c++ Shared library + QList assigment issue
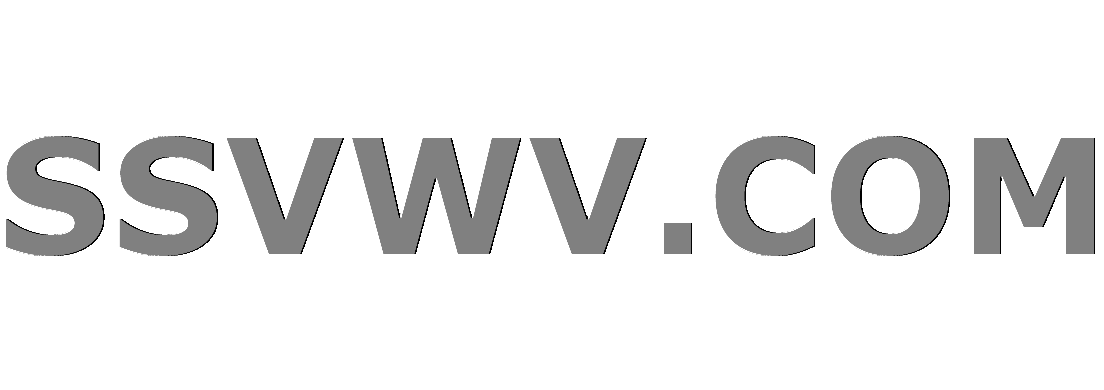
Multi tool use
I've built a shared library using pimpl idiom.
Below there are a few classes related to the problem I'm facing:
class PlotPrivate;
class RESTAPILIB_EXPORT Plot : public IFarmObject
{
using IFarmObject::IFarmObject;
Q_DISABLE_COPY(Plot)
Q_OBJECT
...
Q_PROPERTY(QList<QGeoCoordinate> coordinates READ coordinates WRITE setCoordinates NOTIFY coordinatesChanged)
...
public:
void setCoordinates(const QList<QGeoCoordinate> &coordinates);
QList<QGeoCoordinate> coordinates() const;
Q_INVOKABLE QVariantList coordinatesQml() const;
QPolygonF coordinatesPolygon() const;
...
signals:
void coordinatesChanged(QList<QGeoCoordinate> coordinates);
private:
Q_DECLARE_PRIVATE(Plot)
};
...
class PlotPrivate : public IFarmObjectPrivate
{
public:
PlotPrivate(Plot *q, const QString &id, IObject::ObjectType type = IObject::PLOT);
...
public:
QList<QGeoCoordinate> m_coordinates;
Q_DECLARE_PUBLIC(Plot)
};
...
Plot::Plot(const QString &id)
: IFarmObject(new PlotPrivate(this, id))
{
}
...
void Plot::setCoordinates(const QList<QGeoCoordinate> &coordinates)
{
Q_D(Plot);
d->m_coordinates = coordinates;
d->m_area = 0.0;
if (d->m_coordinates.size() > 2)
d->m_area = abs(getArea(coordinatesPolygon()));
//TODO: render m_image
emit coordinatesChanged(d->m_coordinates);
emit areaChanged(d->m_area);
}
...
const QList<QGeoCoordinate> GeoCoordinatesModel::coordinates() const
{
QList<QGeoCoordinate> lst;
if (m_lst.size() > 2)
{
foreach (GeoCoordinateEntry *e, m_lst) {
lst << e->coordinate();
}
}
return lst;
}
...
void wgFarmPlotEditor::savePlot(Plot *plot)
{
Plot *p = new Plot(plot);
QList<QGeoCoordinate> coords = p_coordinatesModel->coordinates();
p->setCoordinates(coords);
newRequestUpdt(p);
p->deleteLater();
p_coordinatesModel->resetModel();
}
In the shared library, Plot is a pointer.
When the slot wgFarmPlotEditor::savePlot() is called, my application crashes on the following part: p->setCoordinates(coords);
I'm trying to understand why this is happening.
I believe theres soemthing to do with implicit sharing, but I dont know why.
Any thoughts?
Note: I've hidden part of code for better reading..
Note2: IFarmObject and IFarmObjectPrivate, both inherits from QObject, also both have their corresponding d_ptr and q_ptr objects.
Note3: I wrote classes following this guide: https://wiki.qt.io/D-Pointer
Note4: wgFarmPlotEditor class
is in another shared library.. not sure if it makes any difference.
Below there is the stack trace of the crash:
1 QGenericAtomicOps<QAtomicOpsBySize<4>>::load<int> qgenericatomic.h 90 0xf2e29d6
2 QBasicAtomicInteger<int>::load qbasicatomic.h 103 0xf2e5dc0
3 QtPrivate::RefCount::deref qrefcount.h 66 0xf2e4701
4 QList<QGeoCoordinate>::~QList<QGeoCoordinate> qlist.h 826 0xf3215d0
5 QList<QGeoCoordinate>::operator= qlist.h 506 0xf3216e5
6 Plot::setCoordinates plot.cpp 40 0xf31f463
7 wgFarmPlotEditor::savePlot wgfarmploteditor.cpp 77 0x3b66417
8 wgFarmPlotEditor::qt_static_metacall moc_wgfarmploteditor.cpp 98 0x3b7341e
9 wgFarmPlotEditor::qt_metacall moc_wgfarmploteditor.cpp 154 0x3b7335a
10 QMetaObject::metacall qmetaobject.cpp 302 0x665b7780
11 QQmlObjectOrGadget::metacall qqmlpropertycache.cpp 1733 0x429eaa1
12 CallMethod qv4qobjectwrapper.cpp 1177 0x41abe23
13 CallPrecise qv4qobjectwrapper.cpp 1437 0x41ac7bc
14 QV4::QObjectMethod::callInternal qv4qobjectwrapper.cpp 1975 0x41aa65a
15 QV4::QObjectMethod::call qv4qobjectwrapper.cpp 1913 0x41aa2de
16 QV4::FunctionObject::call qv4functionobject_p.h 163 0x3fd0714
17 QV4::Runtime::method_callProperty qv4runtime.cpp 1062 0x41d06a6
18 QV4::Moth::VME::exec qv4vme_moth.cpp 800 0x41bfd32
19 QV4::Moth::VME::exec qv4vme_moth_p.h 72 0x3fd5ad0
20 QV4::Function::call qv4function_p.h 72 0x3fd06d0
... <More>
qt qt5
add a comment |
I've built a shared library using pimpl idiom.
Below there are a few classes related to the problem I'm facing:
class PlotPrivate;
class RESTAPILIB_EXPORT Plot : public IFarmObject
{
using IFarmObject::IFarmObject;
Q_DISABLE_COPY(Plot)
Q_OBJECT
...
Q_PROPERTY(QList<QGeoCoordinate> coordinates READ coordinates WRITE setCoordinates NOTIFY coordinatesChanged)
...
public:
void setCoordinates(const QList<QGeoCoordinate> &coordinates);
QList<QGeoCoordinate> coordinates() const;
Q_INVOKABLE QVariantList coordinatesQml() const;
QPolygonF coordinatesPolygon() const;
...
signals:
void coordinatesChanged(QList<QGeoCoordinate> coordinates);
private:
Q_DECLARE_PRIVATE(Plot)
};
...
class PlotPrivate : public IFarmObjectPrivate
{
public:
PlotPrivate(Plot *q, const QString &id, IObject::ObjectType type = IObject::PLOT);
...
public:
QList<QGeoCoordinate> m_coordinates;
Q_DECLARE_PUBLIC(Plot)
};
...
Plot::Plot(const QString &id)
: IFarmObject(new PlotPrivate(this, id))
{
}
...
void Plot::setCoordinates(const QList<QGeoCoordinate> &coordinates)
{
Q_D(Plot);
d->m_coordinates = coordinates;
d->m_area = 0.0;
if (d->m_coordinates.size() > 2)
d->m_area = abs(getArea(coordinatesPolygon()));
//TODO: render m_image
emit coordinatesChanged(d->m_coordinates);
emit areaChanged(d->m_area);
}
...
const QList<QGeoCoordinate> GeoCoordinatesModel::coordinates() const
{
QList<QGeoCoordinate> lst;
if (m_lst.size() > 2)
{
foreach (GeoCoordinateEntry *e, m_lst) {
lst << e->coordinate();
}
}
return lst;
}
...
void wgFarmPlotEditor::savePlot(Plot *plot)
{
Plot *p = new Plot(plot);
QList<QGeoCoordinate> coords = p_coordinatesModel->coordinates();
p->setCoordinates(coords);
newRequestUpdt(p);
p->deleteLater();
p_coordinatesModel->resetModel();
}
In the shared library, Plot is a pointer.
When the slot wgFarmPlotEditor::savePlot() is called, my application crashes on the following part: p->setCoordinates(coords);
I'm trying to understand why this is happening.
I believe theres soemthing to do with implicit sharing, but I dont know why.
Any thoughts?
Note: I've hidden part of code for better reading..
Note2: IFarmObject and IFarmObjectPrivate, both inherits from QObject, also both have their corresponding d_ptr and q_ptr objects.
Note3: I wrote classes following this guide: https://wiki.qt.io/D-Pointer
Note4: wgFarmPlotEditor class
is in another shared library.. not sure if it makes any difference.
Below there is the stack trace of the crash:
1 QGenericAtomicOps<QAtomicOpsBySize<4>>::load<int> qgenericatomic.h 90 0xf2e29d6
2 QBasicAtomicInteger<int>::load qbasicatomic.h 103 0xf2e5dc0
3 QtPrivate::RefCount::deref qrefcount.h 66 0xf2e4701
4 QList<QGeoCoordinate>::~QList<QGeoCoordinate> qlist.h 826 0xf3215d0
5 QList<QGeoCoordinate>::operator= qlist.h 506 0xf3216e5
6 Plot::setCoordinates plot.cpp 40 0xf31f463
7 wgFarmPlotEditor::savePlot wgfarmploteditor.cpp 77 0x3b66417
8 wgFarmPlotEditor::qt_static_metacall moc_wgfarmploteditor.cpp 98 0x3b7341e
9 wgFarmPlotEditor::qt_metacall moc_wgfarmploteditor.cpp 154 0x3b7335a
10 QMetaObject::metacall qmetaobject.cpp 302 0x665b7780
11 QQmlObjectOrGadget::metacall qqmlpropertycache.cpp 1733 0x429eaa1
12 CallMethod qv4qobjectwrapper.cpp 1177 0x41abe23
13 CallPrecise qv4qobjectwrapper.cpp 1437 0x41ac7bc
14 QV4::QObjectMethod::callInternal qv4qobjectwrapper.cpp 1975 0x41aa65a
15 QV4::QObjectMethod::call qv4qobjectwrapper.cpp 1913 0x41aa2de
16 QV4::FunctionObject::call qv4functionobject_p.h 163 0x3fd0714
17 QV4::Runtime::method_callProperty qv4runtime.cpp 1062 0x41d06a6
18 QV4::Moth::VME::exec qv4vme_moth.cpp 800 0x41bfd32
19 QV4::Moth::VME::exec qv4vme_moth_p.h 72 0x3fd5ad0
20 QV4::Function::call qv4function_p.h 72 0x3fd06d0
... <More>
qt qt5
2
Did you try to find the problem using debuger?
– folibis
Jan 2 at 5:40
It would be useful to see howPlot::setCoordinates()
andPlot::Plot(Plot *p)
look like.
– vahancho
Jan 2 at 8:39
@folibis Yes, the crash happens when the QList gets the destructor called, but I can't figure out why!
– Alex Soletti
Jan 2 at 12:53
@vahancho added!
– Alex Soletti
Jan 2 at 12:54
add a comment |
I've built a shared library using pimpl idiom.
Below there are a few classes related to the problem I'm facing:
class PlotPrivate;
class RESTAPILIB_EXPORT Plot : public IFarmObject
{
using IFarmObject::IFarmObject;
Q_DISABLE_COPY(Plot)
Q_OBJECT
...
Q_PROPERTY(QList<QGeoCoordinate> coordinates READ coordinates WRITE setCoordinates NOTIFY coordinatesChanged)
...
public:
void setCoordinates(const QList<QGeoCoordinate> &coordinates);
QList<QGeoCoordinate> coordinates() const;
Q_INVOKABLE QVariantList coordinatesQml() const;
QPolygonF coordinatesPolygon() const;
...
signals:
void coordinatesChanged(QList<QGeoCoordinate> coordinates);
private:
Q_DECLARE_PRIVATE(Plot)
};
...
class PlotPrivate : public IFarmObjectPrivate
{
public:
PlotPrivate(Plot *q, const QString &id, IObject::ObjectType type = IObject::PLOT);
...
public:
QList<QGeoCoordinate> m_coordinates;
Q_DECLARE_PUBLIC(Plot)
};
...
Plot::Plot(const QString &id)
: IFarmObject(new PlotPrivate(this, id))
{
}
...
void Plot::setCoordinates(const QList<QGeoCoordinate> &coordinates)
{
Q_D(Plot);
d->m_coordinates = coordinates;
d->m_area = 0.0;
if (d->m_coordinates.size() > 2)
d->m_area = abs(getArea(coordinatesPolygon()));
//TODO: render m_image
emit coordinatesChanged(d->m_coordinates);
emit areaChanged(d->m_area);
}
...
const QList<QGeoCoordinate> GeoCoordinatesModel::coordinates() const
{
QList<QGeoCoordinate> lst;
if (m_lst.size() > 2)
{
foreach (GeoCoordinateEntry *e, m_lst) {
lst << e->coordinate();
}
}
return lst;
}
...
void wgFarmPlotEditor::savePlot(Plot *plot)
{
Plot *p = new Plot(plot);
QList<QGeoCoordinate> coords = p_coordinatesModel->coordinates();
p->setCoordinates(coords);
newRequestUpdt(p);
p->deleteLater();
p_coordinatesModel->resetModel();
}
In the shared library, Plot is a pointer.
When the slot wgFarmPlotEditor::savePlot() is called, my application crashes on the following part: p->setCoordinates(coords);
I'm trying to understand why this is happening.
I believe theres soemthing to do with implicit sharing, but I dont know why.
Any thoughts?
Note: I've hidden part of code for better reading..
Note2: IFarmObject and IFarmObjectPrivate, both inherits from QObject, also both have their corresponding d_ptr and q_ptr objects.
Note3: I wrote classes following this guide: https://wiki.qt.io/D-Pointer
Note4: wgFarmPlotEditor class
is in another shared library.. not sure if it makes any difference.
Below there is the stack trace of the crash:
1 QGenericAtomicOps<QAtomicOpsBySize<4>>::load<int> qgenericatomic.h 90 0xf2e29d6
2 QBasicAtomicInteger<int>::load qbasicatomic.h 103 0xf2e5dc0
3 QtPrivate::RefCount::deref qrefcount.h 66 0xf2e4701
4 QList<QGeoCoordinate>::~QList<QGeoCoordinate> qlist.h 826 0xf3215d0
5 QList<QGeoCoordinate>::operator= qlist.h 506 0xf3216e5
6 Plot::setCoordinates plot.cpp 40 0xf31f463
7 wgFarmPlotEditor::savePlot wgfarmploteditor.cpp 77 0x3b66417
8 wgFarmPlotEditor::qt_static_metacall moc_wgfarmploteditor.cpp 98 0x3b7341e
9 wgFarmPlotEditor::qt_metacall moc_wgfarmploteditor.cpp 154 0x3b7335a
10 QMetaObject::metacall qmetaobject.cpp 302 0x665b7780
11 QQmlObjectOrGadget::metacall qqmlpropertycache.cpp 1733 0x429eaa1
12 CallMethod qv4qobjectwrapper.cpp 1177 0x41abe23
13 CallPrecise qv4qobjectwrapper.cpp 1437 0x41ac7bc
14 QV4::QObjectMethod::callInternal qv4qobjectwrapper.cpp 1975 0x41aa65a
15 QV4::QObjectMethod::call qv4qobjectwrapper.cpp 1913 0x41aa2de
16 QV4::FunctionObject::call qv4functionobject_p.h 163 0x3fd0714
17 QV4::Runtime::method_callProperty qv4runtime.cpp 1062 0x41d06a6
18 QV4::Moth::VME::exec qv4vme_moth.cpp 800 0x41bfd32
19 QV4::Moth::VME::exec qv4vme_moth_p.h 72 0x3fd5ad0
20 QV4::Function::call qv4function_p.h 72 0x3fd06d0
... <More>
qt qt5
I've built a shared library using pimpl idiom.
Below there are a few classes related to the problem I'm facing:
class PlotPrivate;
class RESTAPILIB_EXPORT Plot : public IFarmObject
{
using IFarmObject::IFarmObject;
Q_DISABLE_COPY(Plot)
Q_OBJECT
...
Q_PROPERTY(QList<QGeoCoordinate> coordinates READ coordinates WRITE setCoordinates NOTIFY coordinatesChanged)
...
public:
void setCoordinates(const QList<QGeoCoordinate> &coordinates);
QList<QGeoCoordinate> coordinates() const;
Q_INVOKABLE QVariantList coordinatesQml() const;
QPolygonF coordinatesPolygon() const;
...
signals:
void coordinatesChanged(QList<QGeoCoordinate> coordinates);
private:
Q_DECLARE_PRIVATE(Plot)
};
...
class PlotPrivate : public IFarmObjectPrivate
{
public:
PlotPrivate(Plot *q, const QString &id, IObject::ObjectType type = IObject::PLOT);
...
public:
QList<QGeoCoordinate> m_coordinates;
Q_DECLARE_PUBLIC(Plot)
};
...
Plot::Plot(const QString &id)
: IFarmObject(new PlotPrivate(this, id))
{
}
...
void Plot::setCoordinates(const QList<QGeoCoordinate> &coordinates)
{
Q_D(Plot);
d->m_coordinates = coordinates;
d->m_area = 0.0;
if (d->m_coordinates.size() > 2)
d->m_area = abs(getArea(coordinatesPolygon()));
//TODO: render m_image
emit coordinatesChanged(d->m_coordinates);
emit areaChanged(d->m_area);
}
...
const QList<QGeoCoordinate> GeoCoordinatesModel::coordinates() const
{
QList<QGeoCoordinate> lst;
if (m_lst.size() > 2)
{
foreach (GeoCoordinateEntry *e, m_lst) {
lst << e->coordinate();
}
}
return lst;
}
...
void wgFarmPlotEditor::savePlot(Plot *plot)
{
Plot *p = new Plot(plot);
QList<QGeoCoordinate> coords = p_coordinatesModel->coordinates();
p->setCoordinates(coords);
newRequestUpdt(p);
p->deleteLater();
p_coordinatesModel->resetModel();
}
In the shared library, Plot is a pointer.
When the slot wgFarmPlotEditor::savePlot() is called, my application crashes on the following part: p->setCoordinates(coords);
I'm trying to understand why this is happening.
I believe theres soemthing to do with implicit sharing, but I dont know why.
Any thoughts?
Note: I've hidden part of code for better reading..
Note2: IFarmObject and IFarmObjectPrivate, both inherits from QObject, also both have their corresponding d_ptr and q_ptr objects.
Note3: I wrote classes following this guide: https://wiki.qt.io/D-Pointer
Note4: wgFarmPlotEditor class
is in another shared library.. not sure if it makes any difference.
Below there is the stack trace of the crash:
1 QGenericAtomicOps<QAtomicOpsBySize<4>>::load<int> qgenericatomic.h 90 0xf2e29d6
2 QBasicAtomicInteger<int>::load qbasicatomic.h 103 0xf2e5dc0
3 QtPrivate::RefCount::deref qrefcount.h 66 0xf2e4701
4 QList<QGeoCoordinate>::~QList<QGeoCoordinate> qlist.h 826 0xf3215d0
5 QList<QGeoCoordinate>::operator= qlist.h 506 0xf3216e5
6 Plot::setCoordinates plot.cpp 40 0xf31f463
7 wgFarmPlotEditor::savePlot wgfarmploteditor.cpp 77 0x3b66417
8 wgFarmPlotEditor::qt_static_metacall moc_wgfarmploteditor.cpp 98 0x3b7341e
9 wgFarmPlotEditor::qt_metacall moc_wgfarmploteditor.cpp 154 0x3b7335a
10 QMetaObject::metacall qmetaobject.cpp 302 0x665b7780
11 QQmlObjectOrGadget::metacall qqmlpropertycache.cpp 1733 0x429eaa1
12 CallMethod qv4qobjectwrapper.cpp 1177 0x41abe23
13 CallPrecise qv4qobjectwrapper.cpp 1437 0x41ac7bc
14 QV4::QObjectMethod::callInternal qv4qobjectwrapper.cpp 1975 0x41aa65a
15 QV4::QObjectMethod::call qv4qobjectwrapper.cpp 1913 0x41aa2de
16 QV4::FunctionObject::call qv4functionobject_p.h 163 0x3fd0714
17 QV4::Runtime::method_callProperty qv4runtime.cpp 1062 0x41d06a6
18 QV4::Moth::VME::exec qv4vme_moth.cpp 800 0x41bfd32
19 QV4::Moth::VME::exec qv4vme_moth_p.h 72 0x3fd5ad0
20 QV4::Function::call qv4function_p.h 72 0x3fd06d0
... <More>
qt qt5
qt qt5
edited Jan 2 at 12:52
Alex Soletti
asked Jan 2 at 3:51


Alex SolettiAlex Soletti
135
135
2
Did you try to find the problem using debuger?
– folibis
Jan 2 at 5:40
It would be useful to see howPlot::setCoordinates()
andPlot::Plot(Plot *p)
look like.
– vahancho
Jan 2 at 8:39
@folibis Yes, the crash happens when the QList gets the destructor called, but I can't figure out why!
– Alex Soletti
Jan 2 at 12:53
@vahancho added!
– Alex Soletti
Jan 2 at 12:54
add a comment |
2
Did you try to find the problem using debuger?
– folibis
Jan 2 at 5:40
It would be useful to see howPlot::setCoordinates()
andPlot::Plot(Plot *p)
look like.
– vahancho
Jan 2 at 8:39
@folibis Yes, the crash happens when the QList gets the destructor called, but I can't figure out why!
– Alex Soletti
Jan 2 at 12:53
@vahancho added!
– Alex Soletti
Jan 2 at 12:54
2
2
Did you try to find the problem using debuger?
– folibis
Jan 2 at 5:40
Did you try to find the problem using debuger?
– folibis
Jan 2 at 5:40
It would be useful to see how
Plot::setCoordinates()
and Plot::Plot(Plot *p)
look like.– vahancho
Jan 2 at 8:39
It would be useful to see how
Plot::setCoordinates()
and Plot::Plot(Plot *p)
look like.– vahancho
Jan 2 at 8:39
@folibis Yes, the crash happens when the QList gets the destructor called, but I can't figure out why!
– Alex Soletti
Jan 2 at 12:53
@folibis Yes, the crash happens when the QList gets the destructor called, but I can't figure out why!
– Alex Soletti
Jan 2 at 12:53
@vahancho added!
– Alex Soletti
Jan 2 at 12:54
@vahancho added!
– Alex Soletti
Jan 2 at 12:54
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54001007%2fc-shared-library-qlist-assigment-issue%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54001007%2fc-shared-library-qlist-assigment-issue%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VCCv614 bwGqjmkEClBOw,e6JOwr0,y9uzOy31JImiZA OJe
2
Did you try to find the problem using debuger?
– folibis
Jan 2 at 5:40
It would be useful to see how
Plot::setCoordinates()
andPlot::Plot(Plot *p)
look like.– vahancho
Jan 2 at 8:39
@folibis Yes, the crash happens when the QList gets the destructor called, but I can't figure out why!
– Alex Soletti
Jan 2 at 12:53
@vahancho added!
– Alex Soletti
Jan 2 at 12:54