How to delete row from db table with 2 primary keys
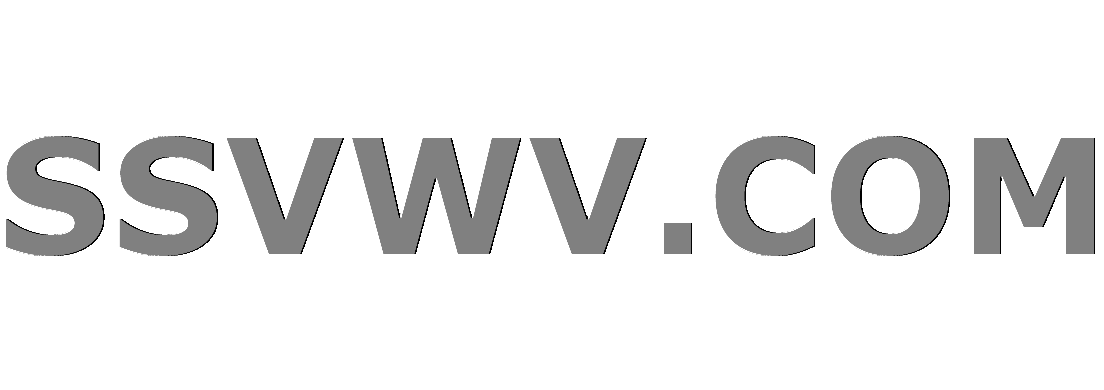
Multi tool use
I'm new using MVC C# on web applications...and I am having troubles deleting a row in the database...the result is an "HTTP Error 400.0 - Bad Request"
I'm not get this error when tables have just 1 primary keys.
Controller:
// GET: DocenteCursoes/Delete/5
public ActionResult Delete(string curso, string docente)
{
if (curso == null && docente == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
DocenteCurso docenteCurso = db.DocenteCurso.Find(curso, docente);
if (docenteCurso == null)
{
return HttpNotFound();
}
return View(docenteCurso);
}
// POST: DocenteCursoes/Delete/5
[HttpPost, ActionName("Delete")]
[ValidateAntiForgeryToken]
public ActionResult DeleteConfirmed(string curso, string docente)
{
DocenteCurso docenteCurso = db.DocenteCurso.Find(curso, docente);
db.DocenteCurso.Remove(docenteCurso);
db.SaveChanges();
return RedirectToAction("Index");
}
protected override void Dispose(bool disposing)
{
if (disposing)
{
db.Dispose();
}
base.Dispose(disposing);
}
Model:
[Table("DocenteCurso")]
public partial class DocenteCurso
{
[Key]
[Column(Order = 0)]
[StringLength(50)]
public string Curso { get; set; }
[Key]
[Column(Order = 1)]
[StringLength(50)]
public string Docente { get; set; }
}
View:
@using (Html.BeginForm()) {
@Html.AntiForgeryToken()
<div class="form-actions no-color">
<input type="submit" value="Delete" class="btn btn-default" /> |
@Html.ActionLink("Back to List", "Index")
</div>
}
c# asp.net-mvc entity-framework
add a comment |
I'm new using MVC C# on web applications...and I am having troubles deleting a row in the database...the result is an "HTTP Error 400.0 - Bad Request"
I'm not get this error when tables have just 1 primary keys.
Controller:
// GET: DocenteCursoes/Delete/5
public ActionResult Delete(string curso, string docente)
{
if (curso == null && docente == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
DocenteCurso docenteCurso = db.DocenteCurso.Find(curso, docente);
if (docenteCurso == null)
{
return HttpNotFound();
}
return View(docenteCurso);
}
// POST: DocenteCursoes/Delete/5
[HttpPost, ActionName("Delete")]
[ValidateAntiForgeryToken]
public ActionResult DeleteConfirmed(string curso, string docente)
{
DocenteCurso docenteCurso = db.DocenteCurso.Find(curso, docente);
db.DocenteCurso.Remove(docenteCurso);
db.SaveChanges();
return RedirectToAction("Index");
}
protected override void Dispose(bool disposing)
{
if (disposing)
{
db.Dispose();
}
base.Dispose(disposing);
}
Model:
[Table("DocenteCurso")]
public partial class DocenteCurso
{
[Key]
[Column(Order = 0)]
[StringLength(50)]
public string Curso { get; set; }
[Key]
[Column(Order = 1)]
[StringLength(50)]
public string Docente { get; set; }
}
View:
@using (Html.BeginForm()) {
@Html.AntiForgeryToken()
<div class="form-actions no-color">
<input type="submit" value="Delete" class="btn btn-default" /> |
@Html.ActionLink("Back to List", "Index")
</div>
}
c# asp.net-mvc entity-framework
1
A table doesn't have two primary keys. A primary key is defined as a single key for the table. A key may be a composite key formed from multiple columns, however.
– Damien_The_Unbeliever
Mar 14 '18 at 8:05
add a comment |
I'm new using MVC C# on web applications...and I am having troubles deleting a row in the database...the result is an "HTTP Error 400.0 - Bad Request"
I'm not get this error when tables have just 1 primary keys.
Controller:
// GET: DocenteCursoes/Delete/5
public ActionResult Delete(string curso, string docente)
{
if (curso == null && docente == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
DocenteCurso docenteCurso = db.DocenteCurso.Find(curso, docente);
if (docenteCurso == null)
{
return HttpNotFound();
}
return View(docenteCurso);
}
// POST: DocenteCursoes/Delete/5
[HttpPost, ActionName("Delete")]
[ValidateAntiForgeryToken]
public ActionResult DeleteConfirmed(string curso, string docente)
{
DocenteCurso docenteCurso = db.DocenteCurso.Find(curso, docente);
db.DocenteCurso.Remove(docenteCurso);
db.SaveChanges();
return RedirectToAction("Index");
}
protected override void Dispose(bool disposing)
{
if (disposing)
{
db.Dispose();
}
base.Dispose(disposing);
}
Model:
[Table("DocenteCurso")]
public partial class DocenteCurso
{
[Key]
[Column(Order = 0)]
[StringLength(50)]
public string Curso { get; set; }
[Key]
[Column(Order = 1)]
[StringLength(50)]
public string Docente { get; set; }
}
View:
@using (Html.BeginForm()) {
@Html.AntiForgeryToken()
<div class="form-actions no-color">
<input type="submit" value="Delete" class="btn btn-default" /> |
@Html.ActionLink("Back to List", "Index")
</div>
}
c# asp.net-mvc entity-framework
I'm new using MVC C# on web applications...and I am having troubles deleting a row in the database...the result is an "HTTP Error 400.0 - Bad Request"
I'm not get this error when tables have just 1 primary keys.
Controller:
// GET: DocenteCursoes/Delete/5
public ActionResult Delete(string curso, string docente)
{
if (curso == null && docente == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
DocenteCurso docenteCurso = db.DocenteCurso.Find(curso, docente);
if (docenteCurso == null)
{
return HttpNotFound();
}
return View(docenteCurso);
}
// POST: DocenteCursoes/Delete/5
[HttpPost, ActionName("Delete")]
[ValidateAntiForgeryToken]
public ActionResult DeleteConfirmed(string curso, string docente)
{
DocenteCurso docenteCurso = db.DocenteCurso.Find(curso, docente);
db.DocenteCurso.Remove(docenteCurso);
db.SaveChanges();
return RedirectToAction("Index");
}
protected override void Dispose(bool disposing)
{
if (disposing)
{
db.Dispose();
}
base.Dispose(disposing);
}
Model:
[Table("DocenteCurso")]
public partial class DocenteCurso
{
[Key]
[Column(Order = 0)]
[StringLength(50)]
public string Curso { get; set; }
[Key]
[Column(Order = 1)]
[StringLength(50)]
public string Docente { get; set; }
}
View:
@using (Html.BeginForm()) {
@Html.AntiForgeryToken()
<div class="form-actions no-color">
<input type="submit" value="Delete" class="btn btn-default" /> |
@Html.ActionLink("Back to List", "Index")
</div>
}
c# asp.net-mvc entity-framework
c# asp.net-mvc entity-framework
edited Mar 13 '18 at 23:06
user3559349
asked Mar 13 '18 at 20:28


Luis AcuñaLuis Acuña
1013
1013
1
A table doesn't have two primary keys. A primary key is defined as a single key for the table. A key may be a composite key formed from multiple columns, however.
– Damien_The_Unbeliever
Mar 14 '18 at 8:05
add a comment |
1
A table doesn't have two primary keys. A primary key is defined as a single key for the table. A key may be a composite key formed from multiple columns, however.
– Damien_The_Unbeliever
Mar 14 '18 at 8:05
1
1
A table doesn't have two primary keys. A primary key is defined as a single key for the table. A key may be a composite key formed from multiple columns, however.
– Damien_The_Unbeliever
Mar 14 '18 at 8:05
A table doesn't have two primary keys. A primary key is defined as a single key for the table. A key may be a composite key formed from multiple columns, however.
– Damien_The_Unbeliever
Mar 14 '18 at 8:05
add a comment |
3 Answers
3
active
oldest
votes
Most probably you are getting BadRequest
due to this condition:
if (curso == null && docente == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Which has nothing to do with whatever or not your entity has a composite primary key.
Make sure you are calling a correct controller action.
Note that Html.BeginForm()
with no parameters, sends an HTTP POST to the current URL.
add a comment |
Ok, let's start with a well-constructed URL like this :
http://yourserver/DocenteCursoes/Delete?curso=firstparam&docente=secondparam
Try that in an Internet browser first, and tell me if you could get rid of the 404 error.
add a comment |
View:
@using (Html.BeginForm(null, null, new { curso = Model.Curso, docente = Model.Docente }))
{
@Html.AntiForgeryToken()
<div class="form-actions no-color">
<input type="submit" value="Delete" class="btn btn-default" /> |
@Html.ActionLink("Back to List", "Index")
</div>
}
Can you provide more information about how this answers the question.
– Underverse
Jan 1 at 3:53
When you use Composite key in ASP.NET MVC Model, you need to define routeValues object in Html.BeginForm().
– Mohammed Ibraheem
Jan 1 at 4:03
Html.BeginForm(string actionName, string controllerName, RouteValueDictionary routeValues);
– Mohammed Ibraheem
Jan 1 at 4:07
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49265327%2fhow-to-delete-row-from-db-table-with-2-primary-keys%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Most probably you are getting BadRequest
due to this condition:
if (curso == null && docente == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Which has nothing to do with whatever or not your entity has a composite primary key.
Make sure you are calling a correct controller action.
Note that Html.BeginForm()
with no parameters, sends an HTTP POST to the current URL.
add a comment |
Most probably you are getting BadRequest
due to this condition:
if (curso == null && docente == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Which has nothing to do with whatever or not your entity has a composite primary key.
Make sure you are calling a correct controller action.
Note that Html.BeginForm()
with no parameters, sends an HTTP POST to the current URL.
add a comment |
Most probably you are getting BadRequest
due to this condition:
if (curso == null && docente == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Which has nothing to do with whatever or not your entity has a composite primary key.
Make sure you are calling a correct controller action.
Note that Html.BeginForm()
with no parameters, sends an HTTP POST to the current URL.
Most probably you are getting BadRequest
due to this condition:
if (curso == null && docente == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Which has nothing to do with whatever or not your entity has a composite primary key.
Make sure you are calling a correct controller action.
Note that Html.BeginForm()
with no parameters, sends an HTTP POST to the current URL.
edited Mar 13 '18 at 20:41
answered Mar 13 '18 at 20:33
koryakinpkoryakinp
1,64921138
1,64921138
add a comment |
add a comment |
Ok, let's start with a well-constructed URL like this :
http://yourserver/DocenteCursoes/Delete?curso=firstparam&docente=secondparam
Try that in an Internet browser first, and tell me if you could get rid of the 404 error.
add a comment |
Ok, let's start with a well-constructed URL like this :
http://yourserver/DocenteCursoes/Delete?curso=firstparam&docente=secondparam
Try that in an Internet browser first, and tell me if you could get rid of the 404 error.
add a comment |
Ok, let's start with a well-constructed URL like this :
http://yourserver/DocenteCursoes/Delete?curso=firstparam&docente=secondparam
Try that in an Internet browser first, and tell me if you could get rid of the 404 error.
Ok, let's start with a well-constructed URL like this :
http://yourserver/DocenteCursoes/Delete?curso=firstparam&docente=secondparam
Try that in an Internet browser first, and tell me if you could get rid of the 404 error.
answered Mar 14 '18 at 7:51


Erlangga Hasto HandokoErlangga Hasto Handoko
27228
27228
add a comment |
add a comment |
View:
@using (Html.BeginForm(null, null, new { curso = Model.Curso, docente = Model.Docente }))
{
@Html.AntiForgeryToken()
<div class="form-actions no-color">
<input type="submit" value="Delete" class="btn btn-default" /> |
@Html.ActionLink("Back to List", "Index")
</div>
}
Can you provide more information about how this answers the question.
– Underverse
Jan 1 at 3:53
When you use Composite key in ASP.NET MVC Model, you need to define routeValues object in Html.BeginForm().
– Mohammed Ibraheem
Jan 1 at 4:03
Html.BeginForm(string actionName, string controllerName, RouteValueDictionary routeValues);
– Mohammed Ibraheem
Jan 1 at 4:07
add a comment |
View:
@using (Html.BeginForm(null, null, new { curso = Model.Curso, docente = Model.Docente }))
{
@Html.AntiForgeryToken()
<div class="form-actions no-color">
<input type="submit" value="Delete" class="btn btn-default" /> |
@Html.ActionLink("Back to List", "Index")
</div>
}
Can you provide more information about how this answers the question.
– Underverse
Jan 1 at 3:53
When you use Composite key in ASP.NET MVC Model, you need to define routeValues object in Html.BeginForm().
– Mohammed Ibraheem
Jan 1 at 4:03
Html.BeginForm(string actionName, string controllerName, RouteValueDictionary routeValues);
– Mohammed Ibraheem
Jan 1 at 4:07
add a comment |
View:
@using (Html.BeginForm(null, null, new { curso = Model.Curso, docente = Model.Docente }))
{
@Html.AntiForgeryToken()
<div class="form-actions no-color">
<input type="submit" value="Delete" class="btn btn-default" /> |
@Html.ActionLink("Back to List", "Index")
</div>
}
View:
@using (Html.BeginForm(null, null, new { curso = Model.Curso, docente = Model.Docente }))
{
@Html.AntiForgeryToken()
<div class="form-actions no-color">
<input type="submit" value="Delete" class="btn btn-default" /> |
@Html.ActionLink("Back to List", "Index")
</div>
}
answered Jan 1 at 3:46
Mohammed IbraheemMohammed Ibraheem
162
162
Can you provide more information about how this answers the question.
– Underverse
Jan 1 at 3:53
When you use Composite key in ASP.NET MVC Model, you need to define routeValues object in Html.BeginForm().
– Mohammed Ibraheem
Jan 1 at 4:03
Html.BeginForm(string actionName, string controllerName, RouteValueDictionary routeValues);
– Mohammed Ibraheem
Jan 1 at 4:07
add a comment |
Can you provide more information about how this answers the question.
– Underverse
Jan 1 at 3:53
When you use Composite key in ASP.NET MVC Model, you need to define routeValues object in Html.BeginForm().
– Mohammed Ibraheem
Jan 1 at 4:03
Html.BeginForm(string actionName, string controllerName, RouteValueDictionary routeValues);
– Mohammed Ibraheem
Jan 1 at 4:07
Can you provide more information about how this answers the question.
– Underverse
Jan 1 at 3:53
Can you provide more information about how this answers the question.
– Underverse
Jan 1 at 3:53
When you use Composite key in ASP.NET MVC Model, you need to define routeValues object in Html.BeginForm().
– Mohammed Ibraheem
Jan 1 at 4:03
When you use Composite key in ASP.NET MVC Model, you need to define routeValues object in Html.BeginForm().
– Mohammed Ibraheem
Jan 1 at 4:03
Html.BeginForm(string actionName, string controllerName, RouteValueDictionary routeValues);
– Mohammed Ibraheem
Jan 1 at 4:07
Html.BeginForm(string actionName, string controllerName, RouteValueDictionary routeValues);
– Mohammed Ibraheem
Jan 1 at 4:07
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49265327%2fhow-to-delete-row-from-db-table-with-2-primary-keys%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Eld5JUjWbBD2MC87dOWAn7aRhR6tfca L 49M,CE
1
A table doesn't have two primary keys. A primary key is defined as a single key for the table. A key may be a composite key formed from multiple columns, however.
– Damien_The_Unbeliever
Mar 14 '18 at 8:05