How to prevent earlier list values from getting deleted in the edited yaml file?
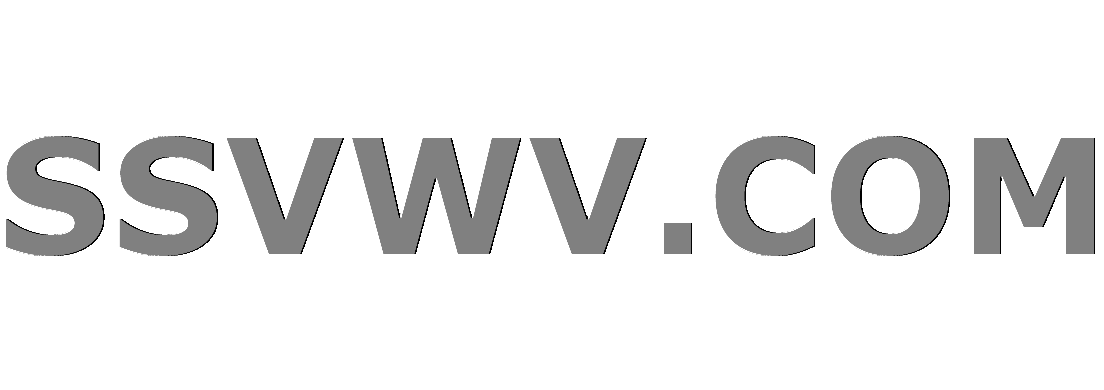
Multi tool use
This is my YAML file (data.yaml
):
- Department: "IT"
name: "abcd"
Education: "Bachlore of Engineering"
And I want to edit it as follows:
- Department: "IT"
name: "abcd"
Education: "Bachlore of Engineering"
- Department: "Production"
name: "xyz"
Education: "Bachlore of Engineering"
- Department: "Management"
name: "ab"
Education: "MBA"
This is my code (currently adding only second list):
from pathlib import Path
from ruamel.yaml import YAML
from ruamel.yaml.scalarstring import SingleQuotedScalarString, DoubleQuotedScalarString
datapath= Path('C:/Users/Master 1TB/source/repos/check2/check2/data.yaml')
with YAML(output=datapath) as yaml:
yaml.indent(sequence=4, offset=2)
code = yaml.load(datapath)
code = [{
"Department": "Production"
"name": "xyz"
"Education": "Bachlore of Engineering"
}]
yaml.dump(code)
Now the problem when code dump the new list in the data.yaml
the earlier list gets deleted, hence my output is:
- Department: "Production"
name: "xyz"
Education: "Bachlore of Engineering"
Instead I want the previous item also in the output and as you explained in the link (How to read a component in YAML file so that I can edit it's key value using ruamel.yaml? ), I have to append the new list value, but this is possible only if I have one list value.
What can be done in this scenario?
Also I am going to add more list values in data.yaml
(preserving all the earlier list in the same YAML file).
ruamel.yaml
add a comment |
This is my YAML file (data.yaml
):
- Department: "IT"
name: "abcd"
Education: "Bachlore of Engineering"
And I want to edit it as follows:
- Department: "IT"
name: "abcd"
Education: "Bachlore of Engineering"
- Department: "Production"
name: "xyz"
Education: "Bachlore of Engineering"
- Department: "Management"
name: "ab"
Education: "MBA"
This is my code (currently adding only second list):
from pathlib import Path
from ruamel.yaml import YAML
from ruamel.yaml.scalarstring import SingleQuotedScalarString, DoubleQuotedScalarString
datapath= Path('C:/Users/Master 1TB/source/repos/check2/check2/data.yaml')
with YAML(output=datapath) as yaml:
yaml.indent(sequence=4, offset=2)
code = yaml.load(datapath)
code = [{
"Department": "Production"
"name": "xyz"
"Education": "Bachlore of Engineering"
}]
yaml.dump(code)
Now the problem when code dump the new list in the data.yaml
the earlier list gets deleted, hence my output is:
- Department: "Production"
name: "xyz"
Education: "Bachlore of Engineering"
Instead I want the previous item also in the output and as you explained in the link (How to read a component in YAML file so that I can edit it's key value using ruamel.yaml? ), I have to append the new list value, but this is possible only if I have one list value.
What can be done in this scenario?
Also I am going to add more list values in data.yaml
(preserving all the earlier list in the same YAML file).
ruamel.yaml
add a comment |
This is my YAML file (data.yaml
):
- Department: "IT"
name: "abcd"
Education: "Bachlore of Engineering"
And I want to edit it as follows:
- Department: "IT"
name: "abcd"
Education: "Bachlore of Engineering"
- Department: "Production"
name: "xyz"
Education: "Bachlore of Engineering"
- Department: "Management"
name: "ab"
Education: "MBA"
This is my code (currently adding only second list):
from pathlib import Path
from ruamel.yaml import YAML
from ruamel.yaml.scalarstring import SingleQuotedScalarString, DoubleQuotedScalarString
datapath= Path('C:/Users/Master 1TB/source/repos/check2/check2/data.yaml')
with YAML(output=datapath) as yaml:
yaml.indent(sequence=4, offset=2)
code = yaml.load(datapath)
code = [{
"Department": "Production"
"name": "xyz"
"Education": "Bachlore of Engineering"
}]
yaml.dump(code)
Now the problem when code dump the new list in the data.yaml
the earlier list gets deleted, hence my output is:
- Department: "Production"
name: "xyz"
Education: "Bachlore of Engineering"
Instead I want the previous item also in the output and as you explained in the link (How to read a component in YAML file so that I can edit it's key value using ruamel.yaml? ), I have to append the new list value, but this is possible only if I have one list value.
What can be done in this scenario?
Also I am going to add more list values in data.yaml
(preserving all the earlier list in the same YAML file).
ruamel.yaml
This is my YAML file (data.yaml
):
- Department: "IT"
name: "abcd"
Education: "Bachlore of Engineering"
And I want to edit it as follows:
- Department: "IT"
name: "abcd"
Education: "Bachlore of Engineering"
- Department: "Production"
name: "xyz"
Education: "Bachlore of Engineering"
- Department: "Management"
name: "ab"
Education: "MBA"
This is my code (currently adding only second list):
from pathlib import Path
from ruamel.yaml import YAML
from ruamel.yaml.scalarstring import SingleQuotedScalarString, DoubleQuotedScalarString
datapath= Path('C:/Users/Master 1TB/source/repos/check2/check2/data.yaml')
with YAML(output=datapath) as yaml:
yaml.indent(sequence=4, offset=2)
code = yaml.load(datapath)
code = [{
"Department": "Production"
"name": "xyz"
"Education": "Bachlore of Engineering"
}]
yaml.dump(code)
Now the problem when code dump the new list in the data.yaml
the earlier list gets deleted, hence my output is:
- Department: "Production"
name: "xyz"
Education: "Bachlore of Engineering"
Instead I want the previous item also in the output and as you explained in the link (How to read a component in YAML file so that I can edit it's key value using ruamel.yaml? ), I have to append the new list value, but this is possible only if I have one list value.
What can be done in this scenario?
Also I am going to add more list values in data.yaml
(preserving all the earlier list in the same YAML file).
ruamel.yaml
ruamel.yaml
edited Dec 29 '18 at 22:11
Anthon
29.4k1693145
29.4k1693145
asked Dec 29 '18 at 21:09


MEHUL SOLANKIMEHUL SOLANKI
174
174
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You have a sequence at the root level of your data.yaml
file, and when you load that you
get a list in your variable code. After that you assigne to code
and what you need to do
is append
an item to that list, or extend
that list with a list of one or more items.
The other thing you cannot really do is have the same file for reading
and writing when you use the output
parameter to the with statement. Either write to a different file
or load from the file then dump the updated structure to the same file.
You should also make sure you have commas between the key-value pairs, as it is now your code will not run.
import sys
from pathlib import Path
from ruamel.yaml import YAML
from ruamel.yaml.scalarstring import SingleQuotedScalarString, DoubleQuotedScalarString
datapath = Path('data.yaml')
yaml = YAML()
code = yaml.load(datapath)
code.extend([{
"Department": "Production",
"name": "xyz",
"Education": "Bachlore of Engineering",
}])
yaml.dump(code, datapath)
print(datapath.read_text())
which gives:
- Department: IT
name: abcd
Education: Bachlore of Engineering
- Department: Production
name: xyz
Education: Bachlore of Engineering
Thanks Anthon!!
– MEHUL SOLANKI
Dec 30 '18 at 20:17
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53973357%2fhow-to-prevent-earlier-list-values-from-getting-deleted-in-the-edited-yaml-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You have a sequence at the root level of your data.yaml
file, and when you load that you
get a list in your variable code. After that you assigne to code
and what you need to do
is append
an item to that list, or extend
that list with a list of one or more items.
The other thing you cannot really do is have the same file for reading
and writing when you use the output
parameter to the with statement. Either write to a different file
or load from the file then dump the updated structure to the same file.
You should also make sure you have commas between the key-value pairs, as it is now your code will not run.
import sys
from pathlib import Path
from ruamel.yaml import YAML
from ruamel.yaml.scalarstring import SingleQuotedScalarString, DoubleQuotedScalarString
datapath = Path('data.yaml')
yaml = YAML()
code = yaml.load(datapath)
code.extend([{
"Department": "Production",
"name": "xyz",
"Education": "Bachlore of Engineering",
}])
yaml.dump(code, datapath)
print(datapath.read_text())
which gives:
- Department: IT
name: abcd
Education: Bachlore of Engineering
- Department: Production
name: xyz
Education: Bachlore of Engineering
Thanks Anthon!!
– MEHUL SOLANKI
Dec 30 '18 at 20:17
add a comment |
You have a sequence at the root level of your data.yaml
file, and when you load that you
get a list in your variable code. After that you assigne to code
and what you need to do
is append
an item to that list, or extend
that list with a list of one or more items.
The other thing you cannot really do is have the same file for reading
and writing when you use the output
parameter to the with statement. Either write to a different file
or load from the file then dump the updated structure to the same file.
You should also make sure you have commas between the key-value pairs, as it is now your code will not run.
import sys
from pathlib import Path
from ruamel.yaml import YAML
from ruamel.yaml.scalarstring import SingleQuotedScalarString, DoubleQuotedScalarString
datapath = Path('data.yaml')
yaml = YAML()
code = yaml.load(datapath)
code.extend([{
"Department": "Production",
"name": "xyz",
"Education": "Bachlore of Engineering",
}])
yaml.dump(code, datapath)
print(datapath.read_text())
which gives:
- Department: IT
name: abcd
Education: Bachlore of Engineering
- Department: Production
name: xyz
Education: Bachlore of Engineering
Thanks Anthon!!
– MEHUL SOLANKI
Dec 30 '18 at 20:17
add a comment |
You have a sequence at the root level of your data.yaml
file, and when you load that you
get a list in your variable code. After that you assigne to code
and what you need to do
is append
an item to that list, or extend
that list with a list of one or more items.
The other thing you cannot really do is have the same file for reading
and writing when you use the output
parameter to the with statement. Either write to a different file
or load from the file then dump the updated structure to the same file.
You should also make sure you have commas between the key-value pairs, as it is now your code will not run.
import sys
from pathlib import Path
from ruamel.yaml import YAML
from ruamel.yaml.scalarstring import SingleQuotedScalarString, DoubleQuotedScalarString
datapath = Path('data.yaml')
yaml = YAML()
code = yaml.load(datapath)
code.extend([{
"Department": "Production",
"name": "xyz",
"Education": "Bachlore of Engineering",
}])
yaml.dump(code, datapath)
print(datapath.read_text())
which gives:
- Department: IT
name: abcd
Education: Bachlore of Engineering
- Department: Production
name: xyz
Education: Bachlore of Engineering
You have a sequence at the root level of your data.yaml
file, and when you load that you
get a list in your variable code. After that you assigne to code
and what you need to do
is append
an item to that list, or extend
that list with a list of one or more items.
The other thing you cannot really do is have the same file for reading
and writing when you use the output
parameter to the with statement. Either write to a different file
or load from the file then dump the updated structure to the same file.
You should also make sure you have commas between the key-value pairs, as it is now your code will not run.
import sys
from pathlib import Path
from ruamel.yaml import YAML
from ruamel.yaml.scalarstring import SingleQuotedScalarString, DoubleQuotedScalarString
datapath = Path('data.yaml')
yaml = YAML()
code = yaml.load(datapath)
code.extend([{
"Department": "Production",
"name": "xyz",
"Education": "Bachlore of Engineering",
}])
yaml.dump(code, datapath)
print(datapath.read_text())
which gives:
- Department: IT
name: abcd
Education: Bachlore of Engineering
- Department: Production
name: xyz
Education: Bachlore of Engineering
answered Dec 29 '18 at 22:10
AnthonAnthon
29.4k1693145
29.4k1693145
Thanks Anthon!!
– MEHUL SOLANKI
Dec 30 '18 at 20:17
add a comment |
Thanks Anthon!!
– MEHUL SOLANKI
Dec 30 '18 at 20:17
Thanks Anthon!!
– MEHUL SOLANKI
Dec 30 '18 at 20:17
Thanks Anthon!!
– MEHUL SOLANKI
Dec 30 '18 at 20:17
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53973357%2fhow-to-prevent-earlier-list-values-from-getting-deleted-in-the-edited-yaml-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
dI6PsZ7,XYN53M5FEiuO,x X,H,Y5U18sQ,twWm,wKXM