortblad " + txtKlantnaam.Text + "-" + txtReferentie.Text + ".docx");
for (intAAntalPrints = 0; intAAntalPrints <= intAantalPoorten; intAAntalPrints++)
{
info.Verb = "Print";
info.CreateNoWindow = true;
info.WindowStyle = ProcessWindowStyle.Hidden;
Process.Start(info);
}
c# winforms
Is there a way to print the number of copies given in a textbox in a C# windows form application?
With my current code the document gets printed the required number of prints, but after the first print there is a dialog that says that the document already is opened and I have to open a copy. When I open this copy, the document prints again, but only if I accept to open the copy. Is there a way to print the document multiple times without getting the dialog of the copy of the document every time?
When the form is filled in correct, I want it to be stored on my hard drive and want it to print the number given in a textbox on a previous form. The value from this textbox is stored in the variable intAantalPoorten.
Thanks a lot!
Regards Bert
CreateWordDocument(@"N:De wienesProductieformulierenSjablonenPoortblad.docx", @"N:De wienesProductieformulierenProducties poortbladenPoortblad " + txtKlantnaam.Text + "-" + txtReferentie.Text + ".docx");
ProcessStartInfo info = new ProcessStartInfo(@"N:De wienesProductieformulierenProducties poortbladenPoortblad " + txtKlantnaam.Text + "-" + txtReferentie.Text + ".docx");
for (intAAntalPrints = 0; intAAntalPrints <= intAantalPoorten; intAAntalPrints++)
{
info.Verb = "Print";
info.CreateNoWindow = true;
info.WindowStyle = ProcessWindowStyle.Hidden;
Process.Start(info);
}
c# winforms
c# winforms
asked Dec 31 '18 at 14:12


Bert SwinnenBert Swinnen
103
103
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You're probably not using the best programming model. The thing you're doing is relying on the windows shell to open the document and invoke the registered verb (as defined by Word in the local registry). This is the most primitive and least functional way to get the job done. For example, there's no way to change which printer you're printing to...or how many copies or which pages, etc.
Instead, there's a rich programming model that Word (and all the Office apps) provide. You can open documents, and print them specifying the number of copies that you want.
To get access to this programming model, you need to reference the .COM interop assembly for Word, which is:
...and the Office Core interop assembly:
Then, it's just a matter of driving Word to do what you want. For example:
using System;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Form1: Form
{
public Form1( )
{
InitializeComponent( );
}
private void button1_Click( object sender, EventArgs e )
{
//--> assumes the textBox1.Text contains the file to open...
var app = new Microsoft.Office.Interop.Word.Application( );
var doc = app.Documents.Open( textBox1.Text, ReadOnly: true );
doc.PrintOut( Copies: 1 );
doc.Close( );
app.Quit( );
}
}
}
Note that this requires Word to be installed on the local machine. For more information, check out the word object model.
Thanks for your answer! I've tried this, but I am getting an error: 'Application' is an ambiguous reference between 'System.Windows.Forms.Application' and 'Microsoft.Office.Interop.Word.Application'. Also I would like to print on a button click, not in the static void Main. I know this is not the best solution for the problem, but due to the fact I am a beginner, all my code are programmed on a button-click event. I am learning to use classes, but at this moment I am not really good at it.
– Bert Swinnen
Jan 2 at 14:56
No worries...I only put the console app in as the smallest/simplest example. You could just put the 5 lines in the main inside your button click. As for the ambiguous reference, it's because there's anApplication
object in both theforms
world and theword
world. You just have to prefix the application with the namespace...I edited the answer to illustrate.
– Clay
Jan 2 at 17:34
This is the solution I was looking for! Thanks a lot Clay!
– Bert Swinnen
Jan 2 at 19:46
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53988415%2fprinting-copies-of-document-from-form-textbox%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You're probably not using the best programming model. The thing you're doing is relying on the windows shell to open the document and invoke the registered verb (as defined by Word in the local registry). This is the most primitive and least functional way to get the job done. For example, there's no way to change which printer you're printing to...or how many copies or which pages, etc.
Instead, there's a rich programming model that Word (and all the Office apps) provide. You can open documents, and print them specifying the number of copies that you want.
To get access to this programming model, you need to reference the .COM interop assembly for Word, which is:
...and the Office Core interop assembly:
Then, it's just a matter of driving Word to do what you want. For example:
using System;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Form1: Form
{
public Form1( )
{
InitializeComponent( );
}
private void button1_Click( object sender, EventArgs e )
{
//--> assumes the textBox1.Text contains the file to open...
var app = new Microsoft.Office.Interop.Word.Application( );
var doc = app.Documents.Open( textBox1.Text, ReadOnly: true );
doc.PrintOut( Copies: 1 );
doc.Close( );
app.Quit( );
}
}
}
Note that this requires Word to be installed on the local machine. For more information, check out the word object model.
Thanks for your answer! I've tried this, but I am getting an error: 'Application' is an ambiguous reference between 'System.Windows.Forms.Application' and 'Microsoft.Office.Interop.Word.Application'. Also I would like to print on a button click, not in the static void Main. I know this is not the best solution for the problem, but due to the fact I am a beginner, all my code are programmed on a button-click event. I am learning to use classes, but at this moment I am not really good at it.
– Bert Swinnen
Jan 2 at 14:56
No worries...I only put the console app in as the smallest/simplest example. You could just put the 5 lines in the main inside your button click. As for the ambiguous reference, it's because there's anApplication
object in both theforms
world and theword
world. You just have to prefix the application with the namespace...I edited the answer to illustrate.
– Clay
Jan 2 at 17:34
This is the solution I was looking for! Thanks a lot Clay!
– Bert Swinnen
Jan 2 at 19:46
add a comment |
You're probably not using the best programming model. The thing you're doing is relying on the windows shell to open the document and invoke the registered verb (as defined by Word in the local registry). This is the most primitive and least functional way to get the job done. For example, there's no way to change which printer you're printing to...or how many copies or which pages, etc.
Instead, there's a rich programming model that Word (and all the Office apps) provide. You can open documents, and print them specifying the number of copies that you want.
To get access to this programming model, you need to reference the .COM interop assembly for Word, which is:
...and the Office Core interop assembly:
Then, it's just a matter of driving Word to do what you want. For example:
using System;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Form1: Form
{
public Form1( )
{
InitializeComponent( );
}
private void button1_Click( object sender, EventArgs e )
{
//--> assumes the textBox1.Text contains the file to open...
var app = new Microsoft.Office.Interop.Word.Application( );
var doc = app.Documents.Open( textBox1.Text, ReadOnly: true );
doc.PrintOut( Copies: 1 );
doc.Close( );
app.Quit( );
}
}
}
Note that this requires Word to be installed on the local machine. For more information, check out the word object model.
Thanks for your answer! I've tried this, but I am getting an error: 'Application' is an ambiguous reference between 'System.Windows.Forms.Application' and 'Microsoft.Office.Interop.Word.Application'. Also I would like to print on a button click, not in the static void Main. I know this is not the best solution for the problem, but due to the fact I am a beginner, all my code are programmed on a button-click event. I am learning to use classes, but at this moment I am not really good at it.
– Bert Swinnen
Jan 2 at 14:56
No worries...I only put the console app in as the smallest/simplest example. You could just put the 5 lines in the main inside your button click. As for the ambiguous reference, it's because there's anApplication
object in both theforms
world and theword
world. You just have to prefix the application with the namespace...I edited the answer to illustrate.
– Clay
Jan 2 at 17:34
This is the solution I was looking for! Thanks a lot Clay!
– Bert Swinnen
Jan 2 at 19:46
add a comment |
You're probably not using the best programming model. The thing you're doing is relying on the windows shell to open the document and invoke the registered verb (as defined by Word in the local registry). This is the most primitive and least functional way to get the job done. For example, there's no way to change which printer you're printing to...or how many copies or which pages, etc.
Instead, there's a rich programming model that Word (and all the Office apps) provide. You can open documents, and print them specifying the number of copies that you want.
To get access to this programming model, you need to reference the .COM interop assembly for Word, which is:
...and the Office Core interop assembly:
Then, it's just a matter of driving Word to do what you want. For example:
using System;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Form1: Form
{
public Form1( )
{
InitializeComponent( );
}
private void button1_Click( object sender, EventArgs e )
{
//--> assumes the textBox1.Text contains the file to open...
var app = new Microsoft.Office.Interop.Word.Application( );
var doc = app.Documents.Open( textBox1.Text, ReadOnly: true );
doc.PrintOut( Copies: 1 );
doc.Close( );
app.Quit( );
}
}
}
Note that this requires Word to be installed on the local machine. For more information, check out the word object model.
You're probably not using the best programming model. The thing you're doing is relying on the windows shell to open the document and invoke the registered verb (as defined by Word in the local registry). This is the most primitive and least functional way to get the job done. For example, there's no way to change which printer you're printing to...or how many copies or which pages, etc.
Instead, there's a rich programming model that Word (and all the Office apps) provide. You can open documents, and print them specifying the number of copies that you want.
To get access to this programming model, you need to reference the .COM interop assembly for Word, which is:
...and the Office Core interop assembly:
Then, it's just a matter of driving Word to do what you want. For example:
using System;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Form1: Form
{
public Form1( )
{
InitializeComponent( );
}
private void button1_Click( object sender, EventArgs e )
{
//--> assumes the textBox1.Text contains the file to open...
var app = new Microsoft.Office.Interop.Word.Application( );
var doc = app.Documents.Open( textBox1.Text, ReadOnly: true );
doc.PrintOut( Copies: 1 );
doc.Close( );
app.Quit( );
}
}
}
Note that this requires Word to be installed on the local machine. For more information, check out the word object model.
edited Jan 2 at 17:33
answered Dec 31 '18 at 22:02


ClayClay
2,98611734
2,98611734
Thanks for your answer! I've tried this, but I am getting an error: 'Application' is an ambiguous reference between 'System.Windows.Forms.Application' and 'Microsoft.Office.Interop.Word.Application'. Also I would like to print on a button click, not in the static void Main. I know this is not the best solution for the problem, but due to the fact I am a beginner, all my code are programmed on a button-click event. I am learning to use classes, but at this moment I am not really good at it.
– Bert Swinnen
Jan 2 at 14:56
No worries...I only put the console app in as the smallest/simplest example. You could just put the 5 lines in the main inside your button click. As for the ambiguous reference, it's because there's anApplication
object in both theforms
world and theword
world. You just have to prefix the application with the namespace...I edited the answer to illustrate.
– Clay
Jan 2 at 17:34
This is the solution I was looking for! Thanks a lot Clay!
– Bert Swinnen
Jan 2 at 19:46
add a comment |
Thanks for your answer! I've tried this, but I am getting an error: 'Application' is an ambiguous reference between 'System.Windows.Forms.Application' and 'Microsoft.Office.Interop.Word.Application'. Also I would like to print on a button click, not in the static void Main. I know this is not the best solution for the problem, but due to the fact I am a beginner, all my code are programmed on a button-click event. I am learning to use classes, but at this moment I am not really good at it.
– Bert Swinnen
Jan 2 at 14:56
No worries...I only put the console app in as the smallest/simplest example. You could just put the 5 lines in the main inside your button click. As for the ambiguous reference, it's because there's anApplication
object in both theforms
world and theword
world. You just have to prefix the application with the namespace...I edited the answer to illustrate.
– Clay
Jan 2 at 17:34
This is the solution I was looking for! Thanks a lot Clay!
– Bert Swinnen
Jan 2 at 19:46
Thanks for your answer! I've tried this, but I am getting an error: 'Application' is an ambiguous reference between 'System.Windows.Forms.Application' and 'Microsoft.Office.Interop.Word.Application'. Also I would like to print on a button click, not in the static void Main. I know this is not the best solution for the problem, but due to the fact I am a beginner, all my code are programmed on a button-click event. I am learning to use classes, but at this moment I am not really good at it.
– Bert Swinnen
Jan 2 at 14:56
Thanks for your answer! I've tried this, but I am getting an error: 'Application' is an ambiguous reference between 'System.Windows.Forms.Application' and 'Microsoft.Office.Interop.Word.Application'. Also I would like to print on a button click, not in the static void Main. I know this is not the best solution for the problem, but due to the fact I am a beginner, all my code are programmed on a button-click event. I am learning to use classes, but at this moment I am not really good at it.
– Bert Swinnen
Jan 2 at 14:56
No worries...I only put the console app in as the smallest/simplest example. You could just put the 5 lines in the main inside your button click. As for the ambiguous reference, it's because there's an
Application
object in both the forms
world and the word
world. You just have to prefix the application with the namespace...I edited the answer to illustrate.– Clay
Jan 2 at 17:34
No worries...I only put the console app in as the smallest/simplest example. You could just put the 5 lines in the main inside your button click. As for the ambiguous reference, it's because there's an
Application
object in both the forms
world and the word
world. You just have to prefix the application with the namespace...I edited the answer to illustrate.– Clay
Jan 2 at 17:34
This is the solution I was looking for! Thanks a lot Clay!
– Bert Swinnen
Jan 2 at 19:46
This is the solution I was looking for! Thanks a lot Clay!
– Bert Swinnen
Jan 2 at 19:46
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53988415%2fprinting-copies-of-document-from-form-textbox%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5FEw9BOE IO5Gy,JCxqT8b,Z,KT,SJC,L8eYjOwUoSJfn