How do I allow backspace in a textbox while capturing keydown events
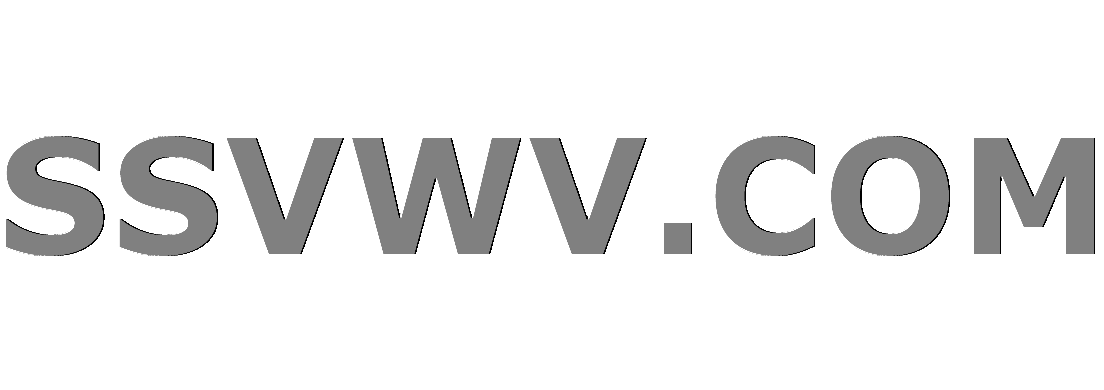
Multi tool use
I've got an interactive graph built with d3, and I capture the backspace key to remove elements from the graph. However, I also have a text box on the page, and I'd like to be able to use backspace while typing. Is there a way to allow the backspace through in the text box, or to only capture it in the main svg of the graph?
My code:
var BACKSPACE_KEY = 8;
d3.select(window).on("keydown", function() {
switch (d3.event.keyCode) {
case BACKSPACE_KEY:
// So that the browser doesn't go back
d3.event.preventDefault();
// Clean up my graph with the backspace key
// ...
}
})
A fiddle demonstrating the problem: https://jsfiddle.net/5pamrn3m/
javascript d3.js
add a comment |
I've got an interactive graph built with d3, and I capture the backspace key to remove elements from the graph. However, I also have a text box on the page, and I'd like to be able to use backspace while typing. Is there a way to allow the backspace through in the text box, or to only capture it in the main svg of the graph?
My code:
var BACKSPACE_KEY = 8;
d3.select(window).on("keydown", function() {
switch (d3.event.keyCode) {
case BACKSPACE_KEY:
// So that the browser doesn't go back
d3.event.preventDefault();
// Clean up my graph with the backspace key
// ...
}
})
A fiddle demonstrating the problem: https://jsfiddle.net/5pamrn3m/
javascript d3.js
1
I might be being very stupid here, but just not using preventDefault seems to do the trick
– TKoL
Oct 17 '17 at 14:08
1
@TKoL yeah, but he wants to preventDefault when event occurs outside of input and in some browsers (in most of them until recently) backspace navigated browser to previous page.
– Walk
Oct 17 '17 at 14:17
I guess I've got the site set to no let you leave without saving, so that would probably work even if the browser did try to send you back. But yeah, Walk is right about the reasons why it's there
– Kyle Heuton
Oct 17 '17 at 15:09
add a comment |
I've got an interactive graph built with d3, and I capture the backspace key to remove elements from the graph. However, I also have a text box on the page, and I'd like to be able to use backspace while typing. Is there a way to allow the backspace through in the text box, or to only capture it in the main svg of the graph?
My code:
var BACKSPACE_KEY = 8;
d3.select(window).on("keydown", function() {
switch (d3.event.keyCode) {
case BACKSPACE_KEY:
// So that the browser doesn't go back
d3.event.preventDefault();
// Clean up my graph with the backspace key
// ...
}
})
A fiddle demonstrating the problem: https://jsfiddle.net/5pamrn3m/
javascript d3.js
I've got an interactive graph built with d3, and I capture the backspace key to remove elements from the graph. However, I also have a text box on the page, and I'd like to be able to use backspace while typing. Is there a way to allow the backspace through in the text box, or to only capture it in the main svg of the graph?
My code:
var BACKSPACE_KEY = 8;
d3.select(window).on("keydown", function() {
switch (d3.event.keyCode) {
case BACKSPACE_KEY:
// So that the browser doesn't go back
d3.event.preventDefault();
// Clean up my graph with the backspace key
// ...
}
})
A fiddle demonstrating the problem: https://jsfiddle.net/5pamrn3m/
javascript d3.js
javascript d3.js
asked Oct 17 '17 at 14:06
Kyle HeutonKyle Heuton
5,20032540
5,20032540
1
I might be being very stupid here, but just not using preventDefault seems to do the trick
– TKoL
Oct 17 '17 at 14:08
1
@TKoL yeah, but he wants to preventDefault when event occurs outside of input and in some browsers (in most of them until recently) backspace navigated browser to previous page.
– Walk
Oct 17 '17 at 14:17
I guess I've got the site set to no let you leave without saving, so that would probably work even if the browser did try to send you back. But yeah, Walk is right about the reasons why it's there
– Kyle Heuton
Oct 17 '17 at 15:09
add a comment |
1
I might be being very stupid here, but just not using preventDefault seems to do the trick
– TKoL
Oct 17 '17 at 14:08
1
@TKoL yeah, but he wants to preventDefault when event occurs outside of input and in some browsers (in most of them until recently) backspace navigated browser to previous page.
– Walk
Oct 17 '17 at 14:17
I guess I've got the site set to no let you leave without saving, so that would probably work even if the browser did try to send you back. But yeah, Walk is right about the reasons why it's there
– Kyle Heuton
Oct 17 '17 at 15:09
1
1
I might be being very stupid here, but just not using preventDefault seems to do the trick
– TKoL
Oct 17 '17 at 14:08
I might be being very stupid here, but just not using preventDefault seems to do the trick
– TKoL
Oct 17 '17 at 14:08
1
1
@TKoL yeah, but he wants to preventDefault when event occurs outside of input and in some browsers (in most of them until recently) backspace navigated browser to previous page.
– Walk
Oct 17 '17 at 14:17
@TKoL yeah, but he wants to preventDefault when event occurs outside of input and in some browsers (in most of them until recently) backspace navigated browser to previous page.
– Walk
Oct 17 '17 at 14:17
I guess I've got the site set to no let you leave without saving, so that would probably work even if the browser did try to send you back. But yeah, Walk is right about the reasons why it's there
– Kyle Heuton
Oct 17 '17 at 15:09
I guess I've got the site set to no let you leave without saving, so that would probably work even if the browser did try to send you back. But yeah, Walk is right about the reasons why it's there
– Kyle Heuton
Oct 17 '17 at 15:09
add a comment |
4 Answers
4
active
oldest
votes
Use event taget (MDN) to check that it was <input>
to be able to break the procedure:
var BACKSPACE_KEY = 8;
d3.select(window).on("keydown", function() {
switch (d3.event.keyCode) {
case BACKSPACE_KEY:
// So that the browser doesn't go back
d3.event.preventDefault();
// BACKSPACE_KEY was fired in <input id="textbox">
if(d3.event.target.id === 'textbox') {
break;
}
// Clean up my graph with the backspace key
// ...
}
})
I used id
due to your fiddle, but the condition could be more generic
// BACKSPACE_KEY was fired in any <input>
if(d3.event.target.nodeName.toLowerCase() === 'input') {
break;
}
add a comment |
Use event.stopPropagation();
https://jsfiddle.net/x64hcktt/
More about event propagation: https://developer.mozilla.org/en-US/docs/Web/API/Document_Object_Model/Examples#Example_5:_Event_Propagation
add a comment |
You can prevent the default behaviour of the backspace key on the whole page except a certain input if you check for the focused element's id using :
if (document.activeElement.id !== "textbox")
d3.event.preventDefault();
See this Fiddle
add a comment |
Here is another way to do it:
d3.select('input').on('keydown', () => d3.event.stopPropagation())
This stops the keydown event from propagating to other handlers.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f46792160%2fhow-do-i-allow-backspace-in-a-textbox-while-capturing-keydown-events%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
Use event taget (MDN) to check that it was <input>
to be able to break the procedure:
var BACKSPACE_KEY = 8;
d3.select(window).on("keydown", function() {
switch (d3.event.keyCode) {
case BACKSPACE_KEY:
// So that the browser doesn't go back
d3.event.preventDefault();
// BACKSPACE_KEY was fired in <input id="textbox">
if(d3.event.target.id === 'textbox') {
break;
}
// Clean up my graph with the backspace key
// ...
}
})
I used id
due to your fiddle, but the condition could be more generic
// BACKSPACE_KEY was fired in any <input>
if(d3.event.target.nodeName.toLowerCase() === 'input') {
break;
}
add a comment |
Use event taget (MDN) to check that it was <input>
to be able to break the procedure:
var BACKSPACE_KEY = 8;
d3.select(window).on("keydown", function() {
switch (d3.event.keyCode) {
case BACKSPACE_KEY:
// So that the browser doesn't go back
d3.event.preventDefault();
// BACKSPACE_KEY was fired in <input id="textbox">
if(d3.event.target.id === 'textbox') {
break;
}
// Clean up my graph with the backspace key
// ...
}
})
I used id
due to your fiddle, but the condition could be more generic
// BACKSPACE_KEY was fired in any <input>
if(d3.event.target.nodeName.toLowerCase() === 'input') {
break;
}
add a comment |
Use event taget (MDN) to check that it was <input>
to be able to break the procedure:
var BACKSPACE_KEY = 8;
d3.select(window).on("keydown", function() {
switch (d3.event.keyCode) {
case BACKSPACE_KEY:
// So that the browser doesn't go back
d3.event.preventDefault();
// BACKSPACE_KEY was fired in <input id="textbox">
if(d3.event.target.id === 'textbox') {
break;
}
// Clean up my graph with the backspace key
// ...
}
})
I used id
due to your fiddle, but the condition could be more generic
// BACKSPACE_KEY was fired in any <input>
if(d3.event.target.nodeName.toLowerCase() === 'input') {
break;
}
Use event taget (MDN) to check that it was <input>
to be able to break the procedure:
var BACKSPACE_KEY = 8;
d3.select(window).on("keydown", function() {
switch (d3.event.keyCode) {
case BACKSPACE_KEY:
// So that the browser doesn't go back
d3.event.preventDefault();
// BACKSPACE_KEY was fired in <input id="textbox">
if(d3.event.target.id === 'textbox') {
break;
}
// Clean up my graph with the backspace key
// ...
}
})
I used id
due to your fiddle, but the condition could be more generic
// BACKSPACE_KEY was fired in any <input>
if(d3.event.target.nodeName.toLowerCase() === 'input') {
break;
}
edited Oct 17 '17 at 14:20
answered Oct 17 '17 at 14:09


dhiltdhilt
8,07742143
8,07742143
add a comment |
add a comment |
Use event.stopPropagation();
https://jsfiddle.net/x64hcktt/
More about event propagation: https://developer.mozilla.org/en-US/docs/Web/API/Document_Object_Model/Examples#Example_5:_Event_Propagation
add a comment |
Use event.stopPropagation();
https://jsfiddle.net/x64hcktt/
More about event propagation: https://developer.mozilla.org/en-US/docs/Web/API/Document_Object_Model/Examples#Example_5:_Event_Propagation
add a comment |
Use event.stopPropagation();
https://jsfiddle.net/x64hcktt/
More about event propagation: https://developer.mozilla.org/en-US/docs/Web/API/Document_Object_Model/Examples#Example_5:_Event_Propagation
Use event.stopPropagation();
https://jsfiddle.net/x64hcktt/
More about event propagation: https://developer.mozilla.org/en-US/docs/Web/API/Document_Object_Model/Examples#Example_5:_Event_Propagation
answered Oct 17 '17 at 14:12
WalkWalk
596313
596313
add a comment |
add a comment |
You can prevent the default behaviour of the backspace key on the whole page except a certain input if you check for the focused element's id using :
if (document.activeElement.id !== "textbox")
d3.event.preventDefault();
See this Fiddle
add a comment |
You can prevent the default behaviour of the backspace key on the whole page except a certain input if you check for the focused element's id using :
if (document.activeElement.id !== "textbox")
d3.event.preventDefault();
See this Fiddle
add a comment |
You can prevent the default behaviour of the backspace key on the whole page except a certain input if you check for the focused element's id using :
if (document.activeElement.id !== "textbox")
d3.event.preventDefault();
See this Fiddle
You can prevent the default behaviour of the backspace key on the whole page except a certain input if you check for the focused element's id using :
if (document.activeElement.id !== "textbox")
d3.event.preventDefault();
See this Fiddle
answered Oct 17 '17 at 14:17
Paul-EtiennePaul-Etienne
573616
573616
add a comment |
add a comment |
Here is another way to do it:
d3.select('input').on('keydown', () => d3.event.stopPropagation())
This stops the keydown event from propagating to other handlers.
add a comment |
Here is another way to do it:
d3.select('input').on('keydown', () => d3.event.stopPropagation())
This stops the keydown event from propagating to other handlers.
add a comment |
Here is another way to do it:
d3.select('input').on('keydown', () => d3.event.stopPropagation())
This stops the keydown event from propagating to other handlers.
Here is another way to do it:
d3.select('input').on('keydown', () => d3.event.stopPropagation())
This stops the keydown event from propagating to other handlers.
answered Jan 2 at 3:40
mvrmvr
30735
30735
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f46792160%2fhow-do-i-allow-backspace-in-a-textbox-while-capturing-keydown-events%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
gq CuDkc6GGyk isTlwLeiYzv7mvxLYcq9MXiHHF,M,NVA,7L9Uuw 57PYP
1
I might be being very stupid here, but just not using preventDefault seems to do the trick
– TKoL
Oct 17 '17 at 14:08
1
@TKoL yeah, but he wants to preventDefault when event occurs outside of input and in some browsers (in most of them until recently) backspace navigated browser to previous page.
– Walk
Oct 17 '17 at 14:17
I guess I've got the site set to no let you leave without saving, so that would probably work even if the browser did try to send you back. But yeah, Walk is right about the reasons why it's there
– Kyle Heuton
Oct 17 '17 at 15:09