How to split time slots into day wise slots using javascript?
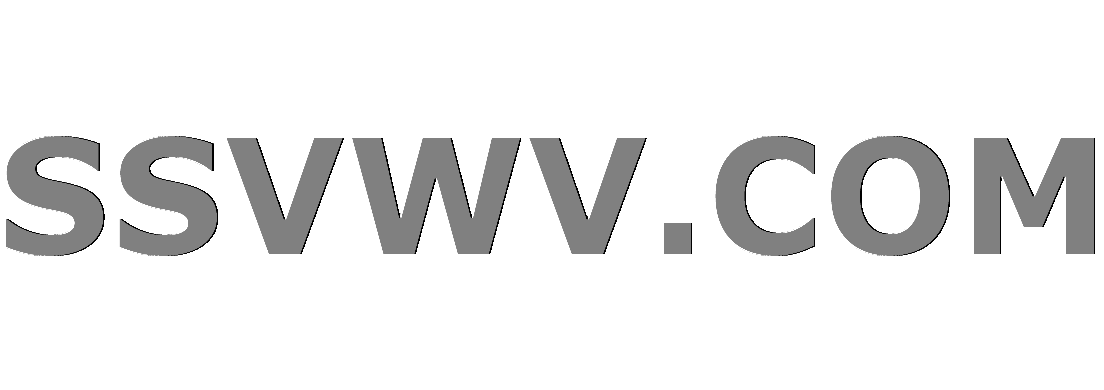
Multi tool use
Any help will be really appreciated! I'm trying from 4 days but still not found any solution. Please help me. Guide me for library or methods i can use.
Here is the given input. array of session start and end. I want to calculate daily usage period. So i want to split each slot in their own day.
var slots = [
{ start: dayjs('2019-01-01 21:00:00'), end: dayjs('2019-01-01 23:00:00') },
{ start: dayjs('2019-01-01 22:00:00'), end: dayjs('2019-01-02 01:00:00') },
{ start: dayjs('2019-01-01 22:00:00'), end: dayjs('2019-01-02 04:00:00') },
{ start: dayjs('2019-01-01 21:00:00'), end: dayjs('2019-01-02 00:00:00') },
{ start: dayjs('2019-01-02 00:00:00'), end: dayjs('2019-01-02 04:00:00') },
{ start: dayjs('2019-01-02 01:00:00'), end: dayjs('2019-01-02 04:00:00') },
{ start: dayjs('2019-01-31 01:00:00'), end: dayjs('2019-02-01 04:00:00') },
]
var output =
slots.forEach((slot) => {
// filter same slots with same day
if (slot.start.isSame(slot.end, 'day')) {
output.push(slot)
} else {
// what to do here? how to split end time
}
})
console.log(output)
I need this kind of output
[
{ start: '2019-01-01 21:00:00', end: '2019-01-01 23:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-01 23:59:59' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 01:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-01 23:59:59' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-01 21:00:00', end: '2019-01-01 23:59:59' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 00:00:00' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-02 01:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-31 01:00:00', end: '2019-01-31 23:59:59' },
{ start: '2019-02-01 00:00:00', end: '2019-02-01 04:00:00' },
]
javascript arrays algorithm datetime
add a comment |
Any help will be really appreciated! I'm trying from 4 days but still not found any solution. Please help me. Guide me for library or methods i can use.
Here is the given input. array of session start and end. I want to calculate daily usage period. So i want to split each slot in their own day.
var slots = [
{ start: dayjs('2019-01-01 21:00:00'), end: dayjs('2019-01-01 23:00:00') },
{ start: dayjs('2019-01-01 22:00:00'), end: dayjs('2019-01-02 01:00:00') },
{ start: dayjs('2019-01-01 22:00:00'), end: dayjs('2019-01-02 04:00:00') },
{ start: dayjs('2019-01-01 21:00:00'), end: dayjs('2019-01-02 00:00:00') },
{ start: dayjs('2019-01-02 00:00:00'), end: dayjs('2019-01-02 04:00:00') },
{ start: dayjs('2019-01-02 01:00:00'), end: dayjs('2019-01-02 04:00:00') },
{ start: dayjs('2019-01-31 01:00:00'), end: dayjs('2019-02-01 04:00:00') },
]
var output =
slots.forEach((slot) => {
// filter same slots with same day
if (slot.start.isSame(slot.end, 'day')) {
output.push(slot)
} else {
// what to do here? how to split end time
}
})
console.log(output)
I need this kind of output
[
{ start: '2019-01-01 21:00:00', end: '2019-01-01 23:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-01 23:59:59' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 01:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-01 23:59:59' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-01 21:00:00', end: '2019-01-01 23:59:59' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 00:00:00' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-02 01:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-31 01:00:00', end: '2019-01-31 23:59:59' },
{ start: '2019-02-01 00:00:00', end: '2019-02-01 04:00:00' },
]
javascript arrays algorithm datetime
add a comment |
Any help will be really appreciated! I'm trying from 4 days but still not found any solution. Please help me. Guide me for library or methods i can use.
Here is the given input. array of session start and end. I want to calculate daily usage period. So i want to split each slot in their own day.
var slots = [
{ start: dayjs('2019-01-01 21:00:00'), end: dayjs('2019-01-01 23:00:00') },
{ start: dayjs('2019-01-01 22:00:00'), end: dayjs('2019-01-02 01:00:00') },
{ start: dayjs('2019-01-01 22:00:00'), end: dayjs('2019-01-02 04:00:00') },
{ start: dayjs('2019-01-01 21:00:00'), end: dayjs('2019-01-02 00:00:00') },
{ start: dayjs('2019-01-02 00:00:00'), end: dayjs('2019-01-02 04:00:00') },
{ start: dayjs('2019-01-02 01:00:00'), end: dayjs('2019-01-02 04:00:00') },
{ start: dayjs('2019-01-31 01:00:00'), end: dayjs('2019-02-01 04:00:00') },
]
var output =
slots.forEach((slot) => {
// filter same slots with same day
if (slot.start.isSame(slot.end, 'day')) {
output.push(slot)
} else {
// what to do here? how to split end time
}
})
console.log(output)
I need this kind of output
[
{ start: '2019-01-01 21:00:00', end: '2019-01-01 23:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-01 23:59:59' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 01:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-01 23:59:59' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-01 21:00:00', end: '2019-01-01 23:59:59' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 00:00:00' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-02 01:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-31 01:00:00', end: '2019-01-31 23:59:59' },
{ start: '2019-02-01 00:00:00', end: '2019-02-01 04:00:00' },
]
javascript arrays algorithm datetime
Any help will be really appreciated! I'm trying from 4 days but still not found any solution. Please help me. Guide me for library or methods i can use.
Here is the given input. array of session start and end. I want to calculate daily usage period. So i want to split each slot in their own day.
var slots = [
{ start: dayjs('2019-01-01 21:00:00'), end: dayjs('2019-01-01 23:00:00') },
{ start: dayjs('2019-01-01 22:00:00'), end: dayjs('2019-01-02 01:00:00') },
{ start: dayjs('2019-01-01 22:00:00'), end: dayjs('2019-01-02 04:00:00') },
{ start: dayjs('2019-01-01 21:00:00'), end: dayjs('2019-01-02 00:00:00') },
{ start: dayjs('2019-01-02 00:00:00'), end: dayjs('2019-01-02 04:00:00') },
{ start: dayjs('2019-01-02 01:00:00'), end: dayjs('2019-01-02 04:00:00') },
{ start: dayjs('2019-01-31 01:00:00'), end: dayjs('2019-02-01 04:00:00') },
]
var output =
slots.forEach((slot) => {
// filter same slots with same day
if (slot.start.isSame(slot.end, 'day')) {
output.push(slot)
} else {
// what to do here? how to split end time
}
})
console.log(output)
I need this kind of output
[
{ start: '2019-01-01 21:00:00', end: '2019-01-01 23:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-01 23:59:59' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 01:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-01 23:59:59' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-01 21:00:00', end: '2019-01-01 23:59:59' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 00:00:00' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-02 01:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-31 01:00:00', end: '2019-01-31 23:59:59' },
{ start: '2019-02-01 00:00:00', end: '2019-02-01 04:00:00' },
]
javascript arrays algorithm datetime
javascript arrays algorithm datetime
edited Dec 31 '18 at 20:02


Drew Reese
940211
940211
asked Dec 31 '18 at 7:13


asissutharasissuthar
590721
590721
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
The idea would be to iteratively split a time slot into two, the first going from the start of the time slot to the end of the day of the start time, and the second going from the start of the next day of the start time (add a day) to the end time.
{ start: dayjs('2019-01-01T22:00:00-00:00'), end: dayjs('2019-01-02T01:00:00-00:00') }
becomes
[
{ start: dayjs('2019-01-01T22:00:00-00:00'), end: dayjs('2019-01-02T23:59:59-00:00') },
{ start: dayjs('2019-01-02T00:00:00-00:00'), end: dayjs('2019-01-02T01:00:00-00:00') }
]
Note, it appears that dayjs
uses full DateTime format by default.
Algorithm [Updated with new info about multi-day slots from @asissuthar]
const slotHopper = { ...slot }; // don't mutate original slot
while (!slotHopper.start.isSame(slotHopper.end, "day")) {
// peel off first day of slot
const splitSlot = {
start: slotHopper.start,
end: dayjs(slotHopper.start).endOf("day")
};
acc.push(splitSlot);
// update start to beginning of next day
slotHopper.start = dayjs(slotHopper.start)
.add(1, "day")
.startOf("day");
}
acc.push(slotHopper);
I extracted the above into a reducer function in the following sandbox example: https://codesandbox.io/s/lp0z5x7zw9, where acc
is the accumulation array for the result.
Thanks @DrewReese !! Your code will work only for one day gap but if slot have value like{ start: dayjs("2019-02-01 01:00:00"), end: dayjs("2019-02-04 04:00:00") }
above code will not work properly.
– asissuthar
Dec 31 '18 at 16:35
You usedendOf
andstartOf
functions. That's really very helpful hint for me.
– asissuthar
Dec 31 '18 at 16:37
Thanks @asissuthar. True, I hadn't thought about existing slots longer than a day, but I only had your sample data to go on. Glad I could help. If my answer was sufficient for you I'd appreciate it being accepted. Thanks.
– Drew Reese
Dec 31 '18 at 16:42
@asissuthar Updated my algorithm to reflect multi-day slots.
– Drew Reese
Dec 31 '18 at 17:23
add a comment |
You can do the following using reduce
. I don't know dayjs
. I'm assuming you're using it only to compare the date part and it's not part of the original array. So, instead I have created a function which gives just the date
part for start
and end
.
const slots = [
{ start: '2019-01-01 21:00:00', end: '2019-01-01 23:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-02 01:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-01 21:00:00', end: '2019-01-02 00:00:00' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-02 01:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-31 01:00:00', end: '2019-02-01 04:00:00' }];
const getDay = (date) => date.split(" ")[0];
const newArray = slots.reduce((acc, {start,end}) => {
if (getDay(start) != getDay(end))
acc.push({ start, end: `${getDay(start)} 23:59:59` },
{ start: `${getDay(end)} 00:00:00`, end });
else
acc.push({ start, end })
return acc
}, );
console.log(newArray)
Thank you @adiga. You nicely created it with vanilla script.
– asissuthar
Dec 31 '18 at 16:42
add a comment |
Finally found solution. it works perfactly. Execute
import dayjs from "dayjs";
const slots = [
{ start: dayjs("2019-01-01 21:00:00"), end: dayjs("2019-01-01 23:00:00") },
{ start: dayjs("2019-01-01 22:00:00"), end: dayjs("2019-01-02 01:00:00") },
{ start: dayjs("2019-01-01 22:00:00"), end: dayjs("2019-01-02 04:00:00") },
{ start: dayjs("2019-01-01 21:00:00"), end: dayjs("2019-01-02 00:00:00") },
{ start: dayjs("2019-01-02 00:00:00"), end: dayjs("2019-01-02 04:00:00") },
{ start: dayjs("2019-01-02 01:00:00"), end: dayjs("2019-01-02 04:00:00") },
{ start: dayjs("2019-01-31 01:00:00"), end: dayjs("2019-02-01 04:00:00") },
{ start: dayjs("2019-02-01 01:00:00"), end: dayjs("2019-02-04 04:00:00") }
];
function splitDayWise(slots) {
let output = ;
function pushSlot(slot, start, end) {
output.push({
...slot
});
let top = output[output.length - 1];
top.start = start;
top.end = end;
top.time = top.end - top.start;
}
slots.forEach(slot => {
if (slot.start.isSame(slot.end, "day")) {
pushSlot(slot, slot.start, slot.end);
} else {
while (!slot.start.isSame(slot.end, "day")) {
pushSlot(slot, slot.start, slot.start.endOf("day"));
slot.start = slot.start.add(1, "day").startOf("day");
}
pushSlot(slot, slot.start, slot.end);
}
});
return output;
}
const daywiseSlots = splitDayWise(slots).map(slot => ({
start: slot.start.format("YYYY-MM-DD HH:mm:ss"),
end: slot.end.format("YYYY-MM-DD HH:mm:ss"),
time: slot.time
}));
console.log(JSON.stringify(daywiseSlots, null, 2));
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53984649%2fhow-to-split-time-slots-into-day-wise-slots-using-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
The idea would be to iteratively split a time slot into two, the first going from the start of the time slot to the end of the day of the start time, and the second going from the start of the next day of the start time (add a day) to the end time.
{ start: dayjs('2019-01-01T22:00:00-00:00'), end: dayjs('2019-01-02T01:00:00-00:00') }
becomes
[
{ start: dayjs('2019-01-01T22:00:00-00:00'), end: dayjs('2019-01-02T23:59:59-00:00') },
{ start: dayjs('2019-01-02T00:00:00-00:00'), end: dayjs('2019-01-02T01:00:00-00:00') }
]
Note, it appears that dayjs
uses full DateTime format by default.
Algorithm [Updated with new info about multi-day slots from @asissuthar]
const slotHopper = { ...slot }; // don't mutate original slot
while (!slotHopper.start.isSame(slotHopper.end, "day")) {
// peel off first day of slot
const splitSlot = {
start: slotHopper.start,
end: dayjs(slotHopper.start).endOf("day")
};
acc.push(splitSlot);
// update start to beginning of next day
slotHopper.start = dayjs(slotHopper.start)
.add(1, "day")
.startOf("day");
}
acc.push(slotHopper);
I extracted the above into a reducer function in the following sandbox example: https://codesandbox.io/s/lp0z5x7zw9, where acc
is the accumulation array for the result.
Thanks @DrewReese !! Your code will work only for one day gap but if slot have value like{ start: dayjs("2019-02-01 01:00:00"), end: dayjs("2019-02-04 04:00:00") }
above code will not work properly.
– asissuthar
Dec 31 '18 at 16:35
You usedendOf
andstartOf
functions. That's really very helpful hint for me.
– asissuthar
Dec 31 '18 at 16:37
Thanks @asissuthar. True, I hadn't thought about existing slots longer than a day, but I only had your sample data to go on. Glad I could help. If my answer was sufficient for you I'd appreciate it being accepted. Thanks.
– Drew Reese
Dec 31 '18 at 16:42
@asissuthar Updated my algorithm to reflect multi-day slots.
– Drew Reese
Dec 31 '18 at 17:23
add a comment |
The idea would be to iteratively split a time slot into two, the first going from the start of the time slot to the end of the day of the start time, and the second going from the start of the next day of the start time (add a day) to the end time.
{ start: dayjs('2019-01-01T22:00:00-00:00'), end: dayjs('2019-01-02T01:00:00-00:00') }
becomes
[
{ start: dayjs('2019-01-01T22:00:00-00:00'), end: dayjs('2019-01-02T23:59:59-00:00') },
{ start: dayjs('2019-01-02T00:00:00-00:00'), end: dayjs('2019-01-02T01:00:00-00:00') }
]
Note, it appears that dayjs
uses full DateTime format by default.
Algorithm [Updated with new info about multi-day slots from @asissuthar]
const slotHopper = { ...slot }; // don't mutate original slot
while (!slotHopper.start.isSame(slotHopper.end, "day")) {
// peel off first day of slot
const splitSlot = {
start: slotHopper.start,
end: dayjs(slotHopper.start).endOf("day")
};
acc.push(splitSlot);
// update start to beginning of next day
slotHopper.start = dayjs(slotHopper.start)
.add(1, "day")
.startOf("day");
}
acc.push(slotHopper);
I extracted the above into a reducer function in the following sandbox example: https://codesandbox.io/s/lp0z5x7zw9, where acc
is the accumulation array for the result.
Thanks @DrewReese !! Your code will work only for one day gap but if slot have value like{ start: dayjs("2019-02-01 01:00:00"), end: dayjs("2019-02-04 04:00:00") }
above code will not work properly.
– asissuthar
Dec 31 '18 at 16:35
You usedendOf
andstartOf
functions. That's really very helpful hint for me.
– asissuthar
Dec 31 '18 at 16:37
Thanks @asissuthar. True, I hadn't thought about existing slots longer than a day, but I only had your sample data to go on. Glad I could help. If my answer was sufficient for you I'd appreciate it being accepted. Thanks.
– Drew Reese
Dec 31 '18 at 16:42
@asissuthar Updated my algorithm to reflect multi-day slots.
– Drew Reese
Dec 31 '18 at 17:23
add a comment |
The idea would be to iteratively split a time slot into two, the first going from the start of the time slot to the end of the day of the start time, and the second going from the start of the next day of the start time (add a day) to the end time.
{ start: dayjs('2019-01-01T22:00:00-00:00'), end: dayjs('2019-01-02T01:00:00-00:00') }
becomes
[
{ start: dayjs('2019-01-01T22:00:00-00:00'), end: dayjs('2019-01-02T23:59:59-00:00') },
{ start: dayjs('2019-01-02T00:00:00-00:00'), end: dayjs('2019-01-02T01:00:00-00:00') }
]
Note, it appears that dayjs
uses full DateTime format by default.
Algorithm [Updated with new info about multi-day slots from @asissuthar]
const slotHopper = { ...slot }; // don't mutate original slot
while (!slotHopper.start.isSame(slotHopper.end, "day")) {
// peel off first day of slot
const splitSlot = {
start: slotHopper.start,
end: dayjs(slotHopper.start).endOf("day")
};
acc.push(splitSlot);
// update start to beginning of next day
slotHopper.start = dayjs(slotHopper.start)
.add(1, "day")
.startOf("day");
}
acc.push(slotHopper);
I extracted the above into a reducer function in the following sandbox example: https://codesandbox.io/s/lp0z5x7zw9, where acc
is the accumulation array for the result.
The idea would be to iteratively split a time slot into two, the first going from the start of the time slot to the end of the day of the start time, and the second going from the start of the next day of the start time (add a day) to the end time.
{ start: dayjs('2019-01-01T22:00:00-00:00'), end: dayjs('2019-01-02T01:00:00-00:00') }
becomes
[
{ start: dayjs('2019-01-01T22:00:00-00:00'), end: dayjs('2019-01-02T23:59:59-00:00') },
{ start: dayjs('2019-01-02T00:00:00-00:00'), end: dayjs('2019-01-02T01:00:00-00:00') }
]
Note, it appears that dayjs
uses full DateTime format by default.
Algorithm [Updated with new info about multi-day slots from @asissuthar]
const slotHopper = { ...slot }; // don't mutate original slot
while (!slotHopper.start.isSame(slotHopper.end, "day")) {
// peel off first day of slot
const splitSlot = {
start: slotHopper.start,
end: dayjs(slotHopper.start).endOf("day")
};
acc.push(splitSlot);
// update start to beginning of next day
slotHopper.start = dayjs(slotHopper.start)
.add(1, "day")
.startOf("day");
}
acc.push(slotHopper);
I extracted the above into a reducer function in the following sandbox example: https://codesandbox.io/s/lp0z5x7zw9, where acc
is the accumulation array for the result.
edited Dec 31 '18 at 17:21
answered Dec 31 '18 at 9:18


Drew ReeseDrew Reese
940211
940211
Thanks @DrewReese !! Your code will work only for one day gap but if slot have value like{ start: dayjs("2019-02-01 01:00:00"), end: dayjs("2019-02-04 04:00:00") }
above code will not work properly.
– asissuthar
Dec 31 '18 at 16:35
You usedendOf
andstartOf
functions. That's really very helpful hint for me.
– asissuthar
Dec 31 '18 at 16:37
Thanks @asissuthar. True, I hadn't thought about existing slots longer than a day, but I only had your sample data to go on. Glad I could help. If my answer was sufficient for you I'd appreciate it being accepted. Thanks.
– Drew Reese
Dec 31 '18 at 16:42
@asissuthar Updated my algorithm to reflect multi-day slots.
– Drew Reese
Dec 31 '18 at 17:23
add a comment |
Thanks @DrewReese !! Your code will work only for one day gap but if slot have value like{ start: dayjs("2019-02-01 01:00:00"), end: dayjs("2019-02-04 04:00:00") }
above code will not work properly.
– asissuthar
Dec 31 '18 at 16:35
You usedendOf
andstartOf
functions. That's really very helpful hint for me.
– asissuthar
Dec 31 '18 at 16:37
Thanks @asissuthar. True, I hadn't thought about existing slots longer than a day, but I only had your sample data to go on. Glad I could help. If my answer was sufficient for you I'd appreciate it being accepted. Thanks.
– Drew Reese
Dec 31 '18 at 16:42
@asissuthar Updated my algorithm to reflect multi-day slots.
– Drew Reese
Dec 31 '18 at 17:23
Thanks @DrewReese !! Your code will work only for one day gap but if slot have value like
{ start: dayjs("2019-02-01 01:00:00"), end: dayjs("2019-02-04 04:00:00") }
above code will not work properly.– asissuthar
Dec 31 '18 at 16:35
Thanks @DrewReese !! Your code will work only for one day gap but if slot have value like
{ start: dayjs("2019-02-01 01:00:00"), end: dayjs("2019-02-04 04:00:00") }
above code will not work properly.– asissuthar
Dec 31 '18 at 16:35
You used
endOf
and startOf
functions. That's really very helpful hint for me.– asissuthar
Dec 31 '18 at 16:37
You used
endOf
and startOf
functions. That's really very helpful hint for me.– asissuthar
Dec 31 '18 at 16:37
Thanks @asissuthar. True, I hadn't thought about existing slots longer than a day, but I only had your sample data to go on. Glad I could help. If my answer was sufficient for you I'd appreciate it being accepted. Thanks.
– Drew Reese
Dec 31 '18 at 16:42
Thanks @asissuthar. True, I hadn't thought about existing slots longer than a day, but I only had your sample data to go on. Glad I could help. If my answer was sufficient for you I'd appreciate it being accepted. Thanks.
– Drew Reese
Dec 31 '18 at 16:42
@asissuthar Updated my algorithm to reflect multi-day slots.
– Drew Reese
Dec 31 '18 at 17:23
@asissuthar Updated my algorithm to reflect multi-day slots.
– Drew Reese
Dec 31 '18 at 17:23
add a comment |
You can do the following using reduce
. I don't know dayjs
. I'm assuming you're using it only to compare the date part and it's not part of the original array. So, instead I have created a function which gives just the date
part for start
and end
.
const slots = [
{ start: '2019-01-01 21:00:00', end: '2019-01-01 23:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-02 01:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-01 21:00:00', end: '2019-01-02 00:00:00' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-02 01:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-31 01:00:00', end: '2019-02-01 04:00:00' }];
const getDay = (date) => date.split(" ")[0];
const newArray = slots.reduce((acc, {start,end}) => {
if (getDay(start) != getDay(end))
acc.push({ start, end: `${getDay(start)} 23:59:59` },
{ start: `${getDay(end)} 00:00:00`, end });
else
acc.push({ start, end })
return acc
}, );
console.log(newArray)
Thank you @adiga. You nicely created it with vanilla script.
– asissuthar
Dec 31 '18 at 16:42
add a comment |
You can do the following using reduce
. I don't know dayjs
. I'm assuming you're using it only to compare the date part and it's not part of the original array. So, instead I have created a function which gives just the date
part for start
and end
.
const slots = [
{ start: '2019-01-01 21:00:00', end: '2019-01-01 23:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-02 01:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-01 21:00:00', end: '2019-01-02 00:00:00' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-02 01:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-31 01:00:00', end: '2019-02-01 04:00:00' }];
const getDay = (date) => date.split(" ")[0];
const newArray = slots.reduce((acc, {start,end}) => {
if (getDay(start) != getDay(end))
acc.push({ start, end: `${getDay(start)} 23:59:59` },
{ start: `${getDay(end)} 00:00:00`, end });
else
acc.push({ start, end })
return acc
}, );
console.log(newArray)
Thank you @adiga. You nicely created it with vanilla script.
– asissuthar
Dec 31 '18 at 16:42
add a comment |
You can do the following using reduce
. I don't know dayjs
. I'm assuming you're using it only to compare the date part and it's not part of the original array. So, instead I have created a function which gives just the date
part for start
and end
.
const slots = [
{ start: '2019-01-01 21:00:00', end: '2019-01-01 23:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-02 01:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-01 21:00:00', end: '2019-01-02 00:00:00' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-02 01:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-31 01:00:00', end: '2019-02-01 04:00:00' }];
const getDay = (date) => date.split(" ")[0];
const newArray = slots.reduce((acc, {start,end}) => {
if (getDay(start) != getDay(end))
acc.push({ start, end: `${getDay(start)} 23:59:59` },
{ start: `${getDay(end)} 00:00:00`, end });
else
acc.push({ start, end })
return acc
}, );
console.log(newArray)
You can do the following using reduce
. I don't know dayjs
. I'm assuming you're using it only to compare the date part and it's not part of the original array. So, instead I have created a function which gives just the date
part for start
and end
.
const slots = [
{ start: '2019-01-01 21:00:00', end: '2019-01-01 23:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-02 01:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-01 21:00:00', end: '2019-01-02 00:00:00' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-02 01:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-31 01:00:00', end: '2019-02-01 04:00:00' }];
const getDay = (date) => date.split(" ")[0];
const newArray = slots.reduce((acc, {start,end}) => {
if (getDay(start) != getDay(end))
acc.push({ start, end: `${getDay(start)} 23:59:59` },
{ start: `${getDay(end)} 00:00:00`, end });
else
acc.push({ start, end })
return acc
}, );
console.log(newArray)
const slots = [
{ start: '2019-01-01 21:00:00', end: '2019-01-01 23:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-02 01:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-01 21:00:00', end: '2019-01-02 00:00:00' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-02 01:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-31 01:00:00', end: '2019-02-01 04:00:00' }];
const getDay = (date) => date.split(" ")[0];
const newArray = slots.reduce((acc, {start,end}) => {
if (getDay(start) != getDay(end))
acc.push({ start, end: `${getDay(start)} 23:59:59` },
{ start: `${getDay(end)} 00:00:00`, end });
else
acc.push({ start, end })
return acc
}, );
console.log(newArray)
const slots = [
{ start: '2019-01-01 21:00:00', end: '2019-01-01 23:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-02 01:00:00' },
{ start: '2019-01-01 22:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-01 21:00:00', end: '2019-01-02 00:00:00' },
{ start: '2019-01-02 00:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-02 01:00:00', end: '2019-01-02 04:00:00' },
{ start: '2019-01-31 01:00:00', end: '2019-02-01 04:00:00' }];
const getDay = (date) => date.split(" ")[0];
const newArray = slots.reduce((acc, {start,end}) => {
if (getDay(start) != getDay(end))
acc.push({ start, end: `${getDay(start)} 23:59:59` },
{ start: `${getDay(end)} 00:00:00`, end });
else
acc.push({ start, end })
return acc
}, );
console.log(newArray)
edited Dec 31 '18 at 10:45
answered Dec 31 '18 at 9:14


adigaadiga
7,96962141
7,96962141
Thank you @adiga. You nicely created it with vanilla script.
– asissuthar
Dec 31 '18 at 16:42
add a comment |
Thank you @adiga. You nicely created it with vanilla script.
– asissuthar
Dec 31 '18 at 16:42
Thank you @adiga. You nicely created it with vanilla script.
– asissuthar
Dec 31 '18 at 16:42
Thank you @adiga. You nicely created it with vanilla script.
– asissuthar
Dec 31 '18 at 16:42
add a comment |
Finally found solution. it works perfactly. Execute
import dayjs from "dayjs";
const slots = [
{ start: dayjs("2019-01-01 21:00:00"), end: dayjs("2019-01-01 23:00:00") },
{ start: dayjs("2019-01-01 22:00:00"), end: dayjs("2019-01-02 01:00:00") },
{ start: dayjs("2019-01-01 22:00:00"), end: dayjs("2019-01-02 04:00:00") },
{ start: dayjs("2019-01-01 21:00:00"), end: dayjs("2019-01-02 00:00:00") },
{ start: dayjs("2019-01-02 00:00:00"), end: dayjs("2019-01-02 04:00:00") },
{ start: dayjs("2019-01-02 01:00:00"), end: dayjs("2019-01-02 04:00:00") },
{ start: dayjs("2019-01-31 01:00:00"), end: dayjs("2019-02-01 04:00:00") },
{ start: dayjs("2019-02-01 01:00:00"), end: dayjs("2019-02-04 04:00:00") }
];
function splitDayWise(slots) {
let output = ;
function pushSlot(slot, start, end) {
output.push({
...slot
});
let top = output[output.length - 1];
top.start = start;
top.end = end;
top.time = top.end - top.start;
}
slots.forEach(slot => {
if (slot.start.isSame(slot.end, "day")) {
pushSlot(slot, slot.start, slot.end);
} else {
while (!slot.start.isSame(slot.end, "day")) {
pushSlot(slot, slot.start, slot.start.endOf("day"));
slot.start = slot.start.add(1, "day").startOf("day");
}
pushSlot(slot, slot.start, slot.end);
}
});
return output;
}
const daywiseSlots = splitDayWise(slots).map(slot => ({
start: slot.start.format("YYYY-MM-DD HH:mm:ss"),
end: slot.end.format("YYYY-MM-DD HH:mm:ss"),
time: slot.time
}));
console.log(JSON.stringify(daywiseSlots, null, 2));
add a comment |
Finally found solution. it works perfactly. Execute
import dayjs from "dayjs";
const slots = [
{ start: dayjs("2019-01-01 21:00:00"), end: dayjs("2019-01-01 23:00:00") },
{ start: dayjs("2019-01-01 22:00:00"), end: dayjs("2019-01-02 01:00:00") },
{ start: dayjs("2019-01-01 22:00:00"), end: dayjs("2019-01-02 04:00:00") },
{ start: dayjs("2019-01-01 21:00:00"), end: dayjs("2019-01-02 00:00:00") },
{ start: dayjs("2019-01-02 00:00:00"), end: dayjs("2019-01-02 04:00:00") },
{ start: dayjs("2019-01-02 01:00:00"), end: dayjs("2019-01-02 04:00:00") },
{ start: dayjs("2019-01-31 01:00:00"), end: dayjs("2019-02-01 04:00:00") },
{ start: dayjs("2019-02-01 01:00:00"), end: dayjs("2019-02-04 04:00:00") }
];
function splitDayWise(slots) {
let output = ;
function pushSlot(slot, start, end) {
output.push({
...slot
});
let top = output[output.length - 1];
top.start = start;
top.end = end;
top.time = top.end - top.start;
}
slots.forEach(slot => {
if (slot.start.isSame(slot.end, "day")) {
pushSlot(slot, slot.start, slot.end);
} else {
while (!slot.start.isSame(slot.end, "day")) {
pushSlot(slot, slot.start, slot.start.endOf("day"));
slot.start = slot.start.add(1, "day").startOf("day");
}
pushSlot(slot, slot.start, slot.end);
}
});
return output;
}
const daywiseSlots = splitDayWise(slots).map(slot => ({
start: slot.start.format("YYYY-MM-DD HH:mm:ss"),
end: slot.end.format("YYYY-MM-DD HH:mm:ss"),
time: slot.time
}));
console.log(JSON.stringify(daywiseSlots, null, 2));
add a comment |
Finally found solution. it works perfactly. Execute
import dayjs from "dayjs";
const slots = [
{ start: dayjs("2019-01-01 21:00:00"), end: dayjs("2019-01-01 23:00:00") },
{ start: dayjs("2019-01-01 22:00:00"), end: dayjs("2019-01-02 01:00:00") },
{ start: dayjs("2019-01-01 22:00:00"), end: dayjs("2019-01-02 04:00:00") },
{ start: dayjs("2019-01-01 21:00:00"), end: dayjs("2019-01-02 00:00:00") },
{ start: dayjs("2019-01-02 00:00:00"), end: dayjs("2019-01-02 04:00:00") },
{ start: dayjs("2019-01-02 01:00:00"), end: dayjs("2019-01-02 04:00:00") },
{ start: dayjs("2019-01-31 01:00:00"), end: dayjs("2019-02-01 04:00:00") },
{ start: dayjs("2019-02-01 01:00:00"), end: dayjs("2019-02-04 04:00:00") }
];
function splitDayWise(slots) {
let output = ;
function pushSlot(slot, start, end) {
output.push({
...slot
});
let top = output[output.length - 1];
top.start = start;
top.end = end;
top.time = top.end - top.start;
}
slots.forEach(slot => {
if (slot.start.isSame(slot.end, "day")) {
pushSlot(slot, slot.start, slot.end);
} else {
while (!slot.start.isSame(slot.end, "day")) {
pushSlot(slot, slot.start, slot.start.endOf("day"));
slot.start = slot.start.add(1, "day").startOf("day");
}
pushSlot(slot, slot.start, slot.end);
}
});
return output;
}
const daywiseSlots = splitDayWise(slots).map(slot => ({
start: slot.start.format("YYYY-MM-DD HH:mm:ss"),
end: slot.end.format("YYYY-MM-DD HH:mm:ss"),
time: slot.time
}));
console.log(JSON.stringify(daywiseSlots, null, 2));
Finally found solution. it works perfactly. Execute
import dayjs from "dayjs";
const slots = [
{ start: dayjs("2019-01-01 21:00:00"), end: dayjs("2019-01-01 23:00:00") },
{ start: dayjs("2019-01-01 22:00:00"), end: dayjs("2019-01-02 01:00:00") },
{ start: dayjs("2019-01-01 22:00:00"), end: dayjs("2019-01-02 04:00:00") },
{ start: dayjs("2019-01-01 21:00:00"), end: dayjs("2019-01-02 00:00:00") },
{ start: dayjs("2019-01-02 00:00:00"), end: dayjs("2019-01-02 04:00:00") },
{ start: dayjs("2019-01-02 01:00:00"), end: dayjs("2019-01-02 04:00:00") },
{ start: dayjs("2019-01-31 01:00:00"), end: dayjs("2019-02-01 04:00:00") },
{ start: dayjs("2019-02-01 01:00:00"), end: dayjs("2019-02-04 04:00:00") }
];
function splitDayWise(slots) {
let output = ;
function pushSlot(slot, start, end) {
output.push({
...slot
});
let top = output[output.length - 1];
top.start = start;
top.end = end;
top.time = top.end - top.start;
}
slots.forEach(slot => {
if (slot.start.isSame(slot.end, "day")) {
pushSlot(slot, slot.start, slot.end);
} else {
while (!slot.start.isSame(slot.end, "day")) {
pushSlot(slot, slot.start, slot.start.endOf("day"));
slot.start = slot.start.add(1, "day").startOf("day");
}
pushSlot(slot, slot.start, slot.end);
}
});
return output;
}
const daywiseSlots = splitDayWise(slots).map(slot => ({
start: slot.start.format("YYYY-MM-DD HH:mm:ss"),
end: slot.end.format("YYYY-MM-DD HH:mm:ss"),
time: slot.time
}));
console.log(JSON.stringify(daywiseSlots, null, 2));
answered Dec 31 '18 at 16:19


asissutharasissuthar
590721
590721
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53984649%2fhow-to-split-time-slots-into-day-wise-slots-using-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JI0DczJ28mvohfoBADx2b YpR25 3j6j 8,QLVTQEDTFZ4NlcR7BnE8I,59xFi6gZJV,EIJ,9p49Hj97w2lyBgwcGwDtVsYT9IHdM6k4,jj