How to get a list of folders inside multiple folders with python
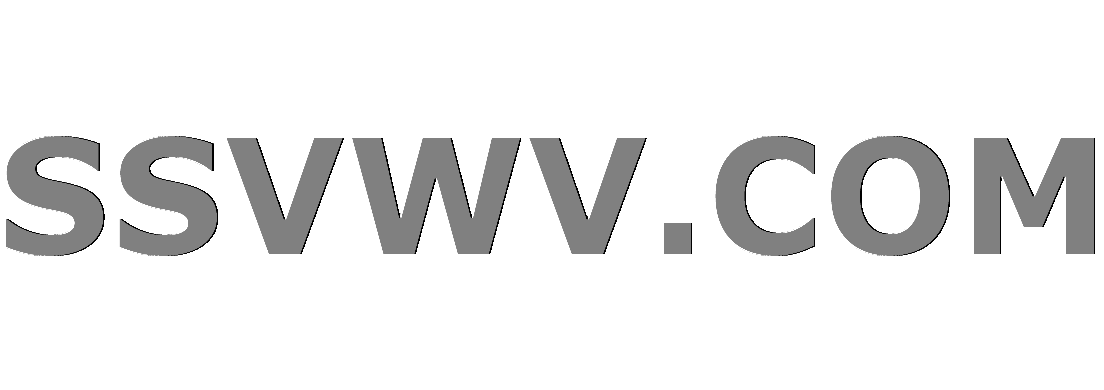
Multi tool use
I've tried a few codes and they all give me the list of my root directory folders or all the files/ folders within a tree. I'm wondering how you get a list of just folders inside folders from your root directory?
Codes I've tried:
This gives me the base list of folders from my root directory.
(next(os.walk(root))[1])
how do I get folders from the next level down? If I try
(next(os.walk(root))[2])
that gives me all the way down to the file.
Thanks!
python
add a comment |
I've tried a few codes and they all give me the list of my root directory folders or all the files/ folders within a tree. I'm wondering how you get a list of just folders inside folders from your root directory?
Codes I've tried:
This gives me the base list of folders from my root directory.
(next(os.walk(root))[1])
how do I get folders from the next level down? If I try
(next(os.walk(root))[2])
that gives me all the way down to the file.
Thanks!
python
You want to recursively traverse the file structure and get a flat list from it? Just to make sure I understand.
– mypetlion
Dec 28 '18 at 21:38
add a comment |
I've tried a few codes and they all give me the list of my root directory folders or all the files/ folders within a tree. I'm wondering how you get a list of just folders inside folders from your root directory?
Codes I've tried:
This gives me the base list of folders from my root directory.
(next(os.walk(root))[1])
how do I get folders from the next level down? If I try
(next(os.walk(root))[2])
that gives me all the way down to the file.
Thanks!
python
I've tried a few codes and they all give me the list of my root directory folders or all the files/ folders within a tree. I'm wondering how you get a list of just folders inside folders from your root directory?
Codes I've tried:
This gives me the base list of folders from my root directory.
(next(os.walk(root))[1])
how do I get folders from the next level down? If I try
(next(os.walk(root))[2])
that gives me all the way down to the file.
Thanks!
python
python
asked Dec 28 '18 at 21:34


Jennifer DuBayJennifer DuBay
1
1
You want to recursively traverse the file structure and get a flat list from it? Just to make sure I understand.
– mypetlion
Dec 28 '18 at 21:38
add a comment |
You want to recursively traverse the file structure and get a flat list from it? Just to make sure I understand.
– mypetlion
Dec 28 '18 at 21:38
You want to recursively traverse the file structure and get a flat list from it? Just to make sure I understand.
– mypetlion
Dec 28 '18 at 21:38
You want to recursively traverse the file structure and get a flat list from it? Just to make sure I understand.
– mypetlion
Dec 28 '18 at 21:38
add a comment |
1 Answer
1
active
oldest
votes
As mentioned in the os.walk() documentation, os.walk()
returns a 3-tuple of (dirpath, dirnames, filenames)
and functions as an iterable. In python, this means that it may be used as the second operand of a for loop i.e. as the iterable in the syntax
for identifier in iterable:
[some operation with "identifer" for each element of "iterable"]
In the case of os.walk()
, we can easily construct a list of all folders with
import os
def get_all_folders(path):
folder_list =
for dirpath, dnames, fnames in os.walk(path):
folder_list += list(dnames)
return folder_list
print(get_all_folders(os.getcwd()))
Or, using the more succinct list comprehension with itertools.chain():
import os
def get_all_folders(path):
return list(chain(*[dir_list for _, dir_list, _ in os.walk(path)]))
print(get_all_folders(os.getcwd()))
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53964505%2fhow-to-get-a-list-of-folders-inside-multiple-folders-with-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
As mentioned in the os.walk() documentation, os.walk()
returns a 3-tuple of (dirpath, dirnames, filenames)
and functions as an iterable. In python, this means that it may be used as the second operand of a for loop i.e. as the iterable in the syntax
for identifier in iterable:
[some operation with "identifer" for each element of "iterable"]
In the case of os.walk()
, we can easily construct a list of all folders with
import os
def get_all_folders(path):
folder_list =
for dirpath, dnames, fnames in os.walk(path):
folder_list += list(dnames)
return folder_list
print(get_all_folders(os.getcwd()))
Or, using the more succinct list comprehension with itertools.chain():
import os
def get_all_folders(path):
return list(chain(*[dir_list for _, dir_list, _ in os.walk(path)]))
print(get_all_folders(os.getcwd()))
add a comment |
As mentioned in the os.walk() documentation, os.walk()
returns a 3-tuple of (dirpath, dirnames, filenames)
and functions as an iterable. In python, this means that it may be used as the second operand of a for loop i.e. as the iterable in the syntax
for identifier in iterable:
[some operation with "identifer" for each element of "iterable"]
In the case of os.walk()
, we can easily construct a list of all folders with
import os
def get_all_folders(path):
folder_list =
for dirpath, dnames, fnames in os.walk(path):
folder_list += list(dnames)
return folder_list
print(get_all_folders(os.getcwd()))
Or, using the more succinct list comprehension with itertools.chain():
import os
def get_all_folders(path):
return list(chain(*[dir_list for _, dir_list, _ in os.walk(path)]))
print(get_all_folders(os.getcwd()))
add a comment |
As mentioned in the os.walk() documentation, os.walk()
returns a 3-tuple of (dirpath, dirnames, filenames)
and functions as an iterable. In python, this means that it may be used as the second operand of a for loop i.e. as the iterable in the syntax
for identifier in iterable:
[some operation with "identifer" for each element of "iterable"]
In the case of os.walk()
, we can easily construct a list of all folders with
import os
def get_all_folders(path):
folder_list =
for dirpath, dnames, fnames in os.walk(path):
folder_list += list(dnames)
return folder_list
print(get_all_folders(os.getcwd()))
Or, using the more succinct list comprehension with itertools.chain():
import os
def get_all_folders(path):
return list(chain(*[dir_list for _, dir_list, _ in os.walk(path)]))
print(get_all_folders(os.getcwd()))
As mentioned in the os.walk() documentation, os.walk()
returns a 3-tuple of (dirpath, dirnames, filenames)
and functions as an iterable. In python, this means that it may be used as the second operand of a for loop i.e. as the iterable in the syntax
for identifier in iterable:
[some operation with "identifer" for each element of "iterable"]
In the case of os.walk()
, we can easily construct a list of all folders with
import os
def get_all_folders(path):
folder_list =
for dirpath, dnames, fnames in os.walk(path):
folder_list += list(dnames)
return folder_list
print(get_all_folders(os.getcwd()))
Or, using the more succinct list comprehension with itertools.chain():
import os
def get_all_folders(path):
return list(chain(*[dir_list for _, dir_list, _ in os.walk(path)]))
print(get_all_folders(os.getcwd()))
edited Dec 28 '18 at 21:48
answered Dec 28 '18 at 21:43
csunday95csunday95
1,109716
1,109716
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53964505%2fhow-to-get-a-list-of-folders-inside-multiple-folders-with-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Xkk59iZOxxos ns,4p,ZuK7ipeUgJBz5KPnD8O0iKxYUQK LJ93dNP 1ZCNQCWYvczDj
You want to recursively traverse the file structure and get a flat list from it? Just to make sure I understand.
– mypetlion
Dec 28 '18 at 21:38