In Python, how do I create a string of n characters in one line of code?
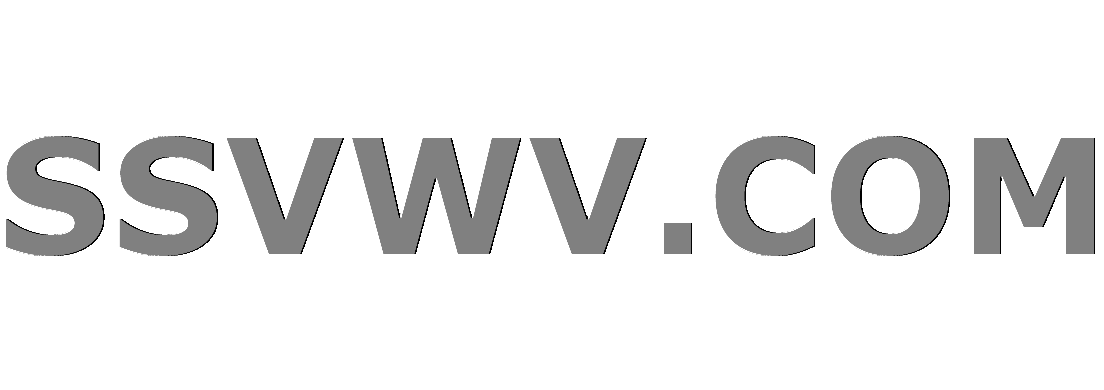
Multi tool use
I need to generate a string with n characters in Python. Is there a one line answer to achieve this with the existing Python library? For instance, I need a string of 10 letters:
string_val = 'abcdefghij'
python string
add a comment |
I need to generate a string with n characters in Python. Is there a one line answer to achieve this with the existing Python library? For instance, I need a string of 10 letters:
string_val = 'abcdefghij'
python string
17
Leave "in one line of code" to code obfuscation contests. When the solution to a problem is naturally written as one line, it will be; otherwise it shouldn't be. Using it as a goal of its own is a guaranteed path to nasty code.
– Glenn Maynard
Sep 14 '09 at 22:32
3
Unless, of course, this is homework. In which case, leave the "in one line of code" but be honest and include the [homework] tag.
– S.Lott
Sep 15 '09 at 0:12
5
It's actually not a homework question, I just needed a string of n length in my test scripts. I forgot that in Python, a char can be multiplied by n where n is a positive integer to achieve what I want.
– Thierry Lam
Sep 15 '09 at 1:08
refer this stackoverflow.com/a/3391106/3779480
– DigviJay Patil
Jun 16 '15 at 11:24
add a comment |
I need to generate a string with n characters in Python. Is there a one line answer to achieve this with the existing Python library? For instance, I need a string of 10 letters:
string_val = 'abcdefghij'
python string
I need to generate a string with n characters in Python. Is there a one line answer to achieve this with the existing Python library? For instance, I need a string of 10 letters:
string_val = 'abcdefghij'
python string
python string
edited May 4 '10 at 0:44
Jon Seigel
10k84786
10k84786
asked Sep 14 '09 at 21:26
Thierry LamThierry Lam
19.4k3396137
19.4k3396137
17
Leave "in one line of code" to code obfuscation contests. When the solution to a problem is naturally written as one line, it will be; otherwise it shouldn't be. Using it as a goal of its own is a guaranteed path to nasty code.
– Glenn Maynard
Sep 14 '09 at 22:32
3
Unless, of course, this is homework. In which case, leave the "in one line of code" but be honest and include the [homework] tag.
– S.Lott
Sep 15 '09 at 0:12
5
It's actually not a homework question, I just needed a string of n length in my test scripts. I forgot that in Python, a char can be multiplied by n where n is a positive integer to achieve what I want.
– Thierry Lam
Sep 15 '09 at 1:08
refer this stackoverflow.com/a/3391106/3779480
– DigviJay Patil
Jun 16 '15 at 11:24
add a comment |
17
Leave "in one line of code" to code obfuscation contests. When the solution to a problem is naturally written as one line, it will be; otherwise it shouldn't be. Using it as a goal of its own is a guaranteed path to nasty code.
– Glenn Maynard
Sep 14 '09 at 22:32
3
Unless, of course, this is homework. In which case, leave the "in one line of code" but be honest and include the [homework] tag.
– S.Lott
Sep 15 '09 at 0:12
5
It's actually not a homework question, I just needed a string of n length in my test scripts. I forgot that in Python, a char can be multiplied by n where n is a positive integer to achieve what I want.
– Thierry Lam
Sep 15 '09 at 1:08
refer this stackoverflow.com/a/3391106/3779480
– DigviJay Patil
Jun 16 '15 at 11:24
17
17
Leave "in one line of code" to code obfuscation contests. When the solution to a problem is naturally written as one line, it will be; otherwise it shouldn't be. Using it as a goal of its own is a guaranteed path to nasty code.
– Glenn Maynard
Sep 14 '09 at 22:32
Leave "in one line of code" to code obfuscation contests. When the solution to a problem is naturally written as one line, it will be; otherwise it shouldn't be. Using it as a goal of its own is a guaranteed path to nasty code.
– Glenn Maynard
Sep 14 '09 at 22:32
3
3
Unless, of course, this is homework. In which case, leave the "in one line of code" but be honest and include the [homework] tag.
– S.Lott
Sep 15 '09 at 0:12
Unless, of course, this is homework. In which case, leave the "in one line of code" but be honest and include the [homework] tag.
– S.Lott
Sep 15 '09 at 0:12
5
5
It's actually not a homework question, I just needed a string of n length in my test scripts. I forgot that in Python, a char can be multiplied by n where n is a positive integer to achieve what I want.
– Thierry Lam
Sep 15 '09 at 1:08
It's actually not a homework question, I just needed a string of n length in my test scripts. I forgot that in Python, a char can be multiplied by n where n is a positive integer to achieve what I want.
– Thierry Lam
Sep 15 '09 at 1:08
refer this stackoverflow.com/a/3391106/3779480
– DigviJay Patil
Jun 16 '15 at 11:24
refer this stackoverflow.com/a/3391106/3779480
– DigviJay Patil
Jun 16 '15 at 11:24
add a comment |
6 Answers
6
active
oldest
votes
To simply repeat the same letter 10 times:
string_val = "x" * 10 # gives you "xxxxxxxxxx"
And if you want something more complex, like n
random lowercase letters, it's still only one line of code (not counting the import statements and defining n
):
from random import choice
from string import ascii_lowercase
n = 10
string_val = "".join(choice(ascii_lowercase) for i in range(n))
add a comment |
The first ten lowercase letters are string.lowercase[:10]
(if you have imported the standard library module string
previously, of course;-).
Other ways to "make a string of 10 characters": 'x'*10
(all the ten characters will be lowercase x
s;-), ''.join(chr(ord('a')+i) for i in xrange(10))
(the first ten lowercase letters again), etc, etc;-).
4
In Python 3.1.1, it's string.ascii_lowercase actually.
– Lasse Vågsæther Karlsen
Sep 14 '09 at 21:31
Yep, python 3 removed.lowercase
(ascii_lowercase
is in recent Python 2's as well as in Python 3).
– Alex Martelli
Sep 14 '09 at 21:37
add a comment |
if you just want any letters:
'a'*10 # gives 'aaaaaaaaaa'
if you want consecutive letters (up to 26):
''.join(['%c' % x for x in range(97, 97+10)]) # gives 'abcdefghij'
add a comment |
Why "one line"? You can fit anything onto one line.
Assuming you want them to start with 'a', and increment by one character each time (with wrapping > 26), here's a line:
>>> mkstring = lambda(x): "".join(map(chr, (ord('a')+(y%26) for y in range(x))))
>>> mkstring(10)
'abcdefghij'
>>> mkstring(30)
'abcdefghijklmnopqrstuvwxyzabcd'
2
You can fit anything into one-line, eh? Quite a claim, re: Python :)
– Gregg Lind
Sep 14 '09 at 21:49
6
Gregg: Python allows semicolons as statement delimiters, so you can put an entire program on one line if desired.
– John Millikin
Sep 14 '09 at 21:58
2
You can't do arbitrary flow control with semicolons though. Nested loops for example.
– recursive
Sep 15 '09 at 0:50
add a comment |
If you can use repeated letters, you can use the *
operator:
>>> 'a'*5
'aaaaa'
add a comment |
This might be a little off the question, but for those interested in the randomness of the generated string, my answer would be:
import os
import string
def _pwd_gen(size=16):
chars = string.letters
chars_len = len(chars)
return str().join(chars[int(ord(c) / 256. * chars_len)] for c in os.urandom(size))
See these answers and random.py
's source for more insight.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f1424005%2fin-python-how-do-i-create-a-string-of-n-characters-in-one-line-of-code%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
To simply repeat the same letter 10 times:
string_val = "x" * 10 # gives you "xxxxxxxxxx"
And if you want something more complex, like n
random lowercase letters, it's still only one line of code (not counting the import statements and defining n
):
from random import choice
from string import ascii_lowercase
n = 10
string_val = "".join(choice(ascii_lowercase) for i in range(n))
add a comment |
To simply repeat the same letter 10 times:
string_val = "x" * 10 # gives you "xxxxxxxxxx"
And if you want something more complex, like n
random lowercase letters, it's still only one line of code (not counting the import statements and defining n
):
from random import choice
from string import ascii_lowercase
n = 10
string_val = "".join(choice(ascii_lowercase) for i in range(n))
add a comment |
To simply repeat the same letter 10 times:
string_val = "x" * 10 # gives you "xxxxxxxxxx"
And if you want something more complex, like n
random lowercase letters, it's still only one line of code (not counting the import statements and defining n
):
from random import choice
from string import ascii_lowercase
n = 10
string_val = "".join(choice(ascii_lowercase) for i in range(n))
To simply repeat the same letter 10 times:
string_val = "x" * 10 # gives you "xxxxxxxxxx"
And if you want something more complex, like n
random lowercase letters, it's still only one line of code (not counting the import statements and defining n
):
from random import choice
from string import ascii_lowercase
n = 10
string_val = "".join(choice(ascii_lowercase) for i in range(n))
edited Jan 1 at 4:25
Community♦
11
11
answered Sep 14 '09 at 21:28
Eli CourtwrightEli Courtwright
121k56196246
121k56196246
add a comment |
add a comment |
The first ten lowercase letters are string.lowercase[:10]
(if you have imported the standard library module string
previously, of course;-).
Other ways to "make a string of 10 characters": 'x'*10
(all the ten characters will be lowercase x
s;-), ''.join(chr(ord('a')+i) for i in xrange(10))
(the first ten lowercase letters again), etc, etc;-).
4
In Python 3.1.1, it's string.ascii_lowercase actually.
– Lasse Vågsæther Karlsen
Sep 14 '09 at 21:31
Yep, python 3 removed.lowercase
(ascii_lowercase
is in recent Python 2's as well as in Python 3).
– Alex Martelli
Sep 14 '09 at 21:37
add a comment |
The first ten lowercase letters are string.lowercase[:10]
(if you have imported the standard library module string
previously, of course;-).
Other ways to "make a string of 10 characters": 'x'*10
(all the ten characters will be lowercase x
s;-), ''.join(chr(ord('a')+i) for i in xrange(10))
(the first ten lowercase letters again), etc, etc;-).
4
In Python 3.1.1, it's string.ascii_lowercase actually.
– Lasse Vågsæther Karlsen
Sep 14 '09 at 21:31
Yep, python 3 removed.lowercase
(ascii_lowercase
is in recent Python 2's as well as in Python 3).
– Alex Martelli
Sep 14 '09 at 21:37
add a comment |
The first ten lowercase letters are string.lowercase[:10]
(if you have imported the standard library module string
previously, of course;-).
Other ways to "make a string of 10 characters": 'x'*10
(all the ten characters will be lowercase x
s;-), ''.join(chr(ord('a')+i) for i in xrange(10))
(the first ten lowercase letters again), etc, etc;-).
The first ten lowercase letters are string.lowercase[:10]
(if you have imported the standard library module string
previously, of course;-).
Other ways to "make a string of 10 characters": 'x'*10
(all the ten characters will be lowercase x
s;-), ''.join(chr(ord('a')+i) for i in xrange(10))
(the first ten lowercase letters again), etc, etc;-).
answered Sep 14 '09 at 21:28
Alex MartelliAlex Martelli
627k12810401280
627k12810401280
4
In Python 3.1.1, it's string.ascii_lowercase actually.
– Lasse Vågsæther Karlsen
Sep 14 '09 at 21:31
Yep, python 3 removed.lowercase
(ascii_lowercase
is in recent Python 2's as well as in Python 3).
– Alex Martelli
Sep 14 '09 at 21:37
add a comment |
4
In Python 3.1.1, it's string.ascii_lowercase actually.
– Lasse Vågsæther Karlsen
Sep 14 '09 at 21:31
Yep, python 3 removed.lowercase
(ascii_lowercase
is in recent Python 2's as well as in Python 3).
– Alex Martelli
Sep 14 '09 at 21:37
4
4
In Python 3.1.1, it's string.ascii_lowercase actually.
– Lasse Vågsæther Karlsen
Sep 14 '09 at 21:31
In Python 3.1.1, it's string.ascii_lowercase actually.
– Lasse Vågsæther Karlsen
Sep 14 '09 at 21:31
Yep, python 3 removed
.lowercase
(ascii_lowercase
is in recent Python 2's as well as in Python 3).– Alex Martelli
Sep 14 '09 at 21:37
Yep, python 3 removed
.lowercase
(ascii_lowercase
is in recent Python 2's as well as in Python 3).– Alex Martelli
Sep 14 '09 at 21:37
add a comment |
if you just want any letters:
'a'*10 # gives 'aaaaaaaaaa'
if you want consecutive letters (up to 26):
''.join(['%c' % x for x in range(97, 97+10)]) # gives 'abcdefghij'
add a comment |
if you just want any letters:
'a'*10 # gives 'aaaaaaaaaa'
if you want consecutive letters (up to 26):
''.join(['%c' % x for x in range(97, 97+10)]) # gives 'abcdefghij'
add a comment |
if you just want any letters:
'a'*10 # gives 'aaaaaaaaaa'
if you want consecutive letters (up to 26):
''.join(['%c' % x for x in range(97, 97+10)]) # gives 'abcdefghij'
if you just want any letters:
'a'*10 # gives 'aaaaaaaaaa'
if you want consecutive letters (up to 26):
''.join(['%c' % x for x in range(97, 97+10)]) # gives 'abcdefghij'
answered Sep 14 '09 at 21:31
PeterPeter
82.7k41157196
82.7k41157196
add a comment |
add a comment |
Why "one line"? You can fit anything onto one line.
Assuming you want them to start with 'a', and increment by one character each time (with wrapping > 26), here's a line:
>>> mkstring = lambda(x): "".join(map(chr, (ord('a')+(y%26) for y in range(x))))
>>> mkstring(10)
'abcdefghij'
>>> mkstring(30)
'abcdefghijklmnopqrstuvwxyzabcd'
2
You can fit anything into one-line, eh? Quite a claim, re: Python :)
– Gregg Lind
Sep 14 '09 at 21:49
6
Gregg: Python allows semicolons as statement delimiters, so you can put an entire program on one line if desired.
– John Millikin
Sep 14 '09 at 21:58
2
You can't do arbitrary flow control with semicolons though. Nested loops for example.
– recursive
Sep 15 '09 at 0:50
add a comment |
Why "one line"? You can fit anything onto one line.
Assuming you want them to start with 'a', and increment by one character each time (with wrapping > 26), here's a line:
>>> mkstring = lambda(x): "".join(map(chr, (ord('a')+(y%26) for y in range(x))))
>>> mkstring(10)
'abcdefghij'
>>> mkstring(30)
'abcdefghijklmnopqrstuvwxyzabcd'
2
You can fit anything into one-line, eh? Quite a claim, re: Python :)
– Gregg Lind
Sep 14 '09 at 21:49
6
Gregg: Python allows semicolons as statement delimiters, so you can put an entire program on one line if desired.
– John Millikin
Sep 14 '09 at 21:58
2
You can't do arbitrary flow control with semicolons though. Nested loops for example.
– recursive
Sep 15 '09 at 0:50
add a comment |
Why "one line"? You can fit anything onto one line.
Assuming you want them to start with 'a', and increment by one character each time (with wrapping > 26), here's a line:
>>> mkstring = lambda(x): "".join(map(chr, (ord('a')+(y%26) for y in range(x))))
>>> mkstring(10)
'abcdefghij'
>>> mkstring(30)
'abcdefghijklmnopqrstuvwxyzabcd'
Why "one line"? You can fit anything onto one line.
Assuming you want them to start with 'a', and increment by one character each time (with wrapping > 26), here's a line:
>>> mkstring = lambda(x): "".join(map(chr, (ord('a')+(y%26) for y in range(x))))
>>> mkstring(10)
'abcdefghij'
>>> mkstring(30)
'abcdefghijklmnopqrstuvwxyzabcd'
answered Sep 14 '09 at 21:30
John MillikinJohn Millikin
156k35190212
156k35190212
2
You can fit anything into one-line, eh? Quite a claim, re: Python :)
– Gregg Lind
Sep 14 '09 at 21:49
6
Gregg: Python allows semicolons as statement delimiters, so you can put an entire program on one line if desired.
– John Millikin
Sep 14 '09 at 21:58
2
You can't do arbitrary flow control with semicolons though. Nested loops for example.
– recursive
Sep 15 '09 at 0:50
add a comment |
2
You can fit anything into one-line, eh? Quite a claim, re: Python :)
– Gregg Lind
Sep 14 '09 at 21:49
6
Gregg: Python allows semicolons as statement delimiters, so you can put an entire program on one line if desired.
– John Millikin
Sep 14 '09 at 21:58
2
You can't do arbitrary flow control with semicolons though. Nested loops for example.
– recursive
Sep 15 '09 at 0:50
2
2
You can fit anything into one-line, eh? Quite a claim, re: Python :)
– Gregg Lind
Sep 14 '09 at 21:49
You can fit anything into one-line, eh? Quite a claim, re: Python :)
– Gregg Lind
Sep 14 '09 at 21:49
6
6
Gregg: Python allows semicolons as statement delimiters, so you can put an entire program on one line if desired.
– John Millikin
Sep 14 '09 at 21:58
Gregg: Python allows semicolons as statement delimiters, so you can put an entire program on one line if desired.
– John Millikin
Sep 14 '09 at 21:58
2
2
You can't do arbitrary flow control with semicolons though. Nested loops for example.
– recursive
Sep 15 '09 at 0:50
You can't do arbitrary flow control with semicolons though. Nested loops for example.
– recursive
Sep 15 '09 at 0:50
add a comment |
If you can use repeated letters, you can use the *
operator:
>>> 'a'*5
'aaaaa'
add a comment |
If you can use repeated letters, you can use the *
operator:
>>> 'a'*5
'aaaaa'
add a comment |
If you can use repeated letters, you can use the *
operator:
>>> 'a'*5
'aaaaa'
If you can use repeated letters, you can use the *
operator:
>>> 'a'*5
'aaaaa'
answered Sep 14 '09 at 21:29
FragsworthFragsworth
12.4k226793
12.4k226793
add a comment |
add a comment |
This might be a little off the question, but for those interested in the randomness of the generated string, my answer would be:
import os
import string
def _pwd_gen(size=16):
chars = string.letters
chars_len = len(chars)
return str().join(chars[int(ord(c) / 256. * chars_len)] for c in os.urandom(size))
See these answers and random.py
's source for more insight.
add a comment |
This might be a little off the question, but for those interested in the randomness of the generated string, my answer would be:
import os
import string
def _pwd_gen(size=16):
chars = string.letters
chars_len = len(chars)
return str().join(chars[int(ord(c) / 256. * chars_len)] for c in os.urandom(size))
See these answers and random.py
's source for more insight.
add a comment |
This might be a little off the question, but for those interested in the randomness of the generated string, my answer would be:
import os
import string
def _pwd_gen(size=16):
chars = string.letters
chars_len = len(chars)
return str().join(chars[int(ord(c) / 256. * chars_len)] for c in os.urandom(size))
See these answers and random.py
's source for more insight.
This might be a little off the question, but for those interested in the randomness of the generated string, my answer would be:
import os
import string
def _pwd_gen(size=16):
chars = string.letters
chars_len = len(chars)
return str().join(chars[int(ord(c) / 256. * chars_len)] for c in os.urandom(size))
See these answers and random.py
's source for more insight.
edited Jan 17 '14 at 18:32
answered Jan 17 '14 at 18:12
foudfoufoudfou
43339
43339
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f1424005%2fin-python-how-do-i-create-a-string-of-n-characters-in-one-line-of-code%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6XqE56ZA87eSUx,iRbv8Z,O
17
Leave "in one line of code" to code obfuscation contests. When the solution to a problem is naturally written as one line, it will be; otherwise it shouldn't be. Using it as a goal of its own is a guaranteed path to nasty code.
– Glenn Maynard
Sep 14 '09 at 22:32
3
Unless, of course, this is homework. In which case, leave the "in one line of code" but be honest and include the [homework] tag.
– S.Lott
Sep 15 '09 at 0:12
5
It's actually not a homework question, I just needed a string of n length in my test scripts. I forgot that in Python, a char can be multiplied by n where n is a positive integer to achieve what I want.
– Thierry Lam
Sep 15 '09 at 1:08
refer this stackoverflow.com/a/3391106/3779480
– DigviJay Patil
Jun 16 '15 at 11:24