Could not cast value of type 'NSAsynchronousFetchResult' (0x103e13388) to 'NSArray' (0x10435cf28) Swift
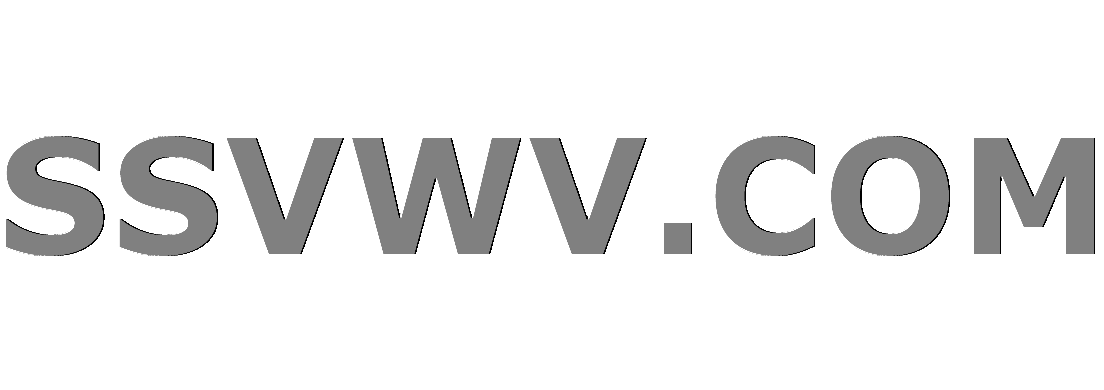
Multi tool use
I am working through a Core Data tutorial and my code throws the following error.
Could not cast value of type 'NSAsynchronousFetchResult' (0x103e13388) to 'NSArray' (0x10435cf28).
2018-12-29 22:54:17.619639+0700 demoCoreData[2670:247527] Could not cast value of type 'NSAsynchronousFetchResult' (0x103e13388) to 'NSArray' (0x10435cf28).
(lldb)
My Question is:
Based on the error provided what is the reason my application is failing ?
Here's my code that won't compile:
import UIKit
import CoreData
class ViewController: UIViewController {
@IBOutlet weak var txtID: UITextField!
@IBOutlet weak var txtTen: UITextField!
@IBOutlet weak var txtTuoi: UITextField!
@IBOutlet weak var lblKetQua: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
@IBAction func btnInsert(_ sender: Any) {
let appDel: AppDelegate = UIApplication.shared.delegate as! AppDelegate // tạo biến để connect giữa iphone với Manage Object Context
let context: NSManagedObjectContext = appDel.persistentContainer.viewContext //tạo biến connect với db
//insert new Khach Hang
let newKH = NSEntityDescription.insertNewObject(forEntityName: "KhachHang", into: context) //insert new object
newKH.setValue(Int(txtID.text!), forKey: "id") //forKey = tên column
newKH.setValue(txtTen.text, forKey: "tenKH")
newKH.setValue(Int(txtTuoi.text!), forKey: "tuoiKH")
do {
try context.save()
print("Insert Success")
}catch {
let err = error as NSError
print("Error is: (err)")
}
}
@IBAction func btnShow(_ sender: Any) {
let appDel: AppDelegate = UIApplication.shared.delegate as! AppDelegate // tạo biến để connect giữa iphone với Manage Object Context
let context: NSManagedObjectContext = appDel.persistentContainer.viewContext // tạo biến connect với db
let request = NSFetchRequest<NSFetchRequestResult>(entityName: "KhachHang")// tạo biến truy vấn đến table "KhachHang"
request.returnsObjectsAsFaults = false // có trả về những kết quả sai hay ko
do {
let results = try context.execute(request)
print("Truy Van Success")
for re in results as! [NSManagedObject] {
print(re.value(forKey: "tenKH"))
}
}catch {
let err = error as NSError
print("Excute err: (err)")
}
}
}
Here is my *.xcdatamodeld file
https://drive.google.com/open?id=18b1iJYz3jWAFkxYK2V4RrkxCTeWuR5yr
What I would really like to know, is how to investigate this on my own.
Thanks in advance for any help!
swift core-data
add a comment |
I am working through a Core Data tutorial and my code throws the following error.
Could not cast value of type 'NSAsynchronousFetchResult' (0x103e13388) to 'NSArray' (0x10435cf28).
2018-12-29 22:54:17.619639+0700 demoCoreData[2670:247527] Could not cast value of type 'NSAsynchronousFetchResult' (0x103e13388) to 'NSArray' (0x10435cf28).
(lldb)
My Question is:
Based on the error provided what is the reason my application is failing ?
Here's my code that won't compile:
import UIKit
import CoreData
class ViewController: UIViewController {
@IBOutlet weak var txtID: UITextField!
@IBOutlet weak var txtTen: UITextField!
@IBOutlet weak var txtTuoi: UITextField!
@IBOutlet weak var lblKetQua: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
@IBAction func btnInsert(_ sender: Any) {
let appDel: AppDelegate = UIApplication.shared.delegate as! AppDelegate // tạo biến để connect giữa iphone với Manage Object Context
let context: NSManagedObjectContext = appDel.persistentContainer.viewContext //tạo biến connect với db
//insert new Khach Hang
let newKH = NSEntityDescription.insertNewObject(forEntityName: "KhachHang", into: context) //insert new object
newKH.setValue(Int(txtID.text!), forKey: "id") //forKey = tên column
newKH.setValue(txtTen.text, forKey: "tenKH")
newKH.setValue(Int(txtTuoi.text!), forKey: "tuoiKH")
do {
try context.save()
print("Insert Success")
}catch {
let err = error as NSError
print("Error is: (err)")
}
}
@IBAction func btnShow(_ sender: Any) {
let appDel: AppDelegate = UIApplication.shared.delegate as! AppDelegate // tạo biến để connect giữa iphone với Manage Object Context
let context: NSManagedObjectContext = appDel.persistentContainer.viewContext // tạo biến connect với db
let request = NSFetchRequest<NSFetchRequestResult>(entityName: "KhachHang")// tạo biến truy vấn đến table "KhachHang"
request.returnsObjectsAsFaults = false // có trả về những kết quả sai hay ko
do {
let results = try context.execute(request)
print("Truy Van Success")
for re in results as! [NSManagedObject] {
print(re.value(forKey: "tenKH"))
}
}catch {
let err = error as NSError
print("Excute err: (err)")
}
}
}
Here is my *.xcdatamodeld file
https://drive.google.com/open?id=18b1iJYz3jWAFkxYK2V4RrkxCTeWuR5yr
What I would really like to know, is how to investigate this on my own.
Thanks in advance for any help!
swift core-data
The error message is very clear. You have aNSAsynchronousFetchResult
(the result of a call toNSManagedObjectContext.execute()
that returns a ` NSPersistentStoreResult, but you're trying to force cast it to an
NSArray`, which it isn't. Read the documentation.
– Alexander
Dec 29 '18 at 16:24
In for loop, the original code is like below: for re in results { print(re.value(forKey: "tenKH")) } I tried that, but it did not work.
– vaart12345
Dec 29 '18 at 16:31
add a comment |
I am working through a Core Data tutorial and my code throws the following error.
Could not cast value of type 'NSAsynchronousFetchResult' (0x103e13388) to 'NSArray' (0x10435cf28).
2018-12-29 22:54:17.619639+0700 demoCoreData[2670:247527] Could not cast value of type 'NSAsynchronousFetchResult' (0x103e13388) to 'NSArray' (0x10435cf28).
(lldb)
My Question is:
Based on the error provided what is the reason my application is failing ?
Here's my code that won't compile:
import UIKit
import CoreData
class ViewController: UIViewController {
@IBOutlet weak var txtID: UITextField!
@IBOutlet weak var txtTen: UITextField!
@IBOutlet weak var txtTuoi: UITextField!
@IBOutlet weak var lblKetQua: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
@IBAction func btnInsert(_ sender: Any) {
let appDel: AppDelegate = UIApplication.shared.delegate as! AppDelegate // tạo biến để connect giữa iphone với Manage Object Context
let context: NSManagedObjectContext = appDel.persistentContainer.viewContext //tạo biến connect với db
//insert new Khach Hang
let newKH = NSEntityDescription.insertNewObject(forEntityName: "KhachHang", into: context) //insert new object
newKH.setValue(Int(txtID.text!), forKey: "id") //forKey = tên column
newKH.setValue(txtTen.text, forKey: "tenKH")
newKH.setValue(Int(txtTuoi.text!), forKey: "tuoiKH")
do {
try context.save()
print("Insert Success")
}catch {
let err = error as NSError
print("Error is: (err)")
}
}
@IBAction func btnShow(_ sender: Any) {
let appDel: AppDelegate = UIApplication.shared.delegate as! AppDelegate // tạo biến để connect giữa iphone với Manage Object Context
let context: NSManagedObjectContext = appDel.persistentContainer.viewContext // tạo biến connect với db
let request = NSFetchRequest<NSFetchRequestResult>(entityName: "KhachHang")// tạo biến truy vấn đến table "KhachHang"
request.returnsObjectsAsFaults = false // có trả về những kết quả sai hay ko
do {
let results = try context.execute(request)
print("Truy Van Success")
for re in results as! [NSManagedObject] {
print(re.value(forKey: "tenKH"))
}
}catch {
let err = error as NSError
print("Excute err: (err)")
}
}
}
Here is my *.xcdatamodeld file
https://drive.google.com/open?id=18b1iJYz3jWAFkxYK2V4RrkxCTeWuR5yr
What I would really like to know, is how to investigate this on my own.
Thanks in advance for any help!
swift core-data
I am working through a Core Data tutorial and my code throws the following error.
Could not cast value of type 'NSAsynchronousFetchResult' (0x103e13388) to 'NSArray' (0x10435cf28).
2018-12-29 22:54:17.619639+0700 demoCoreData[2670:247527] Could not cast value of type 'NSAsynchronousFetchResult' (0x103e13388) to 'NSArray' (0x10435cf28).
(lldb)
My Question is:
Based on the error provided what is the reason my application is failing ?
Here's my code that won't compile:
import UIKit
import CoreData
class ViewController: UIViewController {
@IBOutlet weak var txtID: UITextField!
@IBOutlet weak var txtTen: UITextField!
@IBOutlet weak var txtTuoi: UITextField!
@IBOutlet weak var lblKetQua: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
@IBAction func btnInsert(_ sender: Any) {
let appDel: AppDelegate = UIApplication.shared.delegate as! AppDelegate // tạo biến để connect giữa iphone với Manage Object Context
let context: NSManagedObjectContext = appDel.persistentContainer.viewContext //tạo biến connect với db
//insert new Khach Hang
let newKH = NSEntityDescription.insertNewObject(forEntityName: "KhachHang", into: context) //insert new object
newKH.setValue(Int(txtID.text!), forKey: "id") //forKey = tên column
newKH.setValue(txtTen.text, forKey: "tenKH")
newKH.setValue(Int(txtTuoi.text!), forKey: "tuoiKH")
do {
try context.save()
print("Insert Success")
}catch {
let err = error as NSError
print("Error is: (err)")
}
}
@IBAction func btnShow(_ sender: Any) {
let appDel: AppDelegate = UIApplication.shared.delegate as! AppDelegate // tạo biến để connect giữa iphone với Manage Object Context
let context: NSManagedObjectContext = appDel.persistentContainer.viewContext // tạo biến connect với db
let request = NSFetchRequest<NSFetchRequestResult>(entityName: "KhachHang")// tạo biến truy vấn đến table "KhachHang"
request.returnsObjectsAsFaults = false // có trả về những kết quả sai hay ko
do {
let results = try context.execute(request)
print("Truy Van Success")
for re in results as! [NSManagedObject] {
print(re.value(forKey: "tenKH"))
}
}catch {
let err = error as NSError
print("Excute err: (err)")
}
}
}
Here is my *.xcdatamodeld file
https://drive.google.com/open?id=18b1iJYz3jWAFkxYK2V4RrkxCTeWuR5yr
What I would really like to know, is how to investigate this on my own.
Thanks in advance for any help!
swift core-data
swift core-data
edited Dec 29 '18 at 16:25
vaart12345
asked Dec 29 '18 at 16:19


vaart12345vaart12345
163
163
The error message is very clear. You have aNSAsynchronousFetchResult
(the result of a call toNSManagedObjectContext.execute()
that returns a ` NSPersistentStoreResult, but you're trying to force cast it to an
NSArray`, which it isn't. Read the documentation.
– Alexander
Dec 29 '18 at 16:24
In for loop, the original code is like below: for re in results { print(re.value(forKey: "tenKH")) } I tried that, but it did not work.
– vaart12345
Dec 29 '18 at 16:31
add a comment |
The error message is very clear. You have aNSAsynchronousFetchResult
(the result of a call toNSManagedObjectContext.execute()
that returns a ` NSPersistentStoreResult, but you're trying to force cast it to an
NSArray`, which it isn't. Read the documentation.
– Alexander
Dec 29 '18 at 16:24
In for loop, the original code is like below: for re in results { print(re.value(forKey: "tenKH")) } I tried that, but it did not work.
– vaart12345
Dec 29 '18 at 16:31
The error message is very clear. You have a
NSAsynchronousFetchResult
(the result of a call to NSManagedObjectContext.execute()
that returns a ` NSPersistentStoreResult, but you're trying to force cast it to an
NSArray`, which it isn't. Read the documentation.– Alexander
Dec 29 '18 at 16:24
The error message is very clear. You have a
NSAsynchronousFetchResult
(the result of a call to NSManagedObjectContext.execute()
that returns a ` NSPersistentStoreResult, but you're trying to force cast it to an
NSArray`, which it isn't. Read the documentation.– Alexander
Dec 29 '18 at 16:24
In for loop, the original code is like below: for re in results { print(re.value(forKey: "tenKH")) } I tried that, but it did not work.
– vaart12345
Dec 29 '18 at 16:31
In for loop, the original code is like below: for re in results { print(re.value(forKey: "tenKH")) } I tried that, but it did not work.
– vaart12345
Dec 29 '18 at 16:31
add a comment |
1 Answer
1
active
oldest
votes
From your code:
let results = try context.execute(request)
for re in results as! [NSManagedObject] {
print(re.value(forKey: "tenKH"))
}
The execute
method you're using returns an instance of NSPersistentStoreResult
. This is an abstract class. The NSAsynchronousFetchResult
mentioned in your error message is a concrete subclass of NSPersistentStoreResult
.
Next, you attempt to cast this to [NSManagedObject]
, which is a different, unrelated class. The error you see happens because it's not possible to typecast an NSAsynchronousFetchResult
to an array, because they are not related or even similar to each other.
It looks like you meant to use fetch(request)
instead of execute(request)
, because fetch
returns an array of managed objects.
What I would really like to know, is how to investigate this on my own.
I wouldn't mention it but since you asked specifically: This is something you'd resolve by looking at Apple's documentation for the classes and methods you're using. The execute
method is documented to return something different from what your code expects, and the error message gives a big clue to the nature of the problem.
@tom_Harrington, thank you for your detail explaining! I am a will follow your advice!
– vaart12345
Dec 30 '18 at 5:17
thank you again for your help. My problem was solved.
– vaart12345
Dec 30 '18 at 15:18
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53971228%2fcould-not-cast-value-of-type-nsasynchronousfetchresult-0x103e13388-to-nsarr%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
From your code:
let results = try context.execute(request)
for re in results as! [NSManagedObject] {
print(re.value(forKey: "tenKH"))
}
The execute
method you're using returns an instance of NSPersistentStoreResult
. This is an abstract class. The NSAsynchronousFetchResult
mentioned in your error message is a concrete subclass of NSPersistentStoreResult
.
Next, you attempt to cast this to [NSManagedObject]
, which is a different, unrelated class. The error you see happens because it's not possible to typecast an NSAsynchronousFetchResult
to an array, because they are not related or even similar to each other.
It looks like you meant to use fetch(request)
instead of execute(request)
, because fetch
returns an array of managed objects.
What I would really like to know, is how to investigate this on my own.
I wouldn't mention it but since you asked specifically: This is something you'd resolve by looking at Apple's documentation for the classes and methods you're using. The execute
method is documented to return something different from what your code expects, and the error message gives a big clue to the nature of the problem.
@tom_Harrington, thank you for your detail explaining! I am a will follow your advice!
– vaart12345
Dec 30 '18 at 5:17
thank you again for your help. My problem was solved.
– vaart12345
Dec 30 '18 at 15:18
add a comment |
From your code:
let results = try context.execute(request)
for re in results as! [NSManagedObject] {
print(re.value(forKey: "tenKH"))
}
The execute
method you're using returns an instance of NSPersistentStoreResult
. This is an abstract class. The NSAsynchronousFetchResult
mentioned in your error message is a concrete subclass of NSPersistentStoreResult
.
Next, you attempt to cast this to [NSManagedObject]
, which is a different, unrelated class. The error you see happens because it's not possible to typecast an NSAsynchronousFetchResult
to an array, because they are not related or even similar to each other.
It looks like you meant to use fetch(request)
instead of execute(request)
, because fetch
returns an array of managed objects.
What I would really like to know, is how to investigate this on my own.
I wouldn't mention it but since you asked specifically: This is something you'd resolve by looking at Apple's documentation for the classes and methods you're using. The execute
method is documented to return something different from what your code expects, and the error message gives a big clue to the nature of the problem.
@tom_Harrington, thank you for your detail explaining! I am a will follow your advice!
– vaart12345
Dec 30 '18 at 5:17
thank you again for your help. My problem was solved.
– vaart12345
Dec 30 '18 at 15:18
add a comment |
From your code:
let results = try context.execute(request)
for re in results as! [NSManagedObject] {
print(re.value(forKey: "tenKH"))
}
The execute
method you're using returns an instance of NSPersistentStoreResult
. This is an abstract class. The NSAsynchronousFetchResult
mentioned in your error message is a concrete subclass of NSPersistentStoreResult
.
Next, you attempt to cast this to [NSManagedObject]
, which is a different, unrelated class. The error you see happens because it's not possible to typecast an NSAsynchronousFetchResult
to an array, because they are not related or even similar to each other.
It looks like you meant to use fetch(request)
instead of execute(request)
, because fetch
returns an array of managed objects.
What I would really like to know, is how to investigate this on my own.
I wouldn't mention it but since you asked specifically: This is something you'd resolve by looking at Apple's documentation for the classes and methods you're using. The execute
method is documented to return something different from what your code expects, and the error message gives a big clue to the nature of the problem.
From your code:
let results = try context.execute(request)
for re in results as! [NSManagedObject] {
print(re.value(forKey: "tenKH"))
}
The execute
method you're using returns an instance of NSPersistentStoreResult
. This is an abstract class. The NSAsynchronousFetchResult
mentioned in your error message is a concrete subclass of NSPersistentStoreResult
.
Next, you attempt to cast this to [NSManagedObject]
, which is a different, unrelated class. The error you see happens because it's not possible to typecast an NSAsynchronousFetchResult
to an array, because they are not related or even similar to each other.
It looks like you meant to use fetch(request)
instead of execute(request)
, because fetch
returns an array of managed objects.
What I would really like to know, is how to investigate this on my own.
I wouldn't mention it but since you asked specifically: This is something you'd resolve by looking at Apple's documentation for the classes and methods you're using. The execute
method is documented to return something different from what your code expects, and the error message gives a big clue to the nature of the problem.
answered Dec 30 '18 at 0:38
Tom HarringtonTom Harrington
52.9k5102131
52.9k5102131
@tom_Harrington, thank you for your detail explaining! I am a will follow your advice!
– vaart12345
Dec 30 '18 at 5:17
thank you again for your help. My problem was solved.
– vaart12345
Dec 30 '18 at 15:18
add a comment |
@tom_Harrington, thank you for your detail explaining! I am a will follow your advice!
– vaart12345
Dec 30 '18 at 5:17
thank you again for your help. My problem was solved.
– vaart12345
Dec 30 '18 at 15:18
@tom_Harrington, thank you for your detail explaining! I am a will follow your advice!
– vaart12345
Dec 30 '18 at 5:17
@tom_Harrington, thank you for your detail explaining! I am a will follow your advice!
– vaart12345
Dec 30 '18 at 5:17
thank you again for your help. My problem was solved.
– vaart12345
Dec 30 '18 at 15:18
thank you again for your help. My problem was solved.
– vaart12345
Dec 30 '18 at 15:18
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53971228%2fcould-not-cast-value-of-type-nsasynchronousfetchresult-0x103e13388-to-nsarr%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
G MqtrUpHRct sf DYt,8bqBzy350po
The error message is very clear. You have a
NSAsynchronousFetchResult
(the result of a call toNSManagedObjectContext.execute()
that returns a ` NSPersistentStoreResult, but you're trying to force cast it to an
NSArray`, which it isn't. Read the documentation.– Alexander
Dec 29 '18 at 16:24
In for loop, the original code is like below: for re in results { print(re.value(forKey: "tenKH")) } I tried that, but it did not work.
– vaart12345
Dec 29 '18 at 16:31