Should I be using the “using” statement here or leave it as is?
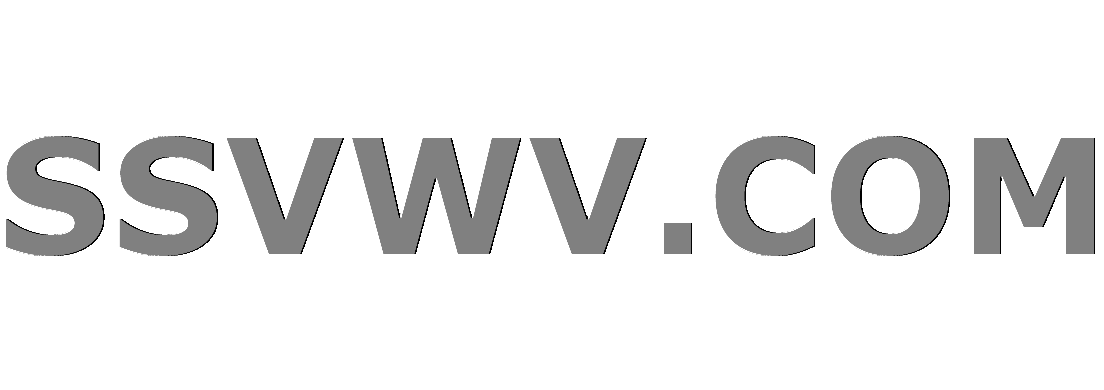
Multi tool use
I found out this way of creating DbContext instances a few years ago and only updated it slightly.
My code works, but I am wondering if it will cause any problems in the future.
My question is, should I use the "using" statement for my context calls or leave it as is?
This is for RAGEMP, a GTAV modification. Server syncs players and makes calls to the MySQL database when needed.
public class DefaultDbContext : DbContext
{
public DefaultDbContext(DbContextOptions options) : base(options)
{
}
// Accounts table
public DbSet<Account> Accounts { get; set; }
}
public class ContextFactory : IDesignTimeDbContextFactory<DefaultDbContext>
{
private static DefaultDbContext _instance;
public DefaultDbContext CreateDbContext(string args)
{
var builder = new DbContextOptionsBuilder<DefaultDbContext>();
builder.
UseMySql(@"Server=localhost;
database=efcore;
uid=root;
pwd=;",
optionsBuilder => optionsBuilder.MigrationsAssembly(typeof(DefaultDbContext).GetTypeInfo().Assembly.GetName().Name));
return new DefaultDbContext(builder.Options);
}
public static DefaultDbContext Instance
{
get
{
if (_instance != null) return _instance;
return _instance = new ContextFactory().CreateDbContext(new string { });
}
private set { }
}
// somewhere else
// create a new Account object
var account = new Account
{
Username = "test",
Password = "test"
};
// Add this account data to the current context
ContextFactory.Instance.Accounts.Add(account);
// And finally insert the data into the database
ContextFactory.Instance.SaveChanges();
c# ef-core-2.0
add a comment |
I found out this way of creating DbContext instances a few years ago and only updated it slightly.
My code works, but I am wondering if it will cause any problems in the future.
My question is, should I use the "using" statement for my context calls or leave it as is?
This is for RAGEMP, a GTAV modification. Server syncs players and makes calls to the MySQL database when needed.
public class DefaultDbContext : DbContext
{
public DefaultDbContext(DbContextOptions options) : base(options)
{
}
// Accounts table
public DbSet<Account> Accounts { get; set; }
}
public class ContextFactory : IDesignTimeDbContextFactory<DefaultDbContext>
{
private static DefaultDbContext _instance;
public DefaultDbContext CreateDbContext(string args)
{
var builder = new DbContextOptionsBuilder<DefaultDbContext>();
builder.
UseMySql(@"Server=localhost;
database=efcore;
uid=root;
pwd=;",
optionsBuilder => optionsBuilder.MigrationsAssembly(typeof(DefaultDbContext).GetTypeInfo().Assembly.GetName().Name));
return new DefaultDbContext(builder.Options);
}
public static DefaultDbContext Instance
{
get
{
if (_instance != null) return _instance;
return _instance = new ContextFactory().CreateDbContext(new string { });
}
private set { }
}
// somewhere else
// create a new Account object
var account = new Account
{
Username = "test",
Password = "test"
};
// Add this account data to the current context
ContextFactory.Instance.Accounts.Add(account);
// And finally insert the data into the database
ContextFactory.Instance.SaveChanges();
c# ef-core-2.0
add a comment |
I found out this way of creating DbContext instances a few years ago and only updated it slightly.
My code works, but I am wondering if it will cause any problems in the future.
My question is, should I use the "using" statement for my context calls or leave it as is?
This is for RAGEMP, a GTAV modification. Server syncs players and makes calls to the MySQL database when needed.
public class DefaultDbContext : DbContext
{
public DefaultDbContext(DbContextOptions options) : base(options)
{
}
// Accounts table
public DbSet<Account> Accounts { get; set; }
}
public class ContextFactory : IDesignTimeDbContextFactory<DefaultDbContext>
{
private static DefaultDbContext _instance;
public DefaultDbContext CreateDbContext(string args)
{
var builder = new DbContextOptionsBuilder<DefaultDbContext>();
builder.
UseMySql(@"Server=localhost;
database=efcore;
uid=root;
pwd=;",
optionsBuilder => optionsBuilder.MigrationsAssembly(typeof(DefaultDbContext).GetTypeInfo().Assembly.GetName().Name));
return new DefaultDbContext(builder.Options);
}
public static DefaultDbContext Instance
{
get
{
if (_instance != null) return _instance;
return _instance = new ContextFactory().CreateDbContext(new string { });
}
private set { }
}
// somewhere else
// create a new Account object
var account = new Account
{
Username = "test",
Password = "test"
};
// Add this account data to the current context
ContextFactory.Instance.Accounts.Add(account);
// And finally insert the data into the database
ContextFactory.Instance.SaveChanges();
c# ef-core-2.0
I found out this way of creating DbContext instances a few years ago and only updated it slightly.
My code works, but I am wondering if it will cause any problems in the future.
My question is, should I use the "using" statement for my context calls or leave it as is?
This is for RAGEMP, a GTAV modification. Server syncs players and makes calls to the MySQL database when needed.
public class DefaultDbContext : DbContext
{
public DefaultDbContext(DbContextOptions options) : base(options)
{
}
// Accounts table
public DbSet<Account> Accounts { get; set; }
}
public class ContextFactory : IDesignTimeDbContextFactory<DefaultDbContext>
{
private static DefaultDbContext _instance;
public DefaultDbContext CreateDbContext(string args)
{
var builder = new DbContextOptionsBuilder<DefaultDbContext>();
builder.
UseMySql(@"Server=localhost;
database=efcore;
uid=root;
pwd=;",
optionsBuilder => optionsBuilder.MigrationsAssembly(typeof(DefaultDbContext).GetTypeInfo().Assembly.GetName().Name));
return new DefaultDbContext(builder.Options);
}
public static DefaultDbContext Instance
{
get
{
if (_instance != null) return _instance;
return _instance = new ContextFactory().CreateDbContext(new string { });
}
private set { }
}
// somewhere else
// create a new Account object
var account = new Account
{
Username = "test",
Password = "test"
};
// Add this account data to the current context
ContextFactory.Instance.Accounts.Add(account);
// And finally insert the data into the database
ContextFactory.Instance.SaveChanges();
c# ef-core-2.0
c# ef-core-2.0
asked Dec 29 '18 at 2:35
xForcerxForcer
134
134
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
There is nothing wrong with this approach if you are keeping your DbContext short lived and not trying to cache them or overly reuse an instance.
However, personally i find this a little verbose. For inhouse-applications, i tend to keep setup and connection strings in app.config and just use the using
statement .
using(var db = new MyContext())
{
var lotsOfStuff = db.SomeTable.Where(x => x.IsAwesome);
//
}
On saying that, there is really only a few rules you need to abide by (without this being an opinionated answer)
- Don't try to overly use a DbContext. They are internally cached, and there is little overhead in creating them and closing them.
- Don't try to hide everything behind layers of abstractions unnecessarily.
- Always code for readability and maintainability first, unless you have a need to code for performance.
Update
Maybe I am misunderstanding something but if I am saving changes to
the database more often than not, is my approach then bad? Little
things get updated when something is changed, not big chunk of data
here and there
It depends how long you are keeping open your DefaultDbContext
, I mean if its only for a couple of queries year thats fine.
Context are designed to be opened and closed fairly quickly, they are not designed to stay open and alive for long periods of time. Doing so will sometimes cause you more issues than not.
Saving to the database often, while making perfect sense, its not really the issue here.
Thank you for answering! Maybe I am misunderstanding something but if I am saving changes to the database more often than not, is my approach then bad? Little things get updated when something is changed, not big chunk of data here and there. E.g. Player money In Game, player clothes... It's saving changes to the database whenever there is a change in game, the same moment. So TL;DR small changes, but often.
– xForcer
Dec 29 '18 at 17:38
@xForcer updated
– TheGeneral
Dec 30 '18 at 0:40
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53966236%2fshould-i-be-using-the-using-statement-here-or-leave-it-as-is%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
There is nothing wrong with this approach if you are keeping your DbContext short lived and not trying to cache them or overly reuse an instance.
However, personally i find this a little verbose. For inhouse-applications, i tend to keep setup and connection strings in app.config and just use the using
statement .
using(var db = new MyContext())
{
var lotsOfStuff = db.SomeTable.Where(x => x.IsAwesome);
//
}
On saying that, there is really only a few rules you need to abide by (without this being an opinionated answer)
- Don't try to overly use a DbContext. They are internally cached, and there is little overhead in creating them and closing them.
- Don't try to hide everything behind layers of abstractions unnecessarily.
- Always code for readability and maintainability first, unless you have a need to code for performance.
Update
Maybe I am misunderstanding something but if I am saving changes to
the database more often than not, is my approach then bad? Little
things get updated when something is changed, not big chunk of data
here and there
It depends how long you are keeping open your DefaultDbContext
, I mean if its only for a couple of queries year thats fine.
Context are designed to be opened and closed fairly quickly, they are not designed to stay open and alive for long periods of time. Doing so will sometimes cause you more issues than not.
Saving to the database often, while making perfect sense, its not really the issue here.
Thank you for answering! Maybe I am misunderstanding something but if I am saving changes to the database more often than not, is my approach then bad? Little things get updated when something is changed, not big chunk of data here and there. E.g. Player money In Game, player clothes... It's saving changes to the database whenever there is a change in game, the same moment. So TL;DR small changes, but often.
– xForcer
Dec 29 '18 at 17:38
@xForcer updated
– TheGeneral
Dec 30 '18 at 0:40
add a comment |
There is nothing wrong with this approach if you are keeping your DbContext short lived and not trying to cache them or overly reuse an instance.
However, personally i find this a little verbose. For inhouse-applications, i tend to keep setup and connection strings in app.config and just use the using
statement .
using(var db = new MyContext())
{
var lotsOfStuff = db.SomeTable.Where(x => x.IsAwesome);
//
}
On saying that, there is really only a few rules you need to abide by (without this being an opinionated answer)
- Don't try to overly use a DbContext. They are internally cached, and there is little overhead in creating them and closing them.
- Don't try to hide everything behind layers of abstractions unnecessarily.
- Always code for readability and maintainability first, unless you have a need to code for performance.
Update
Maybe I am misunderstanding something but if I am saving changes to
the database more often than not, is my approach then bad? Little
things get updated when something is changed, not big chunk of data
here and there
It depends how long you are keeping open your DefaultDbContext
, I mean if its only for a couple of queries year thats fine.
Context are designed to be opened and closed fairly quickly, they are not designed to stay open and alive for long periods of time. Doing so will sometimes cause you more issues than not.
Saving to the database often, while making perfect sense, its not really the issue here.
Thank you for answering! Maybe I am misunderstanding something but if I am saving changes to the database more often than not, is my approach then bad? Little things get updated when something is changed, not big chunk of data here and there. E.g. Player money In Game, player clothes... It's saving changes to the database whenever there is a change in game, the same moment. So TL;DR small changes, but often.
– xForcer
Dec 29 '18 at 17:38
@xForcer updated
– TheGeneral
Dec 30 '18 at 0:40
add a comment |
There is nothing wrong with this approach if you are keeping your DbContext short lived and not trying to cache them or overly reuse an instance.
However, personally i find this a little verbose. For inhouse-applications, i tend to keep setup and connection strings in app.config and just use the using
statement .
using(var db = new MyContext())
{
var lotsOfStuff = db.SomeTable.Where(x => x.IsAwesome);
//
}
On saying that, there is really only a few rules you need to abide by (without this being an opinionated answer)
- Don't try to overly use a DbContext. They are internally cached, and there is little overhead in creating them and closing them.
- Don't try to hide everything behind layers of abstractions unnecessarily.
- Always code for readability and maintainability first, unless you have a need to code for performance.
Update
Maybe I am misunderstanding something but if I am saving changes to
the database more often than not, is my approach then bad? Little
things get updated when something is changed, not big chunk of data
here and there
It depends how long you are keeping open your DefaultDbContext
, I mean if its only for a couple of queries year thats fine.
Context are designed to be opened and closed fairly quickly, they are not designed to stay open and alive for long periods of time. Doing so will sometimes cause you more issues than not.
Saving to the database often, while making perfect sense, its not really the issue here.
There is nothing wrong with this approach if you are keeping your DbContext short lived and not trying to cache them or overly reuse an instance.
However, personally i find this a little verbose. For inhouse-applications, i tend to keep setup and connection strings in app.config and just use the using
statement .
using(var db = new MyContext())
{
var lotsOfStuff = db.SomeTable.Where(x => x.IsAwesome);
//
}
On saying that, there is really only a few rules you need to abide by (without this being an opinionated answer)
- Don't try to overly use a DbContext. They are internally cached, and there is little overhead in creating them and closing them.
- Don't try to hide everything behind layers of abstractions unnecessarily.
- Always code for readability and maintainability first, unless you have a need to code for performance.
Update
Maybe I am misunderstanding something but if I am saving changes to
the database more often than not, is my approach then bad? Little
things get updated when something is changed, not big chunk of data
here and there
It depends how long you are keeping open your DefaultDbContext
, I mean if its only for a couple of queries year thats fine.
Context are designed to be opened and closed fairly quickly, they are not designed to stay open and alive for long periods of time. Doing so will sometimes cause you more issues than not.
Saving to the database often, while making perfect sense, its not really the issue here.
edited Dec 30 '18 at 0:40
answered Dec 29 '18 at 3:12


TheGeneralTheGeneral
29.2k63465
29.2k63465
Thank you for answering! Maybe I am misunderstanding something but if I am saving changes to the database more often than not, is my approach then bad? Little things get updated when something is changed, not big chunk of data here and there. E.g. Player money In Game, player clothes... It's saving changes to the database whenever there is a change in game, the same moment. So TL;DR small changes, but often.
– xForcer
Dec 29 '18 at 17:38
@xForcer updated
– TheGeneral
Dec 30 '18 at 0:40
add a comment |
Thank you for answering! Maybe I am misunderstanding something but if I am saving changes to the database more often than not, is my approach then bad? Little things get updated when something is changed, not big chunk of data here and there. E.g. Player money In Game, player clothes... It's saving changes to the database whenever there is a change in game, the same moment. So TL;DR small changes, but often.
– xForcer
Dec 29 '18 at 17:38
@xForcer updated
– TheGeneral
Dec 30 '18 at 0:40
Thank you for answering! Maybe I am misunderstanding something but if I am saving changes to the database more often than not, is my approach then bad? Little things get updated when something is changed, not big chunk of data here and there. E.g. Player money In Game, player clothes... It's saving changes to the database whenever there is a change in game, the same moment. So TL;DR small changes, but often.
– xForcer
Dec 29 '18 at 17:38
Thank you for answering! Maybe I am misunderstanding something but if I am saving changes to the database more often than not, is my approach then bad? Little things get updated when something is changed, not big chunk of data here and there. E.g. Player money In Game, player clothes... It's saving changes to the database whenever there is a change in game, the same moment. So TL;DR small changes, but often.
– xForcer
Dec 29 '18 at 17:38
@xForcer updated
– TheGeneral
Dec 30 '18 at 0:40
@xForcer updated
– TheGeneral
Dec 30 '18 at 0:40
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53966236%2fshould-i-be-using-the-using-statement-here-or-leave-it-as-is%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
h8v,0waWz2yWKg,T,Bll5fEE,b