Rails not saving entry after clicking submit
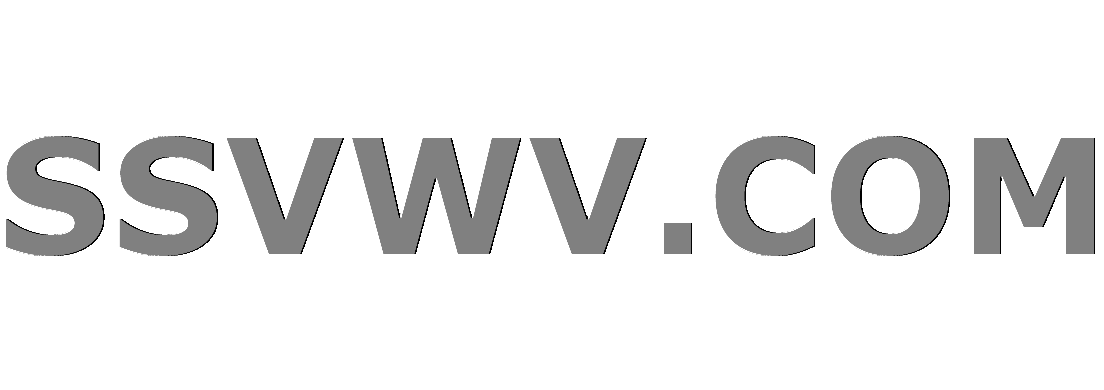
Multi tool use
My app worked perfectly fine before installing the Devise gem. After installing Devise, when I click submit on the form it reloads the same page and doesn't save the entry into the table. It should redirect to the 'ask' page where it asks the user if there are more entries. I have tried multiple solutions from this site but none of them seem to work so I wanted to post this question to get it to work and an understanding of what is going on. The naming might get confusing. My first model is Members, with fields input, gifttext, spouse, and spousegift. After installing Devise I put a users model with the fields email and encrypted_password.
My members_controller.rb:
class MembersController < ApplicationController
include MembersHelper
def new
@member = Member.new
end
def index
@members = Member.all
end
def destroy
@member = Member.find(params[:id])
@members = Member.destroy(params[:id])
redirect_to members_path
end
def create
@member = Member.new(member_params)
if @member.save
redirect_to :action => 'ask'
else
render :action => 'new'
end
end
def ask
end
def show
end
def update
@article = Member.find(params[:id])
if @member.update(member_params)
redirect_to @member
else
render 'edit'
end
end
def results
@matches = prints
end
def member_params
params.require(:member).permit(:input, :gifttext, :spouse, :spousegift)
end
end
My application controller:
class ApplicationController < ActionController::Base
before_action :authenticate_user!
end
My member.rb:
class Member < ApplicationRecord
belongs_to :user
end
My user.rb
class User < ApplicationRecord
# Include default devise modules. Others available are:
# :confirmable, :lockable, :timeoutable, :trackable and :omniauthable
devise :database_authenticatable, :registerable,
:recoverable, :rememberable, :validatable
end
My _form.html.erb:
<%= form_with(model: @member, local: true ) do |f| %>
<p>Who is the participant?</p>
<div class="field">
<%= f.label :person %>
<%= f.text_field :input %>
</div>
<p>What gifts would they like?</p>
<div class="field">
<%= f.label :gifts %>
<%= f.text_field :gifttext %>
</div>
<p>Who, if anyone, is their spouse? If they don't have one you can leave this field blank</p>
<div class="field">
<%= f.label :spouse %>
<%= f.text_field :spouse %>
</div>
<p>What gifts would they like? If there is no spouse then you can leave this field blank.</p>
<div class="field">
<%= f.label :gifts %>
<%= f.text_field :spousegift %>
</div>
<div class="action">
<%= f.submit "Submit" %>
</div>
<%= link_to 'Matches', members_results_path(@member) %>
<% end %>
My routes.rb:
Rails.application.routes.draw do
devise_for :users
get 'members/new'
get 'members/results'
get 'members/index'
get 'names/new'
get 'names/results'
get 'names/index'
root 'members#new'
post 'members/new'
get 'members/ask'
resources :members
# For details on the DSL available within this file, see http://guides.rubyonrails.org/routing.html
end
My migration for the users model:
# frozen_string_literal: true
class AddDeviseToUsers < ActiveRecord::Migration[5.2]
def self.up
change_table :users do |t|
## Database authenticatable
t.string :email, null: false, default: ""
t.string :encrypted_password, null: false, default: ""
## Recoverable
t.string :reset_password_token
t.datetime :reset_password_sent_at
## Rememberable
t.datetime :remember_created_at
## Trackable
# t.integer :sign_in_count, default: 0, null: false
# t.datetime :current_sign_in_at
# t.datetime :last_sign_in_at
# t.inet :current_sign_in_ip
# t.inet :last_sign_in_ip
## Confirmable
# t.string :confirmation_token
# t.datetime :confirmed_at
# t.datetime :confirmation_sent_at
# t.string :unconfirmed_email # Only if using reconfirmable
## Lockable
# t.integer :failed_attempts, default: 0, null: false # Only if lock strategy is :failed_attempts
# t.string :unlock_token # Only if unlock strategy is :email or :both
# t.datetime :locked_at
# Uncomment below if timestamps were not included in your original model.
# t.timestamps null: false
end
add_index :users, :email, unique: true
add_index :users, :reset_password_token, unique: true
# add_index :users, :confirmation_token, unique: true
# add_index :users, :unlock_token, unique: true
end
def self.down
# By default, we don't want to make any assumption about how to roll back a migration when your
# model already existed. Please edit below which fields you would like to remove in this migration.
raise ActiveRecord::IrreversibleMigration
end
end
My Members migration:
class CreateMembers < ActiveRecord::Migration[5.2]
def change
create_table :members do |t|
t.string :input
t.string :gifttext
t.string :spouse
t.string :spousegift
t.timestamps
end
end
end
Let me know if you need any additional info.
ruby-on-rails devise
add a comment |
My app worked perfectly fine before installing the Devise gem. After installing Devise, when I click submit on the form it reloads the same page and doesn't save the entry into the table. It should redirect to the 'ask' page where it asks the user if there are more entries. I have tried multiple solutions from this site but none of them seem to work so I wanted to post this question to get it to work and an understanding of what is going on. The naming might get confusing. My first model is Members, with fields input, gifttext, spouse, and spousegift. After installing Devise I put a users model with the fields email and encrypted_password.
My members_controller.rb:
class MembersController < ApplicationController
include MembersHelper
def new
@member = Member.new
end
def index
@members = Member.all
end
def destroy
@member = Member.find(params[:id])
@members = Member.destroy(params[:id])
redirect_to members_path
end
def create
@member = Member.new(member_params)
if @member.save
redirect_to :action => 'ask'
else
render :action => 'new'
end
end
def ask
end
def show
end
def update
@article = Member.find(params[:id])
if @member.update(member_params)
redirect_to @member
else
render 'edit'
end
end
def results
@matches = prints
end
def member_params
params.require(:member).permit(:input, :gifttext, :spouse, :spousegift)
end
end
My application controller:
class ApplicationController < ActionController::Base
before_action :authenticate_user!
end
My member.rb:
class Member < ApplicationRecord
belongs_to :user
end
My user.rb
class User < ApplicationRecord
# Include default devise modules. Others available are:
# :confirmable, :lockable, :timeoutable, :trackable and :omniauthable
devise :database_authenticatable, :registerable,
:recoverable, :rememberable, :validatable
end
My _form.html.erb:
<%= form_with(model: @member, local: true ) do |f| %>
<p>Who is the participant?</p>
<div class="field">
<%= f.label :person %>
<%= f.text_field :input %>
</div>
<p>What gifts would they like?</p>
<div class="field">
<%= f.label :gifts %>
<%= f.text_field :gifttext %>
</div>
<p>Who, if anyone, is their spouse? If they don't have one you can leave this field blank</p>
<div class="field">
<%= f.label :spouse %>
<%= f.text_field :spouse %>
</div>
<p>What gifts would they like? If there is no spouse then you can leave this field blank.</p>
<div class="field">
<%= f.label :gifts %>
<%= f.text_field :spousegift %>
</div>
<div class="action">
<%= f.submit "Submit" %>
</div>
<%= link_to 'Matches', members_results_path(@member) %>
<% end %>
My routes.rb:
Rails.application.routes.draw do
devise_for :users
get 'members/new'
get 'members/results'
get 'members/index'
get 'names/new'
get 'names/results'
get 'names/index'
root 'members#new'
post 'members/new'
get 'members/ask'
resources :members
# For details on the DSL available within this file, see http://guides.rubyonrails.org/routing.html
end
My migration for the users model:
# frozen_string_literal: true
class AddDeviseToUsers < ActiveRecord::Migration[5.2]
def self.up
change_table :users do |t|
## Database authenticatable
t.string :email, null: false, default: ""
t.string :encrypted_password, null: false, default: ""
## Recoverable
t.string :reset_password_token
t.datetime :reset_password_sent_at
## Rememberable
t.datetime :remember_created_at
## Trackable
# t.integer :sign_in_count, default: 0, null: false
# t.datetime :current_sign_in_at
# t.datetime :last_sign_in_at
# t.inet :current_sign_in_ip
# t.inet :last_sign_in_ip
## Confirmable
# t.string :confirmation_token
# t.datetime :confirmed_at
# t.datetime :confirmation_sent_at
# t.string :unconfirmed_email # Only if using reconfirmable
## Lockable
# t.integer :failed_attempts, default: 0, null: false # Only if lock strategy is :failed_attempts
# t.string :unlock_token # Only if unlock strategy is :email or :both
# t.datetime :locked_at
# Uncomment below if timestamps were not included in your original model.
# t.timestamps null: false
end
add_index :users, :email, unique: true
add_index :users, :reset_password_token, unique: true
# add_index :users, :confirmation_token, unique: true
# add_index :users, :unlock_token, unique: true
end
def self.down
# By default, we don't want to make any assumption about how to roll back a migration when your
# model already existed. Please edit below which fields you would like to remove in this migration.
raise ActiveRecord::IrreversibleMigration
end
end
My Members migration:
class CreateMembers < ActiveRecord::Migration[5.2]
def change
create_table :members do |t|
t.string :input
t.string :gifttext
t.string :spouse
t.string :spousegift
t.timestamps
end
end
end
Let me know if you need any additional info.
ruby-on-rails devise
1
Probably@member
record is invalid. Increate
method, usesave!
instead ofsave
. It will raise an exception (if the record is invalid) that might help (please add it to your question)
– MrShemek
Dec 29 '18 at 16:13
@article = Member.find(params[:id])
should be@member = Member.find(params[:id])
instead
– Ana María Martínez Gómez
Dec 29 '18 at 18:32
It seems to me as you want to authorize member instead of user (it is an instance of it but the name of the class is different)
– Ana María Martínez Gómez
Dec 29 '18 at 18:34
add a comment |
My app worked perfectly fine before installing the Devise gem. After installing Devise, when I click submit on the form it reloads the same page and doesn't save the entry into the table. It should redirect to the 'ask' page where it asks the user if there are more entries. I have tried multiple solutions from this site but none of them seem to work so I wanted to post this question to get it to work and an understanding of what is going on. The naming might get confusing. My first model is Members, with fields input, gifttext, spouse, and spousegift. After installing Devise I put a users model with the fields email and encrypted_password.
My members_controller.rb:
class MembersController < ApplicationController
include MembersHelper
def new
@member = Member.new
end
def index
@members = Member.all
end
def destroy
@member = Member.find(params[:id])
@members = Member.destroy(params[:id])
redirect_to members_path
end
def create
@member = Member.new(member_params)
if @member.save
redirect_to :action => 'ask'
else
render :action => 'new'
end
end
def ask
end
def show
end
def update
@article = Member.find(params[:id])
if @member.update(member_params)
redirect_to @member
else
render 'edit'
end
end
def results
@matches = prints
end
def member_params
params.require(:member).permit(:input, :gifttext, :spouse, :spousegift)
end
end
My application controller:
class ApplicationController < ActionController::Base
before_action :authenticate_user!
end
My member.rb:
class Member < ApplicationRecord
belongs_to :user
end
My user.rb
class User < ApplicationRecord
# Include default devise modules. Others available are:
# :confirmable, :lockable, :timeoutable, :trackable and :omniauthable
devise :database_authenticatable, :registerable,
:recoverable, :rememberable, :validatable
end
My _form.html.erb:
<%= form_with(model: @member, local: true ) do |f| %>
<p>Who is the participant?</p>
<div class="field">
<%= f.label :person %>
<%= f.text_field :input %>
</div>
<p>What gifts would they like?</p>
<div class="field">
<%= f.label :gifts %>
<%= f.text_field :gifttext %>
</div>
<p>Who, if anyone, is their spouse? If they don't have one you can leave this field blank</p>
<div class="field">
<%= f.label :spouse %>
<%= f.text_field :spouse %>
</div>
<p>What gifts would they like? If there is no spouse then you can leave this field blank.</p>
<div class="field">
<%= f.label :gifts %>
<%= f.text_field :spousegift %>
</div>
<div class="action">
<%= f.submit "Submit" %>
</div>
<%= link_to 'Matches', members_results_path(@member) %>
<% end %>
My routes.rb:
Rails.application.routes.draw do
devise_for :users
get 'members/new'
get 'members/results'
get 'members/index'
get 'names/new'
get 'names/results'
get 'names/index'
root 'members#new'
post 'members/new'
get 'members/ask'
resources :members
# For details on the DSL available within this file, see http://guides.rubyonrails.org/routing.html
end
My migration for the users model:
# frozen_string_literal: true
class AddDeviseToUsers < ActiveRecord::Migration[5.2]
def self.up
change_table :users do |t|
## Database authenticatable
t.string :email, null: false, default: ""
t.string :encrypted_password, null: false, default: ""
## Recoverable
t.string :reset_password_token
t.datetime :reset_password_sent_at
## Rememberable
t.datetime :remember_created_at
## Trackable
# t.integer :sign_in_count, default: 0, null: false
# t.datetime :current_sign_in_at
# t.datetime :last_sign_in_at
# t.inet :current_sign_in_ip
# t.inet :last_sign_in_ip
## Confirmable
# t.string :confirmation_token
# t.datetime :confirmed_at
# t.datetime :confirmation_sent_at
# t.string :unconfirmed_email # Only if using reconfirmable
## Lockable
# t.integer :failed_attempts, default: 0, null: false # Only if lock strategy is :failed_attempts
# t.string :unlock_token # Only if unlock strategy is :email or :both
# t.datetime :locked_at
# Uncomment below if timestamps were not included in your original model.
# t.timestamps null: false
end
add_index :users, :email, unique: true
add_index :users, :reset_password_token, unique: true
# add_index :users, :confirmation_token, unique: true
# add_index :users, :unlock_token, unique: true
end
def self.down
# By default, we don't want to make any assumption about how to roll back a migration when your
# model already existed. Please edit below which fields you would like to remove in this migration.
raise ActiveRecord::IrreversibleMigration
end
end
My Members migration:
class CreateMembers < ActiveRecord::Migration[5.2]
def change
create_table :members do |t|
t.string :input
t.string :gifttext
t.string :spouse
t.string :spousegift
t.timestamps
end
end
end
Let me know if you need any additional info.
ruby-on-rails devise
My app worked perfectly fine before installing the Devise gem. After installing Devise, when I click submit on the form it reloads the same page and doesn't save the entry into the table. It should redirect to the 'ask' page where it asks the user if there are more entries. I have tried multiple solutions from this site but none of them seem to work so I wanted to post this question to get it to work and an understanding of what is going on. The naming might get confusing. My first model is Members, with fields input, gifttext, spouse, and spousegift. After installing Devise I put a users model with the fields email and encrypted_password.
My members_controller.rb:
class MembersController < ApplicationController
include MembersHelper
def new
@member = Member.new
end
def index
@members = Member.all
end
def destroy
@member = Member.find(params[:id])
@members = Member.destroy(params[:id])
redirect_to members_path
end
def create
@member = Member.new(member_params)
if @member.save
redirect_to :action => 'ask'
else
render :action => 'new'
end
end
def ask
end
def show
end
def update
@article = Member.find(params[:id])
if @member.update(member_params)
redirect_to @member
else
render 'edit'
end
end
def results
@matches = prints
end
def member_params
params.require(:member).permit(:input, :gifttext, :spouse, :spousegift)
end
end
My application controller:
class ApplicationController < ActionController::Base
before_action :authenticate_user!
end
My member.rb:
class Member < ApplicationRecord
belongs_to :user
end
My user.rb
class User < ApplicationRecord
# Include default devise modules. Others available are:
# :confirmable, :lockable, :timeoutable, :trackable and :omniauthable
devise :database_authenticatable, :registerable,
:recoverable, :rememberable, :validatable
end
My _form.html.erb:
<%= form_with(model: @member, local: true ) do |f| %>
<p>Who is the participant?</p>
<div class="field">
<%= f.label :person %>
<%= f.text_field :input %>
</div>
<p>What gifts would they like?</p>
<div class="field">
<%= f.label :gifts %>
<%= f.text_field :gifttext %>
</div>
<p>Who, if anyone, is their spouse? If they don't have one you can leave this field blank</p>
<div class="field">
<%= f.label :spouse %>
<%= f.text_field :spouse %>
</div>
<p>What gifts would they like? If there is no spouse then you can leave this field blank.</p>
<div class="field">
<%= f.label :gifts %>
<%= f.text_field :spousegift %>
</div>
<div class="action">
<%= f.submit "Submit" %>
</div>
<%= link_to 'Matches', members_results_path(@member) %>
<% end %>
My routes.rb:
Rails.application.routes.draw do
devise_for :users
get 'members/new'
get 'members/results'
get 'members/index'
get 'names/new'
get 'names/results'
get 'names/index'
root 'members#new'
post 'members/new'
get 'members/ask'
resources :members
# For details on the DSL available within this file, see http://guides.rubyonrails.org/routing.html
end
My migration for the users model:
# frozen_string_literal: true
class AddDeviseToUsers < ActiveRecord::Migration[5.2]
def self.up
change_table :users do |t|
## Database authenticatable
t.string :email, null: false, default: ""
t.string :encrypted_password, null: false, default: ""
## Recoverable
t.string :reset_password_token
t.datetime :reset_password_sent_at
## Rememberable
t.datetime :remember_created_at
## Trackable
# t.integer :sign_in_count, default: 0, null: false
# t.datetime :current_sign_in_at
# t.datetime :last_sign_in_at
# t.inet :current_sign_in_ip
# t.inet :last_sign_in_ip
## Confirmable
# t.string :confirmation_token
# t.datetime :confirmed_at
# t.datetime :confirmation_sent_at
# t.string :unconfirmed_email # Only if using reconfirmable
## Lockable
# t.integer :failed_attempts, default: 0, null: false # Only if lock strategy is :failed_attempts
# t.string :unlock_token # Only if unlock strategy is :email or :both
# t.datetime :locked_at
# Uncomment below if timestamps were not included in your original model.
# t.timestamps null: false
end
add_index :users, :email, unique: true
add_index :users, :reset_password_token, unique: true
# add_index :users, :confirmation_token, unique: true
# add_index :users, :unlock_token, unique: true
end
def self.down
# By default, we don't want to make any assumption about how to roll back a migration when your
# model already existed. Please edit below which fields you would like to remove in this migration.
raise ActiveRecord::IrreversibleMigration
end
end
My Members migration:
class CreateMembers < ActiveRecord::Migration[5.2]
def change
create_table :members do |t|
t.string :input
t.string :gifttext
t.string :spouse
t.string :spousegift
t.timestamps
end
end
end
Let me know if you need any additional info.
ruby-on-rails devise
ruby-on-rails devise
edited Jan 2 at 0:56
Alex
asked Dec 29 '18 at 16:10


AlexAlex
23
23
1
Probably@member
record is invalid. Increate
method, usesave!
instead ofsave
. It will raise an exception (if the record is invalid) that might help (please add it to your question)
– MrShemek
Dec 29 '18 at 16:13
@article = Member.find(params[:id])
should be@member = Member.find(params[:id])
instead
– Ana María Martínez Gómez
Dec 29 '18 at 18:32
It seems to me as you want to authorize member instead of user (it is an instance of it but the name of the class is different)
– Ana María Martínez Gómez
Dec 29 '18 at 18:34
add a comment |
1
Probably@member
record is invalid. Increate
method, usesave!
instead ofsave
. It will raise an exception (if the record is invalid) that might help (please add it to your question)
– MrShemek
Dec 29 '18 at 16:13
@article = Member.find(params[:id])
should be@member = Member.find(params[:id])
instead
– Ana María Martínez Gómez
Dec 29 '18 at 18:32
It seems to me as you want to authorize member instead of user (it is an instance of it but the name of the class is different)
– Ana María Martínez Gómez
Dec 29 '18 at 18:34
1
1
Probably
@member
record is invalid. In create
method, use save!
instead of save
. It will raise an exception (if the record is invalid) that might help (please add it to your question)– MrShemek
Dec 29 '18 at 16:13
Probably
@member
record is invalid. In create
method, use save!
instead of save
. It will raise an exception (if the record is invalid) that might help (please add it to your question)– MrShemek
Dec 29 '18 at 16:13
@article = Member.find(params[:id])
should be @member = Member.find(params[:id])
instead– Ana María Martínez Gómez
Dec 29 '18 at 18:32
@article = Member.find(params[:id])
should be @member = Member.find(params[:id])
instead– Ana María Martínez Gómez
Dec 29 '18 at 18:32
It seems to me as you want to authorize member instead of user (it is an instance of it but the name of the class is different)
– Ana María Martínez Gómez
Dec 29 '18 at 18:34
It seems to me as you want to authorize member instead of user (it is an instance of it but the name of the class is different)
– Ana María Martínez Gómez
Dec 29 '18 at 18:34
add a comment |
1 Answer
1
active
oldest
votes
Your MembersController#update method uses a @article
instance variable. You probably meant @member
:
def update
@member = Member.find(params[:id])
if @member.update(member_params)
redirect_to @member
else
render 'edit'
end
end
Your create action doesn't look quite right either you need to set the user and redirect correctly:
def create
@member = Member.new(member_params)
@member.user = current_user
if @member.save
redirect_to ask_member_path(@member)
else
render 'new'
end
end
_form.html.erb
<%= @member.errors.inspect %>
I made these changes but unfortunately, it still does not save the data. Any more suggestions?
– Alex
Dec 30 '18 at 22:31
You need to see the@member.errors
in the form, so you can see why it is not being saved. My wildest guess is, that since it belongs to a user you need to assign the current_user. I made the change in my comment above.
– eikes
Dec 31 '18 at 15:13
I'm getting this on the form page after putting <%= @member.errors.inspect %>: #<ActiveModel::Errors:0x000000000c6ebcf0 @base=#<Member id: nil, input: nil, gifttext: nil, spouse: nil, spousegift: nil, created_at: nil, updated_at: nil>, @messages={:person=>, :input=>, :gifts=>, :gifttext=>, :spouse=>, :spousegift=>}, @details={}>. And when I make the changes to the create action I get this error: ActiveModel::MissingAttributeError in MembersController#create can't write unknown attributeuser_id
– Alex
Dec 31 '18 at 17:03
what does your members table look like? It should have a user_id column when you are usingbelongs_to :user
– eikes
Jan 1 at 21:39
This may actually be the case. I edited the question to show when I added the Members migration for when I created the table so you can see the columns. How would I go about adding a user_id column after the table is already created? Hope this fixes it.
– Alex
Jan 2 at 0:58
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53971161%2frails-not-saving-entry-after-clicking-submit%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your MembersController#update method uses a @article
instance variable. You probably meant @member
:
def update
@member = Member.find(params[:id])
if @member.update(member_params)
redirect_to @member
else
render 'edit'
end
end
Your create action doesn't look quite right either you need to set the user and redirect correctly:
def create
@member = Member.new(member_params)
@member.user = current_user
if @member.save
redirect_to ask_member_path(@member)
else
render 'new'
end
end
_form.html.erb
<%= @member.errors.inspect %>
I made these changes but unfortunately, it still does not save the data. Any more suggestions?
– Alex
Dec 30 '18 at 22:31
You need to see the@member.errors
in the form, so you can see why it is not being saved. My wildest guess is, that since it belongs to a user you need to assign the current_user. I made the change in my comment above.
– eikes
Dec 31 '18 at 15:13
I'm getting this on the form page after putting <%= @member.errors.inspect %>: #<ActiveModel::Errors:0x000000000c6ebcf0 @base=#<Member id: nil, input: nil, gifttext: nil, spouse: nil, spousegift: nil, created_at: nil, updated_at: nil>, @messages={:person=>, :input=>, :gifts=>, :gifttext=>, :spouse=>, :spousegift=>}, @details={}>. And when I make the changes to the create action I get this error: ActiveModel::MissingAttributeError in MembersController#create can't write unknown attributeuser_id
– Alex
Dec 31 '18 at 17:03
what does your members table look like? It should have a user_id column when you are usingbelongs_to :user
– eikes
Jan 1 at 21:39
This may actually be the case. I edited the question to show when I added the Members migration for when I created the table so you can see the columns. How would I go about adding a user_id column after the table is already created? Hope this fixes it.
– Alex
Jan 2 at 0:58
|
show 1 more comment
Your MembersController#update method uses a @article
instance variable. You probably meant @member
:
def update
@member = Member.find(params[:id])
if @member.update(member_params)
redirect_to @member
else
render 'edit'
end
end
Your create action doesn't look quite right either you need to set the user and redirect correctly:
def create
@member = Member.new(member_params)
@member.user = current_user
if @member.save
redirect_to ask_member_path(@member)
else
render 'new'
end
end
_form.html.erb
<%= @member.errors.inspect %>
I made these changes but unfortunately, it still does not save the data. Any more suggestions?
– Alex
Dec 30 '18 at 22:31
You need to see the@member.errors
in the form, so you can see why it is not being saved. My wildest guess is, that since it belongs to a user you need to assign the current_user. I made the change in my comment above.
– eikes
Dec 31 '18 at 15:13
I'm getting this on the form page after putting <%= @member.errors.inspect %>: #<ActiveModel::Errors:0x000000000c6ebcf0 @base=#<Member id: nil, input: nil, gifttext: nil, spouse: nil, spousegift: nil, created_at: nil, updated_at: nil>, @messages={:person=>, :input=>, :gifts=>, :gifttext=>, :spouse=>, :spousegift=>}, @details={}>. And when I make the changes to the create action I get this error: ActiveModel::MissingAttributeError in MembersController#create can't write unknown attributeuser_id
– Alex
Dec 31 '18 at 17:03
what does your members table look like? It should have a user_id column when you are usingbelongs_to :user
– eikes
Jan 1 at 21:39
This may actually be the case. I edited the question to show when I added the Members migration for when I created the table so you can see the columns. How would I go about adding a user_id column after the table is already created? Hope this fixes it.
– Alex
Jan 2 at 0:58
|
show 1 more comment
Your MembersController#update method uses a @article
instance variable. You probably meant @member
:
def update
@member = Member.find(params[:id])
if @member.update(member_params)
redirect_to @member
else
render 'edit'
end
end
Your create action doesn't look quite right either you need to set the user and redirect correctly:
def create
@member = Member.new(member_params)
@member.user = current_user
if @member.save
redirect_to ask_member_path(@member)
else
render 'new'
end
end
_form.html.erb
<%= @member.errors.inspect %>
Your MembersController#update method uses a @article
instance variable. You probably meant @member
:
def update
@member = Member.find(params[:id])
if @member.update(member_params)
redirect_to @member
else
render 'edit'
end
end
Your create action doesn't look quite right either you need to set the user and redirect correctly:
def create
@member = Member.new(member_params)
@member.user = current_user
if @member.save
redirect_to ask_member_path(@member)
else
render 'new'
end
end
_form.html.erb
<%= @member.errors.inspect %>
edited Dec 31 '18 at 15:10
answered Dec 29 '18 at 18:03
eikeseikes
2,24912018
2,24912018
I made these changes but unfortunately, it still does not save the data. Any more suggestions?
– Alex
Dec 30 '18 at 22:31
You need to see the@member.errors
in the form, so you can see why it is not being saved. My wildest guess is, that since it belongs to a user you need to assign the current_user. I made the change in my comment above.
– eikes
Dec 31 '18 at 15:13
I'm getting this on the form page after putting <%= @member.errors.inspect %>: #<ActiveModel::Errors:0x000000000c6ebcf0 @base=#<Member id: nil, input: nil, gifttext: nil, spouse: nil, spousegift: nil, created_at: nil, updated_at: nil>, @messages={:person=>, :input=>, :gifts=>, :gifttext=>, :spouse=>, :spousegift=>}, @details={}>. And when I make the changes to the create action I get this error: ActiveModel::MissingAttributeError in MembersController#create can't write unknown attributeuser_id
– Alex
Dec 31 '18 at 17:03
what does your members table look like? It should have a user_id column when you are usingbelongs_to :user
– eikes
Jan 1 at 21:39
This may actually be the case. I edited the question to show when I added the Members migration for when I created the table so you can see the columns. How would I go about adding a user_id column after the table is already created? Hope this fixes it.
– Alex
Jan 2 at 0:58
|
show 1 more comment
I made these changes but unfortunately, it still does not save the data. Any more suggestions?
– Alex
Dec 30 '18 at 22:31
You need to see the@member.errors
in the form, so you can see why it is not being saved. My wildest guess is, that since it belongs to a user you need to assign the current_user. I made the change in my comment above.
– eikes
Dec 31 '18 at 15:13
I'm getting this on the form page after putting <%= @member.errors.inspect %>: #<ActiveModel::Errors:0x000000000c6ebcf0 @base=#<Member id: nil, input: nil, gifttext: nil, spouse: nil, spousegift: nil, created_at: nil, updated_at: nil>, @messages={:person=>, :input=>, :gifts=>, :gifttext=>, :spouse=>, :spousegift=>}, @details={}>. And when I make the changes to the create action I get this error: ActiveModel::MissingAttributeError in MembersController#create can't write unknown attributeuser_id
– Alex
Dec 31 '18 at 17:03
what does your members table look like? It should have a user_id column when you are usingbelongs_to :user
– eikes
Jan 1 at 21:39
This may actually be the case. I edited the question to show when I added the Members migration for when I created the table so you can see the columns. How would I go about adding a user_id column after the table is already created? Hope this fixes it.
– Alex
Jan 2 at 0:58
I made these changes but unfortunately, it still does not save the data. Any more suggestions?
– Alex
Dec 30 '18 at 22:31
I made these changes but unfortunately, it still does not save the data. Any more suggestions?
– Alex
Dec 30 '18 at 22:31
You need to see the
@member.errors
in the form, so you can see why it is not being saved. My wildest guess is, that since it belongs to a user you need to assign the current_user. I made the change in my comment above.– eikes
Dec 31 '18 at 15:13
You need to see the
@member.errors
in the form, so you can see why it is not being saved. My wildest guess is, that since it belongs to a user you need to assign the current_user. I made the change in my comment above.– eikes
Dec 31 '18 at 15:13
I'm getting this on the form page after putting <%= @member.errors.inspect %>: #<ActiveModel::Errors:0x000000000c6ebcf0 @base=#<Member id: nil, input: nil, gifttext: nil, spouse: nil, spousegift: nil, created_at: nil, updated_at: nil>, @messages={:person=>, :input=>, :gifts=>, :gifttext=>, :spouse=>, :spousegift=>}, @details={}>. And when I make the changes to the create action I get this error: ActiveModel::MissingAttributeError in MembersController#create can't write unknown attribute
user_id
– Alex
Dec 31 '18 at 17:03
I'm getting this on the form page after putting <%= @member.errors.inspect %>: #<ActiveModel::Errors:0x000000000c6ebcf0 @base=#<Member id: nil, input: nil, gifttext: nil, spouse: nil, spousegift: nil, created_at: nil, updated_at: nil>, @messages={:person=>, :input=>, :gifts=>, :gifttext=>, :spouse=>, :spousegift=>}, @details={}>. And when I make the changes to the create action I get this error: ActiveModel::MissingAttributeError in MembersController#create can't write unknown attribute
user_id
– Alex
Dec 31 '18 at 17:03
what does your members table look like? It should have a user_id column when you are using
belongs_to :user
– eikes
Jan 1 at 21:39
what does your members table look like? It should have a user_id column when you are using
belongs_to :user
– eikes
Jan 1 at 21:39
This may actually be the case. I edited the question to show when I added the Members migration for when I created the table so you can see the columns. How would I go about adding a user_id column after the table is already created? Hope this fixes it.
– Alex
Jan 2 at 0:58
This may actually be the case. I edited the question to show when I added the Members migration for when I created the table so you can see the columns. How would I go about adding a user_id column after the table is already created? Hope this fixes it.
– Alex
Jan 2 at 0:58
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53971161%2frails-not-saving-entry-after-clicking-submit%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
tHC 7NCw9zn4EWYI59v,4OcP7 hgfu,oKkbv,DOaWevU3wyuxRFlT8 w6K5UeCceGQhga6yQq H,xu9yDw
1
Probably
@member
record is invalid. Increate
method, usesave!
instead ofsave
. It will raise an exception (if the record is invalid) that might help (please add it to your question)– MrShemek
Dec 29 '18 at 16:13
@article = Member.find(params[:id])
should be@member = Member.find(params[:id])
instead– Ana María Martínez Gómez
Dec 29 '18 at 18:32
It seems to me as you want to authorize member instead of user (it is an instance of it but the name of the class is different)
– Ana María Martínez Gómez
Dec 29 '18 at 18:34