TableView cells disappearing when tapped / reloadData not changing cells?
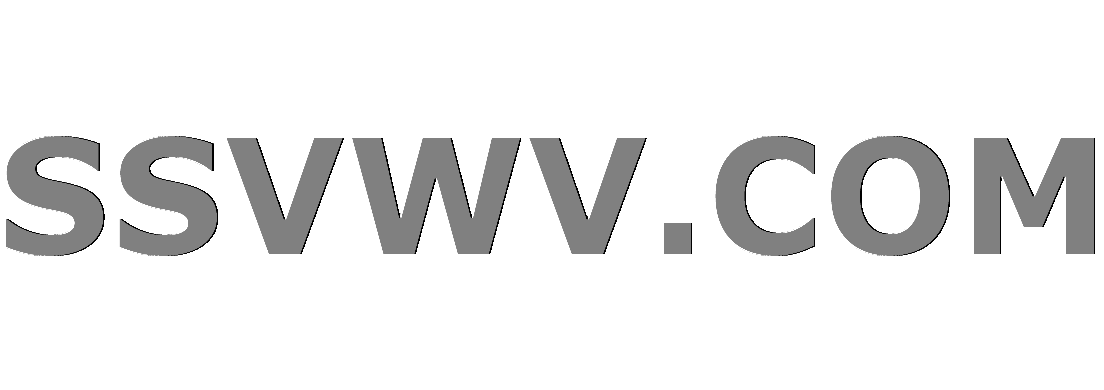
Multi tool use
I am having two problems with my TableView; the first is that whenever a cell is tapped, it disappears. The content view of the cell fades away and leaves a gap between the upper and lower cells. Nothing in my didSelect manipulates the content view.
The second issue is that reloadData doesn't actually update the TableView's data. My TableView is supposed to represent a calendar, and whenever the next or previous day buttons are called (nextDay()
and prevDay()
in the code below), the displayDay
int is changed and tableView.reloadData()
is called. cellForRowAt
relies on displayDay
to determine which day's event should be displayed in the cell, so in theory changing displayDay
changes the content of the cells. However, what happens instead is that whenever the next or previous day buttons are pressed, the content of the cells does not change at all (ie, it still displays the first day's events). The only thing that changes is if the first day has fewer events than the second day, for example if the first day has 3 events and the second day 5, the table view will display the second day's last 2 events at the bottom of the first day's events. My code is below
import UIKit
import Foundation
import Alamofire
import SwiftyJSON
class ScheduleTableViewController: UITableViewController {
var schedule: [DayItem]
var displayDay: Int
var numDays: Int?
@IBOutlet weak var prevDayButton: UIBarButtonItem!
@IBOutlet weak var nextDayButton: UIBarButtonItem!
override func viewDidLoad() {
super.viewDidLoad()
// Uncomment the following line to preserve selection between presentations
// self.clearsSelectionOnViewWillAppear = false
// Uncomment the following line to display an Edit button in the navigation bar for this view controller.
// self.navigationItem.rightBarButtonItem = self.editButtonItem
//schedule = scrapeSchedule()
// let add = UIBarButtonItem(barButtonSystemItem: .add, target: self, action: nil)//action: #selector(addTapped))
// navigationController!.toolbarItems = [add]
let rightSwipe = UISwipeGestureRecognizer(target: self, action: #selector(handleSwipes(_:)))
rightSwipe.direction = .right
view.addGestureRecognizer(rightSwipe)
let leftSwipe = UISwipeGestureRecognizer(target: self, action: #selector(handleSwipes(_:)))
leftSwipe.direction = .left
view.addGestureRecognizer(leftSwipe)
}
required init?(coder aDecoder: NSCoder) {
self.displayDay = 0
self.schedule = [DayItem]()
self.numDays = 0
super.init(coder: aDecoder)
refresh(self)
}
@objc func handleSwipes(_ sender: UISwipeGestureRecognizer) {
if sender.direction == .right {
if prevDayButton.isEnabled {
prevDay(self)
} else {
dismiss(animated: true, completion: nil)
}
} else if sender.direction == .left {
if nextDayButton.isEnabled {
nextDay(self)
}
}
}
func reloadData() {
tableView.reloadData()
if schedule.count != 0 {
self.navigationItem.title = self.schedule[self.displayDay].day
}
}
func configureDayButtons() {
if schedule.count == 0 {
prevDayButton.isEnabled = false
nextDayButton.isEnabled = false
} else {
prevDayButton.isEnabled = true
nextDayButton.isEnabled = true
if displayDay == 0 {
prevDayButton.isEnabled = false
}
if displayDay == numDays! - 1 {
nextDayButton.isEnabled = false
}
}
}
@IBAction func prevDay(_ sender: Any) {
if displayDay != 0 {
displayDay -= 1
configureDayButtons()
reloadData()
}
}
@IBAction func nextDay(_ sender: Any) {
if displayDay != numDays! - 1 {
displayDay += 1
configureDayButtons()
reloadData()
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
override func numberOfSections(in tableView: UITableView) -> Int {
// #warning Incomplete implementation, return the number of sections
return 1
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
// #warning Incomplete implementation, return the number of rows
if schedule.count != 0 {
return schedule[displayDay].events.count
} else {
return 0
}
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let dayItem = schedule[displayDay]
print("display day = (displayDay)")
let eventItem = dayItem.events[indexPath.row]
let identifier = eventItem.identifier
let eventOrTimeText = eventItem.event
let cell = tableView.dequeueReusableCell(withIdentifier: identifier, for: indexPath)
let label = cell.viewWithTag(1000) as! UILabel
label.text = eventOrTimeText
if identifier == "event" || identifier == "eventDDI" {
label.adjustsFontSizeToFitWidth = true
} else if identifier == "time" || identifier == "location" {
label.sizeToFit()
}
return UITableViewCell()
}
override func tableView(_ tableView: UITableView, accessoryButtonTappedForRowWith indexPath: IndexPath) {
self.tableView(self.tableView, didSelectRowAt: indexPath)
}
override func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
tableView.deselectRow(at: indexPath, animated: true)
if schedule[displayDay].events[indexPath.row].information != nil {
let message = schedule[displayDay].events[indexPath.row].information
let alert = UIAlertController(title: schedule[displayDay].events[indexPath.row].event, message: message, preferredStyle: .alert)
let action = UIAlertAction(title: "Close", style: .default, handler: nil)
alert.addAction(action)
present(alert, animated: true, completion: nil)
}
}
@IBAction func close(_ sender: Any) {
dismiss(animated: true, completion: nil)
}
@IBAction func refresh(_ sender: Any) {
scrapeSchedule { schedule in
self.schedule = schedule!
DispatchQueue.main.async {
self.reloadData()
self.numDays = self.schedule.count
self.configureDayButtons()
}
}
}
override func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
// if schedule.
if schedule.count != 0 {
let event = schedule[displayDay].events[indexPath.row]
if event.identifier == "time" && event.event.count > 51 {
return 75
} else if event.identifier == "time" && event.event.count > 31 {
let additionalChars = event.event.count - 31
var quotient: Float = Float(additionalChars) / 20.0
quotient = quotient.rounded(.up)
return CGFloat(quotient * 14 + 40)
}
}
return 34
}
func scrapeSchedule(completion: @escaping ([DayItem]?) -> Void) {
var schedule = [DayItem]()
URLCache.shared.removeAllCachedResponses()
Alamofire.request("my api that functions correctly", method: .get).validate().responseData { response in
switch response.result {
case .success(let data):
do {
let json = try JSON(data: data)
for (index,day):(String, JSON) in json {
schedule.append(self.organizeScheduleJSON(dayJSON: day))
}
completion(schedule)
} catch {
completion(nil)
}
case .failure(let error):
print(error.localizedDescription)
completion(nil)
}
}
}
func organizeScheduleJSON(dayJSON: JSON) -> DayItem {
var events = [EventItem]()
for (index,event):(String, JSON) in dayJSON["eventTimes"] {
if event["information"] != nil {
events.append(EventItem(event: event["event"].string!, identifier: "eventDDI", information: event["information"].string!))
} else {
events.append(EventItem(event: event["event"].string!, identifier: "event"))
}
if event["location"] != nil {
events.append(EventItem(event: event["location"].string!, identifier: "location"))
}
if event["times"] != nil {
var timeArray = event["times"].arrayValue.map({$0.stringValue})
for time in timeArray {
events.append(EventItem(event: time, identifier: "time"))
}
}
}
return DayItem(day: dayJSON["day"].string!, events: events)
}
}
ios swift uitableview uikit
add a comment |
I am having two problems with my TableView; the first is that whenever a cell is tapped, it disappears. The content view of the cell fades away and leaves a gap between the upper and lower cells. Nothing in my didSelect manipulates the content view.
The second issue is that reloadData doesn't actually update the TableView's data. My TableView is supposed to represent a calendar, and whenever the next or previous day buttons are called (nextDay()
and prevDay()
in the code below), the displayDay
int is changed and tableView.reloadData()
is called. cellForRowAt
relies on displayDay
to determine which day's event should be displayed in the cell, so in theory changing displayDay
changes the content of the cells. However, what happens instead is that whenever the next or previous day buttons are pressed, the content of the cells does not change at all (ie, it still displays the first day's events). The only thing that changes is if the first day has fewer events than the second day, for example if the first day has 3 events and the second day 5, the table view will display the second day's last 2 events at the bottom of the first day's events. My code is below
import UIKit
import Foundation
import Alamofire
import SwiftyJSON
class ScheduleTableViewController: UITableViewController {
var schedule: [DayItem]
var displayDay: Int
var numDays: Int?
@IBOutlet weak var prevDayButton: UIBarButtonItem!
@IBOutlet weak var nextDayButton: UIBarButtonItem!
override func viewDidLoad() {
super.viewDidLoad()
// Uncomment the following line to preserve selection between presentations
// self.clearsSelectionOnViewWillAppear = false
// Uncomment the following line to display an Edit button in the navigation bar for this view controller.
// self.navigationItem.rightBarButtonItem = self.editButtonItem
//schedule = scrapeSchedule()
// let add = UIBarButtonItem(barButtonSystemItem: .add, target: self, action: nil)//action: #selector(addTapped))
// navigationController!.toolbarItems = [add]
let rightSwipe = UISwipeGestureRecognizer(target: self, action: #selector(handleSwipes(_:)))
rightSwipe.direction = .right
view.addGestureRecognizer(rightSwipe)
let leftSwipe = UISwipeGestureRecognizer(target: self, action: #selector(handleSwipes(_:)))
leftSwipe.direction = .left
view.addGestureRecognizer(leftSwipe)
}
required init?(coder aDecoder: NSCoder) {
self.displayDay = 0
self.schedule = [DayItem]()
self.numDays = 0
super.init(coder: aDecoder)
refresh(self)
}
@objc func handleSwipes(_ sender: UISwipeGestureRecognizer) {
if sender.direction == .right {
if prevDayButton.isEnabled {
prevDay(self)
} else {
dismiss(animated: true, completion: nil)
}
} else if sender.direction == .left {
if nextDayButton.isEnabled {
nextDay(self)
}
}
}
func reloadData() {
tableView.reloadData()
if schedule.count != 0 {
self.navigationItem.title = self.schedule[self.displayDay].day
}
}
func configureDayButtons() {
if schedule.count == 0 {
prevDayButton.isEnabled = false
nextDayButton.isEnabled = false
} else {
prevDayButton.isEnabled = true
nextDayButton.isEnabled = true
if displayDay == 0 {
prevDayButton.isEnabled = false
}
if displayDay == numDays! - 1 {
nextDayButton.isEnabled = false
}
}
}
@IBAction func prevDay(_ sender: Any) {
if displayDay != 0 {
displayDay -= 1
configureDayButtons()
reloadData()
}
}
@IBAction func nextDay(_ sender: Any) {
if displayDay != numDays! - 1 {
displayDay += 1
configureDayButtons()
reloadData()
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
override func numberOfSections(in tableView: UITableView) -> Int {
// #warning Incomplete implementation, return the number of sections
return 1
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
// #warning Incomplete implementation, return the number of rows
if schedule.count != 0 {
return schedule[displayDay].events.count
} else {
return 0
}
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let dayItem = schedule[displayDay]
print("display day = (displayDay)")
let eventItem = dayItem.events[indexPath.row]
let identifier = eventItem.identifier
let eventOrTimeText = eventItem.event
let cell = tableView.dequeueReusableCell(withIdentifier: identifier, for: indexPath)
let label = cell.viewWithTag(1000) as! UILabel
label.text = eventOrTimeText
if identifier == "event" || identifier == "eventDDI" {
label.adjustsFontSizeToFitWidth = true
} else if identifier == "time" || identifier == "location" {
label.sizeToFit()
}
return UITableViewCell()
}
override func tableView(_ tableView: UITableView, accessoryButtonTappedForRowWith indexPath: IndexPath) {
self.tableView(self.tableView, didSelectRowAt: indexPath)
}
override func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
tableView.deselectRow(at: indexPath, animated: true)
if schedule[displayDay].events[indexPath.row].information != nil {
let message = schedule[displayDay].events[indexPath.row].information
let alert = UIAlertController(title: schedule[displayDay].events[indexPath.row].event, message: message, preferredStyle: .alert)
let action = UIAlertAction(title: "Close", style: .default, handler: nil)
alert.addAction(action)
present(alert, animated: true, completion: nil)
}
}
@IBAction func close(_ sender: Any) {
dismiss(animated: true, completion: nil)
}
@IBAction func refresh(_ sender: Any) {
scrapeSchedule { schedule in
self.schedule = schedule!
DispatchQueue.main.async {
self.reloadData()
self.numDays = self.schedule.count
self.configureDayButtons()
}
}
}
override func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
// if schedule.
if schedule.count != 0 {
let event = schedule[displayDay].events[indexPath.row]
if event.identifier == "time" && event.event.count > 51 {
return 75
} else if event.identifier == "time" && event.event.count > 31 {
let additionalChars = event.event.count - 31
var quotient: Float = Float(additionalChars) / 20.0
quotient = quotient.rounded(.up)
return CGFloat(quotient * 14 + 40)
}
}
return 34
}
func scrapeSchedule(completion: @escaping ([DayItem]?) -> Void) {
var schedule = [DayItem]()
URLCache.shared.removeAllCachedResponses()
Alamofire.request("my api that functions correctly", method: .get).validate().responseData { response in
switch response.result {
case .success(let data):
do {
let json = try JSON(data: data)
for (index,day):(String, JSON) in json {
schedule.append(self.organizeScheduleJSON(dayJSON: day))
}
completion(schedule)
} catch {
completion(nil)
}
case .failure(let error):
print(error.localizedDescription)
completion(nil)
}
}
}
func organizeScheduleJSON(dayJSON: JSON) -> DayItem {
var events = [EventItem]()
for (index,event):(String, JSON) in dayJSON["eventTimes"] {
if event["information"] != nil {
events.append(EventItem(event: event["event"].string!, identifier: "eventDDI", information: event["information"].string!))
} else {
events.append(EventItem(event: event["event"].string!, identifier: "event"))
}
if event["location"] != nil {
events.append(EventItem(event: event["location"].string!, identifier: "location"))
}
if event["times"] != nil {
var timeArray = event["times"].arrayValue.map({$0.stringValue})
for time in timeArray {
events.append(EventItem(event: time, identifier: "time"))
}
}
}
return DayItem(day: dayJSON["day"].string!, events: events)
}
}
ios swift uitableview uikit
1
YourcellForRow(at:)
doesn't look right. It alwaysreturn UITableViewCell()
rather than thecell
you configured.
– Paulw11
Dec 29 '18 at 22:15
That solved it...don't know how I missed that. Thanks!
– Kevin2566
Dec 29 '18 at 22:19
add a comment |
I am having two problems with my TableView; the first is that whenever a cell is tapped, it disappears. The content view of the cell fades away and leaves a gap between the upper and lower cells. Nothing in my didSelect manipulates the content view.
The second issue is that reloadData doesn't actually update the TableView's data. My TableView is supposed to represent a calendar, and whenever the next or previous day buttons are called (nextDay()
and prevDay()
in the code below), the displayDay
int is changed and tableView.reloadData()
is called. cellForRowAt
relies on displayDay
to determine which day's event should be displayed in the cell, so in theory changing displayDay
changes the content of the cells. However, what happens instead is that whenever the next or previous day buttons are pressed, the content of the cells does not change at all (ie, it still displays the first day's events). The only thing that changes is if the first day has fewer events than the second day, for example if the first day has 3 events and the second day 5, the table view will display the second day's last 2 events at the bottom of the first day's events. My code is below
import UIKit
import Foundation
import Alamofire
import SwiftyJSON
class ScheduleTableViewController: UITableViewController {
var schedule: [DayItem]
var displayDay: Int
var numDays: Int?
@IBOutlet weak var prevDayButton: UIBarButtonItem!
@IBOutlet weak var nextDayButton: UIBarButtonItem!
override func viewDidLoad() {
super.viewDidLoad()
// Uncomment the following line to preserve selection between presentations
// self.clearsSelectionOnViewWillAppear = false
// Uncomment the following line to display an Edit button in the navigation bar for this view controller.
// self.navigationItem.rightBarButtonItem = self.editButtonItem
//schedule = scrapeSchedule()
// let add = UIBarButtonItem(barButtonSystemItem: .add, target: self, action: nil)//action: #selector(addTapped))
// navigationController!.toolbarItems = [add]
let rightSwipe = UISwipeGestureRecognizer(target: self, action: #selector(handleSwipes(_:)))
rightSwipe.direction = .right
view.addGestureRecognizer(rightSwipe)
let leftSwipe = UISwipeGestureRecognizer(target: self, action: #selector(handleSwipes(_:)))
leftSwipe.direction = .left
view.addGestureRecognizer(leftSwipe)
}
required init?(coder aDecoder: NSCoder) {
self.displayDay = 0
self.schedule = [DayItem]()
self.numDays = 0
super.init(coder: aDecoder)
refresh(self)
}
@objc func handleSwipes(_ sender: UISwipeGestureRecognizer) {
if sender.direction == .right {
if prevDayButton.isEnabled {
prevDay(self)
} else {
dismiss(animated: true, completion: nil)
}
} else if sender.direction == .left {
if nextDayButton.isEnabled {
nextDay(self)
}
}
}
func reloadData() {
tableView.reloadData()
if schedule.count != 0 {
self.navigationItem.title = self.schedule[self.displayDay].day
}
}
func configureDayButtons() {
if schedule.count == 0 {
prevDayButton.isEnabled = false
nextDayButton.isEnabled = false
} else {
prevDayButton.isEnabled = true
nextDayButton.isEnabled = true
if displayDay == 0 {
prevDayButton.isEnabled = false
}
if displayDay == numDays! - 1 {
nextDayButton.isEnabled = false
}
}
}
@IBAction func prevDay(_ sender: Any) {
if displayDay != 0 {
displayDay -= 1
configureDayButtons()
reloadData()
}
}
@IBAction func nextDay(_ sender: Any) {
if displayDay != numDays! - 1 {
displayDay += 1
configureDayButtons()
reloadData()
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
override func numberOfSections(in tableView: UITableView) -> Int {
// #warning Incomplete implementation, return the number of sections
return 1
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
// #warning Incomplete implementation, return the number of rows
if schedule.count != 0 {
return schedule[displayDay].events.count
} else {
return 0
}
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let dayItem = schedule[displayDay]
print("display day = (displayDay)")
let eventItem = dayItem.events[indexPath.row]
let identifier = eventItem.identifier
let eventOrTimeText = eventItem.event
let cell = tableView.dequeueReusableCell(withIdentifier: identifier, for: indexPath)
let label = cell.viewWithTag(1000) as! UILabel
label.text = eventOrTimeText
if identifier == "event" || identifier == "eventDDI" {
label.adjustsFontSizeToFitWidth = true
} else if identifier == "time" || identifier == "location" {
label.sizeToFit()
}
return UITableViewCell()
}
override func tableView(_ tableView: UITableView, accessoryButtonTappedForRowWith indexPath: IndexPath) {
self.tableView(self.tableView, didSelectRowAt: indexPath)
}
override func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
tableView.deselectRow(at: indexPath, animated: true)
if schedule[displayDay].events[indexPath.row].information != nil {
let message = schedule[displayDay].events[indexPath.row].information
let alert = UIAlertController(title: schedule[displayDay].events[indexPath.row].event, message: message, preferredStyle: .alert)
let action = UIAlertAction(title: "Close", style: .default, handler: nil)
alert.addAction(action)
present(alert, animated: true, completion: nil)
}
}
@IBAction func close(_ sender: Any) {
dismiss(animated: true, completion: nil)
}
@IBAction func refresh(_ sender: Any) {
scrapeSchedule { schedule in
self.schedule = schedule!
DispatchQueue.main.async {
self.reloadData()
self.numDays = self.schedule.count
self.configureDayButtons()
}
}
}
override func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
// if schedule.
if schedule.count != 0 {
let event = schedule[displayDay].events[indexPath.row]
if event.identifier == "time" && event.event.count > 51 {
return 75
} else if event.identifier == "time" && event.event.count > 31 {
let additionalChars = event.event.count - 31
var quotient: Float = Float(additionalChars) / 20.0
quotient = quotient.rounded(.up)
return CGFloat(quotient * 14 + 40)
}
}
return 34
}
func scrapeSchedule(completion: @escaping ([DayItem]?) -> Void) {
var schedule = [DayItem]()
URLCache.shared.removeAllCachedResponses()
Alamofire.request("my api that functions correctly", method: .get).validate().responseData { response in
switch response.result {
case .success(let data):
do {
let json = try JSON(data: data)
for (index,day):(String, JSON) in json {
schedule.append(self.organizeScheduleJSON(dayJSON: day))
}
completion(schedule)
} catch {
completion(nil)
}
case .failure(let error):
print(error.localizedDescription)
completion(nil)
}
}
}
func organizeScheduleJSON(dayJSON: JSON) -> DayItem {
var events = [EventItem]()
for (index,event):(String, JSON) in dayJSON["eventTimes"] {
if event["information"] != nil {
events.append(EventItem(event: event["event"].string!, identifier: "eventDDI", information: event["information"].string!))
} else {
events.append(EventItem(event: event["event"].string!, identifier: "event"))
}
if event["location"] != nil {
events.append(EventItem(event: event["location"].string!, identifier: "location"))
}
if event["times"] != nil {
var timeArray = event["times"].arrayValue.map({$0.stringValue})
for time in timeArray {
events.append(EventItem(event: time, identifier: "time"))
}
}
}
return DayItem(day: dayJSON["day"].string!, events: events)
}
}
ios swift uitableview uikit
I am having two problems with my TableView; the first is that whenever a cell is tapped, it disappears. The content view of the cell fades away and leaves a gap between the upper and lower cells. Nothing in my didSelect manipulates the content view.
The second issue is that reloadData doesn't actually update the TableView's data. My TableView is supposed to represent a calendar, and whenever the next or previous day buttons are called (nextDay()
and prevDay()
in the code below), the displayDay
int is changed and tableView.reloadData()
is called. cellForRowAt
relies on displayDay
to determine which day's event should be displayed in the cell, so in theory changing displayDay
changes the content of the cells. However, what happens instead is that whenever the next or previous day buttons are pressed, the content of the cells does not change at all (ie, it still displays the first day's events). The only thing that changes is if the first day has fewer events than the second day, for example if the first day has 3 events and the second day 5, the table view will display the second day's last 2 events at the bottom of the first day's events. My code is below
import UIKit
import Foundation
import Alamofire
import SwiftyJSON
class ScheduleTableViewController: UITableViewController {
var schedule: [DayItem]
var displayDay: Int
var numDays: Int?
@IBOutlet weak var prevDayButton: UIBarButtonItem!
@IBOutlet weak var nextDayButton: UIBarButtonItem!
override func viewDidLoad() {
super.viewDidLoad()
// Uncomment the following line to preserve selection between presentations
// self.clearsSelectionOnViewWillAppear = false
// Uncomment the following line to display an Edit button in the navigation bar for this view controller.
// self.navigationItem.rightBarButtonItem = self.editButtonItem
//schedule = scrapeSchedule()
// let add = UIBarButtonItem(barButtonSystemItem: .add, target: self, action: nil)//action: #selector(addTapped))
// navigationController!.toolbarItems = [add]
let rightSwipe = UISwipeGestureRecognizer(target: self, action: #selector(handleSwipes(_:)))
rightSwipe.direction = .right
view.addGestureRecognizer(rightSwipe)
let leftSwipe = UISwipeGestureRecognizer(target: self, action: #selector(handleSwipes(_:)))
leftSwipe.direction = .left
view.addGestureRecognizer(leftSwipe)
}
required init?(coder aDecoder: NSCoder) {
self.displayDay = 0
self.schedule = [DayItem]()
self.numDays = 0
super.init(coder: aDecoder)
refresh(self)
}
@objc func handleSwipes(_ sender: UISwipeGestureRecognizer) {
if sender.direction == .right {
if prevDayButton.isEnabled {
prevDay(self)
} else {
dismiss(animated: true, completion: nil)
}
} else if sender.direction == .left {
if nextDayButton.isEnabled {
nextDay(self)
}
}
}
func reloadData() {
tableView.reloadData()
if schedule.count != 0 {
self.navigationItem.title = self.schedule[self.displayDay].day
}
}
func configureDayButtons() {
if schedule.count == 0 {
prevDayButton.isEnabled = false
nextDayButton.isEnabled = false
} else {
prevDayButton.isEnabled = true
nextDayButton.isEnabled = true
if displayDay == 0 {
prevDayButton.isEnabled = false
}
if displayDay == numDays! - 1 {
nextDayButton.isEnabled = false
}
}
}
@IBAction func prevDay(_ sender: Any) {
if displayDay != 0 {
displayDay -= 1
configureDayButtons()
reloadData()
}
}
@IBAction func nextDay(_ sender: Any) {
if displayDay != numDays! - 1 {
displayDay += 1
configureDayButtons()
reloadData()
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
override func numberOfSections(in tableView: UITableView) -> Int {
// #warning Incomplete implementation, return the number of sections
return 1
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
// #warning Incomplete implementation, return the number of rows
if schedule.count != 0 {
return schedule[displayDay].events.count
} else {
return 0
}
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let dayItem = schedule[displayDay]
print("display day = (displayDay)")
let eventItem = dayItem.events[indexPath.row]
let identifier = eventItem.identifier
let eventOrTimeText = eventItem.event
let cell = tableView.dequeueReusableCell(withIdentifier: identifier, for: indexPath)
let label = cell.viewWithTag(1000) as! UILabel
label.text = eventOrTimeText
if identifier == "event" || identifier == "eventDDI" {
label.adjustsFontSizeToFitWidth = true
} else if identifier == "time" || identifier == "location" {
label.sizeToFit()
}
return UITableViewCell()
}
override func tableView(_ tableView: UITableView, accessoryButtonTappedForRowWith indexPath: IndexPath) {
self.tableView(self.tableView, didSelectRowAt: indexPath)
}
override func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
tableView.deselectRow(at: indexPath, animated: true)
if schedule[displayDay].events[indexPath.row].information != nil {
let message = schedule[displayDay].events[indexPath.row].information
let alert = UIAlertController(title: schedule[displayDay].events[indexPath.row].event, message: message, preferredStyle: .alert)
let action = UIAlertAction(title: "Close", style: .default, handler: nil)
alert.addAction(action)
present(alert, animated: true, completion: nil)
}
}
@IBAction func close(_ sender: Any) {
dismiss(animated: true, completion: nil)
}
@IBAction func refresh(_ sender: Any) {
scrapeSchedule { schedule in
self.schedule = schedule!
DispatchQueue.main.async {
self.reloadData()
self.numDays = self.schedule.count
self.configureDayButtons()
}
}
}
override func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
// if schedule.
if schedule.count != 0 {
let event = schedule[displayDay].events[indexPath.row]
if event.identifier == "time" && event.event.count > 51 {
return 75
} else if event.identifier == "time" && event.event.count > 31 {
let additionalChars = event.event.count - 31
var quotient: Float = Float(additionalChars) / 20.0
quotient = quotient.rounded(.up)
return CGFloat(quotient * 14 + 40)
}
}
return 34
}
func scrapeSchedule(completion: @escaping ([DayItem]?) -> Void) {
var schedule = [DayItem]()
URLCache.shared.removeAllCachedResponses()
Alamofire.request("my api that functions correctly", method: .get).validate().responseData { response in
switch response.result {
case .success(let data):
do {
let json = try JSON(data: data)
for (index,day):(String, JSON) in json {
schedule.append(self.organizeScheduleJSON(dayJSON: day))
}
completion(schedule)
} catch {
completion(nil)
}
case .failure(let error):
print(error.localizedDescription)
completion(nil)
}
}
}
func organizeScheduleJSON(dayJSON: JSON) -> DayItem {
var events = [EventItem]()
for (index,event):(String, JSON) in dayJSON["eventTimes"] {
if event["information"] != nil {
events.append(EventItem(event: event["event"].string!, identifier: "eventDDI", information: event["information"].string!))
} else {
events.append(EventItem(event: event["event"].string!, identifier: "event"))
}
if event["location"] != nil {
events.append(EventItem(event: event["location"].string!, identifier: "location"))
}
if event["times"] != nil {
var timeArray = event["times"].arrayValue.map({$0.stringValue})
for time in timeArray {
events.append(EventItem(event: time, identifier: "time"))
}
}
}
return DayItem(day: dayJSON["day"].string!, events: events)
}
}
ios swift uitableview uikit
ios swift uitableview uikit
edited Dec 29 '18 at 22:12


Paulw11
68k1084102
68k1084102
asked Dec 29 '18 at 22:08
Kevin2566Kevin2566
146
146
1
YourcellForRow(at:)
doesn't look right. It alwaysreturn UITableViewCell()
rather than thecell
you configured.
– Paulw11
Dec 29 '18 at 22:15
That solved it...don't know how I missed that. Thanks!
– Kevin2566
Dec 29 '18 at 22:19
add a comment |
1
YourcellForRow(at:)
doesn't look right. It alwaysreturn UITableViewCell()
rather than thecell
you configured.
– Paulw11
Dec 29 '18 at 22:15
That solved it...don't know how I missed that. Thanks!
– Kevin2566
Dec 29 '18 at 22:19
1
1
Your
cellForRow(at:)
doesn't look right. It always return UITableViewCell()
rather than the cell
you configured.– Paulw11
Dec 29 '18 at 22:15
Your
cellForRow(at:)
doesn't look right. It always return UITableViewCell()
rather than the cell
you configured.– Paulw11
Dec 29 '18 at 22:15
That solved it...don't know how I missed that. Thanks!
– Kevin2566
Dec 29 '18 at 22:19
That solved it...don't know how I missed that. Thanks!
– Kevin2566
Dec 29 '18 at 22:19
add a comment |
1 Answer
1
active
oldest
votes
Your cellForRow(at:)
is always returning an empty UITableViewCell
rather than your configured cell
object.
Replace return UITableViewCell()
with return cell
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53973712%2ftableview-cells-disappearing-when-tapped-reloaddata-not-changing-cells%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your cellForRow(at:)
is always returning an empty UITableViewCell
rather than your configured cell
object.
Replace return UITableViewCell()
with return cell
add a comment |
Your cellForRow(at:)
is always returning an empty UITableViewCell
rather than your configured cell
object.
Replace return UITableViewCell()
with return cell
add a comment |
Your cellForRow(at:)
is always returning an empty UITableViewCell
rather than your configured cell
object.
Replace return UITableViewCell()
with return cell
Your cellForRow(at:)
is always returning an empty UITableViewCell
rather than your configured cell
object.
Replace return UITableViewCell()
with return cell
answered Dec 29 '18 at 22:22


Paulw11Paulw11
68k1084102
68k1084102
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53973712%2ftableview-cells-disappearing-when-tapped-reloaddata-not-changing-cells%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
FscelIUIroCmEASvQzn687P9I0pHU0tsR,Nnlr
1
Your
cellForRow(at:)
doesn't look right. It alwaysreturn UITableViewCell()
rather than thecell
you configured.– Paulw11
Dec 29 '18 at 22:15
That solved it...don't know how I missed that. Thanks!
– Kevin2566
Dec 29 '18 at 22:19