Compiler does not recognize button
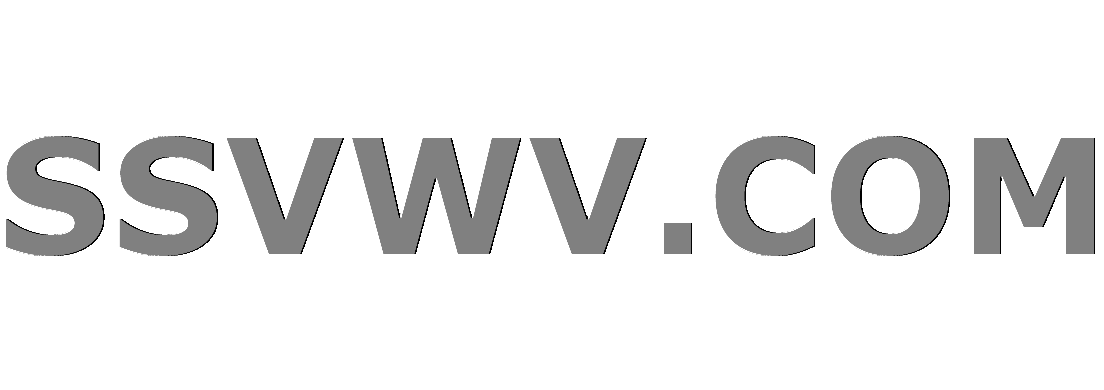
Multi tool use
So I was attempting to create my first app after a long break, and found that something basic has slipped my knowledge, and for whatever reason, even the tutorials for it did not help. I created a button, but wanted it to be able to do things when clicked, so tried adding the code to MainActivity
, though it caused error messages when I tried, wouldn't recognized "Button", "View", or "button_id", and I'm pretty much stumped. Below, I will include the activity_main.xml
code, as well as the .java's code for reference.
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="48dp"
android:text="@string/button"
app:layout_constraintBottom_toTopOf="@+id/textView"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
</android.support.constraint.ConstraintLayout>
Now for the .java file.
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import com.google.android.gms.ads.MobileAds;
import com.google.android.gms.ads.InterstitialAd;
import com.google.android.gms.ads.AdRequest;
public class MainActivity extends AppCompatActivity {
private InterstitialAd mInterstitialAd;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
MobileAds.initialize(this, "ca-app-pub-3940256099942544~3347511713");
mInterstitialAd = new InterstitialAd(this);
mInterstitialAd.setAdUnitId("ca-app-pub-3940256099942544/1033173712");
mInterstitialAd.loadAd(new AdRequest.Builder().build());
Button mButton = (Button) findViewById(R.id.button);
final Button button = findViewById(R.id.button_id);
button.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// Code here executes on main thread after user presses button
}
});
}
}
java

add a comment |
So I was attempting to create my first app after a long break, and found that something basic has slipped my knowledge, and for whatever reason, even the tutorials for it did not help. I created a button, but wanted it to be able to do things when clicked, so tried adding the code to MainActivity
, though it caused error messages when I tried, wouldn't recognized "Button", "View", or "button_id", and I'm pretty much stumped. Below, I will include the activity_main.xml
code, as well as the .java's code for reference.
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="48dp"
android:text="@string/button"
app:layout_constraintBottom_toTopOf="@+id/textView"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
</android.support.constraint.ConstraintLayout>
Now for the .java file.
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import com.google.android.gms.ads.MobileAds;
import com.google.android.gms.ads.InterstitialAd;
import com.google.android.gms.ads.AdRequest;
public class MainActivity extends AppCompatActivity {
private InterstitialAd mInterstitialAd;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
MobileAds.initialize(this, "ca-app-pub-3940256099942544~3347511713");
mInterstitialAd = new InterstitialAd(this);
mInterstitialAd.setAdUnitId("ca-app-pub-3940256099942544/1033173712");
mInterstitialAd.loadAd(new AdRequest.Builder().build());
Button mButton = (Button) findViewById(R.id.button);
final Button button = findViewById(R.id.button_id);
button.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// Code here executes on main thread after user presses button
}
});
}
}
java

add a comment |
So I was attempting to create my first app after a long break, and found that something basic has slipped my knowledge, and for whatever reason, even the tutorials for it did not help. I created a button, but wanted it to be able to do things when clicked, so tried adding the code to MainActivity
, though it caused error messages when I tried, wouldn't recognized "Button", "View", or "button_id", and I'm pretty much stumped. Below, I will include the activity_main.xml
code, as well as the .java's code for reference.
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="48dp"
android:text="@string/button"
app:layout_constraintBottom_toTopOf="@+id/textView"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
</android.support.constraint.ConstraintLayout>
Now for the .java file.
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import com.google.android.gms.ads.MobileAds;
import com.google.android.gms.ads.InterstitialAd;
import com.google.android.gms.ads.AdRequest;
public class MainActivity extends AppCompatActivity {
private InterstitialAd mInterstitialAd;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
MobileAds.initialize(this, "ca-app-pub-3940256099942544~3347511713");
mInterstitialAd = new InterstitialAd(this);
mInterstitialAd.setAdUnitId("ca-app-pub-3940256099942544/1033173712");
mInterstitialAd.loadAd(new AdRequest.Builder().build());
Button mButton = (Button) findViewById(R.id.button);
final Button button = findViewById(R.id.button_id);
button.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// Code here executes on main thread after user presses button
}
});
}
}
java

So I was attempting to create my first app after a long break, and found that something basic has slipped my knowledge, and for whatever reason, even the tutorials for it did not help. I created a button, but wanted it to be able to do things when clicked, so tried adding the code to MainActivity
, though it caused error messages when I tried, wouldn't recognized "Button", "View", or "button_id", and I'm pretty much stumped. Below, I will include the activity_main.xml
code, as well as the .java's code for reference.
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="48dp"
android:text="@string/button"
app:layout_constraintBottom_toTopOf="@+id/textView"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
</android.support.constraint.ConstraintLayout>
Now for the .java file.
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import com.google.android.gms.ads.MobileAds;
import com.google.android.gms.ads.InterstitialAd;
import com.google.android.gms.ads.AdRequest;
public class MainActivity extends AppCompatActivity {
private InterstitialAd mInterstitialAd;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
MobileAds.initialize(this, "ca-app-pub-3940256099942544~3347511713");
mInterstitialAd = new InterstitialAd(this);
mInterstitialAd.setAdUnitId("ca-app-pub-3940256099942544/1033173712");
mInterstitialAd.loadAd(new AdRequest.Builder().build());
Button mButton = (Button) findViewById(R.id.button);
final Button button = findViewById(R.id.button_id);
button.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// Code here executes on main thread after user presses button
}
});
}
}
java

java

edited Dec 30 '18 at 5:10


Andrew Thompson
153k27163339
153k27163339
asked Dec 29 '18 at 21:12
Josh DuvallJosh Duvall
62
62
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
The Button
and View
classes need to be imported; e.g.
import android.widget.Button;
import android.view.View;
Your class is in the default package, so all classes that it uses (apart from those in the java.lang
package) need to be imported.
The reference to button_id
is probably a mistake.
Button mButton = (Button) findViewById(R.id.button);
final Button button = findViewById(R.id.button_id); // Delete this line
Alternatively, maybe you need to declare another button in your "activity_main.xml" file whose id is android:id="@+id/button_id"
Thanks a lot, after following your advice, I was able to get it working! :)
– Josh Duvall
Dec 31 '18 at 10:55
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53973374%2fcompiler-does-not-recognize-button%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The Button
and View
classes need to be imported; e.g.
import android.widget.Button;
import android.view.View;
Your class is in the default package, so all classes that it uses (apart from those in the java.lang
package) need to be imported.
The reference to button_id
is probably a mistake.
Button mButton = (Button) findViewById(R.id.button);
final Button button = findViewById(R.id.button_id); // Delete this line
Alternatively, maybe you need to declare another button in your "activity_main.xml" file whose id is android:id="@+id/button_id"
Thanks a lot, after following your advice, I was able to get it working! :)
– Josh Duvall
Dec 31 '18 at 10:55
add a comment |
The Button
and View
classes need to be imported; e.g.
import android.widget.Button;
import android.view.View;
Your class is in the default package, so all classes that it uses (apart from those in the java.lang
package) need to be imported.
The reference to button_id
is probably a mistake.
Button mButton = (Button) findViewById(R.id.button);
final Button button = findViewById(R.id.button_id); // Delete this line
Alternatively, maybe you need to declare another button in your "activity_main.xml" file whose id is android:id="@+id/button_id"
Thanks a lot, after following your advice, I was able to get it working! :)
– Josh Duvall
Dec 31 '18 at 10:55
add a comment |
The Button
and View
classes need to be imported; e.g.
import android.widget.Button;
import android.view.View;
Your class is in the default package, so all classes that it uses (apart from those in the java.lang
package) need to be imported.
The reference to button_id
is probably a mistake.
Button mButton = (Button) findViewById(R.id.button);
final Button button = findViewById(R.id.button_id); // Delete this line
Alternatively, maybe you need to declare another button in your "activity_main.xml" file whose id is android:id="@+id/button_id"
The Button
and View
classes need to be imported; e.g.
import android.widget.Button;
import android.view.View;
Your class is in the default package, so all classes that it uses (apart from those in the java.lang
package) need to be imported.
The reference to button_id
is probably a mistake.
Button mButton = (Button) findViewById(R.id.button);
final Button button = findViewById(R.id.button_id); // Delete this line
Alternatively, maybe you need to declare another button in your "activity_main.xml" file whose id is android:id="@+id/button_id"
answered Dec 30 '18 at 5:20
community wiki
Stephen C
Thanks a lot, after following your advice, I was able to get it working! :)
– Josh Duvall
Dec 31 '18 at 10:55
add a comment |
Thanks a lot, after following your advice, I was able to get it working! :)
– Josh Duvall
Dec 31 '18 at 10:55
Thanks a lot, after following your advice, I was able to get it working! :)
– Josh Duvall
Dec 31 '18 at 10:55
Thanks a lot, after following your advice, I was able to get it working! :)
– Josh Duvall
Dec 31 '18 at 10:55
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53973374%2fcompiler-does-not-recognize-button%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
b60qQycAy