ValueError: invalid literal for int() with base 10: ''
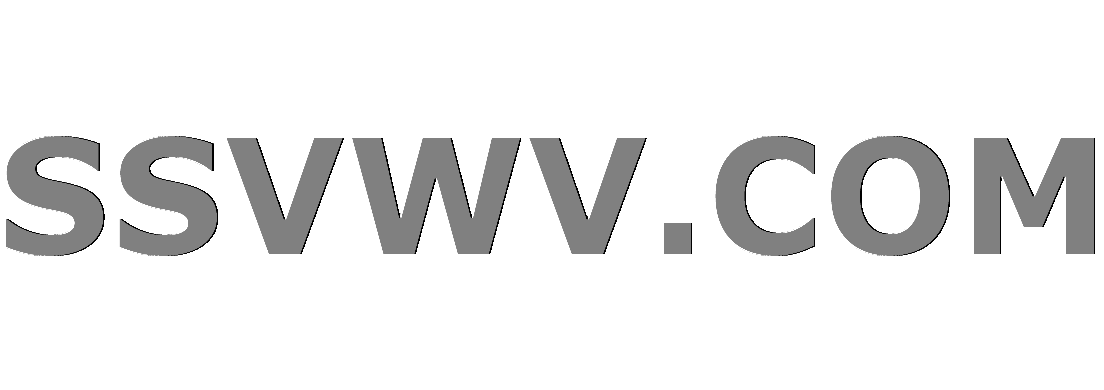
Multi tool use
I am creating a program that reads a file and if the first line of the file is not blank, it reads the next four lines. Calculations are performed on those lines and then the next line is read. If that line is not empty it continues. However, I am getting this error:
ValueError: invalid literal for int() with base 10: ''.`
It is reading the first line but can't convert it to an integer.
What can I do to fix this problem?
The Code:
file_to_read = raw_input("Enter file name of tests (empty string to end program):")
try:
infile = open(file_to_read, 'r')
while file_to_read != " ":
file_to_write = raw_input("Enter output file name (.csv will be appended to it):")
file_to_write = file_to_write + ".csv"
outfile = open(file_to_write, "w")
readings = (infile.readline())
print readings
while readings != 0:
global count
readings = int(readings)
minimum = (infile.readline())
maximum = (infile.readline())
python
add a comment |
I am creating a program that reads a file and if the first line of the file is not blank, it reads the next four lines. Calculations are performed on those lines and then the next line is read. If that line is not empty it continues. However, I am getting this error:
ValueError: invalid literal for int() with base 10: ''.`
It is reading the first line but can't convert it to an integer.
What can I do to fix this problem?
The Code:
file_to_read = raw_input("Enter file name of tests (empty string to end program):")
try:
infile = open(file_to_read, 'r')
while file_to_read != " ":
file_to_write = raw_input("Enter output file name (.csv will be appended to it):")
file_to_write = file_to_write + ".csv"
outfile = open(file_to_write, "w")
readings = (infile.readline())
print readings
while readings != 0:
global count
readings = int(readings)
minimum = (infile.readline())
maximum = (infile.readline())
python
You should consider usingwith open(file_to_read, 'r') as infile:
there.
– Omnifarious
Sep 14 '17 at 11:48
add a comment |
I am creating a program that reads a file and if the first line of the file is not blank, it reads the next four lines. Calculations are performed on those lines and then the next line is read. If that line is not empty it continues. However, I am getting this error:
ValueError: invalid literal for int() with base 10: ''.`
It is reading the first line but can't convert it to an integer.
What can I do to fix this problem?
The Code:
file_to_read = raw_input("Enter file name of tests (empty string to end program):")
try:
infile = open(file_to_read, 'r')
while file_to_read != " ":
file_to_write = raw_input("Enter output file name (.csv will be appended to it):")
file_to_write = file_to_write + ".csv"
outfile = open(file_to_write, "w")
readings = (infile.readline())
print readings
while readings != 0:
global count
readings = int(readings)
minimum = (infile.readline())
maximum = (infile.readline())
python
I am creating a program that reads a file and if the first line of the file is not blank, it reads the next four lines. Calculations are performed on those lines and then the next line is read. If that line is not empty it continues. However, I am getting this error:
ValueError: invalid literal for int() with base 10: ''.`
It is reading the first line but can't convert it to an integer.
What can I do to fix this problem?
The Code:
file_to_read = raw_input("Enter file name of tests (empty string to end program):")
try:
infile = open(file_to_read, 'r')
while file_to_read != " ":
file_to_write = raw_input("Enter output file name (.csv will be appended to it):")
file_to_write = file_to_write + ".csv"
outfile = open(file_to_write, "w")
readings = (infile.readline())
print readings
while readings != 0:
global count
readings = int(readings)
minimum = (infile.readline())
maximum = (infile.readline())
python
python
edited Dec 9 '09 at 10:18


Peter Mortensen
13.6k1984111
13.6k1984111
asked Dec 3 '09 at 17:34
Sarah CoxSarah Cox
904273
904273
You should consider usingwith open(file_to_read, 'r') as infile:
there.
– Omnifarious
Sep 14 '17 at 11:48
add a comment |
You should consider usingwith open(file_to_read, 'r') as infile:
there.
– Omnifarious
Sep 14 '17 at 11:48
You should consider using
with open(file_to_read, 'r') as infile:
there.– Omnifarious
Sep 14 '17 at 11:48
You should consider using
with open(file_to_read, 'r') as infile:
there.– Omnifarious
Sep 14 '17 at 11:48
add a comment |
12 Answers
12
active
oldest
votes
Just for the record:
>>> int('55063.000000')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 10: '55063.000000'
Got me here...
>>> float('55063.000000')
55063.0
Has to be used!
65
I'll just add, to provide more clarity for future readers, that indeed, int(float('1.0')) works when int('1.0') throws the ValueError.
– katyhuff
Apr 26 '13 at 16:53
3
This should be the accepted top answer to this question. I almost closed the page and didn't see it. Thanks!
– iTurki
Jun 21 '16 at 21:44
2
add to answer int(float('55063.000000')) as question is get int(). Than it will realy top answer
– Max
Mar 15 '17 at 8:41
Why does this happen? @katyhuff
– Muhamed Huseinbašić
Jun 8 '17 at 14:03
2
This answer doesn't appear to have anything to do with the question. The question is asking how one could prevent a ValueError when you callint()
on an empty string. "Use float() instead" doesn't solve that problem. You still get a ValueError.
– Kevin
May 1 '18 at 18:50
add a comment |
Pythonic way of iterating over a file and converting to int:
for line in open(fname):
if line.strip(): # line contains eol character(s)
n = int(line) # assuming single integer on each line
What you're trying to do is slightly more complicated, but still not straight-forward:
h = open(fname)
for line in h:
if line.strip():
[int(next(h).strip()) for _ in range(4)] # list of integers
This way it processes 5 lines at the time. Use h.next()
instead of next(h)
prior to Python 2.6.
The reason you had ValueError
is because int
cannot convert an empty string to the integer. In this case you'd need to either check the content of the string before conversion, or except an error:
try:
int('')
except ValueError:
pass # or whatever
4
Your try/except doesn't distinguish between something reasonable expectable (blank/empty line) and something nasty like a non-integer.
– John Machin
Dec 3 '09 at 19:47
and why would you want to distinguish those things?
– SilentGhost
Dec 4 '09 at 1:34
2
because one is reasonably expectable and ignorable but the other is indicative of an error
– John Machin
Dec 4 '09 at 21:07
3
and why is a blank line reasonably expectable and non-integer is not?
– SilentGhost
Dec 5 '09 at 2:18
this is good if you are sure that your list is actually a list of stringified integers. If you are not sure you can don = int(line) if line.is_integer() else int(float(line))
– Mike Furlender
Jul 25 '18 at 15:30
add a comment |
The following are totally acceptable in python:
- passing a string representation of an integer into
int
- passing a string representation of a float into
float
- passing a string representation of an integer into
float
- passing a float into
int
- passing an integer into
float
But you get a ValueError
if you pass a string representation of a float into int
, or a string representation of anything but an integer (including empty string). If you do want to pass a string representation of a float to an int
, as @katyhuff points out above, you can convert to a float first, then to an integer:
>>> int('5')
5
>>> float('5.0')
5.0
>>> float('5')
5.0
>>> int(5.0)
5
>>> float(5)
5.0
>>> int('5.0')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 10: '5.0'
>>> int(float('5.0'))
5
2
This answer doesn't appear to have anything to do with the question. The question is asking how one could prevent a ValueError when you callint()
on an empty string. "Use float() instead" doesn't solve that problem. You still get a ValueError.
– Kevin
May 1 '18 at 18:53
add a comment |
Reason Is that You are getting a empty string or string as a argument into int
check it before is it empty or it contains alpha characters or not if it contains than ignore that part simply.
1
This looks more like a comment. When you have sufficient reputation you will be able to comment on any post.
– Joshua Drake
Jun 23 '17 at 13:39
Ok Reputation...!!!
– rajender kumar
Jun 24 '17 at 9:12
add a comment |
You've got a problem with this line:
while file_to_read != " ":
This does not find an empty string. It finds a string consisting of one space. Presumably this is not what you are looking for.
Listen to everyone else's advice. This is not very idiomatic python code, and would be much clearer if you iterate over the file directly, but I think this problem is worth noting as well.
add a comment |
Please test this function (split()
) on a simple file. I was facing the same issue and found that it was because split()
was not written properly (exception handling).
add a comment |
The reason you are getting this error is that you are trying to convert a space character to an integer, which is totally impossible and restricted.And that's why you are getting this error.
Check your code and correct it, it will work fine
add a comment |
readings = (infile.readline())
print readings
while readings != 0:
global count
readings = int(readings)
There's a problem with that code. readings
is a new line read from the file - it's a string. Therefore you should not compare it to 0. Further, you can't just convert it to an integer unless you're sure it's indeed one. For example, empty lines will produce errors here (as you've surely found out).
And why do you need the global count? That's most certainly bad design in Python.
add a comment |
I am creating a program that reads a
file and if the first line of the file
is not blank, it reads the next four
lines. Calculations are performed on
those lines and then the next line is
read.
Something like this should work:
for line in infile:
next_lines =
if line.strip():
for i in xrange(4):
try:
next_lines.append(infile.next())
except StopIteration:
break
# Do your calculation with "4 lines" here
add a comment |
I was getting similar errors, turns out that the dataset had blank values which python could not convert to integer.
3
Could you elaborate a bit more with some examples on how the OP could solve it?
– fmendez
Mar 12 '13 at 20:00
add a comment |
This could also happen when you have to map space separated integers to a list but you enter the integers line by line using the .input()
.
Like for example I was solving this problem on HackerRank Bon-Appetit, and the got the following error while compiling
So instead of giving input to the program line by line try to map the space separated integers into a list using the map()
method.
add a comment |
I got into the same issue when trying to use readlines() inside for loop for same file object... My suspicion is firing readling() inside readline() for same file object caused this error.
Best solution can be use seek(0) to reset file pointer or
Handle condition with setting some flag then create new object for same file by checking set condition....
add a comment |
protected by Community♦ Feb 16 '18 at 17:03
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
12 Answers
12
active
oldest
votes
12 Answers
12
active
oldest
votes
active
oldest
votes
active
oldest
votes
Just for the record:
>>> int('55063.000000')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 10: '55063.000000'
Got me here...
>>> float('55063.000000')
55063.0
Has to be used!
65
I'll just add, to provide more clarity for future readers, that indeed, int(float('1.0')) works when int('1.0') throws the ValueError.
– katyhuff
Apr 26 '13 at 16:53
3
This should be the accepted top answer to this question. I almost closed the page and didn't see it. Thanks!
– iTurki
Jun 21 '16 at 21:44
2
add to answer int(float('55063.000000')) as question is get int(). Than it will realy top answer
– Max
Mar 15 '17 at 8:41
Why does this happen? @katyhuff
– Muhamed Huseinbašić
Jun 8 '17 at 14:03
2
This answer doesn't appear to have anything to do with the question. The question is asking how one could prevent a ValueError when you callint()
on an empty string. "Use float() instead" doesn't solve that problem. You still get a ValueError.
– Kevin
May 1 '18 at 18:50
add a comment |
Just for the record:
>>> int('55063.000000')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 10: '55063.000000'
Got me here...
>>> float('55063.000000')
55063.0
Has to be used!
65
I'll just add, to provide more clarity for future readers, that indeed, int(float('1.0')) works when int('1.0') throws the ValueError.
– katyhuff
Apr 26 '13 at 16:53
3
This should be the accepted top answer to this question. I almost closed the page and didn't see it. Thanks!
– iTurki
Jun 21 '16 at 21:44
2
add to answer int(float('55063.000000')) as question is get int(). Than it will realy top answer
– Max
Mar 15 '17 at 8:41
Why does this happen? @katyhuff
– Muhamed Huseinbašić
Jun 8 '17 at 14:03
2
This answer doesn't appear to have anything to do with the question. The question is asking how one could prevent a ValueError when you callint()
on an empty string. "Use float() instead" doesn't solve that problem. You still get a ValueError.
– Kevin
May 1 '18 at 18:50
add a comment |
Just for the record:
>>> int('55063.000000')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 10: '55063.000000'
Got me here...
>>> float('55063.000000')
55063.0
Has to be used!
Just for the record:
>>> int('55063.000000')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 10: '55063.000000'
Got me here...
>>> float('55063.000000')
55063.0
Has to be used!
answered Jan 20 '12 at 21:49
FdoBadFdoBad
2,115192
2,115192
65
I'll just add, to provide more clarity for future readers, that indeed, int(float('1.0')) works when int('1.0') throws the ValueError.
– katyhuff
Apr 26 '13 at 16:53
3
This should be the accepted top answer to this question. I almost closed the page and didn't see it. Thanks!
– iTurki
Jun 21 '16 at 21:44
2
add to answer int(float('55063.000000')) as question is get int(). Than it will realy top answer
– Max
Mar 15 '17 at 8:41
Why does this happen? @katyhuff
– Muhamed Huseinbašić
Jun 8 '17 at 14:03
2
This answer doesn't appear to have anything to do with the question. The question is asking how one could prevent a ValueError when you callint()
on an empty string. "Use float() instead" doesn't solve that problem. You still get a ValueError.
– Kevin
May 1 '18 at 18:50
add a comment |
65
I'll just add, to provide more clarity for future readers, that indeed, int(float('1.0')) works when int('1.0') throws the ValueError.
– katyhuff
Apr 26 '13 at 16:53
3
This should be the accepted top answer to this question. I almost closed the page and didn't see it. Thanks!
– iTurki
Jun 21 '16 at 21:44
2
add to answer int(float('55063.000000')) as question is get int(). Than it will realy top answer
– Max
Mar 15 '17 at 8:41
Why does this happen? @katyhuff
– Muhamed Huseinbašić
Jun 8 '17 at 14:03
2
This answer doesn't appear to have anything to do with the question. The question is asking how one could prevent a ValueError when you callint()
on an empty string. "Use float() instead" doesn't solve that problem. You still get a ValueError.
– Kevin
May 1 '18 at 18:50
65
65
I'll just add, to provide more clarity for future readers, that indeed, int(float('1.0')) works when int('1.0') throws the ValueError.
– katyhuff
Apr 26 '13 at 16:53
I'll just add, to provide more clarity for future readers, that indeed, int(float('1.0')) works when int('1.0') throws the ValueError.
– katyhuff
Apr 26 '13 at 16:53
3
3
This should be the accepted top answer to this question. I almost closed the page and didn't see it. Thanks!
– iTurki
Jun 21 '16 at 21:44
This should be the accepted top answer to this question. I almost closed the page and didn't see it. Thanks!
– iTurki
Jun 21 '16 at 21:44
2
2
add to answer int(float('55063.000000')) as question is get int(). Than it will realy top answer
– Max
Mar 15 '17 at 8:41
add to answer int(float('55063.000000')) as question is get int(). Than it will realy top answer
– Max
Mar 15 '17 at 8:41
Why does this happen? @katyhuff
– Muhamed Huseinbašić
Jun 8 '17 at 14:03
Why does this happen? @katyhuff
– Muhamed Huseinbašić
Jun 8 '17 at 14:03
2
2
This answer doesn't appear to have anything to do with the question. The question is asking how one could prevent a ValueError when you call
int()
on an empty string. "Use float() instead" doesn't solve that problem. You still get a ValueError.– Kevin
May 1 '18 at 18:50
This answer doesn't appear to have anything to do with the question. The question is asking how one could prevent a ValueError when you call
int()
on an empty string. "Use float() instead" doesn't solve that problem. You still get a ValueError.– Kevin
May 1 '18 at 18:50
add a comment |
Pythonic way of iterating over a file and converting to int:
for line in open(fname):
if line.strip(): # line contains eol character(s)
n = int(line) # assuming single integer on each line
What you're trying to do is slightly more complicated, but still not straight-forward:
h = open(fname)
for line in h:
if line.strip():
[int(next(h).strip()) for _ in range(4)] # list of integers
This way it processes 5 lines at the time. Use h.next()
instead of next(h)
prior to Python 2.6.
The reason you had ValueError
is because int
cannot convert an empty string to the integer. In this case you'd need to either check the content of the string before conversion, or except an error:
try:
int('')
except ValueError:
pass # or whatever
4
Your try/except doesn't distinguish between something reasonable expectable (blank/empty line) and something nasty like a non-integer.
– John Machin
Dec 3 '09 at 19:47
and why would you want to distinguish those things?
– SilentGhost
Dec 4 '09 at 1:34
2
because one is reasonably expectable and ignorable but the other is indicative of an error
– John Machin
Dec 4 '09 at 21:07
3
and why is a blank line reasonably expectable and non-integer is not?
– SilentGhost
Dec 5 '09 at 2:18
this is good if you are sure that your list is actually a list of stringified integers. If you are not sure you can don = int(line) if line.is_integer() else int(float(line))
– Mike Furlender
Jul 25 '18 at 15:30
add a comment |
Pythonic way of iterating over a file and converting to int:
for line in open(fname):
if line.strip(): # line contains eol character(s)
n = int(line) # assuming single integer on each line
What you're trying to do is slightly more complicated, but still not straight-forward:
h = open(fname)
for line in h:
if line.strip():
[int(next(h).strip()) for _ in range(4)] # list of integers
This way it processes 5 lines at the time. Use h.next()
instead of next(h)
prior to Python 2.6.
The reason you had ValueError
is because int
cannot convert an empty string to the integer. In this case you'd need to either check the content of the string before conversion, or except an error:
try:
int('')
except ValueError:
pass # or whatever
4
Your try/except doesn't distinguish between something reasonable expectable (blank/empty line) and something nasty like a non-integer.
– John Machin
Dec 3 '09 at 19:47
and why would you want to distinguish those things?
– SilentGhost
Dec 4 '09 at 1:34
2
because one is reasonably expectable and ignorable but the other is indicative of an error
– John Machin
Dec 4 '09 at 21:07
3
and why is a blank line reasonably expectable and non-integer is not?
– SilentGhost
Dec 5 '09 at 2:18
this is good if you are sure that your list is actually a list of stringified integers. If you are not sure you can don = int(line) if line.is_integer() else int(float(line))
– Mike Furlender
Jul 25 '18 at 15:30
add a comment |
Pythonic way of iterating over a file and converting to int:
for line in open(fname):
if line.strip(): # line contains eol character(s)
n = int(line) # assuming single integer on each line
What you're trying to do is slightly more complicated, but still not straight-forward:
h = open(fname)
for line in h:
if line.strip():
[int(next(h).strip()) for _ in range(4)] # list of integers
This way it processes 5 lines at the time. Use h.next()
instead of next(h)
prior to Python 2.6.
The reason you had ValueError
is because int
cannot convert an empty string to the integer. In this case you'd need to either check the content of the string before conversion, or except an error:
try:
int('')
except ValueError:
pass # or whatever
Pythonic way of iterating over a file and converting to int:
for line in open(fname):
if line.strip(): # line contains eol character(s)
n = int(line) # assuming single integer on each line
What you're trying to do is slightly more complicated, but still not straight-forward:
h = open(fname)
for line in h:
if line.strip():
[int(next(h).strip()) for _ in range(4)] # list of integers
This way it processes 5 lines at the time. Use h.next()
instead of next(h)
prior to Python 2.6.
The reason you had ValueError
is because int
cannot convert an empty string to the integer. In this case you'd need to either check the content of the string before conversion, or except an error:
try:
int('')
except ValueError:
pass # or whatever
edited Dec 9 '09 at 10:21


Peter Mortensen
13.6k1984111
13.6k1984111
answered Dec 3 '09 at 17:40
SilentGhostSilentGhost
194k47265263
194k47265263
4
Your try/except doesn't distinguish between something reasonable expectable (blank/empty line) and something nasty like a non-integer.
– John Machin
Dec 3 '09 at 19:47
and why would you want to distinguish those things?
– SilentGhost
Dec 4 '09 at 1:34
2
because one is reasonably expectable and ignorable but the other is indicative of an error
– John Machin
Dec 4 '09 at 21:07
3
and why is a blank line reasonably expectable and non-integer is not?
– SilentGhost
Dec 5 '09 at 2:18
this is good if you are sure that your list is actually a list of stringified integers. If you are not sure you can don = int(line) if line.is_integer() else int(float(line))
– Mike Furlender
Jul 25 '18 at 15:30
add a comment |
4
Your try/except doesn't distinguish between something reasonable expectable (blank/empty line) and something nasty like a non-integer.
– John Machin
Dec 3 '09 at 19:47
and why would you want to distinguish those things?
– SilentGhost
Dec 4 '09 at 1:34
2
because one is reasonably expectable and ignorable but the other is indicative of an error
– John Machin
Dec 4 '09 at 21:07
3
and why is a blank line reasonably expectable and non-integer is not?
– SilentGhost
Dec 5 '09 at 2:18
this is good if you are sure that your list is actually a list of stringified integers. If you are not sure you can don = int(line) if line.is_integer() else int(float(line))
– Mike Furlender
Jul 25 '18 at 15:30
4
4
Your try/except doesn't distinguish between something reasonable expectable (blank/empty line) and something nasty like a non-integer.
– John Machin
Dec 3 '09 at 19:47
Your try/except doesn't distinguish between something reasonable expectable (blank/empty line) and something nasty like a non-integer.
– John Machin
Dec 3 '09 at 19:47
and why would you want to distinguish those things?
– SilentGhost
Dec 4 '09 at 1:34
and why would you want to distinguish those things?
– SilentGhost
Dec 4 '09 at 1:34
2
2
because one is reasonably expectable and ignorable but the other is indicative of an error
– John Machin
Dec 4 '09 at 21:07
because one is reasonably expectable and ignorable but the other is indicative of an error
– John Machin
Dec 4 '09 at 21:07
3
3
and why is a blank line reasonably expectable and non-integer is not?
– SilentGhost
Dec 5 '09 at 2:18
and why is a blank line reasonably expectable and non-integer is not?
– SilentGhost
Dec 5 '09 at 2:18
this is good if you are sure that your list is actually a list of stringified integers. If you are not sure you can do
n = int(line) if line.is_integer() else int(float(line))
– Mike Furlender
Jul 25 '18 at 15:30
this is good if you are sure that your list is actually a list of stringified integers. If you are not sure you can do
n = int(line) if line.is_integer() else int(float(line))
– Mike Furlender
Jul 25 '18 at 15:30
add a comment |
The following are totally acceptable in python:
- passing a string representation of an integer into
int
- passing a string representation of a float into
float
- passing a string representation of an integer into
float
- passing a float into
int
- passing an integer into
float
But you get a ValueError
if you pass a string representation of a float into int
, or a string representation of anything but an integer (including empty string). If you do want to pass a string representation of a float to an int
, as @katyhuff points out above, you can convert to a float first, then to an integer:
>>> int('5')
5
>>> float('5.0')
5.0
>>> float('5')
5.0
>>> int(5.0)
5
>>> float(5)
5.0
>>> int('5.0')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 10: '5.0'
>>> int(float('5.0'))
5
2
This answer doesn't appear to have anything to do with the question. The question is asking how one could prevent a ValueError when you callint()
on an empty string. "Use float() instead" doesn't solve that problem. You still get a ValueError.
– Kevin
May 1 '18 at 18:53
add a comment |
The following are totally acceptable in python:
- passing a string representation of an integer into
int
- passing a string representation of a float into
float
- passing a string representation of an integer into
float
- passing a float into
int
- passing an integer into
float
But you get a ValueError
if you pass a string representation of a float into int
, or a string representation of anything but an integer (including empty string). If you do want to pass a string representation of a float to an int
, as @katyhuff points out above, you can convert to a float first, then to an integer:
>>> int('5')
5
>>> float('5.0')
5.0
>>> float('5')
5.0
>>> int(5.0)
5
>>> float(5)
5.0
>>> int('5.0')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 10: '5.0'
>>> int(float('5.0'))
5
2
This answer doesn't appear to have anything to do with the question. The question is asking how one could prevent a ValueError when you callint()
on an empty string. "Use float() instead" doesn't solve that problem. You still get a ValueError.
– Kevin
May 1 '18 at 18:53
add a comment |
The following are totally acceptable in python:
- passing a string representation of an integer into
int
- passing a string representation of a float into
float
- passing a string representation of an integer into
float
- passing a float into
int
- passing an integer into
float
But you get a ValueError
if you pass a string representation of a float into int
, or a string representation of anything but an integer (including empty string). If you do want to pass a string representation of a float to an int
, as @katyhuff points out above, you can convert to a float first, then to an integer:
>>> int('5')
5
>>> float('5.0')
5.0
>>> float('5')
5.0
>>> int(5.0)
5
>>> float(5)
5.0
>>> int('5.0')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 10: '5.0'
>>> int(float('5.0'))
5
The following are totally acceptable in python:
- passing a string representation of an integer into
int
- passing a string representation of a float into
float
- passing a string representation of an integer into
float
- passing a float into
int
- passing an integer into
float
But you get a ValueError
if you pass a string representation of a float into int
, or a string representation of anything but an integer (including empty string). If you do want to pass a string representation of a float to an int
, as @katyhuff points out above, you can convert to a float first, then to an integer:
>>> int('5')
5
>>> float('5.0')
5.0
>>> float('5')
5.0
>>> int(5.0)
5
>>> float(5)
5.0
>>> int('5.0')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 10: '5.0'
>>> int(float('5.0'))
5
edited May 23 '18 at 0:24
answered Dec 12 '17 at 2:26


Peter Skewes-CoxPeter Skewes-Cox
45147
45147
2
This answer doesn't appear to have anything to do with the question. The question is asking how one could prevent a ValueError when you callint()
on an empty string. "Use float() instead" doesn't solve that problem. You still get a ValueError.
– Kevin
May 1 '18 at 18:53
add a comment |
2
This answer doesn't appear to have anything to do with the question. The question is asking how one could prevent a ValueError when you callint()
on an empty string. "Use float() instead" doesn't solve that problem. You still get a ValueError.
– Kevin
May 1 '18 at 18:53
2
2
This answer doesn't appear to have anything to do with the question. The question is asking how one could prevent a ValueError when you call
int()
on an empty string. "Use float() instead" doesn't solve that problem. You still get a ValueError.– Kevin
May 1 '18 at 18:53
This answer doesn't appear to have anything to do with the question. The question is asking how one could prevent a ValueError when you call
int()
on an empty string. "Use float() instead" doesn't solve that problem. You still get a ValueError.– Kevin
May 1 '18 at 18:53
add a comment |
Reason Is that You are getting a empty string or string as a argument into int
check it before is it empty or it contains alpha characters or not if it contains than ignore that part simply.
1
This looks more like a comment. When you have sufficient reputation you will be able to comment on any post.
– Joshua Drake
Jun 23 '17 at 13:39
Ok Reputation...!!!
– rajender kumar
Jun 24 '17 at 9:12
add a comment |
Reason Is that You are getting a empty string or string as a argument into int
check it before is it empty or it contains alpha characters or not if it contains than ignore that part simply.
1
This looks more like a comment. When you have sufficient reputation you will be able to comment on any post.
– Joshua Drake
Jun 23 '17 at 13:39
Ok Reputation...!!!
– rajender kumar
Jun 24 '17 at 9:12
add a comment |
Reason Is that You are getting a empty string or string as a argument into int
check it before is it empty or it contains alpha characters or not if it contains than ignore that part simply.
Reason Is that You are getting a empty string or string as a argument into int
check it before is it empty or it contains alpha characters or not if it contains than ignore that part simply.
answered Jun 23 '17 at 13:19
rajender kumarrajender kumar
13616
13616
1
This looks more like a comment. When you have sufficient reputation you will be able to comment on any post.
– Joshua Drake
Jun 23 '17 at 13:39
Ok Reputation...!!!
– rajender kumar
Jun 24 '17 at 9:12
add a comment |
1
This looks more like a comment. When you have sufficient reputation you will be able to comment on any post.
– Joshua Drake
Jun 23 '17 at 13:39
Ok Reputation...!!!
– rajender kumar
Jun 24 '17 at 9:12
1
1
This looks more like a comment. When you have sufficient reputation you will be able to comment on any post.
– Joshua Drake
Jun 23 '17 at 13:39
This looks more like a comment. When you have sufficient reputation you will be able to comment on any post.
– Joshua Drake
Jun 23 '17 at 13:39
Ok Reputation...!!!
– rajender kumar
Jun 24 '17 at 9:12
Ok Reputation...!!!
– rajender kumar
Jun 24 '17 at 9:12
add a comment |
You've got a problem with this line:
while file_to_read != " ":
This does not find an empty string. It finds a string consisting of one space. Presumably this is not what you are looking for.
Listen to everyone else's advice. This is not very idiomatic python code, and would be much clearer if you iterate over the file directly, but I think this problem is worth noting as well.
add a comment |
You've got a problem with this line:
while file_to_read != " ":
This does not find an empty string. It finds a string consisting of one space. Presumably this is not what you are looking for.
Listen to everyone else's advice. This is not very idiomatic python code, and would be much clearer if you iterate over the file directly, but I think this problem is worth noting as well.
add a comment |
You've got a problem with this line:
while file_to_read != " ":
This does not find an empty string. It finds a string consisting of one space. Presumably this is not what you are looking for.
Listen to everyone else's advice. This is not very idiomatic python code, and would be much clearer if you iterate over the file directly, but I think this problem is worth noting as well.
You've got a problem with this line:
while file_to_read != " ":
This does not find an empty string. It finds a string consisting of one space. Presumably this is not what you are looking for.
Listen to everyone else's advice. This is not very idiomatic python code, and would be much clearer if you iterate over the file directly, but I think this problem is worth noting as well.
answered Dec 3 '09 at 17:56
jcdyerjcdyer
14k33345
14k33345
add a comment |
add a comment |
Please test this function (split()
) on a simple file. I was facing the same issue and found that it was because split()
was not written properly (exception handling).
add a comment |
Please test this function (split()
) on a simple file. I was facing the same issue and found that it was because split()
was not written properly (exception handling).
add a comment |
Please test this function (split()
) on a simple file. I was facing the same issue and found that it was because split()
was not written properly (exception handling).
Please test this function (split()
) on a simple file. I was facing the same issue and found that it was because split()
was not written properly (exception handling).
edited Jun 5 '14 at 15:00


Ed Cottrell♦
36.8k125380
36.8k125380
answered Jun 5 '14 at 11:46
user3446207
add a comment |
add a comment |
The reason you are getting this error is that you are trying to convert a space character to an integer, which is totally impossible and restricted.And that's why you are getting this error.
Check your code and correct it, it will work fine
add a comment |
The reason you are getting this error is that you are trying to convert a space character to an integer, which is totally impossible and restricted.And that's why you are getting this error.
Check your code and correct it, it will work fine
add a comment |
The reason you are getting this error is that you are trying to convert a space character to an integer, which is totally impossible and restricted.And that's why you are getting this error.
Check your code and correct it, it will work fine
The reason you are getting this error is that you are trying to convert a space character to an integer, which is totally impossible and restricted.And that's why you are getting this error.
Check your code and correct it, it will work fine
answered Mar 27 '18 at 6:59
JoishJoish
419412
419412
add a comment |
add a comment |
readings = (infile.readline())
print readings
while readings != 0:
global count
readings = int(readings)
There's a problem with that code. readings
is a new line read from the file - it's a string. Therefore you should not compare it to 0. Further, you can't just convert it to an integer unless you're sure it's indeed one. For example, empty lines will produce errors here (as you've surely found out).
And why do you need the global count? That's most certainly bad design in Python.
add a comment |
readings = (infile.readline())
print readings
while readings != 0:
global count
readings = int(readings)
There's a problem with that code. readings
is a new line read from the file - it's a string. Therefore you should not compare it to 0. Further, you can't just convert it to an integer unless you're sure it's indeed one. For example, empty lines will produce errors here (as you've surely found out).
And why do you need the global count? That's most certainly bad design in Python.
add a comment |
readings = (infile.readline())
print readings
while readings != 0:
global count
readings = int(readings)
There's a problem with that code. readings
is a new line read from the file - it's a string. Therefore you should not compare it to 0. Further, you can't just convert it to an integer unless you're sure it's indeed one. For example, empty lines will produce errors here (as you've surely found out).
And why do you need the global count? That's most certainly bad design in Python.
readings = (infile.readline())
print readings
while readings != 0:
global count
readings = int(readings)
There's a problem with that code. readings
is a new line read from the file - it's a string. Therefore you should not compare it to 0. Further, you can't just convert it to an integer unless you're sure it's indeed one. For example, empty lines will produce errors here (as you've surely found out).
And why do you need the global count? That's most certainly bad design in Python.
answered Dec 3 '09 at 17:43
Eli BenderskyEli Bendersky
165k67297370
165k67297370
add a comment |
add a comment |
I am creating a program that reads a
file and if the first line of the file
is not blank, it reads the next four
lines. Calculations are performed on
those lines and then the next line is
read.
Something like this should work:
for line in infile:
next_lines =
if line.strip():
for i in xrange(4):
try:
next_lines.append(infile.next())
except StopIteration:
break
# Do your calculation with "4 lines" here
add a comment |
I am creating a program that reads a
file and if the first line of the file
is not blank, it reads the next four
lines. Calculations are performed on
those lines and then the next line is
read.
Something like this should work:
for line in infile:
next_lines =
if line.strip():
for i in xrange(4):
try:
next_lines.append(infile.next())
except StopIteration:
break
# Do your calculation with "4 lines" here
add a comment |
I am creating a program that reads a
file and if the first line of the file
is not blank, it reads the next four
lines. Calculations are performed on
those lines and then the next line is
read.
Something like this should work:
for line in infile:
next_lines =
if line.strip():
for i in xrange(4):
try:
next_lines.append(infile.next())
except StopIteration:
break
# Do your calculation with "4 lines" here
I am creating a program that reads a
file and if the first line of the file
is not blank, it reads the next four
lines. Calculations are performed on
those lines and then the next line is
read.
Something like this should work:
for line in infile:
next_lines =
if line.strip():
for i in xrange(4):
try:
next_lines.append(infile.next())
except StopIteration:
break
# Do your calculation with "4 lines" here
answered Dec 3 '09 at 17:49
ImranImran
44.1k2085116
44.1k2085116
add a comment |
add a comment |
I was getting similar errors, turns out that the dataset had blank values which python could not convert to integer.
3
Could you elaborate a bit more with some examples on how the OP could solve it?
– fmendez
Mar 12 '13 at 20:00
add a comment |
I was getting similar errors, turns out that the dataset had blank values which python could not convert to integer.
3
Could you elaborate a bit more with some examples on how the OP could solve it?
– fmendez
Mar 12 '13 at 20:00
add a comment |
I was getting similar errors, turns out that the dataset had blank values which python could not convert to integer.
I was getting similar errors, turns out that the dataset had blank values which python could not convert to integer.
answered Mar 12 '13 at 19:38
user2162611user2162611
727
727
3
Could you elaborate a bit more with some examples on how the OP could solve it?
– fmendez
Mar 12 '13 at 20:00
add a comment |
3
Could you elaborate a bit more with some examples on how the OP could solve it?
– fmendez
Mar 12 '13 at 20:00
3
3
Could you elaborate a bit more with some examples on how the OP could solve it?
– fmendez
Mar 12 '13 at 20:00
Could you elaborate a bit more with some examples on how the OP could solve it?
– fmendez
Mar 12 '13 at 20:00
add a comment |
This could also happen when you have to map space separated integers to a list but you enter the integers line by line using the .input()
.
Like for example I was solving this problem on HackerRank Bon-Appetit, and the got the following error while compiling
So instead of giving input to the program line by line try to map the space separated integers into a list using the map()
method.
add a comment |
This could also happen when you have to map space separated integers to a list but you enter the integers line by line using the .input()
.
Like for example I was solving this problem on HackerRank Bon-Appetit, and the got the following error while compiling
So instead of giving input to the program line by line try to map the space separated integers into a list using the map()
method.
add a comment |
This could also happen when you have to map space separated integers to a list but you enter the integers line by line using the .input()
.
Like for example I was solving this problem on HackerRank Bon-Appetit, and the got the following error while compiling
So instead of giving input to the program line by line try to map the space separated integers into a list using the map()
method.
This could also happen when you have to map space separated integers to a list but you enter the integers line by line using the .input()
.
Like for example I was solving this problem on HackerRank Bon-Appetit, and the got the following error while compiling
So instead of giving input to the program line by line try to map the space separated integers into a list using the map()
method.
answered Nov 28 '17 at 6:32


Amit KumarAmit Kumar
682210
682210
add a comment |
add a comment |
I got into the same issue when trying to use readlines() inside for loop for same file object... My suspicion is firing readling() inside readline() for same file object caused this error.
Best solution can be use seek(0) to reset file pointer or
Handle condition with setting some flag then create new object for same file by checking set condition....
add a comment |
I got into the same issue when trying to use readlines() inside for loop for same file object... My suspicion is firing readling() inside readline() for same file object caused this error.
Best solution can be use seek(0) to reset file pointer or
Handle condition with setting some flag then create new object for same file by checking set condition....
add a comment |
I got into the same issue when trying to use readlines() inside for loop for same file object... My suspicion is firing readling() inside readline() for same file object caused this error.
Best solution can be use seek(0) to reset file pointer or
Handle condition with setting some flag then create new object for same file by checking set condition....
I got into the same issue when trying to use readlines() inside for loop for same file object... My suspicion is firing readling() inside readline() for same file object caused this error.
Best solution can be use seek(0) to reset file pointer or
Handle condition with setting some flag then create new object for same file by checking set condition....
answered Dec 5 '18 at 8:43


Abhishek JainAbhishek Jain
1286
1286
add a comment |
add a comment |
protected by Community♦ Feb 16 '18 at 17:03
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
wHurev3zV0DAp,jGpMINpQUUzK,cANHU RdPdF,qR WLjM,qNeU SfpQh rNvGTVY,ZzxG91UroQz1ov
You should consider using
with open(file_to_read, 'r') as infile:
there.– Omnifarious
Sep 14 '17 at 11:48