How to get previous selected value from another selection into current selection onclick jquery script...
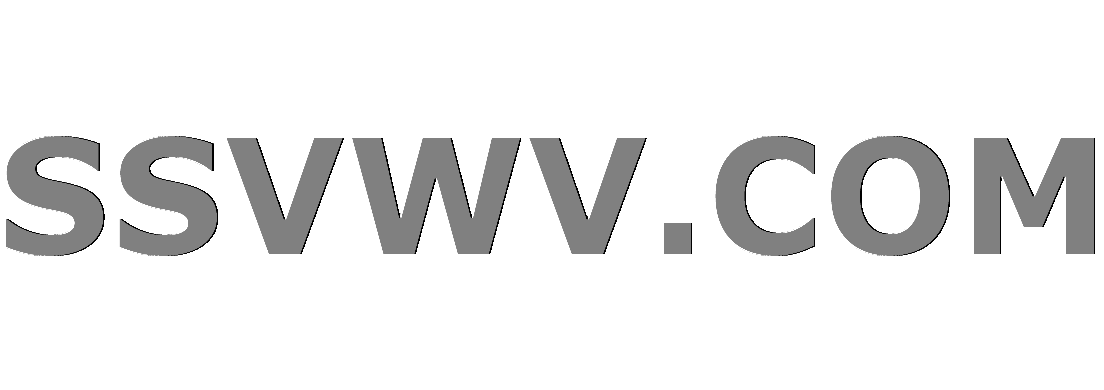
Multi tool use
I have 3 selection. The jQuery onchange occur when user select the third selection and post the variable to another php file so I can check the database based on the id selected. The problem is, how I can get the first and second selection variable to post it together with the third selection?
These are tables I used:
fromlist table :
| id | fromname |
| 1 | A |
| 2 | B |
| 3 | C |
tolist table:
| id | toname |
| 1 | Z |
productlist table:
| id | name |
| 1 | CPO |
| 2 | PK |
transport table:
| id | product_id | product | to_id | from_id | price |
| 1 | 100 | 1 | 1 | 1 | 100.00 |
| 2 | 101 | 2 | 1 | 1 | 200.00 |
| 3 | 102 | 1 | 1 | 2 | 300.00 |
| 4 | 103 | 2 | 1 | 2 | 400.00 |
| 5 | 104 | 2 | 1 | 3 | 500.00 |
Here is the code :
<form name = "transport" method = "GET" action = "http://interlink2u.com/transport/transportfiles/verify.php">
<select name = "phive_product_id" id = "phive_product_id" hidden></select>
<label>From:</label>
<select name = "select_from" id = "select_from" required>
<option id = "" value = "">None</option>
<?php
$fromlist = mysqli_query($connection, "SELECT * FROM fromlist ORDER BY fromname ASC");
while ($row = mysqli_fetch_assoc($fromlist))
{
$getID = $row['id'];
$getName = $row['fromname'];
?><option id = "<?php echo $getID; ?>" value = "<?php echo $getName; ?>"><?php echo $getName;?></option><?php
}
?>
</select><br><br>
<label>To:</label>
<select name = "select_to" id = "select_to" required>
<option id = "" value = "">None</option>
<?php
$tolist = mysqli_query($connection, "SELECT * FROM tolist ORDER BY toname ASC");
while ($row = mysqli_fetch_assoc($tolist))
{
$getID = $row['id'];
$getName = $row['toname'];
?><option id = "<?php echo $getID; ?>" value = "<?php echo $getName; ?>"><?php echo $getName;?></option><?php
}
?>
</select>
<br><br>
<label>Product:</label>
<select name = "select_product" id = "select_product" required>
<option id = "" value = "">None</option>
<?php
$products = mysqli_query($connection, "SELECT * FROM products ORDER BY name ASC");
while ($row = mysqli_fetch_assoc($products))
{
$getID = $row['id'];
$getName = $row['name'];
?><option id = "<?php echo $getID; ?>" value = "<?php echo $getName; ?>"><?php echo $getName;?></option><?php
}
?>
</select>
<select name = "phive_booked_price" id = "phive_booked_price" hidden></select>
<br><br>
<input type = "submit" value = "Submit" id="disablebutton">
</form><br><br><br>
Here is the script :
jQuery(document).on("click", 'select#select_product', function(e)
{
var productID = jQuery(this).children(":selected").attr("id");
var fromID = jQuery('#select_from').children(":selected").attr("id");
var toID = jQuery('#select_to').children(":selected").attr("id");
$.ajax
({
type: "POST",
data: {productID: productID, fromID: fromID, toID: toID},
url: 'http://interlink2u.com/transport/transportfiles/getprice.php',
dataType: 'json',
success: function(json)
{
var $el4 = jQuery("select#phive_product_id");
$el4.empty();
$.each(json, function(k, v)
{
$el4.append("<option id = '" + v.product_id + "'value = '" + v.product_id + "'>" + v.product_id + "</option>");
});
var $el5 = jQuery("select#phive_booked_price");
$el5.empty();
$.each(json, function(k, v)
{
$el5.append("<option id = '" + v.product_id + "'value = '" + v.price + "'>" + v.price + "</option>");
});
}
});
})
getprice.php code :
<?php
include_once 'config/config.php';
$productID= $_POST['productID'];
$result = mysqli_query($connection, "SELECT * FROM transport
INNER JOIN tolist ON transport.to_name = tolist.id
INNER JOIN products ON transport.product = products.id
WHERE product = '" .$productID. "'");
$results = ;
while ($row = mysqli_fetch_assoc($result))
{
$results = $row;
}
header('Content-Type: application/json');
echo json_encode($results);
?>
I want to get the first and second selected values and post it to getprice.php so I can query the result like this :
<?php
include_once 'config/config.php';
$fromID = $_POST['fromID'];
$toID = $_POST['toID'];
$productID = $_POST['productID'];
$result = mysqli_query($connection, "SELECT * FROM transport
INNER JOIN tolist ON transport.to_name = tolist.id
INNER JOIN products ON transport.product = products.id
WHERE from_id = '" .$fromID. "'
AND to_name = '".$toID."'
AND product = '".$productID."'");
$results = ;
while ($row = mysqli_fetch_assoc($result))
{
$results = $row;
}
header('Content-Type: application/json');
echo json_encode($results);
?>
php jquery mysql wordpress
add a comment |
I have 3 selection. The jQuery onchange occur when user select the third selection and post the variable to another php file so I can check the database based on the id selected. The problem is, how I can get the first and second selection variable to post it together with the third selection?
These are tables I used:
fromlist table :
| id | fromname |
| 1 | A |
| 2 | B |
| 3 | C |
tolist table:
| id | toname |
| 1 | Z |
productlist table:
| id | name |
| 1 | CPO |
| 2 | PK |
transport table:
| id | product_id | product | to_id | from_id | price |
| 1 | 100 | 1 | 1 | 1 | 100.00 |
| 2 | 101 | 2 | 1 | 1 | 200.00 |
| 3 | 102 | 1 | 1 | 2 | 300.00 |
| 4 | 103 | 2 | 1 | 2 | 400.00 |
| 5 | 104 | 2 | 1 | 3 | 500.00 |
Here is the code :
<form name = "transport" method = "GET" action = "http://interlink2u.com/transport/transportfiles/verify.php">
<select name = "phive_product_id" id = "phive_product_id" hidden></select>
<label>From:</label>
<select name = "select_from" id = "select_from" required>
<option id = "" value = "">None</option>
<?php
$fromlist = mysqli_query($connection, "SELECT * FROM fromlist ORDER BY fromname ASC");
while ($row = mysqli_fetch_assoc($fromlist))
{
$getID = $row['id'];
$getName = $row['fromname'];
?><option id = "<?php echo $getID; ?>" value = "<?php echo $getName; ?>"><?php echo $getName;?></option><?php
}
?>
</select><br><br>
<label>To:</label>
<select name = "select_to" id = "select_to" required>
<option id = "" value = "">None</option>
<?php
$tolist = mysqli_query($connection, "SELECT * FROM tolist ORDER BY toname ASC");
while ($row = mysqli_fetch_assoc($tolist))
{
$getID = $row['id'];
$getName = $row['toname'];
?><option id = "<?php echo $getID; ?>" value = "<?php echo $getName; ?>"><?php echo $getName;?></option><?php
}
?>
</select>
<br><br>
<label>Product:</label>
<select name = "select_product" id = "select_product" required>
<option id = "" value = "">None</option>
<?php
$products = mysqli_query($connection, "SELECT * FROM products ORDER BY name ASC");
while ($row = mysqli_fetch_assoc($products))
{
$getID = $row['id'];
$getName = $row['name'];
?><option id = "<?php echo $getID; ?>" value = "<?php echo $getName; ?>"><?php echo $getName;?></option><?php
}
?>
</select>
<select name = "phive_booked_price" id = "phive_booked_price" hidden></select>
<br><br>
<input type = "submit" value = "Submit" id="disablebutton">
</form><br><br><br>
Here is the script :
jQuery(document).on("click", 'select#select_product', function(e)
{
var productID = jQuery(this).children(":selected").attr("id");
var fromID = jQuery('#select_from').children(":selected").attr("id");
var toID = jQuery('#select_to').children(":selected").attr("id");
$.ajax
({
type: "POST",
data: {productID: productID, fromID: fromID, toID: toID},
url: 'http://interlink2u.com/transport/transportfiles/getprice.php',
dataType: 'json',
success: function(json)
{
var $el4 = jQuery("select#phive_product_id");
$el4.empty();
$.each(json, function(k, v)
{
$el4.append("<option id = '" + v.product_id + "'value = '" + v.product_id + "'>" + v.product_id + "</option>");
});
var $el5 = jQuery("select#phive_booked_price");
$el5.empty();
$.each(json, function(k, v)
{
$el5.append("<option id = '" + v.product_id + "'value = '" + v.price + "'>" + v.price + "</option>");
});
}
});
})
getprice.php code :
<?php
include_once 'config/config.php';
$productID= $_POST['productID'];
$result = mysqli_query($connection, "SELECT * FROM transport
INNER JOIN tolist ON transport.to_name = tolist.id
INNER JOIN products ON transport.product = products.id
WHERE product = '" .$productID. "'");
$results = ;
while ($row = mysqli_fetch_assoc($result))
{
$results = $row;
}
header('Content-Type: application/json');
echo json_encode($results);
?>
I want to get the first and second selected values and post it to getprice.php so I can query the result like this :
<?php
include_once 'config/config.php';
$fromID = $_POST['fromID'];
$toID = $_POST['toID'];
$productID = $_POST['productID'];
$result = mysqli_query($connection, "SELECT * FROM transport
INNER JOIN tolist ON transport.to_name = tolist.id
INNER JOIN products ON transport.product = products.id
WHERE from_id = '" .$fromID. "'
AND to_name = '".$toID."'
AND product = '".$productID."'");
$results = ;
while ($row = mysqli_fetch_assoc($result))
{
$results = $row;
}
header('Content-Type: application/json');
echo json_encode($results);
?>
php jquery mysql wordpress
add a comment |
I have 3 selection. The jQuery onchange occur when user select the third selection and post the variable to another php file so I can check the database based on the id selected. The problem is, how I can get the first and second selection variable to post it together with the third selection?
These are tables I used:
fromlist table :
| id | fromname |
| 1 | A |
| 2 | B |
| 3 | C |
tolist table:
| id | toname |
| 1 | Z |
productlist table:
| id | name |
| 1 | CPO |
| 2 | PK |
transport table:
| id | product_id | product | to_id | from_id | price |
| 1 | 100 | 1 | 1 | 1 | 100.00 |
| 2 | 101 | 2 | 1 | 1 | 200.00 |
| 3 | 102 | 1 | 1 | 2 | 300.00 |
| 4 | 103 | 2 | 1 | 2 | 400.00 |
| 5 | 104 | 2 | 1 | 3 | 500.00 |
Here is the code :
<form name = "transport" method = "GET" action = "http://interlink2u.com/transport/transportfiles/verify.php">
<select name = "phive_product_id" id = "phive_product_id" hidden></select>
<label>From:</label>
<select name = "select_from" id = "select_from" required>
<option id = "" value = "">None</option>
<?php
$fromlist = mysqli_query($connection, "SELECT * FROM fromlist ORDER BY fromname ASC");
while ($row = mysqli_fetch_assoc($fromlist))
{
$getID = $row['id'];
$getName = $row['fromname'];
?><option id = "<?php echo $getID; ?>" value = "<?php echo $getName; ?>"><?php echo $getName;?></option><?php
}
?>
</select><br><br>
<label>To:</label>
<select name = "select_to" id = "select_to" required>
<option id = "" value = "">None</option>
<?php
$tolist = mysqli_query($connection, "SELECT * FROM tolist ORDER BY toname ASC");
while ($row = mysqli_fetch_assoc($tolist))
{
$getID = $row['id'];
$getName = $row['toname'];
?><option id = "<?php echo $getID; ?>" value = "<?php echo $getName; ?>"><?php echo $getName;?></option><?php
}
?>
</select>
<br><br>
<label>Product:</label>
<select name = "select_product" id = "select_product" required>
<option id = "" value = "">None</option>
<?php
$products = mysqli_query($connection, "SELECT * FROM products ORDER BY name ASC");
while ($row = mysqli_fetch_assoc($products))
{
$getID = $row['id'];
$getName = $row['name'];
?><option id = "<?php echo $getID; ?>" value = "<?php echo $getName; ?>"><?php echo $getName;?></option><?php
}
?>
</select>
<select name = "phive_booked_price" id = "phive_booked_price" hidden></select>
<br><br>
<input type = "submit" value = "Submit" id="disablebutton">
</form><br><br><br>
Here is the script :
jQuery(document).on("click", 'select#select_product', function(e)
{
var productID = jQuery(this).children(":selected").attr("id");
var fromID = jQuery('#select_from').children(":selected").attr("id");
var toID = jQuery('#select_to').children(":selected").attr("id");
$.ajax
({
type: "POST",
data: {productID: productID, fromID: fromID, toID: toID},
url: 'http://interlink2u.com/transport/transportfiles/getprice.php',
dataType: 'json',
success: function(json)
{
var $el4 = jQuery("select#phive_product_id");
$el4.empty();
$.each(json, function(k, v)
{
$el4.append("<option id = '" + v.product_id + "'value = '" + v.product_id + "'>" + v.product_id + "</option>");
});
var $el5 = jQuery("select#phive_booked_price");
$el5.empty();
$.each(json, function(k, v)
{
$el5.append("<option id = '" + v.product_id + "'value = '" + v.price + "'>" + v.price + "</option>");
});
}
});
})
getprice.php code :
<?php
include_once 'config/config.php';
$productID= $_POST['productID'];
$result = mysqli_query($connection, "SELECT * FROM transport
INNER JOIN tolist ON transport.to_name = tolist.id
INNER JOIN products ON transport.product = products.id
WHERE product = '" .$productID. "'");
$results = ;
while ($row = mysqli_fetch_assoc($result))
{
$results = $row;
}
header('Content-Type: application/json');
echo json_encode($results);
?>
I want to get the first and second selected values and post it to getprice.php so I can query the result like this :
<?php
include_once 'config/config.php';
$fromID = $_POST['fromID'];
$toID = $_POST['toID'];
$productID = $_POST['productID'];
$result = mysqli_query($connection, "SELECT * FROM transport
INNER JOIN tolist ON transport.to_name = tolist.id
INNER JOIN products ON transport.product = products.id
WHERE from_id = '" .$fromID. "'
AND to_name = '".$toID."'
AND product = '".$productID."'");
$results = ;
while ($row = mysqli_fetch_assoc($result))
{
$results = $row;
}
header('Content-Type: application/json');
echo json_encode($results);
?>
php jquery mysql wordpress
I have 3 selection. The jQuery onchange occur when user select the third selection and post the variable to another php file so I can check the database based on the id selected. The problem is, how I can get the first and second selection variable to post it together with the third selection?
These are tables I used:
fromlist table :
| id | fromname |
| 1 | A |
| 2 | B |
| 3 | C |
tolist table:
| id | toname |
| 1 | Z |
productlist table:
| id | name |
| 1 | CPO |
| 2 | PK |
transport table:
| id | product_id | product | to_id | from_id | price |
| 1 | 100 | 1 | 1 | 1 | 100.00 |
| 2 | 101 | 2 | 1 | 1 | 200.00 |
| 3 | 102 | 1 | 1 | 2 | 300.00 |
| 4 | 103 | 2 | 1 | 2 | 400.00 |
| 5 | 104 | 2 | 1 | 3 | 500.00 |
Here is the code :
<form name = "transport" method = "GET" action = "http://interlink2u.com/transport/transportfiles/verify.php">
<select name = "phive_product_id" id = "phive_product_id" hidden></select>
<label>From:</label>
<select name = "select_from" id = "select_from" required>
<option id = "" value = "">None</option>
<?php
$fromlist = mysqli_query($connection, "SELECT * FROM fromlist ORDER BY fromname ASC");
while ($row = mysqli_fetch_assoc($fromlist))
{
$getID = $row['id'];
$getName = $row['fromname'];
?><option id = "<?php echo $getID; ?>" value = "<?php echo $getName; ?>"><?php echo $getName;?></option><?php
}
?>
</select><br><br>
<label>To:</label>
<select name = "select_to" id = "select_to" required>
<option id = "" value = "">None</option>
<?php
$tolist = mysqli_query($connection, "SELECT * FROM tolist ORDER BY toname ASC");
while ($row = mysqli_fetch_assoc($tolist))
{
$getID = $row['id'];
$getName = $row['toname'];
?><option id = "<?php echo $getID; ?>" value = "<?php echo $getName; ?>"><?php echo $getName;?></option><?php
}
?>
</select>
<br><br>
<label>Product:</label>
<select name = "select_product" id = "select_product" required>
<option id = "" value = "">None</option>
<?php
$products = mysqli_query($connection, "SELECT * FROM products ORDER BY name ASC");
while ($row = mysqli_fetch_assoc($products))
{
$getID = $row['id'];
$getName = $row['name'];
?><option id = "<?php echo $getID; ?>" value = "<?php echo $getName; ?>"><?php echo $getName;?></option><?php
}
?>
</select>
<select name = "phive_booked_price" id = "phive_booked_price" hidden></select>
<br><br>
<input type = "submit" value = "Submit" id="disablebutton">
</form><br><br><br>
Here is the script :
jQuery(document).on("click", 'select#select_product', function(e)
{
var productID = jQuery(this).children(":selected").attr("id");
var fromID = jQuery('#select_from').children(":selected").attr("id");
var toID = jQuery('#select_to').children(":selected").attr("id");
$.ajax
({
type: "POST",
data: {productID: productID, fromID: fromID, toID: toID},
url: 'http://interlink2u.com/transport/transportfiles/getprice.php',
dataType: 'json',
success: function(json)
{
var $el4 = jQuery("select#phive_product_id");
$el4.empty();
$.each(json, function(k, v)
{
$el4.append("<option id = '" + v.product_id + "'value = '" + v.product_id + "'>" + v.product_id + "</option>");
});
var $el5 = jQuery("select#phive_booked_price");
$el5.empty();
$.each(json, function(k, v)
{
$el5.append("<option id = '" + v.product_id + "'value = '" + v.price + "'>" + v.price + "</option>");
});
}
});
})
getprice.php code :
<?php
include_once 'config/config.php';
$productID= $_POST['productID'];
$result = mysqli_query($connection, "SELECT * FROM transport
INNER JOIN tolist ON transport.to_name = tolist.id
INNER JOIN products ON transport.product = products.id
WHERE product = '" .$productID. "'");
$results = ;
while ($row = mysqli_fetch_assoc($result))
{
$results = $row;
}
header('Content-Type: application/json');
echo json_encode($results);
?>
I want to get the first and second selected values and post it to getprice.php so I can query the result like this :
<?php
include_once 'config/config.php';
$fromID = $_POST['fromID'];
$toID = $_POST['toID'];
$productID = $_POST['productID'];
$result = mysqli_query($connection, "SELECT * FROM transport
INNER JOIN tolist ON transport.to_name = tolist.id
INNER JOIN products ON transport.product = products.id
WHERE from_id = '" .$fromID. "'
AND to_name = '".$toID."'
AND product = '".$productID."'");
$results = ;
while ($row = mysqli_fetch_assoc($result))
{
$results = $row;
}
header('Content-Type: application/json');
echo json_encode($results);
?>
php jquery mysql wordpress
php jquery mysql wordpress
edited Jan 2 at 9:06
Richmond
asked Jan 2 at 3:18
RichmondRichmond
419
419
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
You are only sending productID in your ajax:
data: {productID: productID},
If you change your line to:
data: $('form[name="transport"]').serialize(),
Read more on serialize. Your jquery call will now look like:
jQuery(document).on("click", 'select#select_product', function(e)
{
$.ajax
({
type: "POST",
data: $('form[name="transport"]').serialize(),
url: 'http://interlink2u.com/transport/transportfiles/getprice.php',
dataType: 'json',
success: function(json)
{
var $el4 = jQuery("select#phive_product_id");
$el4.empty();
$.each(json, function(k, v)
{
$el4.append("<option id = '" + v.product_id + "'value = '" + v.product_id + "'>" + v.product_id + "</option>");
});
var $el5 = jQuery("select#phive_booked_price");
$el5.empty();
$.each(json, function(k, v)
{
$el5.append("<option id = '" + v.product_id + "'value = '" + v.price + "'>" + v.price + "</option>");
});
}
});
})
Then in your getprice.php code you would need to update:
$productID = $_POST['select_product']; // now select_product as per your selectbox name
But you also now have available:
$_POST['select_from']
$_POST['select_to']
$_POST['phive_booked_price']
$_POST['phive_product_id']
EDIT:
To include them the way you asked in the comments you would need to update:
var productID = $('#select_product').val();
var fromID = $('#select_from').val();
var toID= $('#select_to').val();
I still don't get it right. Is it possible to add more variable from the outside selection todata: {productID: productID},
?
– Richmond
Jan 2 at 5:57
for exampledata: {productID: productID, fromID: fromID, toID: toID},
something like this
– Richmond
Jan 2 at 5:58
Updated my anwer, check the bottom, it is possible
– Second2None
Jan 2 at 6:02
Thanks for the help. I have found the solution myself. It's working now. I have updated the code
– Richmond
Jan 2 at 8:51
add a comment |
In your script, you are sending data like this : data: {productID: productID, fromID: fromID, toID: toID},
so, in getprice.php, you should receive those like this:
$fromID = $_POST['fromID'];
$toID = $_POST['toID'];
$productID = $_POST['productID'];
I have already try that but still not displaying the price and product id from the query. From the information in wordpress, sometimes this"$"
symbol must change to"jQuery"
in script but I also have tried that. Nothing seems to work. Is there anyway to monitor how the variable passes?
– Richmond
Jan 2 at 7:38
I have tried thisvar productID = jQuery(this).children(":selected").attr("id");
in the script. It works but productID is in the third selection. So how to get the id of the first and second option since both selection were outside from the third selection script so that I can post all 3 selection in one go instead of one by one ?
– Richmond
Jan 2 at 7:40
you can print the data in console to check whether they are passing or not. for this, in getprice.php, write:echo json_encode(array("fromID "=>$fromID ,"toID "=>$toID ,"productID "=>$productID ));
And then in ajax success function, write:console.log(json.fromID );console.log(json.toID);console.log(json.productID );
If values pass successfully, you can see these values in browser console. Its for checking only
– Nazem Mahmud
Jan 2 at 7:46
Thanks for the help. I have found the solution myself. It's working now. I have updated the code.
– Richmond
Jan 2 at 8:50
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54000846%2fhow-to-get-previous-selected-value-from-another-selection-into-current-selection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You are only sending productID in your ajax:
data: {productID: productID},
If you change your line to:
data: $('form[name="transport"]').serialize(),
Read more on serialize. Your jquery call will now look like:
jQuery(document).on("click", 'select#select_product', function(e)
{
$.ajax
({
type: "POST",
data: $('form[name="transport"]').serialize(),
url: 'http://interlink2u.com/transport/transportfiles/getprice.php',
dataType: 'json',
success: function(json)
{
var $el4 = jQuery("select#phive_product_id");
$el4.empty();
$.each(json, function(k, v)
{
$el4.append("<option id = '" + v.product_id + "'value = '" + v.product_id + "'>" + v.product_id + "</option>");
});
var $el5 = jQuery("select#phive_booked_price");
$el5.empty();
$.each(json, function(k, v)
{
$el5.append("<option id = '" + v.product_id + "'value = '" + v.price + "'>" + v.price + "</option>");
});
}
});
})
Then in your getprice.php code you would need to update:
$productID = $_POST['select_product']; // now select_product as per your selectbox name
But you also now have available:
$_POST['select_from']
$_POST['select_to']
$_POST['phive_booked_price']
$_POST['phive_product_id']
EDIT:
To include them the way you asked in the comments you would need to update:
var productID = $('#select_product').val();
var fromID = $('#select_from').val();
var toID= $('#select_to').val();
I still don't get it right. Is it possible to add more variable from the outside selection todata: {productID: productID},
?
– Richmond
Jan 2 at 5:57
for exampledata: {productID: productID, fromID: fromID, toID: toID},
something like this
– Richmond
Jan 2 at 5:58
Updated my anwer, check the bottom, it is possible
– Second2None
Jan 2 at 6:02
Thanks for the help. I have found the solution myself. It's working now. I have updated the code
– Richmond
Jan 2 at 8:51
add a comment |
You are only sending productID in your ajax:
data: {productID: productID},
If you change your line to:
data: $('form[name="transport"]').serialize(),
Read more on serialize. Your jquery call will now look like:
jQuery(document).on("click", 'select#select_product', function(e)
{
$.ajax
({
type: "POST",
data: $('form[name="transport"]').serialize(),
url: 'http://interlink2u.com/transport/transportfiles/getprice.php',
dataType: 'json',
success: function(json)
{
var $el4 = jQuery("select#phive_product_id");
$el4.empty();
$.each(json, function(k, v)
{
$el4.append("<option id = '" + v.product_id + "'value = '" + v.product_id + "'>" + v.product_id + "</option>");
});
var $el5 = jQuery("select#phive_booked_price");
$el5.empty();
$.each(json, function(k, v)
{
$el5.append("<option id = '" + v.product_id + "'value = '" + v.price + "'>" + v.price + "</option>");
});
}
});
})
Then in your getprice.php code you would need to update:
$productID = $_POST['select_product']; // now select_product as per your selectbox name
But you also now have available:
$_POST['select_from']
$_POST['select_to']
$_POST['phive_booked_price']
$_POST['phive_product_id']
EDIT:
To include them the way you asked in the comments you would need to update:
var productID = $('#select_product').val();
var fromID = $('#select_from').val();
var toID= $('#select_to').val();
I still don't get it right. Is it possible to add more variable from the outside selection todata: {productID: productID},
?
– Richmond
Jan 2 at 5:57
for exampledata: {productID: productID, fromID: fromID, toID: toID},
something like this
– Richmond
Jan 2 at 5:58
Updated my anwer, check the bottom, it is possible
– Second2None
Jan 2 at 6:02
Thanks for the help. I have found the solution myself. It's working now. I have updated the code
– Richmond
Jan 2 at 8:51
add a comment |
You are only sending productID in your ajax:
data: {productID: productID},
If you change your line to:
data: $('form[name="transport"]').serialize(),
Read more on serialize. Your jquery call will now look like:
jQuery(document).on("click", 'select#select_product', function(e)
{
$.ajax
({
type: "POST",
data: $('form[name="transport"]').serialize(),
url: 'http://interlink2u.com/transport/transportfiles/getprice.php',
dataType: 'json',
success: function(json)
{
var $el4 = jQuery("select#phive_product_id");
$el4.empty();
$.each(json, function(k, v)
{
$el4.append("<option id = '" + v.product_id + "'value = '" + v.product_id + "'>" + v.product_id + "</option>");
});
var $el5 = jQuery("select#phive_booked_price");
$el5.empty();
$.each(json, function(k, v)
{
$el5.append("<option id = '" + v.product_id + "'value = '" + v.price + "'>" + v.price + "</option>");
});
}
});
})
Then in your getprice.php code you would need to update:
$productID = $_POST['select_product']; // now select_product as per your selectbox name
But you also now have available:
$_POST['select_from']
$_POST['select_to']
$_POST['phive_booked_price']
$_POST['phive_product_id']
EDIT:
To include them the way you asked in the comments you would need to update:
var productID = $('#select_product').val();
var fromID = $('#select_from').val();
var toID= $('#select_to').val();
You are only sending productID in your ajax:
data: {productID: productID},
If you change your line to:
data: $('form[name="transport"]').serialize(),
Read more on serialize. Your jquery call will now look like:
jQuery(document).on("click", 'select#select_product', function(e)
{
$.ajax
({
type: "POST",
data: $('form[name="transport"]').serialize(),
url: 'http://interlink2u.com/transport/transportfiles/getprice.php',
dataType: 'json',
success: function(json)
{
var $el4 = jQuery("select#phive_product_id");
$el4.empty();
$.each(json, function(k, v)
{
$el4.append("<option id = '" + v.product_id + "'value = '" + v.product_id + "'>" + v.product_id + "</option>");
});
var $el5 = jQuery("select#phive_booked_price");
$el5.empty();
$.each(json, function(k, v)
{
$el5.append("<option id = '" + v.product_id + "'value = '" + v.price + "'>" + v.price + "</option>");
});
}
});
})
Then in your getprice.php code you would need to update:
$productID = $_POST['select_product']; // now select_product as per your selectbox name
But you also now have available:
$_POST['select_from']
$_POST['select_to']
$_POST['phive_booked_price']
$_POST['phive_product_id']
EDIT:
To include them the way you asked in the comments you would need to update:
var productID = $('#select_product').val();
var fromID = $('#select_from').val();
var toID= $('#select_to').val();
edited Jan 2 at 6:02
answered Jan 2 at 4:09


Second2NoneSecond2None
1,1301315
1,1301315
I still don't get it right. Is it possible to add more variable from the outside selection todata: {productID: productID},
?
– Richmond
Jan 2 at 5:57
for exampledata: {productID: productID, fromID: fromID, toID: toID},
something like this
– Richmond
Jan 2 at 5:58
Updated my anwer, check the bottom, it is possible
– Second2None
Jan 2 at 6:02
Thanks for the help. I have found the solution myself. It's working now. I have updated the code
– Richmond
Jan 2 at 8:51
add a comment |
I still don't get it right. Is it possible to add more variable from the outside selection todata: {productID: productID},
?
– Richmond
Jan 2 at 5:57
for exampledata: {productID: productID, fromID: fromID, toID: toID},
something like this
– Richmond
Jan 2 at 5:58
Updated my anwer, check the bottom, it is possible
– Second2None
Jan 2 at 6:02
Thanks for the help. I have found the solution myself. It's working now. I have updated the code
– Richmond
Jan 2 at 8:51
I still don't get it right. Is it possible to add more variable from the outside selection to
data: {productID: productID},
?– Richmond
Jan 2 at 5:57
I still don't get it right. Is it possible to add more variable from the outside selection to
data: {productID: productID},
?– Richmond
Jan 2 at 5:57
for example
data: {productID: productID, fromID: fromID, toID: toID},
something like this– Richmond
Jan 2 at 5:58
for example
data: {productID: productID, fromID: fromID, toID: toID},
something like this– Richmond
Jan 2 at 5:58
Updated my anwer, check the bottom, it is possible
– Second2None
Jan 2 at 6:02
Updated my anwer, check the bottom, it is possible
– Second2None
Jan 2 at 6:02
Thanks for the help. I have found the solution myself. It's working now. I have updated the code
– Richmond
Jan 2 at 8:51
Thanks for the help. I have found the solution myself. It's working now. I have updated the code
– Richmond
Jan 2 at 8:51
add a comment |
In your script, you are sending data like this : data: {productID: productID, fromID: fromID, toID: toID},
so, in getprice.php, you should receive those like this:
$fromID = $_POST['fromID'];
$toID = $_POST['toID'];
$productID = $_POST['productID'];
I have already try that but still not displaying the price and product id from the query. From the information in wordpress, sometimes this"$"
symbol must change to"jQuery"
in script but I also have tried that. Nothing seems to work. Is there anyway to monitor how the variable passes?
– Richmond
Jan 2 at 7:38
I have tried thisvar productID = jQuery(this).children(":selected").attr("id");
in the script. It works but productID is in the third selection. So how to get the id of the first and second option since both selection were outside from the third selection script so that I can post all 3 selection in one go instead of one by one ?
– Richmond
Jan 2 at 7:40
you can print the data in console to check whether they are passing or not. for this, in getprice.php, write:echo json_encode(array("fromID "=>$fromID ,"toID "=>$toID ,"productID "=>$productID ));
And then in ajax success function, write:console.log(json.fromID );console.log(json.toID);console.log(json.productID );
If values pass successfully, you can see these values in browser console. Its for checking only
– Nazem Mahmud
Jan 2 at 7:46
Thanks for the help. I have found the solution myself. It's working now. I have updated the code.
– Richmond
Jan 2 at 8:50
add a comment |
In your script, you are sending data like this : data: {productID: productID, fromID: fromID, toID: toID},
so, in getprice.php, you should receive those like this:
$fromID = $_POST['fromID'];
$toID = $_POST['toID'];
$productID = $_POST['productID'];
I have already try that but still not displaying the price and product id from the query. From the information in wordpress, sometimes this"$"
symbol must change to"jQuery"
in script but I also have tried that. Nothing seems to work. Is there anyway to monitor how the variable passes?
– Richmond
Jan 2 at 7:38
I have tried thisvar productID = jQuery(this).children(":selected").attr("id");
in the script. It works but productID is in the third selection. So how to get the id of the first and second option since both selection were outside from the third selection script so that I can post all 3 selection in one go instead of one by one ?
– Richmond
Jan 2 at 7:40
you can print the data in console to check whether they are passing or not. for this, in getprice.php, write:echo json_encode(array("fromID "=>$fromID ,"toID "=>$toID ,"productID "=>$productID ));
And then in ajax success function, write:console.log(json.fromID );console.log(json.toID);console.log(json.productID );
If values pass successfully, you can see these values in browser console. Its for checking only
– Nazem Mahmud
Jan 2 at 7:46
Thanks for the help. I have found the solution myself. It's working now. I have updated the code.
– Richmond
Jan 2 at 8:50
add a comment |
In your script, you are sending data like this : data: {productID: productID, fromID: fromID, toID: toID},
so, in getprice.php, you should receive those like this:
$fromID = $_POST['fromID'];
$toID = $_POST['toID'];
$productID = $_POST['productID'];
In your script, you are sending data like this : data: {productID: productID, fromID: fromID, toID: toID},
so, in getprice.php, you should receive those like this:
$fromID = $_POST['fromID'];
$toID = $_POST['toID'];
$productID = $_POST['productID'];
answered Jan 2 at 7:18
Nazem MahmudNazem Mahmud
12614
12614
I have already try that but still not displaying the price and product id from the query. From the information in wordpress, sometimes this"$"
symbol must change to"jQuery"
in script but I also have tried that. Nothing seems to work. Is there anyway to monitor how the variable passes?
– Richmond
Jan 2 at 7:38
I have tried thisvar productID = jQuery(this).children(":selected").attr("id");
in the script. It works but productID is in the third selection. So how to get the id of the first and second option since both selection were outside from the third selection script so that I can post all 3 selection in one go instead of one by one ?
– Richmond
Jan 2 at 7:40
you can print the data in console to check whether they are passing or not. for this, in getprice.php, write:echo json_encode(array("fromID "=>$fromID ,"toID "=>$toID ,"productID "=>$productID ));
And then in ajax success function, write:console.log(json.fromID );console.log(json.toID);console.log(json.productID );
If values pass successfully, you can see these values in browser console. Its for checking only
– Nazem Mahmud
Jan 2 at 7:46
Thanks for the help. I have found the solution myself. It's working now. I have updated the code.
– Richmond
Jan 2 at 8:50
add a comment |
I have already try that but still not displaying the price and product id from the query. From the information in wordpress, sometimes this"$"
symbol must change to"jQuery"
in script but I also have tried that. Nothing seems to work. Is there anyway to monitor how the variable passes?
– Richmond
Jan 2 at 7:38
I have tried thisvar productID = jQuery(this).children(":selected").attr("id");
in the script. It works but productID is in the third selection. So how to get the id of the first and second option since both selection were outside from the third selection script so that I can post all 3 selection in one go instead of one by one ?
– Richmond
Jan 2 at 7:40
you can print the data in console to check whether they are passing or not. for this, in getprice.php, write:echo json_encode(array("fromID "=>$fromID ,"toID "=>$toID ,"productID "=>$productID ));
And then in ajax success function, write:console.log(json.fromID );console.log(json.toID);console.log(json.productID );
If values pass successfully, you can see these values in browser console. Its for checking only
– Nazem Mahmud
Jan 2 at 7:46
Thanks for the help. I have found the solution myself. It's working now. I have updated the code.
– Richmond
Jan 2 at 8:50
I have already try that but still not displaying the price and product id from the query. From the information in wordpress, sometimes this
"$"
symbol must change to "jQuery"
in script but I also have tried that. Nothing seems to work. Is there anyway to monitor how the variable passes?– Richmond
Jan 2 at 7:38
I have already try that but still not displaying the price and product id from the query. From the information in wordpress, sometimes this
"$"
symbol must change to "jQuery"
in script but I also have tried that. Nothing seems to work. Is there anyway to monitor how the variable passes?– Richmond
Jan 2 at 7:38
I have tried this
var productID = jQuery(this).children(":selected").attr("id");
in the script. It works but productID is in the third selection. So how to get the id of the first and second option since both selection were outside from the third selection script so that I can post all 3 selection in one go instead of one by one ?– Richmond
Jan 2 at 7:40
I have tried this
var productID = jQuery(this).children(":selected").attr("id");
in the script. It works but productID is in the third selection. So how to get the id of the first and second option since both selection were outside from the third selection script so that I can post all 3 selection in one go instead of one by one ?– Richmond
Jan 2 at 7:40
you can print the data in console to check whether they are passing or not. for this, in getprice.php, write:
echo json_encode(array("fromID "=>$fromID ,"toID "=>$toID ,"productID "=>$productID ));
And then in ajax success function, write: console.log(json.fromID );console.log(json.toID);console.log(json.productID );
If values pass successfully, you can see these values in browser console. Its for checking only– Nazem Mahmud
Jan 2 at 7:46
you can print the data in console to check whether they are passing or not. for this, in getprice.php, write:
echo json_encode(array("fromID "=>$fromID ,"toID "=>$toID ,"productID "=>$productID ));
And then in ajax success function, write: console.log(json.fromID );console.log(json.toID);console.log(json.productID );
If values pass successfully, you can see these values in browser console. Its for checking only– Nazem Mahmud
Jan 2 at 7:46
Thanks for the help. I have found the solution myself. It's working now. I have updated the code.
– Richmond
Jan 2 at 8:50
Thanks for the help. I have found the solution myself. It's working now. I have updated the code.
– Richmond
Jan 2 at 8:50
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54000846%2fhow-to-get-previous-selected-value-from-another-selection-into-current-selection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
y2o,5GoSR0AfBjPFmxubSIRe,et,s kf