getJSON method returns 'undefined' in jquery
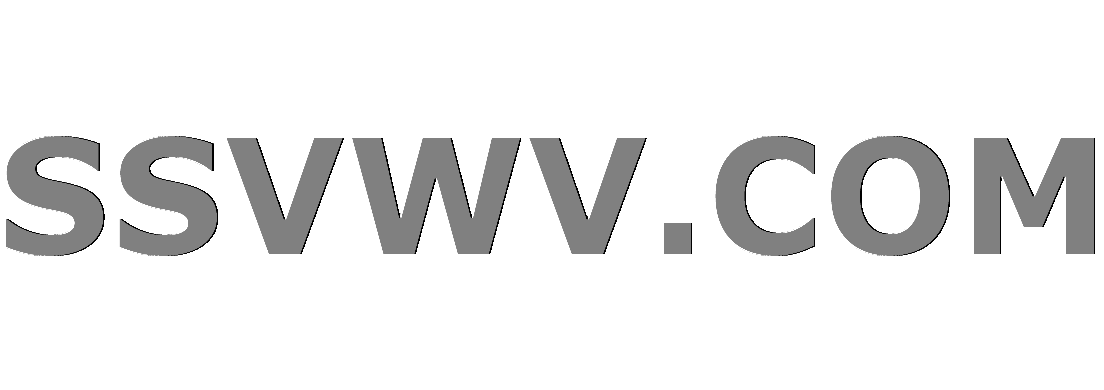
Multi tool use
I am working on an Asp.net core 2.1 project and have the below model
Person
public class Person
{
[Key]
public int ID { get; set; }
[Required]
[MaxLength(150)]
public string Name { get; set; }
[Required]
[MaxLength(150)]
public string Family { get; set; }
[Required]
public int Age { get; set; }
}
There is a web API controller in my project that you can see it.
Web API Controller
[Produces("application/json")]
[Route("api/People")]
public class PeopleController : ControllerBase
{
private readonly ApplicationContext _db;
public PeopleController(ApplicationContext db)
{
_db = db;
}
[HttpGet]
public IEnumerable<Person> GetPerson()
{
return _db.Person;
}
}
Now I want to get data from my web API controller and show in index.cshtml
Index.cshtml
<h2>List Person</h2>
<table class="table table-bordered" id="list">
<tr>
<th>ID</th>
<th>Name</th>
<th>Family</th>
<th>Age</th>
</tr>
</table>
<script>
$.getJSON("/api/People", function(res) {
$.each(res, function(key, val) {
alert(val.Age);
var item =
"<tr><td>" +
val.ID +
"</td><td>" +
val.Name +
"</td><td>" +
val.Family +
"</td><td>" +
val.Age +
"</td><td></td></tr>";
$("#list").append(item);
});
});
</script>
Actual Json in Network Tab in browser
[{"id":1,"name":"jack","family":"bfd","age":30},{"id":2,"name":"mr john","family":"sobhany","age":36}]
But there is a problem. All data shows in my view but all values are undefined
.
What is wrong in my codes?
jquery asp.net-core entity-framework-core asp.net-core-webapi
|
show 3 more comments
I am working on an Asp.net core 2.1 project and have the below model
Person
public class Person
{
[Key]
public int ID { get; set; }
[Required]
[MaxLength(150)]
public string Name { get; set; }
[Required]
[MaxLength(150)]
public string Family { get; set; }
[Required]
public int Age { get; set; }
}
There is a web API controller in my project that you can see it.
Web API Controller
[Produces("application/json")]
[Route("api/People")]
public class PeopleController : ControllerBase
{
private readonly ApplicationContext _db;
public PeopleController(ApplicationContext db)
{
_db = db;
}
[HttpGet]
public IEnumerable<Person> GetPerson()
{
return _db.Person;
}
}
Now I want to get data from my web API controller and show in index.cshtml
Index.cshtml
<h2>List Person</h2>
<table class="table table-bordered" id="list">
<tr>
<th>ID</th>
<th>Name</th>
<th>Family</th>
<th>Age</th>
</tr>
</table>
<script>
$.getJSON("/api/People", function(res) {
$.each(res, function(key, val) {
alert(val.Age);
var item =
"<tr><td>" +
val.ID +
"</td><td>" +
val.Name +
"</td><td>" +
val.Family +
"</td><td>" +
val.Age +
"</td><td></td></tr>";
$("#list").append(item);
});
});
</script>
Actual Json in Network Tab in browser
[{"id":1,"name":"jack","family":"bfd","age":30},{"id":2,"name":"mr john","family":"sobhany","age":36}]
But there is a problem. All data shows in my view but all values are undefined
.
What is wrong in my codes?
jquery asp.net-core entity-framework-core asp.net-core-webapi
1
What does the actual JSON look like (e.g., in the browser's Network tab)?
– T.J. Crowder
Dec 31 '18 at 14:02
Network tab is empty in my browser @T.J.Crowder
– saeed
Dec 31 '18 at 14:06
Then you need to ensure you perform the request with the network tab open (I guess you're using Edge or some other browser where the network tab only records things when it's open). If you're geting a response at all, clearly a network request is happening, which will show up in the network tab.
– T.J. Crowder
Dec 31 '18 at 14:08
Now i perform it with chrome browser andNetwork
tab andResponse
shows value correctly. But showsundefined
in my view. @T.J. Crowder
– saeed
Dec 31 '18 at 14:16
1
i edited question and add actual json in network tab @T.J.Crowder
– saeed
Dec 31 '18 at 14:22
|
show 3 more comments
I am working on an Asp.net core 2.1 project and have the below model
Person
public class Person
{
[Key]
public int ID { get; set; }
[Required]
[MaxLength(150)]
public string Name { get; set; }
[Required]
[MaxLength(150)]
public string Family { get; set; }
[Required]
public int Age { get; set; }
}
There is a web API controller in my project that you can see it.
Web API Controller
[Produces("application/json")]
[Route("api/People")]
public class PeopleController : ControllerBase
{
private readonly ApplicationContext _db;
public PeopleController(ApplicationContext db)
{
_db = db;
}
[HttpGet]
public IEnumerable<Person> GetPerson()
{
return _db.Person;
}
}
Now I want to get data from my web API controller and show in index.cshtml
Index.cshtml
<h2>List Person</h2>
<table class="table table-bordered" id="list">
<tr>
<th>ID</th>
<th>Name</th>
<th>Family</th>
<th>Age</th>
</tr>
</table>
<script>
$.getJSON("/api/People", function(res) {
$.each(res, function(key, val) {
alert(val.Age);
var item =
"<tr><td>" +
val.ID +
"</td><td>" +
val.Name +
"</td><td>" +
val.Family +
"</td><td>" +
val.Age +
"</td><td></td></tr>";
$("#list").append(item);
});
});
</script>
Actual Json in Network Tab in browser
[{"id":1,"name":"jack","family":"bfd","age":30},{"id":2,"name":"mr john","family":"sobhany","age":36}]
But there is a problem. All data shows in my view but all values are undefined
.
What is wrong in my codes?
jquery asp.net-core entity-framework-core asp.net-core-webapi
I am working on an Asp.net core 2.1 project and have the below model
Person
public class Person
{
[Key]
public int ID { get; set; }
[Required]
[MaxLength(150)]
public string Name { get; set; }
[Required]
[MaxLength(150)]
public string Family { get; set; }
[Required]
public int Age { get; set; }
}
There is a web API controller in my project that you can see it.
Web API Controller
[Produces("application/json")]
[Route("api/People")]
public class PeopleController : ControllerBase
{
private readonly ApplicationContext _db;
public PeopleController(ApplicationContext db)
{
_db = db;
}
[HttpGet]
public IEnumerable<Person> GetPerson()
{
return _db.Person;
}
}
Now I want to get data from my web API controller and show in index.cshtml
Index.cshtml
<h2>List Person</h2>
<table class="table table-bordered" id="list">
<tr>
<th>ID</th>
<th>Name</th>
<th>Family</th>
<th>Age</th>
</tr>
</table>
<script>
$.getJSON("/api/People", function(res) {
$.each(res, function(key, val) {
alert(val.Age);
var item =
"<tr><td>" +
val.ID +
"</td><td>" +
val.Name +
"</td><td>" +
val.Family +
"</td><td>" +
val.Age +
"</td><td></td></tr>";
$("#list").append(item);
});
});
</script>
Actual Json in Network Tab in browser
[{"id":1,"name":"jack","family":"bfd","age":30},{"id":2,"name":"mr john","family":"sobhany","age":36}]
But there is a problem. All data shows in my view but all values are undefined
.
What is wrong in my codes?
jquery asp.net-core entity-framework-core asp.net-core-webapi
jquery asp.net-core entity-framework-core asp.net-core-webapi
edited Dec 31 '18 at 14:20
saeed
asked Dec 31 '18 at 14:01
saeedsaeed
396418
396418
1
What does the actual JSON look like (e.g., in the browser's Network tab)?
– T.J. Crowder
Dec 31 '18 at 14:02
Network tab is empty in my browser @T.J.Crowder
– saeed
Dec 31 '18 at 14:06
Then you need to ensure you perform the request with the network tab open (I guess you're using Edge or some other browser where the network tab only records things when it's open). If you're geting a response at all, clearly a network request is happening, which will show up in the network tab.
– T.J. Crowder
Dec 31 '18 at 14:08
Now i perform it with chrome browser andNetwork
tab andResponse
shows value correctly. But showsundefined
in my view. @T.J. Crowder
– saeed
Dec 31 '18 at 14:16
1
i edited question and add actual json in network tab @T.J.Crowder
– saeed
Dec 31 '18 at 14:22
|
show 3 more comments
1
What does the actual JSON look like (e.g., in the browser's Network tab)?
– T.J. Crowder
Dec 31 '18 at 14:02
Network tab is empty in my browser @T.J.Crowder
– saeed
Dec 31 '18 at 14:06
Then you need to ensure you perform the request with the network tab open (I guess you're using Edge or some other browser where the network tab only records things when it's open). If you're geting a response at all, clearly a network request is happening, which will show up in the network tab.
– T.J. Crowder
Dec 31 '18 at 14:08
Now i perform it with chrome browser andNetwork
tab andResponse
shows value correctly. But showsundefined
in my view. @T.J. Crowder
– saeed
Dec 31 '18 at 14:16
1
i edited question and add actual json in network tab @T.J.Crowder
– saeed
Dec 31 '18 at 14:22
1
1
What does the actual JSON look like (e.g., in the browser's Network tab)?
– T.J. Crowder
Dec 31 '18 at 14:02
What does the actual JSON look like (e.g., in the browser's Network tab)?
– T.J. Crowder
Dec 31 '18 at 14:02
Network tab is empty in my browser @T.J.Crowder
– saeed
Dec 31 '18 at 14:06
Network tab is empty in my browser @T.J.Crowder
– saeed
Dec 31 '18 at 14:06
Then you need to ensure you perform the request with the network tab open (I guess you're using Edge or some other browser where the network tab only records things when it's open). If you're geting a response at all, clearly a network request is happening, which will show up in the network tab.
– T.J. Crowder
Dec 31 '18 at 14:08
Then you need to ensure you perform the request with the network tab open (I guess you're using Edge or some other browser where the network tab only records things when it's open). If you're geting a response at all, clearly a network request is happening, which will show up in the network tab.
– T.J. Crowder
Dec 31 '18 at 14:08
Now i perform it with chrome browser and
Network
tab and Response
shows value correctly. But shows undefined
in my view. @T.J. Crowder– saeed
Dec 31 '18 at 14:16
Now i perform it with chrome browser and
Network
tab and Response
shows value correctly. But shows undefined
in my view. @T.J. Crowder– saeed
Dec 31 '18 at 14:16
1
1
i edited question and add actual json in network tab @T.J.Crowder
– saeed
Dec 31 '18 at 14:22
i edited question and add actual json in network tab @T.J.Crowder
– saeed
Dec 31 '18 at 14:22
|
show 3 more comments
1 Answer
1
active
oldest
votes
As you can see looking at the JSON, your JSON serializer is converting your C#-oriented names like Name
(note: first letter capitalized) to JavaScript-oriented ones like name
(note: first letter in lower case):
[{"id":1,"name":"jack","family":"bfd","age":30},{"id":2,"name":"mr john","family":"sobhany","age":36}]
Note ----^^^^^^
JavaScript is a case-sensitive language, so naturally val.Name
is undefined
because there's no Name
property on val
; you want val.name
instead (and so on for id
, family
, bfd
, age
...).
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53988311%2fgetjson-method-returns-undefined-in-jquery%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
As you can see looking at the JSON, your JSON serializer is converting your C#-oriented names like Name
(note: first letter capitalized) to JavaScript-oriented ones like name
(note: first letter in lower case):
[{"id":1,"name":"jack","family":"bfd","age":30},{"id":2,"name":"mr john","family":"sobhany","age":36}]
Note ----^^^^^^
JavaScript is a case-sensitive language, so naturally val.Name
is undefined
because there's no Name
property on val
; you want val.name
instead (and so on for id
, family
, bfd
, age
...).
add a comment |
As you can see looking at the JSON, your JSON serializer is converting your C#-oriented names like Name
(note: first letter capitalized) to JavaScript-oriented ones like name
(note: first letter in lower case):
[{"id":1,"name":"jack","family":"bfd","age":30},{"id":2,"name":"mr john","family":"sobhany","age":36}]
Note ----^^^^^^
JavaScript is a case-sensitive language, so naturally val.Name
is undefined
because there's no Name
property on val
; you want val.name
instead (and so on for id
, family
, bfd
, age
...).
add a comment |
As you can see looking at the JSON, your JSON serializer is converting your C#-oriented names like Name
(note: first letter capitalized) to JavaScript-oriented ones like name
(note: first letter in lower case):
[{"id":1,"name":"jack","family":"bfd","age":30},{"id":2,"name":"mr john","family":"sobhany","age":36}]
Note ----^^^^^^
JavaScript is a case-sensitive language, so naturally val.Name
is undefined
because there's no Name
property on val
; you want val.name
instead (and so on for id
, family
, bfd
, age
...).
As you can see looking at the JSON, your JSON serializer is converting your C#-oriented names like Name
(note: first letter capitalized) to JavaScript-oriented ones like name
(note: first letter in lower case):
[{"id":1,"name":"jack","family":"bfd","age":30},{"id":2,"name":"mr john","family":"sobhany","age":36}]
Note ----^^^^^^
JavaScript is a case-sensitive language, so naturally val.Name
is undefined
because there's no Name
property on val
; you want val.name
instead (and so on for id
, family
, bfd
, age
...).
edited Dec 31 '18 at 14:43
answered Dec 31 '18 at 14:25
T.J. CrowderT.J. Crowder
686k12112191311
686k12112191311
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53988311%2fgetjson-method-returns-undefined-in-jquery%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
x7rZdinayztj96fqViyB8zcVIF9bv7
1
What does the actual JSON look like (e.g., in the browser's Network tab)?
– T.J. Crowder
Dec 31 '18 at 14:02
Network tab is empty in my browser @T.J.Crowder
– saeed
Dec 31 '18 at 14:06
Then you need to ensure you perform the request with the network tab open (I guess you're using Edge or some other browser where the network tab only records things when it's open). If you're geting a response at all, clearly a network request is happening, which will show up in the network tab.
– T.J. Crowder
Dec 31 '18 at 14:08
Now i perform it with chrome browser and
Network
tab andResponse
shows value correctly. But showsundefined
in my view. @T.J. Crowder– saeed
Dec 31 '18 at 14:16
1
i edited question and add actual json in network tab @T.J.Crowder
– saeed
Dec 31 '18 at 14:22