Blazor onclick event passing in counter from loop
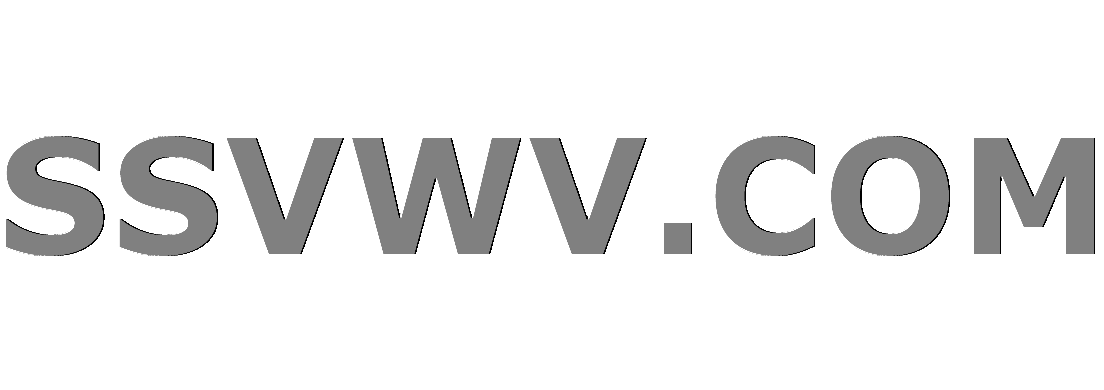
Multi tool use
I'm currently implementing table paging via a home grown solution in Blazor and coming across some difficulty. The troublesome piece of code is below (this is for rendering the paging buttons below a grid):
@for (int i = 0; i < vm.TotalPages; i++)
{
<button id="pg-button-@i" class="btn btn-primary btn-sm" type="button" onclick="@(() => GetTablePage(i))">@i</button>
}
Notice in the onclick event, I am calling a function and passing in i
, the counter for the current interation of the loop.
The GetTablePage
method looks as follows:
protected async Task GetTablePage(int page)
{
Console.WriteLine("page number: " + page);
}
My issue is that EVERY button will call this function with i
set as the length of vm.TotalPages
.
Here is an example to try to make this more clear:
View Markup (notice the id
of each button is set appropriately):
<button id="pg-button-0" class="btn btn-primary btn-sm" type="button">0</button>
<button id="pg-button-1" class="btn btn-primary btn-sm" type="button">1</button>
<button id="pg-button-2" class="btn btn-primary btn-sm" type="button">2</button>
<button id="pg-button-3" class="btn btn-primary btn-sm" type="button">3</button>
<button id="pg-button-4" class="btn btn-primary btn-sm" type="button">4</button>
<button id="pg-button-5" class="btn btn-primary btn-sm" type="button">5</button>
<button id="pg-button-6" class="btn btn-primary btn-sm" type="button">6</button>
Upon clicking ANY of these buttons, my GetTablePage
function is writing 7
to the console which is the length of the vm.TotalPages
collection.
Why is this happening and how can I overcome it?
.net-core single-page-application blazor
add a comment |
I'm currently implementing table paging via a home grown solution in Blazor and coming across some difficulty. The troublesome piece of code is below (this is for rendering the paging buttons below a grid):
@for (int i = 0; i < vm.TotalPages; i++)
{
<button id="pg-button-@i" class="btn btn-primary btn-sm" type="button" onclick="@(() => GetTablePage(i))">@i</button>
}
Notice in the onclick event, I am calling a function and passing in i
, the counter for the current interation of the loop.
The GetTablePage
method looks as follows:
protected async Task GetTablePage(int page)
{
Console.WriteLine("page number: " + page);
}
My issue is that EVERY button will call this function with i
set as the length of vm.TotalPages
.
Here is an example to try to make this more clear:
View Markup (notice the id
of each button is set appropriately):
<button id="pg-button-0" class="btn btn-primary btn-sm" type="button">0</button>
<button id="pg-button-1" class="btn btn-primary btn-sm" type="button">1</button>
<button id="pg-button-2" class="btn btn-primary btn-sm" type="button">2</button>
<button id="pg-button-3" class="btn btn-primary btn-sm" type="button">3</button>
<button id="pg-button-4" class="btn btn-primary btn-sm" type="button">4</button>
<button id="pg-button-5" class="btn btn-primary btn-sm" type="button">5</button>
<button id="pg-button-6" class="btn btn-primary btn-sm" type="button">6</button>
Upon clicking ANY of these buttons, my GetTablePage
function is writing 7
to the console which is the length of the vm.TotalPages
collection.
Why is this happening and how can I overcome it?
.net-core single-page-application blazor
add a comment |
I'm currently implementing table paging via a home grown solution in Blazor and coming across some difficulty. The troublesome piece of code is below (this is for rendering the paging buttons below a grid):
@for (int i = 0; i < vm.TotalPages; i++)
{
<button id="pg-button-@i" class="btn btn-primary btn-sm" type="button" onclick="@(() => GetTablePage(i))">@i</button>
}
Notice in the onclick event, I am calling a function and passing in i
, the counter for the current interation of the loop.
The GetTablePage
method looks as follows:
protected async Task GetTablePage(int page)
{
Console.WriteLine("page number: " + page);
}
My issue is that EVERY button will call this function with i
set as the length of vm.TotalPages
.
Here is an example to try to make this more clear:
View Markup (notice the id
of each button is set appropriately):
<button id="pg-button-0" class="btn btn-primary btn-sm" type="button">0</button>
<button id="pg-button-1" class="btn btn-primary btn-sm" type="button">1</button>
<button id="pg-button-2" class="btn btn-primary btn-sm" type="button">2</button>
<button id="pg-button-3" class="btn btn-primary btn-sm" type="button">3</button>
<button id="pg-button-4" class="btn btn-primary btn-sm" type="button">4</button>
<button id="pg-button-5" class="btn btn-primary btn-sm" type="button">5</button>
<button id="pg-button-6" class="btn btn-primary btn-sm" type="button">6</button>
Upon clicking ANY of these buttons, my GetTablePage
function is writing 7
to the console which is the length of the vm.TotalPages
collection.
Why is this happening and how can I overcome it?
.net-core single-page-application blazor
I'm currently implementing table paging via a home grown solution in Blazor and coming across some difficulty. The troublesome piece of code is below (this is for rendering the paging buttons below a grid):
@for (int i = 0; i < vm.TotalPages; i++)
{
<button id="pg-button-@i" class="btn btn-primary btn-sm" type="button" onclick="@(() => GetTablePage(i))">@i</button>
}
Notice in the onclick event, I am calling a function and passing in i
, the counter for the current interation of the loop.
The GetTablePage
method looks as follows:
protected async Task GetTablePage(int page)
{
Console.WriteLine("page number: " + page);
}
My issue is that EVERY button will call this function with i
set as the length of vm.TotalPages
.
Here is an example to try to make this more clear:
View Markup (notice the id
of each button is set appropriately):
<button id="pg-button-0" class="btn btn-primary btn-sm" type="button">0</button>
<button id="pg-button-1" class="btn btn-primary btn-sm" type="button">1</button>
<button id="pg-button-2" class="btn btn-primary btn-sm" type="button">2</button>
<button id="pg-button-3" class="btn btn-primary btn-sm" type="button">3</button>
<button id="pg-button-4" class="btn btn-primary btn-sm" type="button">4</button>
<button id="pg-button-5" class="btn btn-primary btn-sm" type="button">5</button>
<button id="pg-button-6" class="btn btn-primary btn-sm" type="button">6</button>
Upon clicking ANY of these buttons, my GetTablePage
function is writing 7
to the console which is the length of the vm.TotalPages
collection.
Why is this happening and how can I overcome it?
.net-core single-page-application blazor
.net-core single-page-application blazor
asked Dec 28 '18 at 21:38
GregHGregH
2,3681448
2,3681448
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Because i
is a variable and the for loop is always finished when you click, it is 7 on that moment
You need to do something like:
@for (int i = 0; i < vm.TotalPages; i++)
{
var tempint = i;
<button id="pg-button-@i" class="btn btn-primary btn-sm" type="button" onclick="@(() => GetTablePage(tempint))">@i</button>
}
add a comment |
This is a standard C# behavior where the lambda expression @(() => GetTablePage(i)) has access to a variable and not to the value of the variable, the result of which is that the lambda expression always calls GetTablePage(i) and i equals 7 at the end of the loop. To solve this you can define a variable scoped locally to the loop like the following:
@for (int i = 0; i < vm.TotalPages; i++)
{
var temp = i;
<button id="pg-button-@temp " class="btn btn-primary btn-sm" type="button" onclick="@(() => GetTablePage(temp ))">@temp </button>
}
Hope this helps...
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53964536%2fblazor-onclick-event-passing-in-counter-from-loop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Because i
is a variable and the for loop is always finished when you click, it is 7 on that moment
You need to do something like:
@for (int i = 0; i < vm.TotalPages; i++)
{
var tempint = i;
<button id="pg-button-@i" class="btn btn-primary btn-sm" type="button" onclick="@(() => GetTablePage(tempint))">@i</button>
}
add a comment |
Because i
is a variable and the for loop is always finished when you click, it is 7 on that moment
You need to do something like:
@for (int i = 0; i < vm.TotalPages; i++)
{
var tempint = i;
<button id="pg-button-@i" class="btn btn-primary btn-sm" type="button" onclick="@(() => GetTablePage(tempint))">@i</button>
}
add a comment |
Because i
is a variable and the for loop is always finished when you click, it is 7 on that moment
You need to do something like:
@for (int i = 0; i < vm.TotalPages; i++)
{
var tempint = i;
<button id="pg-button-@i" class="btn btn-primary btn-sm" type="button" onclick="@(() => GetTablePage(tempint))">@i</button>
}
Because i
is a variable and the for loop is always finished when you click, it is 7 on that moment
You need to do something like:
@for (int i = 0; i < vm.TotalPages; i++)
{
var tempint = i;
<button id="pg-button-@i" class="btn btn-primary btn-sm" type="button" onclick="@(() => GetTablePage(tempint))">@i</button>
}
edited Dec 28 '18 at 21:55
answered Dec 28 '18 at 21:49


FloresFlores
3,11422754
3,11422754
add a comment |
add a comment |
This is a standard C# behavior where the lambda expression @(() => GetTablePage(i)) has access to a variable and not to the value of the variable, the result of which is that the lambda expression always calls GetTablePage(i) and i equals 7 at the end of the loop. To solve this you can define a variable scoped locally to the loop like the following:
@for (int i = 0; i < vm.TotalPages; i++)
{
var temp = i;
<button id="pg-button-@temp " class="btn btn-primary btn-sm" type="button" onclick="@(() => GetTablePage(temp ))">@temp </button>
}
Hope this helps...
add a comment |
This is a standard C# behavior where the lambda expression @(() => GetTablePage(i)) has access to a variable and not to the value of the variable, the result of which is that the lambda expression always calls GetTablePage(i) and i equals 7 at the end of the loop. To solve this you can define a variable scoped locally to the loop like the following:
@for (int i = 0; i < vm.TotalPages; i++)
{
var temp = i;
<button id="pg-button-@temp " class="btn btn-primary btn-sm" type="button" onclick="@(() => GetTablePage(temp ))">@temp </button>
}
Hope this helps...
add a comment |
This is a standard C# behavior where the lambda expression @(() => GetTablePage(i)) has access to a variable and not to the value of the variable, the result of which is that the lambda expression always calls GetTablePage(i) and i equals 7 at the end of the loop. To solve this you can define a variable scoped locally to the loop like the following:
@for (int i = 0; i < vm.TotalPages; i++)
{
var temp = i;
<button id="pg-button-@temp " class="btn btn-primary btn-sm" type="button" onclick="@(() => GetTablePage(temp ))">@temp </button>
}
Hope this helps...
This is a standard C# behavior where the lambda expression @(() => GetTablePage(i)) has access to a variable and not to the value of the variable, the result of which is that the lambda expression always calls GetTablePage(i) and i equals 7 at the end of the loop. To solve this you can define a variable scoped locally to the loop like the following:
@for (int i = 0; i < vm.TotalPages; i++)
{
var temp = i;
<button id="pg-button-@temp " class="btn btn-primary btn-sm" type="button" onclick="@(() => GetTablePage(temp ))">@temp </button>
}
Hope this helps...
edited Dec 28 '18 at 23:39
answered Dec 28 '18 at 22:14
IssacIssac
1,1971312
1,1971312
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53964536%2fblazor-onclick-event-passing-in-counter-from-loop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
du5Y 95HaGNciLIbk1yjzj wGXyZJzvU7,JTtUdLuAEztMTGf6OH n