Whats the better way to reference a mongoose schema objectId
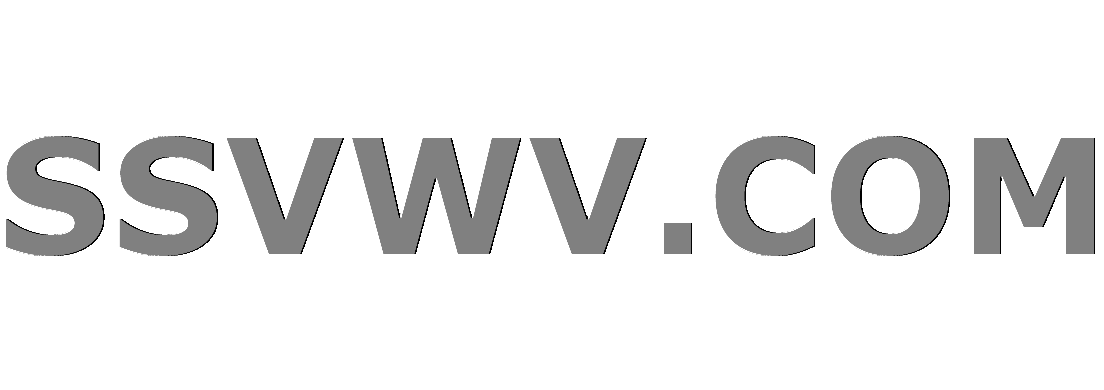
Multi tool use
Assuming that i have the following models:
var author = mongoose.Schema({
name: {
type: String,
required: true
},
isEnabled: {
type: Boolean,
required: true
}
})
var book = mongoose.Schema({
name: {
type: String,
required: true
},
value: {
type: Number,
required: true
}
authorId: {
type: mongoose.Schema.Types.ObjectId,
ref: 'author'
required: true
},
isEnabled: {
type: Boolean,
required: true
}
})
When I try to make a similar aggregate as demonstrated below:
books.aggregate([{$match: { authorId: '599b3b54b8ffff5d1cd323d8'}, { $group: {_id: '$name', total: {$sum: '$value'} } } }])
I did not get any registry due to the fact that mongoose does not transform the authorId into ObjectId, I was able to make it work by turning it into ObjectId before inserting it into the filter, but it did not seem like a good implementation, since I had to give a require in mongoose.
I implemented it differently, changed authorId for String, did it worked very well, my question is if there is a better way to do it?
javascript node.js mongodb mongoose
add a comment |
Assuming that i have the following models:
var author = mongoose.Schema({
name: {
type: String,
required: true
},
isEnabled: {
type: Boolean,
required: true
}
})
var book = mongoose.Schema({
name: {
type: String,
required: true
},
value: {
type: Number,
required: true
}
authorId: {
type: mongoose.Schema.Types.ObjectId,
ref: 'author'
required: true
},
isEnabled: {
type: Boolean,
required: true
}
})
When I try to make a similar aggregate as demonstrated below:
books.aggregate([{$match: { authorId: '599b3b54b8ffff5d1cd323d8'}, { $group: {_id: '$name', total: {$sum: '$value'} } } }])
I did not get any registry due to the fact that mongoose does not transform the authorId into ObjectId, I was able to make it work by turning it into ObjectId before inserting it into the filter, but it did not seem like a good implementation, since I had to give a require in mongoose.
I implemented it differently, changed authorId for String, did it worked very well, my question is if there is a better way to do it?
javascript node.js mongodb mongoose
1
Possible duplicate of stackoverflow.com/questions/36193289/… and more on github.com/Automattic/mongoose/issues/1399
– chridam
Dec 28 '18 at 21:42
add a comment |
Assuming that i have the following models:
var author = mongoose.Schema({
name: {
type: String,
required: true
},
isEnabled: {
type: Boolean,
required: true
}
})
var book = mongoose.Schema({
name: {
type: String,
required: true
},
value: {
type: Number,
required: true
}
authorId: {
type: mongoose.Schema.Types.ObjectId,
ref: 'author'
required: true
},
isEnabled: {
type: Boolean,
required: true
}
})
When I try to make a similar aggregate as demonstrated below:
books.aggregate([{$match: { authorId: '599b3b54b8ffff5d1cd323d8'}, { $group: {_id: '$name', total: {$sum: '$value'} } } }])
I did not get any registry due to the fact that mongoose does not transform the authorId into ObjectId, I was able to make it work by turning it into ObjectId before inserting it into the filter, but it did not seem like a good implementation, since I had to give a require in mongoose.
I implemented it differently, changed authorId for String, did it worked very well, my question is if there is a better way to do it?
javascript node.js mongodb mongoose
Assuming that i have the following models:
var author = mongoose.Schema({
name: {
type: String,
required: true
},
isEnabled: {
type: Boolean,
required: true
}
})
var book = mongoose.Schema({
name: {
type: String,
required: true
},
value: {
type: Number,
required: true
}
authorId: {
type: mongoose.Schema.Types.ObjectId,
ref: 'author'
required: true
},
isEnabled: {
type: Boolean,
required: true
}
})
When I try to make a similar aggregate as demonstrated below:
books.aggregate([{$match: { authorId: '599b3b54b8ffff5d1cd323d8'}, { $group: {_id: '$name', total: {$sum: '$value'} } } }])
I did not get any registry due to the fact that mongoose does not transform the authorId into ObjectId, I was able to make it work by turning it into ObjectId before inserting it into the filter, but it did not seem like a good implementation, since I had to give a require in mongoose.
I implemented it differently, changed authorId for String, did it worked very well, my question is if there is a better way to do it?
javascript node.js mongodb mongoose
javascript node.js mongodb mongoose
asked Dec 28 '18 at 21:25


Vitor DaynnoVitor Daynno
82
82
1
Possible duplicate of stackoverflow.com/questions/36193289/… and more on github.com/Automattic/mongoose/issues/1399
– chridam
Dec 28 '18 at 21:42
add a comment |
1
Possible duplicate of stackoverflow.com/questions/36193289/… and more on github.com/Automattic/mongoose/issues/1399
– chridam
Dec 28 '18 at 21:42
1
1
Possible duplicate of stackoverflow.com/questions/36193289/… and more on github.com/Automattic/mongoose/issues/1399
– chridam
Dec 28 '18 at 21:42
Possible duplicate of stackoverflow.com/questions/36193289/… and more on github.com/Automattic/mongoose/issues/1399
– chridam
Dec 28 '18 at 21:42
add a comment |
1 Answer
1
active
oldest
votes
With mongo4.0 and above you can use $convert to change the data type, or simply $toObjectId
find with string objectId to lookup ObjectId
db.t10.find({$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}})
aggregate
db.t10.aggregate([
{$match : {$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}}}
])
sample collection
> db.t10.find()
{ "_id" : ObjectId("5c269af53f6a8103d9cda006") }
aggregate output
> db.t10.aggregate([{$match : {$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}}}])
{ "_id" : ObjectId("5c269af53f6a8103d9cda006") }
find output
> db.t10.find({$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}})
{ "_id" : ObjectId("5c269af53f6a8103d9cda006") }
>
This solution is very good, although in my case I had to keep as String, because this option is not available in the database, as you yourself specified, as soon as possible I will update the database and implement this solution, thank you very much for your answer.
– Vitor Daynno
Dec 31 '18 at 20:51
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53964442%2fwhats-the-better-way-to-reference-a-mongoose-schema-objectid%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
With mongo4.0 and above you can use $convert to change the data type, or simply $toObjectId
find with string objectId to lookup ObjectId
db.t10.find({$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}})
aggregate
db.t10.aggregate([
{$match : {$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}}}
])
sample collection
> db.t10.find()
{ "_id" : ObjectId("5c269af53f6a8103d9cda006") }
aggregate output
> db.t10.aggregate([{$match : {$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}}}])
{ "_id" : ObjectId("5c269af53f6a8103d9cda006") }
find output
> db.t10.find({$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}})
{ "_id" : ObjectId("5c269af53f6a8103d9cda006") }
>
This solution is very good, although in my case I had to keep as String, because this option is not available in the database, as you yourself specified, as soon as possible I will update the database and implement this solution, thank you very much for your answer.
– Vitor Daynno
Dec 31 '18 at 20:51
add a comment |
With mongo4.0 and above you can use $convert to change the data type, or simply $toObjectId
find with string objectId to lookup ObjectId
db.t10.find({$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}})
aggregate
db.t10.aggregate([
{$match : {$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}}}
])
sample collection
> db.t10.find()
{ "_id" : ObjectId("5c269af53f6a8103d9cda006") }
aggregate output
> db.t10.aggregate([{$match : {$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}}}])
{ "_id" : ObjectId("5c269af53f6a8103d9cda006") }
find output
> db.t10.find({$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}})
{ "_id" : ObjectId("5c269af53f6a8103d9cda006") }
>
This solution is very good, although in my case I had to keep as String, because this option is not available in the database, as you yourself specified, as soon as possible I will update the database and implement this solution, thank you very much for your answer.
– Vitor Daynno
Dec 31 '18 at 20:51
add a comment |
With mongo4.0 and above you can use $convert to change the data type, or simply $toObjectId
find with string objectId to lookup ObjectId
db.t10.find({$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}})
aggregate
db.t10.aggregate([
{$match : {$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}}}
])
sample collection
> db.t10.find()
{ "_id" : ObjectId("5c269af53f6a8103d9cda006") }
aggregate output
> db.t10.aggregate([{$match : {$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}}}])
{ "_id" : ObjectId("5c269af53f6a8103d9cda006") }
find output
> db.t10.find({$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}})
{ "_id" : ObjectId("5c269af53f6a8103d9cda006") }
>
With mongo4.0 and above you can use $convert to change the data type, or simply $toObjectId
find with string objectId to lookup ObjectId
db.t10.find({$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}})
aggregate
db.t10.aggregate([
{$match : {$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}}}
])
sample collection
> db.t10.find()
{ "_id" : ObjectId("5c269af53f6a8103d9cda006") }
aggregate output
> db.t10.aggregate([{$match : {$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}}}])
{ "_id" : ObjectId("5c269af53f6a8103d9cda006") }
find output
> db.t10.find({$expr : {$eq : ["$_id" , {$convert : {input : "5c269af53f6a8103d9cda006", to : "objectId"}}]}})
{ "_id" : ObjectId("5c269af53f6a8103d9cda006") }
>
edited Dec 28 '18 at 22:10
answered Dec 28 '18 at 21:58


SaravanaSaravana
8,22921734
8,22921734
This solution is very good, although in my case I had to keep as String, because this option is not available in the database, as you yourself specified, as soon as possible I will update the database and implement this solution, thank you very much for your answer.
– Vitor Daynno
Dec 31 '18 at 20:51
add a comment |
This solution is very good, although in my case I had to keep as String, because this option is not available in the database, as you yourself specified, as soon as possible I will update the database and implement this solution, thank you very much for your answer.
– Vitor Daynno
Dec 31 '18 at 20:51
This solution is very good, although in my case I had to keep as String, because this option is not available in the database, as you yourself specified, as soon as possible I will update the database and implement this solution, thank you very much for your answer.
– Vitor Daynno
Dec 31 '18 at 20:51
This solution is very good, although in my case I had to keep as String, because this option is not available in the database, as you yourself specified, as soon as possible I will update the database and implement this solution, thank you very much for your answer.
– Vitor Daynno
Dec 31 '18 at 20:51
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53964442%2fwhats-the-better-way-to-reference-a-mongoose-schema-objectid%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
1p yTFoqnaf6qa LTs5O4rZb9seQ
1
Possible duplicate of stackoverflow.com/questions/36193289/… and more on github.com/Automattic/mongoose/issues/1399
– chridam
Dec 28 '18 at 21:42