Array Problems java.lang.ArrayIndexOutOfBoundsException: 9
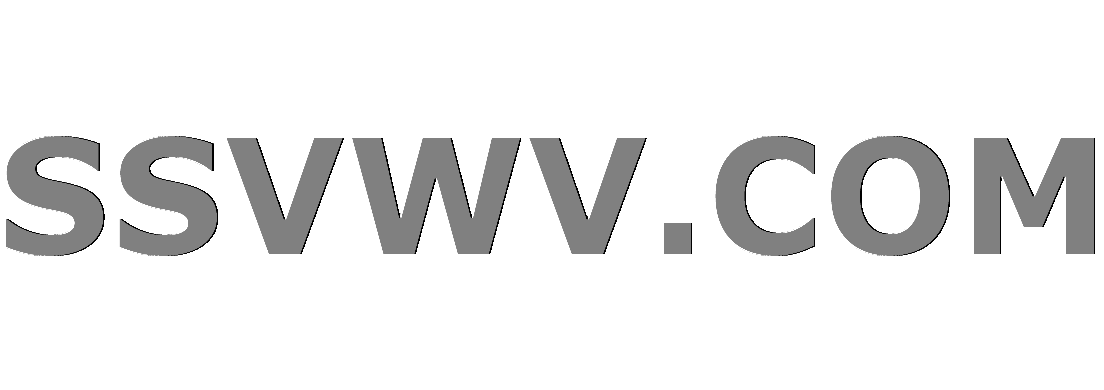
Multi tool use
I am trying to write a class that takes a phone number that the user inputs as a string, then stores each digit in an array. I converted the string to a long using Long.parseLong. Here is the function that is supposed to store each individual digit in an array.
public void storetoarray()
//Turn the strings to longs
//A loop chops of the last digit and stores in an array
{
phonelong = Long.parseLong(initialphone);
phonetemp1 = phonelong;
for (int i = phonelength; i>=0; i--)
{
phonearray[i-1] = phonetemp1%10;
phonetemp2 = phonetemp1 - phonetemp1%10;
phonetemp1 = phonetemp2/10;
System.out.print("Phone temp 2" + phonetemp2 + phonetemp1);
}
}
The logic of that loop is to find the last digit of the phone number using a %10, then subtract that number from the original phone number. That new difference is then set to be phonetemp1/10.
Example 4158884532%10 = 2. That is stored in phonearray[9]. Then phonetemp2 is subtracted giving us 4158884530. Divide that by 10 and you get 415888453. Should be ready to store the next digit.
What is going wrong? Thanks for the help!
java arrays indexoutofboundsexception
add a comment |
I am trying to write a class that takes a phone number that the user inputs as a string, then stores each digit in an array. I converted the string to a long using Long.parseLong. Here is the function that is supposed to store each individual digit in an array.
public void storetoarray()
//Turn the strings to longs
//A loop chops of the last digit and stores in an array
{
phonelong = Long.parseLong(initialphone);
phonetemp1 = phonelong;
for (int i = phonelength; i>=0; i--)
{
phonearray[i-1] = phonetemp1%10;
phonetemp2 = phonetemp1 - phonetemp1%10;
phonetemp1 = phonetemp2/10;
System.out.print("Phone temp 2" + phonetemp2 + phonetemp1);
}
}
The logic of that loop is to find the last digit of the phone number using a %10, then subtract that number from the original phone number. That new difference is then set to be phonetemp1/10.
Example 4158884532%10 = 2. That is stored in phonearray[9]. Then phonetemp2 is subtracted giving us 4158884530. Divide that by 10 and you get 415888453. Should be ready to store the next digit.
What is going wrong? Thanks for the help!
java arrays indexoutofboundsexception
1
Why bother converting to long and then trying to extract digits? Just use substring().
– mclaassen
Jun 1 '13 at 0:40
When do you create phoneArray? Also, why so many phone variables? Mclaassen is right. In any case, you're being North American Numbering Plan-centric. And, where does the input come from in here?
– Eric Jablow
Jun 1 '13 at 0:44
Phone numbers should be treated as strings and not numbers.
– Don Roby
Jun 1 '13 at 0:50
Show these declarations -phonelength
,initialphone
,phonearray
. You are only giving us partial information.
– xagyg
Jun 1 '13 at 2:05
add a comment |
I am trying to write a class that takes a phone number that the user inputs as a string, then stores each digit in an array. I converted the string to a long using Long.parseLong. Here is the function that is supposed to store each individual digit in an array.
public void storetoarray()
//Turn the strings to longs
//A loop chops of the last digit and stores in an array
{
phonelong = Long.parseLong(initialphone);
phonetemp1 = phonelong;
for (int i = phonelength; i>=0; i--)
{
phonearray[i-1] = phonetemp1%10;
phonetemp2 = phonetemp1 - phonetemp1%10;
phonetemp1 = phonetemp2/10;
System.out.print("Phone temp 2" + phonetemp2 + phonetemp1);
}
}
The logic of that loop is to find the last digit of the phone number using a %10, then subtract that number from the original phone number. That new difference is then set to be phonetemp1/10.
Example 4158884532%10 = 2. That is stored in phonearray[9]. Then phonetemp2 is subtracted giving us 4158884530. Divide that by 10 and you get 415888453. Should be ready to store the next digit.
What is going wrong? Thanks for the help!
java arrays indexoutofboundsexception
I am trying to write a class that takes a phone number that the user inputs as a string, then stores each digit in an array. I converted the string to a long using Long.parseLong. Here is the function that is supposed to store each individual digit in an array.
public void storetoarray()
//Turn the strings to longs
//A loop chops of the last digit and stores in an array
{
phonelong = Long.parseLong(initialphone);
phonetemp1 = phonelong;
for (int i = phonelength; i>=0; i--)
{
phonearray[i-1] = phonetemp1%10;
phonetemp2 = phonetemp1 - phonetemp1%10;
phonetemp1 = phonetemp2/10;
System.out.print("Phone temp 2" + phonetemp2 + phonetemp1);
}
}
The logic of that loop is to find the last digit of the phone number using a %10, then subtract that number from the original phone number. That new difference is then set to be phonetemp1/10.
Example 4158884532%10 = 2. That is stored in phonearray[9]. Then phonetemp2 is subtracted giving us 4158884530. Divide that by 10 and you get 415888453. Should be ready to store the next digit.
What is going wrong? Thanks for the help!
java arrays indexoutofboundsexception
java arrays indexoutofboundsexception
edited Oct 30 '18 at 2:01
Dang Nguyen
805522
805522
asked Jun 1 '13 at 0:36
MaxMax
155414
155414
1
Why bother converting to long and then trying to extract digits? Just use substring().
– mclaassen
Jun 1 '13 at 0:40
When do you create phoneArray? Also, why so many phone variables? Mclaassen is right. In any case, you're being North American Numbering Plan-centric. And, where does the input come from in here?
– Eric Jablow
Jun 1 '13 at 0:44
Phone numbers should be treated as strings and not numbers.
– Don Roby
Jun 1 '13 at 0:50
Show these declarations -phonelength
,initialphone
,phonearray
. You are only giving us partial information.
– xagyg
Jun 1 '13 at 2:05
add a comment |
1
Why bother converting to long and then trying to extract digits? Just use substring().
– mclaassen
Jun 1 '13 at 0:40
When do you create phoneArray? Also, why so many phone variables? Mclaassen is right. In any case, you're being North American Numbering Plan-centric. And, where does the input come from in here?
– Eric Jablow
Jun 1 '13 at 0:44
Phone numbers should be treated as strings and not numbers.
– Don Roby
Jun 1 '13 at 0:50
Show these declarations -phonelength
,initialphone
,phonearray
. You are only giving us partial information.
– xagyg
Jun 1 '13 at 2:05
1
1
Why bother converting to long and then trying to extract digits? Just use substring().
– mclaassen
Jun 1 '13 at 0:40
Why bother converting to long and then trying to extract digits? Just use substring().
– mclaassen
Jun 1 '13 at 0:40
When do you create phoneArray? Also, why so many phone variables? Mclaassen is right. In any case, you're being North American Numbering Plan-centric. And, where does the input come from in here?
– Eric Jablow
Jun 1 '13 at 0:44
When do you create phoneArray? Also, why so many phone variables? Mclaassen is right. In any case, you're being North American Numbering Plan-centric. And, where does the input come from in here?
– Eric Jablow
Jun 1 '13 at 0:44
Phone numbers should be treated as strings and not numbers.
– Don Roby
Jun 1 '13 at 0:50
Phone numbers should be treated as strings and not numbers.
– Don Roby
Jun 1 '13 at 0:50
Show these declarations -
phonelength
, initialphone
, phonearray
. You are only giving us partial information.– xagyg
Jun 1 '13 at 2:05
Show these declarations -
phonelength
, initialphone
, phonearray
. You are only giving us partial information.– xagyg
Jun 1 '13 at 2:05
add a comment |
2 Answers
2
active
oldest
votes
Your phonearray
does not have enough space: you are accessing index 9
, but phonearray
has fewer than ten elements.
You need to allocate the array like this:
phonearray = new int[10];
Once you fix this problem, change the loop to avoid accessing index -1
(that's what is going to happen when i
reaches zero).
Finally, there is no need to subtract phonetemp1%10
from phonetemp1
: integer division drops the fraction, so you could do this:
phonearray[i-1] = phonetemp1%10;
phonetemp1 /= 10;
I declared phone array like this long phonearray = new long[phonelength]; where phonelength = initialphone.length(); Doesnt that mean the array will have 10 elements then if initialphone.length() is 10?
– Max
Jun 1 '13 at 0:50
@user2437416 Then theArrayIndexOutOfBoundsException: 9
comes from a different place: if the length of the array is 10, then 9 is a valid index. Your code still has the potential of accessing element -1, when i==0, but then the exception would readArrayIndexOutOfBoundsException: -1
.
– dasblinkenlight
Jun 1 '13 at 0:54
add a comment |
If you have initialized the array correctly with the size same as the length of the initialPhone, you should not see this exception. Your code would rather produce ArrayIndexOutOfBoundException: -1
Rewrote your code a bit, and works as expected:
import java.util.Arrays;
public class TestMain {
public static void main(String args) {
String initialPhone = "4158884532";
int phoneLength = initialPhone.length();
long phoneArray = new long[phoneLength];
Long phoneNum = Long.parseLong(initialPhone);
Long phonetemp1 = phoneNum;
for (int i = phoneLength; i > 0; i--) {
phoneArray[i - 1] = phonetemp1 % 10;
Long phonetemp2 = phonetemp1 - phonetemp1 % 10;
phonetemp1 = phonetemp2 / 10;
System.out.println("Phone temp 2 --> " + phonetemp2 + phonetemp1);
}
System.out.println(Arrays.toString(phoneArray));
}
}
Enjoy!
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f16867875%2farray-problems-java-lang-arrayindexoutofboundsexception-9%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your phonearray
does not have enough space: you are accessing index 9
, but phonearray
has fewer than ten elements.
You need to allocate the array like this:
phonearray = new int[10];
Once you fix this problem, change the loop to avoid accessing index -1
(that's what is going to happen when i
reaches zero).
Finally, there is no need to subtract phonetemp1%10
from phonetemp1
: integer division drops the fraction, so you could do this:
phonearray[i-1] = phonetemp1%10;
phonetemp1 /= 10;
I declared phone array like this long phonearray = new long[phonelength]; where phonelength = initialphone.length(); Doesnt that mean the array will have 10 elements then if initialphone.length() is 10?
– Max
Jun 1 '13 at 0:50
@user2437416 Then theArrayIndexOutOfBoundsException: 9
comes from a different place: if the length of the array is 10, then 9 is a valid index. Your code still has the potential of accessing element -1, when i==0, but then the exception would readArrayIndexOutOfBoundsException: -1
.
– dasblinkenlight
Jun 1 '13 at 0:54
add a comment |
Your phonearray
does not have enough space: you are accessing index 9
, but phonearray
has fewer than ten elements.
You need to allocate the array like this:
phonearray = new int[10];
Once you fix this problem, change the loop to avoid accessing index -1
(that's what is going to happen when i
reaches zero).
Finally, there is no need to subtract phonetemp1%10
from phonetemp1
: integer division drops the fraction, so you could do this:
phonearray[i-1] = phonetemp1%10;
phonetemp1 /= 10;
I declared phone array like this long phonearray = new long[phonelength]; where phonelength = initialphone.length(); Doesnt that mean the array will have 10 elements then if initialphone.length() is 10?
– Max
Jun 1 '13 at 0:50
@user2437416 Then theArrayIndexOutOfBoundsException: 9
comes from a different place: if the length of the array is 10, then 9 is a valid index. Your code still has the potential of accessing element -1, when i==0, but then the exception would readArrayIndexOutOfBoundsException: -1
.
– dasblinkenlight
Jun 1 '13 at 0:54
add a comment |
Your phonearray
does not have enough space: you are accessing index 9
, but phonearray
has fewer than ten elements.
You need to allocate the array like this:
phonearray = new int[10];
Once you fix this problem, change the loop to avoid accessing index -1
(that's what is going to happen when i
reaches zero).
Finally, there is no need to subtract phonetemp1%10
from phonetemp1
: integer division drops the fraction, so you could do this:
phonearray[i-1] = phonetemp1%10;
phonetemp1 /= 10;
Your phonearray
does not have enough space: you are accessing index 9
, but phonearray
has fewer than ten elements.
You need to allocate the array like this:
phonearray = new int[10];
Once you fix this problem, change the loop to avoid accessing index -1
(that's what is going to happen when i
reaches zero).
Finally, there is no need to subtract phonetemp1%10
from phonetemp1
: integer division drops the fraction, so you could do this:
phonearray[i-1] = phonetemp1%10;
phonetemp1 /= 10;
answered Jun 1 '13 at 0:46
dasblinkenlightdasblinkenlight
612k587781197
612k587781197
I declared phone array like this long phonearray = new long[phonelength]; where phonelength = initialphone.length(); Doesnt that mean the array will have 10 elements then if initialphone.length() is 10?
– Max
Jun 1 '13 at 0:50
@user2437416 Then theArrayIndexOutOfBoundsException: 9
comes from a different place: if the length of the array is 10, then 9 is a valid index. Your code still has the potential of accessing element -1, when i==0, but then the exception would readArrayIndexOutOfBoundsException: -1
.
– dasblinkenlight
Jun 1 '13 at 0:54
add a comment |
I declared phone array like this long phonearray = new long[phonelength]; where phonelength = initialphone.length(); Doesnt that mean the array will have 10 elements then if initialphone.length() is 10?
– Max
Jun 1 '13 at 0:50
@user2437416 Then theArrayIndexOutOfBoundsException: 9
comes from a different place: if the length of the array is 10, then 9 is a valid index. Your code still has the potential of accessing element -1, when i==0, but then the exception would readArrayIndexOutOfBoundsException: -1
.
– dasblinkenlight
Jun 1 '13 at 0:54
I declared phone array like this long phonearray = new long[phonelength]; where phonelength = initialphone.length(); Doesnt that mean the array will have 10 elements then if initialphone.length() is 10?
– Max
Jun 1 '13 at 0:50
I declared phone array like this long phonearray = new long[phonelength]; where phonelength = initialphone.length(); Doesnt that mean the array will have 10 elements then if initialphone.length() is 10?
– Max
Jun 1 '13 at 0:50
@user2437416 Then the
ArrayIndexOutOfBoundsException: 9
comes from a different place: if the length of the array is 10, then 9 is a valid index. Your code still has the potential of accessing element -1, when i==0, but then the exception would read ArrayIndexOutOfBoundsException: -1
.– dasblinkenlight
Jun 1 '13 at 0:54
@user2437416 Then the
ArrayIndexOutOfBoundsException: 9
comes from a different place: if the length of the array is 10, then 9 is a valid index. Your code still has the potential of accessing element -1, when i==0, but then the exception would read ArrayIndexOutOfBoundsException: -1
.– dasblinkenlight
Jun 1 '13 at 0:54
add a comment |
If you have initialized the array correctly with the size same as the length of the initialPhone, you should not see this exception. Your code would rather produce ArrayIndexOutOfBoundException: -1
Rewrote your code a bit, and works as expected:
import java.util.Arrays;
public class TestMain {
public static void main(String args) {
String initialPhone = "4158884532";
int phoneLength = initialPhone.length();
long phoneArray = new long[phoneLength];
Long phoneNum = Long.parseLong(initialPhone);
Long phonetemp1 = phoneNum;
for (int i = phoneLength; i > 0; i--) {
phoneArray[i - 1] = phonetemp1 % 10;
Long phonetemp2 = phonetemp1 - phonetemp1 % 10;
phonetemp1 = phonetemp2 / 10;
System.out.println("Phone temp 2 --> " + phonetemp2 + phonetemp1);
}
System.out.println(Arrays.toString(phoneArray));
}
}
Enjoy!
add a comment |
If you have initialized the array correctly with the size same as the length of the initialPhone, you should not see this exception. Your code would rather produce ArrayIndexOutOfBoundException: -1
Rewrote your code a bit, and works as expected:
import java.util.Arrays;
public class TestMain {
public static void main(String args) {
String initialPhone = "4158884532";
int phoneLength = initialPhone.length();
long phoneArray = new long[phoneLength];
Long phoneNum = Long.parseLong(initialPhone);
Long phonetemp1 = phoneNum;
for (int i = phoneLength; i > 0; i--) {
phoneArray[i - 1] = phonetemp1 % 10;
Long phonetemp2 = phonetemp1 - phonetemp1 % 10;
phonetemp1 = phonetemp2 / 10;
System.out.println("Phone temp 2 --> " + phonetemp2 + phonetemp1);
}
System.out.println(Arrays.toString(phoneArray));
}
}
Enjoy!
add a comment |
If you have initialized the array correctly with the size same as the length of the initialPhone, you should not see this exception. Your code would rather produce ArrayIndexOutOfBoundException: -1
Rewrote your code a bit, and works as expected:
import java.util.Arrays;
public class TestMain {
public static void main(String args) {
String initialPhone = "4158884532";
int phoneLength = initialPhone.length();
long phoneArray = new long[phoneLength];
Long phoneNum = Long.parseLong(initialPhone);
Long phonetemp1 = phoneNum;
for (int i = phoneLength; i > 0; i--) {
phoneArray[i - 1] = phonetemp1 % 10;
Long phonetemp2 = phonetemp1 - phonetemp1 % 10;
phonetemp1 = phonetemp2 / 10;
System.out.println("Phone temp 2 --> " + phonetemp2 + phonetemp1);
}
System.out.println(Arrays.toString(phoneArray));
}
}
Enjoy!
If you have initialized the array correctly with the size same as the length of the initialPhone, you should not see this exception. Your code would rather produce ArrayIndexOutOfBoundException: -1
Rewrote your code a bit, and works as expected:
import java.util.Arrays;
public class TestMain {
public static void main(String args) {
String initialPhone = "4158884532";
int phoneLength = initialPhone.length();
long phoneArray = new long[phoneLength];
Long phoneNum = Long.parseLong(initialPhone);
Long phonetemp1 = phoneNum;
for (int i = phoneLength; i > 0; i--) {
phoneArray[i - 1] = phonetemp1 % 10;
Long phonetemp2 = phonetemp1 - phonetemp1 % 10;
phonetemp1 = phonetemp2 / 10;
System.out.println("Phone temp 2 --> " + phonetemp2 + phonetemp1);
}
System.out.println(Arrays.toString(phoneArray));
}
}
Enjoy!
answered Oct 30 '18 at 2:54


moonlightermoonlighter
333111
333111
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f16867875%2farray-problems-java-lang-arrayindexoutofboundsexception-9%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Stm2o XI27znPDmMB9w2c4Un nWhi,t3er s 7xVyV,Sb,OKD0IVDUcRU39UYwT
1
Why bother converting to long and then trying to extract digits? Just use substring().
– mclaassen
Jun 1 '13 at 0:40
When do you create phoneArray? Also, why so many phone variables? Mclaassen is right. In any case, you're being North American Numbering Plan-centric. And, where does the input come from in here?
– Eric Jablow
Jun 1 '13 at 0:44
Phone numbers should be treated as strings and not numbers.
– Don Roby
Jun 1 '13 at 0:50
Show these declarations -
phonelength
,initialphone
,phonearray
. You are only giving us partial information.– xagyg
Jun 1 '13 at 2:05