Compare content of two array
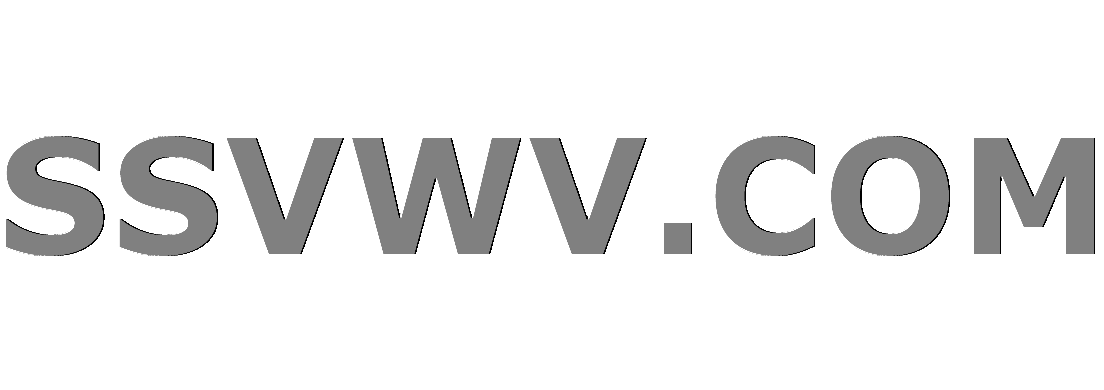
Multi tool use
I need to compare content for two array (source/target) of objects.
If an item in source does not exist in target, I should add item only to include.
If an item in source does exist in target, I should add item only to exclude.
Currently using ramda R.differenceWith()
but I get an issue when target is empty.
I would to know if differenceWith
fit the porpoise here, or I could use another functions. Please provide me an example thanks!
Notes: An answer even without using ramda is ok.
Demo
// source
const pathHash1 = {
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
};
const pathHash2 = {
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
};
const pathHash3 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
};
// target
const pathHash4 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
};
// works
// const source = [pathHash1, pathHash2, pathHash3]
// const target = [pathHash4]
// does no work
const source = [pathHash1, pathHash2, pathHash3]
const target =
// result pathHash1, pathHash2
const resultInclude = R.differenceWith((x,y)=> x.hash === y.hash, source, target)
const resultExclude= R.differenceWith((x,y)=> x.hash !== y.hash, source, target)
console.log('include',resultInclude.map(x=>x.hash))
console.log('exclude',resultExclude.map(x=>x.hash))
javascript ramda.js
add a comment |
I need to compare content for two array (source/target) of objects.
If an item in source does not exist in target, I should add item only to include.
If an item in source does exist in target, I should add item only to exclude.
Currently using ramda R.differenceWith()
but I get an issue when target is empty.
I would to know if differenceWith
fit the porpoise here, or I could use another functions. Please provide me an example thanks!
Notes: An answer even without using ramda is ok.
Demo
// source
const pathHash1 = {
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
};
const pathHash2 = {
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
};
const pathHash3 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
};
// target
const pathHash4 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
};
// works
// const source = [pathHash1, pathHash2, pathHash3]
// const target = [pathHash4]
// does no work
const source = [pathHash1, pathHash2, pathHash3]
const target =
// result pathHash1, pathHash2
const resultInclude = R.differenceWith((x,y)=> x.hash === y.hash, source, target)
const resultExclude= R.differenceWith((x,y)=> x.hash !== y.hash, source, target)
console.log('include',resultInclude.map(x=>x.hash))
console.log('exclude',resultExclude.map(x=>x.hash))
javascript ramda.js
add a comment |
I need to compare content for two array (source/target) of objects.
If an item in source does not exist in target, I should add item only to include.
If an item in source does exist in target, I should add item only to exclude.
Currently using ramda R.differenceWith()
but I get an issue when target is empty.
I would to know if differenceWith
fit the porpoise here, or I could use another functions. Please provide me an example thanks!
Notes: An answer even without using ramda is ok.
Demo
// source
const pathHash1 = {
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
};
const pathHash2 = {
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
};
const pathHash3 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
};
// target
const pathHash4 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
};
// works
// const source = [pathHash1, pathHash2, pathHash3]
// const target = [pathHash4]
// does no work
const source = [pathHash1, pathHash2, pathHash3]
const target =
// result pathHash1, pathHash2
const resultInclude = R.differenceWith((x,y)=> x.hash === y.hash, source, target)
const resultExclude= R.differenceWith((x,y)=> x.hash !== y.hash, source, target)
console.log('include',resultInclude.map(x=>x.hash))
console.log('exclude',resultExclude.map(x=>x.hash))
javascript ramda.js
I need to compare content for two array (source/target) of objects.
If an item in source does not exist in target, I should add item only to include.
If an item in source does exist in target, I should add item only to exclude.
Currently using ramda R.differenceWith()
but I get an issue when target is empty.
I would to know if differenceWith
fit the porpoise here, or I could use another functions. Please provide me an example thanks!
Notes: An answer even without using ramda is ok.
Demo
// source
const pathHash1 = {
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
};
const pathHash2 = {
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
};
const pathHash3 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
};
// target
const pathHash4 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
};
// works
// const source = [pathHash1, pathHash2, pathHash3]
// const target = [pathHash4]
// does no work
const source = [pathHash1, pathHash2, pathHash3]
const target =
// result pathHash1, pathHash2
const resultInclude = R.differenceWith((x,y)=> x.hash === y.hash, source, target)
const resultExclude= R.differenceWith((x,y)=> x.hash !== y.hash, source, target)
console.log('include',resultInclude.map(x=>x.hash))
console.log('exclude',resultExclude.map(x=>x.hash))
javascript ramda.js
javascript ramda.js
asked Dec 29 '18 at 16:01


RadexRadex
93311029
93311029
add a comment |
add a comment |
5 Answers
5
active
oldest
votes
Use R.innerJoin
for the exclude case:
const getInclude = R.differenceWith(R.eqProps('hash'))
const getExclude = R.innerJoin(R.eqProps('hash'))
const pathHash1 = {hash: "c4ca4238a0b923820dcc509a6f75849b",path: "./source/file1.txt"},pathHash2 = {hash: "c81e728d9d4c2f636f067f89cc14862c",path: "./source/file2.txt"},pathHash3 = {hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",path: "./souce/file3.txt"},pathHash4 = {hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",path: "./target/file3.txt"}
const source1 = [pathHash1, pathHash2, pathHash3]
const target1 = [pathHash4]
const source2 = [pathHash1, pathHash2, pathHash3]
const target2 =
const getHash = R.map(R.prop('hash'))
console.log('include1', getHash(getInclude(source1, target1)))
console.log('exclude1', getHash(getExclude(source1, target1)))
console.log('include2', getHash(getInclude(source2, target2)))
console.log('exclude2', getHash(getExclude(source2, target2)))
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
add a comment |
In vanilla js you could consider using this version:
const resultInclude = pathHashListSource.filter(x => !pathHashListTarget.find(y => y.hash === x.hash));
const resultExclude = pathHashListSource.filter(x => pathHashListTarget.find(y => y.hash === x.hash));
This should be an accepted answer. I really dig ramda, but this is the cleanest and least verbose solution here.
– codeepic
Jan 1 at 23:35
add a comment |
I think you could use difference
instead of differenceWith
for simple objects. If you want to find the common objects in both source
and target
, I'd suggest using innerJoin
:
const {difference, innerJoin, equals} = R;
const a = [{x: 1}, {y: 2}, {z: 3}];
const b = [{a: 0}, {x: 1}];
console.log(
difference(a, b)
);
console.log(
innerJoin(equals, a, b)
);
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
add a comment |
The result you get from R.differenceWith() is correct because the source of such a method is:
var differenceWith = _curry3(function differenceWith(pred, first, second) {
var out = ;
var idx = 0;
var firstLen = first.length;
while (idx < firstLen) {
if (!_includesWith(pred, first[idx], second) &&
!_includesWith(pred, first[idx], out)) {
out.push(first[idx]);
}
idx += 1;
}
return out;
});
Like you can see the difference is computed using _includesWith. But, while the second array is empty the on going output array is going to be be filled (no duplicates).
// source
const pathHash1 = {
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
};
const pathHash2 = {
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
};
const pathHash3 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
};
// target
const pathHash4 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
};
// works
// const source = [pathHash1, pathHash2, pathHash3]
// const target = [pathHash4]
// issue
const source = [pathHash1, pathHash2, pathHash3]
const target =
// result pathHash1, pathHash2
const resultInclude = R.differenceWith((x,y)=> x.hash === y.hash, source, target)
const resultExclude= R.differenceWith((x,y)=> x.hash !== y.hash, source, target)
console.log('include',resultInclude.map(x=>x.hash))
console.log('exclude',resultExclude.map(x=>x.hash))
<script src="https://cdn.jsdelivr.net/npm/ramda@latest/dist/ramda.min.js"></script>
add a comment |
Another option here is to build up a native Set
of the target hashes and use R.partition
to split the source list into two lists depending on whether or not the hash exists in the Set
.
const source = [
{
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
},
{
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
},
{
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
}
]
const target = [
{
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
}
]
////
const targetHashes =
target.reduce((hashes, next) => hashes.add(next.hash), new Set)
const [resultExclude, resultInclude] =
R.partition(x => targetHashes.has(x.hash), source)
////
console.log("resultInclude", resultInclude)
console.log("resultExclude", resultExclude)
<script src="//cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53971098%2fcompare-content-of-two-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
Use R.innerJoin
for the exclude case:
const getInclude = R.differenceWith(R.eqProps('hash'))
const getExclude = R.innerJoin(R.eqProps('hash'))
const pathHash1 = {hash: "c4ca4238a0b923820dcc509a6f75849b",path: "./source/file1.txt"},pathHash2 = {hash: "c81e728d9d4c2f636f067f89cc14862c",path: "./source/file2.txt"},pathHash3 = {hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",path: "./souce/file3.txt"},pathHash4 = {hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",path: "./target/file3.txt"}
const source1 = [pathHash1, pathHash2, pathHash3]
const target1 = [pathHash4]
const source2 = [pathHash1, pathHash2, pathHash3]
const target2 =
const getHash = R.map(R.prop('hash'))
console.log('include1', getHash(getInclude(source1, target1)))
console.log('exclude1', getHash(getExclude(source1, target1)))
console.log('include2', getHash(getInclude(source2, target2)))
console.log('exclude2', getHash(getExclude(source2, target2)))
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
add a comment |
Use R.innerJoin
for the exclude case:
const getInclude = R.differenceWith(R.eqProps('hash'))
const getExclude = R.innerJoin(R.eqProps('hash'))
const pathHash1 = {hash: "c4ca4238a0b923820dcc509a6f75849b",path: "./source/file1.txt"},pathHash2 = {hash: "c81e728d9d4c2f636f067f89cc14862c",path: "./source/file2.txt"},pathHash3 = {hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",path: "./souce/file3.txt"},pathHash4 = {hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",path: "./target/file3.txt"}
const source1 = [pathHash1, pathHash2, pathHash3]
const target1 = [pathHash4]
const source2 = [pathHash1, pathHash2, pathHash3]
const target2 =
const getHash = R.map(R.prop('hash'))
console.log('include1', getHash(getInclude(source1, target1)))
console.log('exclude1', getHash(getExclude(source1, target1)))
console.log('include2', getHash(getInclude(source2, target2)))
console.log('exclude2', getHash(getExclude(source2, target2)))
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
add a comment |
Use R.innerJoin
for the exclude case:
const getInclude = R.differenceWith(R.eqProps('hash'))
const getExclude = R.innerJoin(R.eqProps('hash'))
const pathHash1 = {hash: "c4ca4238a0b923820dcc509a6f75849b",path: "./source/file1.txt"},pathHash2 = {hash: "c81e728d9d4c2f636f067f89cc14862c",path: "./source/file2.txt"},pathHash3 = {hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",path: "./souce/file3.txt"},pathHash4 = {hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",path: "./target/file3.txt"}
const source1 = [pathHash1, pathHash2, pathHash3]
const target1 = [pathHash4]
const source2 = [pathHash1, pathHash2, pathHash3]
const target2 =
const getHash = R.map(R.prop('hash'))
console.log('include1', getHash(getInclude(source1, target1)))
console.log('exclude1', getHash(getExclude(source1, target1)))
console.log('include2', getHash(getInclude(source2, target2)))
console.log('exclude2', getHash(getExclude(source2, target2)))
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
Use R.innerJoin
for the exclude case:
const getInclude = R.differenceWith(R.eqProps('hash'))
const getExclude = R.innerJoin(R.eqProps('hash'))
const pathHash1 = {hash: "c4ca4238a0b923820dcc509a6f75849b",path: "./source/file1.txt"},pathHash2 = {hash: "c81e728d9d4c2f636f067f89cc14862c",path: "./source/file2.txt"},pathHash3 = {hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",path: "./souce/file3.txt"},pathHash4 = {hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",path: "./target/file3.txt"}
const source1 = [pathHash1, pathHash2, pathHash3]
const target1 = [pathHash4]
const source2 = [pathHash1, pathHash2, pathHash3]
const target2 =
const getHash = R.map(R.prop('hash'))
console.log('include1', getHash(getInclude(source1, target1)))
console.log('exclude1', getHash(getExclude(source1, target1)))
console.log('include2', getHash(getInclude(source2, target2)))
console.log('exclude2', getHash(getExclude(source2, target2)))
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
const getInclude = R.differenceWith(R.eqProps('hash'))
const getExclude = R.innerJoin(R.eqProps('hash'))
const pathHash1 = {hash: "c4ca4238a0b923820dcc509a6f75849b",path: "./source/file1.txt"},pathHash2 = {hash: "c81e728d9d4c2f636f067f89cc14862c",path: "./source/file2.txt"},pathHash3 = {hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",path: "./souce/file3.txt"},pathHash4 = {hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",path: "./target/file3.txt"}
const source1 = [pathHash1, pathHash2, pathHash3]
const target1 = [pathHash4]
const source2 = [pathHash1, pathHash2, pathHash3]
const target2 =
const getHash = R.map(R.prop('hash'))
console.log('include1', getHash(getInclude(source1, target1)))
console.log('exclude1', getHash(getExclude(source1, target1)))
console.log('include2', getHash(getInclude(source2, target2)))
console.log('exclude2', getHash(getExclude(source2, target2)))
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
const getInclude = R.differenceWith(R.eqProps('hash'))
const getExclude = R.innerJoin(R.eqProps('hash'))
const pathHash1 = {hash: "c4ca4238a0b923820dcc509a6f75849b",path: "./source/file1.txt"},pathHash2 = {hash: "c81e728d9d4c2f636f067f89cc14862c",path: "./source/file2.txt"},pathHash3 = {hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",path: "./souce/file3.txt"},pathHash4 = {hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",path: "./target/file3.txt"}
const source1 = [pathHash1, pathHash2, pathHash3]
const target1 = [pathHash4]
const source2 = [pathHash1, pathHash2, pathHash3]
const target2 =
const getHash = R.map(R.prop('hash'))
console.log('include1', getHash(getInclude(source1, target1)))
console.log('exclude1', getHash(getExclude(source1, target1)))
console.log('include2', getHash(getInclude(source2, target2)))
console.log('exclude2', getHash(getExclude(source2, target2)))
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
answered Dec 29 '18 at 18:00


Ori DroriOri Drori
75.8k138092
75.8k138092
add a comment |
add a comment |
In vanilla js you could consider using this version:
const resultInclude = pathHashListSource.filter(x => !pathHashListTarget.find(y => y.hash === x.hash));
const resultExclude = pathHashListSource.filter(x => pathHashListTarget.find(y => y.hash === x.hash));
This should be an accepted answer. I really dig ramda, but this is the cleanest and least verbose solution here.
– codeepic
Jan 1 at 23:35
add a comment |
In vanilla js you could consider using this version:
const resultInclude = pathHashListSource.filter(x => !pathHashListTarget.find(y => y.hash === x.hash));
const resultExclude = pathHashListSource.filter(x => pathHashListTarget.find(y => y.hash === x.hash));
This should be an accepted answer. I really dig ramda, but this is the cleanest and least verbose solution here.
– codeepic
Jan 1 at 23:35
add a comment |
In vanilla js you could consider using this version:
const resultInclude = pathHashListSource.filter(x => !pathHashListTarget.find(y => y.hash === x.hash));
const resultExclude = pathHashListSource.filter(x => pathHashListTarget.find(y => y.hash === x.hash));
In vanilla js you could consider using this version:
const resultInclude = pathHashListSource.filter(x => !pathHashListTarget.find(y => y.hash === x.hash));
const resultExclude = pathHashListSource.filter(x => pathHashListTarget.find(y => y.hash === x.hash));
edited Dec 29 '18 at 20:22
answered Dec 29 '18 at 16:32


GibboKGibboK
34.4k107317542
34.4k107317542
This should be an accepted answer. I really dig ramda, but this is the cleanest and least verbose solution here.
– codeepic
Jan 1 at 23:35
add a comment |
This should be an accepted answer. I really dig ramda, but this is the cleanest and least verbose solution here.
– codeepic
Jan 1 at 23:35
This should be an accepted answer. I really dig ramda, but this is the cleanest and least verbose solution here.
– codeepic
Jan 1 at 23:35
This should be an accepted answer. I really dig ramda, but this is the cleanest and least verbose solution here.
– codeepic
Jan 1 at 23:35
add a comment |
I think you could use difference
instead of differenceWith
for simple objects. If you want to find the common objects in both source
and target
, I'd suggest using innerJoin
:
const {difference, innerJoin, equals} = R;
const a = [{x: 1}, {y: 2}, {z: 3}];
const b = [{a: 0}, {x: 1}];
console.log(
difference(a, b)
);
console.log(
innerJoin(equals, a, b)
);
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
add a comment |
I think you could use difference
instead of differenceWith
for simple objects. If you want to find the common objects in both source
and target
, I'd suggest using innerJoin
:
const {difference, innerJoin, equals} = R;
const a = [{x: 1}, {y: 2}, {z: 3}];
const b = [{a: 0}, {x: 1}];
console.log(
difference(a, b)
);
console.log(
innerJoin(equals, a, b)
);
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
add a comment |
I think you could use difference
instead of differenceWith
for simple objects. If you want to find the common objects in both source
and target
, I'd suggest using innerJoin
:
const {difference, innerJoin, equals} = R;
const a = [{x: 1}, {y: 2}, {z: 3}];
const b = [{a: 0}, {x: 1}];
console.log(
difference(a, b)
);
console.log(
innerJoin(equals, a, b)
);
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
I think you could use difference
instead of differenceWith
for simple objects. If you want to find the common objects in both source
and target
, I'd suggest using innerJoin
:
const {difference, innerJoin, equals} = R;
const a = [{x: 1}, {y: 2}, {z: 3}];
const b = [{a: 0}, {x: 1}];
console.log(
difference(a, b)
);
console.log(
innerJoin(equals, a, b)
);
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
const {difference, innerJoin, equals} = R;
const a = [{x: 1}, {y: 2}, {z: 3}];
const b = [{a: 0}, {x: 1}];
console.log(
difference(a, b)
);
console.log(
innerJoin(equals, a, b)
);
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
const {difference, innerJoin, equals} = R;
const a = [{x: 1}, {y: 2}, {z: 3}];
const b = [{a: 0}, {x: 1}];
console.log(
difference(a, b)
);
console.log(
innerJoin(equals, a, b)
);
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
answered Dec 29 '18 at 16:22
customcommandercustomcommander
1,417819
1,417819
add a comment |
add a comment |
The result you get from R.differenceWith() is correct because the source of such a method is:
var differenceWith = _curry3(function differenceWith(pred, first, second) {
var out = ;
var idx = 0;
var firstLen = first.length;
while (idx < firstLen) {
if (!_includesWith(pred, first[idx], second) &&
!_includesWith(pred, first[idx], out)) {
out.push(first[idx]);
}
idx += 1;
}
return out;
});
Like you can see the difference is computed using _includesWith. But, while the second array is empty the on going output array is going to be be filled (no duplicates).
// source
const pathHash1 = {
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
};
const pathHash2 = {
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
};
const pathHash3 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
};
// target
const pathHash4 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
};
// works
// const source = [pathHash1, pathHash2, pathHash3]
// const target = [pathHash4]
// issue
const source = [pathHash1, pathHash2, pathHash3]
const target =
// result pathHash1, pathHash2
const resultInclude = R.differenceWith((x,y)=> x.hash === y.hash, source, target)
const resultExclude= R.differenceWith((x,y)=> x.hash !== y.hash, source, target)
console.log('include',resultInclude.map(x=>x.hash))
console.log('exclude',resultExclude.map(x=>x.hash))
<script src="https://cdn.jsdelivr.net/npm/ramda@latest/dist/ramda.min.js"></script>
add a comment |
The result you get from R.differenceWith() is correct because the source of such a method is:
var differenceWith = _curry3(function differenceWith(pred, first, second) {
var out = ;
var idx = 0;
var firstLen = first.length;
while (idx < firstLen) {
if (!_includesWith(pred, first[idx], second) &&
!_includesWith(pred, first[idx], out)) {
out.push(first[idx]);
}
idx += 1;
}
return out;
});
Like you can see the difference is computed using _includesWith. But, while the second array is empty the on going output array is going to be be filled (no duplicates).
// source
const pathHash1 = {
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
};
const pathHash2 = {
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
};
const pathHash3 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
};
// target
const pathHash4 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
};
// works
// const source = [pathHash1, pathHash2, pathHash3]
// const target = [pathHash4]
// issue
const source = [pathHash1, pathHash2, pathHash3]
const target =
// result pathHash1, pathHash2
const resultInclude = R.differenceWith((x,y)=> x.hash === y.hash, source, target)
const resultExclude= R.differenceWith((x,y)=> x.hash !== y.hash, source, target)
console.log('include',resultInclude.map(x=>x.hash))
console.log('exclude',resultExclude.map(x=>x.hash))
<script src="https://cdn.jsdelivr.net/npm/ramda@latest/dist/ramda.min.js"></script>
add a comment |
The result you get from R.differenceWith() is correct because the source of such a method is:
var differenceWith = _curry3(function differenceWith(pred, first, second) {
var out = ;
var idx = 0;
var firstLen = first.length;
while (idx < firstLen) {
if (!_includesWith(pred, first[idx], second) &&
!_includesWith(pred, first[idx], out)) {
out.push(first[idx]);
}
idx += 1;
}
return out;
});
Like you can see the difference is computed using _includesWith. But, while the second array is empty the on going output array is going to be be filled (no duplicates).
// source
const pathHash1 = {
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
};
const pathHash2 = {
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
};
const pathHash3 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
};
// target
const pathHash4 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
};
// works
// const source = [pathHash1, pathHash2, pathHash3]
// const target = [pathHash4]
// issue
const source = [pathHash1, pathHash2, pathHash3]
const target =
// result pathHash1, pathHash2
const resultInclude = R.differenceWith((x,y)=> x.hash === y.hash, source, target)
const resultExclude= R.differenceWith((x,y)=> x.hash !== y.hash, source, target)
console.log('include',resultInclude.map(x=>x.hash))
console.log('exclude',resultExclude.map(x=>x.hash))
<script src="https://cdn.jsdelivr.net/npm/ramda@latest/dist/ramda.min.js"></script>
The result you get from R.differenceWith() is correct because the source of such a method is:
var differenceWith = _curry3(function differenceWith(pred, first, second) {
var out = ;
var idx = 0;
var firstLen = first.length;
while (idx < firstLen) {
if (!_includesWith(pred, first[idx], second) &&
!_includesWith(pred, first[idx], out)) {
out.push(first[idx]);
}
idx += 1;
}
return out;
});
Like you can see the difference is computed using _includesWith. But, while the second array is empty the on going output array is going to be be filled (no duplicates).
// source
const pathHash1 = {
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
};
const pathHash2 = {
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
};
const pathHash3 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
};
// target
const pathHash4 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
};
// works
// const source = [pathHash1, pathHash2, pathHash3]
// const target = [pathHash4]
// issue
const source = [pathHash1, pathHash2, pathHash3]
const target =
// result pathHash1, pathHash2
const resultInclude = R.differenceWith((x,y)=> x.hash === y.hash, source, target)
const resultExclude= R.differenceWith((x,y)=> x.hash !== y.hash, source, target)
console.log('include',resultInclude.map(x=>x.hash))
console.log('exclude',resultExclude.map(x=>x.hash))
<script src="https://cdn.jsdelivr.net/npm/ramda@latest/dist/ramda.min.js"></script>
// source
const pathHash1 = {
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
};
const pathHash2 = {
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
};
const pathHash3 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
};
// target
const pathHash4 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
};
// works
// const source = [pathHash1, pathHash2, pathHash3]
// const target = [pathHash4]
// issue
const source = [pathHash1, pathHash2, pathHash3]
const target =
// result pathHash1, pathHash2
const resultInclude = R.differenceWith((x,y)=> x.hash === y.hash, source, target)
const resultExclude= R.differenceWith((x,y)=> x.hash !== y.hash, source, target)
console.log('include',resultInclude.map(x=>x.hash))
console.log('exclude',resultExclude.map(x=>x.hash))
<script src="https://cdn.jsdelivr.net/npm/ramda@latest/dist/ramda.min.js"></script>
// source
const pathHash1 = {
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
};
const pathHash2 = {
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
};
const pathHash3 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
};
// target
const pathHash4 = {
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
};
// works
// const source = [pathHash1, pathHash2, pathHash3]
// const target = [pathHash4]
// issue
const source = [pathHash1, pathHash2, pathHash3]
const target =
// result pathHash1, pathHash2
const resultInclude = R.differenceWith((x,y)=> x.hash === y.hash, source, target)
const resultExclude= R.differenceWith((x,y)=> x.hash !== y.hash, source, target)
console.log('include',resultInclude.map(x=>x.hash))
console.log('exclude',resultExclude.map(x=>x.hash))
<script src="https://cdn.jsdelivr.net/npm/ramda@latest/dist/ramda.min.js"></script>
answered Dec 29 '18 at 16:59


gaetanoMgaetanoM
29.3k52644
29.3k52644
add a comment |
add a comment |
Another option here is to build up a native Set
of the target hashes and use R.partition
to split the source list into two lists depending on whether or not the hash exists in the Set
.
const source = [
{
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
},
{
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
},
{
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
}
]
const target = [
{
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
}
]
////
const targetHashes =
target.reduce((hashes, next) => hashes.add(next.hash), new Set)
const [resultExclude, resultInclude] =
R.partition(x => targetHashes.has(x.hash), source)
////
console.log("resultInclude", resultInclude)
console.log("resultExclude", resultExclude)
<script src="//cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
add a comment |
Another option here is to build up a native Set
of the target hashes and use R.partition
to split the source list into two lists depending on whether or not the hash exists in the Set
.
const source = [
{
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
},
{
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
},
{
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
}
]
const target = [
{
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
}
]
////
const targetHashes =
target.reduce((hashes, next) => hashes.add(next.hash), new Set)
const [resultExclude, resultInclude] =
R.partition(x => targetHashes.has(x.hash), source)
////
console.log("resultInclude", resultInclude)
console.log("resultExclude", resultExclude)
<script src="//cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
add a comment |
Another option here is to build up a native Set
of the target hashes and use R.partition
to split the source list into two lists depending on whether or not the hash exists in the Set
.
const source = [
{
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
},
{
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
},
{
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
}
]
const target = [
{
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
}
]
////
const targetHashes =
target.reduce((hashes, next) => hashes.add(next.hash), new Set)
const [resultExclude, resultInclude] =
R.partition(x => targetHashes.has(x.hash), source)
////
console.log("resultInclude", resultInclude)
console.log("resultExclude", resultExclude)
<script src="//cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
Another option here is to build up a native Set
of the target hashes and use R.partition
to split the source list into two lists depending on whether or not the hash exists in the Set
.
const source = [
{
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
},
{
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
},
{
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
}
]
const target = [
{
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
}
]
////
const targetHashes =
target.reduce((hashes, next) => hashes.add(next.hash), new Set)
const [resultExclude, resultInclude] =
R.partition(x => targetHashes.has(x.hash), source)
////
console.log("resultInclude", resultInclude)
console.log("resultExclude", resultExclude)
<script src="//cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
const source = [
{
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
},
{
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
},
{
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
}
]
const target = [
{
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
}
]
////
const targetHashes =
target.reduce((hashes, next) => hashes.add(next.hash), new Set)
const [resultExclude, resultInclude] =
R.partition(x => targetHashes.has(x.hash), source)
////
console.log("resultInclude", resultInclude)
console.log("resultExclude", resultExclude)
<script src="//cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
const source = [
{
hash: "c4ca4238a0b923820dcc509a6f75849b",
path: "./source/file1.txt"
},
{
hash: "c81e728d9d4c2f636f067f89cc14862c",
path: "./source/file2.txt"
},
{
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./souce/file3.txt"
}
]
const target = [
{
hash: "eccbc87e4b5ce2fe28308fd9f2a7baf3",
path: "./target/file3.txt"
}
]
////
const targetHashes =
target.reduce((hashes, next) => hashes.add(next.hash), new Set)
const [resultExclude, resultInclude] =
R.partition(x => targetHashes.has(x.hash), source)
////
console.log("resultInclude", resultInclude)
console.log("resultExclude", resultExclude)
<script src="//cdnjs.cloudflare.com/ajax/libs/ramda/0.26.1/ramda.min.js"></script>
answered Dec 29 '18 at 21:49


Scott ChristopherScott Christopher
5,0291223
5,0291223
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53971098%2fcompare-content-of-two-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
b7VKC41 LtHf 99qiXdnaUi9ZpO8F2j7XaYm4