How to put a string into an array
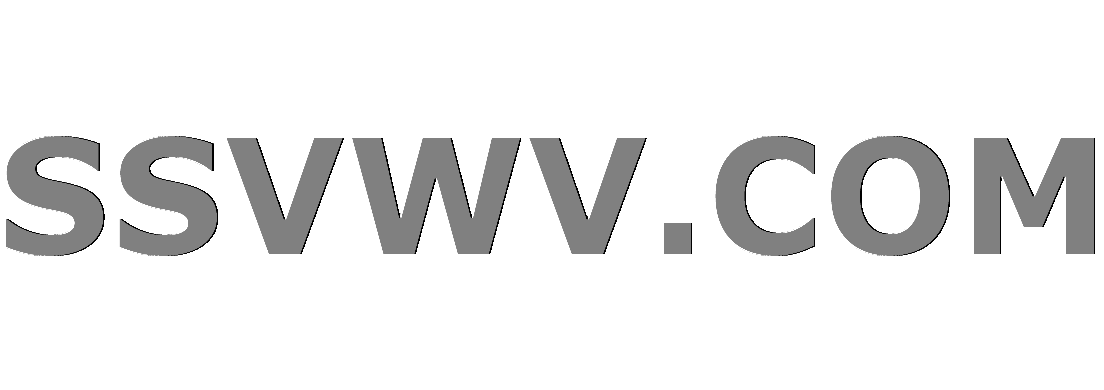
Multi tool use
I am having a hard time putting a string into an array.
The purpose of my program is to generate random strings. And I want those random strings generated to be placed on an array so I can access those strings again later.
char main()
{
srand(time(NULL));
char* rStr[9] = {0};
int i;
for (i = 0; i < 7; i++)
{
randomString(rStr, 8); //This is the function that generates a
//random string with 8 characters
printf("%d---%sn", i, rStr);
}
}
The above code will produce 7 randomly-generated strings with 8 characters. But my problem is I want every strings generated to be placed on an array so I can call them or display them again.
I have tried this below:
char main()
{
srand(time(NULL));
char* rStr[9] = {0};
int i;
for (i = 0; i < 7; i++)
{
rStr[i] = randomString(rStr, 8);
printf("%d---%sn", i, rStr[i]);
}
}
but my program would just crash.
EDIT:
int randomNumber(int min, int max) //This function is responsible for
//the randomness of the string
{
max -= min;
return (rand() % max) +min;
}
char randomString(char *str, int randomCharCount)
{
const char *charSet = "abcdefghijklmnopqrstuvwxyz0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ";
int i;
for (i = 0; i < randomCharCount; i++)
{
str[i] = charSet[randomNumber(0, 35)];
}
}
Credits of the code to the tutorial right here:
https://www.youtube.com/watch?v=3l1TKWVKyAY&index=58&list=PL0170B6E7DD6D8810&fbclid=IwAR2qq87y9qKt4PKPSZrwoRF10j1V9NU9W055j3-1YBXNBU7YtFDMMa1mxMg
https://www.youtube.com/watch?v=3l1TKWVKyAY&index=58&list=PL0170B6E7DD6D8810&fbclid=IwAR2qq87y9qKt4PKPSZrwoRF10j1V9NU9W055j3-1YBXNBU7YtFDMMa1mxMg
c arrays string
add a comment |
I am having a hard time putting a string into an array.
The purpose of my program is to generate random strings. And I want those random strings generated to be placed on an array so I can access those strings again later.
char main()
{
srand(time(NULL));
char* rStr[9] = {0};
int i;
for (i = 0; i < 7; i++)
{
randomString(rStr, 8); //This is the function that generates a
//random string with 8 characters
printf("%d---%sn", i, rStr);
}
}
The above code will produce 7 randomly-generated strings with 8 characters. But my problem is I want every strings generated to be placed on an array so I can call them or display them again.
I have tried this below:
char main()
{
srand(time(NULL));
char* rStr[9] = {0};
int i;
for (i = 0; i < 7; i++)
{
rStr[i] = randomString(rStr, 8);
printf("%d---%sn", i, rStr[i]);
}
}
but my program would just crash.
EDIT:
int randomNumber(int min, int max) //This function is responsible for
//the randomness of the string
{
max -= min;
return (rand() % max) +min;
}
char randomString(char *str, int randomCharCount)
{
const char *charSet = "abcdefghijklmnopqrstuvwxyz0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ";
int i;
for (i = 0; i < randomCharCount; i++)
{
str[i] = charSet[randomNumber(0, 35)];
}
}
Credits of the code to the tutorial right here:
https://www.youtube.com/watch?v=3l1TKWVKyAY&index=58&list=PL0170B6E7DD6D8810&fbclid=IwAR2qq87y9qKt4PKPSZrwoRF10j1V9NU9W055j3-1YBXNBU7YtFDMMa1mxMg
https://www.youtube.com/watch?v=3l1TKWVKyAY&index=58&list=PL0170B6E7DD6D8810&fbclid=IwAR2qq87y9qKt4PKPSZrwoRF10j1V9NU9W055j3-1YBXNBU7YtFDMMa1mxMg
c arrays string
The problem is not in the code you show, but in the code you don't show. What doesrandomString
really do? What does it return? Please create a Minimal, Complete, and Verifiable example to show us.
– Some programmer dude
Jan 2 at 3:45
char* rStr[9] = {0}
creates an array of 9 pointers to char, not an array ofchar [9]
?? (and be very wary of learning how to code from youtube...)
– David C. Rankin
Jan 2 at 4:02
YourrandomString
function is declared to return a singlechar
. And in fact doesn't return anything at all. You also dereference the pointer being passed, which is not the correct type (you pass a pointer to a pointer tochar
, i.e.char **
).
– Some programmer dude
Jan 2 at 4:07
Unless you are coding for a freestanding embedded system without an Operating System your declaration formain
is incorrect. For hosted systems, the proper declarations formain
areint main (void)
andint main (int argc, char **argv)
See: C11 Standard §5.1.2.2.1 Program startup p1 (draft n1570). See also: What should main() return in C and C++?
– David C. Rankin
Jan 2 at 4:09
add a comment |
I am having a hard time putting a string into an array.
The purpose of my program is to generate random strings. And I want those random strings generated to be placed on an array so I can access those strings again later.
char main()
{
srand(time(NULL));
char* rStr[9] = {0};
int i;
for (i = 0; i < 7; i++)
{
randomString(rStr, 8); //This is the function that generates a
//random string with 8 characters
printf("%d---%sn", i, rStr);
}
}
The above code will produce 7 randomly-generated strings with 8 characters. But my problem is I want every strings generated to be placed on an array so I can call them or display them again.
I have tried this below:
char main()
{
srand(time(NULL));
char* rStr[9] = {0};
int i;
for (i = 0; i < 7; i++)
{
rStr[i] = randomString(rStr, 8);
printf("%d---%sn", i, rStr[i]);
}
}
but my program would just crash.
EDIT:
int randomNumber(int min, int max) //This function is responsible for
//the randomness of the string
{
max -= min;
return (rand() % max) +min;
}
char randomString(char *str, int randomCharCount)
{
const char *charSet = "abcdefghijklmnopqrstuvwxyz0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ";
int i;
for (i = 0; i < randomCharCount; i++)
{
str[i] = charSet[randomNumber(0, 35)];
}
}
Credits of the code to the tutorial right here:
https://www.youtube.com/watch?v=3l1TKWVKyAY&index=58&list=PL0170B6E7DD6D8810&fbclid=IwAR2qq87y9qKt4PKPSZrwoRF10j1V9NU9W055j3-1YBXNBU7YtFDMMa1mxMg
https://www.youtube.com/watch?v=3l1TKWVKyAY&index=58&list=PL0170B6E7DD6D8810&fbclid=IwAR2qq87y9qKt4PKPSZrwoRF10j1V9NU9W055j3-1YBXNBU7YtFDMMa1mxMg
c arrays string
I am having a hard time putting a string into an array.
The purpose of my program is to generate random strings. And I want those random strings generated to be placed on an array so I can access those strings again later.
char main()
{
srand(time(NULL));
char* rStr[9] = {0};
int i;
for (i = 0; i < 7; i++)
{
randomString(rStr, 8); //This is the function that generates a
//random string with 8 characters
printf("%d---%sn", i, rStr);
}
}
The above code will produce 7 randomly-generated strings with 8 characters. But my problem is I want every strings generated to be placed on an array so I can call them or display them again.
I have tried this below:
char main()
{
srand(time(NULL));
char* rStr[9] = {0};
int i;
for (i = 0; i < 7; i++)
{
rStr[i] = randomString(rStr, 8);
printf("%d---%sn", i, rStr[i]);
}
}
but my program would just crash.
EDIT:
int randomNumber(int min, int max) //This function is responsible for
//the randomness of the string
{
max -= min;
return (rand() % max) +min;
}
char randomString(char *str, int randomCharCount)
{
const char *charSet = "abcdefghijklmnopqrstuvwxyz0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ";
int i;
for (i = 0; i < randomCharCount; i++)
{
str[i] = charSet[randomNumber(0, 35)];
}
}
Credits of the code to the tutorial right here:
https://www.youtube.com/watch?v=3l1TKWVKyAY&index=58&list=PL0170B6E7DD6D8810&fbclid=IwAR2qq87y9qKt4PKPSZrwoRF10j1V9NU9W055j3-1YBXNBU7YtFDMMa1mxMg
https://www.youtube.com/watch?v=3l1TKWVKyAY&index=58&list=PL0170B6E7DD6D8810&fbclid=IwAR2qq87y9qKt4PKPSZrwoRF10j1V9NU9W055j3-1YBXNBU7YtFDMMa1mxMg
c arrays string
c arrays string
edited Jan 2 at 7:54


Mike
2,0031722
2,0031722
asked Jan 2 at 3:39
ktl159ktl159
287
287
The problem is not in the code you show, but in the code you don't show. What doesrandomString
really do? What does it return? Please create a Minimal, Complete, and Verifiable example to show us.
– Some programmer dude
Jan 2 at 3:45
char* rStr[9] = {0}
creates an array of 9 pointers to char, not an array ofchar [9]
?? (and be very wary of learning how to code from youtube...)
– David C. Rankin
Jan 2 at 4:02
YourrandomString
function is declared to return a singlechar
. And in fact doesn't return anything at all. You also dereference the pointer being passed, which is not the correct type (you pass a pointer to a pointer tochar
, i.e.char **
).
– Some programmer dude
Jan 2 at 4:07
Unless you are coding for a freestanding embedded system without an Operating System your declaration formain
is incorrect. For hosted systems, the proper declarations formain
areint main (void)
andint main (int argc, char **argv)
See: C11 Standard §5.1.2.2.1 Program startup p1 (draft n1570). See also: What should main() return in C and C++?
– David C. Rankin
Jan 2 at 4:09
add a comment |
The problem is not in the code you show, but in the code you don't show. What doesrandomString
really do? What does it return? Please create a Minimal, Complete, and Verifiable example to show us.
– Some programmer dude
Jan 2 at 3:45
char* rStr[9] = {0}
creates an array of 9 pointers to char, not an array ofchar [9]
?? (and be very wary of learning how to code from youtube...)
– David C. Rankin
Jan 2 at 4:02
YourrandomString
function is declared to return a singlechar
. And in fact doesn't return anything at all. You also dereference the pointer being passed, which is not the correct type (you pass a pointer to a pointer tochar
, i.e.char **
).
– Some programmer dude
Jan 2 at 4:07
Unless you are coding for a freestanding embedded system without an Operating System your declaration formain
is incorrect. For hosted systems, the proper declarations formain
areint main (void)
andint main (int argc, char **argv)
See: C11 Standard §5.1.2.2.1 Program startup p1 (draft n1570). See also: What should main() return in C and C++?
– David C. Rankin
Jan 2 at 4:09
The problem is not in the code you show, but in the code you don't show. What does
randomString
really do? What does it return? Please create a Minimal, Complete, and Verifiable example to show us.– Some programmer dude
Jan 2 at 3:45
The problem is not in the code you show, but in the code you don't show. What does
randomString
really do? What does it return? Please create a Minimal, Complete, and Verifiable example to show us.– Some programmer dude
Jan 2 at 3:45
char* rStr[9] = {0}
creates an array of 9 pointers to char, not an array of char [9]
?? (and be very wary of learning how to code from youtube...)– David C. Rankin
Jan 2 at 4:02
char* rStr[9] = {0}
creates an array of 9 pointers to char, not an array of char [9]
?? (and be very wary of learning how to code from youtube...)– David C. Rankin
Jan 2 at 4:02
Your
randomString
function is declared to return a single char
. And in fact doesn't return anything at all. You also dereference the pointer being passed, which is not the correct type (you pass a pointer to a pointer to char
, i.e. char **
).– Some programmer dude
Jan 2 at 4:07
Your
randomString
function is declared to return a single char
. And in fact doesn't return anything at all. You also dereference the pointer being passed, which is not the correct type (you pass a pointer to a pointer to char
, i.e. char **
).– Some programmer dude
Jan 2 at 4:07
Unless you are coding for a freestanding embedded system without an Operating System your declaration for
main
is incorrect. For hosted systems, the proper declarations for main
are int main (void)
and int main (int argc, char **argv)
See: C11 Standard §5.1.2.2.1 Program startup p1 (draft n1570). See also: What should main() return in C and C++?– David C. Rankin
Jan 2 at 4:09
Unless you are coding for a freestanding embedded system without an Operating System your declaration for
main
is incorrect. For hosted systems, the proper declarations for main
are int main (void)
and int main (int argc, char **argv)
See: C11 Standard §5.1.2.2.1 Program startup p1 (draft n1570). See also: What should main() return in C and C++?– David C. Rankin
Jan 2 at 4:09
add a comment |
2 Answers
2
active
oldest
votes
Your code has following problems
- No
return
type formain()
. - You have to declare separate array for saving
strings
.
Try the following code :-
char main()
{
srand(time(NULL));
char rStr[9] = {0};
// sStr is where the rStr are saved .
char sStr[50][9]; // max 50 rStr
int i;
for (i = 0; i < 7; i++)
{
randomString(rStr, 8);
strcpy(sStr[i],rStr);
printf("%d---%sn", i, sStr[i]);
}
// some return value here.
return 0;
}
2
Thank you very much for answering my question and providing a code, anoopknr! This is a huge help for me. God bless!
– ktl159
Jan 2 at 4:18
add a comment |
Think you're looking for strcpy
.
Initialising and then "pushing" to the array:
char stringarray[MAX_NUMBER_STRINGS][MAX_STRING_SIZE];
strcpy(stringarray[0], "blah");
Also main should return an int see valid options below for main:
int main(void);
or:
int main(int argc, char* argv);
References:
How do I create an array of strings in C?
What are the valid signatures for C's main() function?
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54000957%2fhow-to-put-a-string-into-an-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your code has following problems
- No
return
type formain()
. - You have to declare separate array for saving
strings
.
Try the following code :-
char main()
{
srand(time(NULL));
char rStr[9] = {0};
// sStr is where the rStr are saved .
char sStr[50][9]; // max 50 rStr
int i;
for (i = 0; i < 7; i++)
{
randomString(rStr, 8);
strcpy(sStr[i],rStr);
printf("%d---%sn", i, sStr[i]);
}
// some return value here.
return 0;
}
2
Thank you very much for answering my question and providing a code, anoopknr! This is a huge help for me. God bless!
– ktl159
Jan 2 at 4:18
add a comment |
Your code has following problems
- No
return
type formain()
. - You have to declare separate array for saving
strings
.
Try the following code :-
char main()
{
srand(time(NULL));
char rStr[9] = {0};
// sStr is where the rStr are saved .
char sStr[50][9]; // max 50 rStr
int i;
for (i = 0; i < 7; i++)
{
randomString(rStr, 8);
strcpy(sStr[i],rStr);
printf("%d---%sn", i, sStr[i]);
}
// some return value here.
return 0;
}
2
Thank you very much for answering my question and providing a code, anoopknr! This is a huge help for me. God bless!
– ktl159
Jan 2 at 4:18
add a comment |
Your code has following problems
- No
return
type formain()
. - You have to declare separate array for saving
strings
.
Try the following code :-
char main()
{
srand(time(NULL));
char rStr[9] = {0};
// sStr is where the rStr are saved .
char sStr[50][9]; // max 50 rStr
int i;
for (i = 0; i < 7; i++)
{
randomString(rStr, 8);
strcpy(sStr[i],rStr);
printf("%d---%sn", i, sStr[i]);
}
// some return value here.
return 0;
}
Your code has following problems
- No
return
type formain()
. - You have to declare separate array for saving
strings
.
Try the following code :-
char main()
{
srand(time(NULL));
char rStr[9] = {0};
// sStr is where the rStr are saved .
char sStr[50][9]; // max 50 rStr
int i;
for (i = 0; i < 7; i++)
{
randomString(rStr, 8);
strcpy(sStr[i],rStr);
printf("%d---%sn", i, sStr[i]);
}
// some return value here.
return 0;
}
answered Jan 2 at 4:06
anoopknranoopknr
1,7491718
1,7491718
2
Thank you very much for answering my question and providing a code, anoopknr! This is a huge help for me. God bless!
– ktl159
Jan 2 at 4:18
add a comment |
2
Thank you very much for answering my question and providing a code, anoopknr! This is a huge help for me. God bless!
– ktl159
Jan 2 at 4:18
2
2
Thank you very much for answering my question and providing a code, anoopknr! This is a huge help for me. God bless!
– ktl159
Jan 2 at 4:18
Thank you very much for answering my question and providing a code, anoopknr! This is a huge help for me. God bless!
– ktl159
Jan 2 at 4:18
add a comment |
Think you're looking for strcpy
.
Initialising and then "pushing" to the array:
char stringarray[MAX_NUMBER_STRINGS][MAX_STRING_SIZE];
strcpy(stringarray[0], "blah");
Also main should return an int see valid options below for main:
int main(void);
or:
int main(int argc, char* argv);
References:
How do I create an array of strings in C?
What are the valid signatures for C's main() function?
add a comment |
Think you're looking for strcpy
.
Initialising and then "pushing" to the array:
char stringarray[MAX_NUMBER_STRINGS][MAX_STRING_SIZE];
strcpy(stringarray[0], "blah");
Also main should return an int see valid options below for main:
int main(void);
or:
int main(int argc, char* argv);
References:
How do I create an array of strings in C?
What are the valid signatures for C's main() function?
add a comment |
Think you're looking for strcpy
.
Initialising and then "pushing" to the array:
char stringarray[MAX_NUMBER_STRINGS][MAX_STRING_SIZE];
strcpy(stringarray[0], "blah");
Also main should return an int see valid options below for main:
int main(void);
or:
int main(int argc, char* argv);
References:
How do I create an array of strings in C?
What are the valid signatures for C's main() function?
Think you're looking for strcpy
.
Initialising and then "pushing" to the array:
char stringarray[MAX_NUMBER_STRINGS][MAX_STRING_SIZE];
strcpy(stringarray[0], "blah");
Also main should return an int see valid options below for main:
int main(void);
or:
int main(int argc, char* argv);
References:
How do I create an array of strings in C?
What are the valid signatures for C's main() function?
edited Jan 2 at 4:07
answered Jan 2 at 4:04


Adam DadAdam Dad
114
114
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54000957%2fhow-to-put-a-string-into-an-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
R1xSN yAitQv,M6gIxquBlD2DJCUMYggThKaB,Igk,eC KWz,oF5Xo
The problem is not in the code you show, but in the code you don't show. What does
randomString
really do? What does it return? Please create a Minimal, Complete, and Verifiable example to show us.– Some programmer dude
Jan 2 at 3:45
char* rStr[9] = {0}
creates an array of 9 pointers to char, not an array ofchar [9]
?? (and be very wary of learning how to code from youtube...)– David C. Rankin
Jan 2 at 4:02
Your
randomString
function is declared to return a singlechar
. And in fact doesn't return anything at all. You also dereference the pointer being passed, which is not the correct type (you pass a pointer to a pointer tochar
, i.e.char **
).– Some programmer dude
Jan 2 at 4:07
Unless you are coding for a freestanding embedded system without an Operating System your declaration for
main
is incorrect. For hosted systems, the proper declarations formain
areint main (void)
andint main (int argc, char **argv)
See: C11 Standard §5.1.2.2.1 Program startup p1 (draft n1570). See also: What should main() return in C and C++?– David C. Rankin
Jan 2 at 4:09