How to fix my Even-Odd Program java code it writes even or odd but also the number
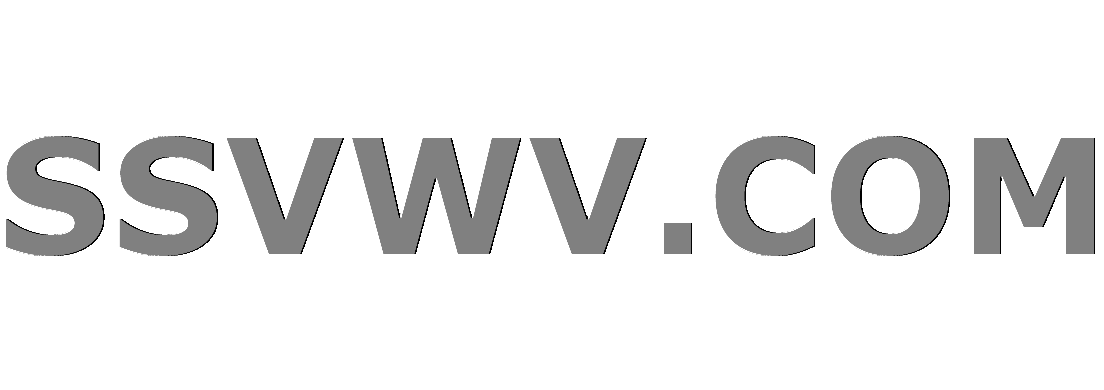
Multi tool use
package practıce1.array;
public class PRACTICE1ARRAY {
public static int evenorodd(int a) {
@author Başar Ballıöz
if (a % 2 == 0) {
System.out.println("Number Is Even");
}
else {
System.out.println("Number Is Odd");
}
return a;
}
public static void main(String args) {
int arr = new int {2,7,9,10,22,31};
for (int i = 0; i < arr.length; i++) {
System.out.println("Element Of Index " + i + " = " + arr[i]);
}
System.out.println(evenorodd(arr[0]));
}
}
Output:
run:
Element Of Index 0 = 2
Element Of Index 1 = 7
Element Of Index 2 = 9
Element Of Index 3 = 10
Element Of Index 4 = 22
Element Of Index 5 = 31
Number Is Even
2
BUILD SUCCESSFUL (total time: 0 seconds)
I dont want to see value of number. I just want to see is number even or odd ?
I think it`s causing because of a mistake which is happened in method
java
add a comment |
package practıce1.array;
public class PRACTICE1ARRAY {
public static int evenorodd(int a) {
@author Başar Ballıöz
if (a % 2 == 0) {
System.out.println("Number Is Even");
}
else {
System.out.println("Number Is Odd");
}
return a;
}
public static void main(String args) {
int arr = new int {2,7,9,10,22,31};
for (int i = 0; i < arr.length; i++) {
System.out.println("Element Of Index " + i + " = " + arr[i]);
}
System.out.println(evenorodd(arr[0]));
}
}
Output:
run:
Element Of Index 0 = 2
Element Of Index 1 = 7
Element Of Index 2 = 9
Element Of Index 3 = 10
Element Of Index 4 = 22
Element Of Index 5 = 31
Number Is Even
2
BUILD SUCCESSFUL (total time: 0 seconds)
I dont want to see value of number. I just want to see is number even or odd ?
I think it`s causing because of a mistake which is happened in method
java
add a comment |
package practıce1.array;
public class PRACTICE1ARRAY {
public static int evenorodd(int a) {
@author Başar Ballıöz
if (a % 2 == 0) {
System.out.println("Number Is Even");
}
else {
System.out.println("Number Is Odd");
}
return a;
}
public static void main(String args) {
int arr = new int {2,7,9,10,22,31};
for (int i = 0; i < arr.length; i++) {
System.out.println("Element Of Index " + i + " = " + arr[i]);
}
System.out.println(evenorodd(arr[0]));
}
}
Output:
run:
Element Of Index 0 = 2
Element Of Index 1 = 7
Element Of Index 2 = 9
Element Of Index 3 = 10
Element Of Index 4 = 22
Element Of Index 5 = 31
Number Is Even
2
BUILD SUCCESSFUL (total time: 0 seconds)
I dont want to see value of number. I just want to see is number even or odd ?
I think it`s causing because of a mistake which is happened in method
java
package practıce1.array;
public class PRACTICE1ARRAY {
public static int evenorodd(int a) {
@author Başar Ballıöz
if (a % 2 == 0) {
System.out.println("Number Is Even");
}
else {
System.out.println("Number Is Odd");
}
return a;
}
public static void main(String args) {
int arr = new int {2,7,9,10,22,31};
for (int i = 0; i < arr.length; i++) {
System.out.println("Element Of Index " + i + " = " + arr[i]);
}
System.out.println(evenorodd(arr[0]));
}
}
Output:
run:
Element Of Index 0 = 2
Element Of Index 1 = 7
Element Of Index 2 = 9
Element Of Index 3 = 10
Element Of Index 4 = 22
Element Of Index 5 = 31
Number Is Even
2
BUILD SUCCESSFUL (total time: 0 seconds)
I dont want to see value of number. I just want to see is number even or odd ?
I think it`s causing because of a mistake which is happened in method
java
java
edited Dec 30 '18 at 19:54
Başar Ballıöz
asked Dec 28 '18 at 21:33
Başar BallıözBaşar Ballıöz
185
185
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Your evenorodd
doesn't need to return the number given to it then, and you don't need to print the value given in. Change your method to this:
public static void evenorodd(int a) {
if (a % 2 == 0) {
System.out.println("Number Is Even");
}
else {
System.out.println("Number Is Odd");
}
}
And when you call it, don't put the method call inside a print statement.
Change this:
System.out.println(evenorodd(arr[0]));
to this:
evenorodd(arr[0])
Thank you so much for helping
– Başar Ballıöz
Dec 28 '18 at 21:43
@BaşarBallıöz Glad I could help! You can accept and/or upvote the answer if you found it helpful.
– mypetlion
Dec 28 '18 at 21:44
But i want to ask what is the difference between writing with system.out and just writing method Why it is also writing value of number when we write our method in System.out ?
– Başar Ballıöz
Dec 28 '18 at 21:44
@BaşarBallıöz The way you had it, the method was returning the value passed in to it. Then the Sop method sent that value that was passed to it to the programs stdout. Since you don't want the value being passed to stdout, we just don't call System.out.println.
– mypetlion
Dec 28 '18 at 21:46
@BaşarBallıöz Returning a passed in argument does not make sense most of the time. The caller knows what he passed to the method. Of course, unless this is done to allow method chaining.
– MC Emperor
Dec 29 '18 at 9:47
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53964499%2fhow-to-fix-my-even-odd-program-java-code-it-writes-even-or-odd-but-also-the-numb%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your evenorodd
doesn't need to return the number given to it then, and you don't need to print the value given in. Change your method to this:
public static void evenorodd(int a) {
if (a % 2 == 0) {
System.out.println("Number Is Even");
}
else {
System.out.println("Number Is Odd");
}
}
And when you call it, don't put the method call inside a print statement.
Change this:
System.out.println(evenorodd(arr[0]));
to this:
evenorodd(arr[0])
Thank you so much for helping
– Başar Ballıöz
Dec 28 '18 at 21:43
@BaşarBallıöz Glad I could help! You can accept and/or upvote the answer if you found it helpful.
– mypetlion
Dec 28 '18 at 21:44
But i want to ask what is the difference between writing with system.out and just writing method Why it is also writing value of number when we write our method in System.out ?
– Başar Ballıöz
Dec 28 '18 at 21:44
@BaşarBallıöz The way you had it, the method was returning the value passed in to it. Then the Sop method sent that value that was passed to it to the programs stdout. Since you don't want the value being passed to stdout, we just don't call System.out.println.
– mypetlion
Dec 28 '18 at 21:46
@BaşarBallıöz Returning a passed in argument does not make sense most of the time. The caller knows what he passed to the method. Of course, unless this is done to allow method chaining.
– MC Emperor
Dec 29 '18 at 9:47
add a comment |
Your evenorodd
doesn't need to return the number given to it then, and you don't need to print the value given in. Change your method to this:
public static void evenorodd(int a) {
if (a % 2 == 0) {
System.out.println("Number Is Even");
}
else {
System.out.println("Number Is Odd");
}
}
And when you call it, don't put the method call inside a print statement.
Change this:
System.out.println(evenorodd(arr[0]));
to this:
evenorodd(arr[0])
Thank you so much for helping
– Başar Ballıöz
Dec 28 '18 at 21:43
@BaşarBallıöz Glad I could help! You can accept and/or upvote the answer if you found it helpful.
– mypetlion
Dec 28 '18 at 21:44
But i want to ask what is the difference between writing with system.out and just writing method Why it is also writing value of number when we write our method in System.out ?
– Başar Ballıöz
Dec 28 '18 at 21:44
@BaşarBallıöz The way you had it, the method was returning the value passed in to it. Then the Sop method sent that value that was passed to it to the programs stdout. Since you don't want the value being passed to stdout, we just don't call System.out.println.
– mypetlion
Dec 28 '18 at 21:46
@BaşarBallıöz Returning a passed in argument does not make sense most of the time. The caller knows what he passed to the method. Of course, unless this is done to allow method chaining.
– MC Emperor
Dec 29 '18 at 9:47
add a comment |
Your evenorodd
doesn't need to return the number given to it then, and you don't need to print the value given in. Change your method to this:
public static void evenorodd(int a) {
if (a % 2 == 0) {
System.out.println("Number Is Even");
}
else {
System.out.println("Number Is Odd");
}
}
And when you call it, don't put the method call inside a print statement.
Change this:
System.out.println(evenorodd(arr[0]));
to this:
evenorodd(arr[0])
Your evenorodd
doesn't need to return the number given to it then, and you don't need to print the value given in. Change your method to this:
public static void evenorodd(int a) {
if (a % 2 == 0) {
System.out.println("Number Is Even");
}
else {
System.out.println("Number Is Odd");
}
}
And when you call it, don't put the method call inside a print statement.
Change this:
System.out.println(evenorodd(arr[0]));
to this:
evenorodd(arr[0])
answered Dec 28 '18 at 21:41


mypetlionmypetlion
1,5661613
1,5661613
Thank you so much for helping
– Başar Ballıöz
Dec 28 '18 at 21:43
@BaşarBallıöz Glad I could help! You can accept and/or upvote the answer if you found it helpful.
– mypetlion
Dec 28 '18 at 21:44
But i want to ask what is the difference between writing with system.out and just writing method Why it is also writing value of number when we write our method in System.out ?
– Başar Ballıöz
Dec 28 '18 at 21:44
@BaşarBallıöz The way you had it, the method was returning the value passed in to it. Then the Sop method sent that value that was passed to it to the programs stdout. Since you don't want the value being passed to stdout, we just don't call System.out.println.
– mypetlion
Dec 28 '18 at 21:46
@BaşarBallıöz Returning a passed in argument does not make sense most of the time. The caller knows what he passed to the method. Of course, unless this is done to allow method chaining.
– MC Emperor
Dec 29 '18 at 9:47
add a comment |
Thank you so much for helping
– Başar Ballıöz
Dec 28 '18 at 21:43
@BaşarBallıöz Glad I could help! You can accept and/or upvote the answer if you found it helpful.
– mypetlion
Dec 28 '18 at 21:44
But i want to ask what is the difference between writing with system.out and just writing method Why it is also writing value of number when we write our method in System.out ?
– Başar Ballıöz
Dec 28 '18 at 21:44
@BaşarBallıöz The way you had it, the method was returning the value passed in to it. Then the Sop method sent that value that was passed to it to the programs stdout. Since you don't want the value being passed to stdout, we just don't call System.out.println.
– mypetlion
Dec 28 '18 at 21:46
@BaşarBallıöz Returning a passed in argument does not make sense most of the time. The caller knows what he passed to the method. Of course, unless this is done to allow method chaining.
– MC Emperor
Dec 29 '18 at 9:47
Thank you so much for helping
– Başar Ballıöz
Dec 28 '18 at 21:43
Thank you so much for helping
– Başar Ballıöz
Dec 28 '18 at 21:43
@BaşarBallıöz Glad I could help! You can accept and/or upvote the answer if you found it helpful.
– mypetlion
Dec 28 '18 at 21:44
@BaşarBallıöz Glad I could help! You can accept and/or upvote the answer if you found it helpful.
– mypetlion
Dec 28 '18 at 21:44
But i want to ask what is the difference between writing with system.out and just writing method Why it is also writing value of number when we write our method in System.out ?
– Başar Ballıöz
Dec 28 '18 at 21:44
But i want to ask what is the difference between writing with system.out and just writing method Why it is also writing value of number when we write our method in System.out ?
– Başar Ballıöz
Dec 28 '18 at 21:44
@BaşarBallıöz The way you had it, the method was returning the value passed in to it. Then the Sop method sent that value that was passed to it to the programs stdout. Since you don't want the value being passed to stdout, we just don't call System.out.println.
– mypetlion
Dec 28 '18 at 21:46
@BaşarBallıöz The way you had it, the method was returning the value passed in to it. Then the Sop method sent that value that was passed to it to the programs stdout. Since you don't want the value being passed to stdout, we just don't call System.out.println.
– mypetlion
Dec 28 '18 at 21:46
@BaşarBallıöz Returning a passed in argument does not make sense most of the time. The caller knows what he passed to the method. Of course, unless this is done to allow method chaining.
– MC Emperor
Dec 29 '18 at 9:47
@BaşarBallıöz Returning a passed in argument does not make sense most of the time. The caller knows what he passed to the method. Of course, unless this is done to allow method chaining.
– MC Emperor
Dec 29 '18 at 9:47
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53964499%2fhow-to-fix-my-even-odd-program-java-code-it-writes-even-or-odd-but-also-the-numb%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LfLF,PhXEkTwnM QCh3BEgp,6Ct4r02 RXckwT 5,bET9gJME D80mNJzIwIEgf3Xt6aK,pPKMr8gqjqDy,wT,K5 mioe o35KC