How to filter an json object by another object - Javascript
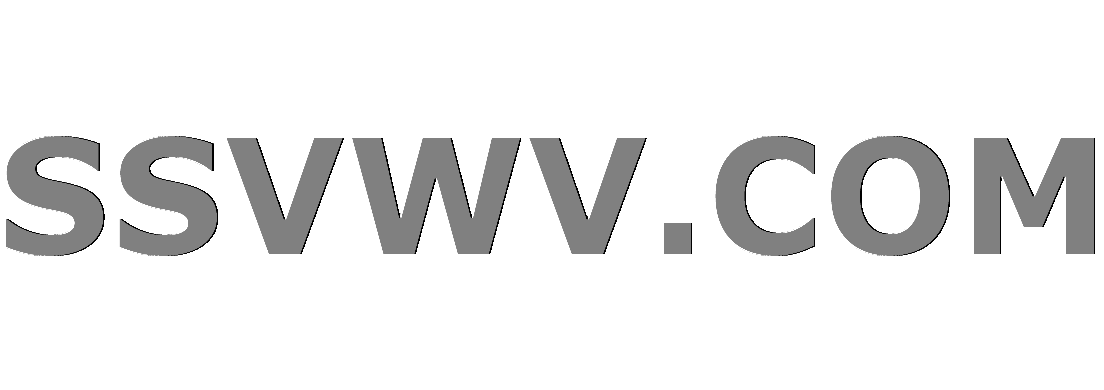
Multi tool use
How can I filter a json object with another object in Javascript (cannot use JQuery)
This is my JSON object
var jsondata = [
{
"firstName": "Sam",
"lastName": "Jones",
"age": "10"
},
{
"firstName": "Sam1",
"lastName": "Jones1",
"age": "10"
},
{
"firstName": "Sam2",
"lastName": "Jones2",
"age": "12"
},
{
"firstName": "Sam3",
"lastName": "Jones3",
"age": "13"
},
{
"firstName": "Sam4",
"lastName": "Jones4",
"age": "14"
},
{
"firstName": "Sam5",
"lastName": "Jones5",
"age": "15"
},
{
"firstName": "Sam",
"lastName": "Jones11",
"age": "16"
},
{
"firstName": "Sam6",
"lastName": "Jones6",
"age": "17"
},
{
"firstName": "Sam7",
"lastName": "Jones7",
"age": "18"
},
{
"firstName": "Sam8",
"lastName": "Jones8",
"age": "19"
},
{
"firstName": "Sam9",
"lastName": "Jones9",
"age": "20"
},
{
"firstName": "Sam10",
"lastName": "Jones10",
"age": "21"
},
{
"firstName": "Sam11",
"lastName": "Jones11",
"age": "22"
},
{
"firstName": "Sam12",
"lastName": "Jones12",
"age": "23"
}
]
The above is the filterable object, now below is the object for filtering
var filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}]
I need to check the the fields of json object (jsondata) like firstName, lastName and match the values of both objects.
Suppose firstname field of jsondata will be compared with id of filterArray and their values will be matched. Alongside lastname will also be compared.
FInally I want to store the filtered object (after eliminating those which are not in filter in a variable). How can I realize this functionality? Please help.
I need to implement this using plain JS, I cannot use Jquery.
javascript json
add a comment |
How can I filter a json object with another object in Javascript (cannot use JQuery)
This is my JSON object
var jsondata = [
{
"firstName": "Sam",
"lastName": "Jones",
"age": "10"
},
{
"firstName": "Sam1",
"lastName": "Jones1",
"age": "10"
},
{
"firstName": "Sam2",
"lastName": "Jones2",
"age": "12"
},
{
"firstName": "Sam3",
"lastName": "Jones3",
"age": "13"
},
{
"firstName": "Sam4",
"lastName": "Jones4",
"age": "14"
},
{
"firstName": "Sam5",
"lastName": "Jones5",
"age": "15"
},
{
"firstName": "Sam",
"lastName": "Jones11",
"age": "16"
},
{
"firstName": "Sam6",
"lastName": "Jones6",
"age": "17"
},
{
"firstName": "Sam7",
"lastName": "Jones7",
"age": "18"
},
{
"firstName": "Sam8",
"lastName": "Jones8",
"age": "19"
},
{
"firstName": "Sam9",
"lastName": "Jones9",
"age": "20"
},
{
"firstName": "Sam10",
"lastName": "Jones10",
"age": "21"
},
{
"firstName": "Sam11",
"lastName": "Jones11",
"age": "22"
},
{
"firstName": "Sam12",
"lastName": "Jones12",
"age": "23"
}
]
The above is the filterable object, now below is the object for filtering
var filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}]
I need to check the the fields of json object (jsondata) like firstName, lastName and match the values of both objects.
Suppose firstname field of jsondata will be compared with id of filterArray and their values will be matched. Alongside lastname will also be compared.
FInally I want to store the filtered object (after eliminating those which are not in filter in a variable). How can I realize this functionality? Please help.
I need to implement this using plain JS, I cannot use Jquery.
javascript json
2
The posted question does not appear to include any attempt at all to solve the problem. StackOverflow expects you to try to solve your own problem first, as your attempts help us to better understand what you want. Please edit the question to show what you've tried, so as to illustrate a specific roadblock you're running into a Minimal, Complete, and Verifiable example. For more information, please see How to Ask and take the tour.
– CertainPerformance
Jan 2 at 3:36
I cannot understand how to implement this. That is why I asked the question.
– tommy123
Jan 2 at 3:38
add a comment |
How can I filter a json object with another object in Javascript (cannot use JQuery)
This is my JSON object
var jsondata = [
{
"firstName": "Sam",
"lastName": "Jones",
"age": "10"
},
{
"firstName": "Sam1",
"lastName": "Jones1",
"age": "10"
},
{
"firstName": "Sam2",
"lastName": "Jones2",
"age": "12"
},
{
"firstName": "Sam3",
"lastName": "Jones3",
"age": "13"
},
{
"firstName": "Sam4",
"lastName": "Jones4",
"age": "14"
},
{
"firstName": "Sam5",
"lastName": "Jones5",
"age": "15"
},
{
"firstName": "Sam",
"lastName": "Jones11",
"age": "16"
},
{
"firstName": "Sam6",
"lastName": "Jones6",
"age": "17"
},
{
"firstName": "Sam7",
"lastName": "Jones7",
"age": "18"
},
{
"firstName": "Sam8",
"lastName": "Jones8",
"age": "19"
},
{
"firstName": "Sam9",
"lastName": "Jones9",
"age": "20"
},
{
"firstName": "Sam10",
"lastName": "Jones10",
"age": "21"
},
{
"firstName": "Sam11",
"lastName": "Jones11",
"age": "22"
},
{
"firstName": "Sam12",
"lastName": "Jones12",
"age": "23"
}
]
The above is the filterable object, now below is the object for filtering
var filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}]
I need to check the the fields of json object (jsondata) like firstName, lastName and match the values of both objects.
Suppose firstname field of jsondata will be compared with id of filterArray and their values will be matched. Alongside lastname will also be compared.
FInally I want to store the filtered object (after eliminating those which are not in filter in a variable). How can I realize this functionality? Please help.
I need to implement this using plain JS, I cannot use Jquery.
javascript json
How can I filter a json object with another object in Javascript (cannot use JQuery)
This is my JSON object
var jsondata = [
{
"firstName": "Sam",
"lastName": "Jones",
"age": "10"
},
{
"firstName": "Sam1",
"lastName": "Jones1",
"age": "10"
},
{
"firstName": "Sam2",
"lastName": "Jones2",
"age": "12"
},
{
"firstName": "Sam3",
"lastName": "Jones3",
"age": "13"
},
{
"firstName": "Sam4",
"lastName": "Jones4",
"age": "14"
},
{
"firstName": "Sam5",
"lastName": "Jones5",
"age": "15"
},
{
"firstName": "Sam",
"lastName": "Jones11",
"age": "16"
},
{
"firstName": "Sam6",
"lastName": "Jones6",
"age": "17"
},
{
"firstName": "Sam7",
"lastName": "Jones7",
"age": "18"
},
{
"firstName": "Sam8",
"lastName": "Jones8",
"age": "19"
},
{
"firstName": "Sam9",
"lastName": "Jones9",
"age": "20"
},
{
"firstName": "Sam10",
"lastName": "Jones10",
"age": "21"
},
{
"firstName": "Sam11",
"lastName": "Jones11",
"age": "22"
},
{
"firstName": "Sam12",
"lastName": "Jones12",
"age": "23"
}
]
The above is the filterable object, now below is the object for filtering
var filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}]
I need to check the the fields of json object (jsondata) like firstName, lastName and match the values of both objects.
Suppose firstname field of jsondata will be compared with id of filterArray and their values will be matched. Alongside lastname will also be compared.
FInally I want to store the filtered object (after eliminating those which are not in filter in a variable). How can I realize this functionality? Please help.
I need to implement this using plain JS, I cannot use Jquery.
javascript json
javascript json
asked Jan 2 at 3:36
tommy123tommy123
11618
11618
2
The posted question does not appear to include any attempt at all to solve the problem. StackOverflow expects you to try to solve your own problem first, as your attempts help us to better understand what you want. Please edit the question to show what you've tried, so as to illustrate a specific roadblock you're running into a Minimal, Complete, and Verifiable example. For more information, please see How to Ask and take the tour.
– CertainPerformance
Jan 2 at 3:36
I cannot understand how to implement this. That is why I asked the question.
– tommy123
Jan 2 at 3:38
add a comment |
2
The posted question does not appear to include any attempt at all to solve the problem. StackOverflow expects you to try to solve your own problem first, as your attempts help us to better understand what you want. Please edit the question to show what you've tried, so as to illustrate a specific roadblock you're running into a Minimal, Complete, and Verifiable example. For more information, please see How to Ask and take the tour.
– CertainPerformance
Jan 2 at 3:36
I cannot understand how to implement this. That is why I asked the question.
– tommy123
Jan 2 at 3:38
2
2
The posted question does not appear to include any attempt at all to solve the problem. StackOverflow expects you to try to solve your own problem first, as your attempts help us to better understand what you want. Please edit the question to show what you've tried, so as to illustrate a specific roadblock you're running into a Minimal, Complete, and Verifiable example. For more information, please see How to Ask and take the tour.
– CertainPerformance
Jan 2 at 3:36
The posted question does not appear to include any attempt at all to solve the problem. StackOverflow expects you to try to solve your own problem first, as your attempts help us to better understand what you want. Please edit the question to show what you've tried, so as to illustrate a specific roadblock you're running into a Minimal, Complete, and Verifiable example. For more information, please see How to Ask and take the tour.
– CertainPerformance
Jan 2 at 3:36
I cannot understand how to implement this. That is why I asked the question.
– tommy123
Jan 2 at 3:38
I cannot understand how to implement this. That is why I asked the question.
– tommy123
Jan 2 at 3:38
add a comment |
2 Answers
2
active
oldest
votes
You can use every
and includes
to make a boolean value that will work with filter()
.
For example:
var jsondata = [{"firstName": "Sam","lastName": "Jones","age": "10"},{"firstName": "Sam1","lastName": "Jones1","age": "10"},{"firstName": "Sam2","lastName": "Jones2","age": "12"},{"firstName": "Sam3","lastName": "Jones3","age": "13"},{"firstName": "Sam4","lastName": "Jones4","age": "14"},{"firstName": "Sam5","lastName": "Jones5","age": "15"},{"firstName": "Sam","lastName": "Jones11","age": "16"},{"firstName": "Sam6","lastName": "Jones6","age": "17"},{"firstName": "Sam7","lastName": "Jones7","age": "18"},{"firstName": "Sam8","lastName": "Jones8","age": "19"},{"firstName": "Sam9","lastName": "Jones9","age": "20"},{"firstName": "Sam10","lastName": "Jones10","age": "21"},{"firstName": "Sam11","lastName": "Jones11","age": "22"},{"firstName": "Sam12","lastName": "Jones12","age": "23"}]
var filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}]
let filtered = jsondata.filter(item => // filter jsondata
filterArray.every( f => // so every member of filter array
f.value.includes(item[f.id])) ) // has a corresponding item[id] in value
console.log(filtered)
The above filter says that for every
item in filterArray
you want the value
array to include the item keyed to id
. This will make it so the item in jsondata
needs to match all the criteria in filterArray
. If you add Sam1
and Jones1
to the arrays, you'll get two items in the filter.
var jsondata = [{"firstName": "Sam","lastName": "Jones","age": "10"},{"firstName": "Sam1","lastName": "Jones1","age": "10"},{"firstName": "Sam2","lastName": "Jones2","age": "12"},{"firstName": "Sam3","lastName": "Jones3","age": "13"},{"firstName": "Sam4","lastName": "Jones4","age": "14"},{"firstName": "Sam5","lastName": "Jones5","age": "15"},{"firstName": "Sam","lastName": "Jones11","age": "16"},{"firstName": "Sam6","lastName": "Jones6","age": "17"},{"firstName": "Sam7","lastName": "Jones7","age": "18"},{"firstName": "Sam8","lastName": "Jones8","age": "19"},{"firstName": "Sam9","lastName": "Jones9","age": "20"},{"firstName": "Sam10","lastName": "Jones10","age": "21"},{"firstName": "Sam11","lastName": "Jones11","age": "22"},{"firstName": "Sam12","lastName": "Jones12","age": "23"}]
var filterArray = [{"id":"firstName","value":["Sam", "Sam1"]},{"id":"lastName","value":["Jones", "Jones1"]}]
let filtered = jsondata.filter(item => // filter jsondata
filterArray.every( f => // so every member of filter array
f.value.includes(item[f.id])) ) // has a corresponding item[id] in value
console.log(filtered)
1
I marked you as correct.
– tommy123
Jan 2 at 3:57
Very consise solution +1
– Henry Howeson
Jan 2 at 4:03
add a comment |
If any of the conditions in the filterArray are false then it will return false, otherwise it will return true. Array.prototype.filter() will create a new array with items for which the function returned true.
const jsondata = jsondata=[{firstName:"Sam",lastName:"Jones",age:"10"},{firstName:"Sam1",lastName:"Jones1",age:"10"},{firstName:"Sam2",lastName:"Jones2",age:"12"},{firstName:"Sam3",lastName:"Jones3",age:"13"},{firstName:"Sam4",lastName:"Jones4",age:"14"},{firstName:"Sam5",lastName:"Jones5",age:"15"},{firstName:"Sam",lastName:"Jones11",age:"16"},{firstName:"Sam6",lastName:"Jones6",age:"17"},{firstName:"Sam7",lastName:"Jones7",age:"18"},{firstName:"Sam8",lastName:"Jones8",age:"19"},{firstName:"Sam9",lastName:"Jones9",age:"20"},{firstName:"Sam10",lastName:"Jones10",age:"21"},{firstName:"Sam11",lastName:"Jones11",age:"22"},{firstName:"Sam12",lastName:"Jones12",age:"23"}];
const filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}];
var match = jsondata.filter(function(element){
for(let i=0; i<filterArray.length; i++){
if(!filterArray[i].value.includes(element[filterArray[i].id])){
return false
}
}
return true;
});
console.log(match);
1
Why is this returningSam1
Jones1
when theSam
Jones
is in the array?
– Mark Meyer
Jan 2 at 3:53
1
@MarkMeyer fixed
– Henry Howeson
Jan 2 at 3:58
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54000937%2fhow-to-filter-an-json-object-by-another-object-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use every
and includes
to make a boolean value that will work with filter()
.
For example:
var jsondata = [{"firstName": "Sam","lastName": "Jones","age": "10"},{"firstName": "Sam1","lastName": "Jones1","age": "10"},{"firstName": "Sam2","lastName": "Jones2","age": "12"},{"firstName": "Sam3","lastName": "Jones3","age": "13"},{"firstName": "Sam4","lastName": "Jones4","age": "14"},{"firstName": "Sam5","lastName": "Jones5","age": "15"},{"firstName": "Sam","lastName": "Jones11","age": "16"},{"firstName": "Sam6","lastName": "Jones6","age": "17"},{"firstName": "Sam7","lastName": "Jones7","age": "18"},{"firstName": "Sam8","lastName": "Jones8","age": "19"},{"firstName": "Sam9","lastName": "Jones9","age": "20"},{"firstName": "Sam10","lastName": "Jones10","age": "21"},{"firstName": "Sam11","lastName": "Jones11","age": "22"},{"firstName": "Sam12","lastName": "Jones12","age": "23"}]
var filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}]
let filtered = jsondata.filter(item => // filter jsondata
filterArray.every( f => // so every member of filter array
f.value.includes(item[f.id])) ) // has a corresponding item[id] in value
console.log(filtered)
The above filter says that for every
item in filterArray
you want the value
array to include the item keyed to id
. This will make it so the item in jsondata
needs to match all the criteria in filterArray
. If you add Sam1
and Jones1
to the arrays, you'll get two items in the filter.
var jsondata = [{"firstName": "Sam","lastName": "Jones","age": "10"},{"firstName": "Sam1","lastName": "Jones1","age": "10"},{"firstName": "Sam2","lastName": "Jones2","age": "12"},{"firstName": "Sam3","lastName": "Jones3","age": "13"},{"firstName": "Sam4","lastName": "Jones4","age": "14"},{"firstName": "Sam5","lastName": "Jones5","age": "15"},{"firstName": "Sam","lastName": "Jones11","age": "16"},{"firstName": "Sam6","lastName": "Jones6","age": "17"},{"firstName": "Sam7","lastName": "Jones7","age": "18"},{"firstName": "Sam8","lastName": "Jones8","age": "19"},{"firstName": "Sam9","lastName": "Jones9","age": "20"},{"firstName": "Sam10","lastName": "Jones10","age": "21"},{"firstName": "Sam11","lastName": "Jones11","age": "22"},{"firstName": "Sam12","lastName": "Jones12","age": "23"}]
var filterArray = [{"id":"firstName","value":["Sam", "Sam1"]},{"id":"lastName","value":["Jones", "Jones1"]}]
let filtered = jsondata.filter(item => // filter jsondata
filterArray.every( f => // so every member of filter array
f.value.includes(item[f.id])) ) // has a corresponding item[id] in value
console.log(filtered)
1
I marked you as correct.
– tommy123
Jan 2 at 3:57
Very consise solution +1
– Henry Howeson
Jan 2 at 4:03
add a comment |
You can use every
and includes
to make a boolean value that will work with filter()
.
For example:
var jsondata = [{"firstName": "Sam","lastName": "Jones","age": "10"},{"firstName": "Sam1","lastName": "Jones1","age": "10"},{"firstName": "Sam2","lastName": "Jones2","age": "12"},{"firstName": "Sam3","lastName": "Jones3","age": "13"},{"firstName": "Sam4","lastName": "Jones4","age": "14"},{"firstName": "Sam5","lastName": "Jones5","age": "15"},{"firstName": "Sam","lastName": "Jones11","age": "16"},{"firstName": "Sam6","lastName": "Jones6","age": "17"},{"firstName": "Sam7","lastName": "Jones7","age": "18"},{"firstName": "Sam8","lastName": "Jones8","age": "19"},{"firstName": "Sam9","lastName": "Jones9","age": "20"},{"firstName": "Sam10","lastName": "Jones10","age": "21"},{"firstName": "Sam11","lastName": "Jones11","age": "22"},{"firstName": "Sam12","lastName": "Jones12","age": "23"}]
var filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}]
let filtered = jsondata.filter(item => // filter jsondata
filterArray.every( f => // so every member of filter array
f.value.includes(item[f.id])) ) // has a corresponding item[id] in value
console.log(filtered)
The above filter says that for every
item in filterArray
you want the value
array to include the item keyed to id
. This will make it so the item in jsondata
needs to match all the criteria in filterArray
. If you add Sam1
and Jones1
to the arrays, you'll get two items in the filter.
var jsondata = [{"firstName": "Sam","lastName": "Jones","age": "10"},{"firstName": "Sam1","lastName": "Jones1","age": "10"},{"firstName": "Sam2","lastName": "Jones2","age": "12"},{"firstName": "Sam3","lastName": "Jones3","age": "13"},{"firstName": "Sam4","lastName": "Jones4","age": "14"},{"firstName": "Sam5","lastName": "Jones5","age": "15"},{"firstName": "Sam","lastName": "Jones11","age": "16"},{"firstName": "Sam6","lastName": "Jones6","age": "17"},{"firstName": "Sam7","lastName": "Jones7","age": "18"},{"firstName": "Sam8","lastName": "Jones8","age": "19"},{"firstName": "Sam9","lastName": "Jones9","age": "20"},{"firstName": "Sam10","lastName": "Jones10","age": "21"},{"firstName": "Sam11","lastName": "Jones11","age": "22"},{"firstName": "Sam12","lastName": "Jones12","age": "23"}]
var filterArray = [{"id":"firstName","value":["Sam", "Sam1"]},{"id":"lastName","value":["Jones", "Jones1"]}]
let filtered = jsondata.filter(item => // filter jsondata
filterArray.every( f => // so every member of filter array
f.value.includes(item[f.id])) ) // has a corresponding item[id] in value
console.log(filtered)
1
I marked you as correct.
– tommy123
Jan 2 at 3:57
Very consise solution +1
– Henry Howeson
Jan 2 at 4:03
add a comment |
You can use every
and includes
to make a boolean value that will work with filter()
.
For example:
var jsondata = [{"firstName": "Sam","lastName": "Jones","age": "10"},{"firstName": "Sam1","lastName": "Jones1","age": "10"},{"firstName": "Sam2","lastName": "Jones2","age": "12"},{"firstName": "Sam3","lastName": "Jones3","age": "13"},{"firstName": "Sam4","lastName": "Jones4","age": "14"},{"firstName": "Sam5","lastName": "Jones5","age": "15"},{"firstName": "Sam","lastName": "Jones11","age": "16"},{"firstName": "Sam6","lastName": "Jones6","age": "17"},{"firstName": "Sam7","lastName": "Jones7","age": "18"},{"firstName": "Sam8","lastName": "Jones8","age": "19"},{"firstName": "Sam9","lastName": "Jones9","age": "20"},{"firstName": "Sam10","lastName": "Jones10","age": "21"},{"firstName": "Sam11","lastName": "Jones11","age": "22"},{"firstName": "Sam12","lastName": "Jones12","age": "23"}]
var filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}]
let filtered = jsondata.filter(item => // filter jsondata
filterArray.every( f => // so every member of filter array
f.value.includes(item[f.id])) ) // has a corresponding item[id] in value
console.log(filtered)
The above filter says that for every
item in filterArray
you want the value
array to include the item keyed to id
. This will make it so the item in jsondata
needs to match all the criteria in filterArray
. If you add Sam1
and Jones1
to the arrays, you'll get two items in the filter.
var jsondata = [{"firstName": "Sam","lastName": "Jones","age": "10"},{"firstName": "Sam1","lastName": "Jones1","age": "10"},{"firstName": "Sam2","lastName": "Jones2","age": "12"},{"firstName": "Sam3","lastName": "Jones3","age": "13"},{"firstName": "Sam4","lastName": "Jones4","age": "14"},{"firstName": "Sam5","lastName": "Jones5","age": "15"},{"firstName": "Sam","lastName": "Jones11","age": "16"},{"firstName": "Sam6","lastName": "Jones6","age": "17"},{"firstName": "Sam7","lastName": "Jones7","age": "18"},{"firstName": "Sam8","lastName": "Jones8","age": "19"},{"firstName": "Sam9","lastName": "Jones9","age": "20"},{"firstName": "Sam10","lastName": "Jones10","age": "21"},{"firstName": "Sam11","lastName": "Jones11","age": "22"},{"firstName": "Sam12","lastName": "Jones12","age": "23"}]
var filterArray = [{"id":"firstName","value":["Sam", "Sam1"]},{"id":"lastName","value":["Jones", "Jones1"]}]
let filtered = jsondata.filter(item => // filter jsondata
filterArray.every( f => // so every member of filter array
f.value.includes(item[f.id])) ) // has a corresponding item[id] in value
console.log(filtered)
You can use every
and includes
to make a boolean value that will work with filter()
.
For example:
var jsondata = [{"firstName": "Sam","lastName": "Jones","age": "10"},{"firstName": "Sam1","lastName": "Jones1","age": "10"},{"firstName": "Sam2","lastName": "Jones2","age": "12"},{"firstName": "Sam3","lastName": "Jones3","age": "13"},{"firstName": "Sam4","lastName": "Jones4","age": "14"},{"firstName": "Sam5","lastName": "Jones5","age": "15"},{"firstName": "Sam","lastName": "Jones11","age": "16"},{"firstName": "Sam6","lastName": "Jones6","age": "17"},{"firstName": "Sam7","lastName": "Jones7","age": "18"},{"firstName": "Sam8","lastName": "Jones8","age": "19"},{"firstName": "Sam9","lastName": "Jones9","age": "20"},{"firstName": "Sam10","lastName": "Jones10","age": "21"},{"firstName": "Sam11","lastName": "Jones11","age": "22"},{"firstName": "Sam12","lastName": "Jones12","age": "23"}]
var filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}]
let filtered = jsondata.filter(item => // filter jsondata
filterArray.every( f => // so every member of filter array
f.value.includes(item[f.id])) ) // has a corresponding item[id] in value
console.log(filtered)
The above filter says that for every
item in filterArray
you want the value
array to include the item keyed to id
. This will make it so the item in jsondata
needs to match all the criteria in filterArray
. If you add Sam1
and Jones1
to the arrays, you'll get two items in the filter.
var jsondata = [{"firstName": "Sam","lastName": "Jones","age": "10"},{"firstName": "Sam1","lastName": "Jones1","age": "10"},{"firstName": "Sam2","lastName": "Jones2","age": "12"},{"firstName": "Sam3","lastName": "Jones3","age": "13"},{"firstName": "Sam4","lastName": "Jones4","age": "14"},{"firstName": "Sam5","lastName": "Jones5","age": "15"},{"firstName": "Sam","lastName": "Jones11","age": "16"},{"firstName": "Sam6","lastName": "Jones6","age": "17"},{"firstName": "Sam7","lastName": "Jones7","age": "18"},{"firstName": "Sam8","lastName": "Jones8","age": "19"},{"firstName": "Sam9","lastName": "Jones9","age": "20"},{"firstName": "Sam10","lastName": "Jones10","age": "21"},{"firstName": "Sam11","lastName": "Jones11","age": "22"},{"firstName": "Sam12","lastName": "Jones12","age": "23"}]
var filterArray = [{"id":"firstName","value":["Sam", "Sam1"]},{"id":"lastName","value":["Jones", "Jones1"]}]
let filtered = jsondata.filter(item => // filter jsondata
filterArray.every( f => // so every member of filter array
f.value.includes(item[f.id])) ) // has a corresponding item[id] in value
console.log(filtered)
var jsondata = [{"firstName": "Sam","lastName": "Jones","age": "10"},{"firstName": "Sam1","lastName": "Jones1","age": "10"},{"firstName": "Sam2","lastName": "Jones2","age": "12"},{"firstName": "Sam3","lastName": "Jones3","age": "13"},{"firstName": "Sam4","lastName": "Jones4","age": "14"},{"firstName": "Sam5","lastName": "Jones5","age": "15"},{"firstName": "Sam","lastName": "Jones11","age": "16"},{"firstName": "Sam6","lastName": "Jones6","age": "17"},{"firstName": "Sam7","lastName": "Jones7","age": "18"},{"firstName": "Sam8","lastName": "Jones8","age": "19"},{"firstName": "Sam9","lastName": "Jones9","age": "20"},{"firstName": "Sam10","lastName": "Jones10","age": "21"},{"firstName": "Sam11","lastName": "Jones11","age": "22"},{"firstName": "Sam12","lastName": "Jones12","age": "23"}]
var filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}]
let filtered = jsondata.filter(item => // filter jsondata
filterArray.every( f => // so every member of filter array
f.value.includes(item[f.id])) ) // has a corresponding item[id] in value
console.log(filtered)
var jsondata = [{"firstName": "Sam","lastName": "Jones","age": "10"},{"firstName": "Sam1","lastName": "Jones1","age": "10"},{"firstName": "Sam2","lastName": "Jones2","age": "12"},{"firstName": "Sam3","lastName": "Jones3","age": "13"},{"firstName": "Sam4","lastName": "Jones4","age": "14"},{"firstName": "Sam5","lastName": "Jones5","age": "15"},{"firstName": "Sam","lastName": "Jones11","age": "16"},{"firstName": "Sam6","lastName": "Jones6","age": "17"},{"firstName": "Sam7","lastName": "Jones7","age": "18"},{"firstName": "Sam8","lastName": "Jones8","age": "19"},{"firstName": "Sam9","lastName": "Jones9","age": "20"},{"firstName": "Sam10","lastName": "Jones10","age": "21"},{"firstName": "Sam11","lastName": "Jones11","age": "22"},{"firstName": "Sam12","lastName": "Jones12","age": "23"}]
var filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}]
let filtered = jsondata.filter(item => // filter jsondata
filterArray.every( f => // so every member of filter array
f.value.includes(item[f.id])) ) // has a corresponding item[id] in value
console.log(filtered)
var jsondata = [{"firstName": "Sam","lastName": "Jones","age": "10"},{"firstName": "Sam1","lastName": "Jones1","age": "10"},{"firstName": "Sam2","lastName": "Jones2","age": "12"},{"firstName": "Sam3","lastName": "Jones3","age": "13"},{"firstName": "Sam4","lastName": "Jones4","age": "14"},{"firstName": "Sam5","lastName": "Jones5","age": "15"},{"firstName": "Sam","lastName": "Jones11","age": "16"},{"firstName": "Sam6","lastName": "Jones6","age": "17"},{"firstName": "Sam7","lastName": "Jones7","age": "18"},{"firstName": "Sam8","lastName": "Jones8","age": "19"},{"firstName": "Sam9","lastName": "Jones9","age": "20"},{"firstName": "Sam10","lastName": "Jones10","age": "21"},{"firstName": "Sam11","lastName": "Jones11","age": "22"},{"firstName": "Sam12","lastName": "Jones12","age": "23"}]
var filterArray = [{"id":"firstName","value":["Sam", "Sam1"]},{"id":"lastName","value":["Jones", "Jones1"]}]
let filtered = jsondata.filter(item => // filter jsondata
filterArray.every( f => // so every member of filter array
f.value.includes(item[f.id])) ) // has a corresponding item[id] in value
console.log(filtered)
var jsondata = [{"firstName": "Sam","lastName": "Jones","age": "10"},{"firstName": "Sam1","lastName": "Jones1","age": "10"},{"firstName": "Sam2","lastName": "Jones2","age": "12"},{"firstName": "Sam3","lastName": "Jones3","age": "13"},{"firstName": "Sam4","lastName": "Jones4","age": "14"},{"firstName": "Sam5","lastName": "Jones5","age": "15"},{"firstName": "Sam","lastName": "Jones11","age": "16"},{"firstName": "Sam6","lastName": "Jones6","age": "17"},{"firstName": "Sam7","lastName": "Jones7","age": "18"},{"firstName": "Sam8","lastName": "Jones8","age": "19"},{"firstName": "Sam9","lastName": "Jones9","age": "20"},{"firstName": "Sam10","lastName": "Jones10","age": "21"},{"firstName": "Sam11","lastName": "Jones11","age": "22"},{"firstName": "Sam12","lastName": "Jones12","age": "23"}]
var filterArray = [{"id":"firstName","value":["Sam", "Sam1"]},{"id":"lastName","value":["Jones", "Jones1"]}]
let filtered = jsondata.filter(item => // filter jsondata
filterArray.every( f => // so every member of filter array
f.value.includes(item[f.id])) ) // has a corresponding item[id] in value
console.log(filtered)
edited Jan 2 at 3:46
answered Jan 2 at 3:41


Mark MeyerMark Meyer
38.9k33160
38.9k33160
1
I marked you as correct.
– tommy123
Jan 2 at 3:57
Very consise solution +1
– Henry Howeson
Jan 2 at 4:03
add a comment |
1
I marked you as correct.
– tommy123
Jan 2 at 3:57
Very consise solution +1
– Henry Howeson
Jan 2 at 4:03
1
1
I marked you as correct.
– tommy123
Jan 2 at 3:57
I marked you as correct.
– tommy123
Jan 2 at 3:57
Very consise solution +1
– Henry Howeson
Jan 2 at 4:03
Very consise solution +1
– Henry Howeson
Jan 2 at 4:03
add a comment |
If any of the conditions in the filterArray are false then it will return false, otherwise it will return true. Array.prototype.filter() will create a new array with items for which the function returned true.
const jsondata = jsondata=[{firstName:"Sam",lastName:"Jones",age:"10"},{firstName:"Sam1",lastName:"Jones1",age:"10"},{firstName:"Sam2",lastName:"Jones2",age:"12"},{firstName:"Sam3",lastName:"Jones3",age:"13"},{firstName:"Sam4",lastName:"Jones4",age:"14"},{firstName:"Sam5",lastName:"Jones5",age:"15"},{firstName:"Sam",lastName:"Jones11",age:"16"},{firstName:"Sam6",lastName:"Jones6",age:"17"},{firstName:"Sam7",lastName:"Jones7",age:"18"},{firstName:"Sam8",lastName:"Jones8",age:"19"},{firstName:"Sam9",lastName:"Jones9",age:"20"},{firstName:"Sam10",lastName:"Jones10",age:"21"},{firstName:"Sam11",lastName:"Jones11",age:"22"},{firstName:"Sam12",lastName:"Jones12",age:"23"}];
const filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}];
var match = jsondata.filter(function(element){
for(let i=0; i<filterArray.length; i++){
if(!filterArray[i].value.includes(element[filterArray[i].id])){
return false
}
}
return true;
});
console.log(match);
1
Why is this returningSam1
Jones1
when theSam
Jones
is in the array?
– Mark Meyer
Jan 2 at 3:53
1
@MarkMeyer fixed
– Henry Howeson
Jan 2 at 3:58
add a comment |
If any of the conditions in the filterArray are false then it will return false, otherwise it will return true. Array.prototype.filter() will create a new array with items for which the function returned true.
const jsondata = jsondata=[{firstName:"Sam",lastName:"Jones",age:"10"},{firstName:"Sam1",lastName:"Jones1",age:"10"},{firstName:"Sam2",lastName:"Jones2",age:"12"},{firstName:"Sam3",lastName:"Jones3",age:"13"},{firstName:"Sam4",lastName:"Jones4",age:"14"},{firstName:"Sam5",lastName:"Jones5",age:"15"},{firstName:"Sam",lastName:"Jones11",age:"16"},{firstName:"Sam6",lastName:"Jones6",age:"17"},{firstName:"Sam7",lastName:"Jones7",age:"18"},{firstName:"Sam8",lastName:"Jones8",age:"19"},{firstName:"Sam9",lastName:"Jones9",age:"20"},{firstName:"Sam10",lastName:"Jones10",age:"21"},{firstName:"Sam11",lastName:"Jones11",age:"22"},{firstName:"Sam12",lastName:"Jones12",age:"23"}];
const filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}];
var match = jsondata.filter(function(element){
for(let i=0; i<filterArray.length; i++){
if(!filterArray[i].value.includes(element[filterArray[i].id])){
return false
}
}
return true;
});
console.log(match);
1
Why is this returningSam1
Jones1
when theSam
Jones
is in the array?
– Mark Meyer
Jan 2 at 3:53
1
@MarkMeyer fixed
– Henry Howeson
Jan 2 at 3:58
add a comment |
If any of the conditions in the filterArray are false then it will return false, otherwise it will return true. Array.prototype.filter() will create a new array with items for which the function returned true.
const jsondata = jsondata=[{firstName:"Sam",lastName:"Jones",age:"10"},{firstName:"Sam1",lastName:"Jones1",age:"10"},{firstName:"Sam2",lastName:"Jones2",age:"12"},{firstName:"Sam3",lastName:"Jones3",age:"13"},{firstName:"Sam4",lastName:"Jones4",age:"14"},{firstName:"Sam5",lastName:"Jones5",age:"15"},{firstName:"Sam",lastName:"Jones11",age:"16"},{firstName:"Sam6",lastName:"Jones6",age:"17"},{firstName:"Sam7",lastName:"Jones7",age:"18"},{firstName:"Sam8",lastName:"Jones8",age:"19"},{firstName:"Sam9",lastName:"Jones9",age:"20"},{firstName:"Sam10",lastName:"Jones10",age:"21"},{firstName:"Sam11",lastName:"Jones11",age:"22"},{firstName:"Sam12",lastName:"Jones12",age:"23"}];
const filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}];
var match = jsondata.filter(function(element){
for(let i=0; i<filterArray.length; i++){
if(!filterArray[i].value.includes(element[filterArray[i].id])){
return false
}
}
return true;
});
console.log(match);
If any of the conditions in the filterArray are false then it will return false, otherwise it will return true. Array.prototype.filter() will create a new array with items for which the function returned true.
const jsondata = jsondata=[{firstName:"Sam",lastName:"Jones",age:"10"},{firstName:"Sam1",lastName:"Jones1",age:"10"},{firstName:"Sam2",lastName:"Jones2",age:"12"},{firstName:"Sam3",lastName:"Jones3",age:"13"},{firstName:"Sam4",lastName:"Jones4",age:"14"},{firstName:"Sam5",lastName:"Jones5",age:"15"},{firstName:"Sam",lastName:"Jones11",age:"16"},{firstName:"Sam6",lastName:"Jones6",age:"17"},{firstName:"Sam7",lastName:"Jones7",age:"18"},{firstName:"Sam8",lastName:"Jones8",age:"19"},{firstName:"Sam9",lastName:"Jones9",age:"20"},{firstName:"Sam10",lastName:"Jones10",age:"21"},{firstName:"Sam11",lastName:"Jones11",age:"22"},{firstName:"Sam12",lastName:"Jones12",age:"23"}];
const filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}];
var match = jsondata.filter(function(element){
for(let i=0; i<filterArray.length; i++){
if(!filterArray[i].value.includes(element[filterArray[i].id])){
return false
}
}
return true;
});
console.log(match);
const jsondata = jsondata=[{firstName:"Sam",lastName:"Jones",age:"10"},{firstName:"Sam1",lastName:"Jones1",age:"10"},{firstName:"Sam2",lastName:"Jones2",age:"12"},{firstName:"Sam3",lastName:"Jones3",age:"13"},{firstName:"Sam4",lastName:"Jones4",age:"14"},{firstName:"Sam5",lastName:"Jones5",age:"15"},{firstName:"Sam",lastName:"Jones11",age:"16"},{firstName:"Sam6",lastName:"Jones6",age:"17"},{firstName:"Sam7",lastName:"Jones7",age:"18"},{firstName:"Sam8",lastName:"Jones8",age:"19"},{firstName:"Sam9",lastName:"Jones9",age:"20"},{firstName:"Sam10",lastName:"Jones10",age:"21"},{firstName:"Sam11",lastName:"Jones11",age:"22"},{firstName:"Sam12",lastName:"Jones12",age:"23"}];
const filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}];
var match = jsondata.filter(function(element){
for(let i=0; i<filterArray.length; i++){
if(!filterArray[i].value.includes(element[filterArray[i].id])){
return false
}
}
return true;
});
console.log(match);
const jsondata = jsondata=[{firstName:"Sam",lastName:"Jones",age:"10"},{firstName:"Sam1",lastName:"Jones1",age:"10"},{firstName:"Sam2",lastName:"Jones2",age:"12"},{firstName:"Sam3",lastName:"Jones3",age:"13"},{firstName:"Sam4",lastName:"Jones4",age:"14"},{firstName:"Sam5",lastName:"Jones5",age:"15"},{firstName:"Sam",lastName:"Jones11",age:"16"},{firstName:"Sam6",lastName:"Jones6",age:"17"},{firstName:"Sam7",lastName:"Jones7",age:"18"},{firstName:"Sam8",lastName:"Jones8",age:"19"},{firstName:"Sam9",lastName:"Jones9",age:"20"},{firstName:"Sam10",lastName:"Jones10",age:"21"},{firstName:"Sam11",lastName:"Jones11",age:"22"},{firstName:"Sam12",lastName:"Jones12",age:"23"}];
const filterArray = [{"id":"firstName","value":["Sam"]},{"id":"lastName","value":["Jones"]}];
var match = jsondata.filter(function(element){
for(let i=0; i<filterArray.length; i++){
if(!filterArray[i].value.includes(element[filterArray[i].id])){
return false
}
}
return true;
});
console.log(match);
edited Jan 2 at 3:57
answered Jan 2 at 3:44
Henry HowesonHenry Howeson
12812
12812
1
Why is this returningSam1
Jones1
when theSam
Jones
is in the array?
– Mark Meyer
Jan 2 at 3:53
1
@MarkMeyer fixed
– Henry Howeson
Jan 2 at 3:58
add a comment |
1
Why is this returningSam1
Jones1
when theSam
Jones
is in the array?
– Mark Meyer
Jan 2 at 3:53
1
@MarkMeyer fixed
– Henry Howeson
Jan 2 at 3:58
1
1
Why is this returning
Sam1
Jones1
when the Sam
Jones
is in the array?– Mark Meyer
Jan 2 at 3:53
Why is this returning
Sam1
Jones1
when the Sam
Jones
is in the array?– Mark Meyer
Jan 2 at 3:53
1
1
@MarkMeyer fixed
– Henry Howeson
Jan 2 at 3:58
@MarkMeyer fixed
– Henry Howeson
Jan 2 at 3:58
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54000937%2fhow-to-filter-an-json-object-by-another-object-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pJEWva,P
2
The posted question does not appear to include any attempt at all to solve the problem. StackOverflow expects you to try to solve your own problem first, as your attempts help us to better understand what you want. Please edit the question to show what you've tried, so as to illustrate a specific roadblock you're running into a Minimal, Complete, and Verifiable example. For more information, please see How to Ask and take the tour.
– CertainPerformance
Jan 2 at 3:36
I cannot understand how to implement this. That is why I asked the question.
– tommy123
Jan 2 at 3:38