How can I stack two views on top of each other?
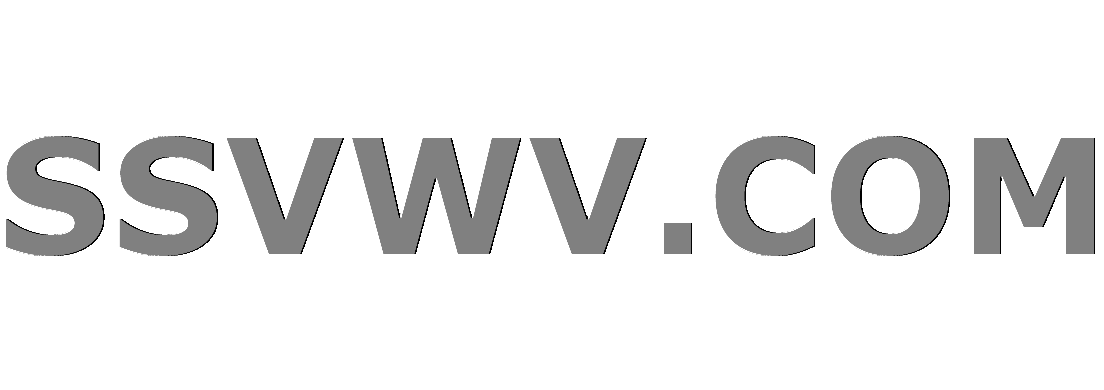
Multi tool use
I have 3 views, playView, gameView and timeView.
gameView is the top left block. timeView is the bottom left block and playView is the right block.
This is a photo of the program I need to remake.
How should I stack those two views on top of each other. And then the other view on the right of those two.
As you can see the bottom left block is a bit bigger than the top left block.
I've tried using GridLayout and BoxLayout but none of those seem to work.
This is what I've tried. It does work but the size is the same of the two left blocks.
public void setGameView(GameView gameView, PlayView playView,TimeView timeView) {
this.gameView = gameView;
this.playView = playView;
this.timeView = timeView;
JPanel subPanel = new JPanel(new BoxLayout(this, BoxLayout.Y_AXIS));
subPanel.add(gameView);
subPanel.add(timeView);
this.add(playView, BorderLayout.EAST);
this.add(subPanel, BorderLayout.WEST);
}
I would like to get the same result as the photo. If someone can steer me the right way. What layout should I use?
java swing layout-manager
add a comment |
I have 3 views, playView, gameView and timeView.
gameView is the top left block. timeView is the bottom left block and playView is the right block.
This is a photo of the program I need to remake.
How should I stack those two views on top of each other. And then the other view on the right of those two.
As you can see the bottom left block is a bit bigger than the top left block.
I've tried using GridLayout and BoxLayout but none of those seem to work.
This is what I've tried. It does work but the size is the same of the two left blocks.
public void setGameView(GameView gameView, PlayView playView,TimeView timeView) {
this.gameView = gameView;
this.playView = playView;
this.timeView = timeView;
JPanel subPanel = new JPanel(new BoxLayout(this, BoxLayout.Y_AXIS));
subPanel.add(gameView);
subPanel.add(timeView);
this.add(playView, BorderLayout.EAST);
this.add(subPanel, BorderLayout.WEST);
}
I would like to get the same result as the photo. If someone can steer me the right way. What layout should I use?
java swing layout-manager
@camickr I edited the image. I want those two blocks on the left like in the image, but I need those two blocks to have different sizes (also like in the image).
– idontunderstandarrays
Dec 29 '18 at 16:00
Either the GridBagLayout or BoxLayout should work. Both of these layout managers will respect thepreferred size
of each component. Then if there is extra space that space will be allocated to each component. This is done automatically by the BoxLayout. For the GridBagLayout you need to specify the weighty constraint of the GridBagConstraints. Read the section from the Swing tutorial on How to use GridBagLayout for more info on constraints. So if it doesn't work the problem is with your GameView and PlayView classes.
– camickr
Dec 29 '18 at 16:05
@camickr I set the size of each component so that it all fits good, but when I use BoxLayout I am not able to place two components on the left side and one above the other.
– idontunderstandarrays
Dec 29 '18 at 16:07
add a comment |
I have 3 views, playView, gameView and timeView.
gameView is the top left block. timeView is the bottom left block and playView is the right block.
This is a photo of the program I need to remake.
How should I stack those two views on top of each other. And then the other view on the right of those two.
As you can see the bottom left block is a bit bigger than the top left block.
I've tried using GridLayout and BoxLayout but none of those seem to work.
This is what I've tried. It does work but the size is the same of the two left blocks.
public void setGameView(GameView gameView, PlayView playView,TimeView timeView) {
this.gameView = gameView;
this.playView = playView;
this.timeView = timeView;
JPanel subPanel = new JPanel(new BoxLayout(this, BoxLayout.Y_AXIS));
subPanel.add(gameView);
subPanel.add(timeView);
this.add(playView, BorderLayout.EAST);
this.add(subPanel, BorderLayout.WEST);
}
I would like to get the same result as the photo. If someone can steer me the right way. What layout should I use?
java swing layout-manager
I have 3 views, playView, gameView and timeView.
gameView is the top left block. timeView is the bottom left block and playView is the right block.
This is a photo of the program I need to remake.
How should I stack those two views on top of each other. And then the other view on the right of those two.
As you can see the bottom left block is a bit bigger than the top left block.
I've tried using GridLayout and BoxLayout but none of those seem to work.
This is what I've tried. It does work but the size is the same of the two left blocks.
public void setGameView(GameView gameView, PlayView playView,TimeView timeView) {
this.gameView = gameView;
this.playView = playView;
this.timeView = timeView;
JPanel subPanel = new JPanel(new BoxLayout(this, BoxLayout.Y_AXIS));
subPanel.add(gameView);
subPanel.add(timeView);
this.add(playView, BorderLayout.EAST);
this.add(subPanel, BorderLayout.WEST);
}
I would like to get the same result as the photo. If someone can steer me the right way. What layout should I use?
java swing layout-manager
java swing layout-manager
edited Dec 29 '18 at 16:00
idontunderstandarrays
asked Dec 29 '18 at 15:49


idontunderstandarraysidontunderstandarrays
899
899
@camickr I edited the image. I want those two blocks on the left like in the image, but I need those two blocks to have different sizes (also like in the image).
– idontunderstandarrays
Dec 29 '18 at 16:00
Either the GridBagLayout or BoxLayout should work. Both of these layout managers will respect thepreferred size
of each component. Then if there is extra space that space will be allocated to each component. This is done automatically by the BoxLayout. For the GridBagLayout you need to specify the weighty constraint of the GridBagConstraints. Read the section from the Swing tutorial on How to use GridBagLayout for more info on constraints. So if it doesn't work the problem is with your GameView and PlayView classes.
– camickr
Dec 29 '18 at 16:05
@camickr I set the size of each component so that it all fits good, but when I use BoxLayout I am not able to place two components on the left side and one above the other.
– idontunderstandarrays
Dec 29 '18 at 16:07
add a comment |
@camickr I edited the image. I want those two blocks on the left like in the image, but I need those two blocks to have different sizes (also like in the image).
– idontunderstandarrays
Dec 29 '18 at 16:00
Either the GridBagLayout or BoxLayout should work. Both of these layout managers will respect thepreferred size
of each component. Then if there is extra space that space will be allocated to each component. This is done automatically by the BoxLayout. For the GridBagLayout you need to specify the weighty constraint of the GridBagConstraints. Read the section from the Swing tutorial on How to use GridBagLayout for more info on constraints. So if it doesn't work the problem is with your GameView and PlayView classes.
– camickr
Dec 29 '18 at 16:05
@camickr I set the size of each component so that it all fits good, but when I use BoxLayout I am not able to place two components on the left side and one above the other.
– idontunderstandarrays
Dec 29 '18 at 16:07
@camickr I edited the image. I want those two blocks on the left like in the image, but I need those two blocks to have different sizes (also like in the image).
– idontunderstandarrays
Dec 29 '18 at 16:00
@camickr I edited the image. I want those two blocks on the left like in the image, but I need those two blocks to have different sizes (also like in the image).
– idontunderstandarrays
Dec 29 '18 at 16:00
Either the GridBagLayout or BoxLayout should work. Both of these layout managers will respect the
preferred size
of each component. Then if there is extra space that space will be allocated to each component. This is done automatically by the BoxLayout. For the GridBagLayout you need to specify the weighty constraint of the GridBagConstraints. Read the section from the Swing tutorial on How to use GridBagLayout for more info on constraints. So if it doesn't work the problem is with your GameView and PlayView classes.– camickr
Dec 29 '18 at 16:05
Either the GridBagLayout or BoxLayout should work. Both of these layout managers will respect the
preferred size
of each component. Then if there is extra space that space will be allocated to each component. This is done automatically by the BoxLayout. For the GridBagLayout you need to specify the weighty constraint of the GridBagConstraints. Read the section from the Swing tutorial on How to use GridBagLayout for more info on constraints. So if it doesn't work the problem is with your GameView and PlayView classes.– camickr
Dec 29 '18 at 16:05
@camickr I set the size of each component so that it all fits good, but when I use BoxLayout I am not able to place two components on the left side and one above the other.
– idontunderstandarrays
Dec 29 '18 at 16:07
@camickr I set the size of each component so that it all fits good, but when I use BoxLayout I am not able to place two components on the left side and one above the other.
– idontunderstandarrays
Dec 29 '18 at 16:07
add a comment |
2 Answers
2
active
oldest
votes
I set the size
You should not set the size.
If you are using components on panels, then each layout manager will determine the preferred size.
If you are doing custom painting then your component should implement the getPreferredSize()
method so layout managers can do their job.
when I use BoxLayout I am not able to place two components on the left side
JPanel subPanel = new JPanel(new BoxLayout(this, BoxLayout.Y_AXIS));
subPanel.add(gameView);
subPanel.add(timeView);
You are setting the wrong panel to use the BoxLayout.
The code should be:
//JPanel subPanel = new JPanel(new BoxLayout(this, BoxLayout.Y_AXIS));
JPanel subPanel = new JPanel();
subPanel.setLayout(new BoxLayout(subPanel, BoxLayout.Y_AXIS));
Or an easier way is to sue the Box
class:
Box subpanel = Box.createVerticalBox();
Ah it works now! Thanks a lot :)
– idontunderstandarrays
Dec 30 '18 at 23:25
add a comment |
In the BorderLayout
, if no element is occupying a particular border, the panel in the CENTER
will extend to the edge of the parent.
In your case, your gamePanel
should be placed in the CENTER
, not the EAST
. Your subpanel should remain where it is in the WEST
.
I edited the image. I want those two blocks on the left like in the image, but I need those two blocks to have different sizes (also like in the image).
– idontunderstandarrays
Dec 29 '18 at 16:01
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53970999%2fhow-can-i-stack-two-views-on-top-of-each-other%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I set the size
You should not set the size.
If you are using components on panels, then each layout manager will determine the preferred size.
If you are doing custom painting then your component should implement the getPreferredSize()
method so layout managers can do their job.
when I use BoxLayout I am not able to place two components on the left side
JPanel subPanel = new JPanel(new BoxLayout(this, BoxLayout.Y_AXIS));
subPanel.add(gameView);
subPanel.add(timeView);
You are setting the wrong panel to use the BoxLayout.
The code should be:
//JPanel subPanel = new JPanel(new BoxLayout(this, BoxLayout.Y_AXIS));
JPanel subPanel = new JPanel();
subPanel.setLayout(new BoxLayout(subPanel, BoxLayout.Y_AXIS));
Or an easier way is to sue the Box
class:
Box subpanel = Box.createVerticalBox();
Ah it works now! Thanks a lot :)
– idontunderstandarrays
Dec 30 '18 at 23:25
add a comment |
I set the size
You should not set the size.
If you are using components on panels, then each layout manager will determine the preferred size.
If you are doing custom painting then your component should implement the getPreferredSize()
method so layout managers can do their job.
when I use BoxLayout I am not able to place two components on the left side
JPanel subPanel = new JPanel(new BoxLayout(this, BoxLayout.Y_AXIS));
subPanel.add(gameView);
subPanel.add(timeView);
You are setting the wrong panel to use the BoxLayout.
The code should be:
//JPanel subPanel = new JPanel(new BoxLayout(this, BoxLayout.Y_AXIS));
JPanel subPanel = new JPanel();
subPanel.setLayout(new BoxLayout(subPanel, BoxLayout.Y_AXIS));
Or an easier way is to sue the Box
class:
Box subpanel = Box.createVerticalBox();
Ah it works now! Thanks a lot :)
– idontunderstandarrays
Dec 30 '18 at 23:25
add a comment |
I set the size
You should not set the size.
If you are using components on panels, then each layout manager will determine the preferred size.
If you are doing custom painting then your component should implement the getPreferredSize()
method so layout managers can do their job.
when I use BoxLayout I am not able to place two components on the left side
JPanel subPanel = new JPanel(new BoxLayout(this, BoxLayout.Y_AXIS));
subPanel.add(gameView);
subPanel.add(timeView);
You are setting the wrong panel to use the BoxLayout.
The code should be:
//JPanel subPanel = new JPanel(new BoxLayout(this, BoxLayout.Y_AXIS));
JPanel subPanel = new JPanel();
subPanel.setLayout(new BoxLayout(subPanel, BoxLayout.Y_AXIS));
Or an easier way is to sue the Box
class:
Box subpanel = Box.createVerticalBox();
I set the size
You should not set the size.
If you are using components on panels, then each layout manager will determine the preferred size.
If you are doing custom painting then your component should implement the getPreferredSize()
method so layout managers can do their job.
when I use BoxLayout I am not able to place two components on the left side
JPanel subPanel = new JPanel(new BoxLayout(this, BoxLayout.Y_AXIS));
subPanel.add(gameView);
subPanel.add(timeView);
You are setting the wrong panel to use the BoxLayout.
The code should be:
//JPanel subPanel = new JPanel(new BoxLayout(this, BoxLayout.Y_AXIS));
JPanel subPanel = new JPanel();
subPanel.setLayout(new BoxLayout(subPanel, BoxLayout.Y_AXIS));
Or an easier way is to sue the Box
class:
Box subpanel = Box.createVerticalBox();
edited Dec 29 '18 at 16:59
answered Dec 29 '18 at 16:12
camickrcamickr
275k15127239
275k15127239
Ah it works now! Thanks a lot :)
– idontunderstandarrays
Dec 30 '18 at 23:25
add a comment |
Ah it works now! Thanks a lot :)
– idontunderstandarrays
Dec 30 '18 at 23:25
Ah it works now! Thanks a lot :)
– idontunderstandarrays
Dec 30 '18 at 23:25
Ah it works now! Thanks a lot :)
– idontunderstandarrays
Dec 30 '18 at 23:25
add a comment |
In the BorderLayout
, if no element is occupying a particular border, the panel in the CENTER
will extend to the edge of the parent.
In your case, your gamePanel
should be placed in the CENTER
, not the EAST
. Your subpanel should remain where it is in the WEST
.
I edited the image. I want those two blocks on the left like in the image, but I need those two blocks to have different sizes (also like in the image).
– idontunderstandarrays
Dec 29 '18 at 16:01
add a comment |
In the BorderLayout
, if no element is occupying a particular border, the panel in the CENTER
will extend to the edge of the parent.
In your case, your gamePanel
should be placed in the CENTER
, not the EAST
. Your subpanel should remain where it is in the WEST
.
I edited the image. I want those two blocks on the left like in the image, but I need those two blocks to have different sizes (also like in the image).
– idontunderstandarrays
Dec 29 '18 at 16:01
add a comment |
In the BorderLayout
, if no element is occupying a particular border, the panel in the CENTER
will extend to the edge of the parent.
In your case, your gamePanel
should be placed in the CENTER
, not the EAST
. Your subpanel should remain where it is in the WEST
.
In the BorderLayout
, if no element is occupying a particular border, the panel in the CENTER
will extend to the edge of the parent.
In your case, your gamePanel
should be placed in the CENTER
, not the EAST
. Your subpanel should remain where it is in the WEST
.
edited Dec 29 '18 at 15:59
answered Dec 29 '18 at 15:52
Joe CJoe C
11.3k62542
11.3k62542
I edited the image. I want those two blocks on the left like in the image, but I need those two blocks to have different sizes (also like in the image).
– idontunderstandarrays
Dec 29 '18 at 16:01
add a comment |
I edited the image. I want those two blocks on the left like in the image, but I need those two blocks to have different sizes (also like in the image).
– idontunderstandarrays
Dec 29 '18 at 16:01
I edited the image. I want those two blocks on the left like in the image, but I need those two blocks to have different sizes (also like in the image).
– idontunderstandarrays
Dec 29 '18 at 16:01
I edited the image. I want those two blocks on the left like in the image, but I need those two blocks to have different sizes (also like in the image).
– idontunderstandarrays
Dec 29 '18 at 16:01
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53970999%2fhow-can-i-stack-two-views-on-top-of-each-other%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
w5QE4Q,HMY4Ffr6vX2nO99vVqC rjDHC
@camickr I edited the image. I want those two blocks on the left like in the image, but I need those two blocks to have different sizes (also like in the image).
– idontunderstandarrays
Dec 29 '18 at 16:00
Either the GridBagLayout or BoxLayout should work. Both of these layout managers will respect the
preferred size
of each component. Then if there is extra space that space will be allocated to each component. This is done automatically by the BoxLayout. For the GridBagLayout you need to specify the weighty constraint of the GridBagConstraints. Read the section from the Swing tutorial on How to use GridBagLayout for more info on constraints. So if it doesn't work the problem is with your GameView and PlayView classes.– camickr
Dec 29 '18 at 16:05
@camickr I set the size of each component so that it all fits good, but when I use BoxLayout I am not able to place two components on the left side and one above the other.
– idontunderstandarrays
Dec 29 '18 at 16:07